The exponential function in MATLAB can be computed using the `exp` function, which calculates \( e^x \) for a given input \( x \).
% Example: Calculate the exponential of 2
result = exp(2);
disp(result); % Displays: 7.3891
What is an Exponential Function?
An exponential function is a mathematical expression in which a constant base is raised to a variable exponent. The general form can be expressed as \( f(x) = a \cdot b^x \), where:
- \( a \) is a constant that represents the initial value,
- \( b \) is the base of the exponential (greater than zero), and
- \( x \) is the exponent.
Exponential functions have unique properties, such as always being positive and having a continuously increasing or decreasing nature, depending on the base. They are foundational in modeling various phenomena in fields such as biology, finance, and physics, such as population growth, radioactive decay, and compound interest.
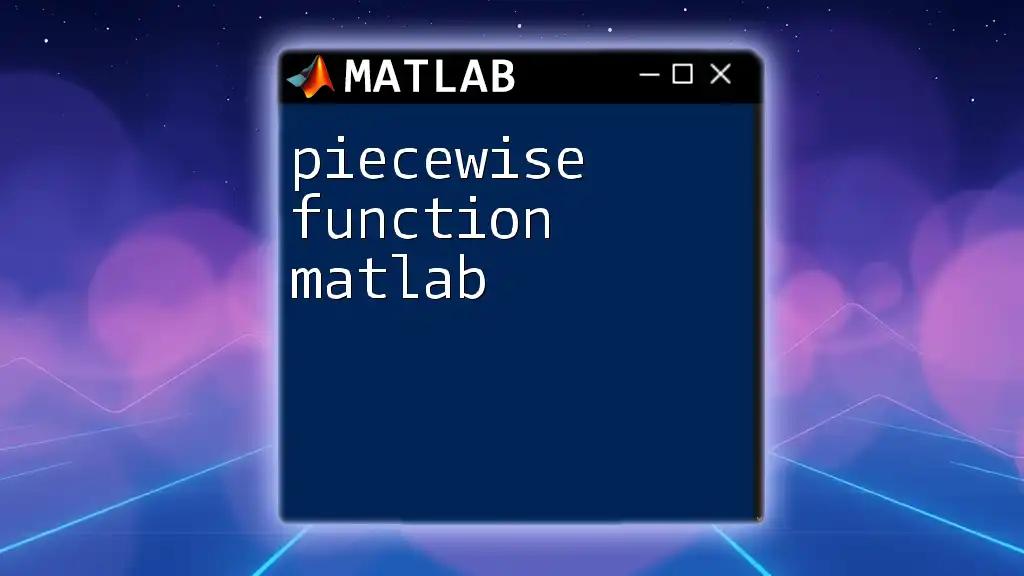
Importance of Exponential Functions in MATLAB
MATLAB is a powerful tool for creating and manipulating exponential functions. Understanding these functions can enhance your skill set in numerous applications, including:
- Engineering: Modeling systems that change rapidly, such as electrical circuits.
- Finance: Analyzing investment growth or depreciation values over time.
- Data Science: Fitting complex data trends to exponential curves.
By mastering the exponential function in MATLAB, you empower yourself to solve real-world problems effectively.
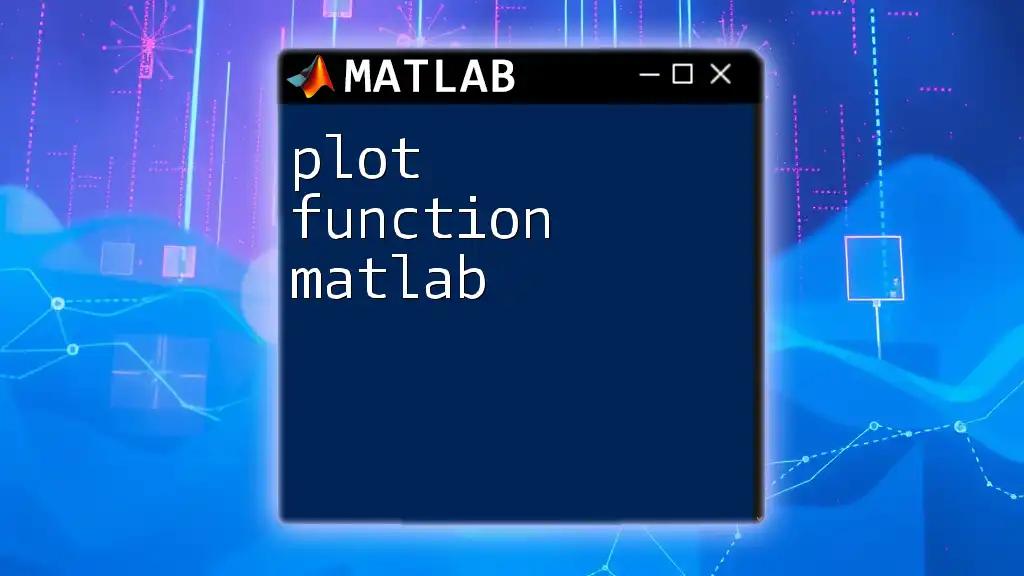
Getting Started with MATLAB
Installing MATLAB
To work with exponential functions in MATLAB, you'll first need to ensure you have the software installed. Download it from the official MathWorks website and follow the installation instructions.
Basic MATLAB Commands
Familiarizing yourself with basic MATLAB commands is crucial. Commands are executed in the Command Window, and scripts or functions can be written and saved in .m files. Start by practicing simple commands and gradually advance to creating scripts.
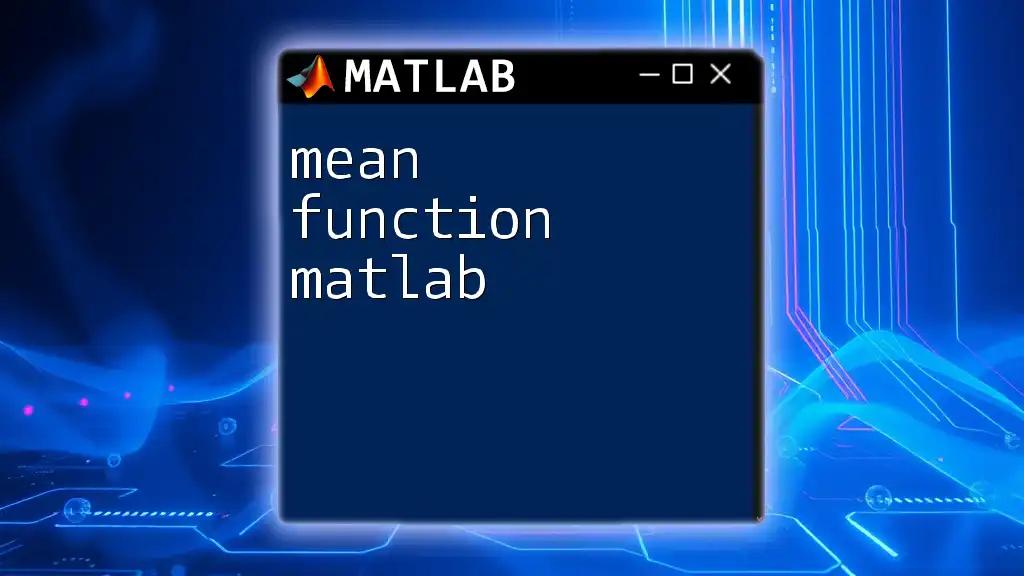
Creating Exponential Functions in MATLAB
Using the exp() Function
MATLAB provides a built-in function, `exp()`, to facilitate calculations involving the mathematical constant e (approximately 2.71828). The syntax is straightforward:
y = exp(x);
Here, x can be a scalar, vector, or matrix, and MATLAB will compute \( e^x \) for each element.
Example:
You can visualize the exponential function by executing the following code:
x = 0:0.1:2; % Create a range of x values
y = exp(x); % Calculate e^x
plot(x, y); % Plotting the exponential function
In this example, the range of x values from 0 to 2 demonstrates how the function exponentially increases, making it easy to observe the characteristic curve.
Custom Exponential Functions
You can also define your own exponential functions in MATLAB using a user-defined function. This adds flexibility for various bases.
For example, you can create a custom function for \( a^x \) as follows:
function y = custom_exp(a, x)
y = a.^x; % Exponential function with base 'a'
end
x = 0:0.1:2; % Create a range
y = custom_exp(2, x); % Calculate 2^x
plot(x, y); % Plotting 2^x
This function allows you to explore exponential growth with bases other than e.
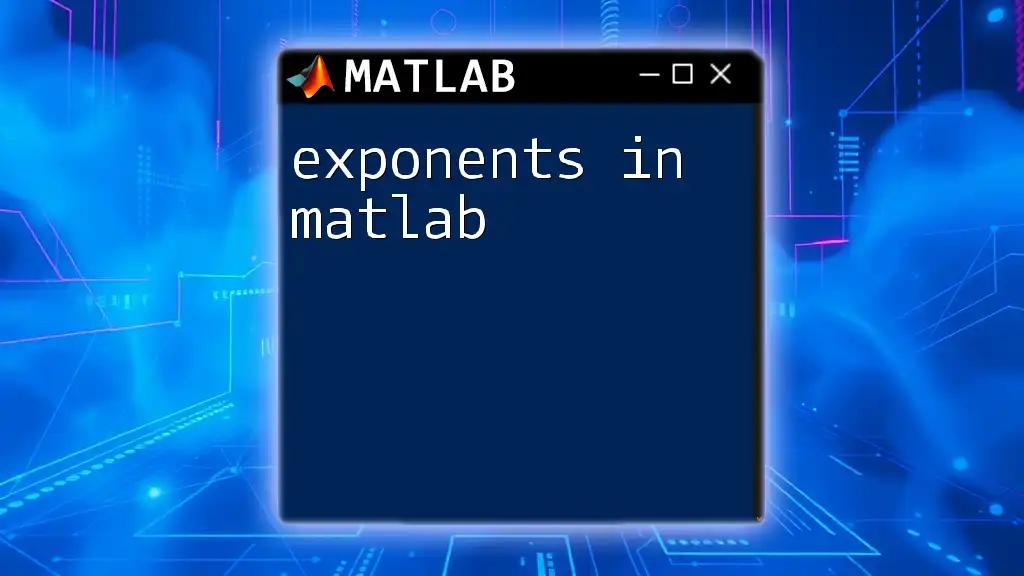
Advanced Exponential Functions
Exponential Growth and Decay
Understanding exponential growth and decay is vital in many sectors. The growth model can be described by the equation \( y = a \cdot e^{rt} \), representing continuous growth, while decay can be represented similarly but with a negative exponent.
Here's how you can visualize both:
t = 0:0.1:5;
a = 1; % Initial amount
r = 0.5; % Rate for growth
y_growth = a * exp(r * t); % Exponential growth formula
y_decay = a * exp(-r * t); % Exponential decay formula
figure;
plot(t, y_growth, 'r', t, y_decay, 'b'); % Plotting both
legend('Growth', 'Decay');
title('Exponential Growth and Decay');
In this code, red represents exponential growth while blue illustrates exponential decay. Observing both plots allows for better understanding of how populations rise and fall or how resources deplete over time.
Using Exponential Functions in Data Analysis
Exponential functions can also be used to fit models to empirical data. MATLAB offers a powerful `fit()` function that can help create a curve-fitting model.
Here's an example:
data_x = [0, 1, 2, 3, 4, 5];
data_y = [2.71, 4.09, 7.39, 13.3, 24.7, 45.4];
fit_model = fit(data_x', data_y', 'exp1'); % Fit an exponential model
plot(fit_model, data_x, data_y); % Plotting the fit against the data
In this case, MATLAB will adjust the exponential curve to fit the provided data points, allowing you to analyze patterns or make predictions based on the fitted model.
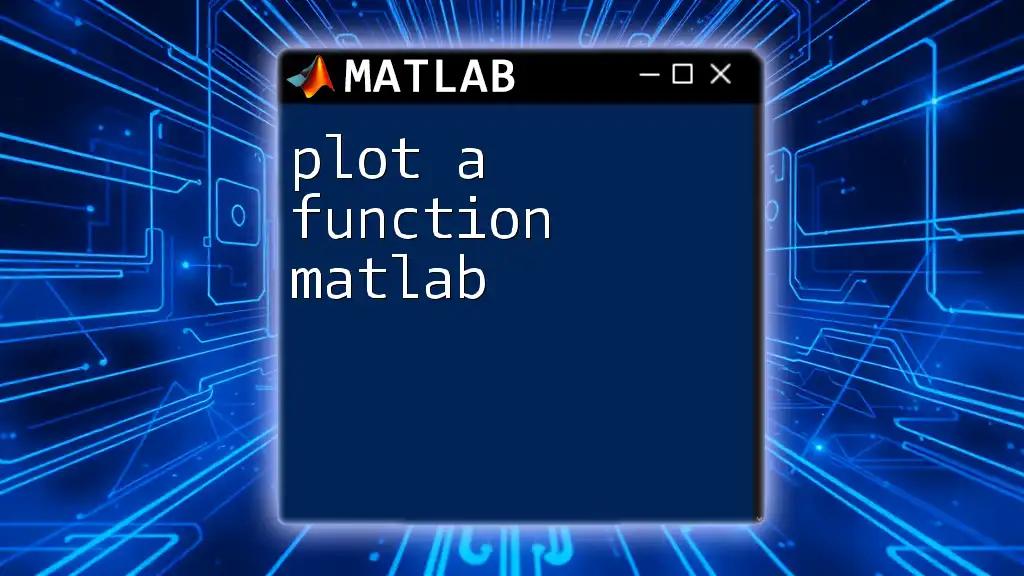
Visualizing Exponential Functions
Plotting Techniques in MATLAB
Visual representation is critical for understanding exponential functions. MATLAB allows for customizable plots, enabling you to enhance the clarity of your data visualization.
Consider this example of a customized plot:
x = 0:0.1:5;
y = exp(x);
plot(x, y, 'g--', 'LineWidth', 2); % Changed color and line style
xlabel('x-axis');
ylabel('e^x');
title('Exponential Function Plot');
grid on; % Adding grid for clarity
By adjusting labels, colors, and styles, you can create visually appealing plots that effectively communicate your findings.
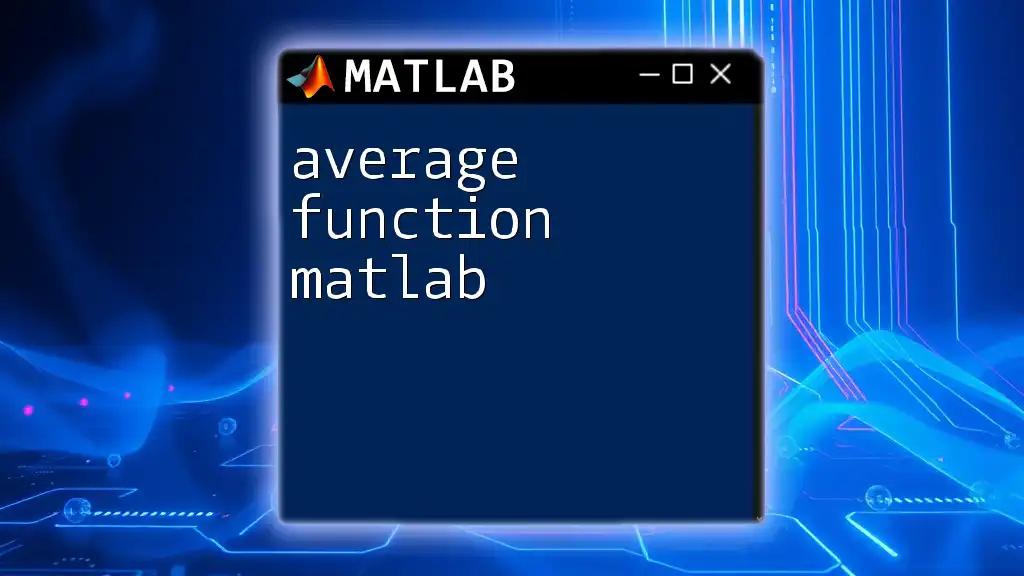
Common Errors and Troubleshooting
Debugging Exponential Function Errors
When working with exponential functions in MATLAB, errors may arise. Common mistakes include misusing the `exp()` function or neglecting to define variable types. Always ensure your input is either a scalar or correctly formatted array.
Performance Considerations
When dealing with large datasets, performance optimization becomes crucial. Avoid loops when executing element-wise operations. Instead, utilize vectorization techniques, as they significantly enhance execution speed in MATLAB, especially for large-scale calculations.
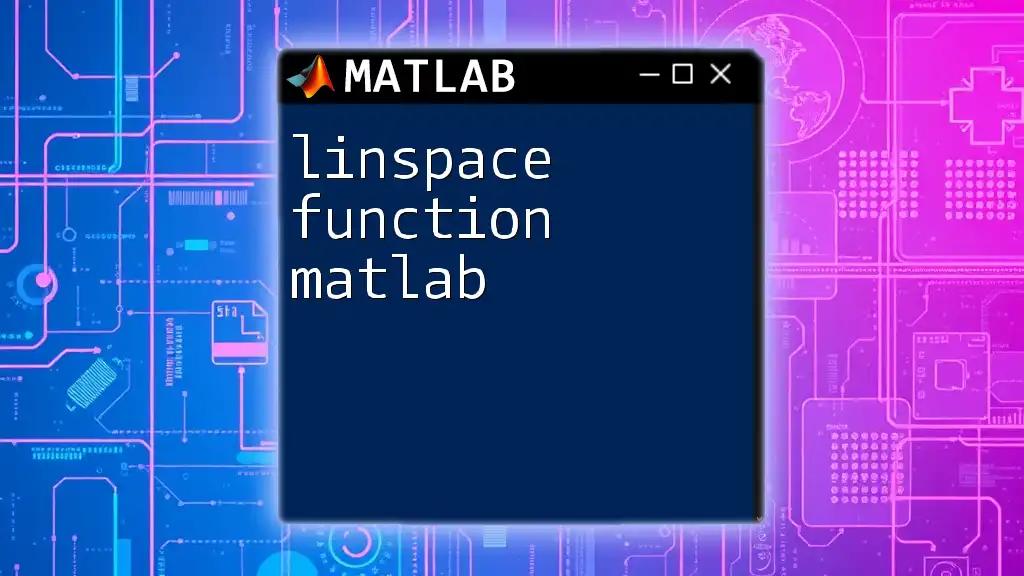
Conclusion
Grasping exponential functions is fundamental for various applications in MATLAB. From understanding basic definitions to creating custom functions and fitting models, your journey through exponential functions empowers you to tackle complex problems efficiently.
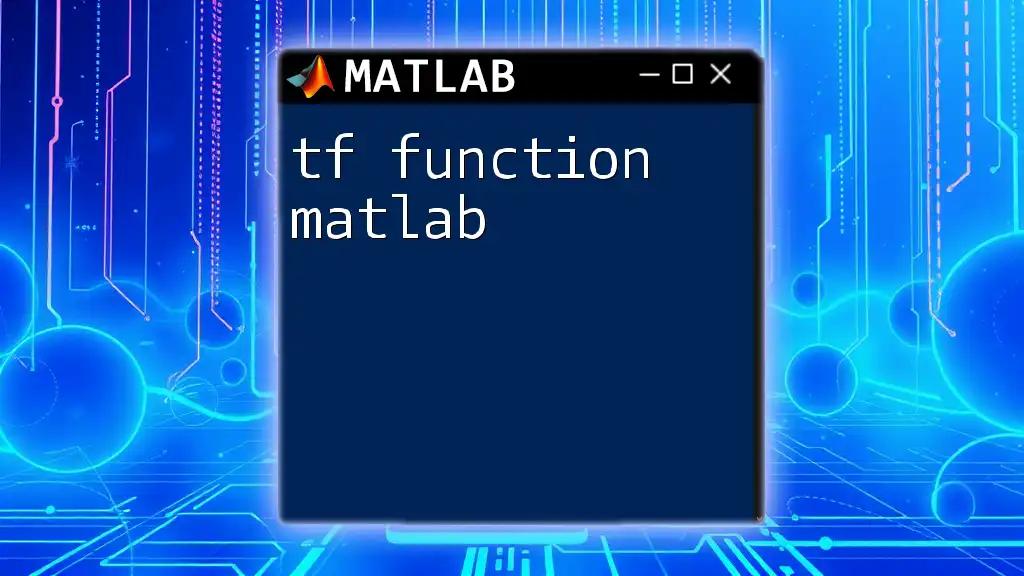
Further Learning Resources
To deepen your understanding of exponential functions and MATLAB, consider exploring additional resources such as books on MATLAB programming, engaging in online courses, or participating in forums dedicated to MATLAB discussions.

Join Our MATLAB Community
We encourage you to subscribe for more tutorials, insights, and hands-on examples related to exponential function in MATLAB. Share your experiences or questions in the comments—let's enhance our skills together!