The `ismember` function in MATLAB checks whether elements of one array are present in another array, returning an array of the same size as the first array, with logical `1` (true) for each element that is a member and `0` (false) otherwise.
Here's a code snippet demonstrating its use:
A = [1, 2, 3, 4, 5];
B = [3, 5, 7];
C = ismember(A, B);
% C will be [0 0 1 0 1]
Understanding the Syntax
Basic Syntax of `ismember`
The `ismember` function in MATLAB compares two arrays and returns a logical array indicating whether each element of the first array is present in the second array. The basic syntax for using `ismember` is as follows:
L = ismember(A, B)
- A: This is the array or cell array that you want to test for membership. It can be of various types, including numeric, character, or cell arrays.
- B: This second array acts as the reference set against which A is checked. It can also be any type of MATLAB array.
- L: The output, a logical array, where each element corresponds to A, returning `true (1)` if the element exists in B, or `false (0)` if it does not.
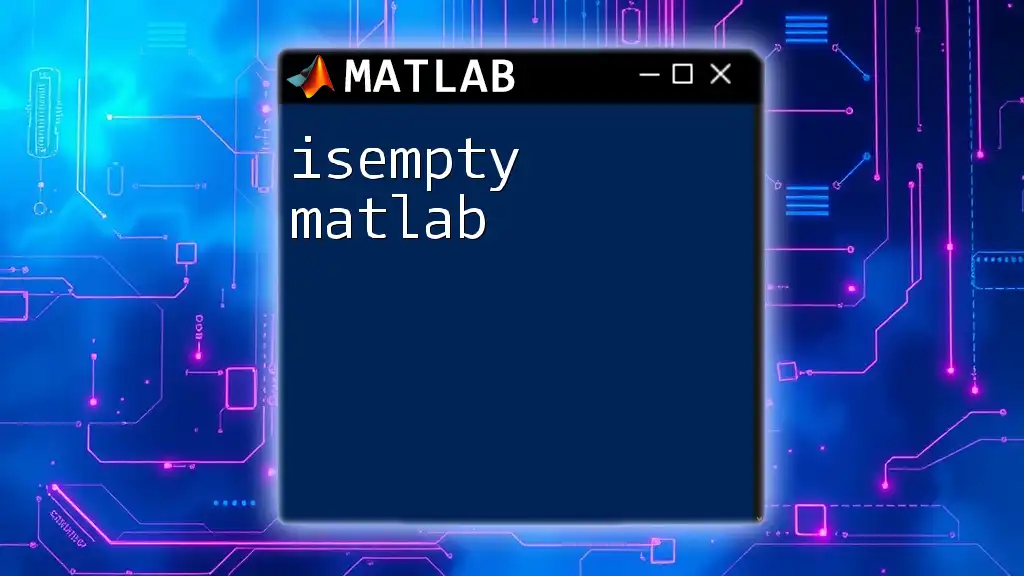
Practical Examples
Example 1: Basic Usage of `ismember`
To demonstrate the fundamental application of `ismember`, consider the following scenario: You have two numeric arrays and you want to check which elements of the first array exist in the second one.
A = [1, 2, 3, 4];
B = [2, 4, 6];
L = ismember(A, B)
In this example, `L` would return:
L =
0 1 0 1
This indicates that the second and fourth elements of A (the numbers 2 and 4) are indeed members of B.
Example 2: Using `ismember` with Strings
`ismember` can also be applied to string data, which is critical in data processing and analysis.
A = {'apple', 'banana', 'cherry'};
B = {'banana', 'kiwi'};
L = ismember(A, B)
The output will be:
L =
0 1 0
This shows that only 'banana' from A exists in B.
Example 3: Working with Cell Arrays
Another specific use case involves checking membership with cell arrays. Here’s how that works:
A = {1, 2, 3, 4};
B = {2, 4, 6};
L = ismember(A, B)
In this case, L will still outputs a logical array:
L =
0 1 0 1
Again confirming that elements 2 and 4 from cell array A are found in array B.
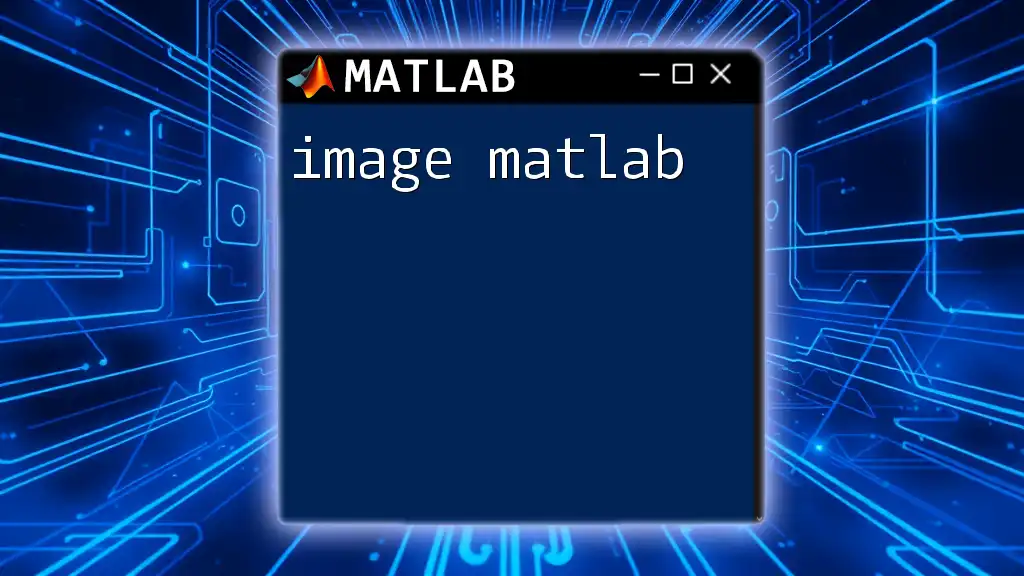
Advanced Usage
Multiple Dimensions and Matrices
When dealing with multi-dimensional arrays, `ismember` remains a useful function. If the dimension of A and B allows for it, you can compare entire matrices—consider this example:
A = [1, 2; 3, 4];
B = [4, 1];
[L, loc] = ismember(A, B)
In this case, `L` will yield a logical array and `loc` will provide the index of `B` corresponding to values in A.
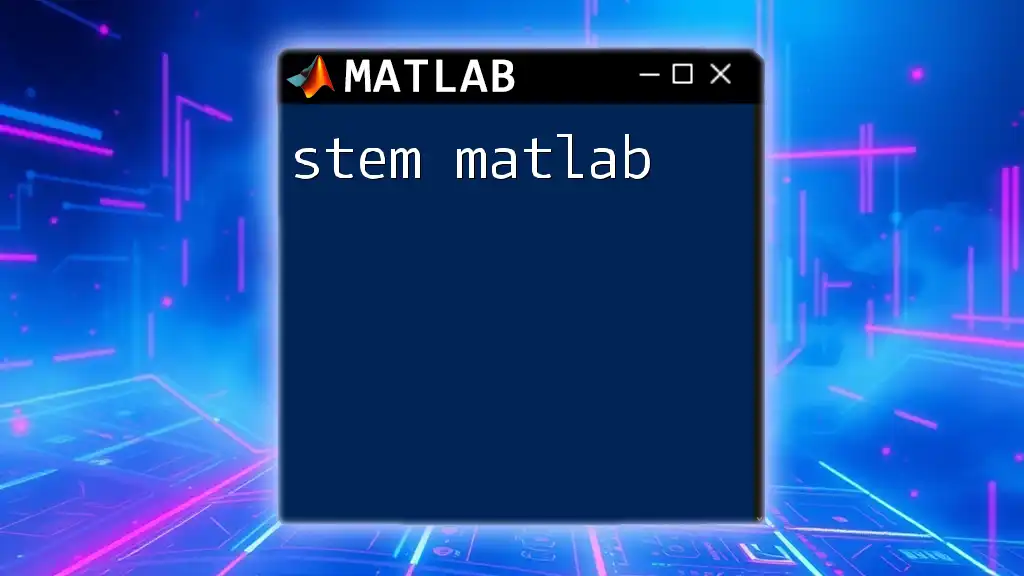
Performance Considerations
Efficiency in Large Datasets
When working with larger datasets, performance becomes critical. The `ismember` function may slow down as the size of A and B increases. For significant performance gains, it's advantageous to sort your arrays before using `ismember`. This way, the search for memberships can be optimized.
Best Practices for Optimizing Use
To boost performance while leveraging `ismember`, consider using sorted arrays combined with logical indexing rather than brute force membership checking. For example:
A = sort([2, 3, 1, 4]);
B = sort([4, 1]);
[L, loc] = ismember(A, B)
Sorting helps mitigate the computational cost and enhances efficiency during function execution.
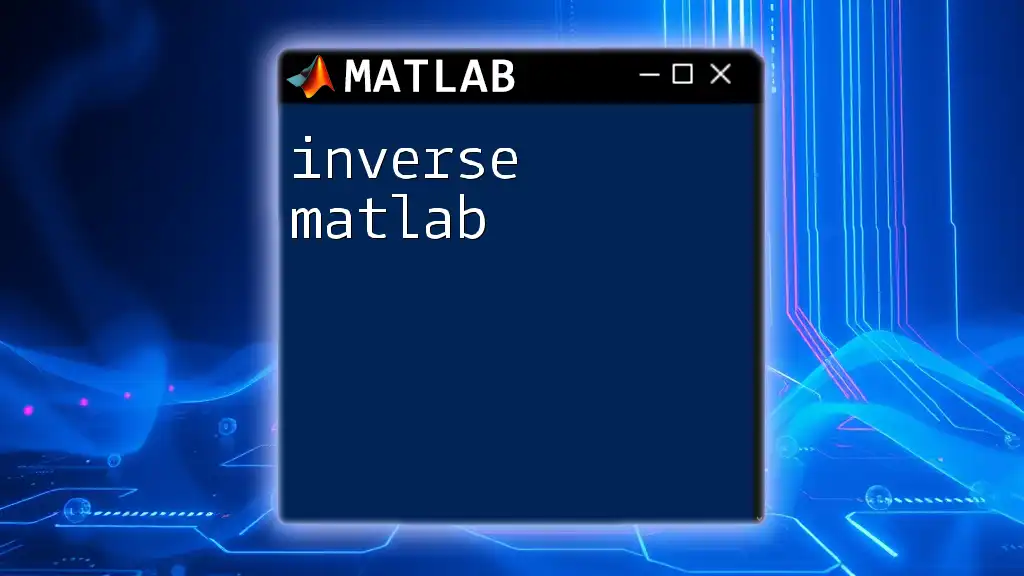
Handling Unique Cases
Dealing with NaN Values
One common challenge in MATLAB programming is managing NaN (Not a Number) values. When using `ismember`, NaN is treated as a unique value. Consider the following code:
A = [1, NaN, 3];
B = [NaN, 2];
L = ismember(A, B)
In this case, the output will reflect that NaN from A is recognized as being part of B:
L =
0 1 0
Case Sensitivity in String Matching
When working with character arrays or strings, it is important to note that `ismember` performs case-sensitive comparisons. This means that:
A = {'Apple', 'banana'};
B = {'apple', 'Banana'};
L = ismember(A, B)
In this case, L will return:
L =
0 0
This outcome signifies that neither 'Apple' nor 'banana' matches 'apple' or 'Banana' because of case differences.
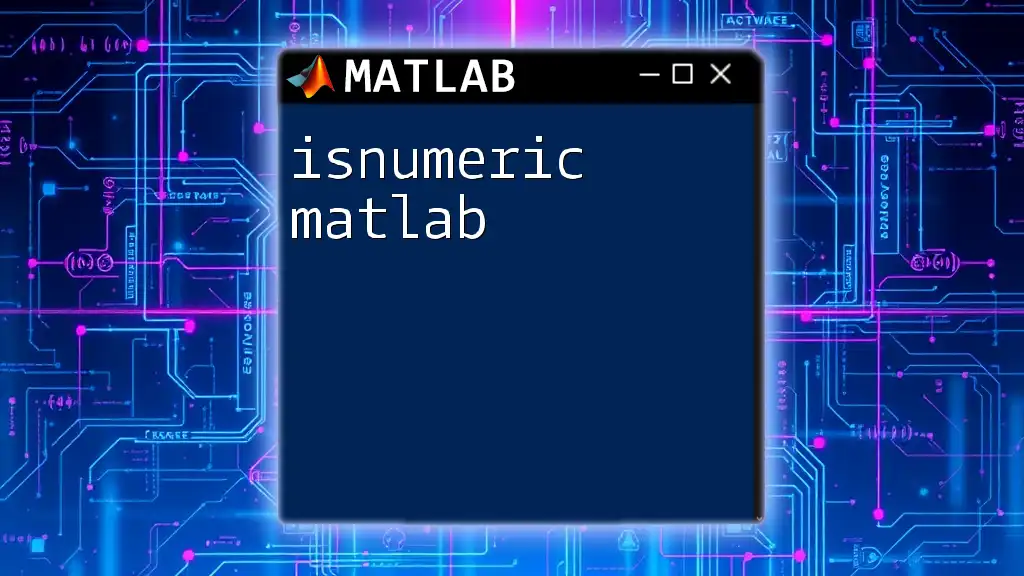
Common Errors and Troubleshooting
Common Mistakes to Avoid
One common error when using `ismember` arises from mismatched data types or dimensions. For example, if you inadvertently attempt to compare arrays of incompatible sizes, MATLAB will throw an error. Additionally, trying to compare numeric data with cell arrays without appropriate conversion can lead to unexpected results.
Debugging Tips
If you encounter issues with `ismember`, consider checking the types and sizes of A and B using the `class` and `size` functions before running `ismember`. This practice helps ensure that you're operating on compatible data types.
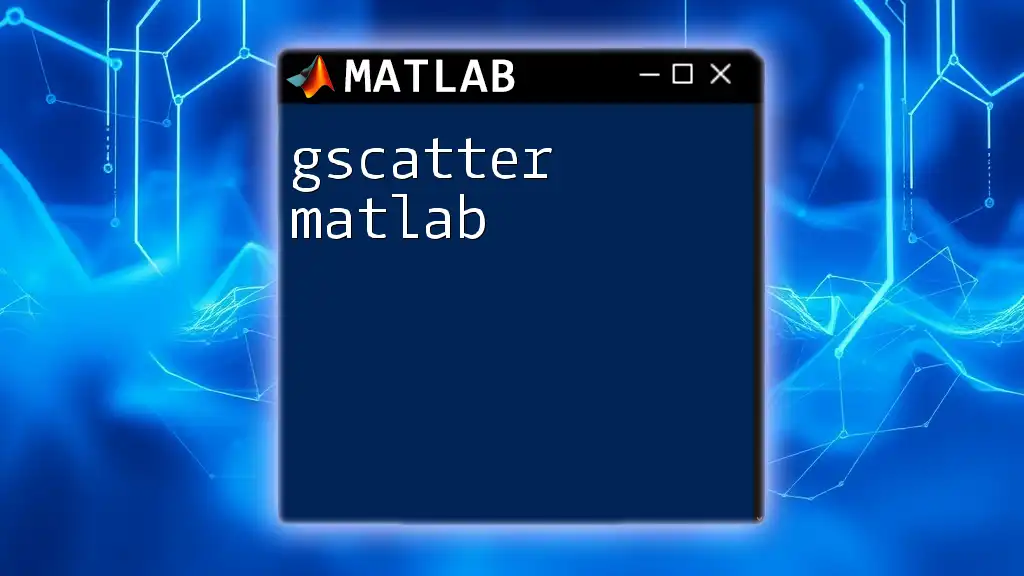
Conclusion
The `ismember` function is a powerful tool in MATLAB, allowing for efficient membership checks across a variety of data types. By mastering its syntax, understanding its performance implications, and knowing how to handle special cases, you can significantly enhance your data processing capabilities. Regular practice with `ismember` will equip you to tackle increasingly complex problems in your MATLAB projects with confidence.
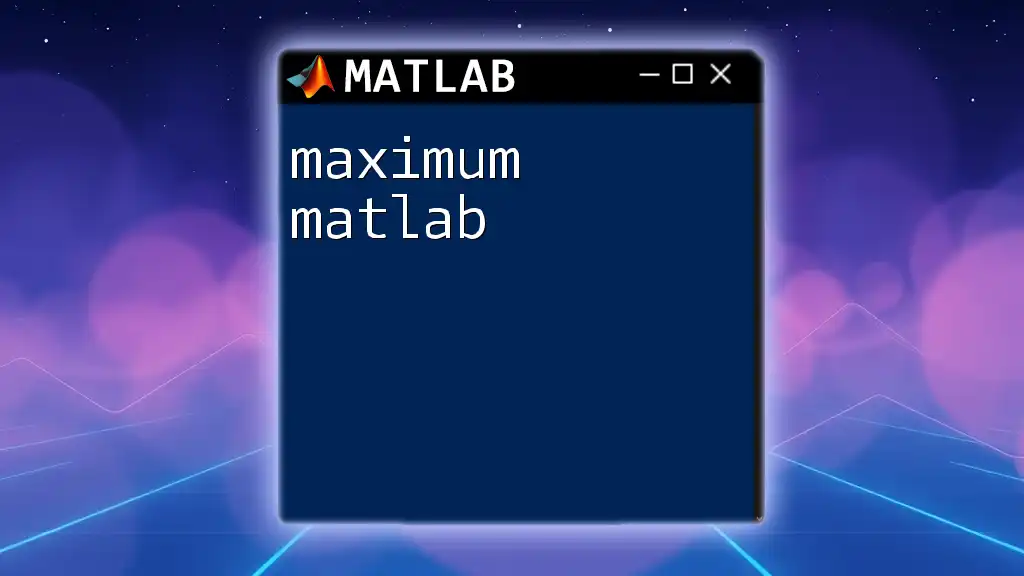
Additional Resources
For those interested in diving deeper into the functionality of `ismember` in MATLAB, consider exploring the official MATLAB documentation for comprehensive examples and use cases. Furthermore, seek additional resources and tutorial videos to broaden your understanding and application of set theory in MATLAB.