In MATLAB, the `tf` command is used to create transfer function models, which are essential for control system analysis and design.
Here's a simple example of using the `tf` command:
% Create a transfer function H(s) = 1 / (s^2 + 3s + 2)
numerator = 1;
denominator = [1 3 2];
H = tf(numerator, denominator);
What is a Transfer Function?
A transfer function is a mathematical representation that describes the input-output relationship of a system in the Laplace domain. It is expressed as the ratio of two polynomials: the numerator represents the system's response while the denominator characterizes the system's dynamics.
In a transfer function, you can often see the following format:
G(s) = (N(s))/(D(s))
Where:
- N(s): The numerator polynomial
- D(s): The denominator polynomial
The poles and zeros of a transfer function are fundamental to understanding system behavior. The poles correspond to the values that make the denominator zero, indicating where the system tends to become unstable. In contrast, the zeros are the values that make the numerator zero, affecting the frequency response of the system.
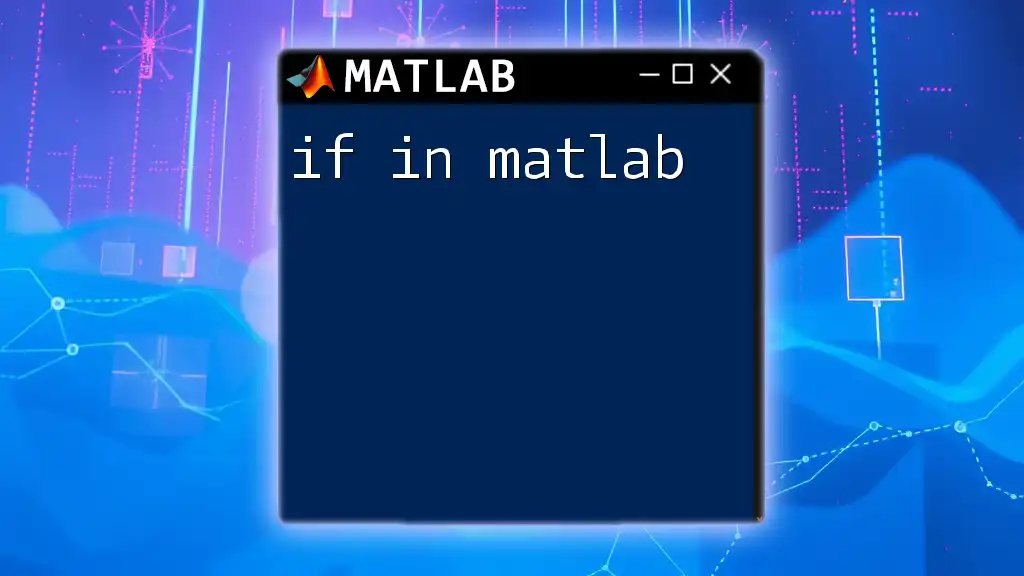
Getting Started with the tf Command in MATLAB
Syntax and Basic Usage
The `tf` command in MATLAB is straightforward and allows you to create transfer function models efficiently. The basic syntax is as follows:
G = tf(num, den);
Here, num is the vector containing the coefficients of the numerator polynomial, and den is the vector containing the coefficients of the denominator polynomial.
To create a simple transfer function, such as:
G(s) = 1 / (s^2 + 2s + 1)
You can use the following code:
G = tf([1], [1, 2, 1]);
This command generates a transfer function that MATLAB can use for further analysis.
Creating Transfer Functions with Arrays
You can create more complex transfer functions by using arrays for the numerator and denominator. For example, to represent a second-order system, you define the coefficients in vectors:
num = [1];
den = [1, 3, 2];
G = tf(num, den);
This creates the transfer function:
G(s) = 1 / (s^2 + 3s + 2)
Understanding Transfer Function Properties
Once you have created a transfer function, accessing its properties is quick and easy. For instance, if you want to inspect the numerator and denominator of your transfer function, you can use the following commands:
G.numerator % Displays numerator: [1]
G.denominator % Displays denominator: [1, 3, 2]
These commands help you understand the structure of your system and validate that you have set up your transfer function correctly.
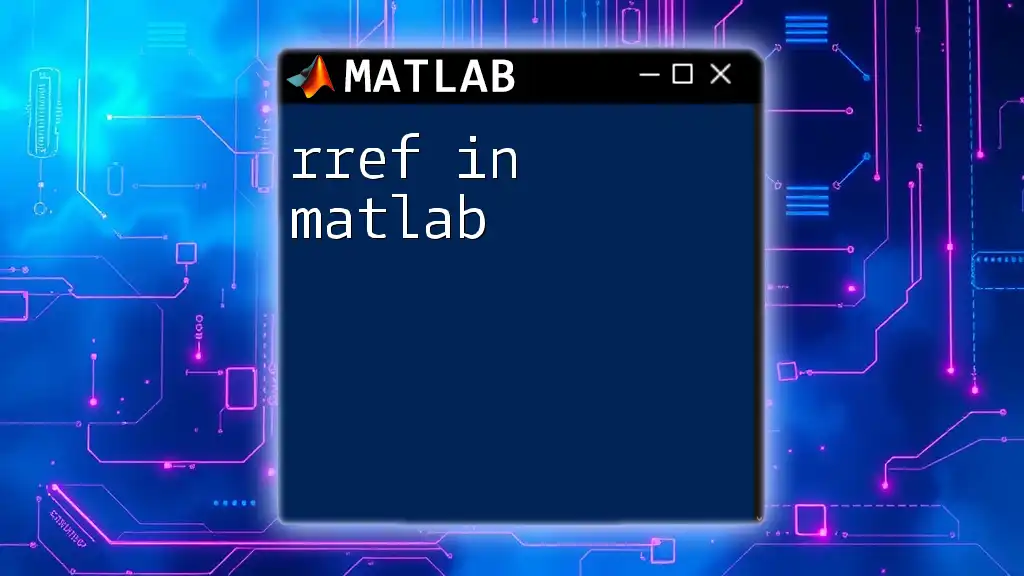
Advanced Features of the tf Command
Creating Transfer Functions with Multiple Inputs and Outputs
MATLAB's `tf` command also supports MIMO (Multiple Input Multiple Output) systems. This is essential when dealing with more complex systems, such as those used in robotics or advanced control systems.
To create a MIMO system, you can use the following:
G = tf([[1, 2], [3, 4]], [1, 5, 6]); % MIMO System
This code creates a transfer function with multiple input-output relationships.
Combining Transfer Functions
In control system design, you often need to combine transfer functions. You can combine them using basic arithmetic operations such as multiplication, addition, or division.
For example, to create a system that operates in parallel (sum of outputs), you can use:
G1 = tf([1], [1, 2]);
G2 = tf([1], [1, 4]);
G_total = G1 + G2; % Parallel
Conversely, for a series (cascade) connection, you would multiply the transfer functions:
G_total = G1 * G2; % Series
Combining transfer functions in this way is crucial for analyzing and designing control systems effectively.
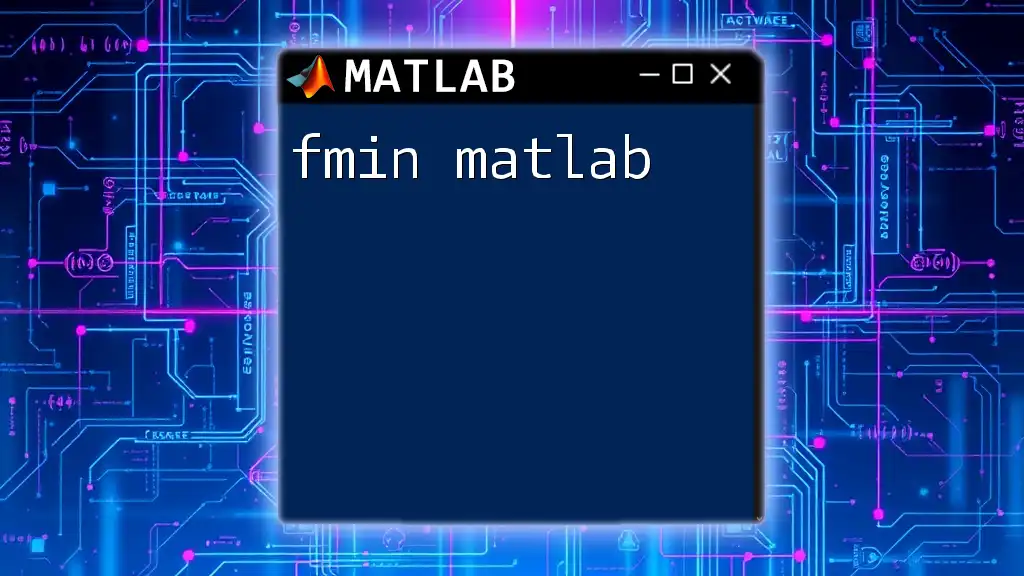
Analyzing Transfer Functions
Step Response Analysis
One of the most important analyses you can perform on a transfer function is the step response. The step response shows how the system reacts over time to a step input, offering insight into its stability and dynamic behavior.
To plot the step response of your transfer function, use:
step(G);
title('Step Response of G');
This command generates a plot that visually represents how the system responds to a sudden change in input, which is vital for understanding the system's performance.
Bode Plot Generation
Another crucial analysis technique is generating a Bode plot, which provides a frequency response of the system. The Bode plot consists of two graphs: one for magnitude and one for phase, both plotted against frequency (on a logarithmic scale).
You can generate a Bode plot for your transfer function using:
bode(G);
title('Bode Plot of G');
Interpreting the Bode plot helps engineers determine the stability, gain margins, and phase margins of the system, guiding adjustments during design.
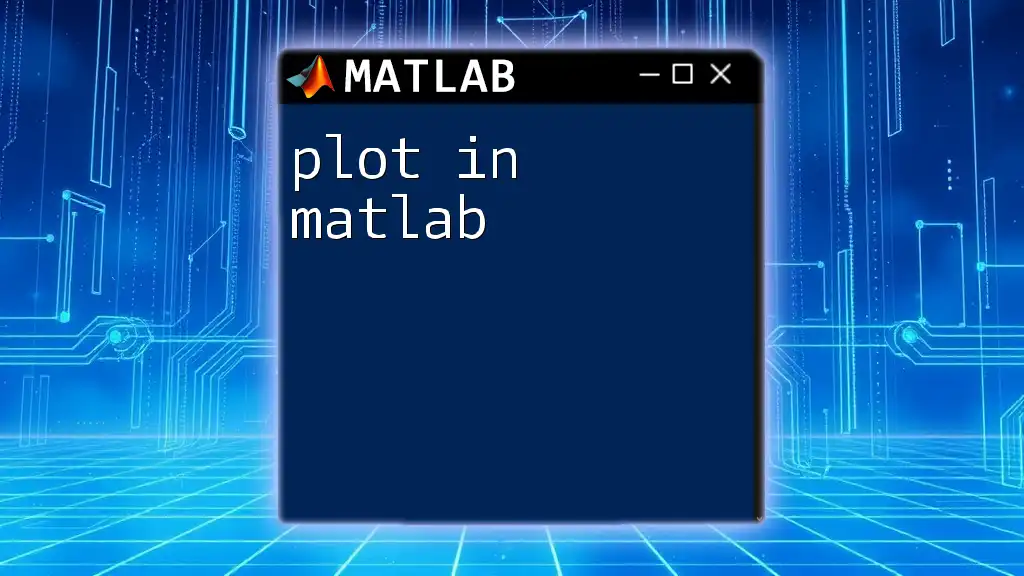
Common Issues and Troubleshooting
Common Errors with tf Command
While working with the `tf` command, you might encounter common errors related to vector sizes. For instance, if the numerator and denominator arrays do not match the expected dimensions or degrees, you may receive error messages.
Carefully check to ensure that the length of the `num` array is equal to the degree of the polynomial plus one (interpreted as the highest power of s), and the same for the `den` array.
Debugging Transfer Function Models
If you suspect your transfer function might be incorrect or unstable, you can debug it by validating its properties. One useful command is:
pole(G); % Check poles for stability
Examining the poles helps determine stability; if any poles have positive real parts, the system is unstable. To ensure reliable performance, refining your transfer function in response to such findings is essential.
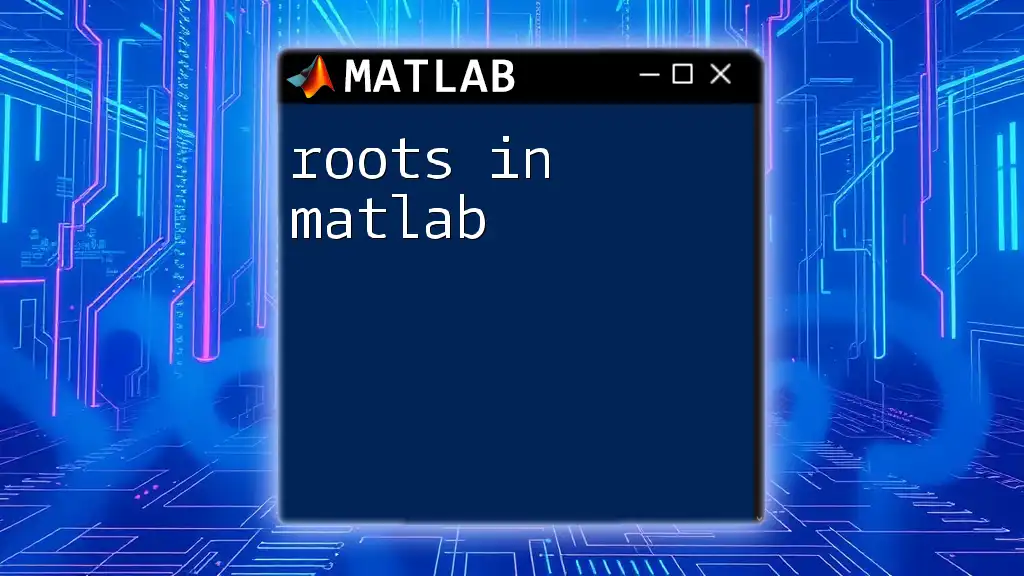
Conclusion
Mastering the use of `tf` in MATLAB is crucial for professionals involved in control systems and engineering applications. This command enables you to succinctly define and analyze transfer functions, gaining insights into system stability and performance.
By practicing how to build, manipulate, and analyze transfer functions in MATLAB, you position yourself to tackle complex engineering challenges effectively. Embrace the process, experiment with different configurations, and expand your skill set in control system design!
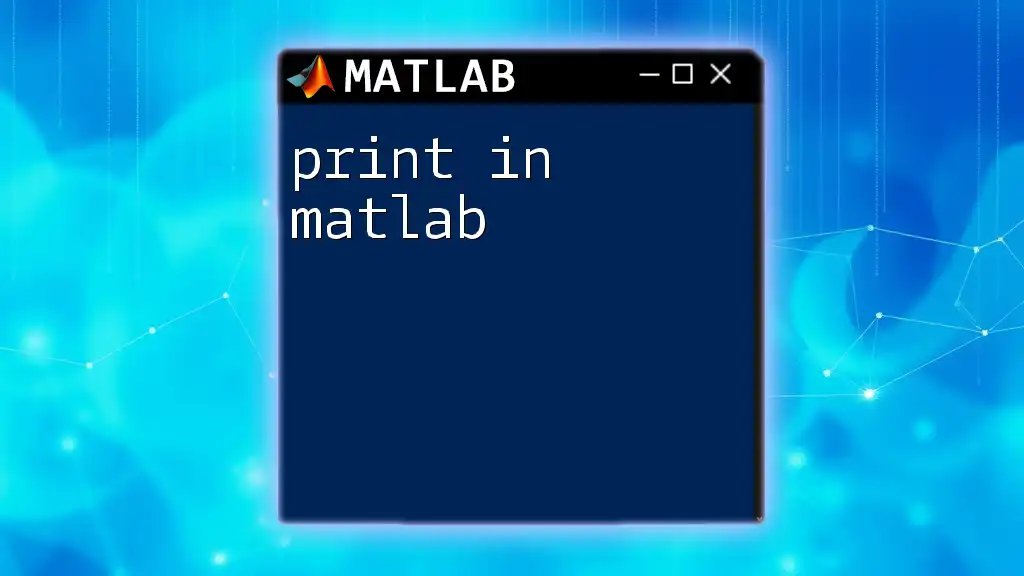
Additional Resources
To enhance your understanding further, consider exploring MATLAB's official documentation on the `tf` command, control system toolbox, and relevant textbooks on control theory. These resources will provide you with a solid foundation to deepen your knowledge and skills.