To create a function in MATLAB, define it using the `function` keyword followed by the output variable, function name, and input parameters in a separate file or within a script.
Here’s a simple example:
function output = squareNumber(input)
output = input^2;
end
Understanding MATLAB Functions
What is a Function?
A function in MATLAB is a self-contained block of code designed to perform a specific task. It takes inputs, processes them, and provides outputs, making it an essential building block in programming. Functions help in organizing code logically, reducing redundancy, and enhancing reusability.
Key Differences between functions and scripts are significant:
- Input Arguments: Functions can accept inputs, whereas scripts cannot.
- Output Arguments: Functions can return outputs, while scripts do not have return values.
- Scope of Variables: Functions have their own workspace, meaning variables defined within a function are not accessible outside of it.
Structure of a MATLAB Function
The basic syntax of a MATLAB function is straightforward. Every function starts with the `function` keyword, followed by the output variables, the function name, and the input variables. The general format is:
function [outputs] = functionName(inputs)
Components of a function include:
- Input Arguments: Variables you pass into the function.
- Output Arguments: Variables that the function returns after execution.
- Function Body: The section where the main code lies to perform the task.
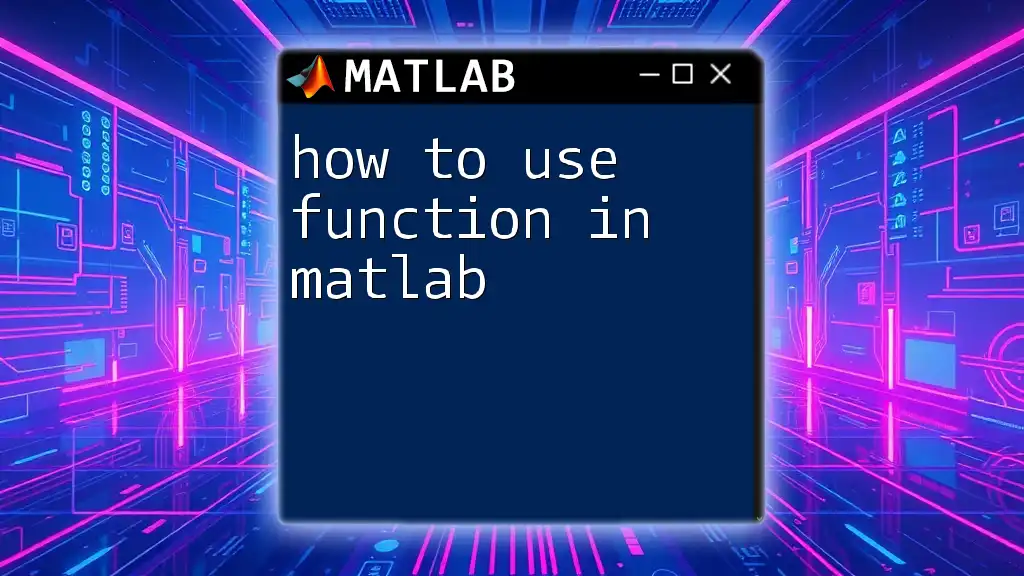
Creating Your First Function
Step-by-Step Guide
Setting up a function in MATLAB begins with creating a new `.m` file. This file should be named the same as the function itself to avoid confusion. For example, if your function is called `addTwoNumbers`, the file should be named `addTwoNumbers.m`.
Example Function: Adding Two Numbers
Here’s a simple example of a function that adds two numbers:
function sum = addTwoNumbers(a, b)
sum = a + b;
end
Explanation of the example code:
- `function sum = addTwoNumbers(a, b)` defines the function and its input/output structure.
- Inside the function body, `sum = a + b;` performs the addition, which is then returned as the output.
To call the function, you simply type:
result = addTwoNumbers(3, 5);
This will return `8` and store it in `result`.
Common Pitfalls
Avoiding Syntax Errors
Proper syntax and structure are crucial for successful function creation. Ensure that the function name matches the filename and that you close all parentheses correctly.
Ensuring Input Parameters Are Valid
It’s essential to check that the inputs are of the expected type. Here's how you can implement basic error handling using an example function:
function result = safeAdd(a, b)
if ~isnumeric(a) || ~isnumeric(b)
error('Inputs must be numeric.');
end
result = a + b;
end
In this example, `safeAdd` ensures that both inputs are numeric before proceeding with the addition. If not, it displays an error message.
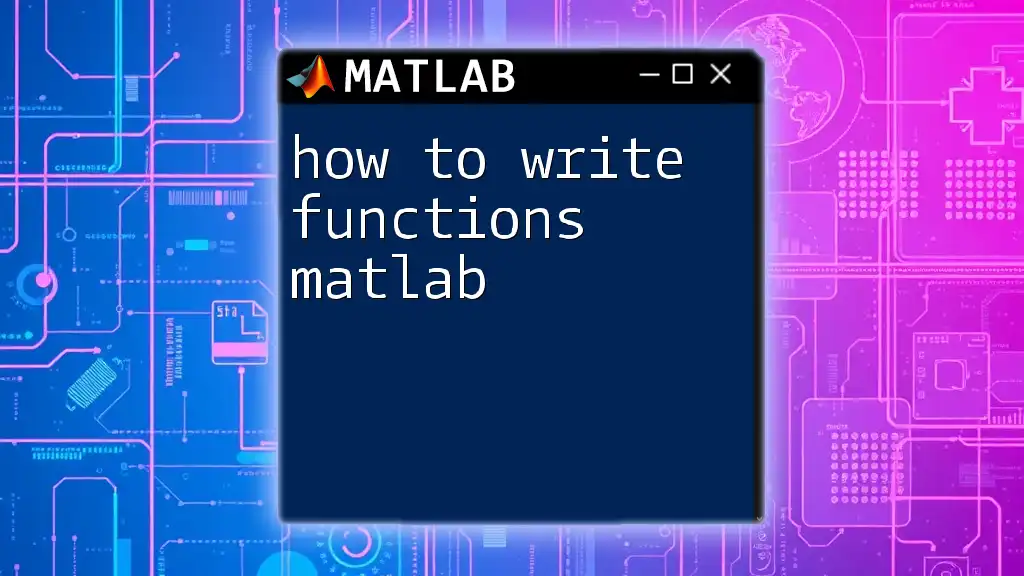
Advanced Function Features
Multiple Inputs and Outputs
MATLAB functions can handle flexible inputs and multiple outputs, which significantly enhances their utility.
Handling Multiple Inputs
To accommodate a variable number of inputs, you can use `varargin`, as shown in this example:
function output = flexibleAdd(varargin)
output = sum([varargin{:}]);
end
This `flexibleAdd` function allows you to input any number of numeric values and returns their sum.
Returning Multiple Outputs
You can define multiple output variables by enclosing them in square brackets. Here's an example:
function [sum, product] = basicMath(a, b)
sum = a + b;
product = a * b;
end
Calling this function would look like this:
[s, p] = basicMath(3, 5);
Here, `s` will store the sum, while `p` will hold the product.
Nested Functions
Nested functions are beneficial for encapsulating functionality and controlling variable scope. A nested function is defined within another function, allowing access to its variables.
Example of Nested Functions
Here's an example of how nested functions work:
function outerFunction()
function innerFunction()
disp('This is an inner function.');
end
innerFunction();
end
In this example, `innerFunction` can access variables from `outerFunction`, which delineates a strong connection between them.
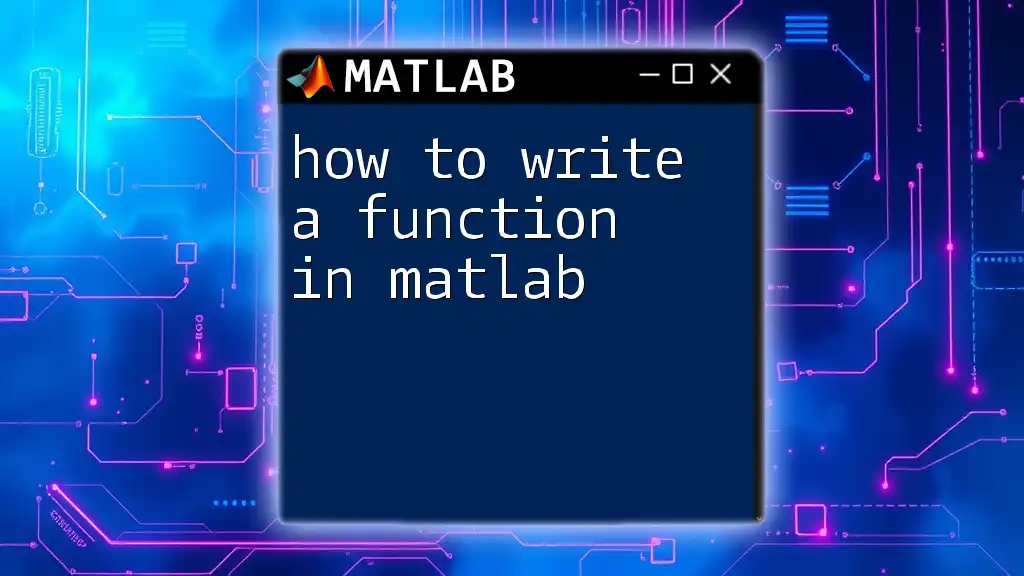
Best Practices for Function Creation
Documentation
Commenting your code is vital for clarity. Documentation helps both the original developer and others who may use the function in the future. Here's an example of well-documented functions:
function sum = addTwoNumbers(a, b)
% This function adds two numbers a and b and returns the sum
sum = a + b;
end
Code Organization
Ensuring that your functions are organized logically is important. Use meaningful names for your functions and variables. Following consistent naming conventions improves readability.
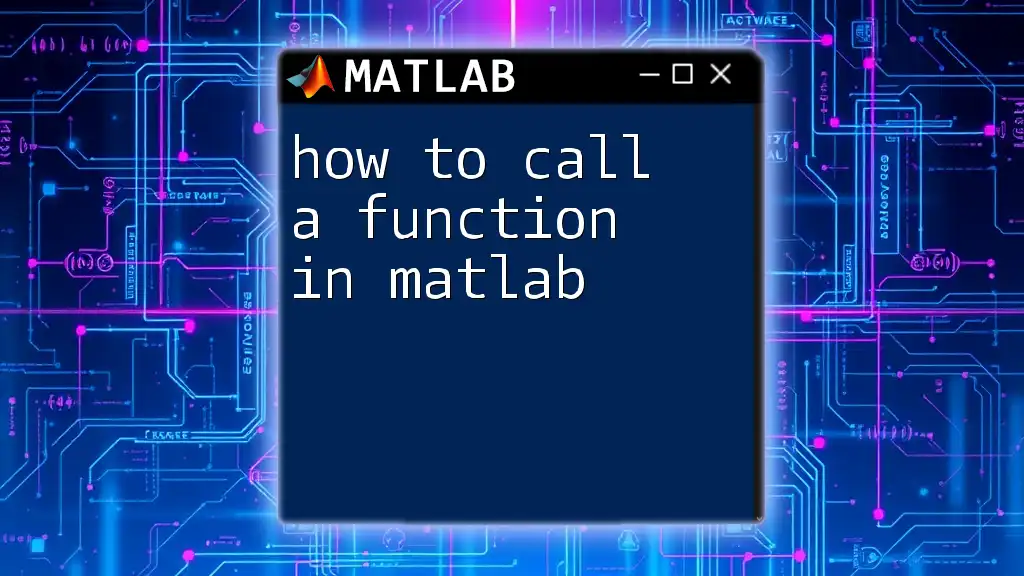
Debugging Your Functions
Common Issues and Solutions
Debugging is an integral part of programming. MATLAB provides robust debugging tools, such as breakpoints, to inspect the state of the program. Here’s a quick description of how to debug your functions:
- Set breakpoints by clicking on the left margin next to the code line.
- Use the command `dbstop if error` to halt execution when an error occurs.
This lets you step through your function step-by-step to identify issues easily.
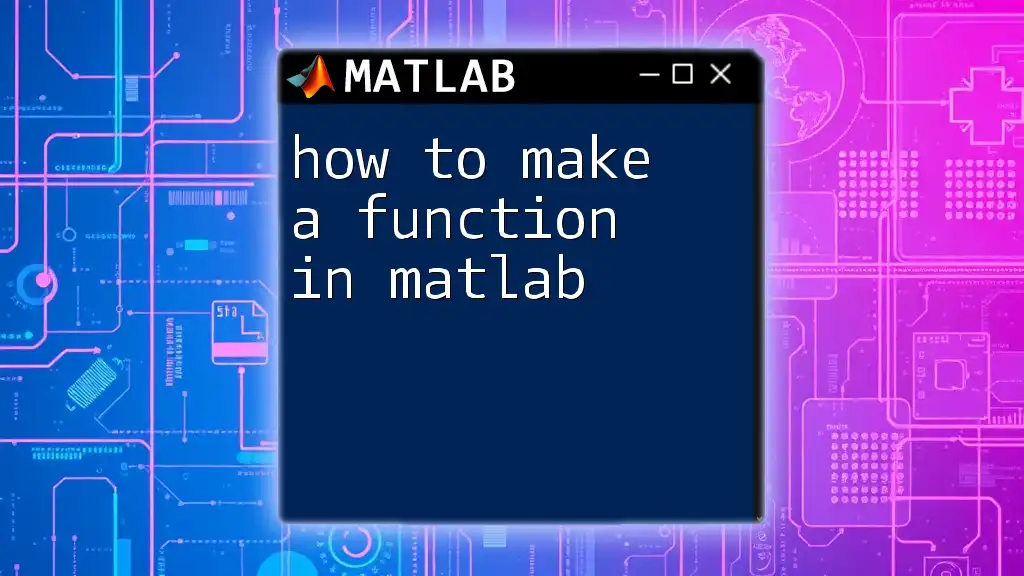
Conclusion
Understanding how to create functions in MATLAB is critical for developing effective code. Functions promote better organization, reduce code redundancy, and enhance code readability. By practicing and implementing the techniques and best practices outlined in this guide, you can master this essential aspect of MATLAB programming. Consider exploring additional resources and communities where you can continue to grow your skills in MATLAB function creation.