To call a function in MATLAB, simply use the function name followed by parentheses, including any input arguments if required.
Here's a code snippet illustrating how to call a built-in function, such as `sqrt`, to calculate the square root of a number:
result = sqrt(25);
Understanding MATLAB Functions
What is a Function?
A function in programming is a block of code designed to perform a specific task. In MATLAB, functions are essential for structuring code efficiently and enabling code reuse. Unlike scripts that operate on data in the main workspace, functions have their own independent workspace, meaning they can use and manipulate their own input and output parameters without affecting other parts of your program.
Structure of a MATLAB Function
A MATLAB function generally contains three core components:
-
Function Signature: The header of a function defines the name of the function and lists its input and output parameters.
An example of a function definition is shown below:
function [output1, output2] = myFunction(input1, input2) % Function code goes here end
-
Input and Output Variables: These are crucial for passing information into and receiving results from functions. Understanding how to handle inputs and outputs is essential to mastering how to call a function in MATLAB.
For instance, consider a function that calculates the area and perimeter of a rectangle based on its width and height:
function [area, perimeter] = rectangleProperties(width, height) area = width * height; perimeter = 2 * (width + height); end
-
Function Body: This part includes the actual code that specifies what the function does, leveraging the input parameters to produce output.
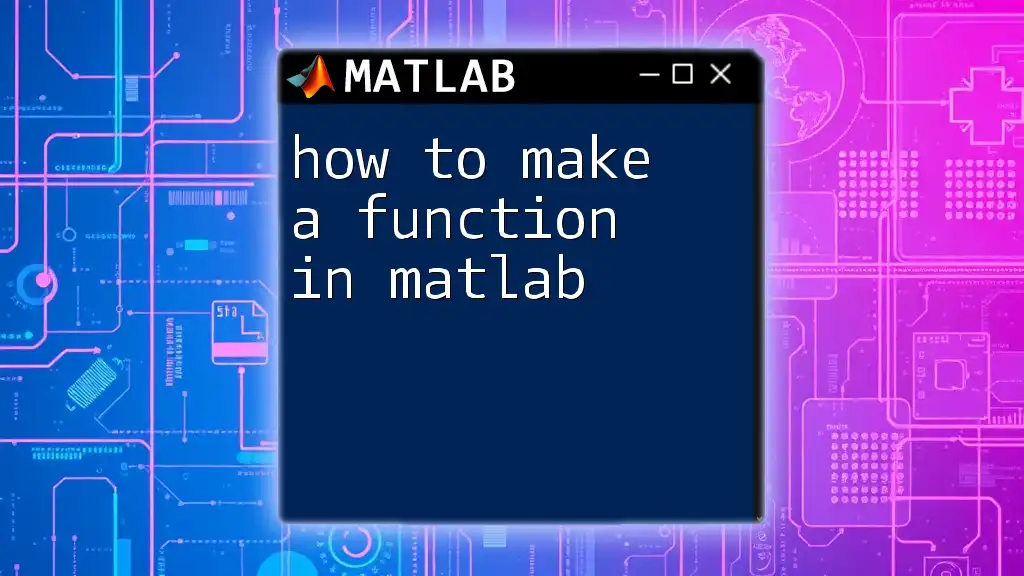
How to Call a Function in MATLAB
Calling Built-in Functions
MATLAB comes with a plethora of built-in functions designed to make numerical computations easier. These can be called simply by using their name followed by parentheses containing the required arguments.
For example, to calculate the square root of 16, you can call the built-in `sqrt` function as follows:
result = sqrt(16);
This call returns the result `4`, demonstrating how straightforward it is to invoke built-in functions in MATLAB.
Calling User-defined Functions
How to Create a User-defined Function
Creating your own function in MATLAB is a powerful way to extend the software’s capabilities. Here are the steps:
-
Naming: The function file should be saved with the same name as the function itself to be recognized properly by MATLAB.
-
Structure: A user-defined function typically has a header, input parameters, and a body of code.
Here’s an example of how to create a user-defined function to calculate the area of a circle:
function area = calculateArea(radius)
area = pi * radius^2;
end
This function simply takes the radius as input and outputs the calculated area.
How to Call Your User-defined Function
Once you've defined your function, calling it is simple. You only need to use the function name followed by the required arguments in parentheses:
a = calculateArea(5);
In this instance, `a` would store the value approximately equal to `78.54`, as it computes the area for a circle with a radius of 5.
When calling functions, it’s crucial to ensure that the data types of inputs match what the function expects, as this maintains efficiency and reduces errors.
Additionally, calling a function from a different file is as easy as ensuring the function's file is in your current working directory or included in MATLAB’s path.
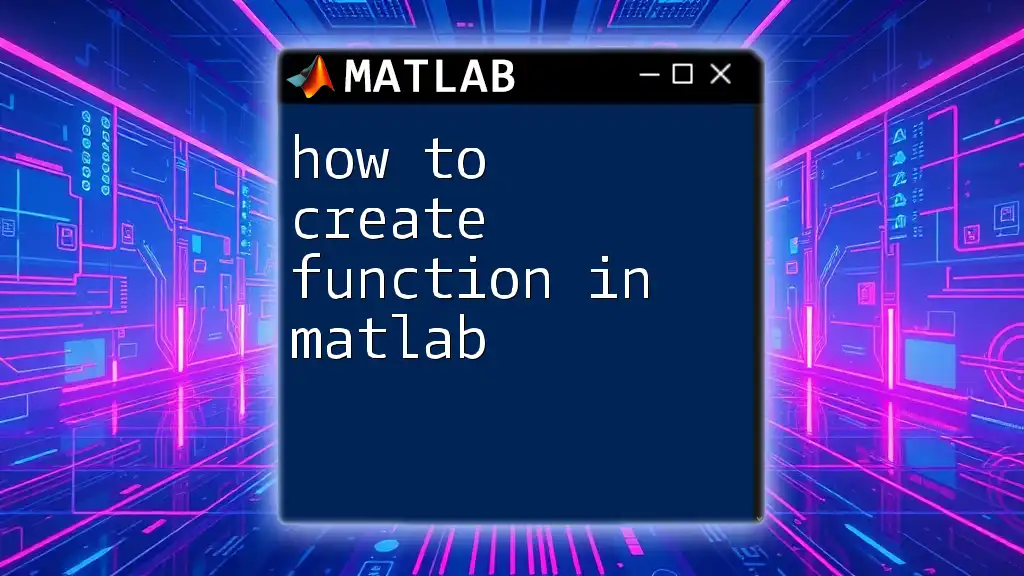
Function Overloading and Default Values
Function Overloading
Function overloading allows you to define multiple versions of a function that can handle different types or numbers of input arguments. MATLAB will select the appropriate version based on the inputs it receives.
Here’s an example demonstrating this concept:
function output = multiply(a, b)
output = a * b; % Works with two numbers
end
function output = multiply(a, b, c)
output = a * b * c; % Works with three numbers
end
In this case, MATLAB will call the two-parameter version if two inputs are provided and the three-parameter version for three inputs.
Setting Default Values for Parameters
Setting default values for parameters allows for flexibility when calling functions. You can use `varargin` to handle variable inputs effectively.
Here’s how you can accomplish this:
function output = myFunction(varargin)
if nargin == 0
output = 'No inputs provided';
else
output = sum(cell2mat(varargin));
end
end
In this example, if no arguments are supplied, the function returns a message. If arguments are provided, it sums them.
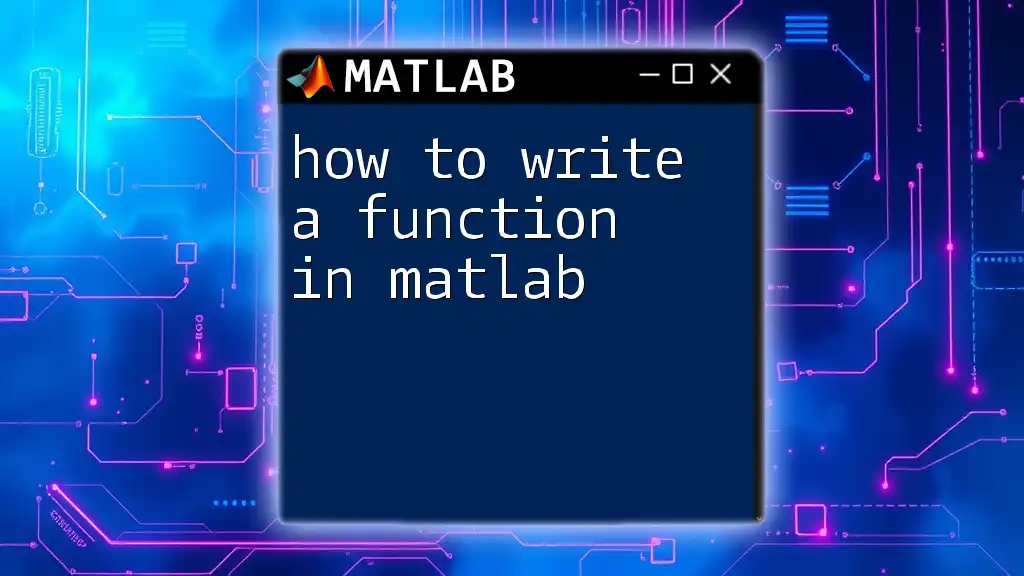
Debugging Function Calls
Common Errors When Calling Functions
When calling functions, you may encounter typical mistakes such as passing incompatible data types, omitting required inputs, or even using an incorrect function name. These mistakes can trigger informative error messages.
For example, calling a function without providing the required number of arguments might produce an error like:
Error using myFunction (line X)
Not enough input arguments.
Understanding how to read and interpret these messages is crucial for effective debugging.
Techniques for Debugging Function Calls
MATLAB provides several debugging tools:
-
Using Breakpoints: You can set breakpoints in your function code to pause execution and inspect variable values at different stages for a clearer understanding of what’s happening.
-
Reviewing Function Code: Debugging often involves reviewing your function to ensure that the logic is sound and follows best practices.
-
Using the MATLAB Debugger: MATLAB’s built-in debugger serves as an essential tool for tracking down errors and understanding function flow.
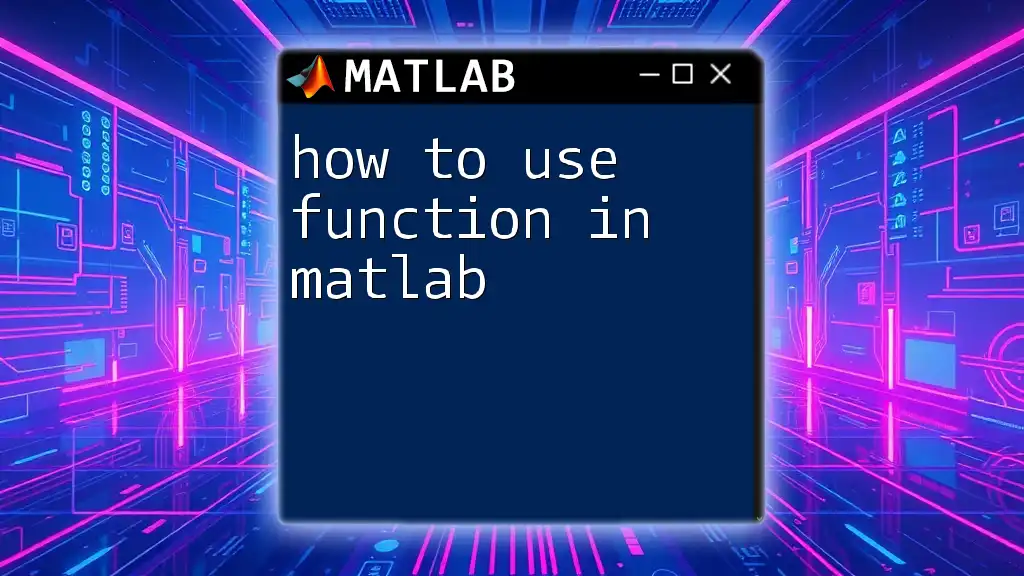
Conclusion
This guide covered the fundamental aspects of how to call a function in MATLAB, from understanding functions and their structure to creating and calling user-defined functions, handling function overloading, and the importance of debugging. By practicing these points, you will become more proficient in using MATLAB for your programming and data analysis needs. Remember, the key to mastering MATLAB is regular practice and experimenting with different functionalities to expand your skill set.