In MATLAB, the `if` statement allows you to execute a block of code conditionally based on whether a specified logical expression evaluates to true.
Here’s a simple code snippet demonstrating the usage of `if` in MATLAB:
x = 10;
if x > 5
disp('x is greater than 5');
end
Understanding the `if` Statement in MATLAB
What is an `if` Statement?
The `if` statement is a fundamental concept in programming that allows you to execute certain blocks of code based on whether a specified condition is true or false. This capability to make decisions is crucial in creating dynamic and responsive applications. In MATLAB, `if` statements are predominantly used for flow control, allowing a program to react differently to varying inputs or states, much like making decisions in real life.
Basic Syntax of the `if` Statement
The syntax for a basic `if` statement in MATLAB is straightforward. It consists of a condition followed by the code to execute if the condition evaluates to true. Here's how it looks:
if condition
% Code to execute if condition is true
end
For example, consider the following snippet:
x = 5;
if x > 0
disp('x is positive');
end
In this scenario, since `x` is indeed greater than zero, MATLAB will display the message "x is positive." This illustrates how conditional logic can help direct program flow.
Using `elseif` and `else`
Sometimes, you want to check multiple conditions in a single `if` construct. MATLAB allows for this through `elseif` and `else`. The `elseif` allows you to specify a new condition if the previous one is false, while `else` provides a default block if none of the conditions are met.
Here's a practical example:
x = 0;
if x > 0
disp('x is positive');
elseif x < 0
disp('x is negative');
else
disp('x is zero');
end
In this case, since `x` equals zero, the output will be "x is zero." This clarity in branching logic helps keep your code readable and efficient.
Nested `if` Statements
To handle more complex situations, you can nest `if` statements, enabling multiple layers of conditions. This approach allows you to check a condition within another condition leading to richer decision-making capabilities.
Here’s an example of nested `if` statements:
x = 10;
if x > 0
if mod(x, 2) == 0
disp('x is positive and even');
else
disp('x is positive and odd');
end
end
In this instance, the program checks if `x` is positive. If it is, it further checks if `x` is even or odd, demonstrating how nested conditions can be effectively used to derive more granular outcomes.
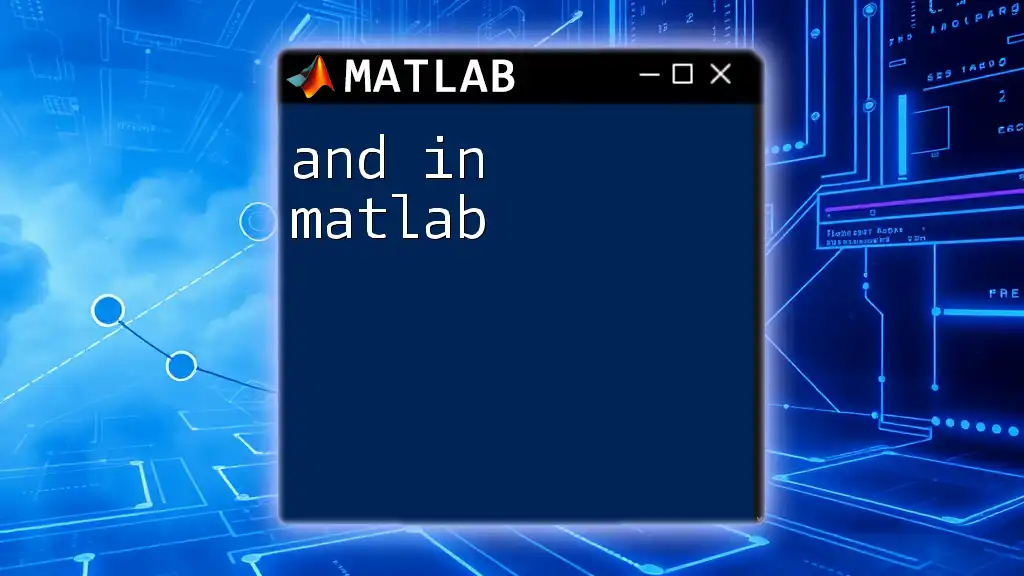
The `in` Keyword in MATLAB
Understanding Logical Indexing
Logical indexing pertains to how we can access and manipulate arrays based on condition checks. The `in` concept in MATLAB is often represented as checking for the presence of an element within an array or set. This can significantly streamline data selection processes in your code.
Using `ismember` to Check Inclusion
In MATLAB, the `ismember()` function serves as the primary means to determine if elements from one array exist in another. The basic syntax is as follows:
C = ismember(B, A);
Here, `B` represents the array you want to check against `A`. For example:
A = [1, 2, 3, 4];
B = [2, 4, 6];
C = ismember(B, A); % Returns [true, true, false]
This code shows that the numbers 2 and 4 are present in array `A`, while 6 is not. Utilizing `ismember` provides a clear and straightforward method for checking membership.
Using `in` with Arrays for Conditional Operations
You can effectively combine the functionality of `in` with `if` statements for conditional checks. This is particularly useful when you want to execute certain code based on array membership.
Consider this example:
A = [1, 2, 3, 4, 5];
numToCheck = 3;
if ismember(numToCheck, A)
disp('The number is in the array.');
else
disp('The number is not in the array.');
end
Here, since `3` is indeed part of array `A`, the output will be "The number is in the array." This integration of logical indexing with conditional checks enhances the granularity of your decision-making.
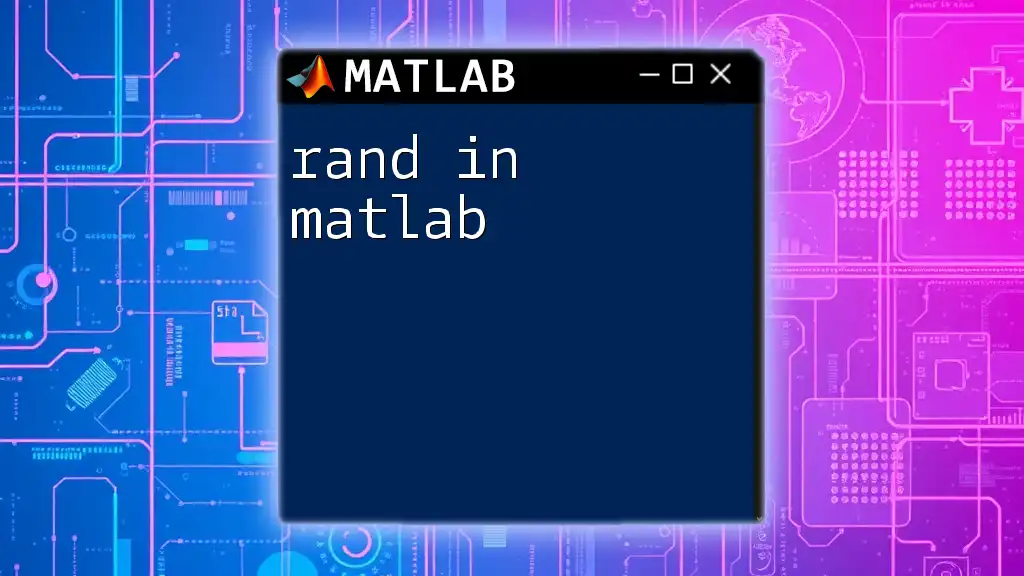
Best Practices for Using `if` and `in` in MATLAB
Clarity and Readability
When writing code, it’s essential to prioritize clarity and readability. A well-structured `if` statement enhances code comprehension by clearly delineating logic paths. Use meaningful variable names and maintain consistent indentation to convey the structure efficiently.
Additionally, incorporating comments in your code snippets significantly aids understanding. These line-by-line explanations can clarify the intent and function of your logic, proving invaluable for future reference.
Performance Considerations
While MATLAB efficiently handles conditional statements, it’s wise to avoid convoluted logic that could impact performance. Stringing too many nested `if` statements can lead to slower execution times. It's often more effective to separate complex logic into simpler functions or utilize vectorized operations when possible.
This approach not only speeds up execution but makes your code cleaner and more maintainable.
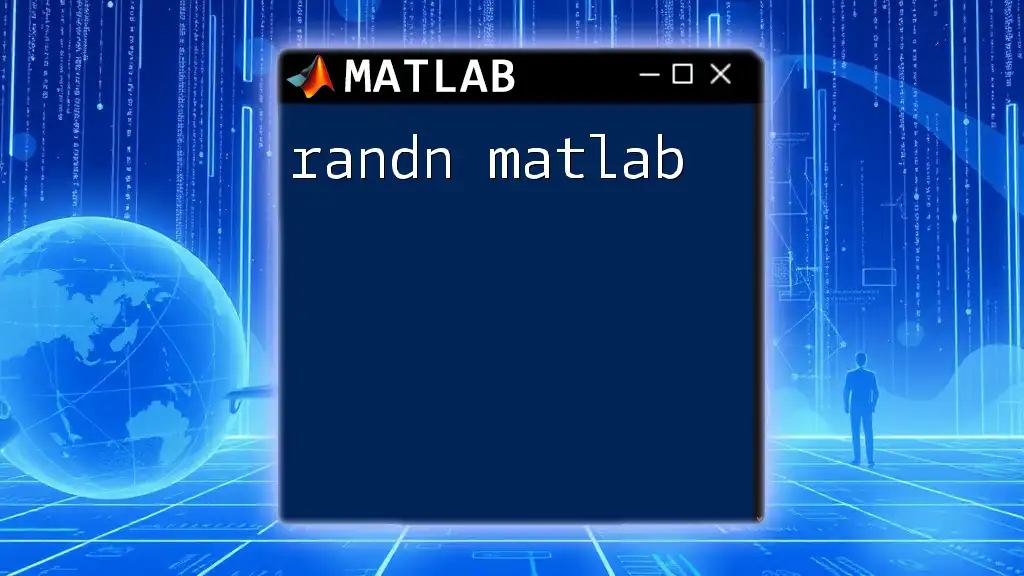
Common Errors and Troubleshooting
Mismatched Data Types
A common error when using `if` statements involves mismatched data types. Ensure that the conditions being evaluated are compatible (e.g., comparing strings against numbers) to avoid runtime errors.
Logic Errors in Conditions
Logic errors—such as using `>` instead of `>=`—can lead to unexpected behavior. Regularly reviewing your logical conditions and possibly using debugging tools can help catch these errors promptly.
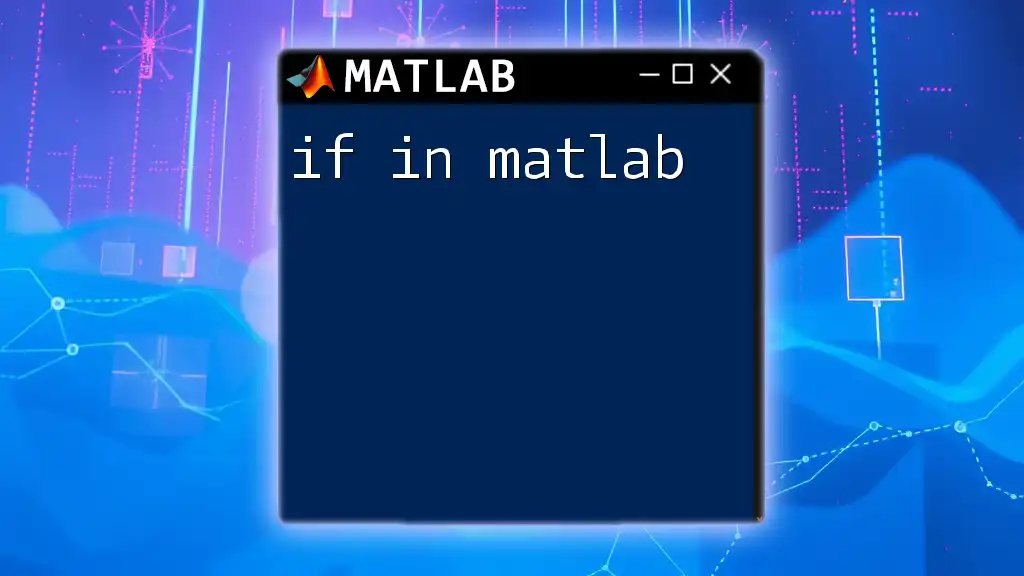
Conclusion
Mastering the use of `if and in matlab` significantly enhances your coding capabilities, allowing you to write dynamic, responsive, and efficient code. Embrace these concepts, and don't shy away from experimenting with different scenarios to deepen your understanding. The ability to make precise, conditional decisions is invaluable to your programming toolkit.
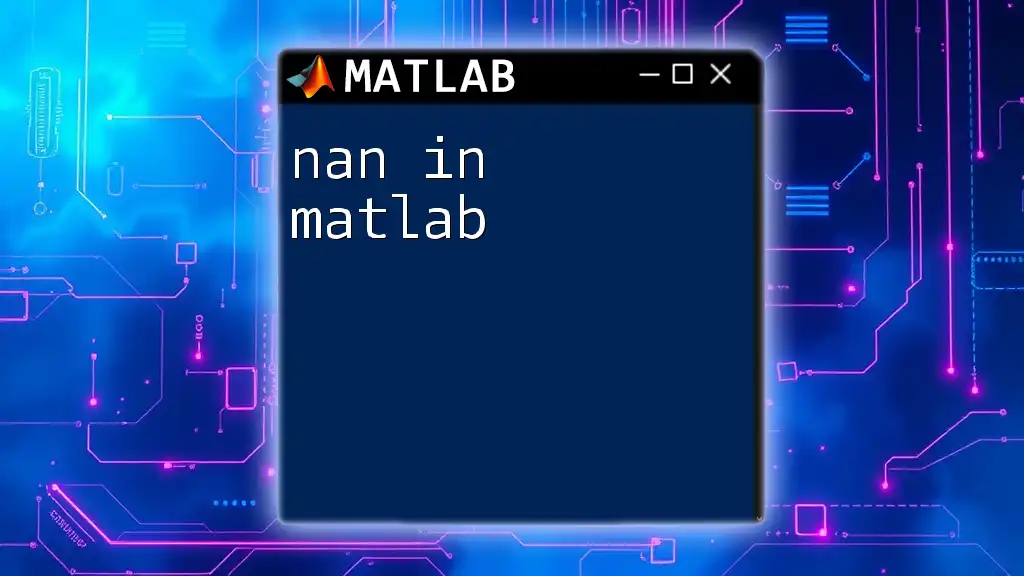
Further Resources
For those eager to learn more, refer to the official MATLAB documentation for more in-depth insights. You can also find numerous practice exercises and examples that will allow you to explore these concepts further and solidify your understanding.