The `roots` function in MATLAB computes the roots of a polynomial given its coefficients in a vector, allowing users to easily find the values of x that make the polynomial equal to zero.
Here’s a code snippet to illustrate its usage:
% Define the coefficients of the polynomial: x^3 - 6x^2 + 11x - 6
coefficients = [1 -6 11 -6];
% Calculate the roots
r = roots(coefficients);
disp(r);
Understanding Polynomials
Definition of a Polynomial
A polynomial is an expression formed by the sum of terms, each consisting of a variable raised to a non-negative integer power multiplied by a coefficient. The general form of a polynomial can be expressed as:
\[ p(x) = a_n x^n + a_{n-1} x^{n-1} + \ldots + a_1 x + a_0 \]
where:
- \( p(x) \) is the polynomial function.
- \( n \) is the degree of the polynomial, which indicates the highest power of the variable \( x \).
- \( a_n, a_{n-1}, \ldots, a_1, a_0 \) are the coefficients.
Examples of Polynomials
To solidify your understanding, let's review a few examples of different types of polynomials:
-
Linear Polynomial: Example: \( p(x) = 3x + 2 \)
-
Quadratic Polynomial: Example: \( p(x) = 2x^2 + 3x + 1 \)
-
Cubic Polynomial: Example: \( p(x) = x^3 - 4x^2 + 2x - 5 \)
These examples illustrate how polynomials can vary in complexity depending on their degree and coefficients.
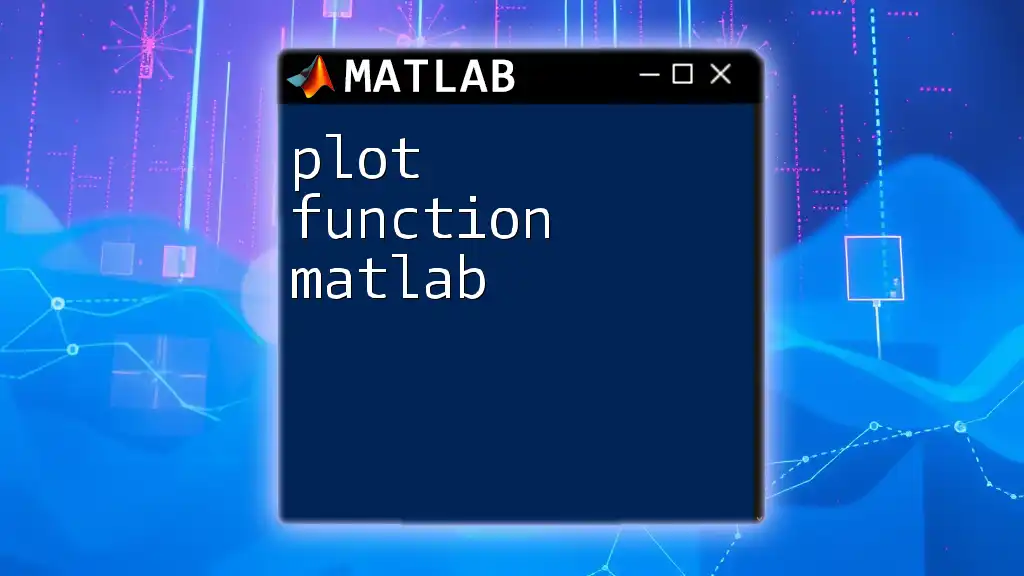
How the Roots Function Works
Syntax of the Roots Function
In MATLAB, the roots function is used to determine the roots of a polynomial given its coefficients. The basic syntax for this function is:
roots(p)
Where `p` is a vector containing the coefficients of the polynomial, starting with the highest degree.
Input Format for the Roots Function
To use the `roots` function correctly, you must input the polynomial in the appropriate format. For a polynomial expressed as
\[ p(x) = ax^2 + bx + c \]
You would input the coefficients as:
p = [a, b, c];
For example, if you have a polynomial \( p(x) = 2x^2 + 3x + 1 \), you can define it in MATLAB like this:
p = [2, 3, 1];
Output of the Roots Function
The output from the `roots` function represents the values of \( x \) for which \( p(x) = 0 \). These values can be real or complex numbers, depending on the characteristics of the polynomial.
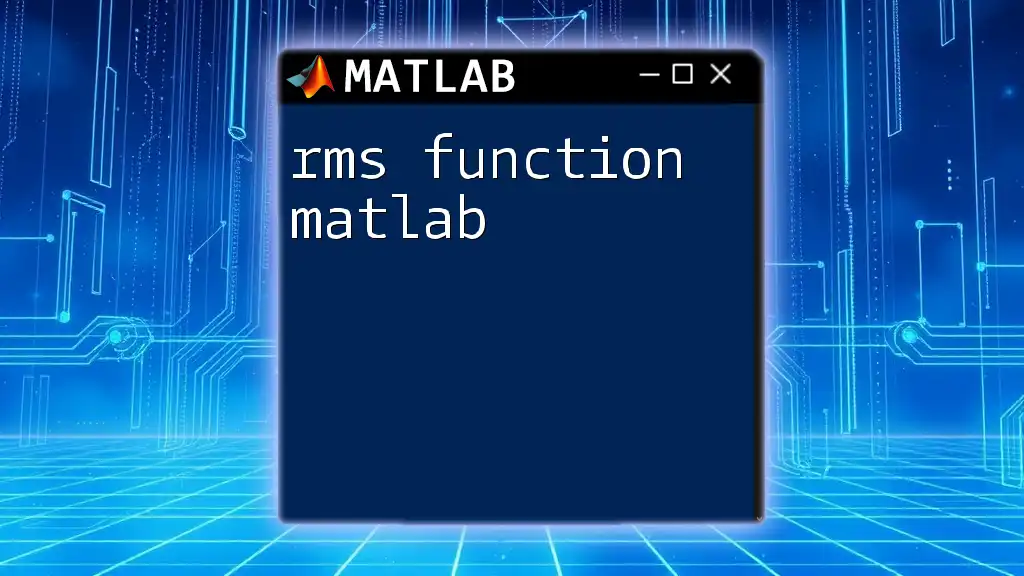
Practical Applications of the Roots Function
Real-World Applications
The `roots function matlab` plays a vital role in fields like engineering, physics, and finance, where polynomial equations frequently arise. Applications include:
- Structural Engineering: Analyzing stress and strain equations.
- Control Systems: Designing systems with specified stability criteria.
- Finance: Understanding the behavior of certain financial instruments, such as options.
Example Problems
To illustrate the application, let’s look at some concrete examples:
Example 1: Finding the Roots of a Quadratic Equation
Consider the quadratic equation \( 2x^2 + 3x + 1 = 0 \). You can find the roots using the following MATLAB code snippet:
p = [2, 3, 1]; % coefficients for 2x^2 + 3x + 1
rootValues = roots(p);
Running this code will output the roots, which are the values of \( x \) that satisfy the equation.
Example 2: Finding Roots of a Cubic Polynomial
Now, let’s analyze a cubic polynomial, say \( x^3 - 4x^2 + 2x - 5 = 0 \). You can compute the roots accordingly:
p = [1, -4, 2, -5]; % coefficients for x^3 - 4x^2 + 2x - 5
rootValues = roots(p);
Again, this will yield the corresponding roots for the polynomial in question.
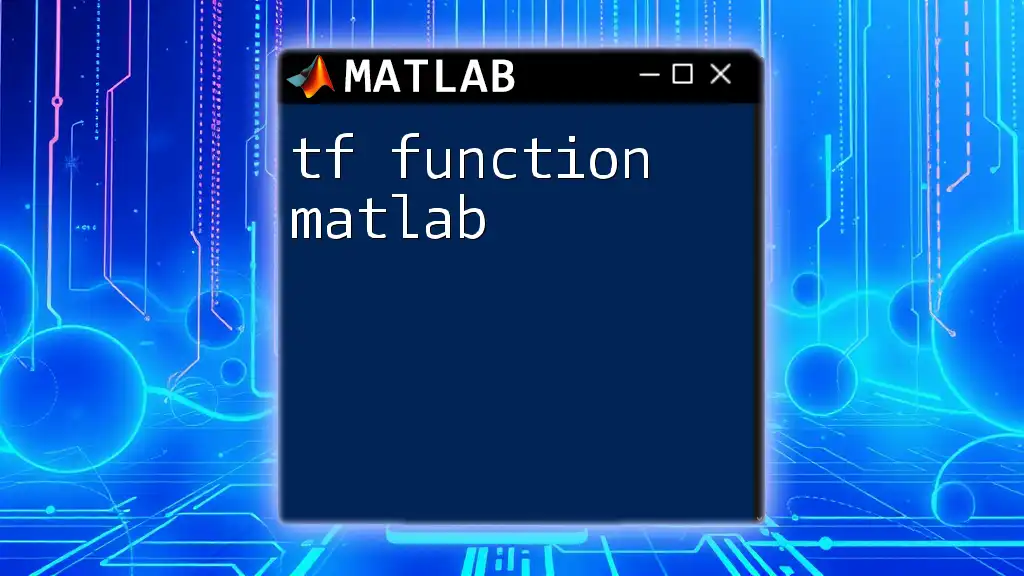
Detailed Example: Step-by-Step Guide
Step 1: Define the Polynomial
Let's define a polynomial as an example: \( 3x^3 - 2x^2 + 5x - 1 \). In MATLAB, we can set it up like this:
p = [3, -2, 5, -1]; % coefficients for 3x^3 - 2x^2 + 5x - 1
Step 2: Call the Roots Function
Next, we can call the `roots` function to compute the roots of this polynomial:
rootValues = roots(p);
Step 3: Interpreting the Results
Upon executing the `roots` command, MATLAB will return an array of values, which are the roots of the polynomial. You might receive both real and complex roots, which you can interpret based on the context of the problem you are solving. If, for instance, you see complex numbers, this indicates that the polynomial does not intersect the x-axis for those values.
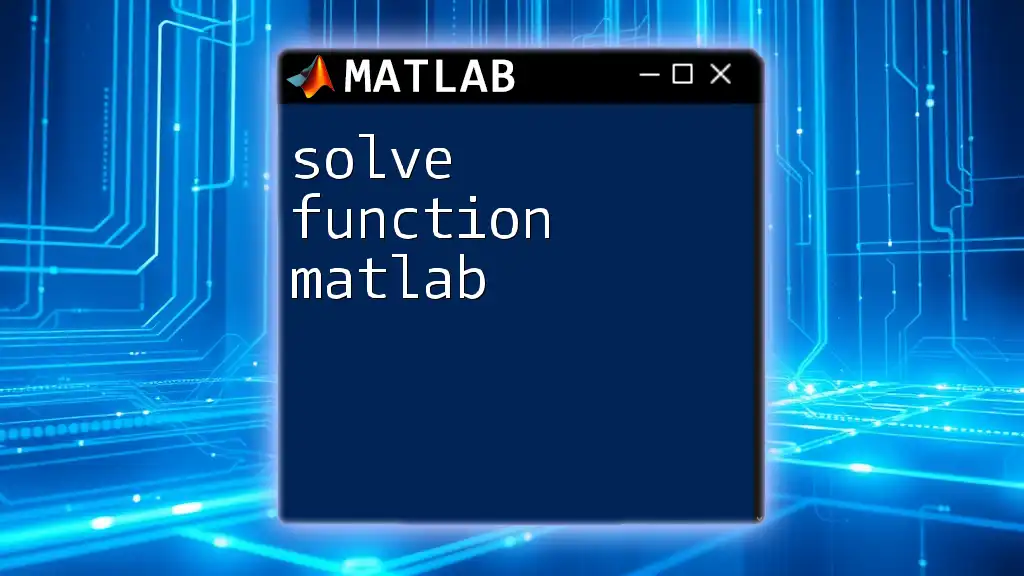
Troubleshooting Common Issues
Understanding Complex Roots
When dealing with the `roots function matlab`, you might encounter complex roots. These occur when the polynomial has no real solutions. For example, a quadratic equation with a negative discriminant will yield complex roots, indicating that the function does not cross the x-axis. Always remember that:
- Complex roots appear in conjugate pairs for polynomials with real coefficients.
- Complex results signify that the polynomial has behavior that may not be visible on a standard graph.
Common Errors and How to Fix Them
Errors frequently arise from incorrectly formatted input. Always ensure that your coefficient vector starts with the highest degree coefficient and follows in descending order. An example of a common mistake would be trying to input:
p = [2, 3]; % Missing constant term for a quadratic polynomial
This should be explicitly defined, or the `roots` function will return misleading or erroneous results.
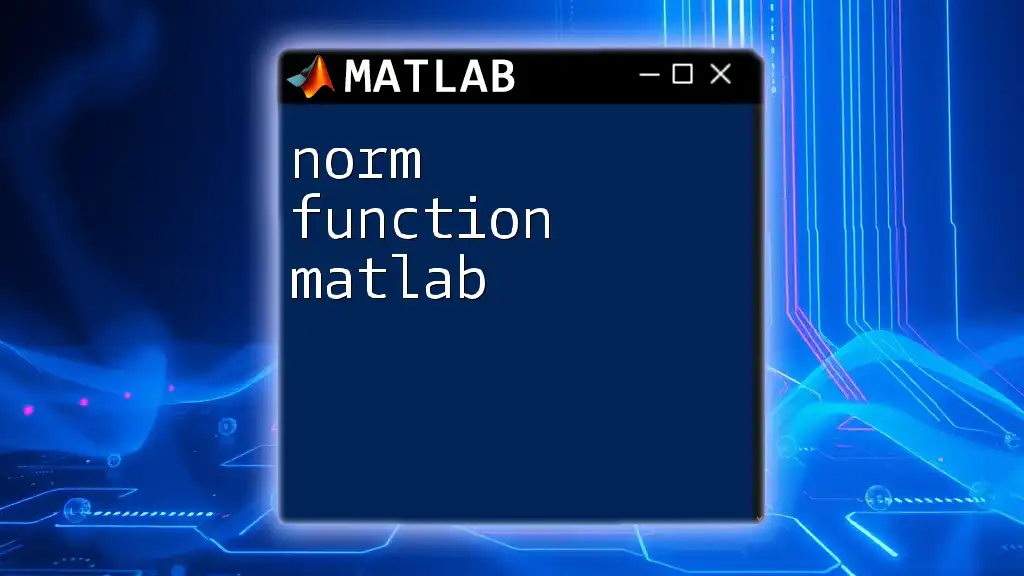
Conclusion
The `roots function matlab` is a powerful tool for solving polynomial equations with applications that span many fields. By understanding how to correctly define polynomials, utilize the `roots` function, and interpret the results, you will be well-equipped to tackle a variety of mathematical problems effectively. Embrace this functionality, and don't hesitate to experiment with different polynomials to deepen your understanding.
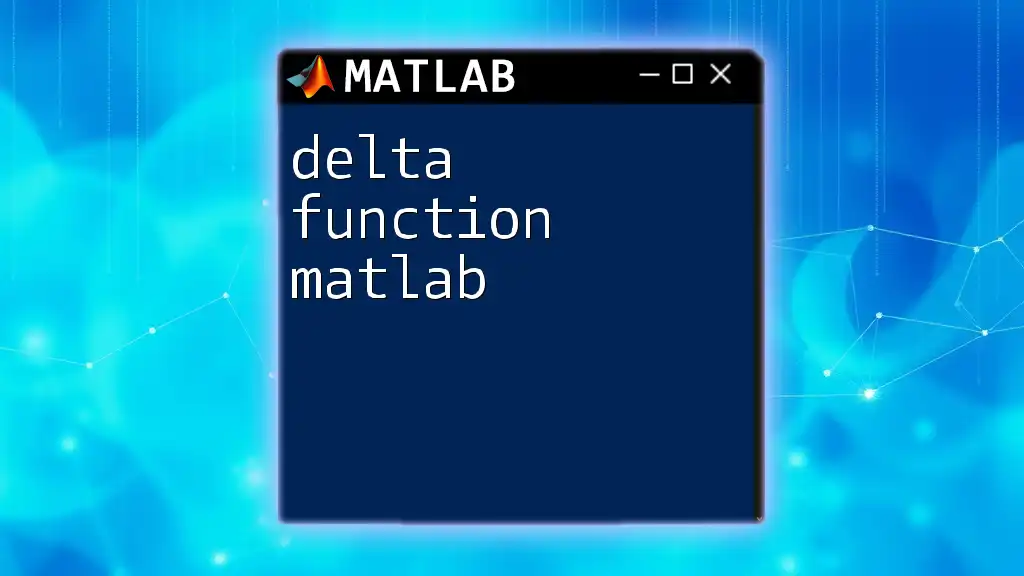
Additional Resources
Further Reading
For those looking to deepen their understanding, the MATLAB documentation offers extensive resources that cover not just the `roots` function but other polynomial-related functions as well.
Online Communities
Joining MATLAB user forums and communities such as MATLAB Central is a fantastic way to explore further, find solutions, and connect with like-minded individuals who share an interest in MATLAB programming.