The average function in MATLAB is typically calculated using the `mean` function, which computes the average value of an array or matrix along a specified dimension.
Here’s a code snippet demonstrating how to use the `mean` function:
% Example of calculating the average of an array
data = [1, 2, 3, 4, 5];
average_value = mean(data);
disp(average_value);
Understanding the Concept of Average
What is Average?
An average is a central value that represents a set of numbers. It acts as a summary measure, illustrating the common or typical value among the data. The most common type of average is the mean, but there are other types such as the median and mode. Each type of average offers unique insights, and their applications can range from statistical analysis to daily decision-making.
Types of Averages in MATLAB
In MATLAB, you can compute several types of averages:
- Mean: The arithmetic average, calculated by dividing the sum of individual values by the number of values. Use it when you want a general idea of the dataset.
- Median: The middle value when the data is ordered. This is helpful when your dataset includes outliers that can skew the mean.
- Mode: The value that appears most frequently in the dataset. This is useful in categorical data analysis.
Understanding when to use each average can significantly affect your data analysis results.
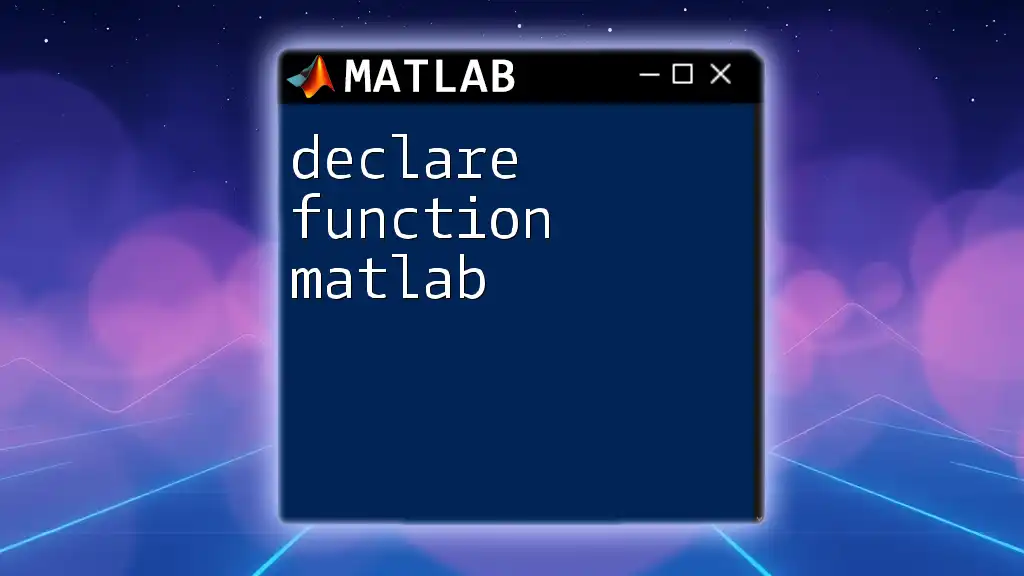
The `mean` Function in MATLAB
Syntax of the `mean` Function
The basic syntax for the `mean` function is as follows:
result = mean(A)
Here, `A` can be a vector or a matrix that contains the numerical data.
Parameters of the `mean` Function
The `mean` function allows an optional second argument that specifies the dimension along which the mean is computed:
result = mean(A, dim)
- If `dim` is `1`, it calculates the mean along the rows.
- If `dim` is `2`, it calculates the mean along the columns.
Example Usage of the `mean` Function
Single-Dimensional Arrays
To calculate the mean of a vector, you might write:
A = [1, 2, 3, 4, 5];
avg_value = mean(A);
The result, `avg_value`, equals `3`, which is the arithmetic average of the elements in the vector.
Two-Dimensional Arrays
When working with a matrix, you can calculate the mean across different dimensions:
B = [1, 2; 3, 4; 5, 6];
row_avg = mean(B, 1); % mean along rows
col_avg = mean(B, 2); % mean along columns
Here:
- `row_avg` yields `[3, 4]`, representing the average of each column.
- `col_avg` gives `[2; 4; 6]`, representing the mean of each row.
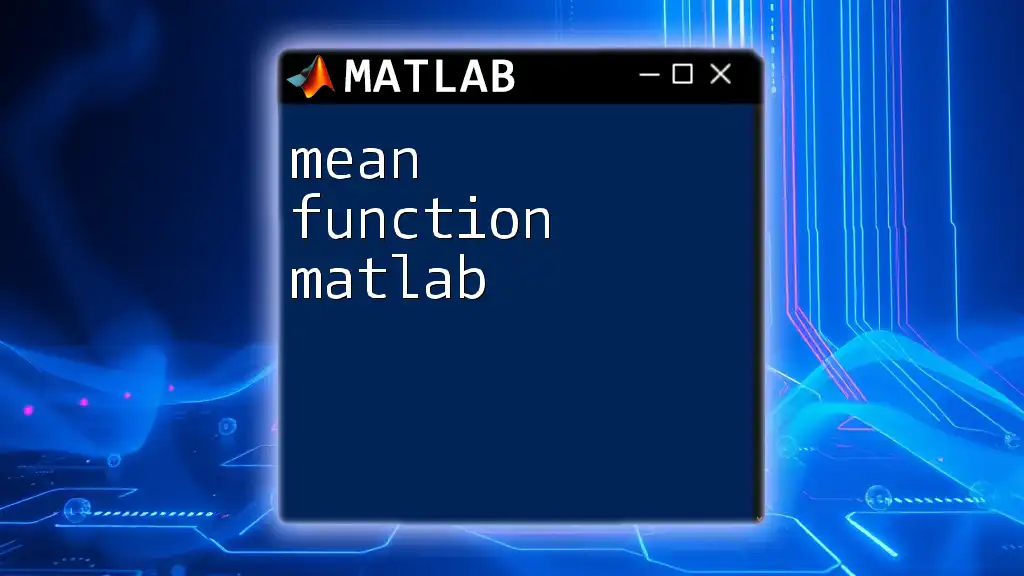
The `median` Function in MATLAB
Using the `median` Function
Similar to the `mean` function, the `median` function also has a straightforward syntax:
result = median(A)
Example Usage of the `median` Function
Single-Dimensional Arrays
To find the median of a given vector:
A = [5, 3, 1, 4, 2];
median_value = median(A);
In this case, `median_value` will return `3`, the middle value of the sorted array.
Two-Dimensional Arrays
When applied to a matrix, the `median` function can be used like this:
B = [5, 3, 1; 4, 2, 6];
median_row = median(B, 1);
median_column = median(B, 2);
For the calculations:
- `median_row` will return `[4, 2, 6]`, representing each column's median.
- `median_column` will return `[3; 4]`, representing each row's median.
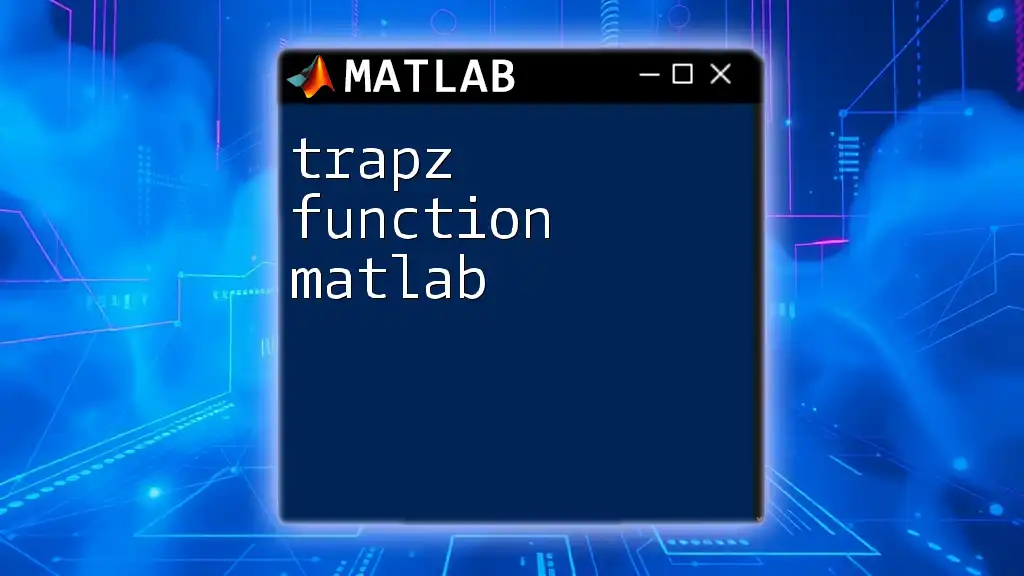
The `mode` Function in MATLAB
Understanding the `mode` Function
The `mode` function identifies the most frequently occurring value within a dataset. Its syntax is as follows:
result = mode(A)
Example Usage of the `mode` Function
Single-Dimensional Arrays
To calculate the mode of an array:
A = [1, 2, 2, 3, 4];
mode_value = mode(A);
In this instance, `mode_value` equals `2`, as it appears most frequently in the vector.
Two-Dimensional Arrays
You can also compute the mode for each dimension in a matrix:
B = [1, 2, 2; 3, 4, 4];
mode_row = mode(B, 1);
mode_column = mode(B, 2);
- `mode_row` results in `[2, 4]`, the most common values per column.
- `mode_column` yields `[2; 4]`, the most frequent values per row.
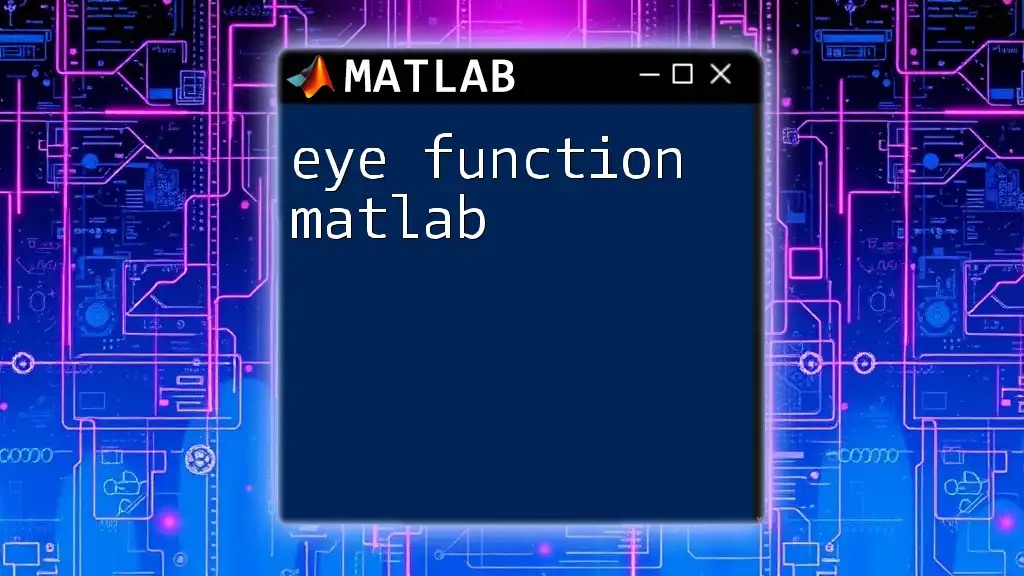
Combining Functions for Advanced Analysis
Calculating Averages in Real-World Scenarios
Being able to compute and compare the mean, median, and mode aids in the analysis of various data sets. For instance, in a financial analysis, you can leverage these functions to determine average sales, identify outliers in profit distribution, and ascertain the most common transaction amounts.
Code Snippet Example
Here’s how to compute all three averages together:
data = [10, 12, 10, 15, 20];
avg_mean = mean(data);
avg_median = median(data);
avg_mode = mode(data);
disp(['Mean: ', num2str(avg_mean), ', Median: ', num2str(avg_median), ', Mode: ', num2str(avg_mode)]);
Executing this snippet would give you a complete overview of the central tendencies within `data`, elucidating potential trends and patterns.
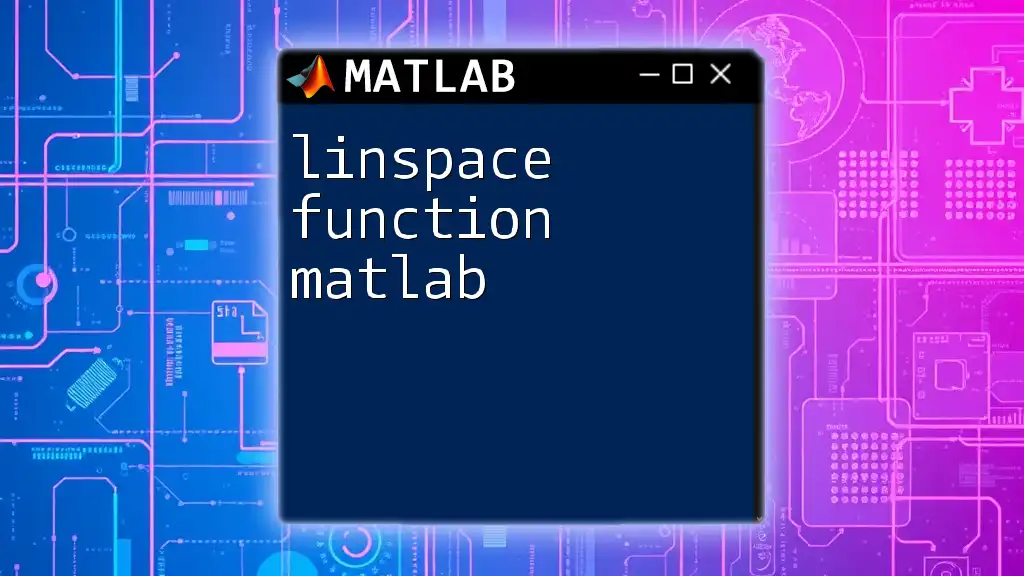
Conclusion
In summary, the average function in MATLAB is invaluable for data analysis, providing critical insights into numerical datasets. By mastering the `mean`, `median`, and `mode` functions, you can greatly enhance your analytical capabilities. Practice these functions to establish a foundational understanding of average calculations in MATLAB, paving the way for more complex data analysis tasks.
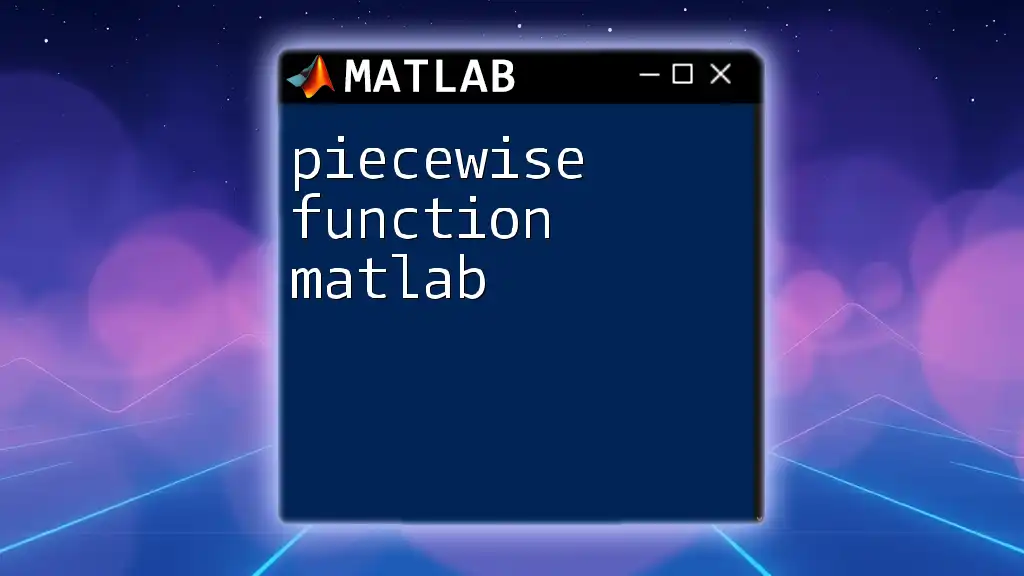
Additional Resources
Links to MATLAB Documentation
Refer to the official MATLAB documentation for deeper learning and additional examples regarding these average functions.
Suggested Exercises
- Compute the mean, median, and mode for datasets representing daily temperatures, sales figures, or test scores.
- Analyze the impact of outliers on mean values by including extreme values in your datasets.
FAQs
-
Can I apply the `mean`, `median`, and `mode` functions to non-numeric data? No, these functions are designed to work with numeric datasets.
-
What should I do when handling missing values in my data? MATLAB provides options for ignoring or filling missing values, which is essential for accurate average calculations. Consider utilizing the `mean(A,'omitnan')` option.
With these insights and examples, you’re well-equipped to leverage the average function in MATLAB effectively!