In MATLAB, the modulus operation can be performed using the `mod` function, which returns the remainder after the division of two numbers.
result = mod(10, 3); % This returns 1, as 10 divided by 3 leaves a remainder of 1.
Understanding the Modulus Operator
What is Modulus?
The term modulus refers to the operation of finding the remainder when one integer is divided by another. In simple terms, when you divide a number (the dividend) by another number (the divisor), the modulus operation returns the leftover value that results from the division, which is known as the remainder. For example, in the division of `10` by `3`, the quotient is `3` and the remainder (or modulus) is `1`.
The Modulus Operator in MATLAB
In MATLAB, the modulus operation is primarily performed using the `mod` function. The syntax is straightforward:
result = mod(a, b)
Here, `a` is the dividend and `b` is the divisor. The function returns the remainder when `a` is divided by `b`. Understanding how to use this function effectively is essential for performing various calculations in MATLAB.
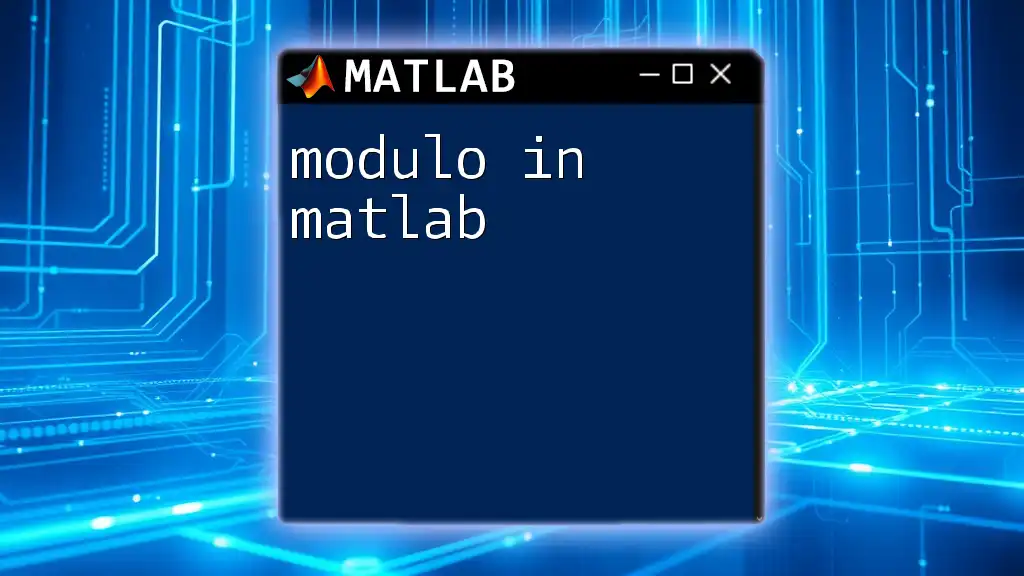
Basic Usage of Modulus in MATLAB
Using the `mod` Function
The `mod` function is fundamental for performing modulus calculations in MATLAB. It operates efficiently and is designed to handle both scalar and array inputs seamlessly.
Example of Basic Modulus Calculation
Let's consider a simple example to illustrate how the `mod` function works:
result = mod(10, 3);
disp(result); % Expected output: 1
In this scenario, `10` divided by `3` gives a quotient of `3` and a remainder of `1`, which is what the `mod` function returns.
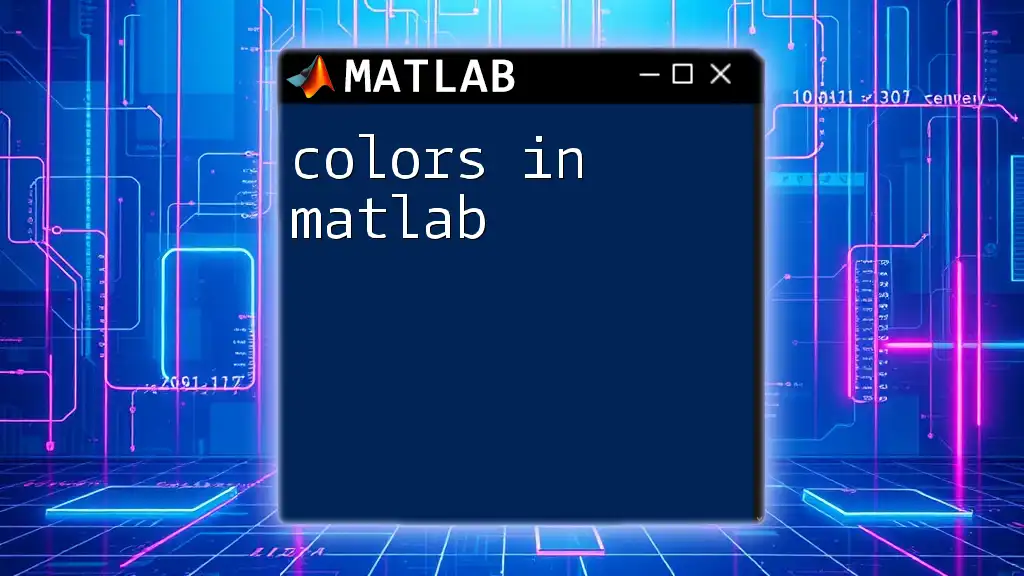
Working with Negative Numbers
Modulus with Negative Dividends
One fascinating aspect of the `mod` function is how it handles negative numbers. When the dividend is negative, MATLAB returns the modulus result that is non-negative.
result = mod(-10, 3);
disp(result); % Expected output: 2
Here, `-10` divided by `3` has a quotient of `-4` and a remainder of `2`. The `mod` function ensures that the output remains non-negative.
Modulus with Negative Divisors
Similarly, the behavior of the `mod` function changes when the divisor is negative. MATLAB will still calculate the modulus correctly but returns a negative output if the dividend is positive.
result = mod(10, -3);
disp(result); % Expected output: -2
In this case, `10` divided by `-3` yields a quotient of `-3` and a remainder of `-2`, reflecting this unique behavior of the modulus operation.
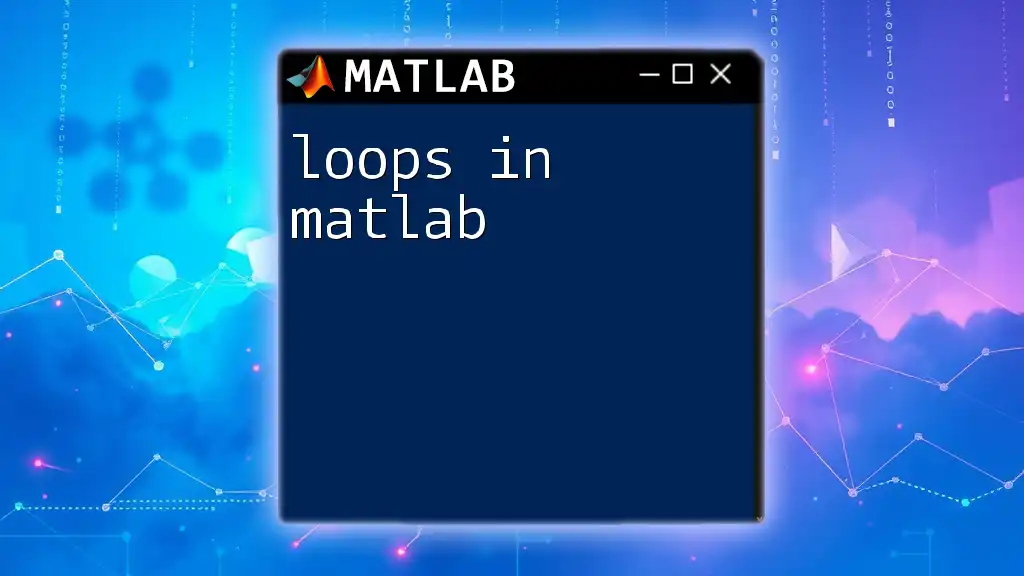
Practical Applications of Modulus
Check for Even or Odd Numbers
One of the most common applications of the modulus operation is checking whether a number is even or odd. In programming, this can be achieved using the condition:
number = 7;
if mod(number, 2) == 0
disp('Even');
else
disp('Odd');
end
This code snippet checks if the remainder of `number` when divided by `2` is zero. If it is, the number is even; otherwise, it is odd.
Periodic Behavior in Signals
The modulus can also play a critical role in signal processing, particularly when modeling periodic functions. The periodic nature of certain signals can be captured through the modulus operation.
t = 0:0.1:10;
signal = mod(t, 2); % Creates a periodic square wave
plot(t, signal);
title('Periodic Signal Example');
xlabel('Time');
ylabel('Signal');
In the above example, the `mod` function is used to create a periodic square wave signal. The time vector `t` gets mapped to a repeating pattern every `2` units.
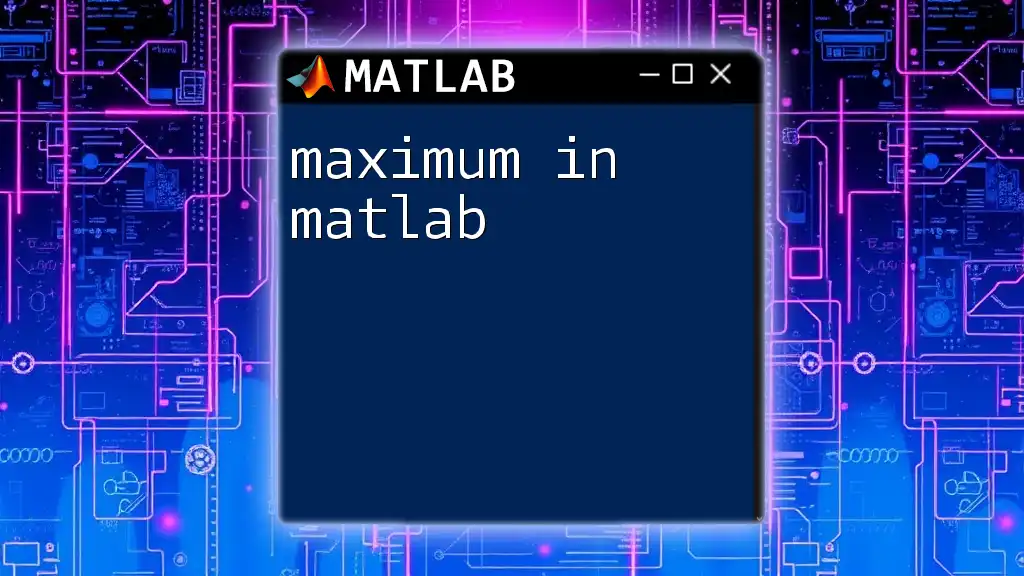
Advanced Uses of Modulus
Vector and Matrix Operations
The `mod` function is versatile and can be applied to vectors and matrices in MATLAB. When you input an array, the `mod` function performs element-wise operations, which simplifies computations significantly.
A = [10, 15, -7; 12, -9, 3];
result = mod(A, 5);
disp(result);
In this example, the `mod` function applies to each element of the matrix `A`, providing an output matrix that contains the remainders when each element is divided by `5`.
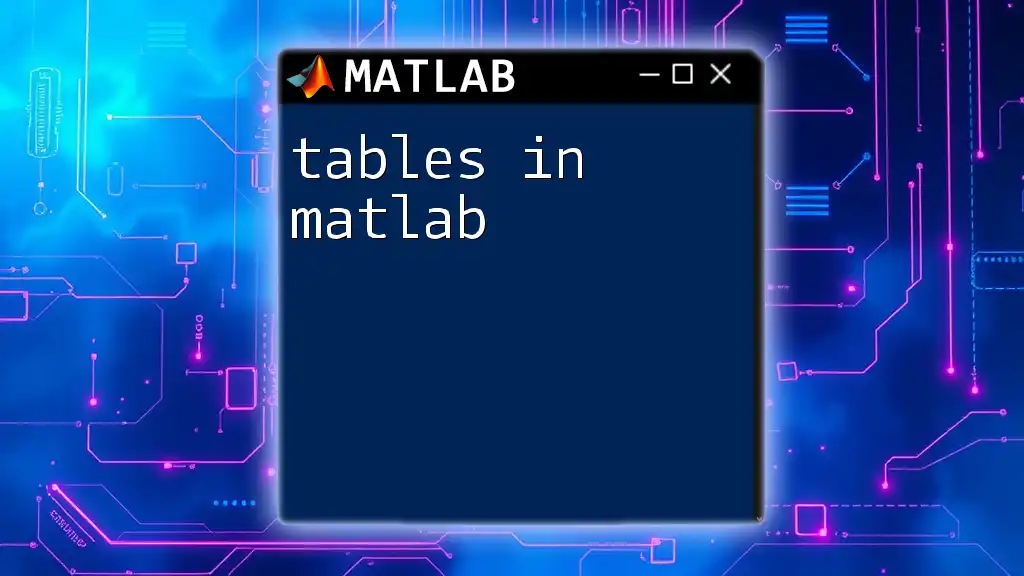
Common Pitfalls and Troubleshooting
Misunderstandings with Data Types
While using the `mod` function, it's crucial to keep in mind that the data type of the operands can affect the output. For instance, using complex numbers with `mod` does not yield meaningful results. Always ensure the inputs are compatible with the modulus operation.
Performance Considerations
When working with large datasets or inside loops, it’s essential to consider the performance of the `mod` function. If you find that your computations are slow, check for vectorization opportunities or look into optimizing your calculations to improve efficiency.
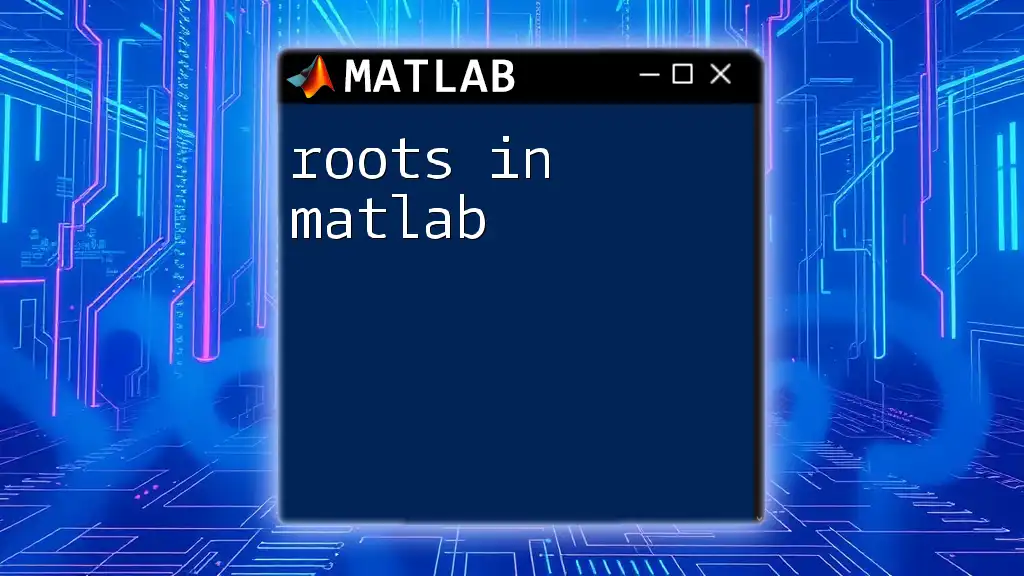
Conclusion
The modulus operation, represented by the `mod` function in MATLAB, is a powerful and versatile tool for handling a variety of mathematical tasks. Its ability to work with both positive and negative numbers, in addition to its applications in determining even and odd numbers or modeling periodic behavior, make it an essential part of your MATLAB toolbox. To fully grasp its potential, practice using modulus in different coding scenarios, and you'll become proficient in this useful operation.
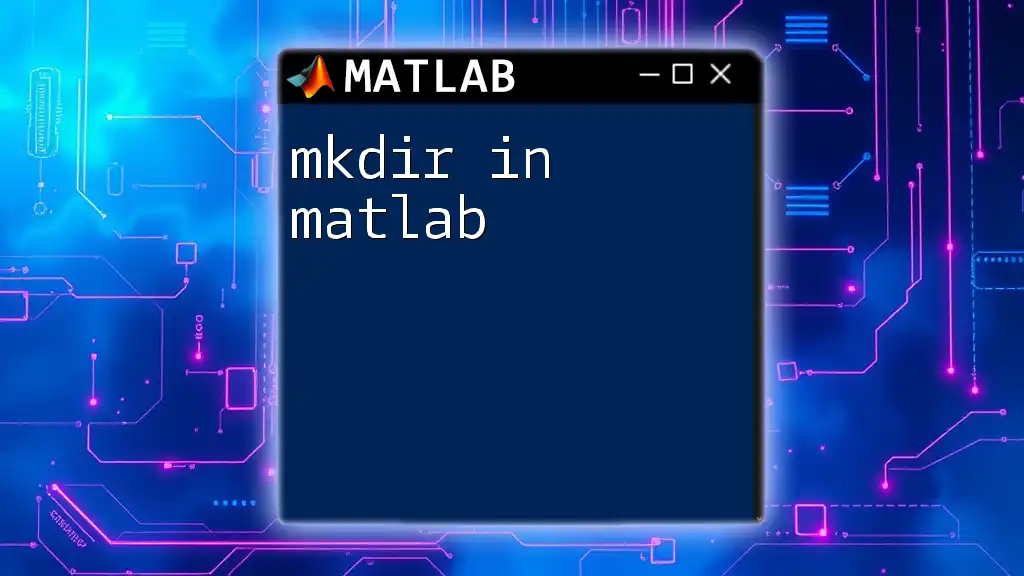
Additional Resources
To deepen your understanding of the modulus operation in MATLAB, explore the official MATLAB documentation and consider tackling exercises that challenge your grasp of the concepts discussed in this guide.