The `append` function in MATLAB is used to concatenate arrays or strings, allowing you to create larger datasets or combine character arrays effectively.
result = [array1, array2]; % Concatenates two arrays
% or
combinedStr = strcat('Hello, ', 'World!'); % Concatenates two strings
What is the `append` Command?
The `append` command in MATLAB serves as a powerful tool in data management, allowing users to concatenate multiple datasets seamlessly. Its versatility makes it beneficial across various applications, whether it’s working with numeric arrays, tables, or even files.
General Syntax and Functionality
The general syntax for the `append` function is:
append(A, B)
Where `A` and `B` represent the datasets you wish to merge. This simple yet effective function is crucial for maintaining a cohesive dataset, especially when dealing with large volumes of data accumulated over time.
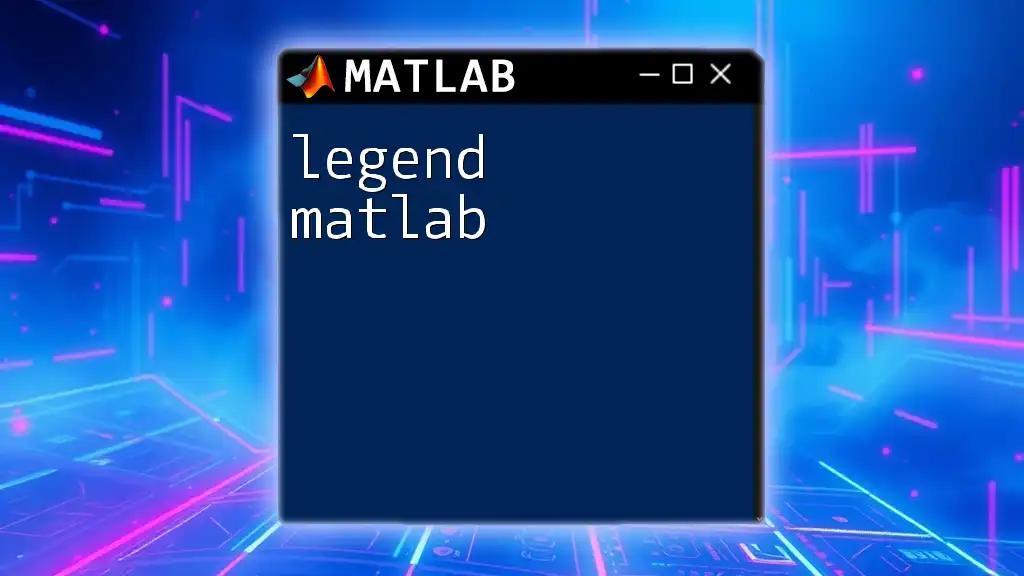
Why Use the `append` Command?
Understanding why to use the `append` command is as critical as knowing how to use it. Appending data rather than replacing it allows users to grow datasets dynamically. This is particularly vital during data analysis workflows or processes that require keeping historical data intact while updating or adding new entries.
Practical applications of this command include merging data from experiments, expanding datasets from simulations, or consolidating results from multiple sources. The flexibility of `append` is invaluable in these scenarios.
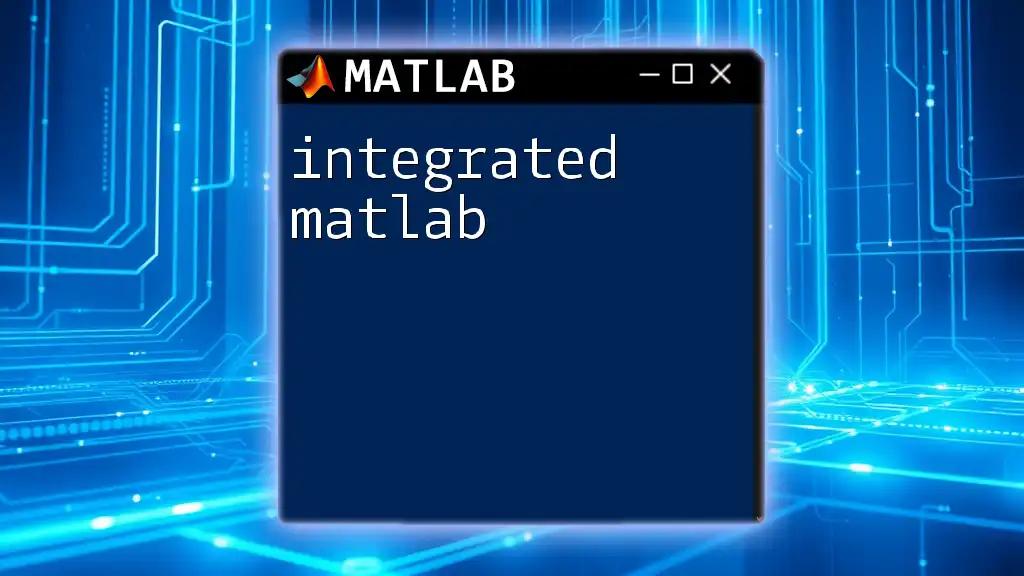
Understanding the Syntax of the `append` Command
Basic Syntax
When you use the `append` function, ensuring the dimensions of the inputs align is key to avoiding errors. For instance, when appending two arrays, they must have compatible dimensions.
Syntax for Different Data Types
Appending Arrays
To append arrays, simply use the concatenation operator. The `append` command does not exist explicitly as a function for arrays, so this method is common:
A = [1, 2, 3];
B = [4, 5, 6];
result = [A, B]; % Result: [1, 2, 3, 4, 5, 6]
This method horizontally concatenates the arrays `A` and `B`. For vertical concatenation, you can use:
result = [A; B]; % If A and B are column vectors
Appending Tables
When working with tables in MATLAB, the `append` function allows you to combine them efficiently. Here's how you can do it:
T1 = table([1; 2], [3; 4], 'VariableNames', {'A', 'B'});
T2 = table([5; 6], [7; 8], 'VariableNames', {'A', 'B'});
T3 = [T1; T2]; % Vertical concatenation
This method merges the rows of tables T1 and T2 into a new table T3.
Appending to Files
Appending data to files is another powerful application of the `append` command principles. Let's see how to append data to a text file:
fid = fopen('data.txt', 'a'); % Open in append mode
fprintf(fid, '%f\n', rand(5,1)); % Append random numbers
fclose(fid);
In this code, the file is opened in append mode, ensuring that any subsequent writes will not overwrite existing data.
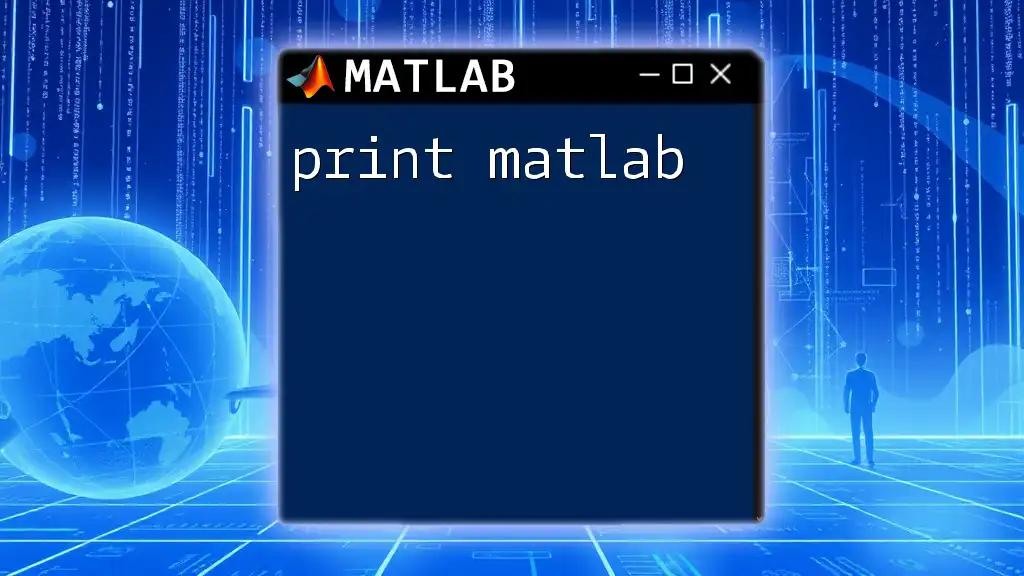
Practical Examples of Using `append`
Example 1: Appending Data to a Numeric Array
Consider a scenario where you're collecting test scores over multiple sessions. Here’s how you could manage that:
scores_session1 = [85, 90, 88];
scores_session2 = [92, 94, 91];
all_scores = [scores_session1, scores_session2]; % Append scores
In this example, all scores from different sessions are combined in a single array for easier analysis.
Example 2: Appending Data to a Table
If you are merging datasets from two separate experiments, you may encounter cases where the table structures must align properly:
Experiment1 = table([1; 2], ["A"; "B"], 'VariableNames', {'ID', 'Result'});
Experiment2 = table([3; 4], ["C"; "D"], 'VariableNames', {'ID', 'Result'});
CombinedResults = [Experiment1; Experiment2]; % Combining results
It's essential to ensure that variable names and types match, enabling a seamless integration of the data.
Example 3: Appending Data to a .mat File
If you're working with complex data types or larger datasets, storing them as `.mat` files is often preferred:
data1 = rand(10, 2); % Sample data
data2 = rand(5, 2);
save('mydata.mat', 'data1'); % Saving first dataset
append('mydata.mat', 'data2'); % Assuming a custom append functionality for .mat files
Note that MATLAB does not have a direct append command for .mat files; you typically have to load the existing data and re-save it. However, if you are familiar with file structures, you can manipulate them using low-level file operations.
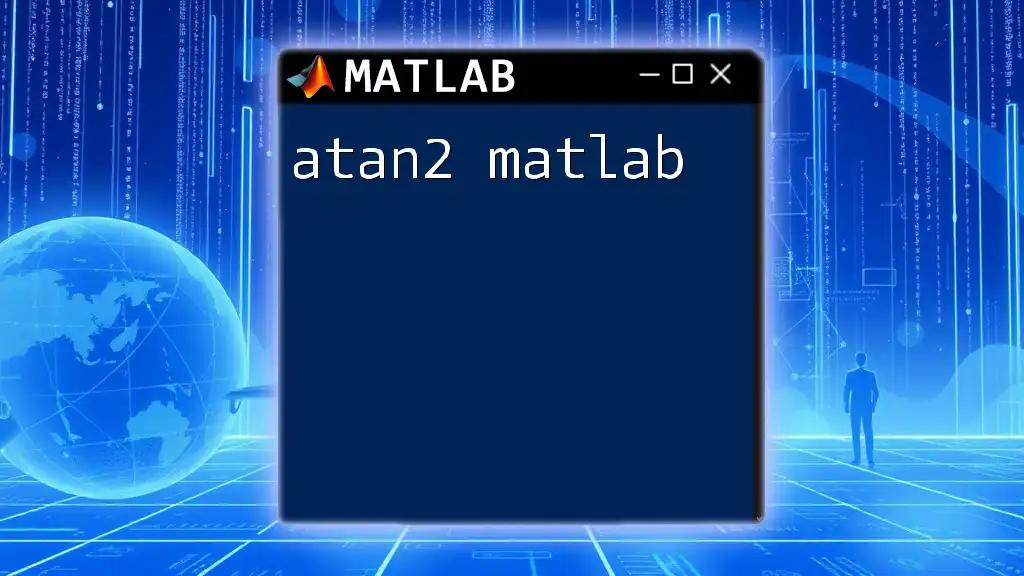
Common Errors and Troubleshooting
When using `append`, users may encounter certain errors.
-
Dimension Mismatch: Attempting to append arrays or tables with incompatible sizes will generate errors. Always check dimensions using `size()` before appending.
-
Variable Name Conflicts in Tables: If the tables contain columns with the same names but different data types, MATLAB will throw warnings. Clarifying data types before merging can prevent these issues.
A good practice is to always validate your datasets before appending:
if size(A, 2) == size(B, 2)
result = [A; B]; % Only append if dimensions are compatible
else
error('Dimension mismatch: A has %d columns, B has %d columns', size(A, 2), size(B, 2));
end

Performance Considerations
Appending data can have performance implications, particularly with very large datasets. Frequent appending can lead to suboptimal performance due to memory reallocation.
It's advisable to allocate memory beforehand when you know the total size required. For instance, if you're iteratively filling an array, pre-allocate it:
N = 100; % Total number of elements
data = zeros(1, N); % Pre-allocation for speed
for i = 1:N
data(i) = i; % Fill data
end
This method avoids the overhead associated with enlarging an array incrementally.
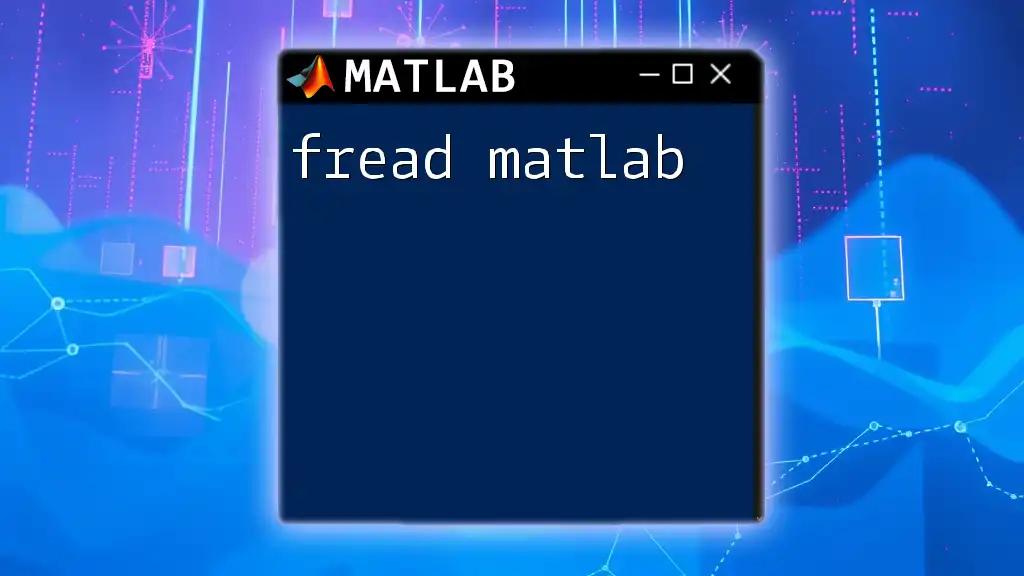
Conclusion
The `append` command in MATLAB is a vital tool for managing and maintaining datasets effectively. By mastering this command, users can ensure their data analysis is robust, organized, and reflective of their ongoing work, especially in fields that rely heavily on data accumulation.
Regular practice with hands-on examples and real-life applications will strengthen your grasp of the `append` command, making it an indispensable tool in your MATLAB skillset.
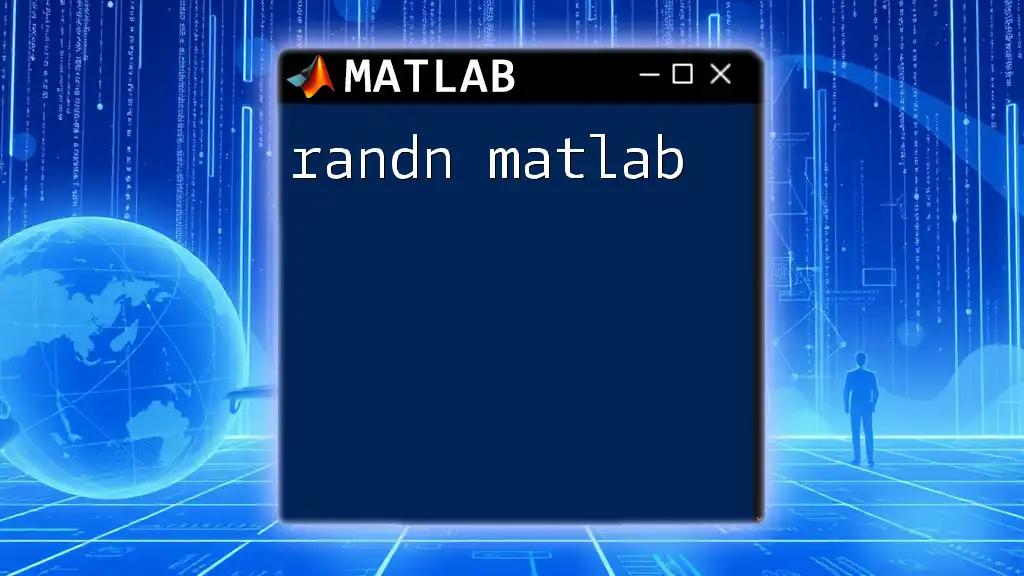
FAQs about the `append` Command
What happens if the dimensions do not match when appending arrays?
If you attempt to append arrays with incompatible sizes, MATLAB will throw an error indicating the mismatch in dimensions. It’s crucial to check dimensions using functions like `size()` before performing operations.
Can I append different data types within one table?
When appending different data types in a table, ensure that column names are unique and the data types align correctly. If not, MATLAB will raise warnings or errors.
How can I efficiently append data in a loop?
The best practice for appending data in a loop involves pre-allocating sufficient space for your data structure. This prevents unnecessary memory allocation and increases performance, allowing your script to run smoother and faster.
By leveraging the knowledge gained from this article, you'll be well on your way to effectively utilizing the `append` command in MATLAB to manipulate and organize your data successfully.