The `trapz` function in MATLAB computes the numerical integral of a set of data points using the trapezoidal rule, providing an effective way to approximate the area under a curve.
Here's a code snippet that demonstrates how to use the `trapz` function:
% Example data points
x = 0:0.1:10;
y = sin(x);
% Calculate the integral using the trapezoidal rule
area = trapz(x, y);
disp(['Approximate area under the curve: ', num2str(area)]);
Understanding the `trapz` Function
What is `trapz`?
The `trapz` function in MATLAB is designed for numerical integration using the trapezoidal rule. This function is especially useful in scenarios where you have discrete data points and need to estimate the area under a curve formed by these points. By approximating the area with trapezoids rather than using analytical integration, `trapz` provides a flexible solution for various datasets.
Unike other methods, the `trapz` function allows for quick calculations, making it particularly advantageous in engineering, physics, and data analysis tasks.
Syntax of the `trapz` Function
The general syntax for using the `trapz` function is straightforward:
y = trapz(X, Y)
In this context:
- X represents the vector of x-coordinates (which is optional).
- Y is the vector or matrix containing the y-coordinates.
For instance, if you have a set of x-values and corresponding y-values, you can compute the integral as follows:
y = trapz(x, y_values)
How the `trapz` Function Works
The Trapezoidal Rule
At the core of the `trapz` function is the trapezoidal rule. This principle involves approximating the area under a curve by decomposing it into a series of trapezoids. The total area is then calculated by summing the areas of all trapezoids. Mathematically, this is expressed as:
\[ \text{Area} \approx \sum_{i=1}^{n-1} \frac{(y_{i} + y_{i+1})}{2} \cdot (x_{i+1} - x_{i}) \]
This method is particularly effective for providing decent approximations, especially when the function is linear between points.
Input Dimensions and Behavior
The `trapz` function can handle various input sizes and shapes. It can process one-dimensional vectors as well as multi-dimensional matrices. If you provide a matrix, `trapz` will integrate over the first dimension by default, treating each column as a separate y-coordinate set.
Consider the following examples:
% One-dimensional case
x = [1, 2, 3, 4];
y = [2, 3, 5, 7];
area = trapz(x, y);
In this example, the area under the curve defined by `x` and `y` is calculated successfully.
For multi-dimensional data:
% Two-dimensional example
Z = [1, 2; 3, 4];
area_2d = trapz(Z);
Here, `trapz` calculates the total area under the surface represented by the matrix `Z`.
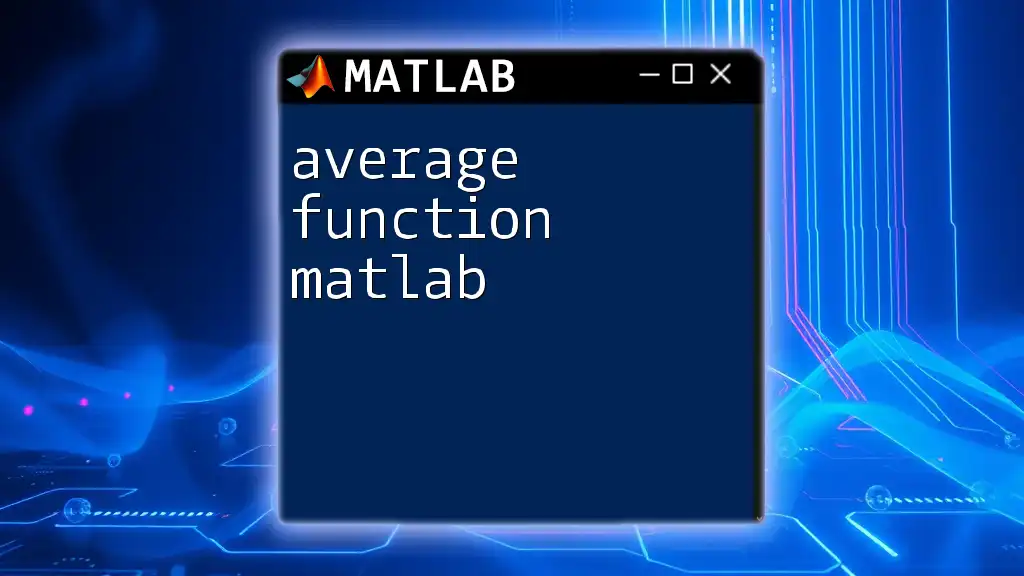
Practical Examples of Using `trapz`
Example 1: Simple Area Calculation
Let’s walk through calculating the area under the sine function using `trapz`. This example helps to understand how to apply the function in a practical scenario.
x = 0:0.1:10; % x values
y = sin(x); % y values (sin function)
area = trapz(x, y); % Calculate area under sin curve
fprintf('Area under the curve: %.2f\n', area);
In this script:
- `x` defines a range from 0 to 10.
- `y` computes the sine values for the given `x`.
- The `trapz` function then calculates the area under the sine curve, which is displayed to the user.
Example 2: Multi-dimensional Data
To illustrate working with two-dimensional data, consider a scenario where you have grid data from a function:
[X, Y] = meshgrid(1:5, 1:5);
Z = X.*Y; % Z = X*Y
area_2d = trapz(X, trapz(Y, Z, 2));
fprintf('Area under the surface: %.2f\n', area_2d);
In this example:
- `meshgrid` creates matrices for `X` and `Y` over the range 1 to 5.
- `Z` is calculated as the product of `X` and `Y`.
- The double application of `trapz` integrates first along `Y`, followed by `X`, providing the total area under the surface defined by `Z`.
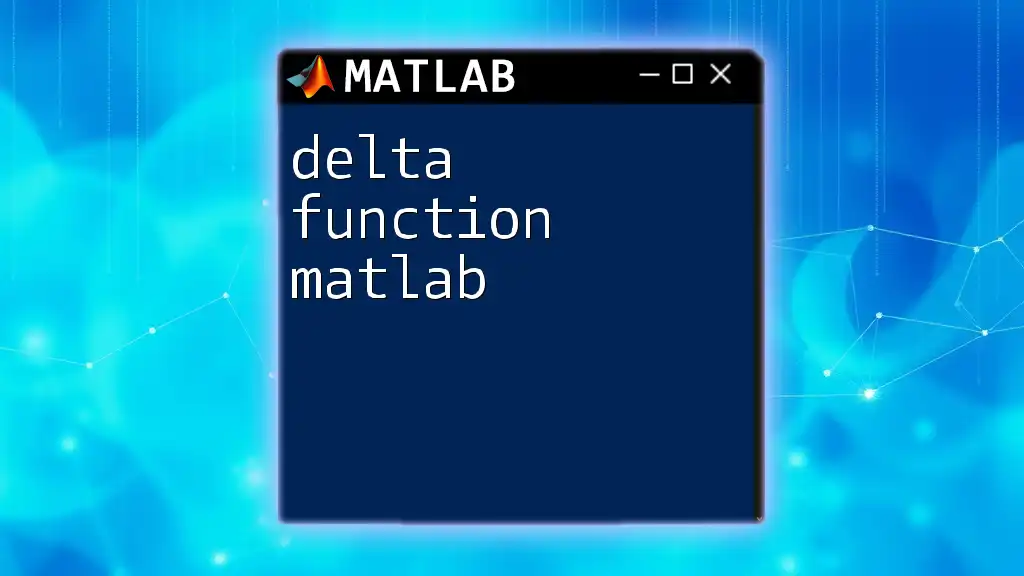
Common Errors and Troubleshooting
Input Size Mismatch
One of the most common errors encountered when using the `trapz` function comes from input size mismatches. Ensure that the dimensions of `X` and `Y` are compatible. If you attempt to integrate with mismatched input sizes, MATLAB will throw an error message indicating the dimensionality issue. Always verify the shapes of your matrices before applying the function.
Incorrect Data Type
The `trapz` function supports various data types including double, single, and logical. If you pass an unsupported data type, MATLAB will generate an error. To avoid this, ensure your data is in a format that `trapz` can handle effectively.
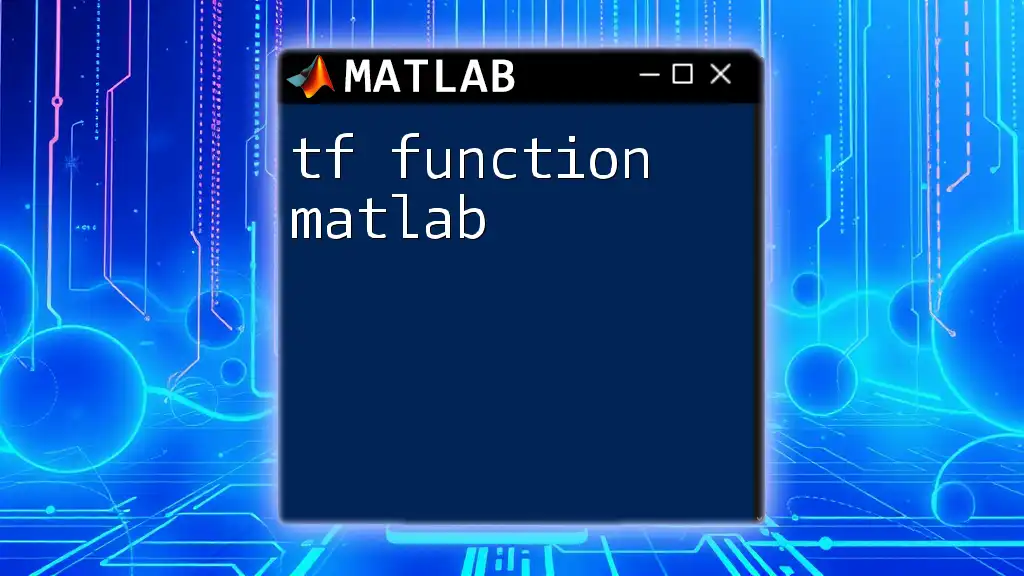
Best Practices for Using `trapz`
Choosing the Correct Input Vectors
When inputting data, the accuracy of the `X` and `Y` vectors is paramount. Ensure that the data accurately represents the function's behavior, and consider the need for uniform spacing in `X`. Non-uniform spacing can lead to inaccuracies in the area calculation.
When to Use `trapz` vs Other Functions
While `trapz` is a powerful tool for numerical integration, be mindful of when to use it. For example, for analytic functions where you can determine the integral algebraically, consider using `integral` or `quad` functions, which may offer more precision. In contrast, for discrete datasets or empirical data points, `trapz` is your go-to function.
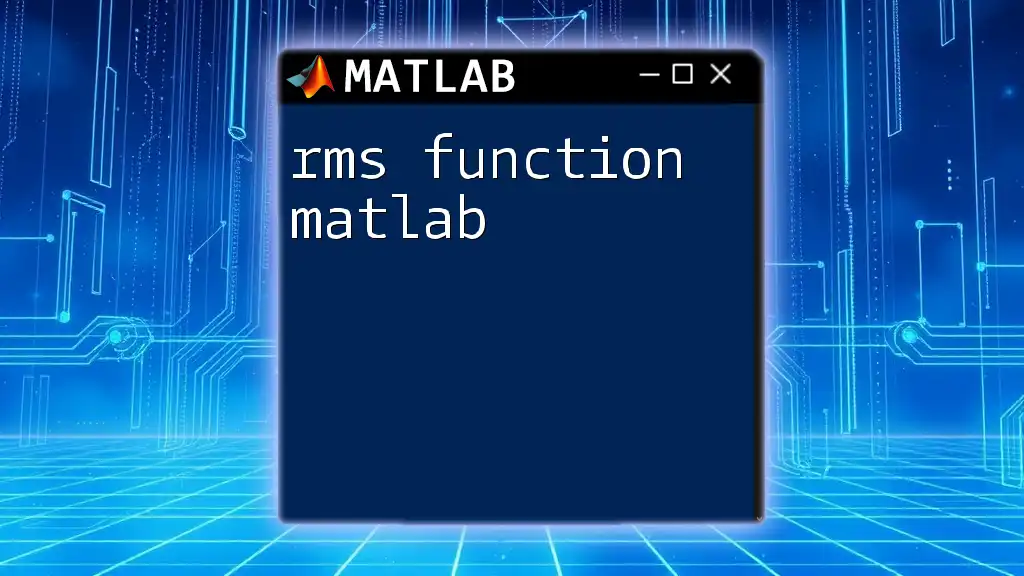
Conclusion
In summary, the `trapz function in MATLAB` is an indispensable tool for numerical integration, especially suitable for discrete data. With a fundamental understanding of its workings, syntax, and practical applications, you can leverage this function effectively in your work. Practice with the examples provided to cement your understanding, and remember to explore additional resources for a more in-depth mastery of MATLAB commands.
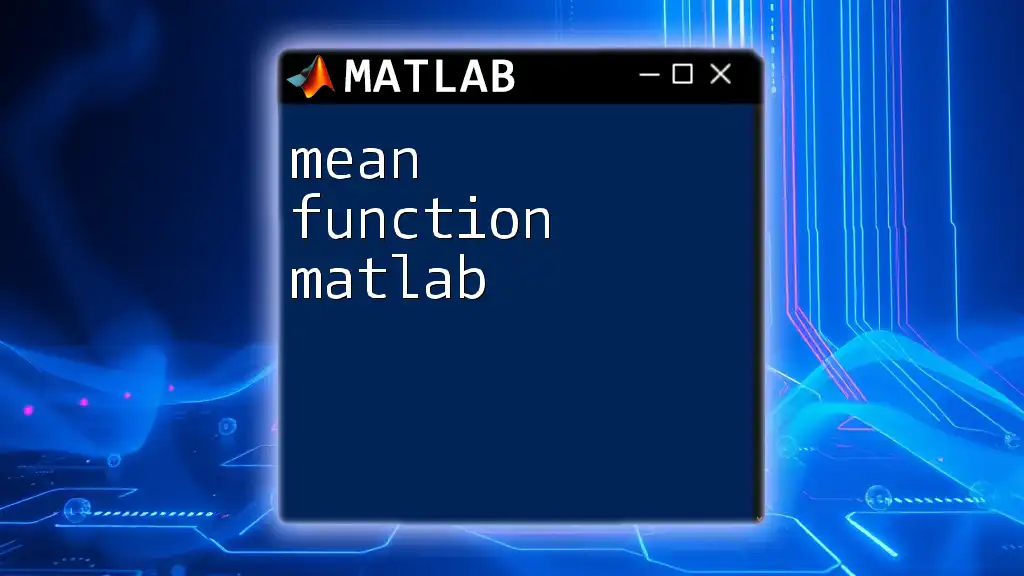
Additional Resources
For further learning, consider exploring the official MATLAB documentation specific to the `trapz` function, consulting recommended books, or participating in MATLAB user communities. These resources can provide invaluable insight and guidance as you refine your MATLAB skills.
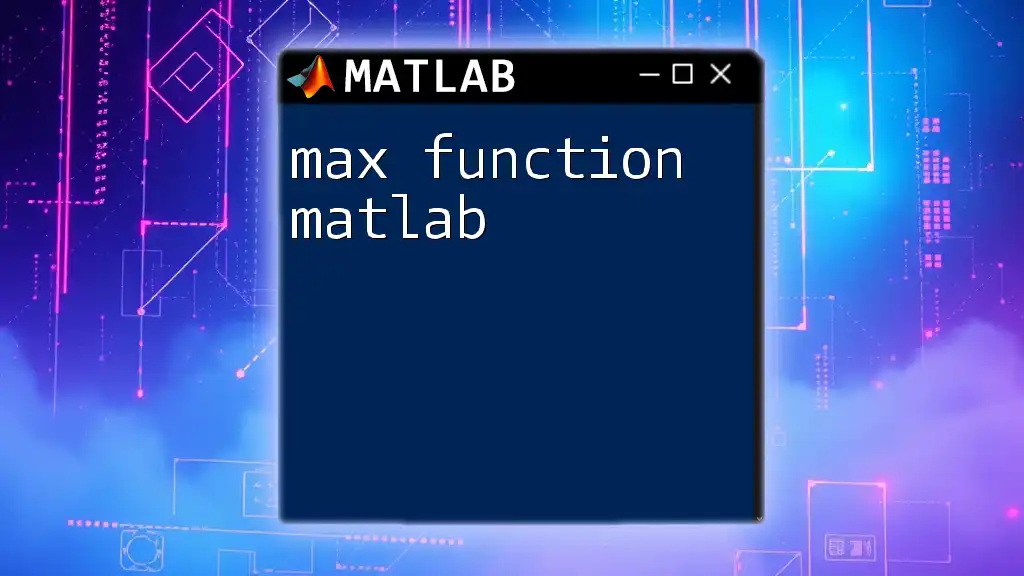
Call to Action
To enhance your proficiency with MATLAB commands and functions like `trapz`, sign up for more tutorials and expert tips that will accelerate your learning journey. Become adept at MATLAB in a quick, short, and concise way!