A histogram in MATLAB is a graphical representation that organizes a group of data points into user-specified ranges, allowing you to easily visualize the distribution of the data.
Here's a simple code snippet to create a histogram in MATLAB:
data = randn(1,1000); % Generate 1000 random samples from a standard normal distribution
histogram(data, 30); % Create a histogram with 30 bins
xlabel('Data Values'); % Label for X-axis
ylabel('Frequency'); % Label for Y-axis
title('Histogram of Random Data'); % Title of the histogram
Understanding Histograms
A histogram is a graphical representation of the distribution of numerical data. It provides a visual insight into the frequency of data points within specific intervals, known as bins. Histograms are essential for understanding the underlying patterns or trends within datasets, allowing analysts to derive key insights.
Why Use MATLAB for Histograms?
MATLAB is renowned for its powerful data visualization tools, making it an ideal environment for creating histograms. The language's syntax is straightforward, and it includes built-in functions that reduce the complexity of data visualization tasks. With MATLAB, users can create, customize, and analyze histograms with efficiency and precision.
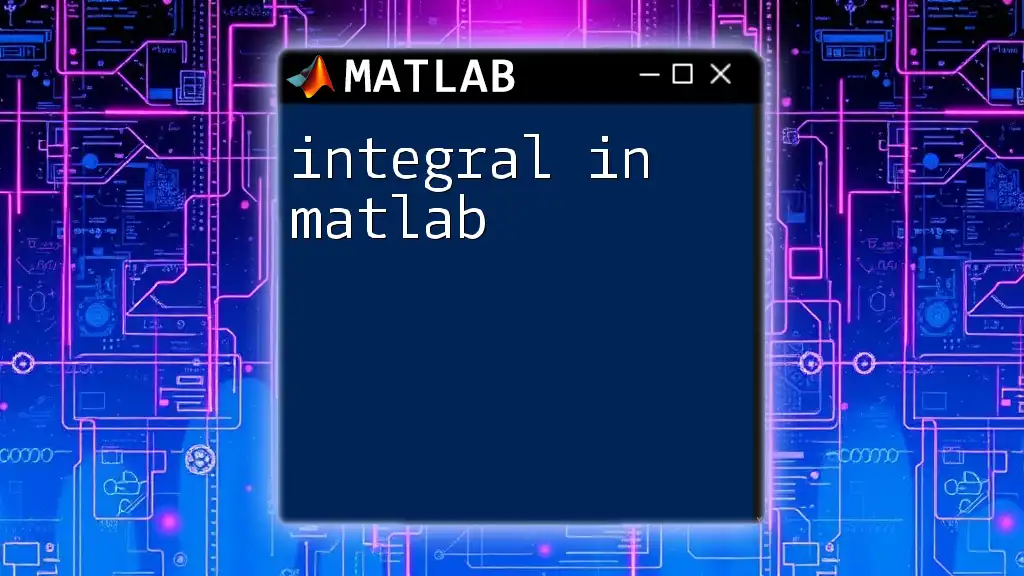
Getting Started with Histograms
Basic Command for Creating a Histogram
To create a histogram in MATLAB, you will primarily use the `histogram` function. This function takes in a dataset and automatically computes the frequency of data points within the specified bins.
Syntax and Parameters
The basic syntax for the `histogram` function is as follows:
histogram(data)
Here, `data` is an array containing the values that you want to visualize.
Sample Data Generation
Before creating a histogram, you typically need sample data. You can easily generate random data points for demonstration purposes. For instance, generating normally distributed data can be done as follows:
% Generate 1000 random data points from a normal distribution
data = randn(1000, 1);
This line of code will give you an array of 1,000 values sampled from a standard normal distribution.
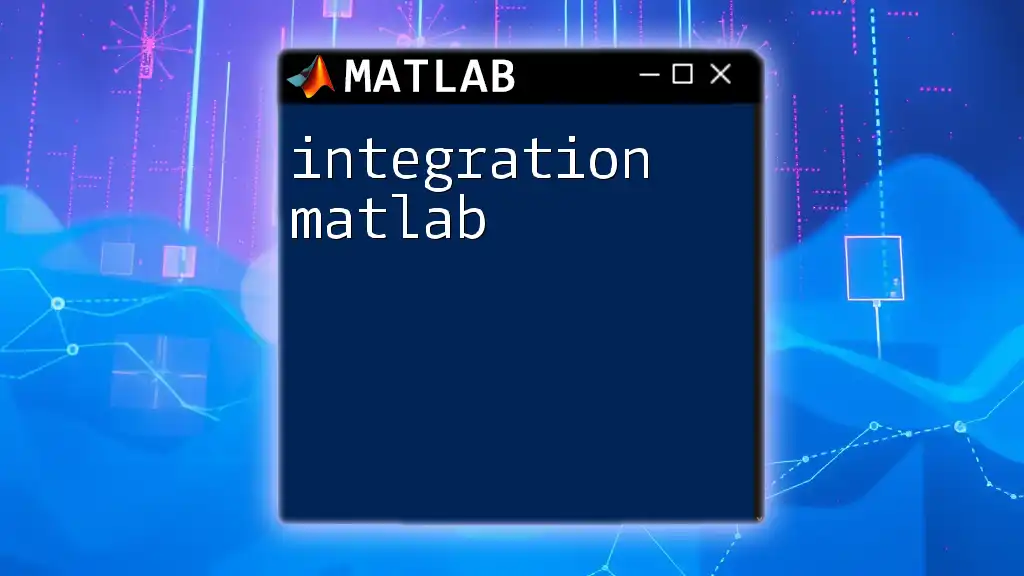
Creating Your First Histogram
Simple Histogram Example
Once you have your data set up, creating a simple histogram is straightforward. Here is how you can generate a basic histogram of your sample data:
% Create a basic histogram
histogram(data);
title('Simple Histogram of Random Data');
xlabel('Data Values');
ylabel('Frequency');
This code will produce a histogram representing the frequency of the randomly generated data points. The title, x-axis, and y-axis labels enhance the understanding of what the histogram represents.
Customizing Your Histogram
Changing the Number of Bins
Bins determine how data is grouped in a histogram. By adjusting the number of bins, you can control the granularity of the histogram. For instance, if you want to have 20 bins instead, you can modify your code like this:
% Create a histogram with 20 bins
histogram(data, 20);
This command will create a more refined view of your data distribution with the specified bin count.
Adding Titles and Labels
Effective titling and labeling improve the readability of your histogram. These annotations provide viewers with essential context about your data. Here’s an example of how to add titles and labels properly:
% Adding titles and labels
histogram(data);
title('Histogram with Custom Bins');
xlabel('Data Values');
ylabel('Frequency');
This enhances the visual communication of your data, making it clear what information the histogram conveys.
Color and Aesthetic Customization
MATLAB allows you to customize the aesthetics of your histogram, including colors and styles, enabling you to make your visualization more appealing. For example, you might want to customize the face color and edge color of the histogram:
% Customizing histogram with color
histogram(data, 'FaceColor', 'blue', 'EdgeColor', 'black');
This customization will change the appearance of your histogram, allowing it to better fit the style of your project or presentation.
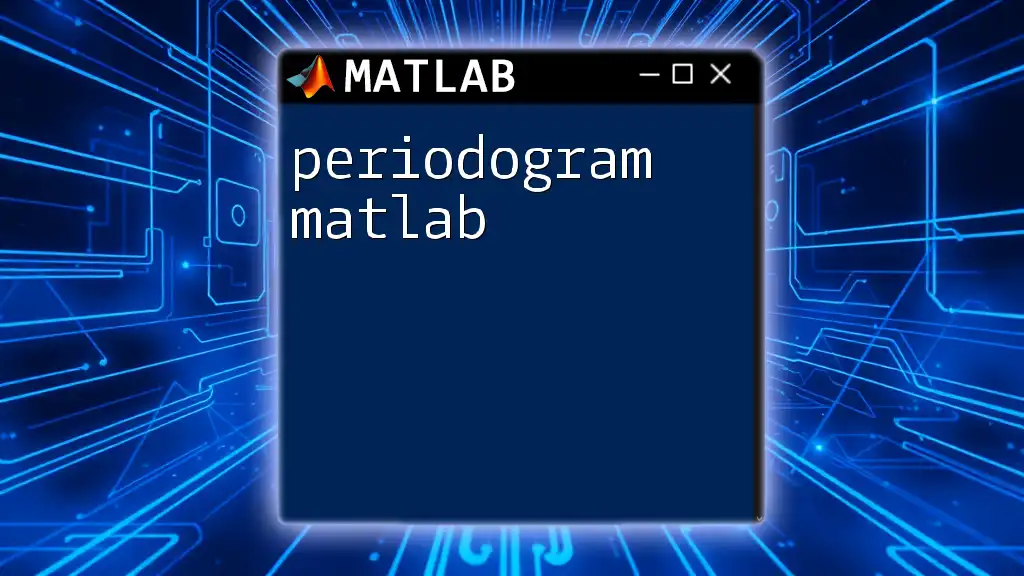
Advanced Histogram Features
Normalizing the Histogram
Normalizing a histogram helps in comparing datasets with different ranges or sizes. By adjusting the histogram to show probabilities instead of frequencies, you can gain valuable insights. Here’s how to create a normalized histogram:
% Create a normalized histogram
histogram(data, 'Normalization', 'pdf');
In this case, the histogram will display the probability density function of your data, helping analysts understand the relative likelihood of the various outcome ranges.
Overlaying Histograms
Overlapping histograms can be particularly useful when comparing multiple datasets. To overlay two histograms, follow these steps:
% Overlaying two histograms
data2 = randn(1000, 1) + 1; % Second data set
histogram(data, 'FaceAlpha', 0.5);
hold on;
histogram(data2, 'FaceAlpha', 0.5);
title('Overlayed Histograms');
legend('Dataset 1', 'Dataset 2');
hold off;
This code will display two histograms on the same plot, with transparency applied so both datasets can be seen. The legend clarifies which color corresponds to each dataset.
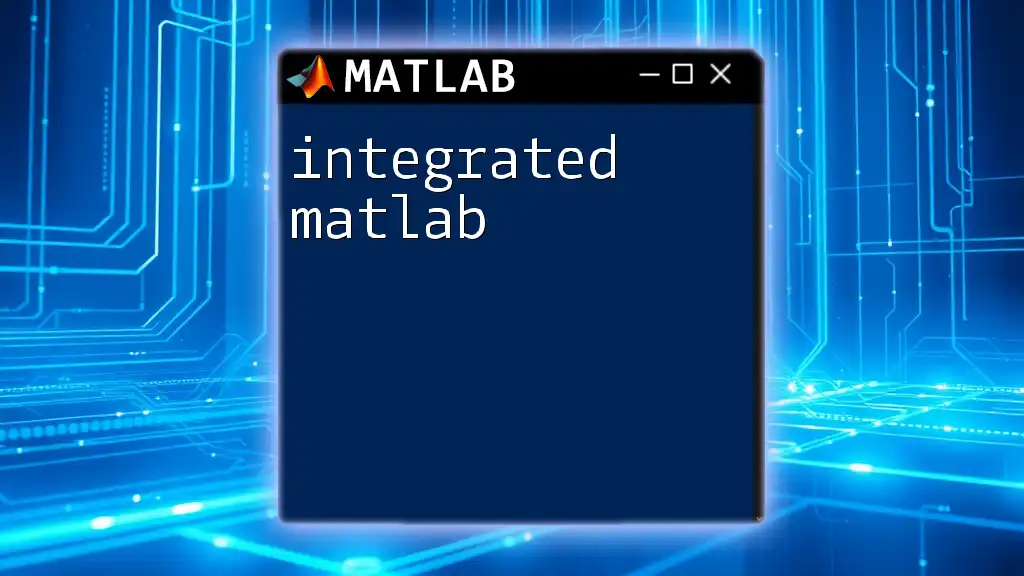
Applications of Histograms in Data Analysis
Statistical Analysis
Histograms play a crucial role in statistical analysis by revealing the distribution of data points. Analysts can quickly observe the skewness, kurtosis, and modality of the data through visual inspection. These factors can influence resultant statistical inferences and model selection.
Use in Image Processing
In image processing, histograms provide insights into pixel intensity values. Analyzing the histogram of an image assists in understanding the brightness and contrast variations, contributing to better image enhancement techniques.
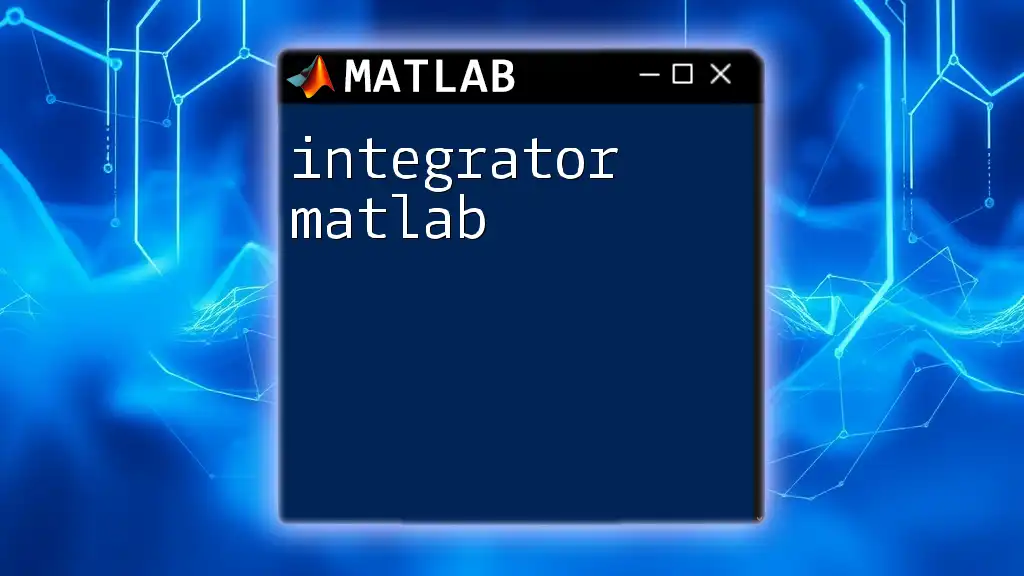
Conclusion
In this article, we thoroughly explored the fundamentals of creating histograms in MATLAB. From generating sample data to customizing your histogram’s appearance, each step highlighted the power and versatility of MATLAB’s visualization tools. A well-crafted histogram can significantly enhance your data analysis efforts, making it easier to interpret complex datasets.
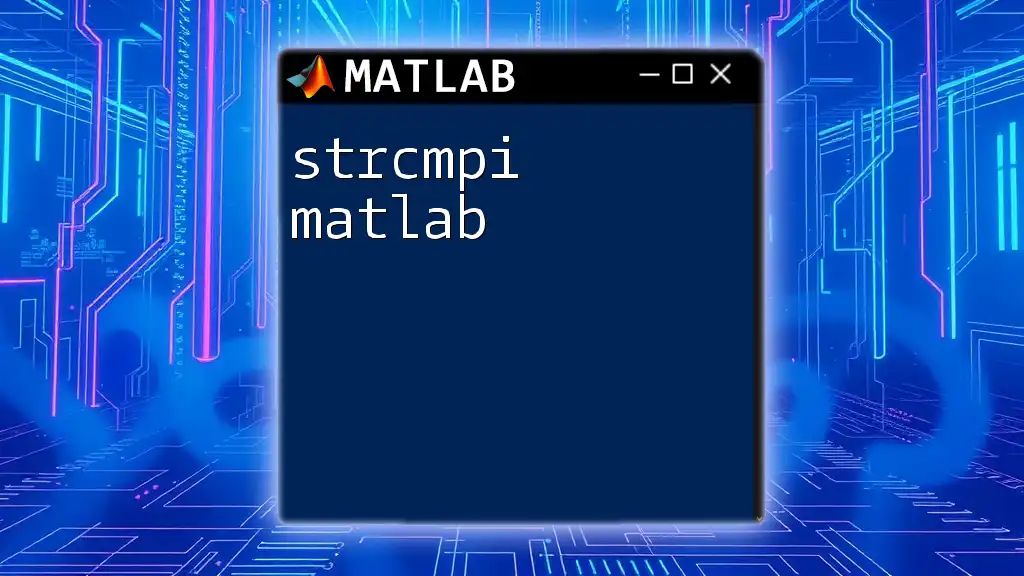
Additional Resources
For further learning, you can refer to the official MATLAB documentation for the `histogram` function. There are numerous tutorials available that can deepen your understanding and application of histograms in MATLAB.
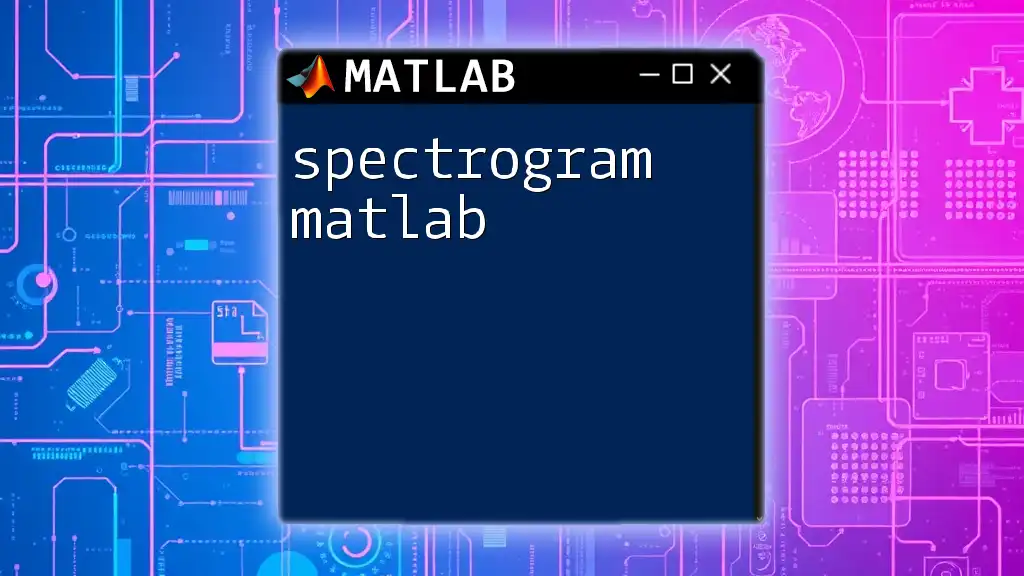
FAQs
Common Questions Regarding MATLAB Histograms
How to fix overlapping bins?
Adjusting the number of bins can often alleviate the problem of overlapping. Additionally, you can use kernel density estimates for clearer visualization.
What to do if the histogram looks cluttered?
You can simplify the histogram by reducing the number of bins or using transparency effects to better distinguish between datasets.
How to export a histogram to a file?
To save your histogram as an image file, use the `saveas` function or `exportgraphics` in newer versions of MATLAB, specifying the desired filename and format. For instance:
saveas(gcf, 'myHistogram.png');
With this comprehensive overview, you’re now equipped to effectively utilize and visualize histograms in MATLAB, unlocking important insights from your data. Happy coding!