The `fread` function in MATLAB is used to read binary data from a file, allowing you to specify the data type and the number of elements to read.
Here’s a simple example of how to use `fread` in MATLAB:
fid = fopen('data.bin', 'rb'); % Open the binary file for reading
data = fread(fid, 100, 'double'); % Read 100 elements of type double
fclose(fid); % Close the file
Understanding `fread`
What is `fread`?
`fread` is a MATLAB function designed to read binary data from a file. It offers a powerful way to handle raw binary files, relevant in various applications such as data processing, scientific computing, and simulation. By using `fread`, you can efficiently extract numerical data without the overhead associated with parsing text formats.
Syntax and Parameters
To properly use `fread`, you'll need to understand its syntax:
A = fread(fileID, sizeA, precision, machinefmt)
Parameters Explained:
- fileID: This is the identifier for the file you want to read from. You can obtain a `fileID` by calling `fopen`, which opens the file for reading.
- sizeA: Specifies the size of the output array, defining how many values you want to read at once.
- precision: Indicates the data type for the output array. The choice of precision can significantly impact the data you read, as it determines how many bytes are read for each value.
- machinefmt: An optional parameter that specifies the byte ordering, which can be particularly important when dealing with files created on different architectures.
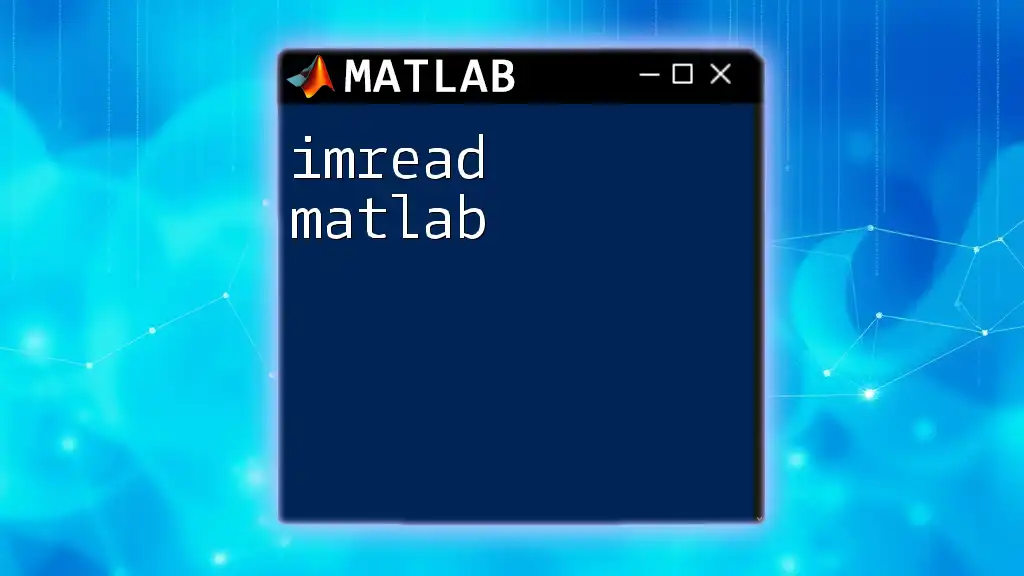
Setting Up for Using `fread`
Opening a File
Before you can read data, you need to open the file. Use the `fopen` function to achieve this:
fileID = fopen('data.bin', 'r');
Checking File Status
After opening the file, it's crucial to ensure that it is valid. If `fileID` returns -1, it indicates that the file could not be opened. You can use a simple conditional statement to check this:
if fileID == -1
error('File cannot be opened. Check the path or file permissions.');
end
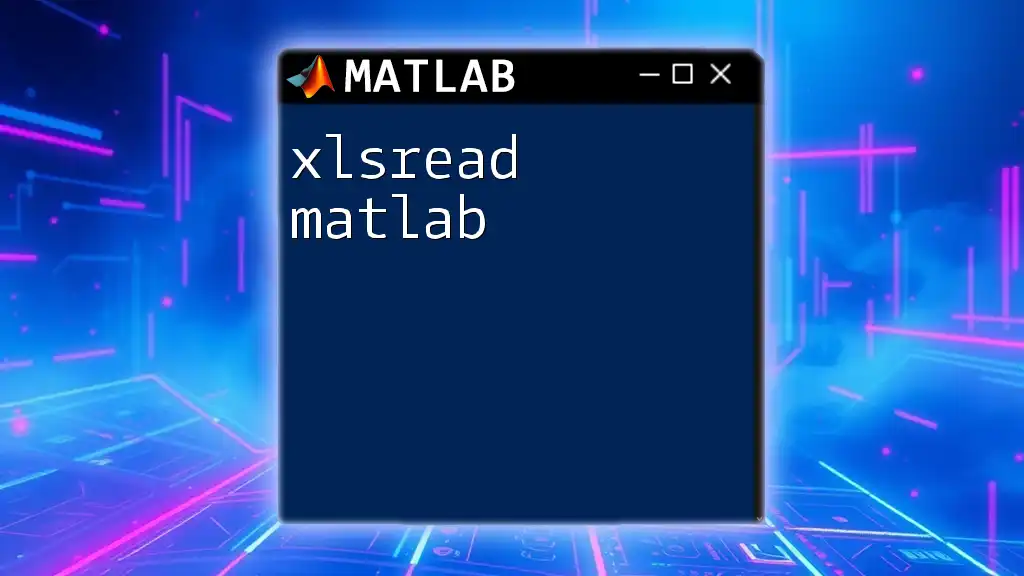
Reading Binary Data with `fread`
Example: Basic Usage
The simplest use of `fread` involves reading a specific number of data points from a binary file. Here’s an example of how to read ten double-precision floating-point numbers:
data = fread(fileID, 10, 'double');
This command will read ten numbers and store them in the array `data`. The output depends entirely on the contents of your binary file.
Reading Different Data Types
Supported Data Types
`fread` supports a variety of data types, including but not limited to:
- 'int16': Signed 16-bit integers
- 'uint8': Unsigned 8-bit integers
- 'float32': 32-bit floating-point numbers
Example: Reading Integer Data
You can also read integers efficiently, as shown in the following example:
intData = fread(fileID, 5, 'int16');
This reads five 16-bit signed integers from the file. Such flexibility allows you to work seamlessly with various binary formats.
Handling Large Files
Specifying Size
When dealing with large files, you might want to specify the size of the output array to avoid excessive memory use. For instance, to read a 100x100 array of doubles:
largeData = fread(fileID, [100, 100], 'double');
This command reads a two-dimensional array, making it easier and more efficient when handling large datasets.
Reading Data in Chunks
If your files are exceptionally large, reading them all at once could strain memory resources. Instead, you can loop through the file in chunks:
while ~feof(fileID)
chunk = fread(fileID, 1000, 'double');
% Process chunk
end
Using a loop can help you manage memory usage better while still effectively processing data.
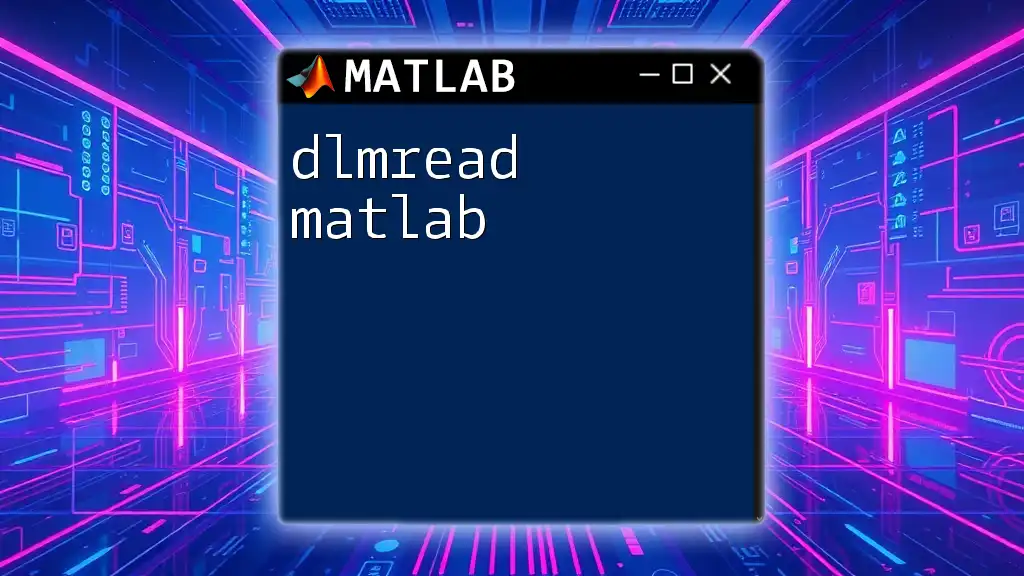
Working with Format and Data Conversion
Using Precision Formats
Choosing the appropriate precision is essential, as it affects how data is stored and read. Each precision specifier corresponds to a different number of bytes. For example, using 'double' means each number occupies 8 bytes in memory, while 'uint8' would take just 1 byte.
Converting Data After Reading
Once you’ve read the data, you may need to convert its type for further analysis. For instance, if you read unsigned integers but need them as doubles:
data = fread(fileID, 10, 'uint8');
convertedData = double(data);
This conversion ensures you can use the data in mathematical computations efficiently.
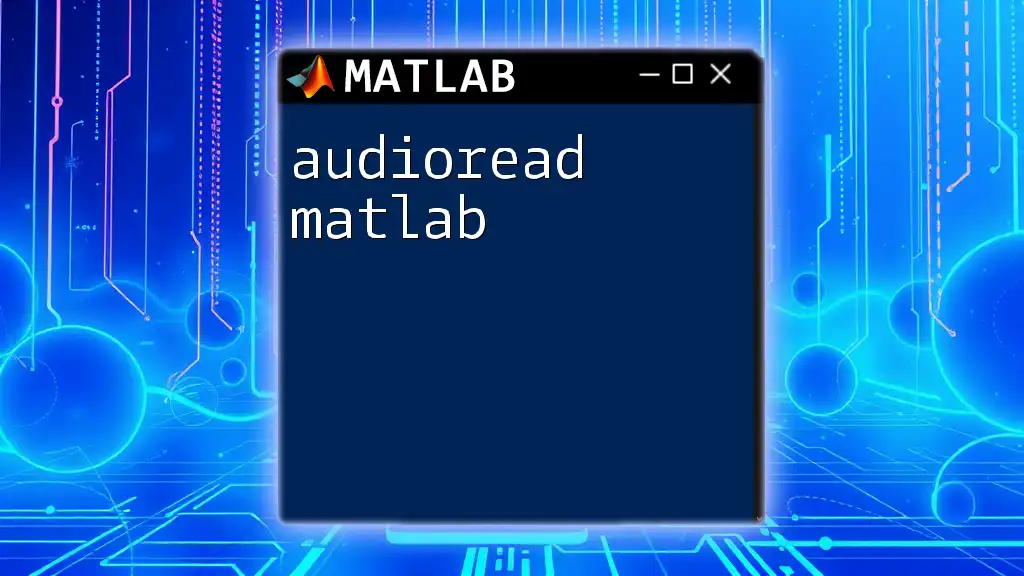
Error Handling in `fread`
Common Errors and Solutions
When working with file operations, errors can occur. One common mistake is trying to read from a file that couldn’t be opened. Use `ferror(fileID)` to identify potential issues:
if ferror(fileID)
disp('Error reading the file.');
end
Recognizing these errors promptly can save you from unexpected problems during data analysis.
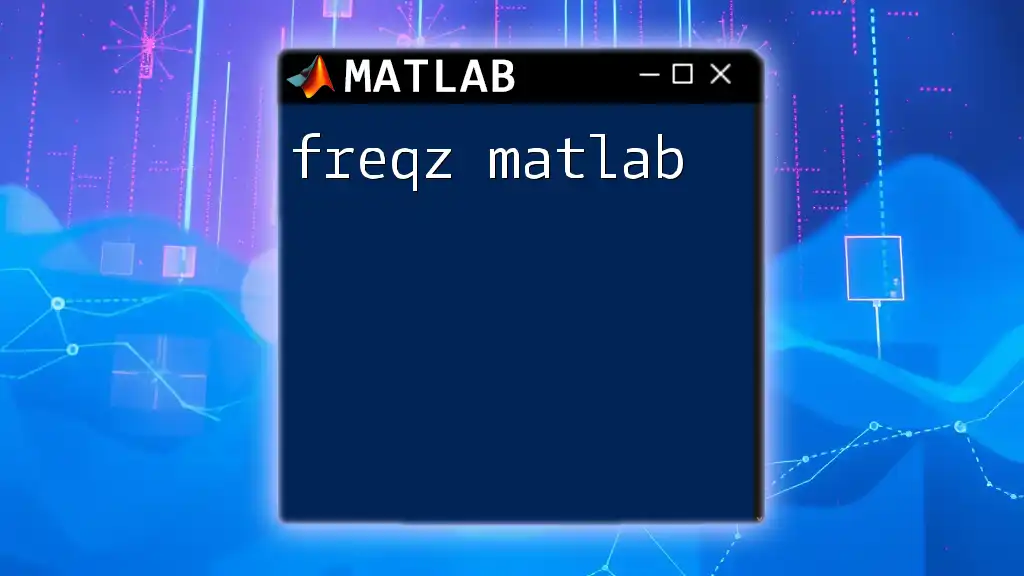
Closing the File
Properly Closing File Identifiers
Once your operations are complete, it is of utmost importance to close the file to free up system resources:
fclose(fileID);
Neglecting to do so could lead to memory leaks and file corruptions, so always ensure you perform this final step.
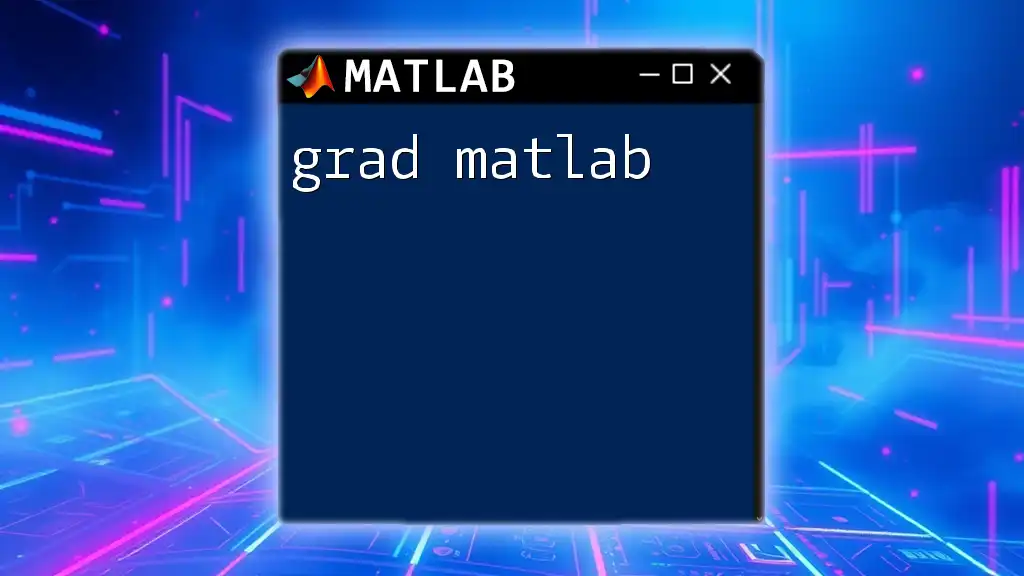
Advanced Usage and Tips
Reading from a Specific Point
You may find that it’s necessary to start reading from a specific point in the file. The `fseek` function can be used to navigate to a desired position:
fseek(fileID, offset, 'bof');
data = fread(fileID, size, 'double');
This allows for much flexibility when working with structured binary data.
Performance Considerations
Consideration of performance is crucial, especially if you are working with large datasets. The size of the chunks you choose to read can affect speed—experiment with different chunk sizes to identify the optimal size for your specific situation.
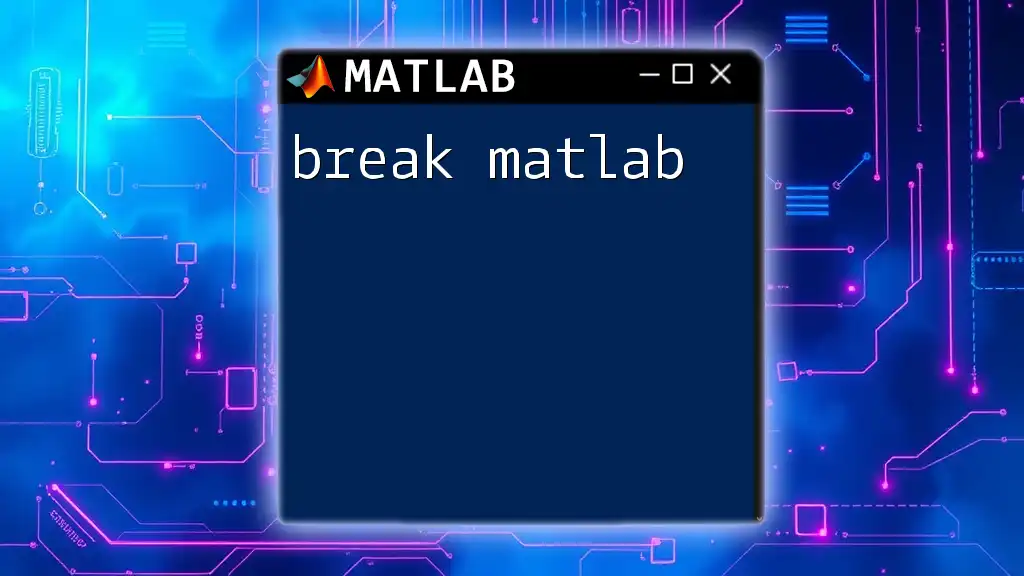
Conclusion
In conclusion, `fread` is an indispensable tool in MATLAB for reading binary data efficiently and effectively. Understanding the intricacies of file handling, data types, and reading strategies can greatly enhance your data processing capabilities. By mastering this function, you can leverage MATLAB’s power to perform intricate data analyses seamlessly.
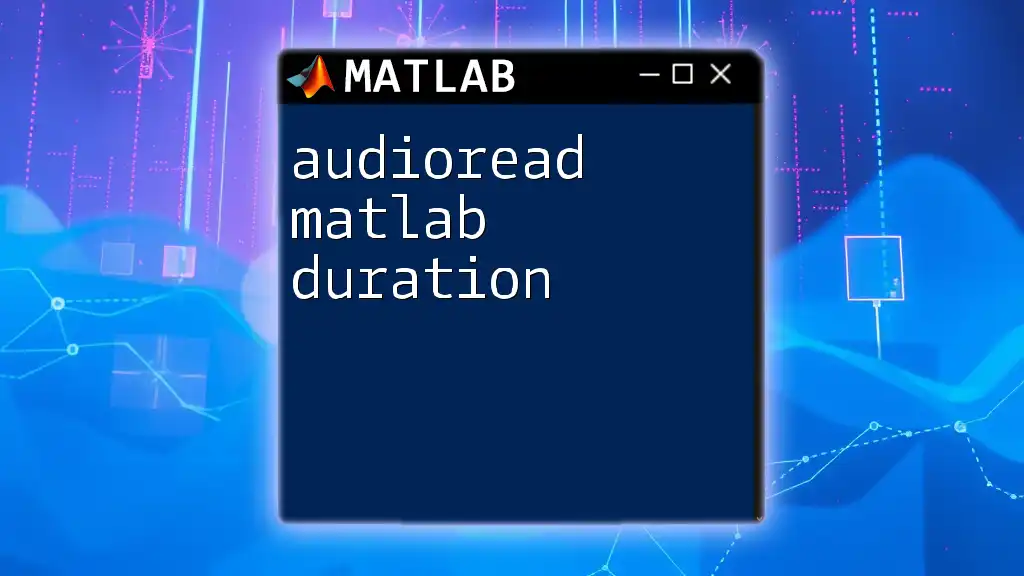
Additional Resources
For further exploration, consult the official MATLAB documentation on `fread`, or check out video tutorials that cover file I/O and data manipulation techniques, giving you a more visual approach to mastering these concepts.