In MATLAB, you declare a function using the `function` keyword followed by the output variables, the function name, and the input variables in parentheses. Here's an example:
function output = myFunction(input)
output = input^2;
end
Understanding Functions in MATLAB
What is a Function?
A function in MATLAB is a block of code that takes inputs, performs tasks, and returns outputs. Unlike scripts, which execute a sequence of commands, functions allow for a more structured programming approach. They encapsulate functionality, enabling better organization and reuse of code.
Importance of Functions
Functions play a critical role in programming for several reasons:
- Reusability: Once defined, functions can be called multiple times throughout your code without rewriting the logic.
- Modularity: Functions enable you to organize code into manageable sections, making it easier to maintain and understand.
- Clarity: By using descriptive function names and clear input/output parameters, the purpose of your code becomes immediately clear to anyone reading it.
Consider a scenario where you need to calculate the area and perimeter of various shapes. Instead of writing the calculations each time, a function can compute these values given the shape's dimensions.
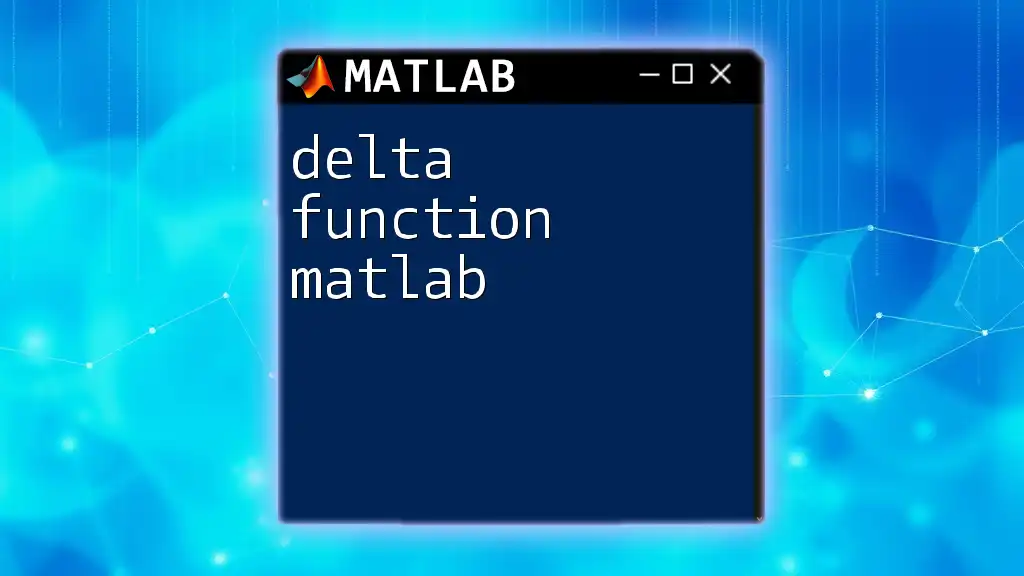
Basic Syntax for Declaring Functions
Function Declaration
To declare a function in MATLAB, you use the following basic syntax:
function [output1, output2] = functionName(input1, input2)
Components of a Function Declaration
- Function keyword: This indicates that you are defining a function.
- Output variables: These represent the values your function will return. You can return multiple values as a comma-separated list.
- Function name: The function must have a valid name. It should be unique and follow MATLAB's naming rules (e.g., no spaces, must start with a letter).
- Input parameters: These are the values your function will accept to perform operations. They allow the function to work with different data.
Example of a Simple Function
Here is an example of a simple MATLAB function that calculates the area and perimeter of a rectangle:
function [area, perimeter] = rectangleProperties(length, width)
area = length * width;
perimeter = 2 * (length + width);
end
In this function:
- `area` and `perimeter` are the output variables.
- `length` and `width` are the input parameters.
- When called, the function will return the area and perimeter based on the provided dimensions.
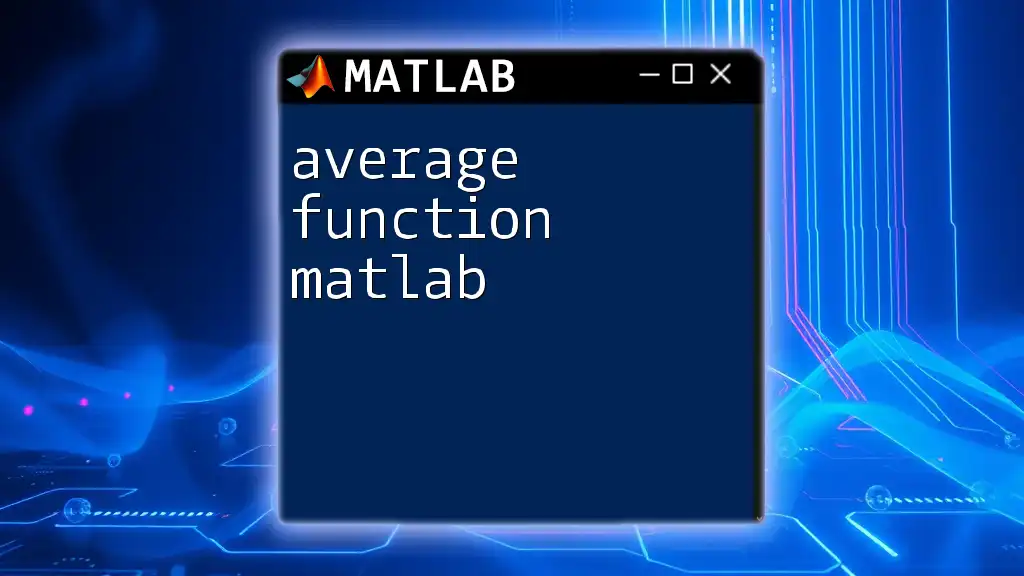
Types of Functions in MATLAB
Built-in Functions
MATLAB comes equipped with a plethora of built-in functions designed for various tasks, ranging from mathematical operations to data handling. For example, `sqrt()` calculates the square root, while the `mean()` function computes the average of an array. Familiarizing yourself with these can significantly enhance your coding efficiency.
User-Defined Functions
Purpose of User-Defined Functions
User-defined functions enable you to encapsulate specific functionality that may not exist in built-in functions. By creating your own functions, you tailor solutions to your specific needs, making code more versatile.
Differences Between Functions and Nested Functions
There are distinctions between regular functions and nested functions. Nested functions are defined within the body of another function and can access the parent function's workspace. This is useful for keeping variables local to the parent function while still utilizing them in complex calculations.
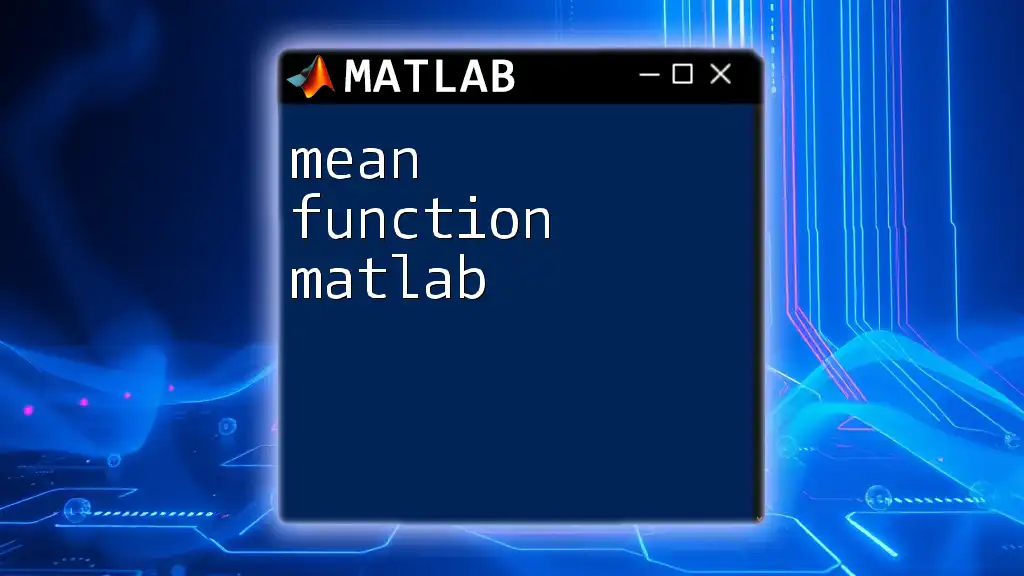
Advanced Function Declarations
Optional Input and Output Arguments
To declare functions that handle optional input arguments, you can check the number of input arguments using `nargin`. This allows your functions to have default behaviors. Consider the following example:
function output = exampleFunction(a, b, c)
if nargin < 3
c = 1; % Default value if c is not provided
end
output = a + b * c;
end
In this function, if the user does not provide the third argument `c`, it will default to `1`.
Variable-Length Input Arguments
Sometimes, you may need to create functions that accept a variable number of input arguments. MATLAB allows this through the use of `varargin`, which collects additional input arguments into a cell array. Here’s an illustration:
function result = sumManyNumbers(varargin)
result = sum(cell2mat(varargin));
end
This function takes any number of numerical arguments, converts them to a matrix, and calculates their sum, showcasing the flexibility of MATLAB functions.
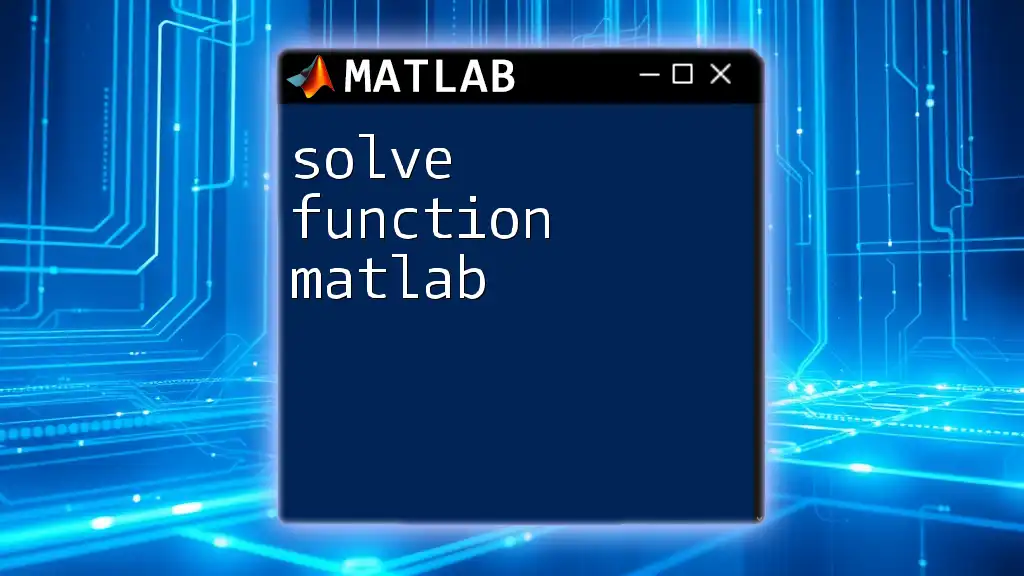
Function File Management
Creating and Saving Function Files
To use your defined functions, create a new file with the `.m` extension in your working directory. The filename must match the function name. For instance, for the function `rectangleProperties`, the file should be named `rectangleProperties.m`.
Best Practices for Naming Function Files
Choosing meaningful names for your function files enhances code readability. Your function name should reflect its purpose clearly; for example, a function that sorts an array could be named `sortArray`.
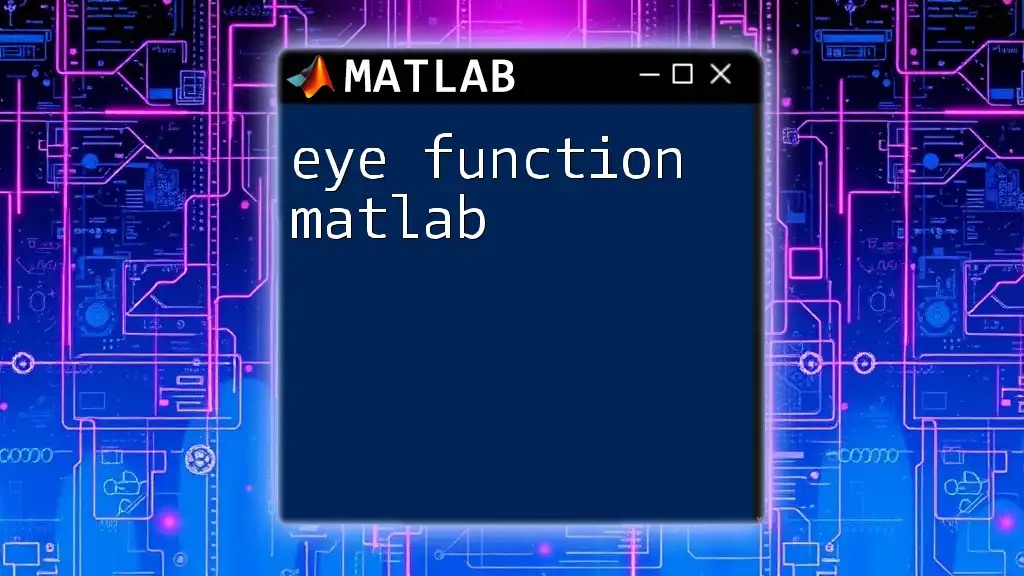
Conclusion
Understanding how to declare functions in MATLAB is essential for programming effectively within this environment. Functions enhance code clarity, reusability, and modularity, making it easier to develop complex applications. By mastering both basic and advanced function declarations, you can tailor your MATLAB experience to suit your needs.
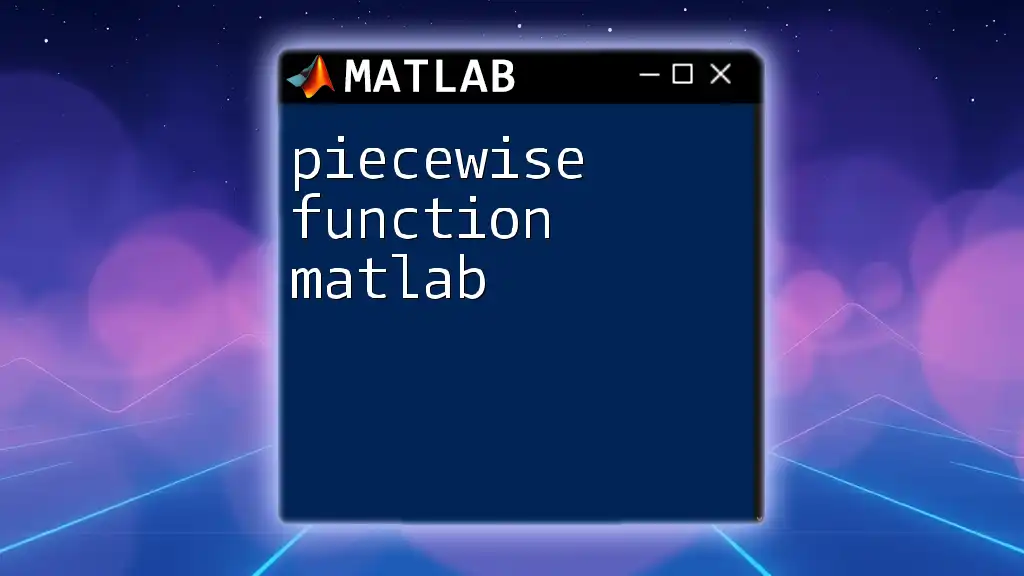
Additional Resources
Recommended Books and Online Tutorials
For those eager to dive deeper into MATLAB, explore resources such as "MATLAB: A Practical Introduction to Programming and Problem Solving" by Stormy Attaway or online platforms like MathWorks' documentation and tutorials.
Community and Support
Engaging with the MATLAB community can provide invaluable support. Platforms such as MATLAB Central and various user forums help troubleshoot issues and enhance your coding skills.