The "if-then-else" structure in MATLAB allows you to execute specific code blocks based on whether a condition is true or false.
x = 10;
if x > 5
disp('x is greater than 5');
else
disp('x is 5 or less');
end
Understanding Conditional Statements
What are Conditional Statements?
Conditional statements are fundamental constructs in programming that allow for decision-making processes within your code. They enable your program to execute different code paths based on certain conditions being evaluated as true or false. In other words, conditional statements help your code determine what to do based on varying inputs or states during runtime.
Syntax of If Then Else in MATLAB
The basic structure for implementing conditional logic in MATLAB revolves around the `if then else` statement. The syntax is straightforward and can be summarized as follows:
if condition
% Code to execute if condition is true
elseif another_condition
% Code to execute if another_condition is true
else
% Code to execute if none of the conditions are true
end
By adhering to this syntax, you can shape how your program behaves under different conditions, enabling complex logic flows.
When to Use If Then Else Statements
Common Use Cases
If then else statements are commonly utilized for a variety of scenarios, such as:
- User input validation: Ensuring that inputs meet specific criteria before proceeding.
- Decision-making processes: Choosing between multiple code paths based on calculated values.
- Error handling: Implementing ways to deal with unexpected conditions.
Best Practices for Writing Conditions
When crafting your conditional statements, it is crucial to make your conditions clear and concise. Here are some best practices:
- Keep conditions simple and understandable: Complicated conditions can confuse readers and increase the chance of errors. Always aim for clarity.
- Avoid deeply nested if statements: Although nesting can be useful, excessive nesting may lead to complex code that's hard to follow. Consider refactoring to maintain readability.
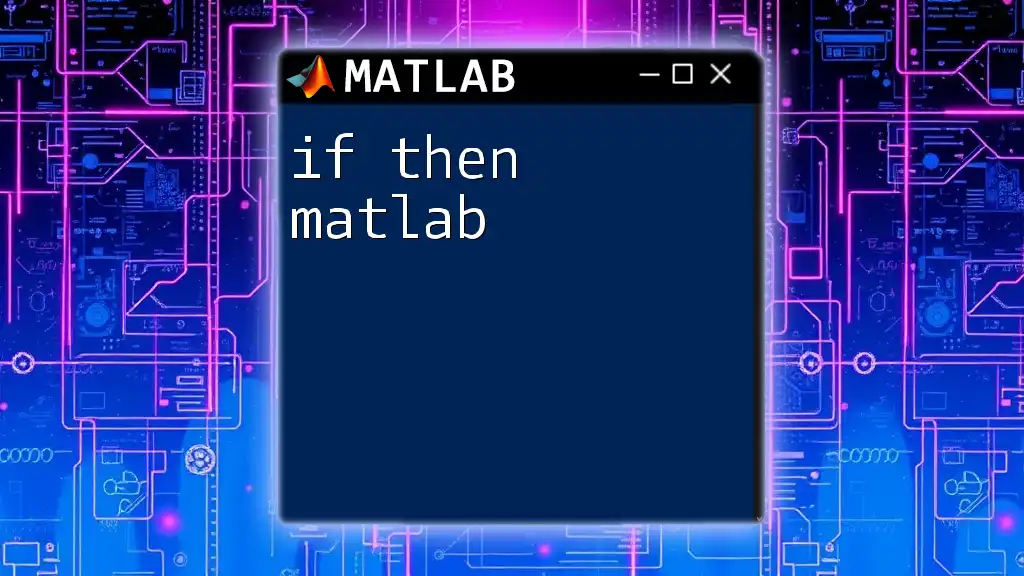
Examples of If Then Else in MATLAB
Simple Example
Let's look at a basic example to understand how the if then else statement functions in practice:
x = 5;
if x > 0
disp('x is positive');
else
disp('x is non-positive');
end
In this code, we declare a variable `x` and check if it is greater than zero. If the condition evaluates to true, the program displays "x is positive." If false, it outputs "x is non-positive." This clear and concise format demonstrates how effectively the if then else structure can dictate program flow.
Using Elseif for Multiple Conditions
To handle multiple conditions more elegantly, the `elseif` statement can be added. Consider the following example:
myScore = 85;
if myScore >= 90
grade = 'A';
elseif myScore >= 80
grade = 'B';
elseif myScore >= 70
grade = 'C';
else
grade = 'D or F';
end
fprintf('Your grade is: %s\n', grade);
Here, we evaluate `myScore` against several thresholds to determine the corresponding grade. The use of `elseif` provides a seamless way to manage multiple conditions while ensuring that only the appropriate block of code is executed.
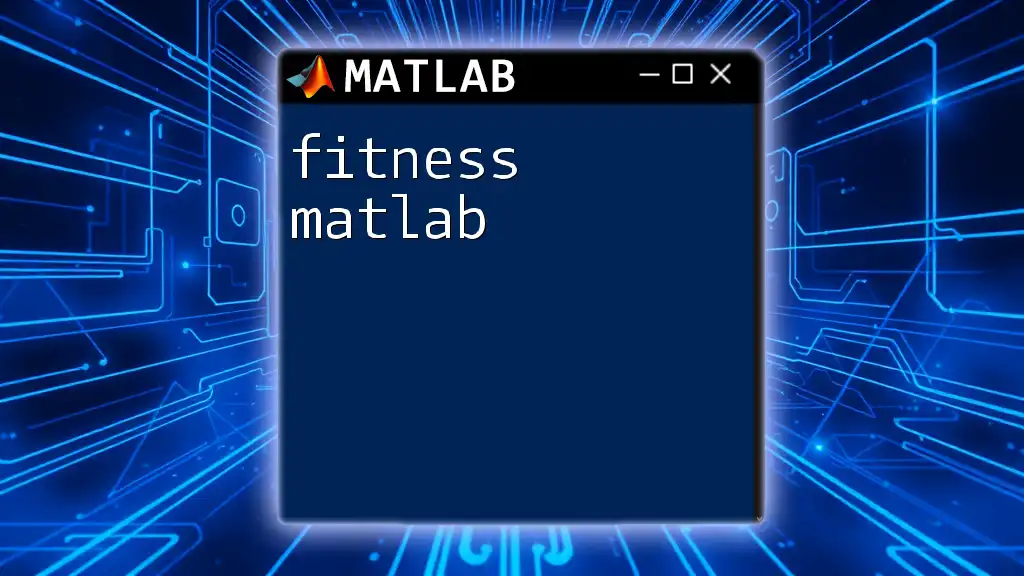
Nested If Then Else Statements
What are Nested Statements?
Nested if statements occur when an if statement is contained within another if statement. While this allows for greater specificity and control, it should be used judiciously to avoid complexity.
Code Example
Here's a demonstration of how nested statements work:
num = 10;
if num > 0
if mod(num, 2) == 0
disp('Number is positive and even');
else
disp('Number is positive and odd');
end
else
disp('Number is non-positive');
end
In this example, we first check if `num` is positive. If it is, we then check if it is even or odd. This layering of conditions can help fine-tune the behavior of your code based on various criteria.
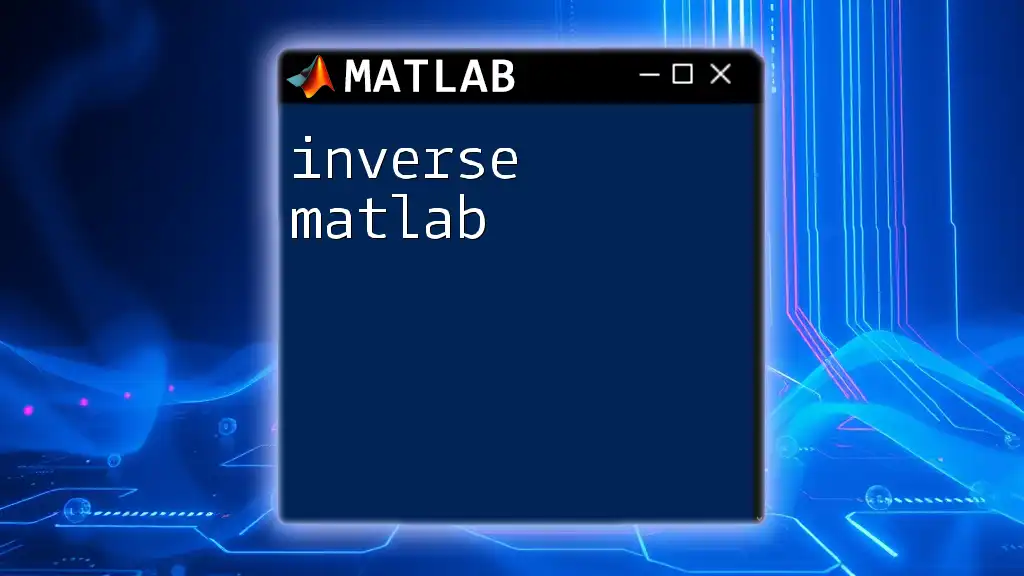
Logical Operators with If Then Else
Using AND, OR, and NOT Operators
MATLAB allows for the use of logical operators in your conditions, which can expand your decision-making capabilities:
- AND (`&&`): Returns true if both conditions are true.
- OR (`||`): Returns true if at least one condition is true.
- NOT (`~`): Inverts the truth value of the condition.
Example of Logical Operators in Conditions
Let’s see how you can incorporate logical operators into your conditional statements:
age = 25;
hasPermit = true;
if age >= 18 && hasPermit
disp('You can drive.');
else
disp('You cannot drive.');
end
In this example, we are checking two conditions: whether the `age` is at least 18 and whether the person has a driving permit. Using the AND operator, both conditions must be true for the function to indicate that the person can drive.
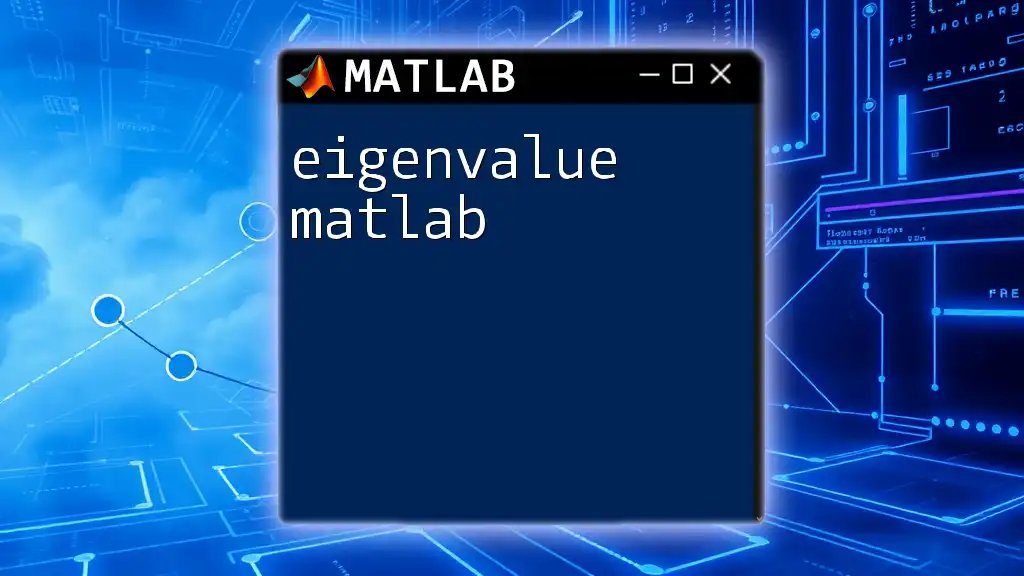
Conclusion
The if then else statement in MATLAB is a powerful tool for steering the flow of execution based on conditions. By mastering this feature, you can write clear, efficient, and dynamic code that reacts to the state of variables or the results of computations.
Engaging with conditional logic enhances your programming skills, allowing you to build interactive applications and perform complex decision-making tasks. Don't hesitate to experiment with the provided examples to see how they can be adapted to fit your needs!
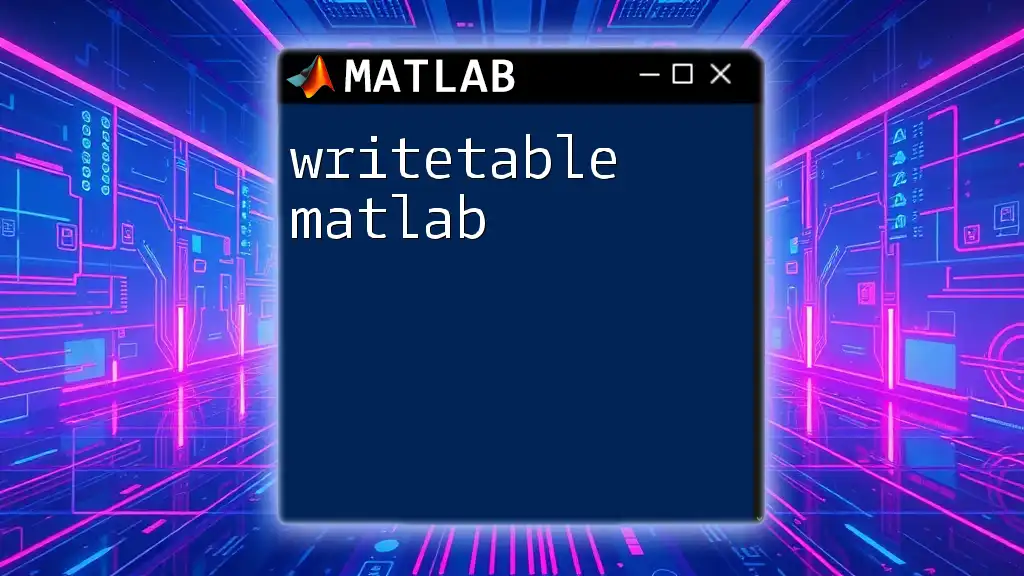
Call to Action
We invite you to share your experiences or queries related to using if then else statements in MATLAB. Engaging in discussions or seeking clarification can significantly bolster your understanding.
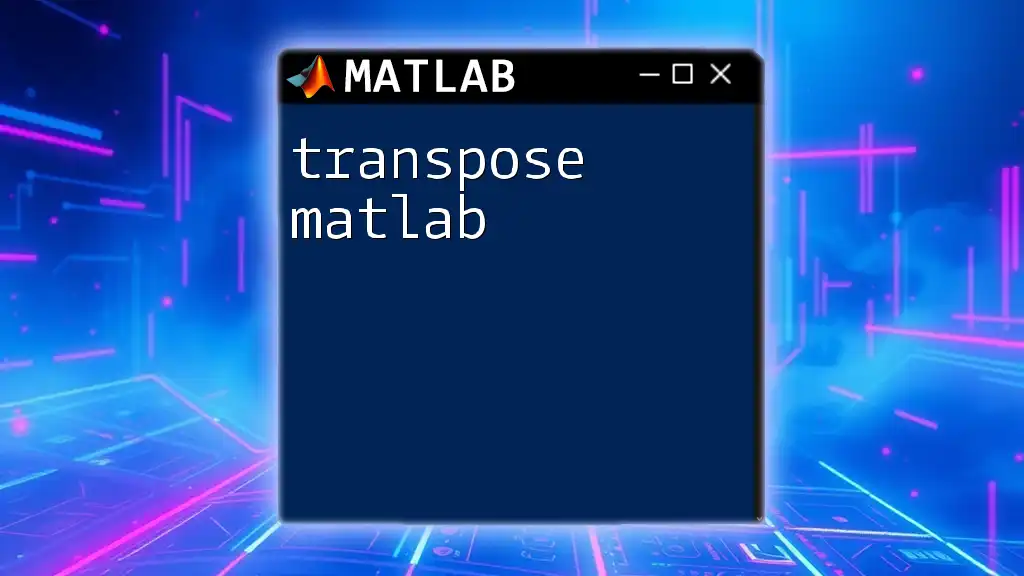
Additional Resources
For further readings, check the official MATLAB documentation on conditional statements, and look for recommended courses that delve deeper into MATLAB programming. Empower yourself with the knowledge necessary to wield the power of if then else in MATLAB!