In MATLAB, a function handle is a reference to a function that allows you to pass functions as arguments to other functions or store them in variables, enabling more flexible code execution.
Here's a code snippet demonstrating how to create and use a function handle:
% Define a simple function
square = @(x) x.^2;
% Use the function handle to compute the square of a number
result = square(5); % result will be 25
Understanding the Basics of Function Handles
What is a Function Handle?
A function handle is a MATLAB data type that allows you to create a reference to a function that can be passed around in your code, similar to a pointer in other programming languages. This powerful feature is essential for flexible programming styles that require functions to be treated as first-class citizens.
The basic syntax to create a function handle is:
f = @myFunction; % Replace 'myFunction' with the name of your actual function
How to Create Function Handles
Creating function handles is straightforward. You can have them in two ways: through anonymous functions or named functions.
Creating Anonymous Functions
An anonymous function is a one-line expression that does not require a separate file. For example:
f = @(x) x^2; % An anonymous function that squares its input
This allows you to create quick snippets of code without cluttering your workspace with multiple function files.
Creating Named Functions
To create a named function, you would typically define it in a separate `.m` file. Here’s an example:
function y = myFunction(x)
y = x^2; % Squares the input x
end
Then, the function handle can be created like this:
f = @myFunction; % Creates a handle to the named function
Properties of Function Handles
Function handles provide remarkable flexibility in how you can work with different types of functions throughout your code. They can reference any function (including anonymous functions) and can even encapsulate functions that have parameters.
Anonymous Functions vs Named Functions
While both types serve their purposes, anonymous functions are generally used for short, simple tasks where creating a whole file isn't necessary. Named functions, on the other hand, are better for larger, more complex operations that require clarity and maintainability.
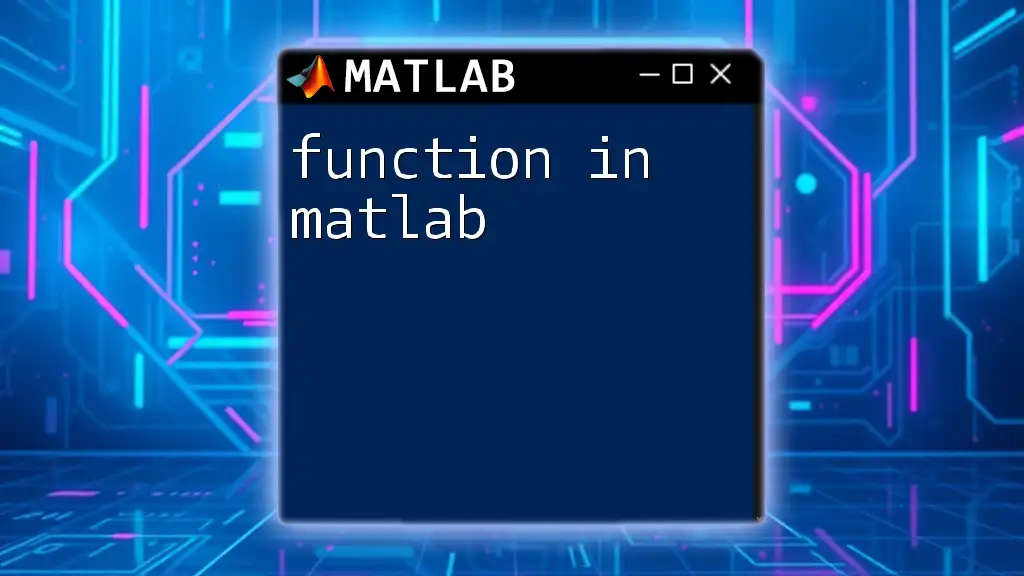
Using Function Handles in MATLAB
Passing Function Handles as Arguments
One of the main advantages of using function handles is that they can be passed as arguments to other functions. Here is an example demonstrating this concept:
function result = integrateFunction(f, a, b)
result = integral(f, a, b); % Uses built-in function integral to compute the area under f from a to b
end
In this case, you can pass a function handle like so:
f = @(x) x.^2; % Define the function we want to integrate
area = integrateFunction(f, 0, 1); % Computes the integral of f from 0 to 1
This modular approach promotes code reuse and keeps functions focused on their tasks.
Function Handles in Array Operations
Function handles can also be utilized for operations on arrays. MATLAB’s vectorization capabilities enable efficient computations, which are often faster than traditional loops. Here’s how you can apply a function handle to an array:
f = @(x) x.^2; % Define an anonymous function that squares its input
values = f(1:5); % Applies the function to each element of the array [1, 2, 3, 4, 5]
In this case, `values` will yield `[1, 4, 9, 16, 25]`, showing how easily you can apply defined logic to multiple inputs.
Using Function Handles with Built-in Functions
MATLAB offers several built-in functions that work seamlessly with function handles.
Examples with `arrayfun`, `cellfun`
The `arrayfun` function applies a function handle to each element of an array:
A = [1, 2, 3, 4];
squaredArray = arrayfun(f, A); % Applies f to each element of A
This results in `squaredArray` being `[1, 4, 9, 16]`.
Similarly, `cellfun` applies a function handle to each element inside a cell array:
C = {1, 2, 3};
squaredCells = cellfun(f, C); % Applies f to each cell in C
This returns a new cell array containing the squared values of the original cells.
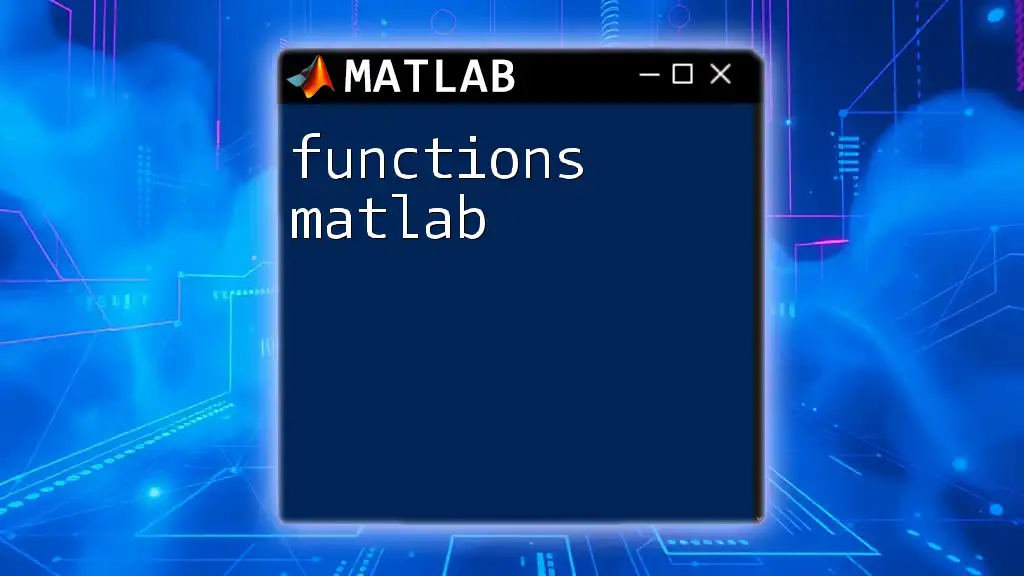
Advanced Topics in Function Handles
Nested Functions and Handle Creation
You can define nested functions that are local to another function's context. This allows you to create a function handle referencing a nested function:
function outerFunction()
a = 10; % Outer variable
nestedHandle = @(x) a + x; % Nested function that uses the outer variable
disp(nestedHandle(5)); % Outputs 15
end
This approach enables the encapsulation of logic and variable scope in a way that adheres to clean programming principles.
Function Handles with Multiple Inputs
It’s important to note that function handles can accept multiple inputs. Create a function handle like so:
multiInput = @(x, y) x + y; % A function that adds two inputs
result = multiInput(2, 3); % Outputs 5
This versatility allows for handling various scenarios where functions might need multiple parameters for computation.
Exploring Function Handle Metadata
Sometimes, you may want to inspect function handles for debugging or understanding their structure. You can use the `functions` command:
funcs = functions(f); % Get information about the function handle
disp(funcs); % Displays details about the function handle
This output will show you the name, type, and workspace for the referenced function, helping you debug or optimize your code.
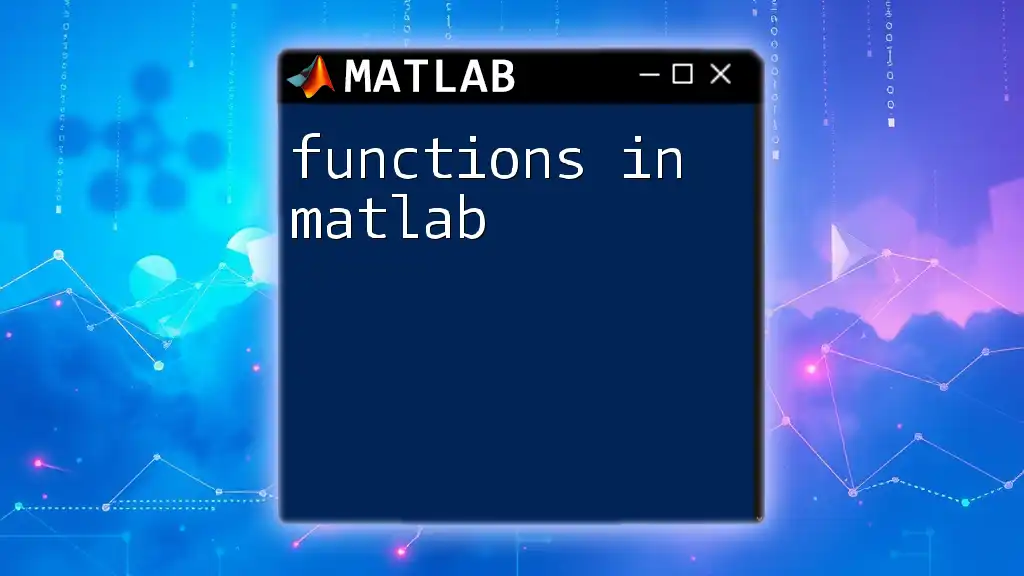
Best Practices for Using Function Handles
When working with function handles, it's essential to keep your code concise and maintain clarity. Here are a few tips to enhance your coding practice:
- Comment Your Code: Always add comments to articulate the purpose and logic behind each function handle, especially for complex operations.
- Use Descriptive Names: Name your anonymous functions meaningfully, as this makes your code self-explanatory.
- Test Reusability: Aim to structure function handles in a way that promotes their reuse across different functions and scripts.
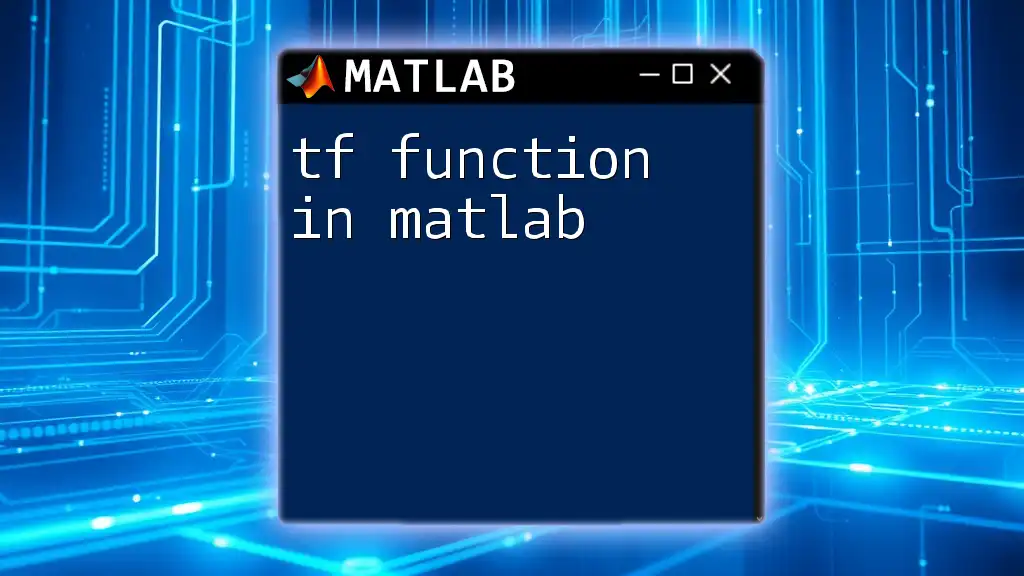
Conclusion
Function handles in MATLAB empower you to create more flexible, reusable, and maintainable code. By understanding their capabilities—from basic creation and usage to advanced applications—you can enhance your MATLAB programming skills. We encourage you to experiment with function handles and think creatively about how to apply them in your projects!
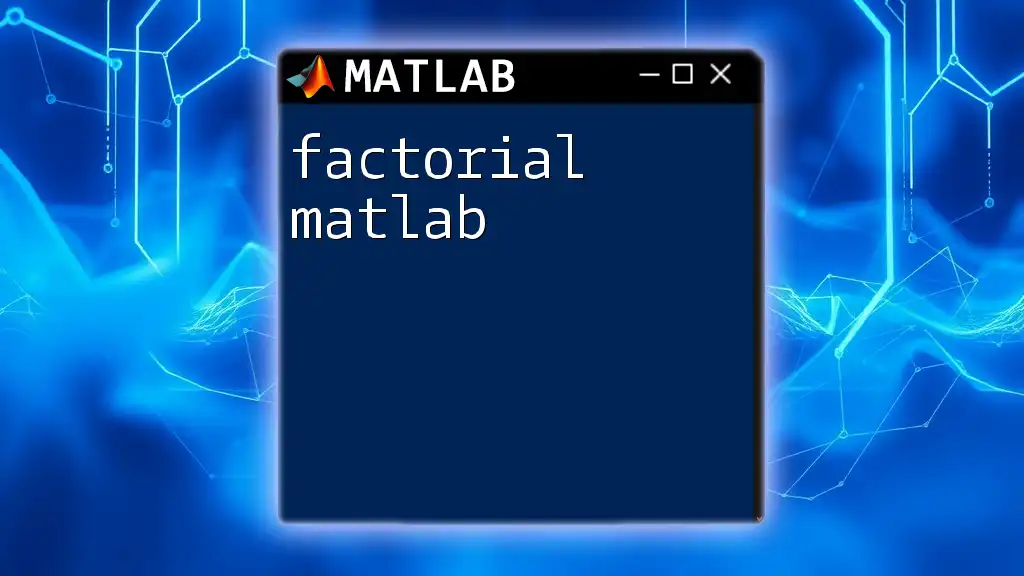
Additional Resources
For further reading, consider exploring MATLAB’s official documentation on function handles, as well as recommended books and tutorials to deepen your understanding of this vital programming aspect.