In MATLAB, you can solve equations quickly using the `solve` function from the Symbolic Math Toolbox, which allows you to find the roots of algebraic expressions efficiently.
syms x; % Define the symbolic variable
equation = x^2 - 4 == 0; % Specify the equation
solution = solve(equation, x); % Solve the equation
disp(solution); % Display the solution
Understanding Equations in MATLAB
Types of Equations
Linear Equations: These equations have a constant slope and can be represented in the form \( ax + b = c \). For example, the equation \( 2x + 3 = 7 \) is linear. Solving such equations using MATLAB involves straightforward syntax and direct application.
Nonlinear Equations: Unlike linear equations, nonlinear equations can take various forms and do not have a constant slope. An example is \( x^2 - 4 = 0 \). These equations typically require numerical methods for solving, making MATLAB an essential tool due to its robust numeric solver capabilities.
Systems of Equations: A system consists of multiple equations involving two or more variables. For instance:
- \( x + y = 10 \)
- \( 2x - y = 3 \)
This type of problem can be solved simultaneously in MATLAB, which showcases its power in handling complex scenarios.
MATLAB's Capabilities
Built-in Functions for Solving Equations: MATLAB provides several built-in functions designed specifically for solving equations. These include:
- `solve`: For symbolic equations.
- `fzero`: A function for finding roots of nonlinear equations.
Symbolic vs. Numeric Solutions: It's crucial to understand that MATLAB can provide both symbolic solutions with exact values (like fractions) and numeric solutions which are approximated. The choice between the two largely depends on the specific requirements of your problem. Use symbolic methods when exact values are needed and numeric approaches when dealing with complex or high-dimensional data.
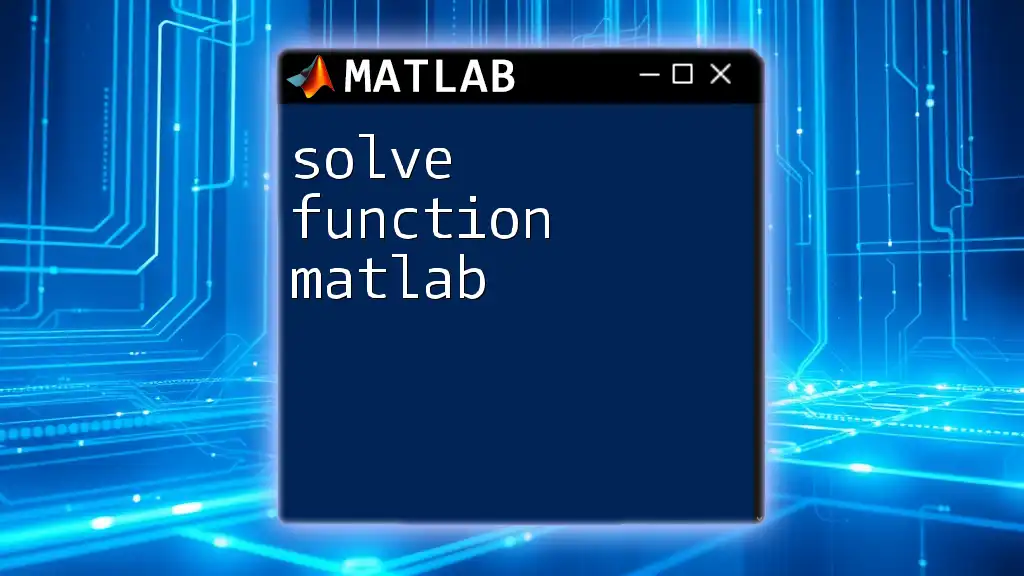
How to Use the `solve` Function
Setting Up the Environment
Installation and Setup: Before you begin, ensure that you have MATLAB installed. You can download it from the official MathWorks website.
Creating and Initializing Variables: In MATLAB, use the `syms` function to declare symbolic variables that can be used in equations. For instance:
syms x y
Basic Syntax and Usage
The `solve` Function Explained: The `solve` function is powerful for algebraic equations. Its syntax is fairly straightforward:
solve(equation, variable)
In practical terms, to solve our earlier linear equation \( 2x + 3 = 7 \), you would write:
syms x;
equation = 2*x + 3 == 7;
solution = solve(equation, x);
disp(solution);
This code snippet initiates a symbolic variable `x`, defines the equation, and computes the solution.
Solving Multiple Equations
Using `solve` for Systems of Equations: For more complex problems involving systems of equations, you can input multiple equations in the `solve` function. Here's an example:
syms x y;
eq1 = x + y == 10;
eq2 = 2*x - y == 3;
solutions = solve([eq1, eq2], [x, y]);
disp(solutions);
This snippet declares the equations and finds the values of `x` and `y` that satisfy both, demonstrating MATLAB's efficiency in solving systems.
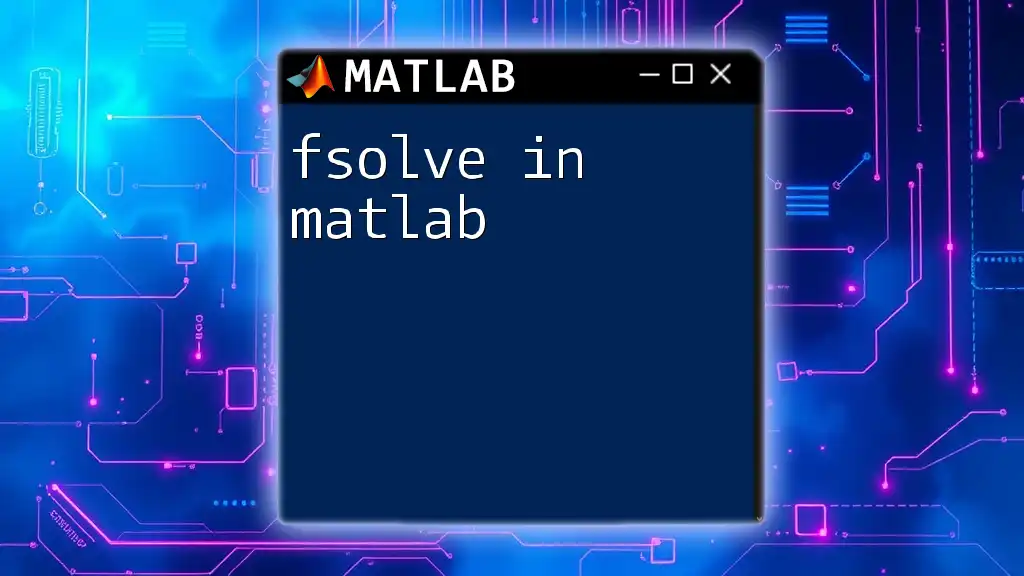
Utilizing Other MATLAB Functions
Numeric Solutions
Using `fzero` for Nonlinear Equations: For solving nonlinear equations, `fzero` is highly effective. For example, to find the root of the equation \( x^2 - 4 = 0 \):
func = @(x) x^2 - 4; % Define the function
root = fzero(func, 1); % Initial guess of 1
disp(root);
In this case, you define a function using an anonymous function handle and provide an initial guess to locate the root.
Optimization with `fminunc`
Finding Minima: MATLAB’s `fminunc` function is another useful tool for solving optimization problems. It finds the minimum of unconstrained multivariable functions. You must define the function and its gradient beforehand. For instance:
function_to_minimize = @(x) (x(1)-1)^2 + (x(2)-2)^2; % Example function
initial_guess = [0, 0]; % Starting point
optimal_solution = fminunc(function_to_minimize, initial_guess);
disp(optimal_solution);
This illustrative snippet shows how to minimize a function defined by a sum of squares, demonstrating the versatility of MATLAB in optimization contexts.
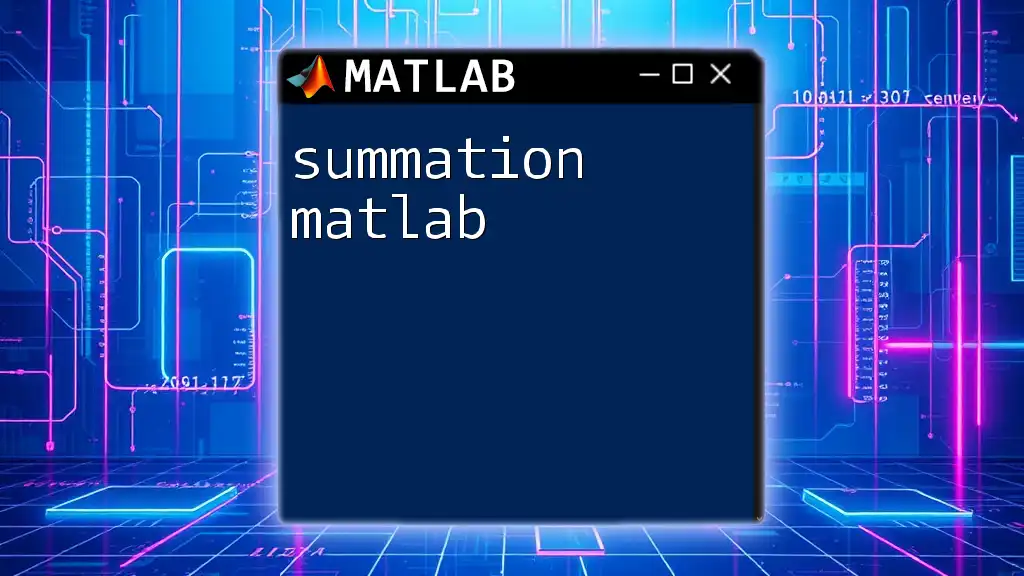
Practical Applications
Engineering Problems
Case Study: Finding Forces in Structures: To apply MATLAB in a real-world scenario, consider a simple structure under load. By setting up equations based on the forces and applying the `solve` function, engineers can determine the values that maintain equilibrium in the structure. For example, using equilibrium conditions, you could set equations like:
syms F A B; % Forces in the structure
eq1 = F + A + B == 0; % For example, all forces equal zero
sol = solve(eq1, A);
disp(sol);
Such application showcases how MATLAB aids in solving complex engineering equations efficiently.
Scientific Research
Example: Population Models: In biological contexts, population dynamics can be modeled using differential equations. By solving these equations numerically in MATLAB, researchers can predict future population sizes. For example:
function population_growth = population_model(t, P)
r = 0.1; % growth rate
K = 100; % carrying capacity
population_growth = r * P * (1 - P/K);
end
Using `ode45` to solve these differential equations allows scientists to simulate and analyze population behaviors over time.
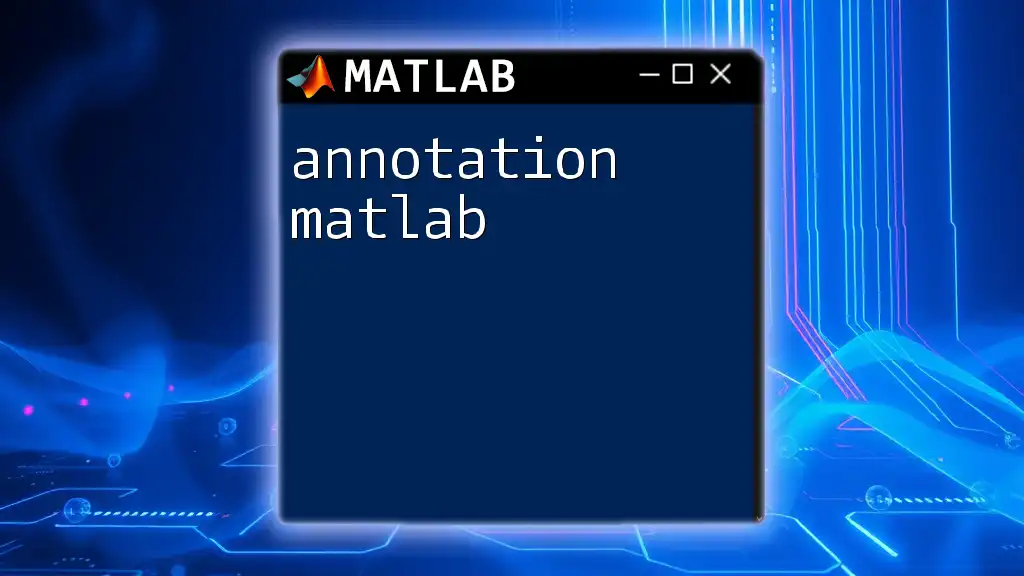
Common Errors and Troubleshooting
Syntax Errors
Common Mistakes When Using `solve`: One prevalent issue is malformed equations. Ensure that equations are correctly formatted, using `==` for equality checks and placing variables in the correct context.
Interpretation of Results
Understanding the Output: MATLAB's results must be interpreted cautiously. When outputting solutions, the results may appear as a symbolic representation or numerical format. Always validate and interpret these in the context of your problem to avoid missteps.
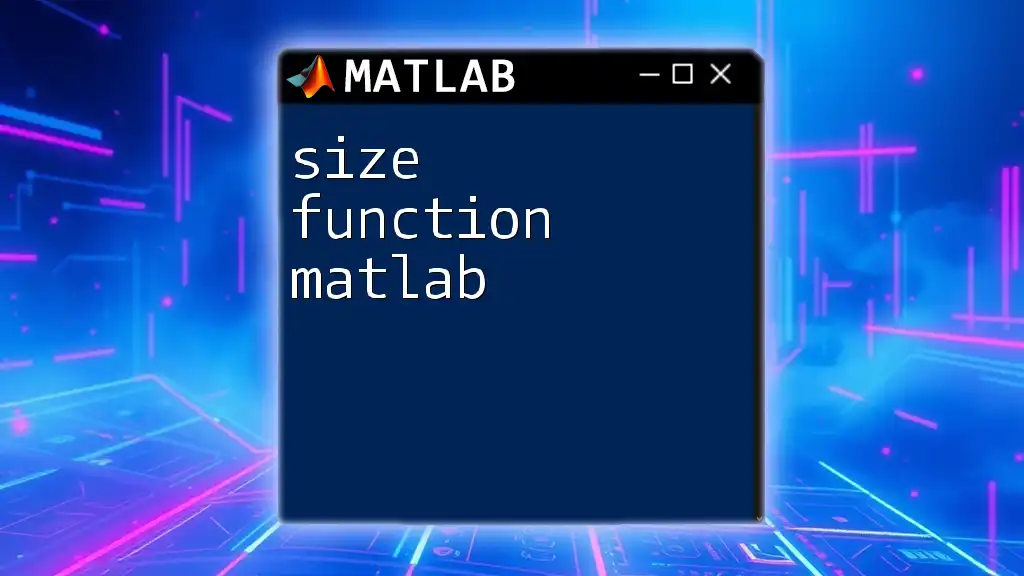
Conclusion
In this guide, we explored the various facets of how to solve equations in MATLAB. From understanding different types of equations and using the `solve` function to applying numerical solvers, the capabilities of MATLAB are vast. As you engage with these tools, consider delving deeper into specific functions and methodologies that can elevate your problem-solving skills. MATLAB is not merely a programming language; it’s a robust environment that allows for innovative solutions across numerous fields.
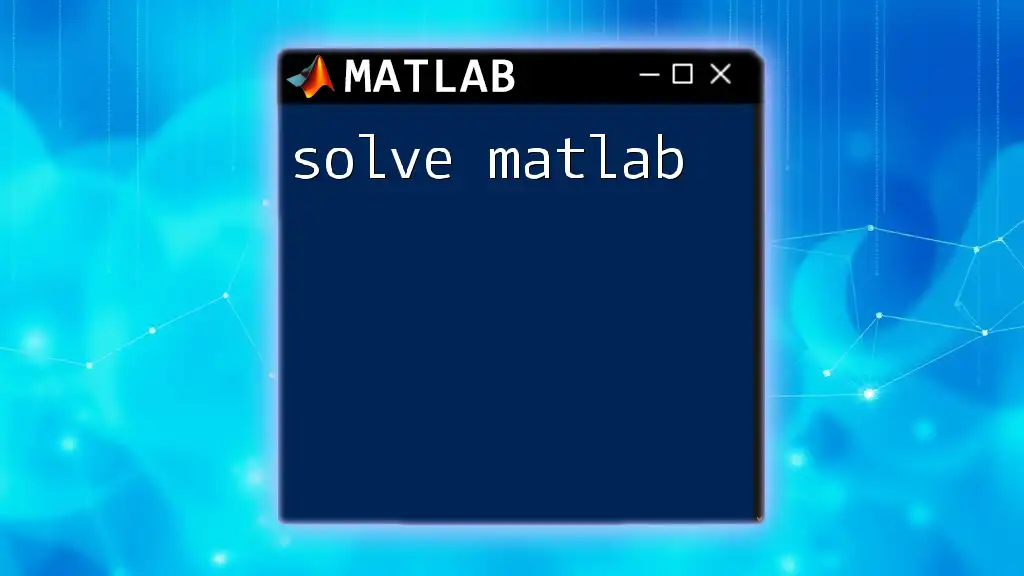
Additional Resources
Recommended Reading and Tutorials
To further enhance your understanding of solving equations in MATLAB, consider exploring the following:
- MATLAB Documentation: The official documentation offers in-depth explanations and examples.
- Online Courses: Platforms like Coursera and LinkedIn Learning provide structured courses on MATLAB.
Community and Support
Engaging with communities can be invaluable. Websites such as MATLAB Answers and various online forums offer support and tutorials from experienced users, enhancing your learning experience.