The absolute function in MATLAB, denoted as `abs()`, returns the absolute value of a number, ensuring that the output is always non-negative.
Here’s a code snippet demonstrating its usage:
% Example of using the abs function in MATLAB
value = -5; % Define a negative value
absolute_value = abs(value); % Calculate the absolute value
disp(absolute_value); % Display the result
Understanding the Absolute Function
What is an Absolute Function?
The absolute function calculates the magnitude of a number regardless of its sign. In simpler terms, it converts negative values into positive ones. Mathematically, the absolute value is represented as:
\[ |x| = \begin{cases} x & \text{if } x \geq 0 \\ -x & \text{if } x < 0 \end{cases} \]
In this equation, x is any real or complex number, and its absolute value is derived from its distance from zero on the number line. Understanding this function is critical in various applications ranging from mathematical computations to data normalization in engineering and scientific research.
Syntax of the Absolute Function in MATLAB
In MATLAB, the primary syntax for the absolute function is simple:
abs(X)
Where X can be a scalar, vector, matrix, or even a complex number. The output is the absolute value of X with the same dimensions as the input. Knowing this allows users to efficiently leverage MATLAB’s powerful capabilities in handling numerical data.
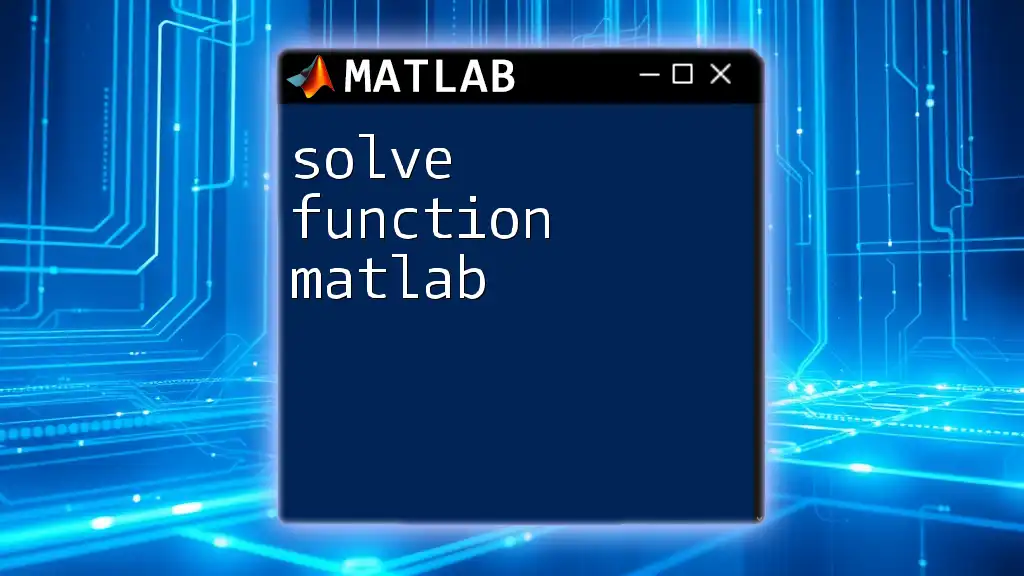
How the Absolute Function Works
Absolute Value of Real Numbers
The absolute function in MATLAB effectively handles real numbers, returning them as positive values. Here’s an example:
% Example: Absolute value of a real number
realNum = -5;
absValue = abs(realNum);
disp(absValue); % Output: 5
In the code above, although the input is a negative number (-5), calling `abs(realNum)` returns 5. This illustrates how the absolute function discards the sign and only retains the magnitude.
Absolute Value of Complex Numbers
When dealing with complex numbers, the absolute function computes the magnitude using the formula:
\[ |a + bi| = \sqrt{a^2 + b^2} \]
Where a is the real part, and b is the imaginary part. A practical example in MATLAB is as follows:
% Example: Absolute value of a complex number
complexNum = 3 + 4i;
absValue = abs(complexNum);
disp(absValue); % Output: 5
In this case, the complex number 3 + 4i has a magnitude of 5. When you execute the `abs(complexNum)` command, MATLAB calculates the result by extracting the square root of the sum of the squares of 3 and 4, which effectively demonstrates the power of the absolute function in handling complex data.
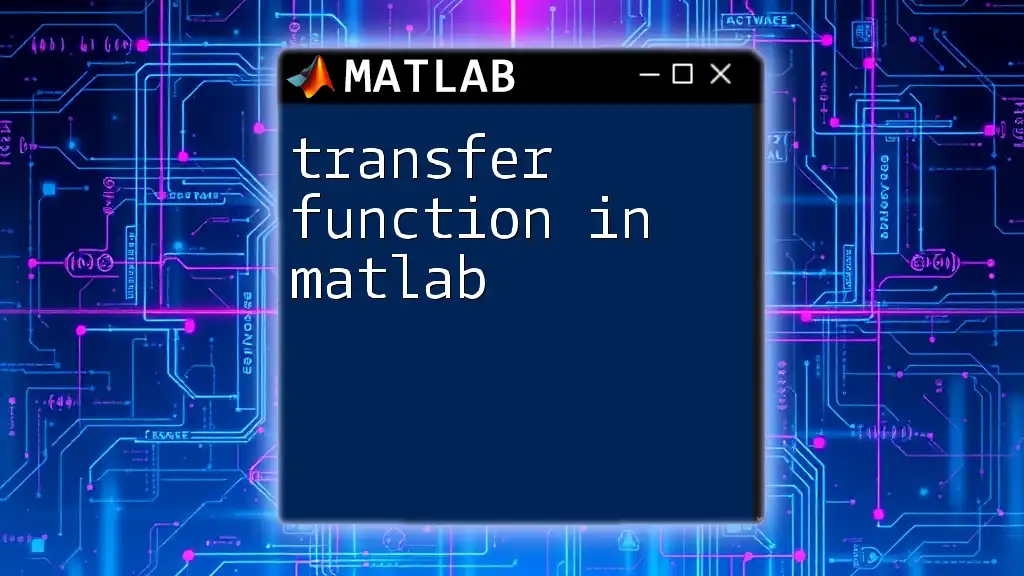
Applications of the Absolute Function
Data Normalization
The absolute function plays a crucial role in data normalization, especially in statistical analyses where it’s essential to negate the effect of negative values. Normalizing data can involve transforming values to fit within a specific range. The absolute values ensure that all data points are positive, aiding in cleaner analysis and comparison.
For instance, if a dataset includes both negative and positive values, using the absolute function will allow you to scale your data uniformly without the bias introduced by negative numbers.
Signal Processing
Signal processing frequently utilizes the absolute function to analyze signals. A common task is to visualize the amplitude of signals without the influence of phase. Consider the following MATLAB example:
% Example: Using abs in a simple signal processing context
t = 0:0.01:1; % Time vector
signal = sin(2 * pi * 5 * t); % Generate a sample signal
absSignal = abs(signal); % Get absolute value
plot(t, absSignal); % Visualize the absolute signal
title('Absolute Value of a Signal');
xlabel('Time (s)');
ylabel('Amplitude');
In this code, a sine wave signal is generated, and applying the absolute function allows it to be plotted as a positive amplitude over time. The resulting graph emphasizes the oscillation without negativity, which can be crucial in understanding peaks and troughs in signal behavior.
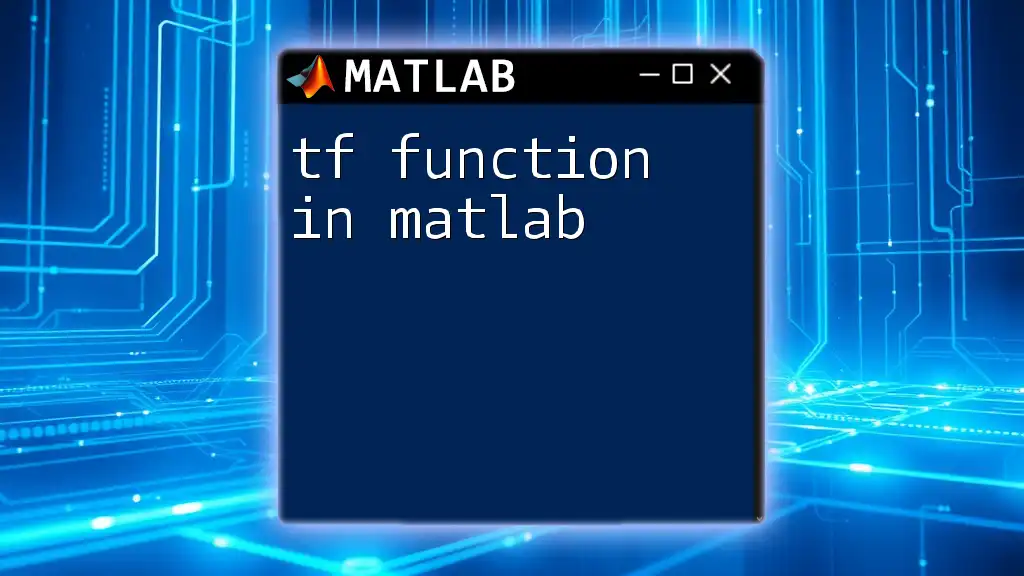
Common Mistakes and Troubleshooting
Misunderstanding Input Types
A common mistake when using the absolute function is inputting non-numeric data types, such as strings or cell arrays, which can lead to errors or unexpected results. To avoid this, always ensure that input values are either numerical scalars, vectors, or matrices. MATLAB will not process non-numeric values and will throw an error, so verifying your data types is essential before applying the absolute function.
Performance Considerations
When working with large datasets, using the absolute function efficiently is paramount. While the absolute operation is generally fast, operating on massive matrices can lead to performance bottlenecks. To optimize your code:
- Preallocate arrays: Instead of dynamically resizing, allocate memory before loops.
- Vectorize operations: Utilize MATLAB’s ability to perform operations on entire arrays at once instead of using loops.
By following these practices, you can improve execution speed whenever you employ the absolute function in MATLAB.
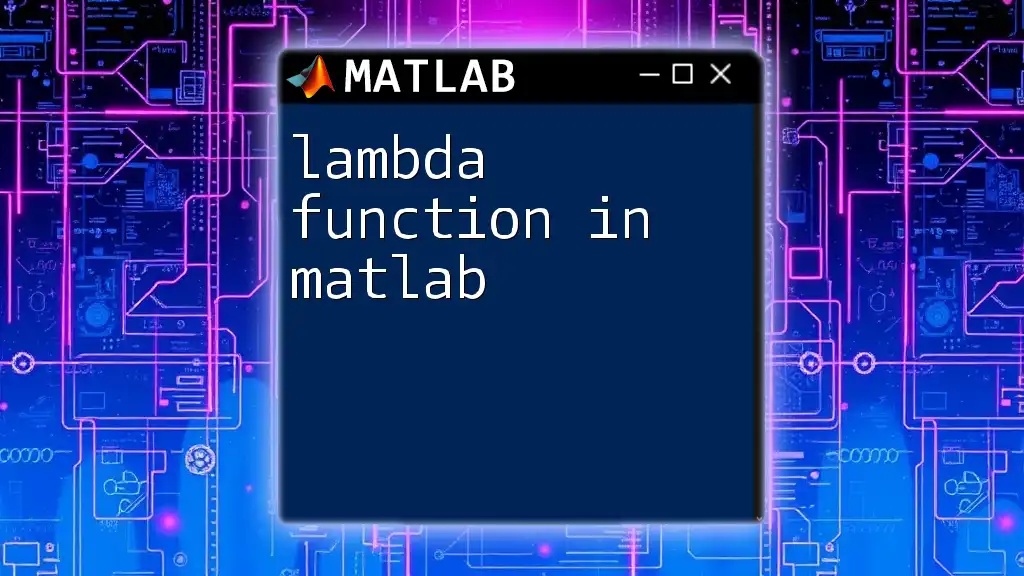
Conclusion
In summary, the absolute function in MATLAB offers a straightforward yet powerful tool to compute magnitudes of both real and complex numbers. Its applications span data normalization to signal processing, showcasing its importance in various fields. As you continue to explore MATLAB, we encourage you to apply the absolute function and experiment with its potential to enhance your programming skills.