The `sort` function in MATLAB is used to arrange the elements of an array in ascending or descending order. Here's a simple example of its usage:
% Example of sorting a vector in ascending order
A = [3, 1, 4, 1, 5];
B = sort(A); % B will be [1, 1, 3, 4, 5]
Understanding the `sort` Function in MATLAB
What is the `sort` Function?
The `sort function` in MATLAB is a fundamental command used for arranging elements of arrays, matrices, and vectors in a specified order. Its primary purpose is to facilitate organized data manipulation, enabling users to easily analyze and visualize information. The syntax is straightforward:
B = sort(A)
Here, `A` represents the array or matrix to be sorted, and `B` is the output variable containing the sorted elements. The sort function can handle various data types, including numerical arrays, strings, and more complex structures.
How Does the `sort` Function Work?
MATLAB algorithmically sorts data using efficient sorting methods, allowing for quick operations even on larger datasets. By utilizing a combination of algorithms optimized for specific data types, MATLAB provides a robust solution for data sorting. Understanding how this function operates is critical for leveraging its capabilities effectively.
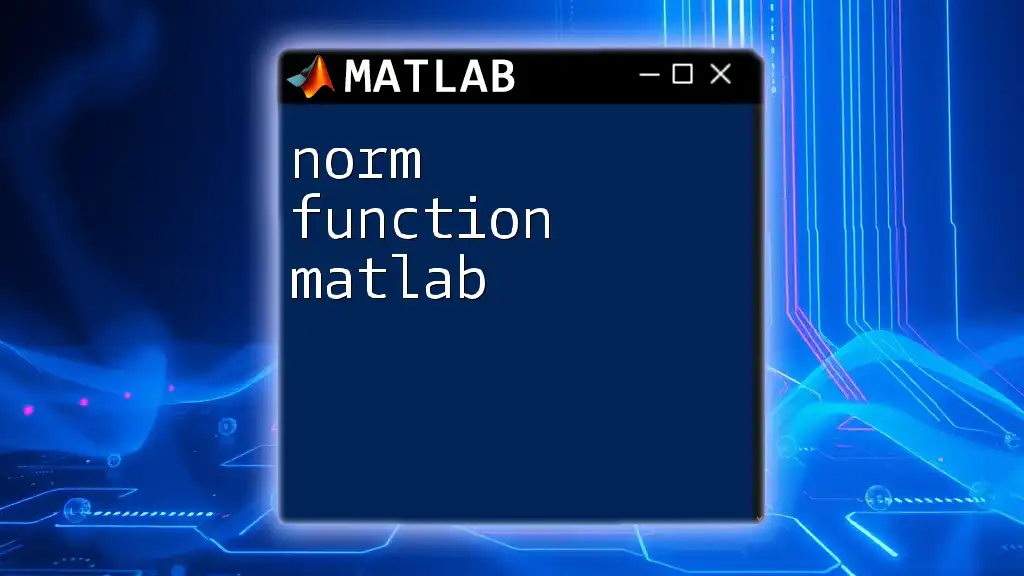
Basic Usage of the `sort` Function
Sorting a Vector
Sorting a numeric vector is one of the simplest applications of the `sort function`. For example, consider this unsorted vector:
A = [4, 2, 5, 1, 3];
B = sort(A);
Running this code produces the output:
B = [1, 2, 3, 4, 5]
This output is the sorted version of vector A in ascending order, showcasing how easily data can be organized.
Sorting a Matrix
Sorting Rows vs. Columns
The `sort` function's versatility extends to matrices, where users can sort data both by rows and columns. By default, MATLAB sorts each column of the matrix. For instance:
A = [4, 2;
1, 3;
6, 5];
B = sort(A); % sorts each column
The resulting matrix `B` will be:
B = [1, 2;
4, 3;
6, 5]
To sort a matrix by rows, simply add an additional argument indicating the dimension:
B = sort(A, 2); % sorts each row
This operation produces:
B = [4, 2;
1, 3;
6, 5]
This flexibility allows users to manipulate data according to their specific needs.
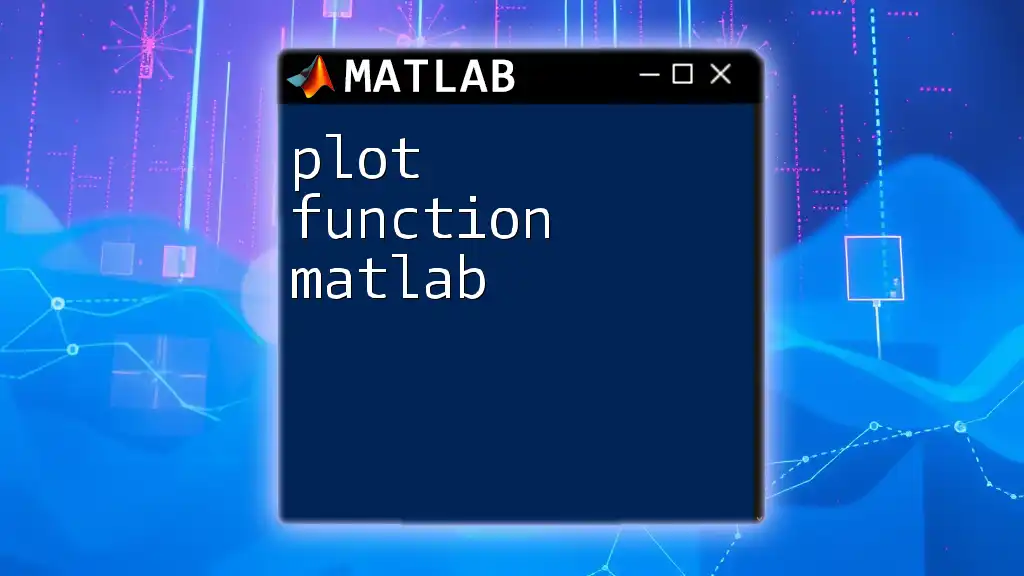
Advanced Features of `sort`
Specifying Sort Order
The default behavior of the `sort function` is to arrange data in ascending order. However, users can easily change this to descending order by specifying:
B = sort(A, 'descend');
This command will output the sorted array from highest to lowest, making it easy to tailor the function to various contexts where ranked data is needed.
Sorting with Indices
Another powerful feature of the `sort function` is the ability to retrieve the indices of the sorted elements alongside the sorted data itself. This can be useful when needing to reference original data points. For instance:
[B, I] = sort(A);
In this case, `B` contains the sorted values while `I` provides the indices of the elements in their original array `A`. This is particularly useful in data analysis scenarios where maintaining a reference to the original data is vital.
Sorting Complex Data Types
The `sort function` can also tackle more complex data structures, such as structs and cell arrays. For example, when sorting a structured dataset:
S.name = {'John'; 'Yvonne'; 'Alice'};
S.score = [23; 30; 21];
[~, sortedIndices] = sort([S.score]);
sortedData = S(sortedIndices);
Here, the scores are sorted, and the corresponding names rearranged accordingly, demonstrating the flexibility and power of sorting functions in MATLAB.
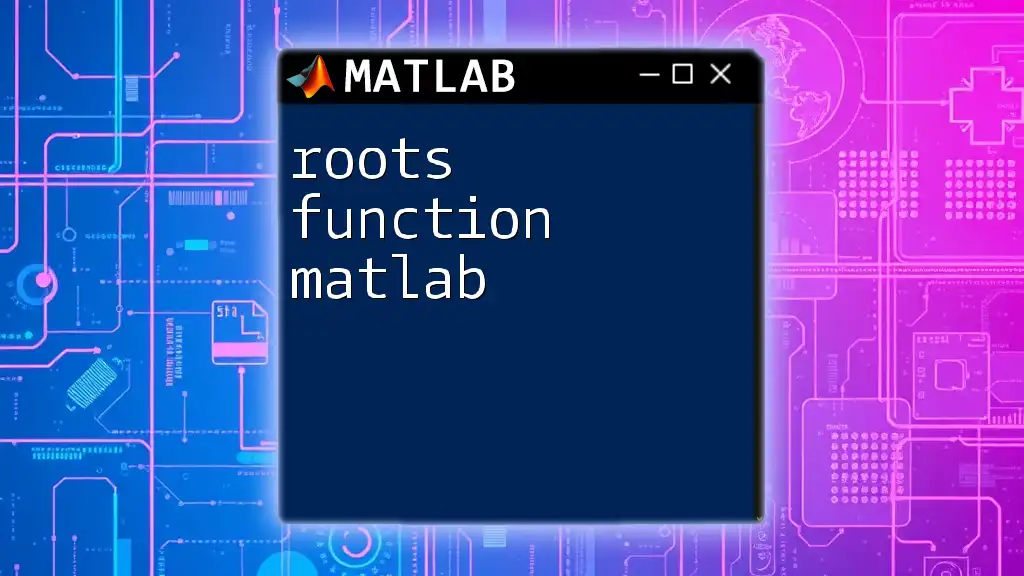
Practical Applications of the `sort` Function
Data Analysis & Visualization
Sorting is a crucial step in data analysis, often serving as a precursor to visualization. For instance, before creating plots or charts, data typically needs to be structured in a specific order. The `sort function` not only enables this but also improves interpretability and insights derived from visual representations.
Use in Algorithms
Beyond simple data manipulation, sorting is foundational in many algorithms, such as binary search. Before performing such searches, sorted data is essential, illustrating how the `sort function` lays groundwork for more complex operations.
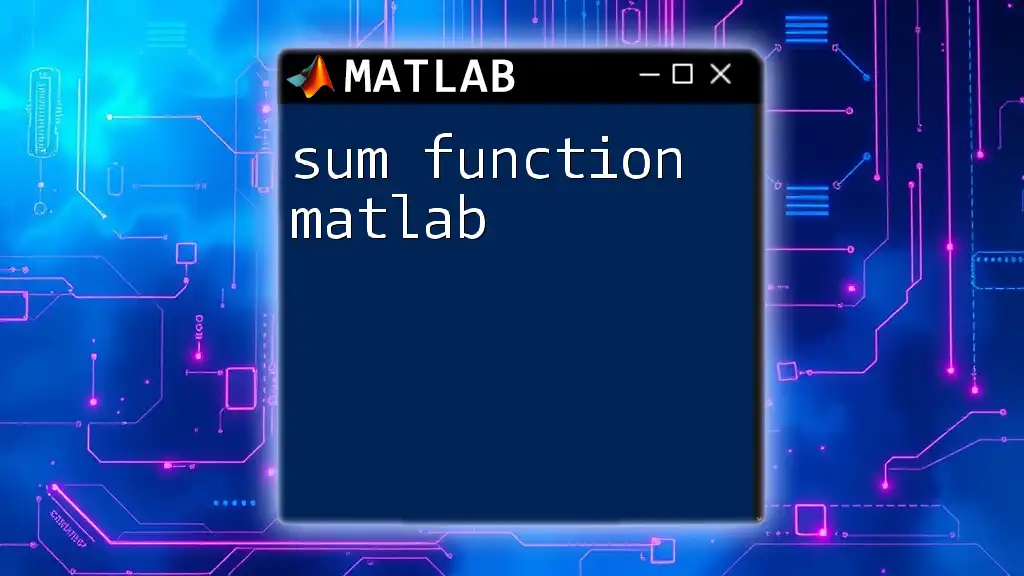
Common Pitfalls and Errors
Performance Issues
While the `sort function` excels in efficiency, users should be aware of performance implications when handling large datasets. For extensive arrays, consider exploring MATLAB's built-in parallel computing options or utilizing alternative sorting algorithms when appropriate.
Understanding Output Dimensions
One common source of confusion can arise from the dimensions of output arrays, particularly when sorting multi-dimensional data. Always ensure awareness of the output's dimensions—whether sorting by rows or columns—to avoid unexpected results.
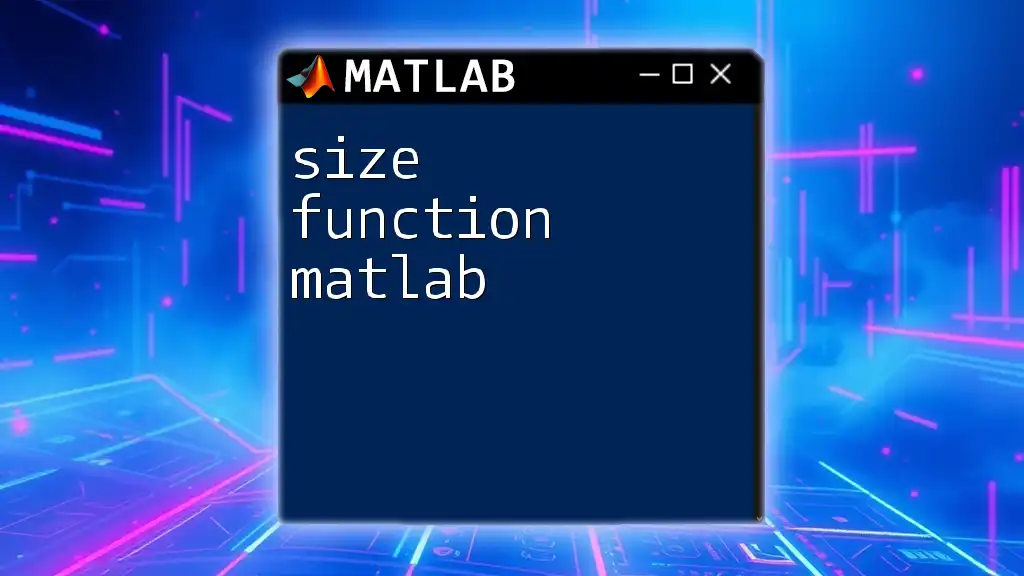
Conclusion
In summary, the `sort function` in MATLAB is an indispensable tool for anyone working with data. Its ability to efficiently organize arrays and matrices aids analysis and visualization, making it essential for effective data manipulation. Practicing the use of `sort` across different datasets will enhance your MATLAB skills and empower you to perform advanced data operations confidently.
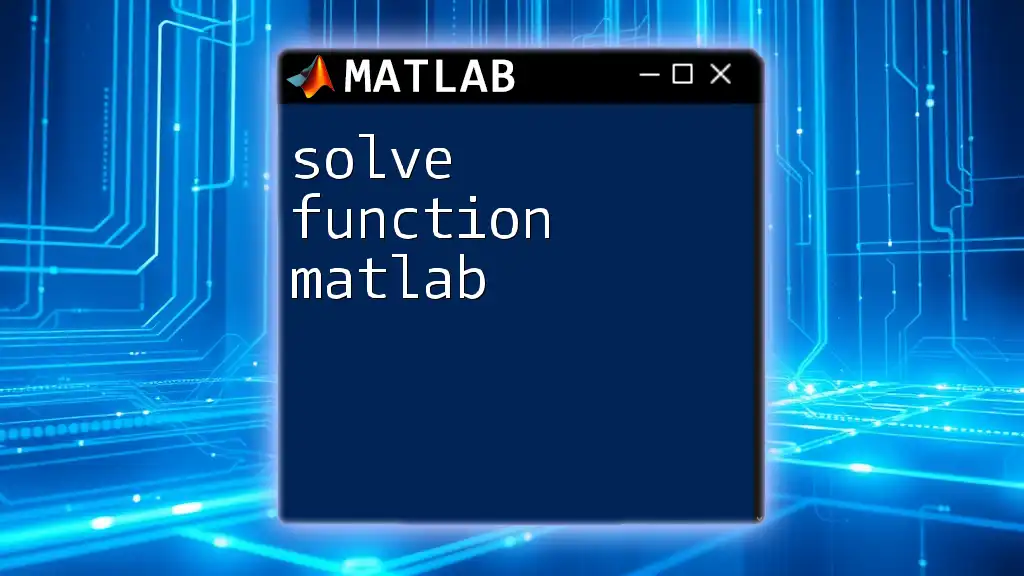
Additional Resources
To further expand your knowledge, consider exploring the [MATLAB official documentation](https://www.mathworks.com/help/matlab/ref/sort.html) for in-depth explanations and examples. Online courses and tutorials tailored for MATLAB users will also provide valuable insights into mastering this powerful function. Engaging with community forums can also be beneficial, allowing for shared learning experiences with fellow MATLAB enthusiasts.