In MATLAB, a lambda function, also known as an anonymous function, allows you to create simple, one-line functions without needing to define a separate function file.
Here's a code snippet demonstrating a lambda function in MATLAB:
% Lambda function that squares its input
square = @(x) x.^2;
% Example usage
result = square(5); % result is 25
Introduction to Lambda Functions
Lambda functions, also known as anonymous functions, serve as powerful tools in MATLAB that allow users to create simple functions without the need to formally define them. These functions can accept any number of inputs but can only return one output. This contrasts with traditional function definitions, which require a separate file or a more extensive code structure.
The benefits of using lambda functions in MATLAB are significant. They simplify code, reduce the need for boilerplate function files, and allow for quick calculations in a clean and concise manner. They are particularly useful for short, one-off operations where defining a full function might be overkill.
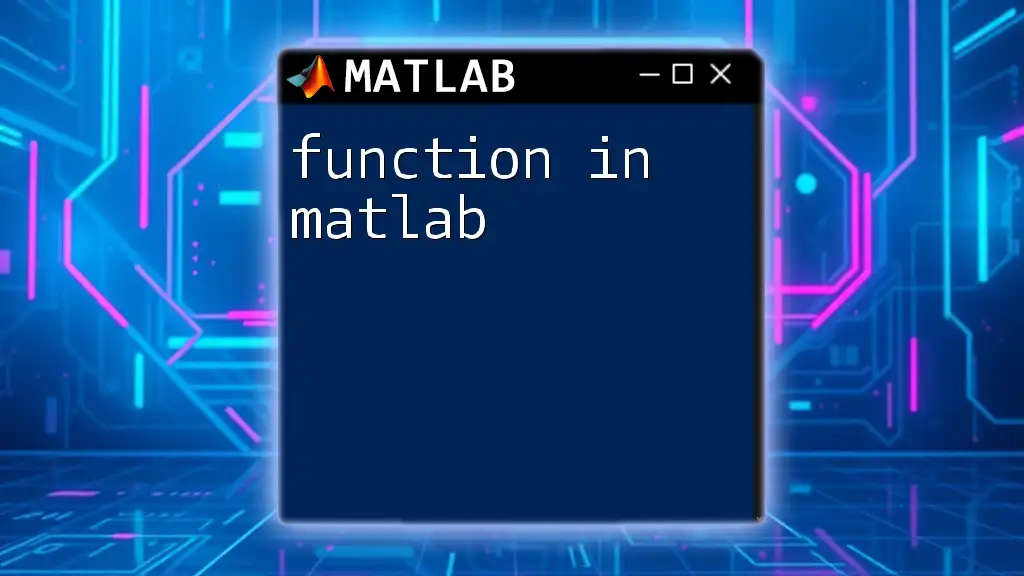
Creating Lambda Functions in MATLAB
Simple Syntax
Creating a lambda function in MATLAB is straightforward and uses the `@` symbol followed by the input variables in parentheses, and a single-line expression. Here's the basic syntax:
f = @(x) x^2;
In this example, `f` is a lambda function that takes a single input `x` and returns its square.
Variables and Parameters in Lambda Functions
Passing parameters to lambda functions is flexible. Lambda functions can handle multiple input variables, allowing you to perform operations that involve several data points.
f = @(x, y) x + y;
In this case, the lambda function `f` sums the two inputs `x` and `y`.
Additionally, using `varargin` allows defining functions that can take a variable number of inputs. This means the function can adapt based on how many arguments you provide:
f = @(varargin) sum(cell2mat(varargin));
This lambda function calculates the sum of all inputs passed to it, regardless of their number, showcasing the flexibility of lambda functions.
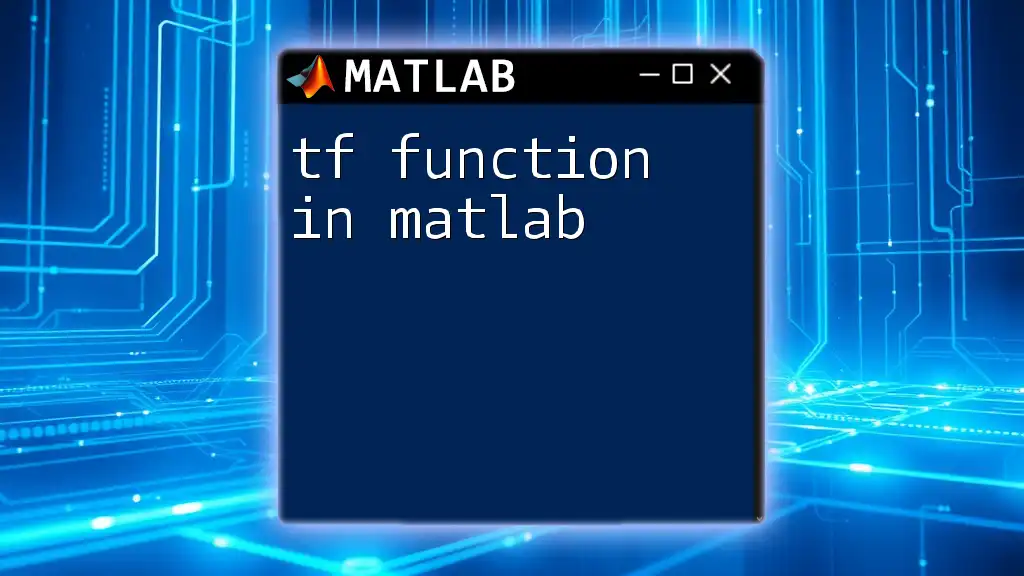
Applications of Lambda Functions
Mathematical Operations
Lambda functions are especially useful in data analysis. You can quickly apply mathematical operations to arrays or matrices. For instance, using `arrayfun`, you can efficiently perform calculations on each element of an array:
result = arrayfun(@(x) x^2, [1, 2, 3, 4]);
Here, `arrayfun` applies the lambda function that squares each element of the input array.
Optimization Problems
In optimization scenarios, lambda functions are invaluable. They can be used in various optimization algorithms to define the objective function—what we aim to minimize or maximize. For example:
f = @(x) (x-3)^2; % Function to minimize
[x_min, f_min] = fminunc(f, 0);
In this example, `fminunc` minimizes the lambda function defined, which is a simple quadratic function. The method finds the value of `x` that minimizes `f(x)`.
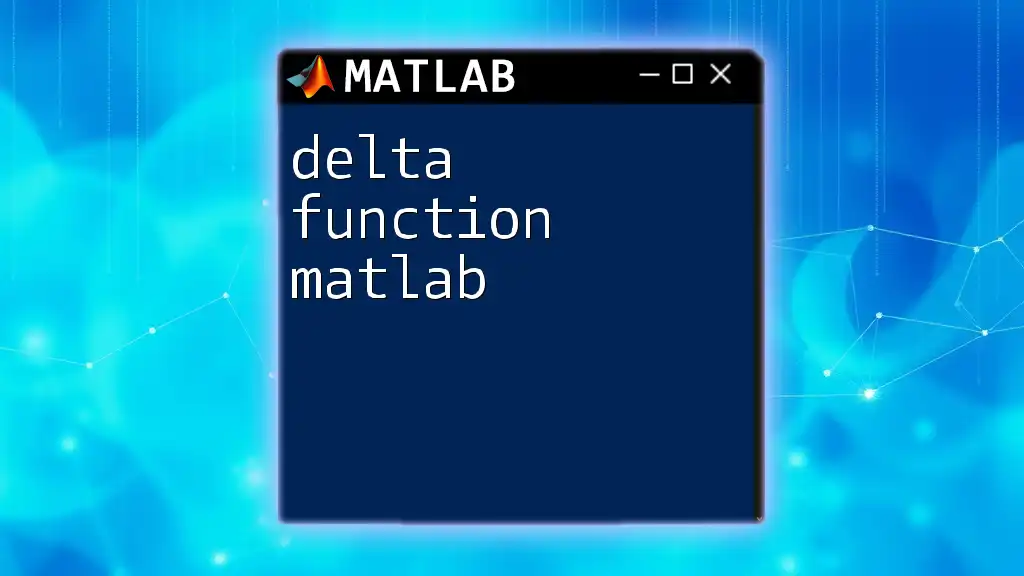
Visualizing Functions with Lambda
Plotting Lambda Functions
Visualizing mathematical functions helps in understanding their behavior. MATLAB's `fplot` function can be paired with lambda functions for plotting:
f = @(x) sin(x);
fplot(f, [-2*pi, 2*pi]);
title('Plot of the sine function');
This code snippet plots the sine function defined through a lambda function over the specified interval, allowing for quick graphical insight into the function’s properties.
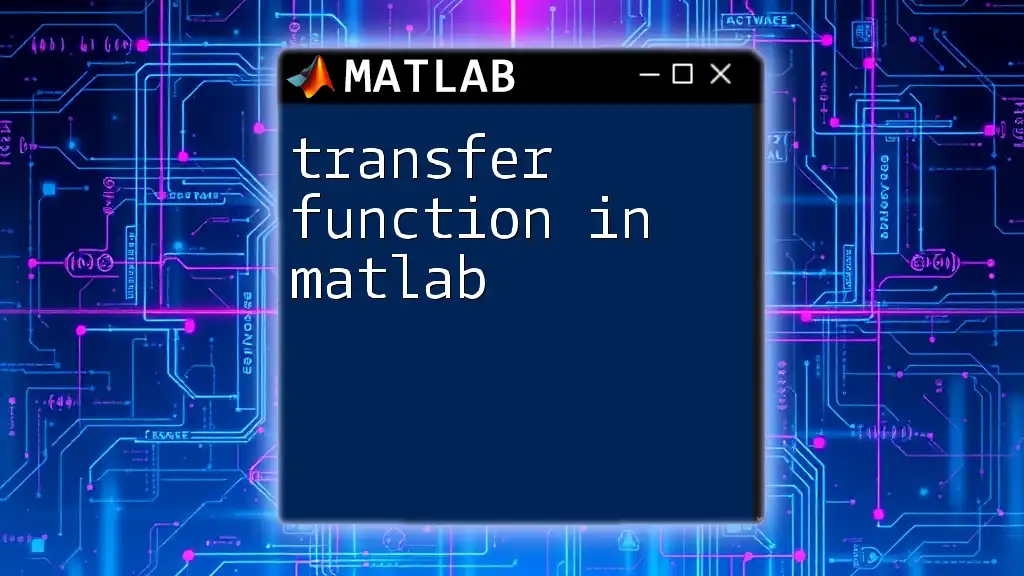
Limitations of Lambda Functions
Scope and Context
While lambda functions offer simplicity, it's essential to understand their scope limitations. Variables defined outside the lambda function are accessible, but there are restrictions on variable persistence. For instance, unlike traditional functions, lambda functions do not allow for persistent or global variables, which can lead to issues if not properly managed.
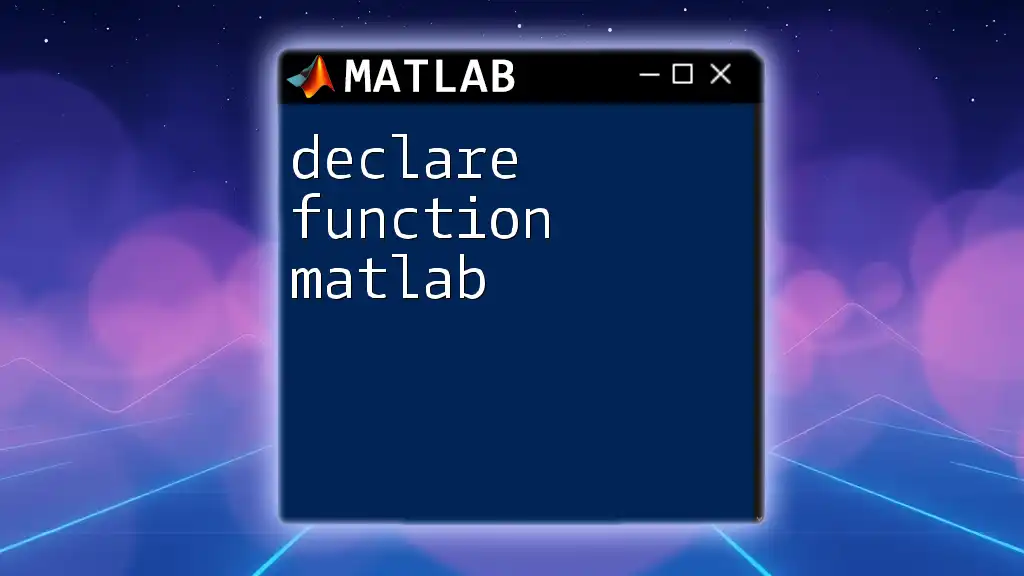
Best Practices for Using Lambda Functions
Readability and Maintainability
When using lambda functions, clarity is vital. While they can simplify coding, it's crucial to write them in a way that retains readability. Avoid overly complex operations in a single expression; instead, consider breaking it down into simpler components.
When to Use Lambda Functions
Knowing when to utilize lambda functions is equally important. They are ideal for quick calculations or when you need a temporary function for a specific task. However, if the operation is complex or reused multiple times, it may be better to define a full function.
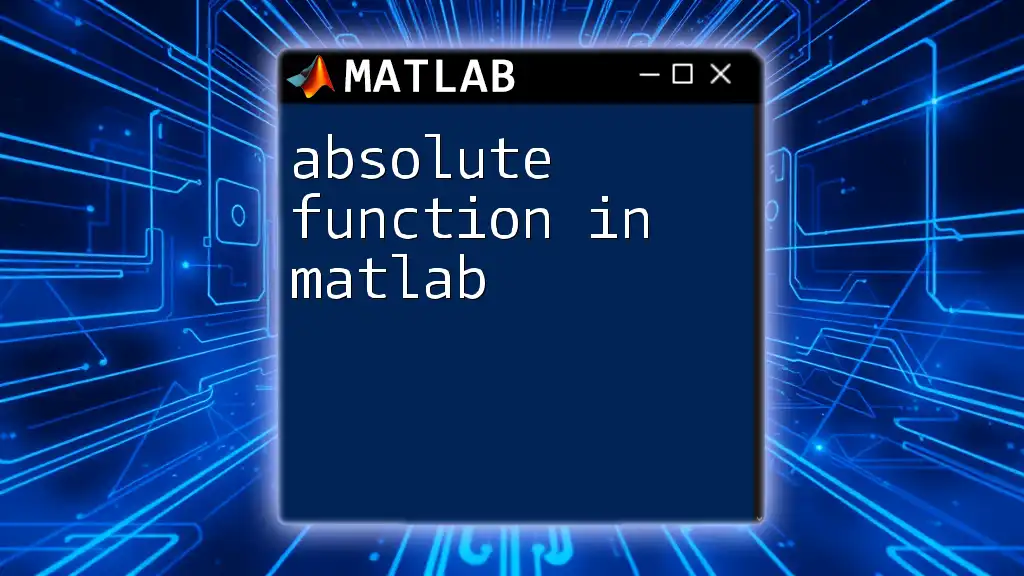
Real-World Examples
Data Processing
In data processing scenarios, lambda functions can serve various purposes. For example, you can apply a lambda function to manipulate a dataset efficiently.
data = [1, 2, 3, 4, 5];
processed_data = arrayfun(@(x) x*2, data);
This snippet effectively doubles each element in the `data` array, demonstrating how lambdas streamline operations on datasets.
Integration with Other MATLAB Functions
Lambda functions can seamlessly integrate with built-in MATLAB functions for enhanced functionality:
myCellArray = {1, 2, 3, 4};
result = cellfun(@(x) x^2, myCellArray);
Here, `cellfun` is used to apply the lambda function that squares each element of the cell array, showcasing the versatility of using lambda functions in conjunction with MATLAB's capabilities.
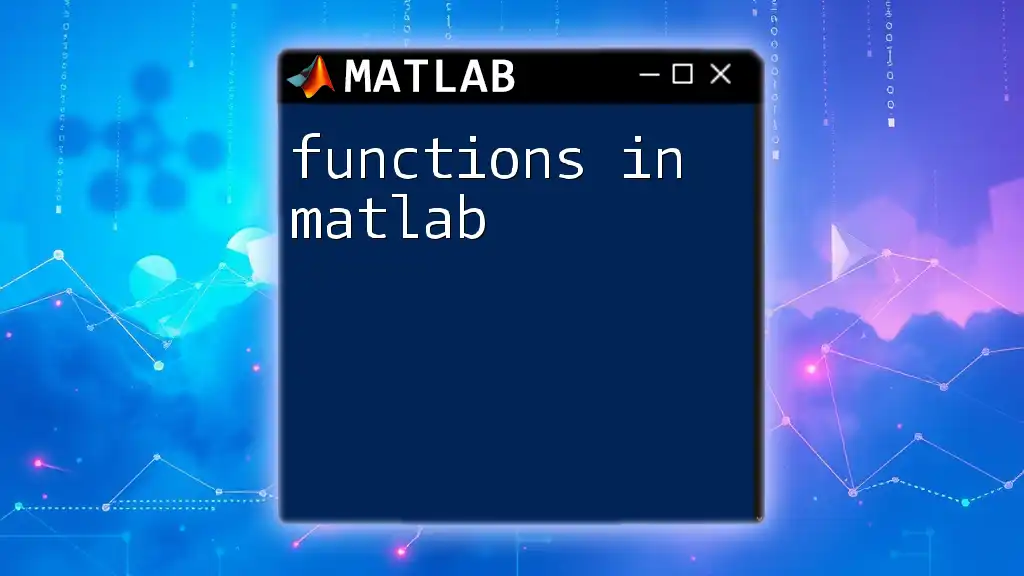
Frequently Asked Questions
It's common for users to have questions when first encountering lambda functions. Some frequently asked questions include:
- How do I debug a lambda function?
- Can lambda functions access variables defined in the main workspace?
By addressing these queries, new users can gain a better understanding of how to effectively use lambda functions in MATLAB.
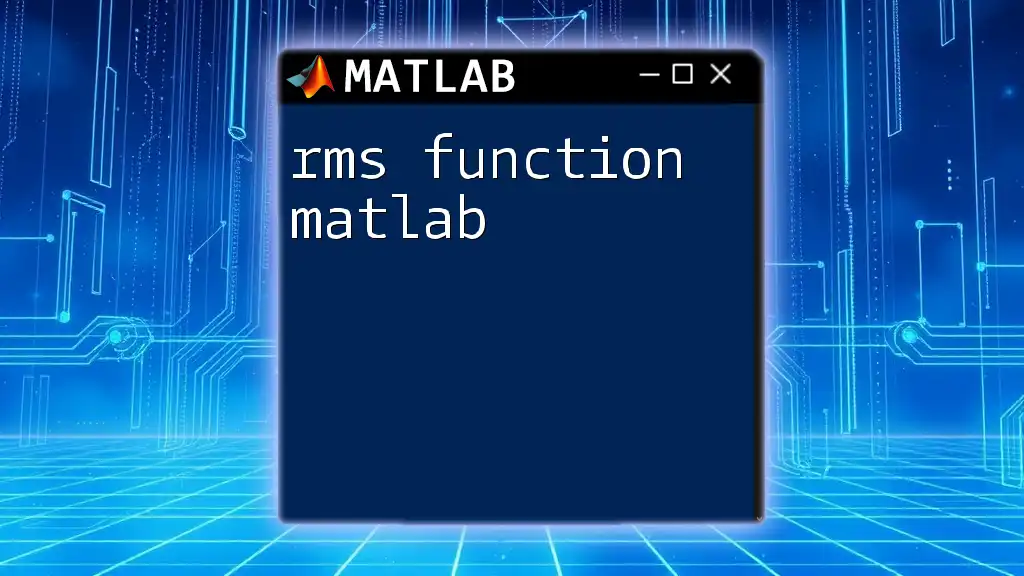
Conclusion
Lambda functions in MATLAB offer a concise and powerful way to define functions without the overhead of formal function files. They are beneficial for quick calculations, data manipulation, and mathematical operations. By grasping their syntax, applications, and limitations, users can effectively leverage lambda functions to enhance their coding experience.
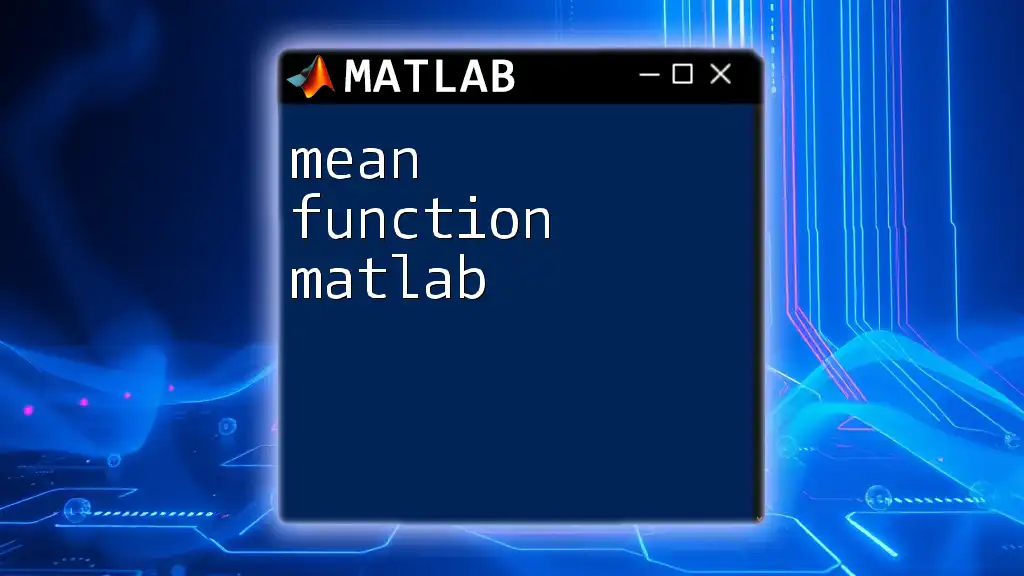
Additional Resources
To further enhance your understanding of lambda functions in MATLAB, consider exploring the official MATLAB documentation, online tutorials, and community examples that provide deeper insights and practical applications in various scenarios.