In MATLAB, the `and` operator is used to perform element-wise logical conjunction between two arrays or conditions, returning true only when both corresponding elements are true.
Here's a simple example:
A = [1, 0, 1];
B = [1, 1, 0];
result = A & B; % This will return [1, 0, 0]
Understanding Logical Operators in MATLAB
What are Logical Operators?
Logical operators are fundamental components of programming languages, including MATLAB. They allow you to create conditions and make decisions based on the truth values of expressions. In MATLAB, there are several common logical operators, such as AND, OR, and NOT. Each of these operators serves a distinct purpose and helps control the flow of your code.
The Significance of the AND Operator
The AND operator, often represented in MATLAB by `&` and `&&`, evaluates whether multiple conditions are true. When using AND, the overall expression evaluates to true only if all individual conditions are true.
For instance, in the expression `A && B`, if both `A` and `B` are true, the result will be true. If either condition is false, the result is false. This property makes the AND operator particularly useful for enforcing multiple conditions simultaneously.
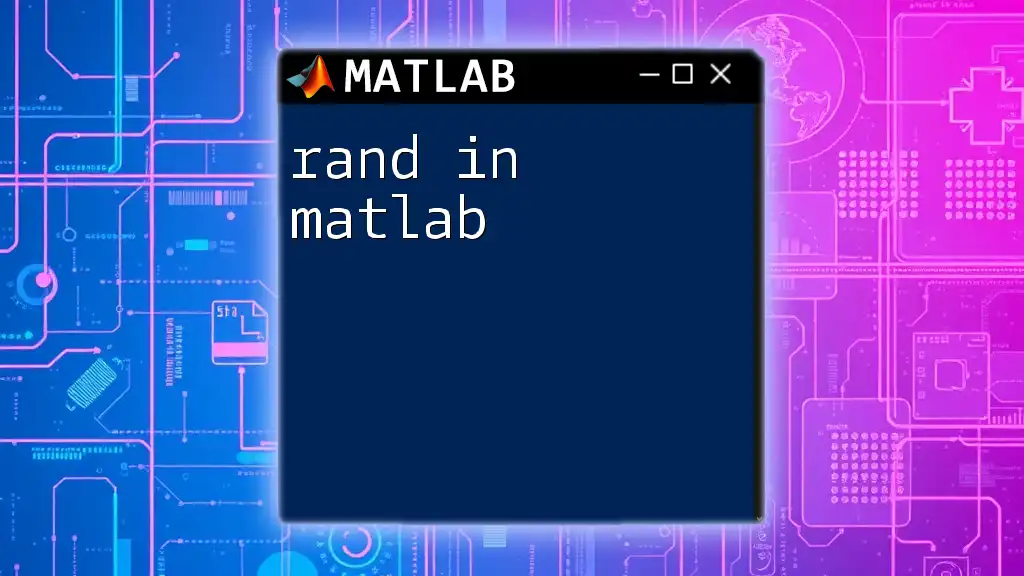
Syntax and Usage of the AND Operator
Using `&` for Element-wise AND Operations
The `&` operator in MATLAB performs element-wise logical AND operations on arrays. This means that it evaluates each corresponding pair of elements from the input arrays.
Here's how it works:
A = [1, 0, 1];
B = [1, 1, 0];
C = A & B; % Result: [1 0 0]
In this example, the result `C` consists of three elements:
- The first element of `C` is `1` because both corresponding elements in `A` and `B` are `1`.
- The second element of `C` is `0` because `A` has `0` and `B` has `1`.
- The third element is `0` because `A` has `1` and `B` has `0`.
This example illustrates how the `&` operator evaluates each element independently, giving you the flexibility to perform logical operations on arrays.
Using `&&` for Short-circuiting AND Operations
In contrast, the `&&` operator is used for short-circuiting logical AND operations. This operator evaluates only the necessary conditions to determine the result.
Consider the following example:
x = true;
y = false;
if x && y
disp('Both are true');
else
disp('At least one is false'); % This will execute
end
In this scenario, `x` is true and `y` is false. The expression `x && y` evaluates to false, and therefore the "At least one is false" message is displayed.
Short-circuiting means that if the first condition is false when using `&&`, MATLAB doesn't evaluate the second condition because the overall result cannot be true. This behavior can enhance performance, especially in more complex expressions where the second condition involves expensive computations or function calls.
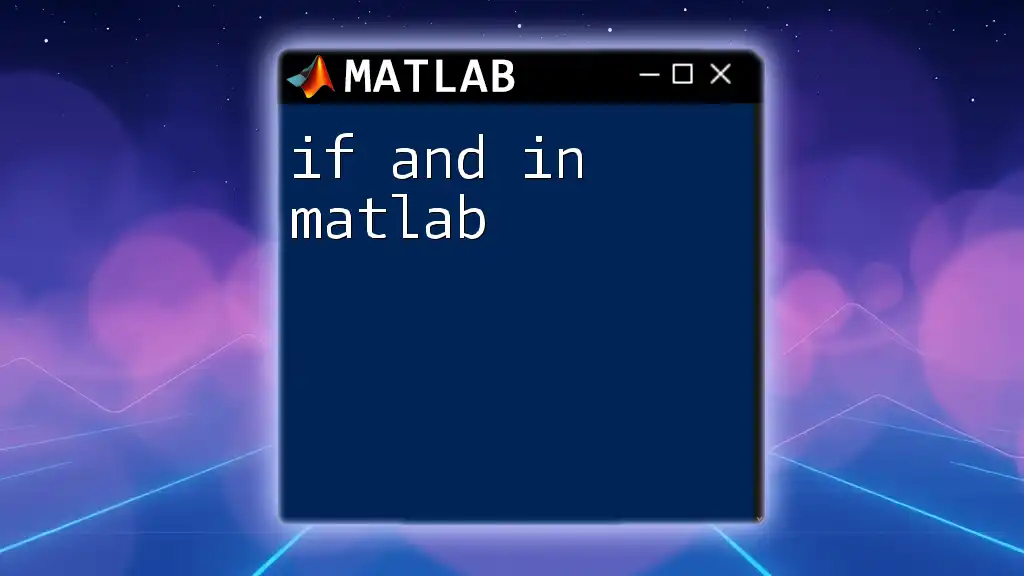
Practical Applications of the AND Operator in MATLAB
Conditional Statements
One of the most common places to use the AND operator is within conditional statements. By combining multiple conditions with AND, you can execute code only when all specified conditions are satisfied.
For example:
a = 5;
b = 10;
if a > 0 && b > 0
disp('Both numbers are positive');
end
In this case, both conditions must be true for the message "Both numbers are positive" to display, demonstrating how the AND operator can be employed to enforce multiple checks.
Filtering Data
The AND operator is highly useful for logical indexing, allowing you to filter arrays based on multiple criteria. Here's an example:
data = [1, 2, 3, 4, 5];
filteredData = data(data > 2 & data < 5); % Result: [3 4]
In this code, the expression `data > 2 & data < 5` creates a logical array that specifies which elements satisfy both conditions. As a result, `filteredData` contains only the elements `3` and `4`, showcasing the practical application of the AND operator in data filtering tasks.
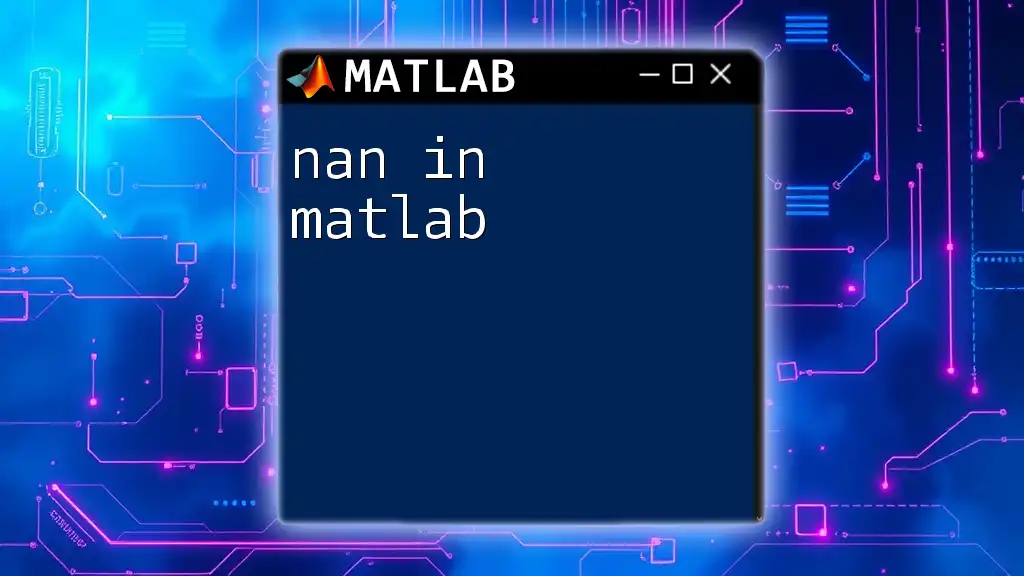
Combining Logical Expressions with AND
Complex Conditions
You can create complex logical conditions by combining multiple expressions using the AND operator. This is particularly beneficial when dealing with more intricate decision-making processes.
For example:
age = 25;
isStudent = true;
if age < 30 && isStudent
disp('Eligible for student discount');
end
In this scenario, the code checks whether the person is below 30 years old and whether they are a student. If both conditions are true, the message about the student discount eligibility is displayed, emphasizing the utility of combining logical expressions for real-world applications.
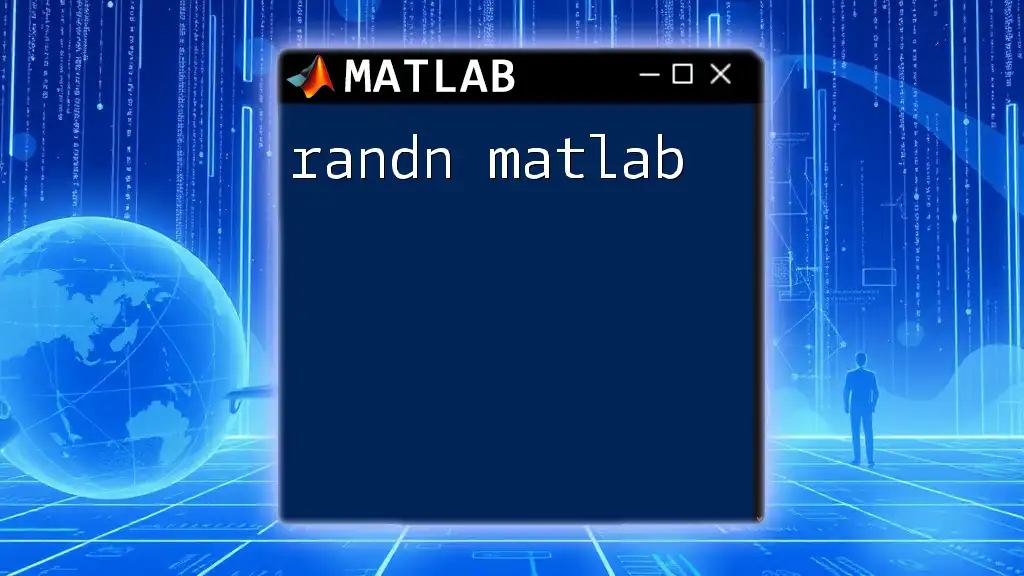
Common Pitfalls and Troubleshooting
Understanding Operator Precedence
One common pitfall when using logical operators in MATLAB is misunderstanding operator precedence. MATLAB evaluates expressions based on specific rules, which can lead to unexpected results if not properly managed.
Here's an example:
x = 1; y = 0; z = 1;
result = x & (y && z); % Correct understanding of logical flow
In this code, it’s essential to recognize how each part of the expression is evaluated. Correctly understanding how different operators interact helps prevent unexpected outcomes.
Debugging Logical Errors
When debugging logical errors, it can be helpful to visualize the conditions being evaluated. When you encounter an issue with an AND operation, check if each condition is producing the expected truth value. Oftentimes, the confusion between `&` and `&&` can lead to bugs, especially in complex logical statements.
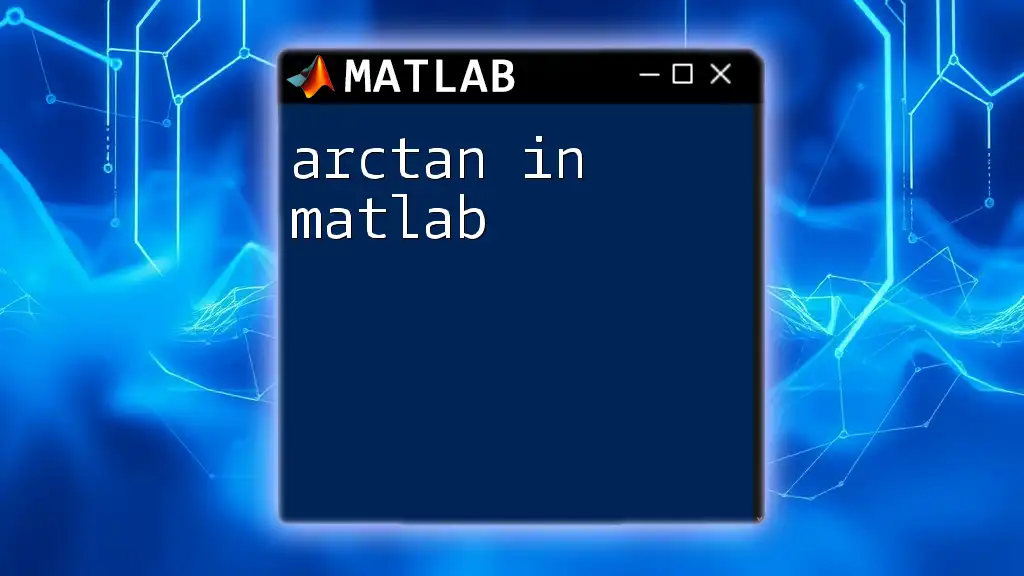
Best Practices when Using AND in MATLAB
Clarity in Code
When writing code, clarity should always be a priority. Using the AND operator can quickly make conditions complex, but prioritizing readability ensures that others (or yourself in the future) can understand your logic quickly. Using comments effectively to explain complex logical conditions can prevent misunderstandings.
Optimization Tips
When using logical operators, especially when combined with larger data sets, consider performance implications. The `&&` operator can perform better due to its short-circuiting behavior. Moreover, try to minimize the number of evaluated conditions by ordering them from most likely to least likely to save processing time.
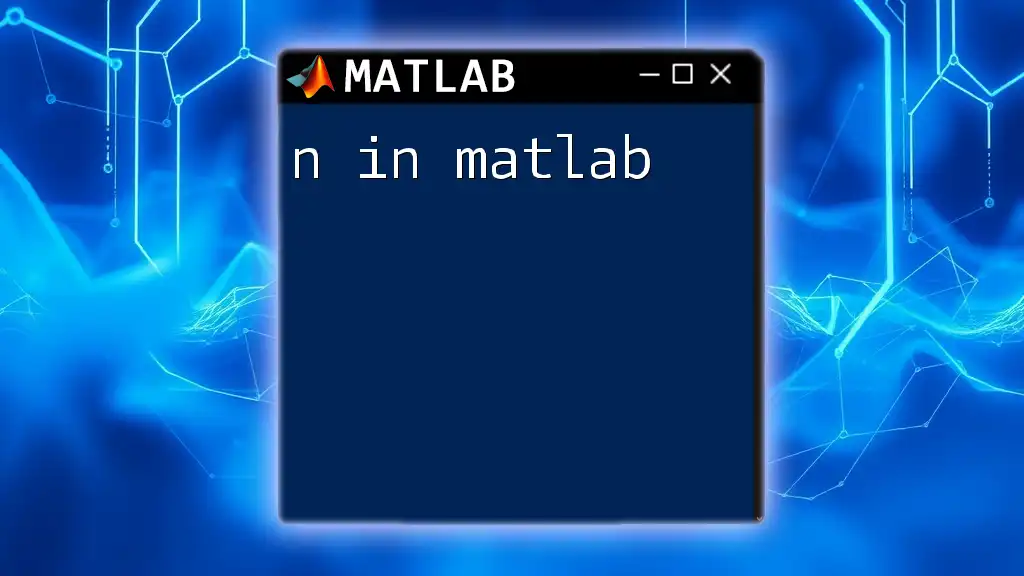
Conclusion
The AND operator in MATLAB is a powerful tool that enables effective decision-making in programming. Understanding how to properly implement and utilize `&` and `&&` will significantly enhance your programming capabilities. It is key to mastering logical operations and optimizing your code for performance and clarity. By experimenting with different scenarios, you can deepen your understanding and leverage the full potential of logical operations in your MATLAB projects.
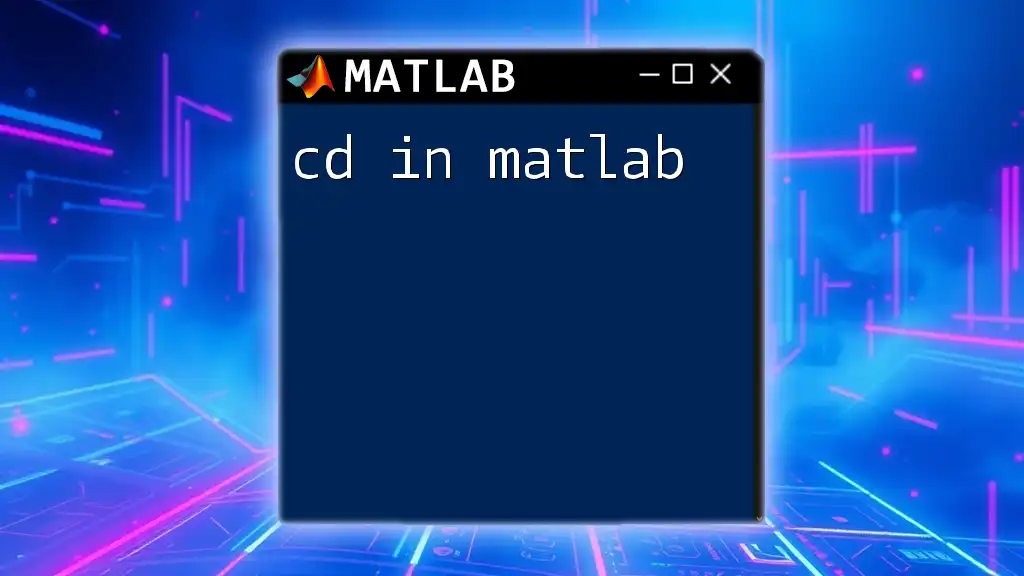
Additional Resources
For further study, explore MATLAB's official documentation on logical operations and delve into advanced tutorials that provide practical strategies for applying these concepts in various programming tasks.