`interp2` is a MATLAB function used for performing two-dimensional interpolation on a given grid of data points to estimate values at specified query points.
Here’s a basic code snippet using `interp2`:
% Define grid points and corresponding values
[X, Y] = meshgrid(1:5, 1:5);
Z = [1 2 3 4 5; 6 7 8 9 10; 11 12 13 14 15; 16 17 18 19 20; 21 22 23 24 25];
% Define query points
xq = 2.5;
yq = 3.5;
% Perform interpolation
vq = interp2(X, Y, Z, xq, yq);
% Display result
disp(vq);
Understanding Interpolation
What is Interpolation?
Interpolation is a mathematical technique used to estimate unknown values that fall within the range of a discrete set of known data points. It is widely used in various fields such as engineering, economics, and scientific computing, enabling analysts to make sense of incomplete datasets.
There are various types of interpolation methods, including:
- Nearest neighbor: Chooses the value of the nearest data point.
- Bilinear: Takes a weighted average of the four nearest grid points in a rectangular grid.
- Bicubic: Applies cubic interpolation for smoother results, taking into account the nearest 16 points.
Why Use `interp2`?
The `interp2` function in MATLAB plays a crucial role in 2D data interpolation, allowing users to estimate values on a grid based on the values of points surrounding it. This is particularly useful for applications like converting image resolutions, estimating geographical data points, or filling in missing data. Understanding when and how to use `interp2` is essential for effective data analysis and visualization.
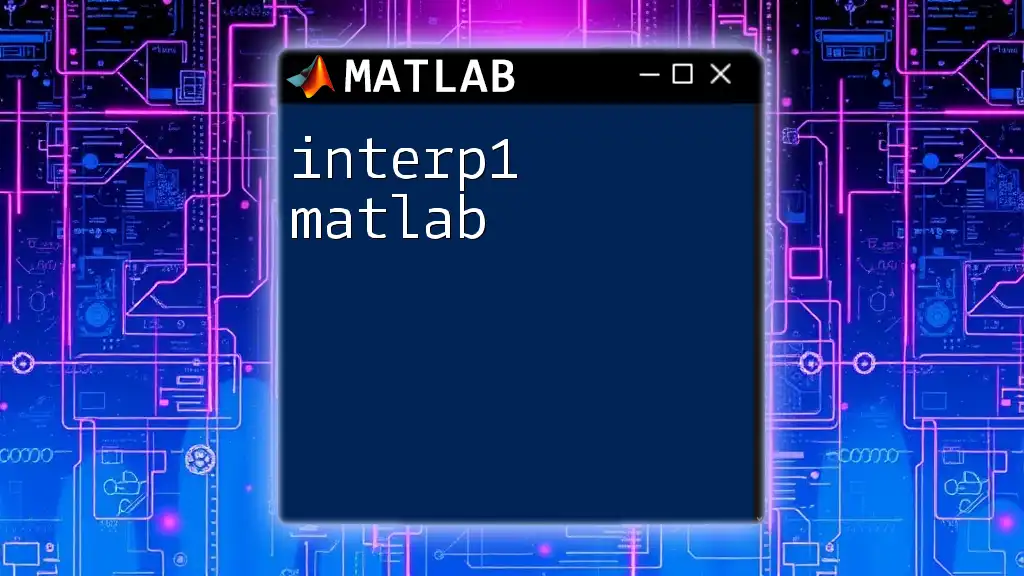
Getting Started with `interp2`
Syntax of `interp2`
The basic syntax for calling the `interp2` function is:
ZI = interp2(X, Y, Z, XI, YI)
Here, `X` and `Y` are matrices defining the coordinates of the data points, `Z` represents the values at those coordinates, and `XI` and `YI` specify where you want the approximated values.
Required Inputs Explained
The Coordinate Matrices
To utilize `interp2`, the input matrices `X` and `Y` must be constructed correctly. These matrices should represent the x and y coordinates of your data in a rectangular grid. MATLAB offers a convenient function, `meshgrid`, to create these matrices easily.
For example, to create a grid from -5 to 5:
[X, Y] = meshgrid(-5:1:5, -5:1:5);
The Data Matrix
The data matrix `Z` contains the actual values that correspond to each pair of coordinates in `X` and `Y`. This matrix should have the same dimension as that of `X` and `Y`. For instance, if you are using a function, you can define `Z` as:
Z = sin(sqrt(X.^2 + Y.^2));
The Interpolation Points
The interpolation points are specified by `XI` and `YI`, which define where you want to estimate new values. You can similarly construct these matrices using `meshgrid`, but with more refined increments to achieve a smoother interpolation. For example:
[XI, YI] = meshgrid(-5:0.1:5, -5:0.1:5);
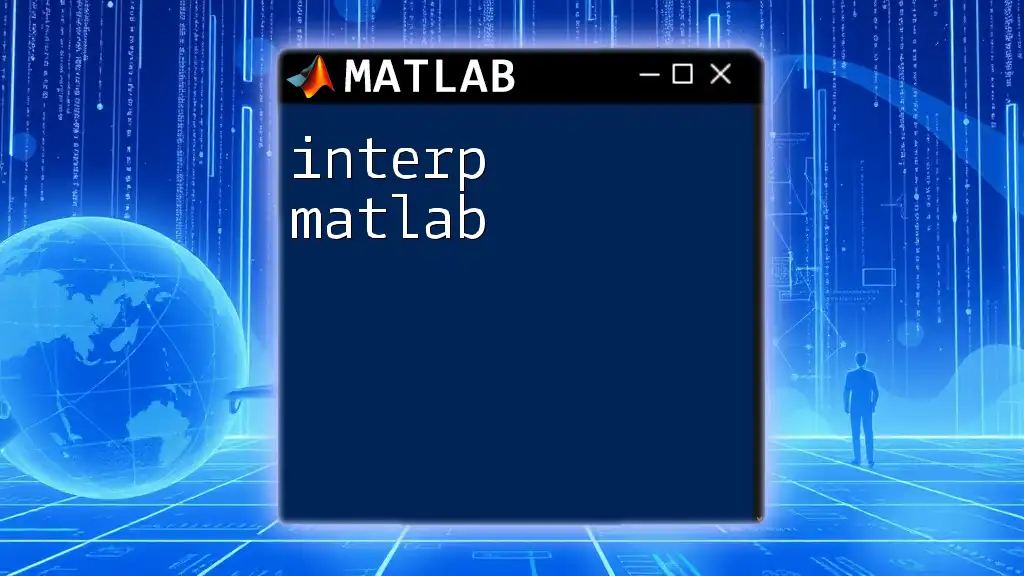
Using `interp2` with Examples
Example 1: Basic Bilinear Interpolation
Now that you have created the necessary matrices, performing a basic bilinear interpolation is straightforward. Here's how you do it:
ZI = interp2(X, Y, Z, XI, YI);
surf(XI, YI, ZI);
In this example, `ZI` now contains the interpolated values calculated at the points defined by `XI` and `YI`. The surface plot using `surf` displays the resulting interpolation visually.
Example 2: Different Interpolation Methods
You can specify different interpolation methods within the `interp2` function to get varying results.
Bilinear Interpolation
For bilinear interpolation, the command remains largely the same as the basic example, but now you explicitly define the method:
ZI_bilinear = interp2(X, Y, Z, XI, YI, 'linear');
Bicubic Interpolation
If you want a smoother appearance, bicubic interpolation can be employed with:
ZI_bicubic = interp2(X, Y, Z, XI, YI, 'cubic');
Example 3: Handling Missing Data
Dealing with missing data is a common issue in data analysis. You can effectively handle this in `Z` using NaN values. Here’s how to replace NaN values before interpolation:
Z(isnan(Z)) = 0; % Replace NaN with 0, then interpolate.
This preprocessing step ensures that your interpolation function has data to work with.
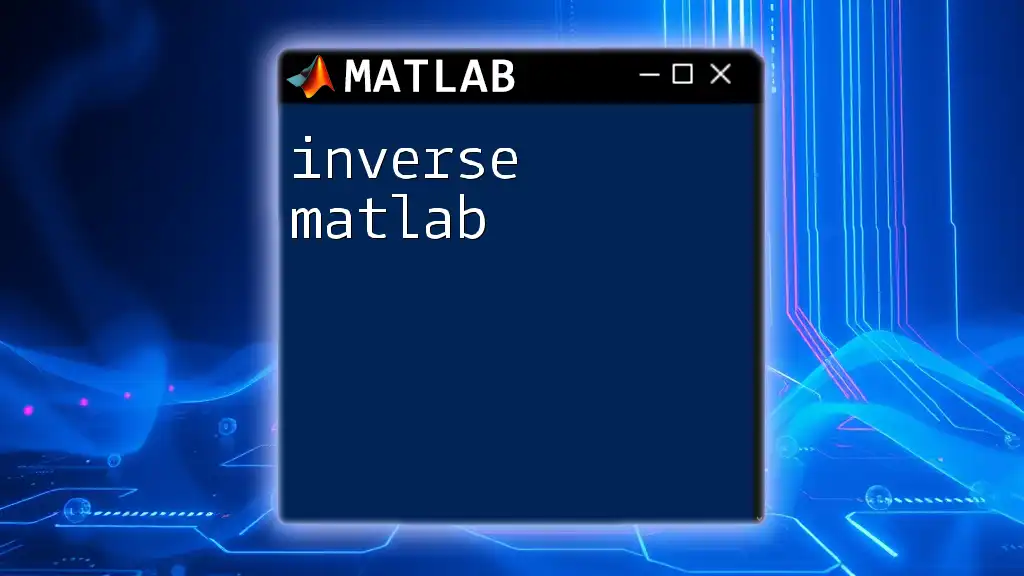
Advanced Features
Output Options
When using `interp2`, you can control the output format, such as specifying the size of the output matrix. This can be useful for achieving better performance or fitting into specific data structures expected in later processing stages.
Extrapolation
The `interp2` function also provides an option for handling values that fall outside the defined grid through extrapolation. This can be achieved like so:
ZI_extrapolated = interp2(X, Y, Z, XI, YI, 'linear', 'extrap');
In this example, points outside the range of `X` and `Y` will be approximated using the specified interpolation method.
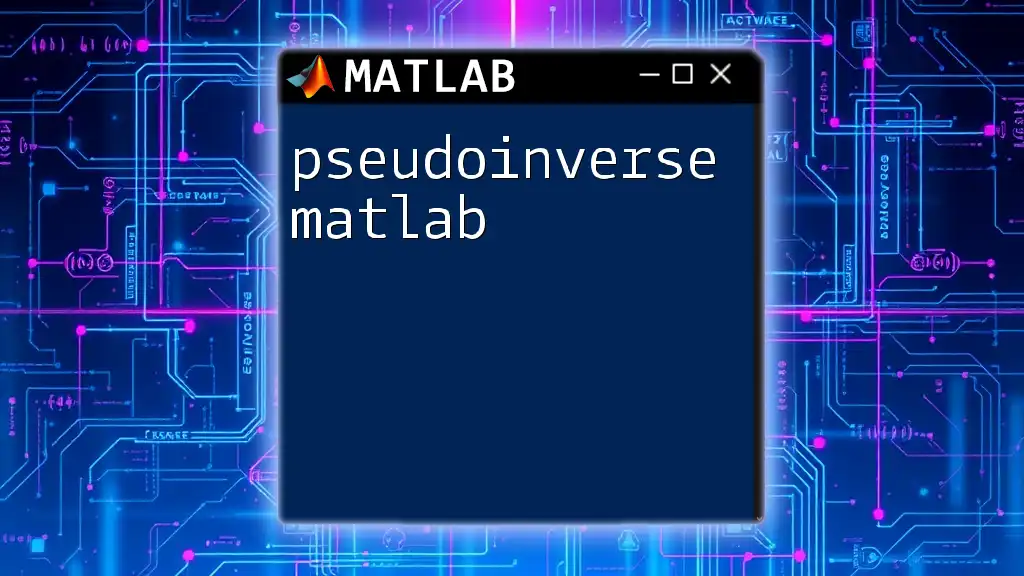
Common Pitfalls
As with any programming task, using `interp2` comes with its challenges. Common mistakes include:
- Dimension Mismatch: Ensure that `X`, `Y`, and `Z` matrices share the same dimensions.
- Interpolation Points Out of Range: Attempting to interpolate outside the bounds of the provided data will yield unexpected results unless extrapolation is used.
- NaN Values: Forgetting to handle NaN values can lead to distorted interpolated results or errors in execution. Always cleanse the data before processing.
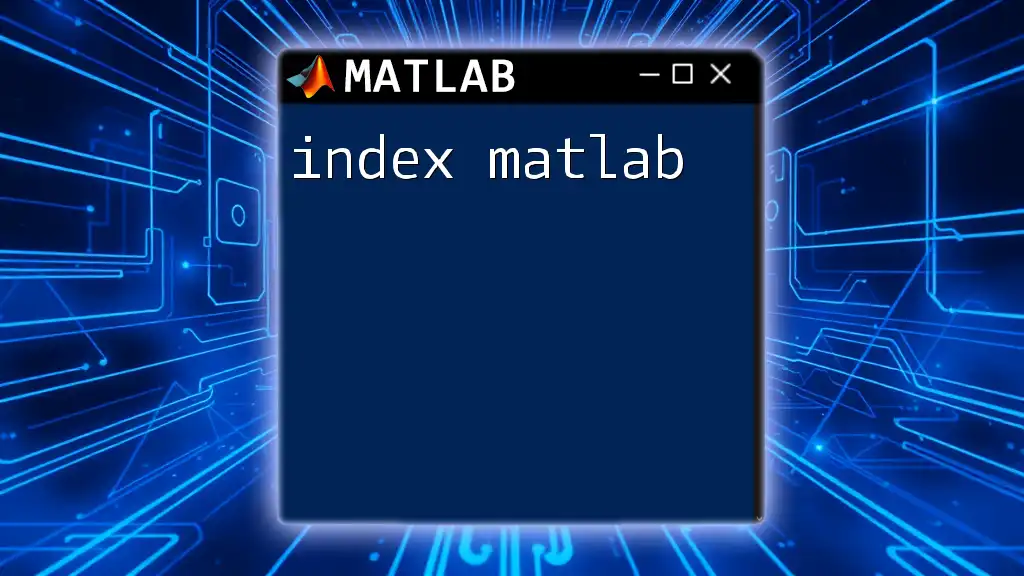
Practical Applications
Case Study: Image Resizing
One practical application of `interp2` is in image processing tasks such as resizing. Using bilinear interpolation, you can upscale an image to improve resolution by estimating pixel values for new coordinate points.
Case Study: Geographic Data Estimation
In geospatial analysis, `interp2` is invaluable for estimating terrain elevations at specific geographic coordinates or resampling environmental data. This can enhance decision-making across various sectors like urban planning and resource management.
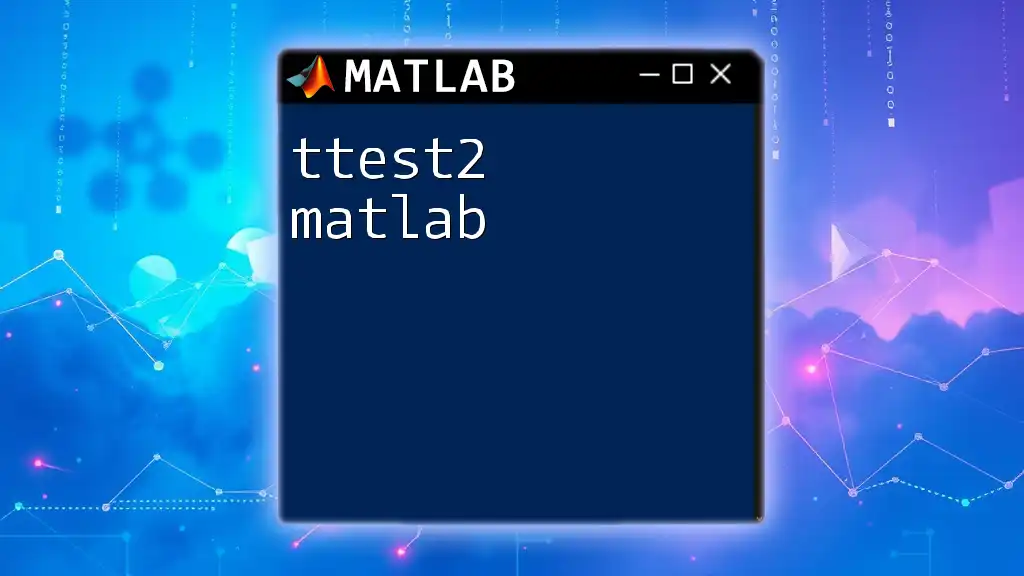
Conclusion
Utilizing `interp2` in MATLAB provides an efficient way to perform 2D interpolation. By thoroughly understanding its syntax and practical applications, you can unlock valuable insights from your data. Experimenting with different datasets and interpolation methods will enhance your skills in data analysis and visualization.
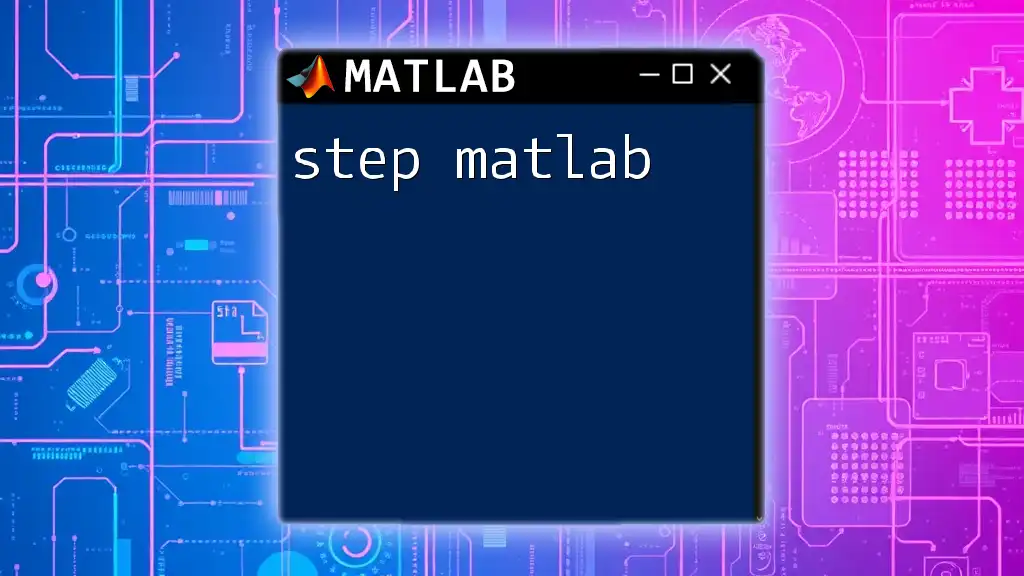
Additional Resources
For further learning, consider exploring the official MATLAB documentation for `interp2`, which offers additional insights and advanced techniques. Online tutorials, courses, and community forums can provide further support as you master this useful MATLAB function.