Bode plots are graphical representations of a system's frequency response, which can be easily created in MATLAB using the `bode` command applied to a transfer function model.
Here's a simple example code snippet to plot a Bode plot in MATLAB:
sys = tf([1], [1, 2, 1]); % Define a transfer function (numerator, denominator)
bode(sys); % Generate the Bode plot
grid on; % Add a grid for better readability
Understanding Bode Plots
Definition and Components
Bode plots are graphical representations of a system's frequency response, primarily used in control theory and signal processing. A Bode plot consists of two main components:
-
Bode Magnitude Plot: Displays the gain of the system in decibels (dB) as a function of frequency (usually represented on a logarithmic scale).
-
Bode Phase Plot: Shows the phase shift introduced by the system as a function of frequency.
These plots help in assessing how a system responds to different input frequencies, ultimately revealing insights into its stability and dynamic behavior.
Applications of Bode Plots
Bode plots are used extensively in various fields, including:
-
Control System Stability Analysis: Engineers use Bode plots to evaluate the stability margins of systems, helping to determine whether a system will behave predictably under various conditions.
-
Frequency Response Analysis: Bode plots allow for a visual assessment of how a system will respond to sinusoidal inputs of varying frequencies, important for designing filters and controllers.
-
System Design and Tuning: By analyzing Bode plots, engineers can adjust system parameters to achieve desired performance characteristics, making them a vital tool for system optimization.
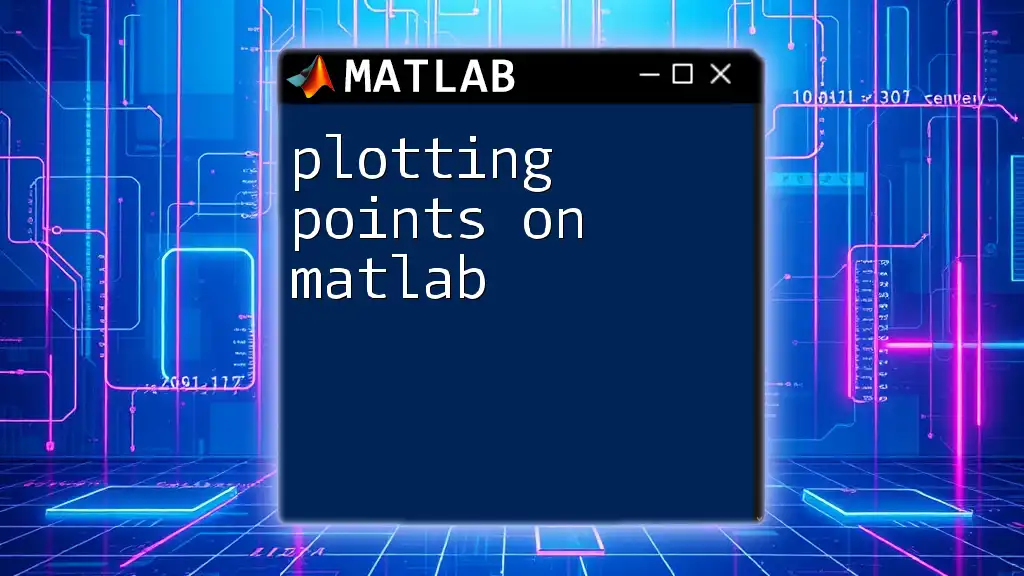
Getting Started with MATLAB
Setting Up MATLAB Environment
Before diving into plotting Bode plots in MATLAB, it's essential to ensure that you have the appropriate environment set up. Start MATLAB and create a new script where you will write your commands. The Control System Toolbox is highly recommended for working with Bode plots, as it contains functions specifically intended for this purpose.
Basic MATLAB Command Structure
MATLAB uses a straightforward command structure. Familiarizing yourself with this syntax will streamline your experience. Here’s a simple example of creating a variable:
x = 5; % Assigns the value 5 to the variable x.
This basic syntax allows for the manipulation and analysis of data efficiently.
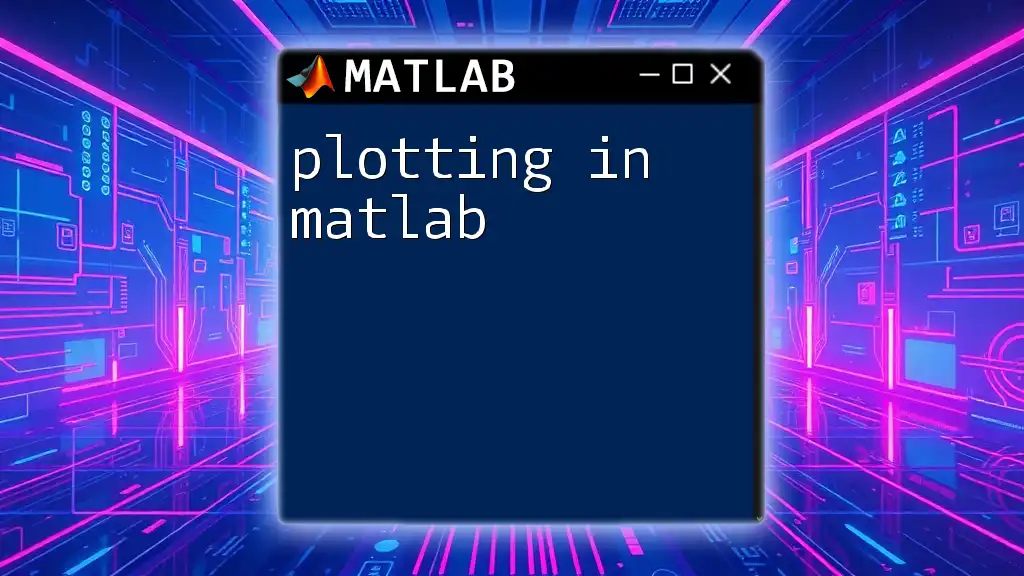
Creating a Transfer Function in MATLAB
Definition of Transfer Function
A transfer function is a mathematical representation of a system that relates the output to the input in the Laplace domain. It characterizes the dynamics of the system and can be expressed as a ratio of polynomials.
How to Create Transfer Functions
To create a transfer function in MATLAB, you can use the `tf` command. Here’s how to define a simple transfer function:
% Example transfer function: G(s) = 1 / (s^2 + 3s + 2)
num = [1]; % Numerator coefficients
den = [1 3 2]; % Denominator coefficients
G = tf(num, den); % Creating the transfer function G
In this example, `G` represents the transfer function \( G(s) = \frac{1}{s^2 + 3s + 2} \).
Visualizing the Transfer Function
To visualize your transfer function, you can simply type the variable name into the command window:
G
This command will display the transfer function's formula.
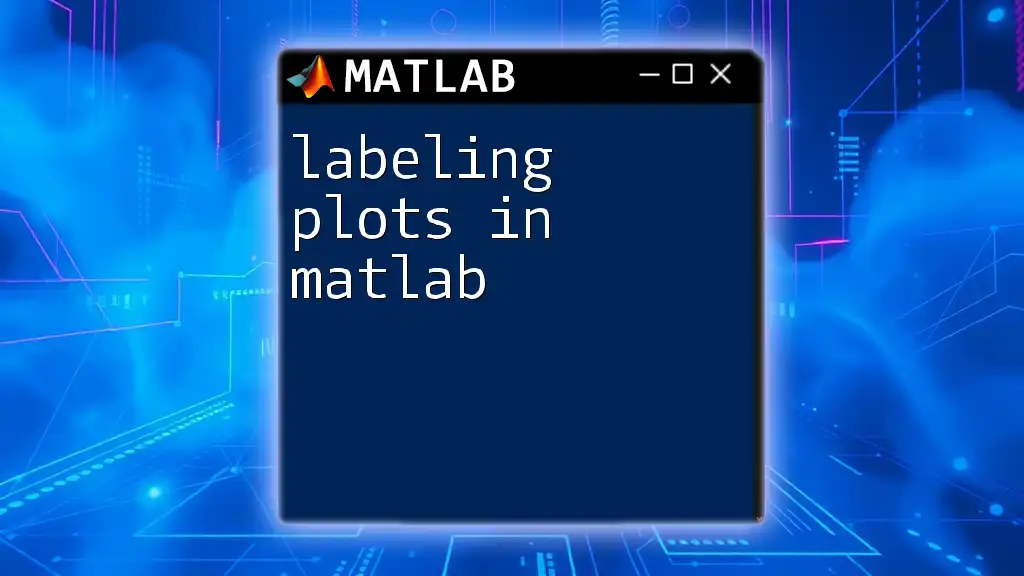
Plotting Bode Plots in MATLAB
Using the Bode Command
The most straightforward way to plot Bode plots in MATLAB is by using the `bode` command. Once you have defined your transfer function, you can generate the plot with a simple command:
% Plotting Bode plot of transfer function G
bode(G)
grid on; % Adding grid for better readability
Executing this command will produce both the magnitude and phase plots of the transfer function `G`.
Customizing Bode Plots
Adjusting Frequency Range
To make your analysis more relevant, you can adjust the range of frequencies over which the Bode plot is generated. Use the `logspace` function to create a vector of frequencies:
% Specify frequency range
w = logspace(-1, 2, 100); % Frequencies from 0.1 to 100 rad/s
bode(G, w);
In this snippet, `w` defines the frequency range from 0.1 to 100 rad/s, allowing you to view the Bode plot over a custom bandwidth.
Customizing Magnitude and Phase
MATLAB allows further customization of your plots, such as changing line styles, colors, and frequency units. You can adjust the options by creating an options structure:
% Customizing Bode plot appearance
opts = bodeoptions;
opts.FreqUnits = 'Hz'; % Change frequency units to Hz
bode(G, opts);
Running this command will generate a Bode plot with frequency represented in Hertz instead of radians, improving interpretability for certain applications.
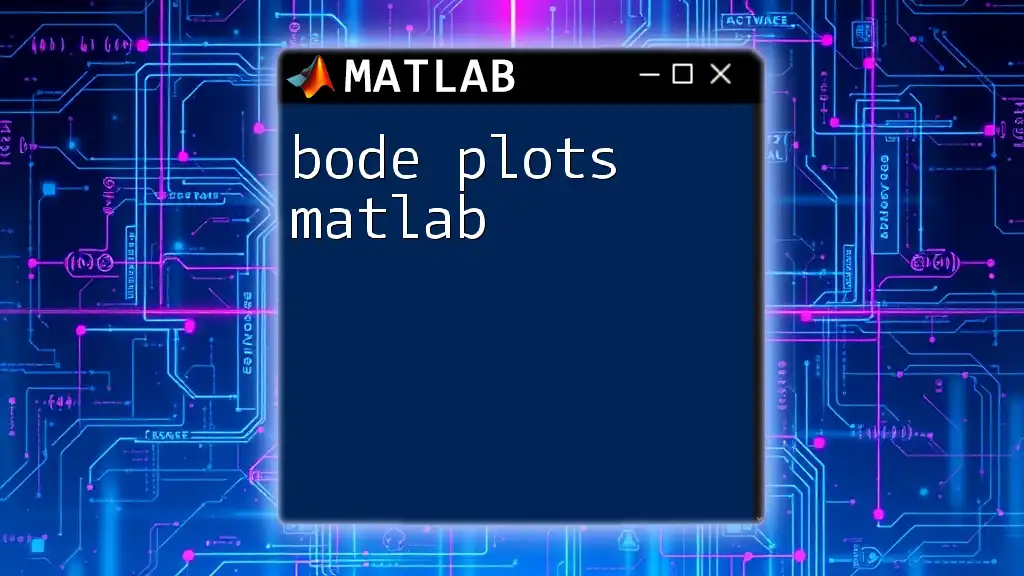
Analyzing Bode Plots
Understanding the Magnitude Plot
When interpreting the magnitude plot, the gain is expressed in decibels. This representation is crucial because dB values provide a more manageable range, especially for systems with high gain.
- A flat magnitude response across a range of frequencies usually indicates a stable system.
- As the frequency increases, a decreasing slope suggests that the system may have stability issues.
Understanding the Phase Plot
The phase plot indicates how much the output lags behind the input over different frequencies, measured in degrees.
- A phase shift around -180 degrees at the crossover frequency can indicate potential instability.
- Understanding the phase characteristics helps engineers design compensators to ensure a stable system response.
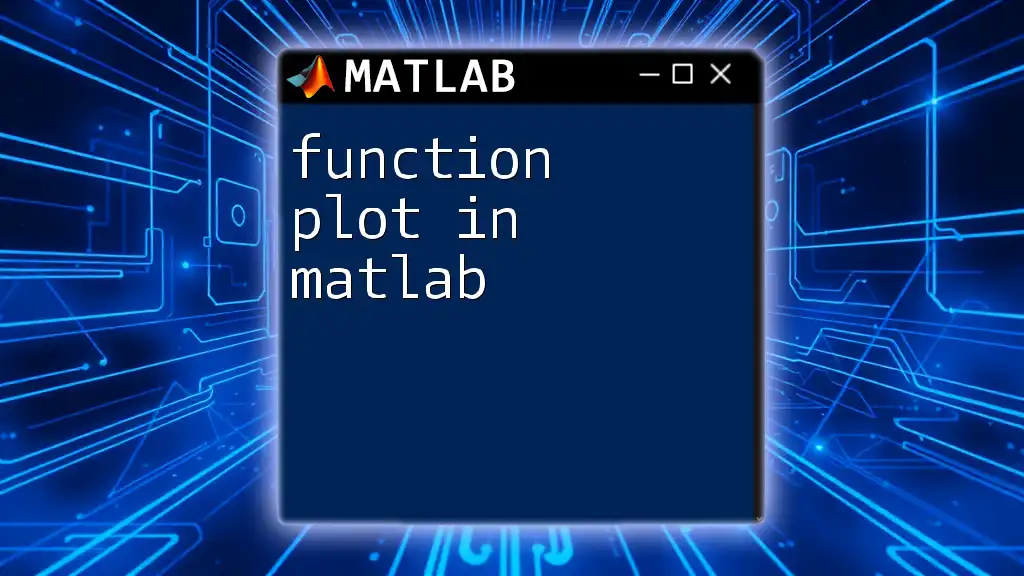
Case Study: Example Dual-Pole System
Example of a Bode Plot for a Given Transfer Function
Let’s consider a dual-pole system represented by the transfer function:
% Example transfer function: G(s) = 1 / (s^2 + 2s + 2)
num = [1];
den = [1 2 2];
G2 = tf(num, den);
bode(G2);
grid on;
In this case, `G2` represents the transfer function \( G(s) = \frac{1}{s^2 + 2s + 2} \).
Interpretation of the Bode Plot Results
Upon generating the Bode plot for this dual-pole system, you will observe:
- The magnitude plot exhibits a certain gain profile, revealing how the system responds to different frequencies.
- The phase plot will likely show a significant phase lag at higher frequencies.
These characteristics can help identify the stability of the system and prompt any necessary adjustments in the design phase.
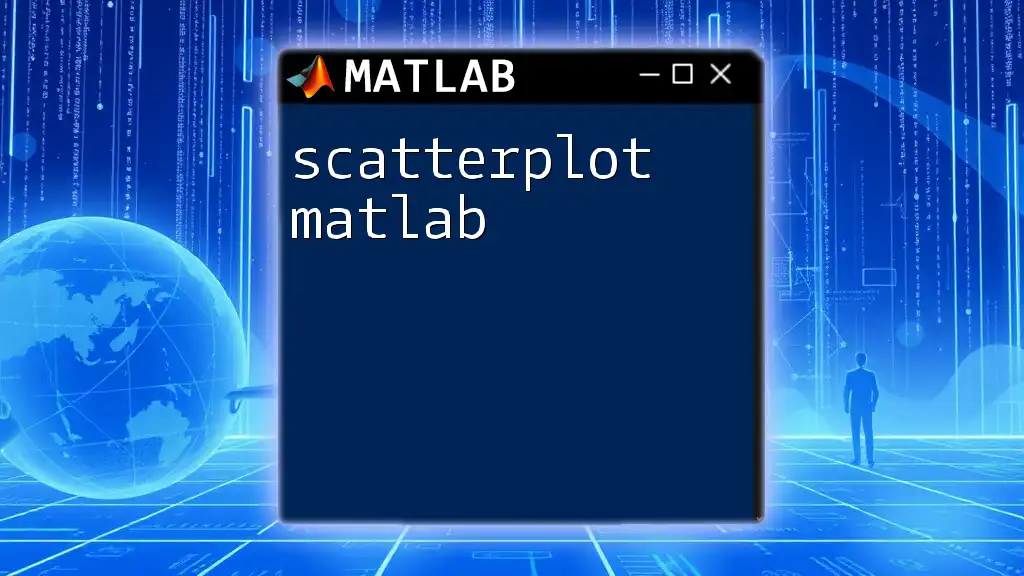
Conclusion
In this guide, we explored the essentials of plotting Bode plots in MATLAB. We delved into the definition of Bode plots, demonstrated how to create transfer functions, and generated and customized Bode plots using MATLAB commands. The analysis of these plots can provide invaluable insights into system behavior, making them fundamental tools for engineers and researchers alike. As you experiment with different transfer functions and frequency ranges, you'll gain ample experience in effectively utilizing Bode plots for control system analysis.
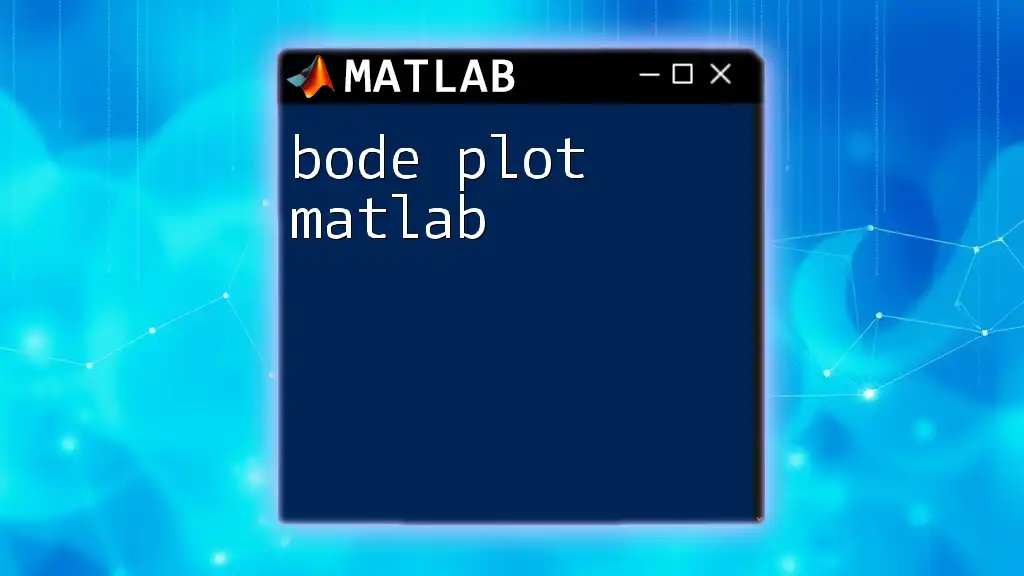
Additional Resources
Recommended Reading
For more in-depth learning, consider exploring the MATLAB documentation on Bode plots and looking into online courses that cover control systems comprehensively.
Community and Support
Engaging with forums and online communities can enhance your learning experience. Don’t hesitate to seek help or collaborate, as the MATLAB user community is rich with knowledge and resources.