In this post, you'll learn how to plot points in MATLAB using the `plot` function to visually represent data on a 2D graph.
x = [1, 2, 3, 4, 5]; % X-coordinates of the points
y = [2, 3, 5, 4, 6]; % Y-coordinates of the points
plot(x, y, 'o'); % Plot the points with circle markers
xlabel('X-axis'); % Label for the x-axis
ylabel('Y-axis'); % Label for the y-axis
title('Plotting Points in MATLAB'); % Title of the plot
grid on; % Turn on the grid for better visibility
Understanding MATLAB Plotting Basics
What is Plotting in MATLAB?
Plotting in MATLAB is a fundamental skill for analyzing data visually. It allows users to represent mathematical functions, datasets, and simulation results graphically. Learning how to effectively plot points can enhance data interpretation and provide insights that raw numbers may obscure.
MATLAB offers flexibility to create various plot types, with 2D and 3D plotting being the most common. While 2D plots are primarily used for simpler visualizations, 3D plots provide a more comprehensive view of datasets that contain three variables.
MATLAB Plotting Functions Overview
MATLAB provides several built-in functions for plotting, each suited for different scenarios:
- `plot`: The most commonly used function for 2D line plots.
- `scatter`: Ideal for creating scattered point diagrams, allowing customization of marker sizes and colors.
- `hold on`: Used to add multiple plots to the same figure without erasing previous plots.
Understanding when and how to use these functions can significantly affect the clarity and effectiveness of your visualizations.
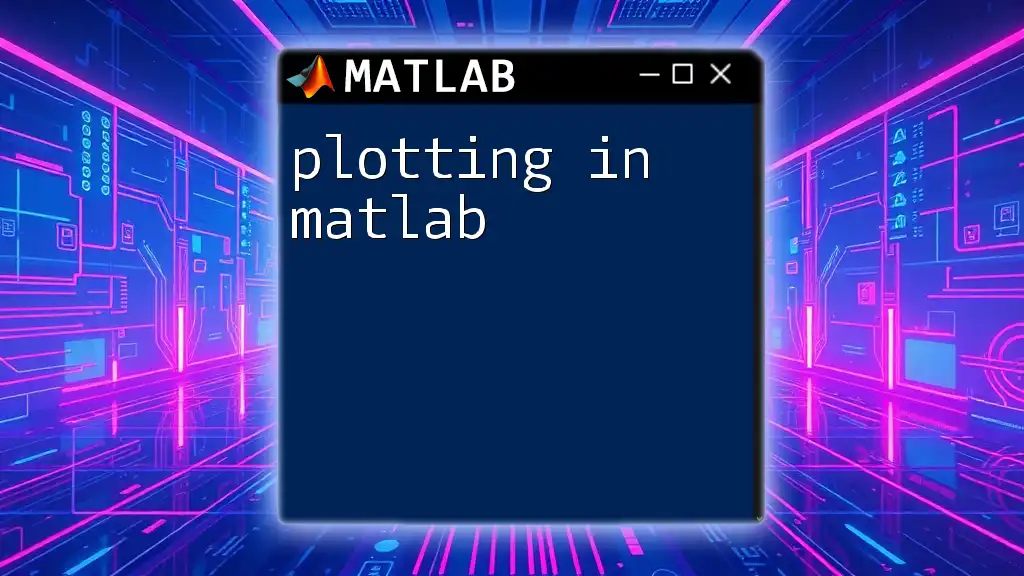
Setting Up Your MATLAB Environment
Downloading and Installing MATLAB
Before diving into plotting points on MATLAB, you need to have MATLAB installed. You can download it via the official MathWorks website. A trial version is available for newcomers, allowing exploration without a long-term commitment.
Creating a New Script
Once MATLAB is installed, creating a new script is straightforward. Open MATLAB, click on New Script in the home tab, and start writing your code. It’s a good practice to save your script regularly to avoid losing changes. After completion, use the Run button to execute your commands and visualize your outputs.
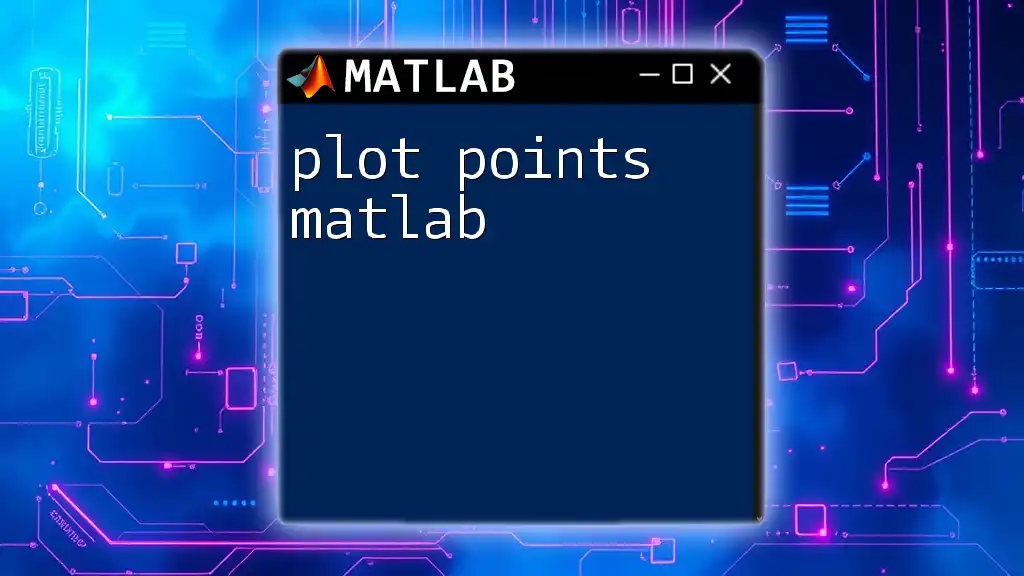
Plotting Points in MATLAB
Basic 2D Plotting with the `plot()` Function
One of the simplest methods for plotting points on MATLAB is by utilizing the `plot` function. It plots data points in a 2D space defined by x and y coordinates.
Here's a basic example showcasing how to create a simple plot:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 1, 4];
plot(x, y, 'o-'); % 'o-' indicates circular markers joined by lines
In this example, `x` represents the horizontal axis, while `y` stands for the vertical axis. The `'o-'` specifies circular markers connected by lines, providing a clear representation of the data points.
Customizing Point Styles
Customization enhances the readability and appeal of your plots. MATLAB allows you to define different marker types and line styles that can be tailored to your dataset.
For instance, you can customize the marker and line style by utilizing the following code snippet:
plot(x, y, 's--r'); % Square markers with dashed red lines
In this example:
- The `'s'` denotes square markers.
- The `'--'` specifies a dashed line style.
- The `'r'` indicates the color red.
Being able to adjust these styles enables you to create visually distinct plots even when working with multiple datasets.
Adding Titles and Labels
Providing context to your plots is crucial. Titles and axis labels make it easier for your audience to understand what each axis represents. Use the following commands to add descriptive titles and labels to your plot:
title('Sample Data Points');
xlabel('X-axis Label');
ylabel('Y-axis Label');
Adding a title helps viewers grasp the essence of the graph, while axis labels clarify what measurements or variables are being represented. This is particularly important in professional and academic contexts, where precise interpretations are essential.
Adding a Legend
When working with multiple datasets in a single plot, adding a legend can guide viewers and improve clarity. The legend indicates what each data series represents, reducing ambiguity.
To add a legend, use the following command:
legend('Data Series 1');
This can be particularly useful when you have several lines or data points representing different categories, helping to distinguish between them effortlessly.
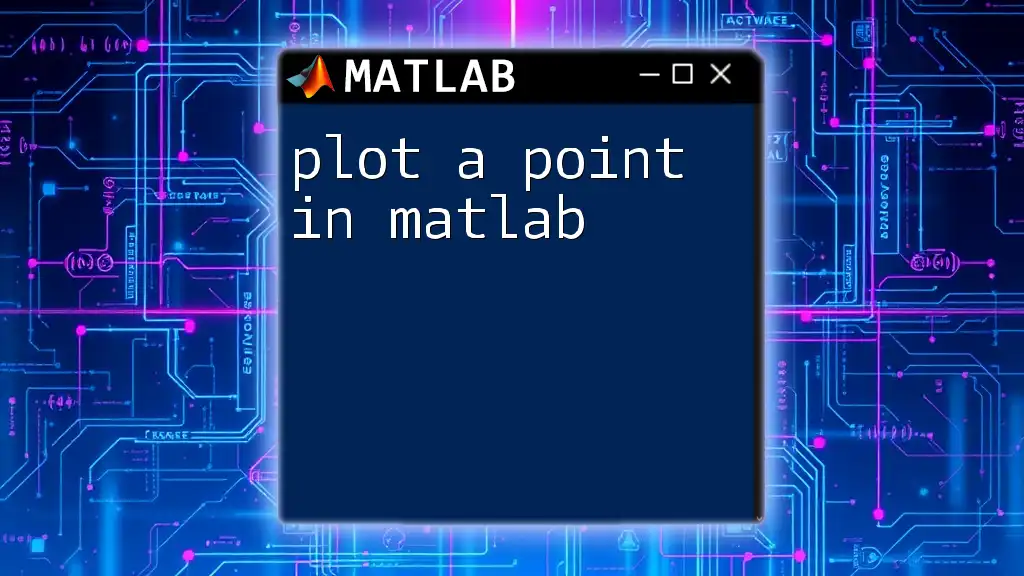
Exploring Advanced Plotting Methods
Using the `scatter()` Function
For datasets where displaying each individual point's relationship is essential, utilize the `scatter` function. Unlike the `plot` function, `scatter` allows you to control the size and color of the markers, offering a richer representation of the data.
Here’s an example:
scatter(x, y, 100, 'filled', 'MarkerEdgeColor', 'k'); % Scatters filled circles
In this code:
- The `100` represents the sizes of the markers.
- The `'filled'` command indicates that the circles are solid.
- The `MarkerEdgeColor` parameter defines the color of the edges.
Using `scatter` is advantageous when you want to emphasize the distribution of points rather than connecting them with a line.
3D Plotting with `plot3()`
When analyzing datasets with three variables, 3D plotting becomes essential. The `plot3` function enables you to visualize relationships in three dimensions, providing insight that isn’t apparent in 2D plots.
An example of a 3D plot is as follows:
z = [1, 4, 9, 16, 25]; % Example Z data
plot3(x, y, z, 'o');
In this example, `x`, `y`, and `z` represent three dimensions. The `plot3` function creates a 3D scatter, representing each point in a three-dimensional space.
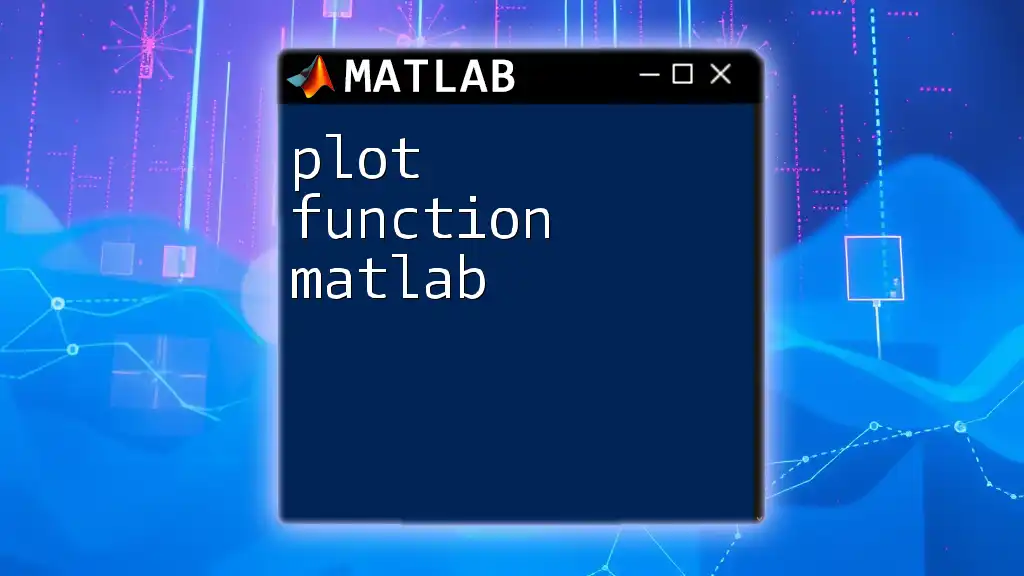
Advanced Customization Techniques
Adding Annotations
Annotations help highlight specific areas or data points on your plot, drawing attention to essential elements. Use the `text` function for this purpose.
Here's how you can annotate a critical point:
text(3, 5, 'Important Point', 'FontSize', 12);
Adding annotations not only makes your graphs more informative but also engages the viewer by directing their focus to areas of interest.
Customizing Axes and Grids
To enhance data readability, adjusting axis limits and activating grid lines can be beneficial. Use the `xlim` and `ylim` commands to set the axis ranges:
xlim([0 6]);
ylim([0 6]);
grid on; % Activating grid lines
Setting appropriate limits can prevent data from feeling cramped and allow for better visual interpretation. Grid lines serve as a helpful reference for estimating values in the plot.
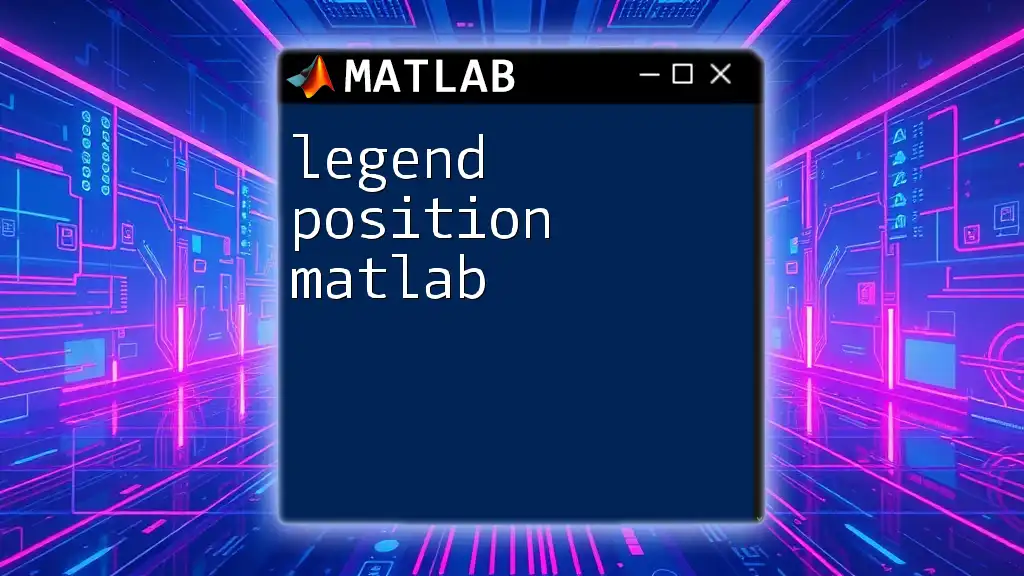
Practical Applications and Examples
Real-World Scenarios
Effective plotting is foundational across various fields like engineering, finance, and academia. For instance:
- Engineering often relies on plotting stress-strain curves to analyze material behavior.
- Finance utilizes line graphs to track stock performance over time.
- Academia employs scatter plots to visualize correlations between two variables, enhancing research findings.
Understanding practical applications can help you visualize how mastering the skills of plotting points on MATLAB can directly impact work in various domains.
User Challenges and Solutions
As you learn to plot points, you may encounter common mistakes such as misconceptions about data ranges or difficulty with legend entries. Here are some solutions:
- Check your input data: Ensuring your data vectors are correctly formatted is essential.
- Utilize MATLAB’s help feature: Typing `doc function_name` in the command window will provide detailed documentation on any plotting function.
- Experiment with plot settings: Don’t hesitate to modify marker types, line styles, or colors to see which combinations work best.
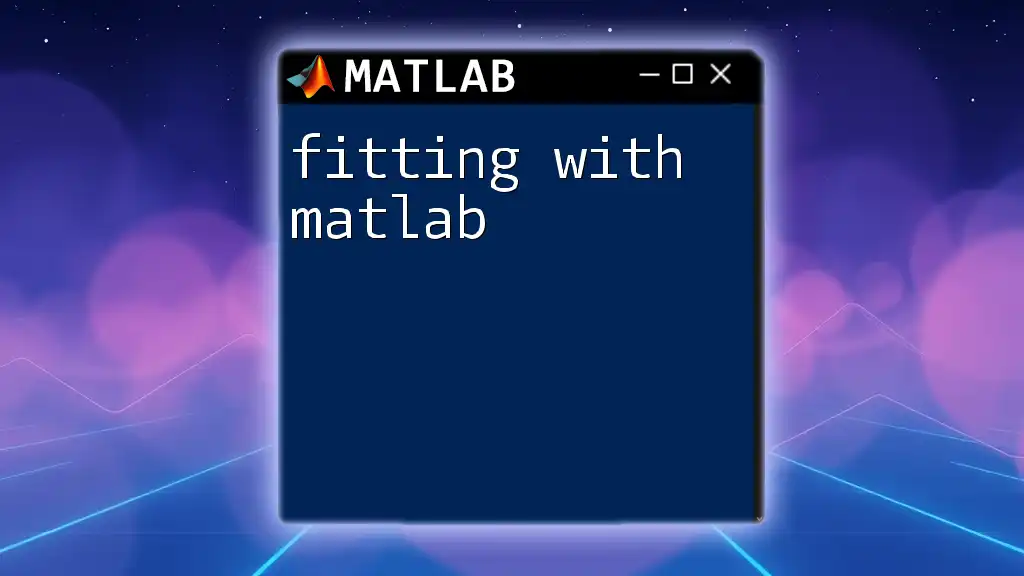
Conclusion
Understanding how to effectively plot points on MATLAB is a crucial skill that enables clearer data presentation and analysis. From basic 2D plots to advanced 3D visualizations, MATLAB provides diverse tools for enhancing data communication. By employing strategic techniques for customizing styles, utilizing annotations, and managing axis properties, you can create compelling visualizations that resonate with your audience.
Mastering these skills empowers you to share insights and findings effectively across various fields of study and industries.
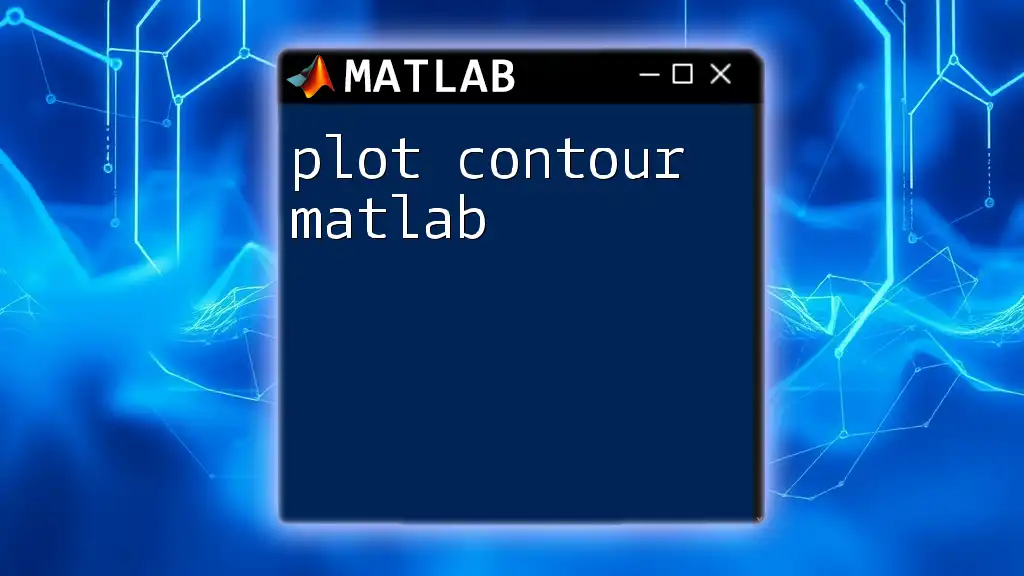
Further Resources
For additional learning, consider exploring online tutorials, MATLAB Central, and community forums. Engaging with these resources can deepen your understanding and proficiency in plotting points on MATLAB.
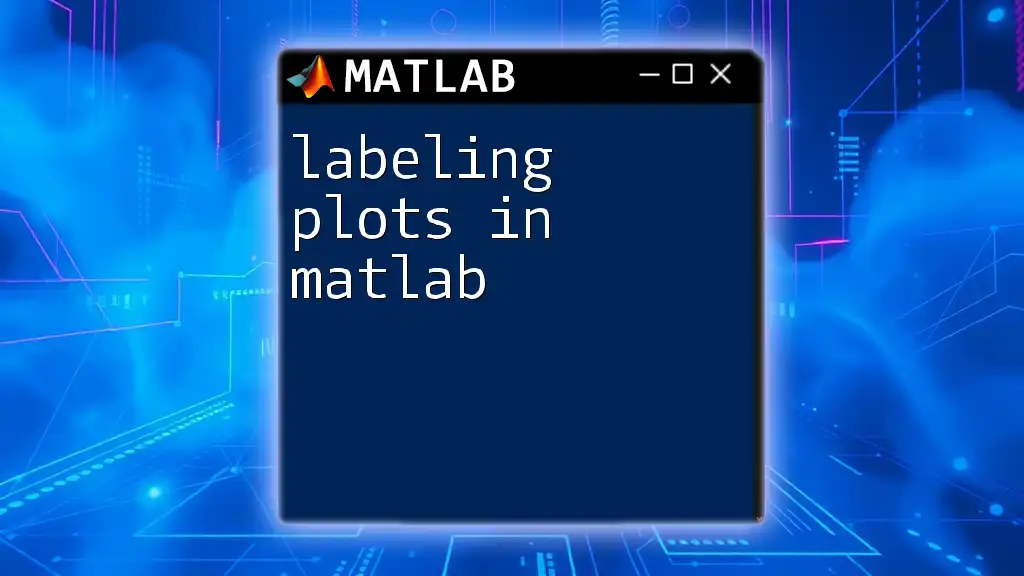
Call to Action
If you want to dive deeper into MATLAB capabilities and enhance your skills through hands-on experience, join our comprehensive training program! Visit our website to explore courses designed for various skill levels and keep getting better at using MATLAB efficiently.