In MATLAB, the `roots` function is used to find the roots of a polynomial given its coefficients in a vector format. Here’s a simple example:
% Define the coefficients of the polynomial: x^2 - 3x + 2
coefficients = [1 -3 2];
% Calculate the roots
r = roots(coefficients);
disp(r);
Understanding Roots of Functions
What Are Roots?
In mathematics, a root is defined as a value at which a function equals zero. When graphed, roots represent the points where the curve intersects the x-axis. For example, if we have a quadratic equation \( f(x) = ax^2 + bx + c \), the roots are the solutions to the equation \( ax^2 + bx + c = 0 \). Finding these points is crucial for understanding the behavior of the function and solving equations in various applications.
Importance of Roots in Various Fields
Roots are significant in multiple domains:
- Physics: Roots help in analyzing motion, forces, and equilibrium points.
- Engineering: Designing systems often requires identifying stability points, which correspond to roots of polynomials.
- Data Science: Root finding is important in optimization problems, such as minimizing error functions in model fitting.
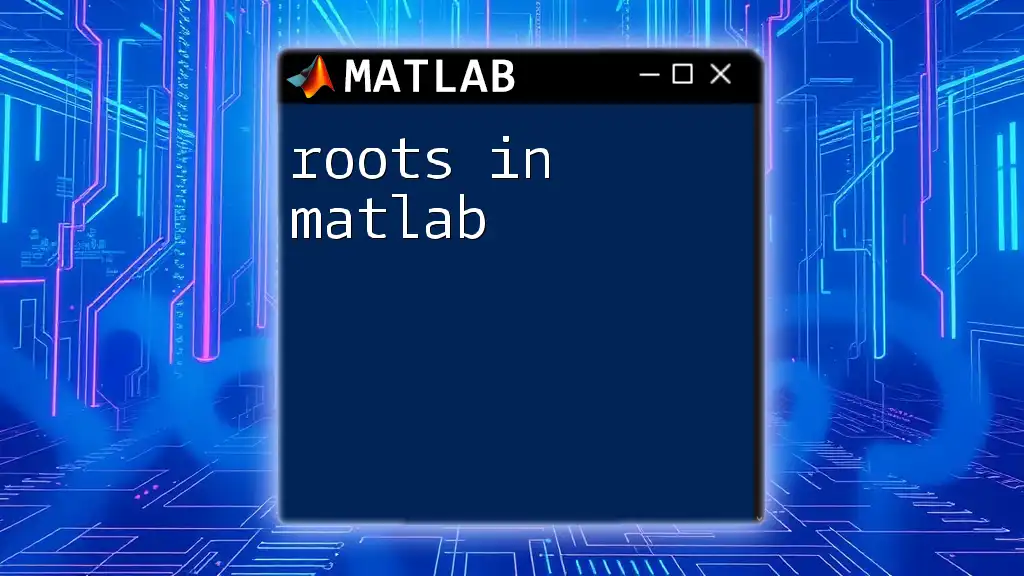
MATLAB Functions for Root Finding
Overview of Root Finding in MATLAB
MATLAB provides a robust suite of built-in functions to assist in finding roots of both polynomial and non-polynomial equations. These functions are efficient and user-friendly, making MATLAB a powerful tool for mathematicians, engineers, and scientists alike.
The `roots` Command
Syntax and Usage
The `roots` function is specifically designed for finding the roots of polynomial equations. The syntax is straightforward:
roots(p)
Here, p is a vector of coefficients in descending order of the polynomial degree.
Example
To find the roots of a polynomial equation, consider:
% Example: Finding roots of the polynomial x^2 - 5x + 6
p = [1 -5 6]; % Coefficients for f(x) = x^2 - 5x + 6
r = roots(p);
disp(r); % Display the roots
In this example, the output will reveal the roots \( x = 2 \) and \( x = 3 \), indicating where the function intersects the x-axis.
The `fzero` Function
Syntax and Usage
The `fzero` function finds a root of a non-linear function, and its syntax is:
fzero(fun, x0)
fun is a handle to the function for which the root is sought, and x0 is an initial guess.
Example
To find the root of the function \( f(x) = x^2 - 4 \):
% Example: Finding a root of the function f(x) = x^2 - 4
f = @(x) x.^2 - 4; % Define function
root = fzero(f, 2); % Initial guess of 2
disp(root); % Display the root
In this case, the output will show \( 2 \) or \( -2 \) as valid roots depending on the initial guess. Choosing an appropriate starting point is critical for the success of methods like these.
The `fsolve` Function
Syntax and Usage
For solving a system of equations, MATLAB utilizes the `fsolve` function:
fsolve(fun, x0)
Here, fun is again a function handle, and x0 is an initial guess or starting point.
Example
To solve a system of equations, consider the following example:
% Example: Solving a system of equations
fun = @(x) [x(1)^2 + x(2)^2 - 4; x(1) - x(2)];
initialGuess = [1; 1];
solution = fsolve(fun, initialGuess);
disp(solution); % Display the solution
The output displays \( x(1) \) and \( x(2) \) that satisfy both equations, demonstrating how both functions intersect.
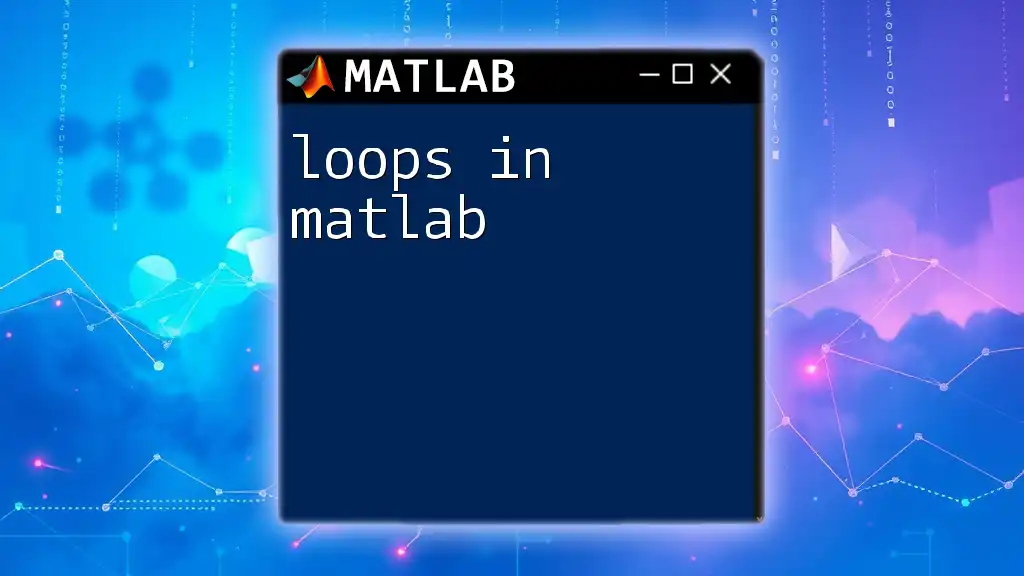
Visualizing Roots
Plotting Functions and Roots
Visualization is a powerful way to comprehend the behavior of functions and their roots. MATLAB's plotting capability allows us to view functions and easily identify their roots.
Example
To visualize the function \( f(x) = x^2 - 4 \) along with its roots, use the following code:
% Example: Plotting the function f(x) = x^2 - 4 along with its roots
f = @(x) x.^2 - 4;
fplot(f, [-5 5]); % Plot the function
hold on;
plot([-2 2], [0 0], 'r--'); % x-axis
plot([-2 2], [2 2], 'g*'); % Roots as green stars
hold off;
title('Plot of the function f(x) = x^2 - 4');
xlabel('x');
ylabel('f(x)');
legend('f(x)', 'Roots');
grid on;
This code generates a visual representation, allowing the viewer to quickly identify where the function crosses the x-axis—confirming the calculated roots at \( 2 \) and \( -2 \).
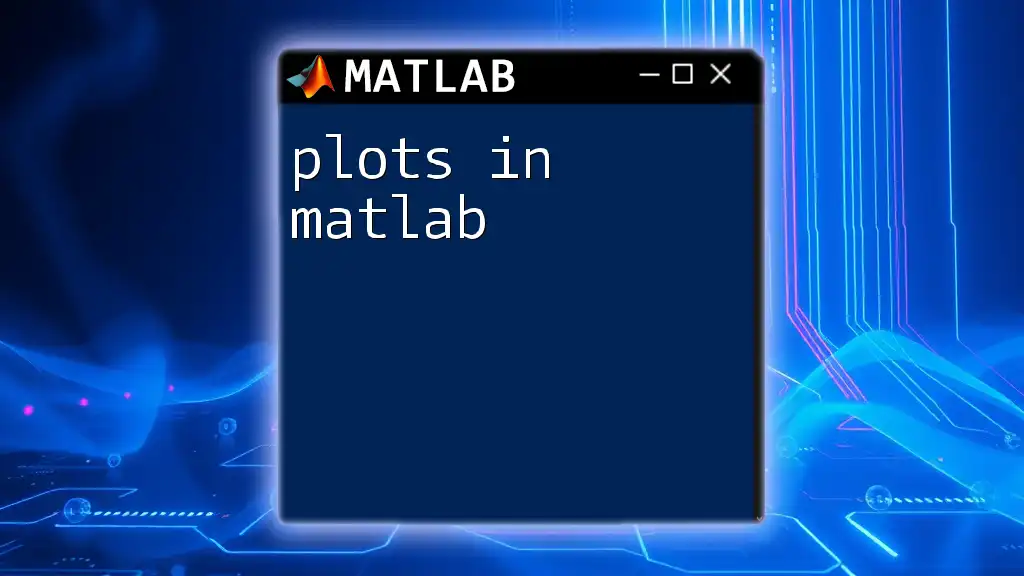
Advanced Techniques for Root Finding
Newton-Raphson Method
The Newton-Raphson method is an iterative root-finding algorithm that converges quickly under certain conditions. It uses the formula: \[ x_{n+1} = x_n - \frac{f(x_n)}{f'(x_n)} \]
Implementation in MATLAB
To implement the Newton-Raphson method for \( f(x) = x^2 - 4 \):
% Example: Using the Newton-Raphson method
f = @(x) x.^2 - 4; % Function
df = @(x) 2*x; % Derivative
x0 = 2; % Initial guess
tol = 1e-6;
maxIter = 100;
iter = 0;
while iter < maxIter
x1 = x0 - f(x0)/df(x0);
if abs(x1 - x0) < tol
break; % Stop if the change is within tolerance
end
x0 = x1;
iter = iter + 1;
end
disp(x1); % Display approximated root
In this example, the output will reveal the approximated root. Understanding convergence criteria is essential; if the initial guess is too far from the actual root, the method might fail.
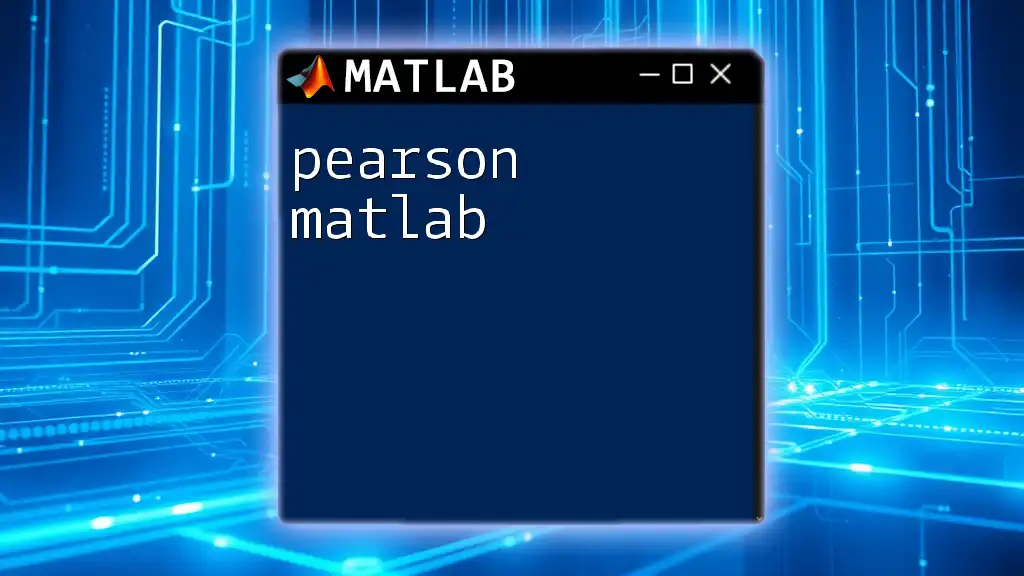
Common Pitfalls and Troubleshooting
Issues with Convergence
Occasionally, root-finding algorithms may struggle to converge, especially if:
- The chosen initial guess is too far from the actual root.
- The function's derivative is near zero at the guessed value (causing division by zero).
- The function has discontinuities or oscillations near the root.
Tips for Successful Root Finding
- Choose Intelligent Initial Guesses: A good initial guess can drastically affect convergence speed.
- Visualize the Function: Always plot the function to understand its behavior and identify roots.
- Use Options Structures: Utilize options in MATLAB functions to set tolerances and maximum iterations according to your needs.
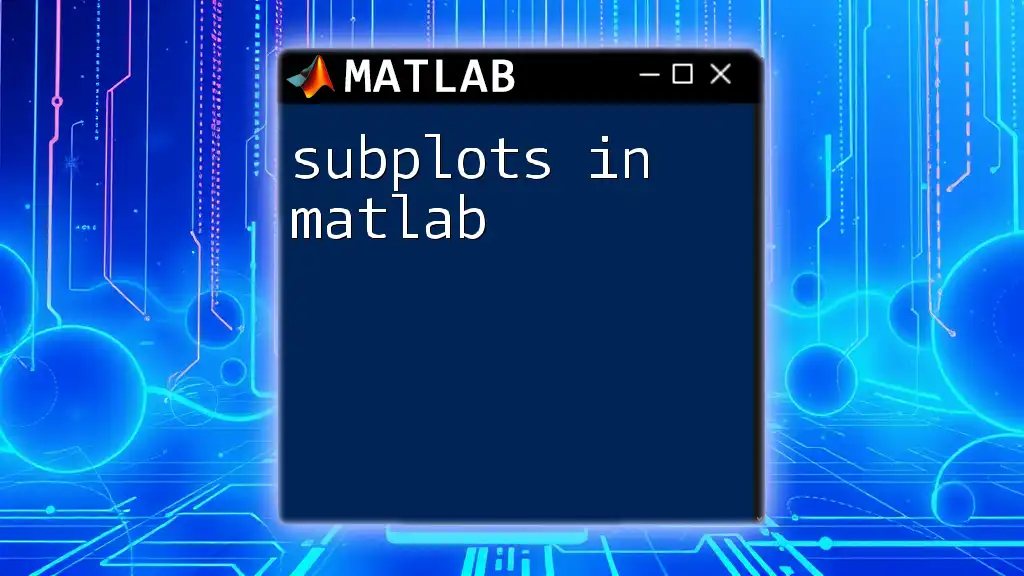
Conclusion
This comprehensive guide highlights the essential methods for finding roots on MATLAB. From understanding roots and their significance to applying effective MATLAB commands like `roots`, `fzero`, and `fsolve`, you now have the tools to explore root-finding in your projects. Experimenting with different functions and visualizing their graphs will further deepen your understanding and ability to apply these concepts effectively.
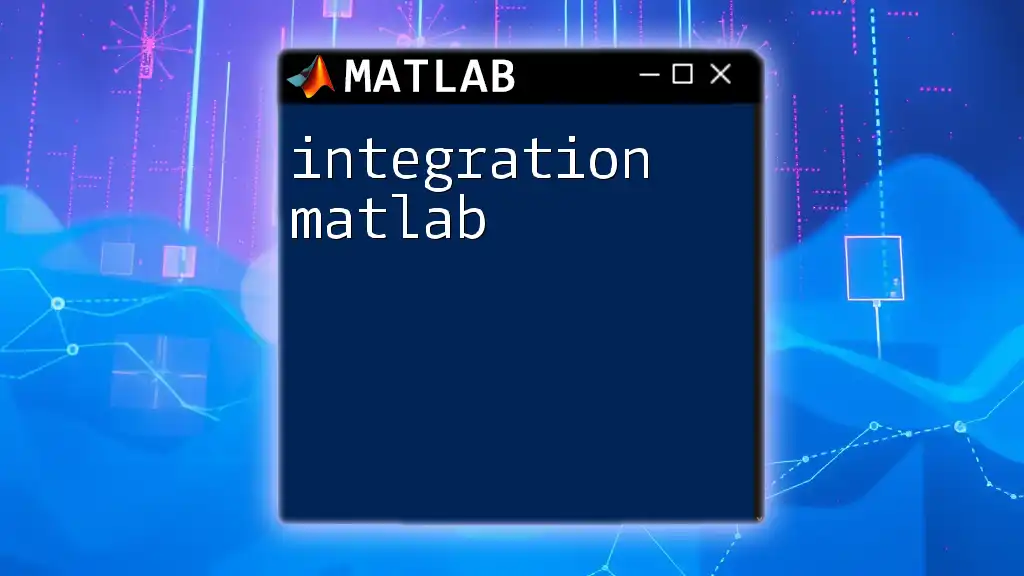
Additional Resources
To further enhance your skills, consider exploring MATLAB's documentation on these functions, relevant textbooks, and online courses that focus on numerical methods and computational mathematics.