In MATLAB, the `if...elseif...else` construct allows for conditional execution of code based on various logical tests, enabling you to define alternative actions depending on which condition is true.
Here's a simple example:
x = 10;
if x < 0
disp('x is negative');
elseif x == 0
disp('x is zero');
else
disp('x is positive');
end
Understanding Conditional Statements
What are Conditional Statements?
Conditional statements are fundamental structures in programming that allow a program to make decisions based on certain conditions. They enable the control flow in code, determining which block of code executes based on whether a specified condition evaluates to true or false.
Overview of the `if` Statement in MATLAB
The `if` statement is the simplest form of a conditional statement in MATLAB. Its primary role is to execute a block of code only when the specified condition is true.
The syntax for a basic `if` statement is as follows:
if condition
% code to execute if condition is true
end
For example:
x = 10;
if x > 5
disp('x is greater than 5');
end
In this example, since the condition `x > 5` is true, the program will output "x is greater than 5."
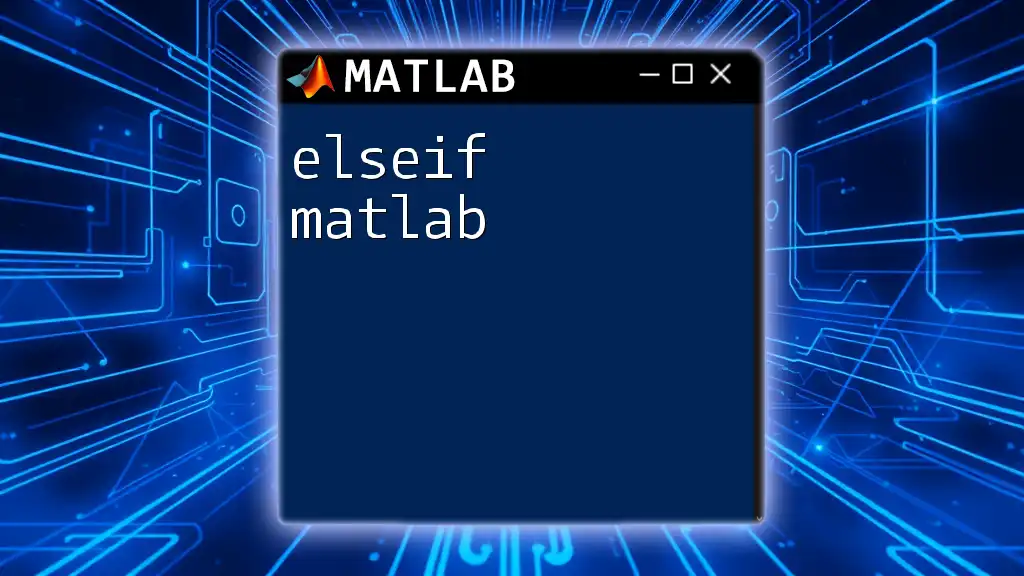
The `if else if` Structure
What is the `else if` Statement?
The `else if` statement, also known as `elseif`, provides a way to check multiple conditions sequentially. If the initial `if` condition is not met, the program will evaluate the next `elseif` condition. This effectively allows for branching logic in your code.
Syntax of `if else if`
The general structure of the `if else if` statement in MATLAB is:
if condition1
% code if condition1 is true
elseif condition2
% code if condition2 is true
else
% code if all conditions are false
end
This structure allows for multiple possible outcomes, giving your MATLAB scripts a flexible approach to decision-making.
Practical Example: Using `if else if`
Let’s illustrate the `if else if` structure through a practical example: a grading system based on a student's score.
score = 85;
if score >= 90
grade = 'A';
elseif score >= 80
grade = 'B';
elseif score >= 70
grade = 'C';
else
grade = 'D';
end
disp(['Your grade is: ', grade]);
In this code snippet, the program checks the score against several conditions. Since the score is 85, the output will be "Your grade is: B".
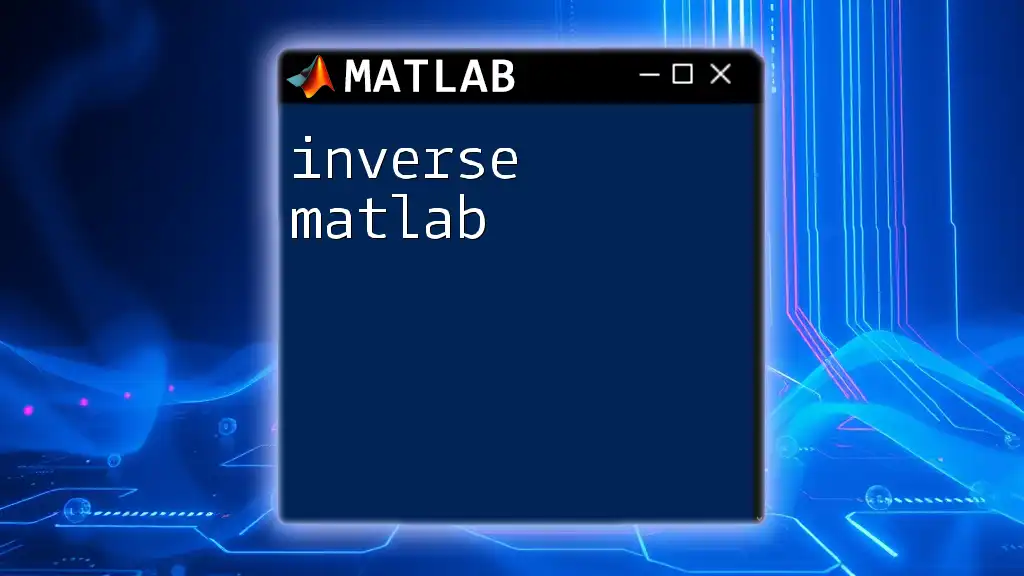
Key Concepts and Best Practices
Logical Operators in MATLAB
Logical operators are essential when combining multiple conditions. Here are some common logical operators in MATLAB:
- `&&` (Logical AND): Returns true if both conditions are true.
- `||` (Logical OR): Returns true if at least one condition is true.
- `~` (Logical NOT): Negates a condition.
Using these operators can enhance `if else if` statements, empowering you to create more complex and robust conditions. For example:
x = 4;
y = -3;
if (x > 0) && (y > 0)
disp('Both x and y are positive.');
else
disp('At least one of x or y is non-positive.');
end
In this example, the condition checks if both `x` and `y` are positive. Since `y` is negative, the output will be "At least one of x or y is non-positive."
Nesting `if else if` Statements
Nesting allows you to place an `if` statement inside another `if` statement. This is useful for scenarios that require multiple levels of conditions. Here is an example:
age = 20;
if age < 18
disp('You are a minor.');
else
if age < 65
disp('You are an adult.');
else
disp('You are a senior.');
end
end
This code checks the age and properly categorizes the person as a minor, adult, or senior depending on their age.
Avoiding Common Mistakes
When using `if else if` constructs, a few common pitfalls may arise, such as:
- Improper nesting: Ensure that each `if` and `elseif` statement is correctly paired with its corresponding `end`.
- Using `=` instead of `==`: Remember that `=` is for assignment and `==` is for comparison.
- Overlapping conditions: Make sure your conditions do not overlap or contradict, as this can lead to unexpected behavior.
Debugging tips to troubleshoot issues include:
- Utilize `disp` statements to output values and track which conditions are being executed.
- Break down complex conditions into smaller, manageable parts to isolate problems.
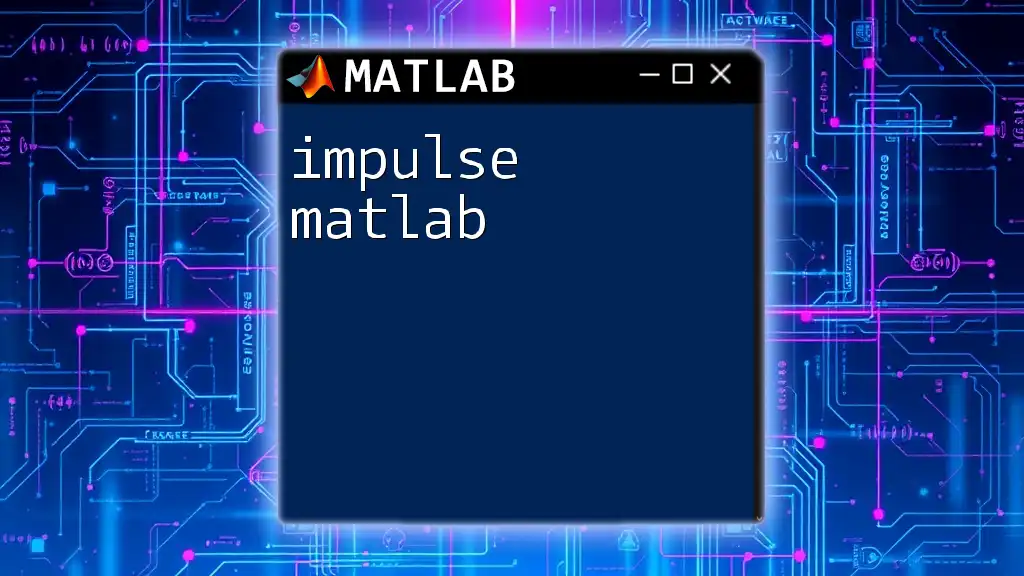
Performance and Optimization
Minimizing Conditions for Efficiency
To enhance performance, always aim to keep your conditions as simple as possible. Avoid unnecessary checks that can lead to redundant computations. For instance, if one condition is guaranteed to cover a significant branch of logic, you should place it first to short-circuit further checks.
Here's an example of coding scenario with unnecessary conditions:
if x > 0
% code
elseif x > 1
% code
end
Instead, the following is more efficient:
if x > 1
% code
elseif x > 0
% code
end
Readability and Code Maintenance
Clear code is easier to read and maintain. Follow these guidelines for writing understandable `if else if` statements:
- Use meaningful variable and condition names: This aids in understanding the purpose of each condition.
- Comment your code: Brief comments can quickly remind you and others of the logic behind complex conditions.
- Limit nesting: Too much nesting can make code difficult to follow. If you find your logic becoming overly complicated, consider refactoring.
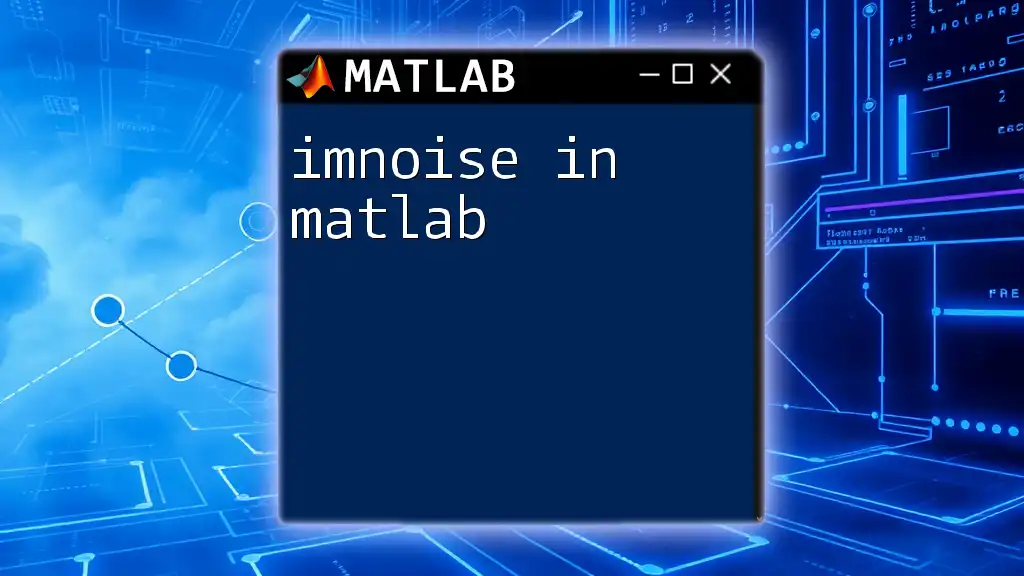
Advanced Topics
Using `switch` Statements as an Alternative
When dealing with multiple discrete values, `switch` statements can sometimes serve as a cleaner alternative to `if else if`. They allow you to evaluate a variable against a series of cases. Here’s how a `switch` statement looks:
value = 'B';
switch value
case 'A'
disp('Excellent!');
case 'B'
disp('Good job!');
case 'C'
disp('You can do better.');
otherwise
disp('Invalid grade.');
end
This structure can be easier to read and maintain when checking a single variable against many possible values.
Practical Applications of `if else if` in MATLAB
The `if else if` structure finds use in various real-world applications, from data analysis where you interpret data values to automated decision-making processes such as adaptive algorithms. For example, in a data analysis context, you might want to categorize a variable based on ranges of values:
temperature = 30;
if temperature < 0
category = 'Freezing';
elseif temperature < 20
category = 'Cold';
elseif temperature < 30
category = 'Warm';
else
category = 'Hot';
end
disp(['Temperature category: ', category]);
This kind of decision-making structure is common in many scripting scenarios.
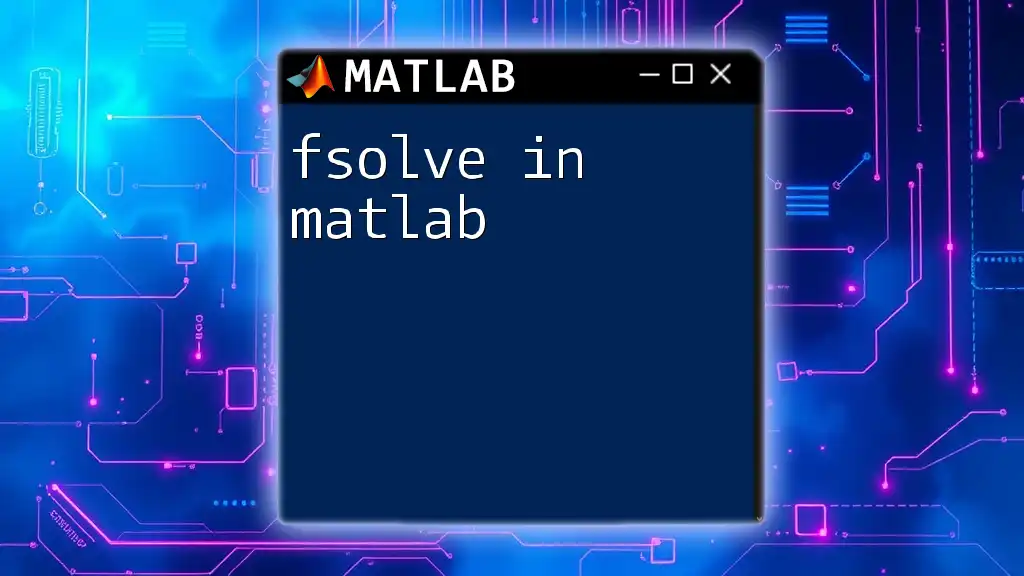
Conclusion
The `if else if` statement is a significant component of writing effective MATLAB code, enabling complex decision trees and logic execution within your programs. By mastering this control structure, along with the associated guidelines and best practices, you will enhance both your coding proficiency and your capability to produce clear, maintainable scripts. Practice with the provided examples and work on your unique challenges to gain confidence in using `if else if` in MATLAB.