To import data into MATLAB, you can use the `readtable` function for CSV files, enabling quick access to your dataset as a table variable.
data = readtable('your_data_file.csv');
Understanding Data Formats
Common Data Formats play a crucial role in the process of importing your data into MATLAB. Knowing the different types of files and how to work with them can significantly enhance your data analysis efficiency.
Text Files (.txt, .csv)
Text files, including .txt and .csv formats, are among the most common ways to store data. These files store data in a plain text format, which makes them easy to read and write along with simple editing. The comma-separated format (CSV) is particularly popular for tabular data because it easily separates values with commas.
Excel Files (.xls, .xlsx)
Excel files are widely used for data storage and analysis across various fields. They allow for complex manipulations of data and support multiple sheets within a single file. The ability to format and visually present data makes Excel a preferred choice for many users.
MAT-files (.mat)
MAT-files are special binary files used by MATLAB to store variables, arrays, and structures efficiently. They are designed specifically to work seamlessly with MATLAB, allowing for quick loading and saving of data. This format is often used to save large datasets or complex structures without the overhead of text file parsing.
Database Connections (SQL, NoSQL)
For larger datasets, connecting directly to databases—both SQL and NoSQL—can become necessary. MATLAB supports robust connections to databases, allowing users to retrieve relevant data dynamically without loading entire files.
Other Formats
Other file formats such as JSON and XML can also be handled in MATLAB, especially for data interchange between web applications. These formats are beneficial when working with APIs or rapid data exchange.
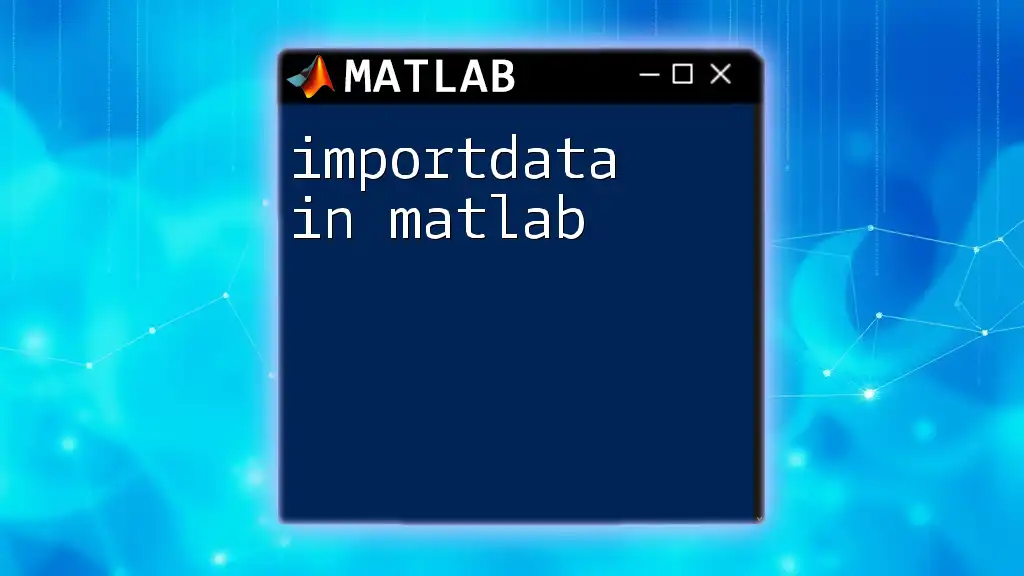
Importing Data into MATLAB
Using Built-in Functions
MATLAB provides several built-in functions for importing your data into MATLAB with ease.
`readtable` for Tabular Data
The `readtable` function is designed for reading tabular data from files like .csv and .txt. This function automatically detects variable names and data types, making it a flexible choice for most data types.
Example:
data = readtable('datafile.csv');
After running this command, the variable `data` contains a table where each column corresponds to a variable from the CSV file.
`load` for MAT-files
For users dealing with MAT-files, the `load` function offers a straightforward way to load data. This function reads binary data directly into MATLAB variables.
Example:
load('datafile.mat');
This command loads all variables from the MAT-file directly into the current workspace, preserving variable names.
`xlsread` for Excel Files
The `xlsread` function is particularly useful for importing data from Excel files. It can read numeric data, text, and raw data, although users should note that newer versions of MATLAB recommend using `readtable` for Excel imports.
Example:
[num, txt, raw] = xlsread('datafile.xlsx');
In this example, `num` contains numeric data, `txt` holds text, and `raw` contains all data without specifying types.
Import Tool: A Visual Approach
The Import Tool in MATLAB offers a user-friendly way to import data visually.
- Step-by-Step Guide:
- Initiating the Tool: You can launch the Import Tool from the Home tab or by using the command `uiimport`.
- Selecting Files: Browse to select your data file.
- Data Preview and Options: The tool generates a preview, allowing you to specify delimiters, variable names, and data types before importing.
- Finalizing the Import: Once satisfied, you can import the data into your workspace.
This approach is particularly helpful for users who prefer visual interactions or are unfamiliar with command-line functions.
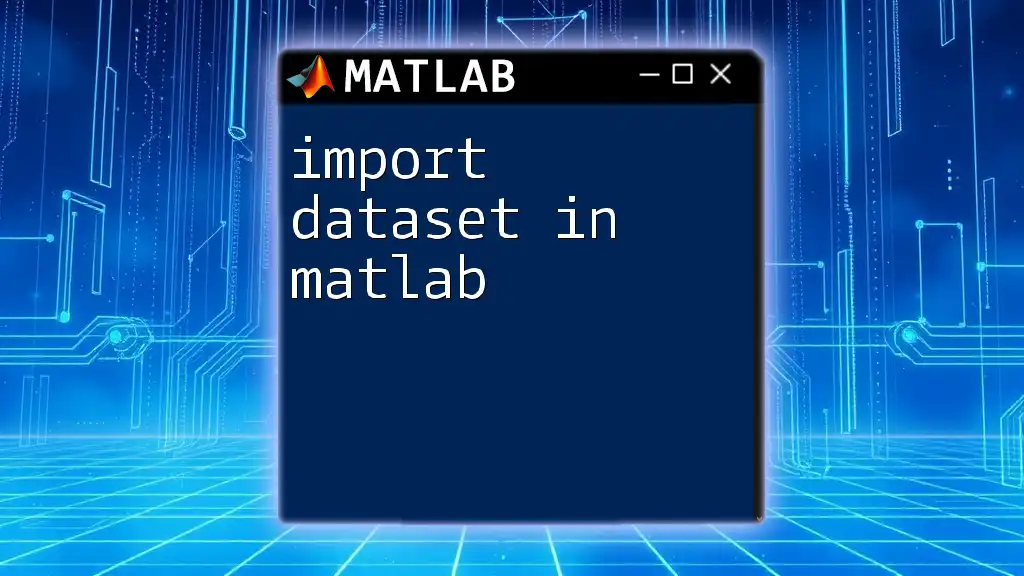
Advanced Data Import Techniques
Importing from Databases
Using the Database Toolbox, you can connect to SQL databases to retrieve data directly. This method is beneficial for dynamically accessing updates from large datasets without needlessly moving huge amounts of data locally.
Example:
conn = database('dbname', 'username', 'password');
data = fetch(conn, 'SELECT * FROM tablename');
close(conn);
In this code snippet, a connection is established with the database, and data is fetched based on a SQL query. Always remember to close the connection to free resources.
Accessing RESTful APIs for Data
For modern web-based projects, accessing data from RESTful APIs may enhance your data richness. MATLAB allows you to use web functions to pull data from APIs seamlessly, enabling innovative data analysis solutions.
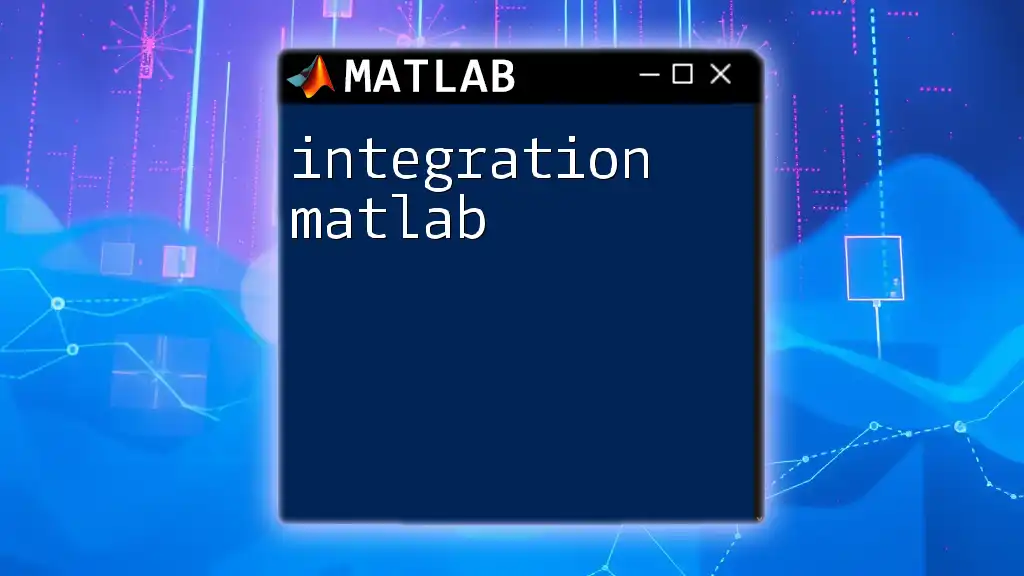
Troubleshooting Common Import Issues
Handling Missing Data
When importing your data into MATLAB, one frequent issue is dealing with missing values. MATLAB represents missing data as NaN (Not a Number). Users can specify how to treat missing values upon import.
Example:
data = readtable('datafile.csv', 'TreatAsEmpty', {'', 'NA'});
In this case, the command instructs MATLAB to treat blank entries or "NA" values as missing during the import process.
Data Parsing Errors
Common parsing issues may arise due to mismatched delimiters, incorrect column data types, or even incompatible header formats. To validate your imports, you can check the dimensions and types of your data after importing.
Using commands like `size(data)` or `class(data)` can help troubleshoot any potential inconsistencies and ensure that the data is imported correctly.
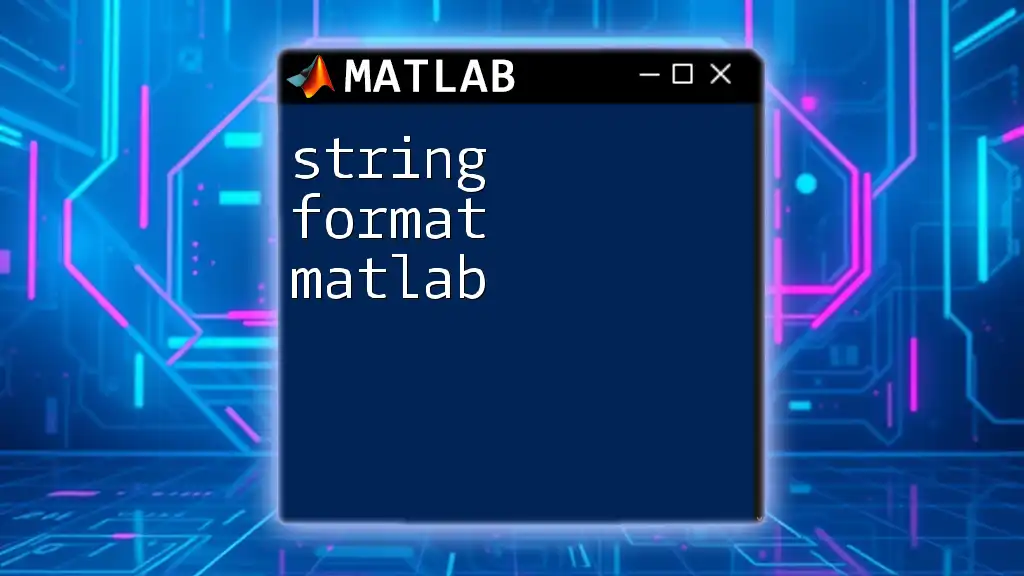
Conclusion
In summary, mastering the process of importing your data into MATLAB can greatly enhance your efficiency and capability in data analysis. With various methods ranging from built-in functions to visual tools and database connections, MATLAB offers a flexible suite to accommodate all data types.
Don't hesitate to experiment with different methods and discover what works best for your specific use cases. For those eager to delve deeper, MATLAB's robust documentation and community resources are invaluable for expanding your skills.