A piecewise function in MATLAB allows you to define a function that takes on different expressions based on the input value, enabling you to handle multiple cases efficiently.
Here’s an example code snippet demonstrating how to define a piecewise function in MATLAB:
f = @(x) (x<0) .* (x.^2) + (x>=0 & x<1) .* (x + 1) + (x>=1) .* (2*x);
Understanding Piecewise Functions
Definition and Mathematical Representation
A piecewise function is defined as a function that has different expressions based on the input value. This type of function is particularly useful when modeling scenarios where a relationship changes based on certain conditions. The general mathematical representation of a piecewise function can be denoted as:
\[ f(x) = \begin{cases} f_1(x) & \text{if } x < a \\ f_2(x) & \text{if } a \leq x < b \\ f_3(x) & \text{if } x \geq b \end{cases} \]
Properties of Piecewise Functions
Piecewise functions can exhibit various properties:
- Continuity: A piecewise function can be continuous or discontinuous depending on how the pieces are defined at the boundaries.
- Differentiability: Differentiability can also vary. A function that’s continuous may not necessarily be differentiable at the transition points.
- Graphical Representation: Graphing piecewise functions often reveals distinct behaviors in different segments, showcasing their utility in visual analysis.
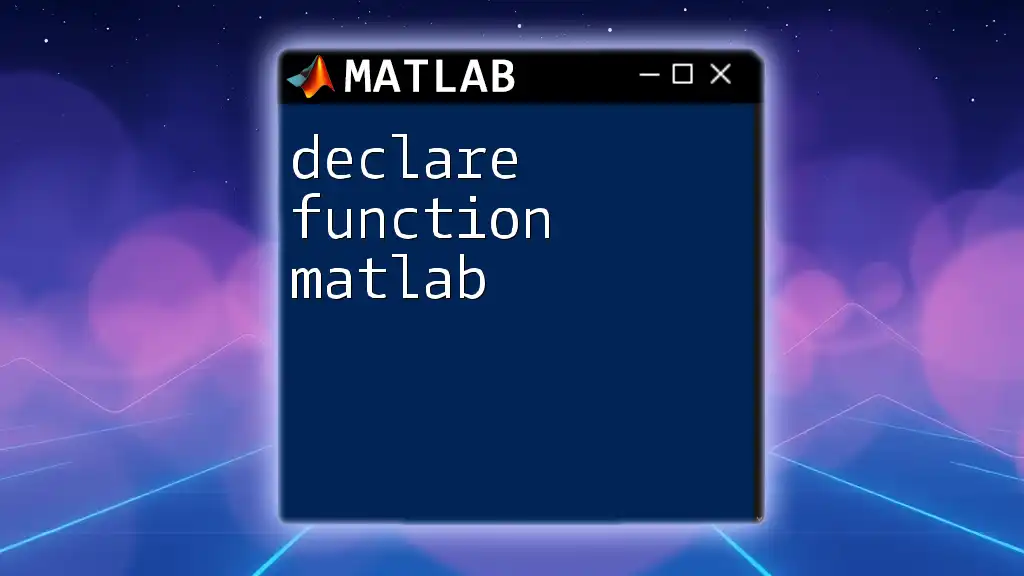
Implementing Piecewise Functions in MATLAB
Syntax and Basic Structure
In MATLAB, the `piecewise` function is a straightforward way to define and work with piecewise functions. The syntax is simple, and its parameters allow for easy configuration of conditions and corresponding outputs.
Creating Piecewise Functions
Example 1: Simple Piecewise Function
To illustrate, consider a simple piecewise function defined as follows:
- \( f(x) = x^2 \) for \( x < 0 \)
- \( f(x) = x + 1 \) for \( x \geq 0 \)
This function can be implemented in MATLAB using:
syms x
f = piecewise(x < 0, x^2, x >= 0, x + 1);
In this example:
- For any input less than 0, \( f(x) \) returns the square of \( x \).
- For inputs greater than or equal to 0, it shifts the input by 1, generating a linear relationship.
Example 2: Piecewise Function with Multiple Conditions
Consider a more complex piecewise function where:
- \( f(x) = -1 \) for \( x < -1 \)
- \( f(x) = x^2 \) for \( -1 \leq x < 0 \)
- \( f(x) = x + 1 \) for \( 0 \leq x < 2 \)
- \( f(x) = 2x \) for \( x \geq 2 \)
This can be structured in MATLAB with the following code:
syms x
f = piecewise(x < -1, -1, -1 <= x < 0, x^2, 0 <= x < 2, x + 1, x >= 2, 2*x);
This function illustrates how varying conditions lead to different outputs and enables the modeling of complex behaviors across specified ranges.
Plotting Piecewise Functions
Visualizing a piecewise function allows for a clearer understanding of its behavior across the defined ranges. Using the `fplot` function in MATLAB, we can display our earlier defined piecewise function:
f = piecewise(x < 0, x^2, x >= 0, x + 1);
fplot(f, [-5, 5]);
grid on;
title('Piecewise Function Visualization');
xlabel('x');
ylabel('f(x)');
This code snippet will create a plot reflecting the defined piecewise function, enabling users to see how outputs change with respect to their inputs.
Handling Complex Scenarios
Example 3: Conditional Statements in MATLAB
In situations where you cannot use the `piecewise` function, or for educational purposes, you might want to define a piecewise function using conditional statements. Here’s how you might implement this:
x = -2:0.1:3; % Define the range
y = zeros(size(x)); % Initialize output array
for i = 1:length(x)
if x(i) < 0
y(i) = x(i)^2; % First piece
elseif x(i) >= 0 && x(i) < 2
y(i) = x(i) + 1; % Second piece
else
y(i) = 2*x(i); % Third piece
end
end
plot(x, y);
This loop iterates through the defined range of \( x \), applying different calculations based on the conditions specified. While this approach is functional, it's worth noting that MATLAB excels with vectorized operations, which enhances performance and clarity.
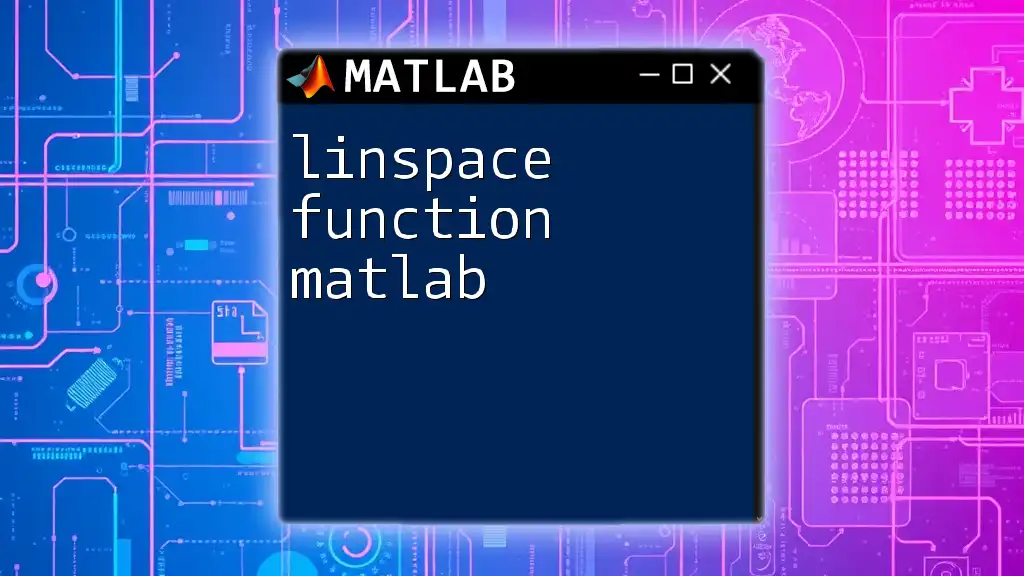
Advanced Applications of Piecewise Functions
Applications in Engineering and Physics
Piecewise functions are fundamental in physics and engineering disciplines. For example, they can model systems that exhibit different behaviors under varying conditions, such as phase transitions in materials or stress-strain relationships in mechanical structures.
Piecewise Functions in Data Analysis
In data analysis, piecewise functions can be used for curve fitting where a dataset may exhibit nonlinear trends within certain ranges. They allow for the construction of models that better capture the underlying relationships in the data, which improves predictions and insights.
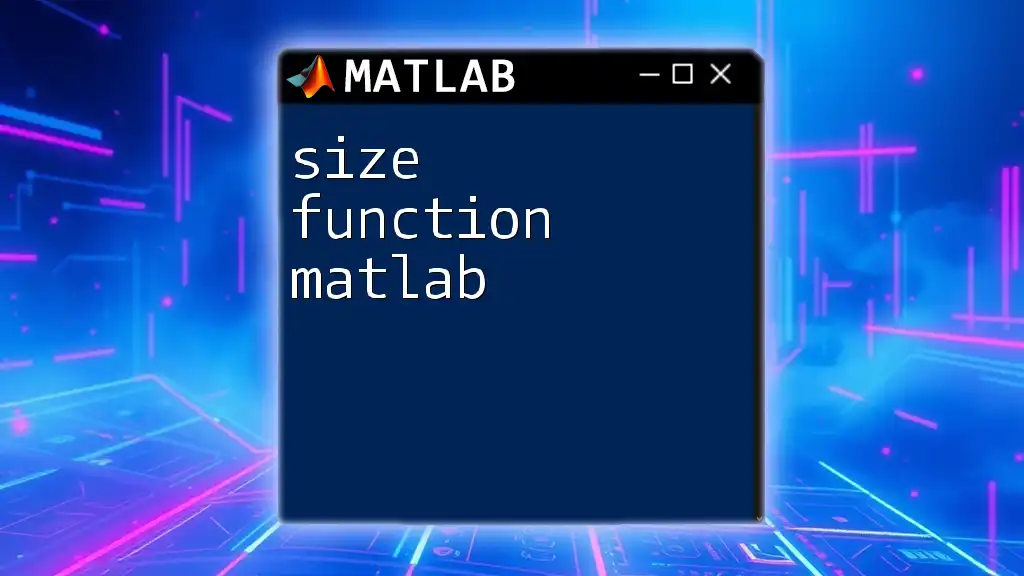
Best Practices for Working with Piecewise Functions in MATLAB
Common Pitfalls to Avoid
When implementing piecewise functions in MATLAB, it is crucial to watch for:
- Incorrect conditions: Ensure that boundaries do not overlap, which could lead to confusion and incorrect outputs.
- Edge cases: Be mindful of how the function behaves at transition points—these are often sources of discontinuities.
Optimizing Your Piecewise Functions
To ensure efficient and effective performance with piecewise functions:
- Leverage vectorization whenever possible to minimize computational overhead.
- Write clean, concise code that avoids redundancy, making the logic of the piecewise conditions easier to follow and maintain.
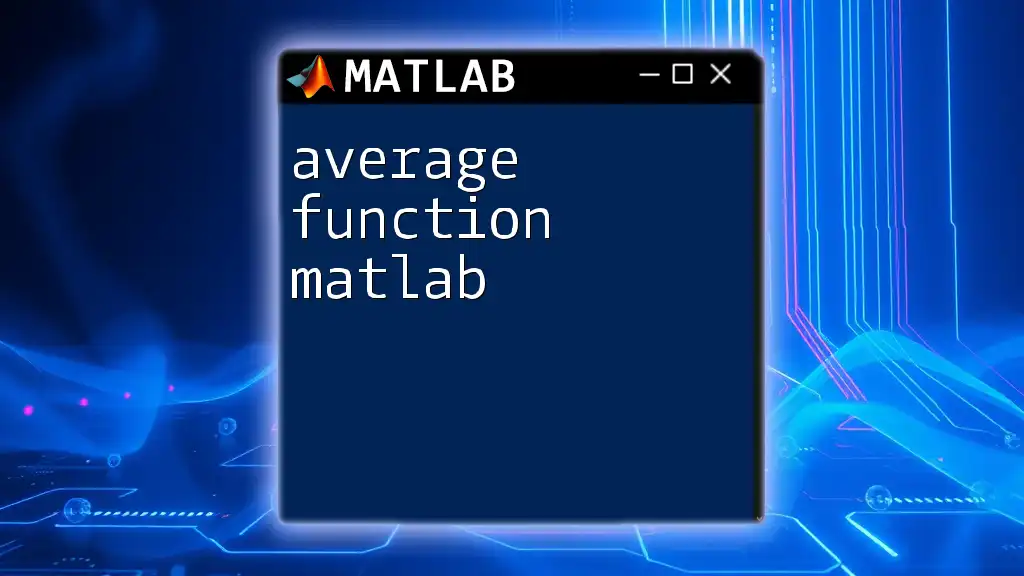
Conclusion
Understanding and implementing piecewise functions in MATLAB can greatly enhance your ability to model complex systems and analyze data effectively. By following best practices and avoiding common pitfalls, you can maximize the utility of these powerful mathematical constructs in your projects.
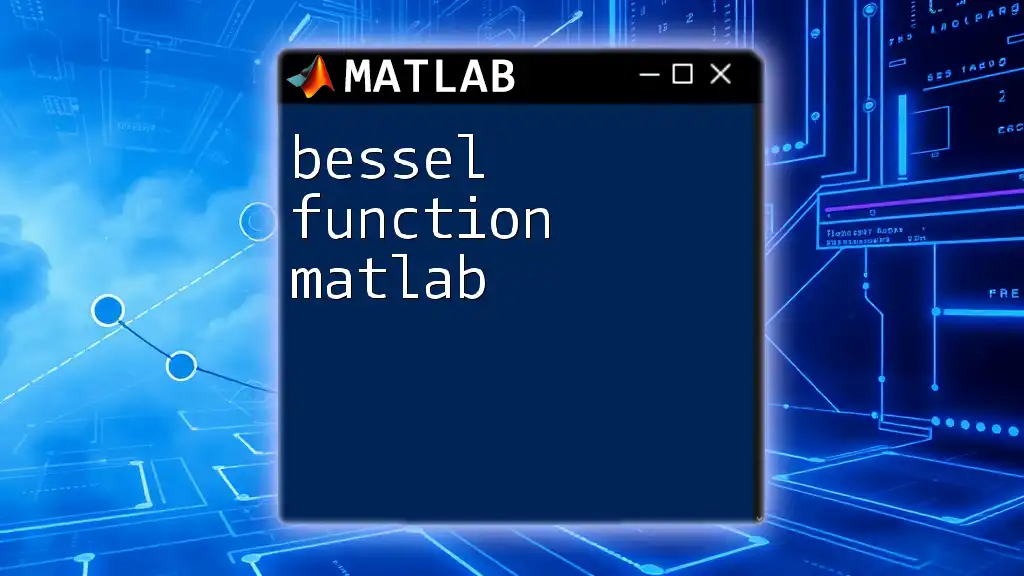
Additional Resources
To deepen your understanding of piecewise functions in MATLAB, consider exploring the official documentation on the `piecewise` function and related tutorials that focus on advanced applications.
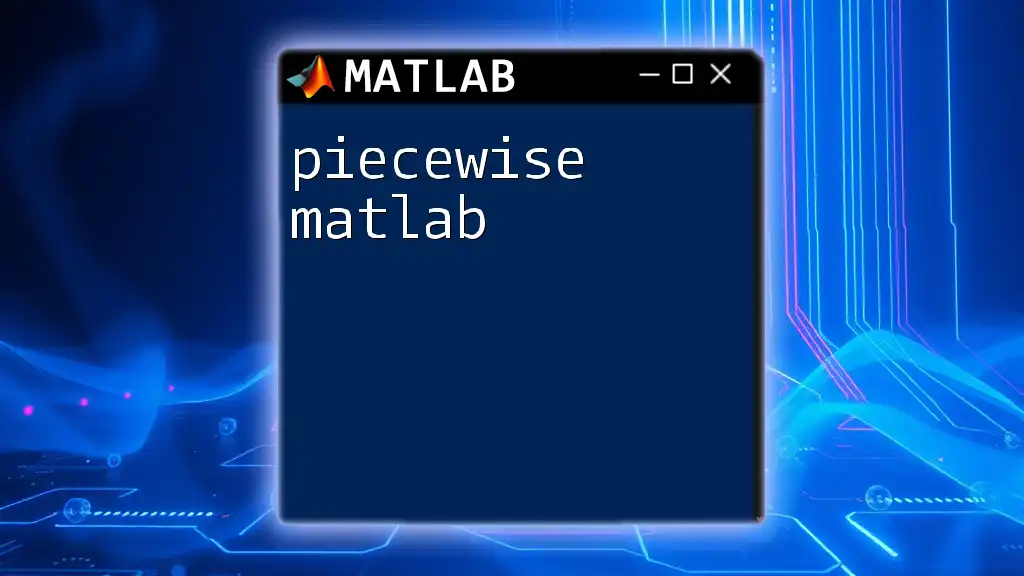
Call to Action
If you found this guide helpful and want to dive deeper into MATLAB programming, consider subscribing for more tutorials. Join our community to engage with fellow learners and share your own experiences with piecewise functions in MATLAB!