In MATLAB, you can visualize the contour of a 2D function using the `contour` command, which creates contour plots representing constant values of a matrix or function. Here’s a simple example:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
contour(X, Y, Z);
xlabel('X-axis');
ylabel('Y-axis');
title('Contour Plot of sin(sqrt(X^2 + Y^2))');
Understanding Contour Plots
What is a Contour Plot?
A contour plot is a graphical representation that illustrates the three-dimensional relationship between variables in a two-dimensional format. It uses contour lines to connect points of equal value, effectively encoding a third dimension (usually represented by the z-axis) within a two-dimensional plane (x and y axes). This type of visualization is crucial in various fields such as engineering, physical sciences, and data analysis, allowing for intuitive insights into the behavior of multi-dimensional data.
Key Terminology
- Contour Lines: These are the lines that represent the value of a third dimension. Each contour line connects locations that have the same value, making it easy to visualize gradients and slopes.
- Levels and Intervals: Levels refer to the specific values that the contour lines represent, while intervals define the spacing between these levels. Choosing the right intervals can greatly influence the readability and informative nature of your contour plot.
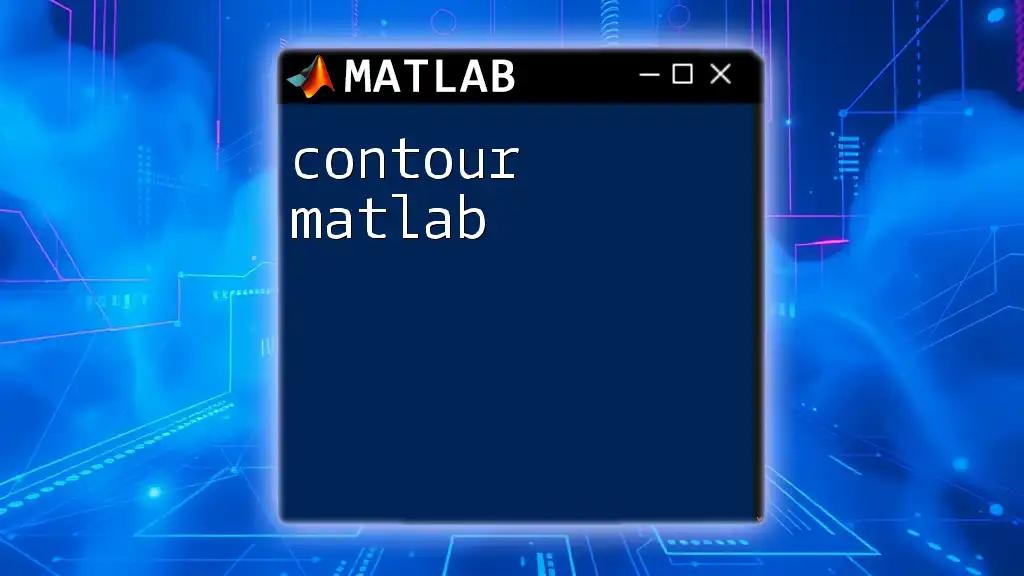
Setting Up Your MATLAB Environment
Installing MATLAB
Before you can start plotting contour in MATLAB, you need to ensure that you have the software installed. Installation typically involves downloading the installer from the MathWorks website and following the on-screen instructions. Make sure you have the latest version to utilize all available features for contour plotting.
Basic MATLAB Commands Review
Familiarizing yourself with some basic commands can ease your learning curve. Begin with the following commands:
- `clc`: Clears the command window.
- `clear`: Removes all variables from the workspace.
- `close all`: Closes all figure windows.
These commands help maintain a clean workspace, allowing you to focus on the task at hand.
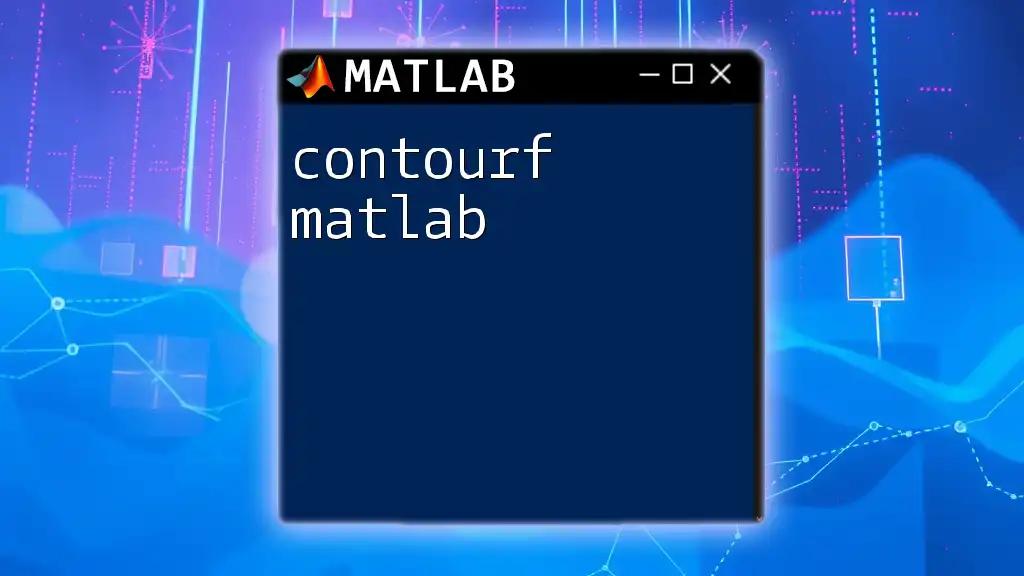
Creating a Basic Contour Plot
Working with Sample Data
To create a contour plot, you first need some sample data. You can generate a grid of data points using MATLAB's `meshgrid` function along with a function like `peaks` to simulate an example dataset.
[X, Y] = meshgrid(-3:0.1:3, -3:0.1:3);
Z = peaks(X, Y); % Sample data
In the above code, `meshgrid` creates a grid of x and y coordinates ranging from -3 to 3, while the `peaks` function generates corresponding z-values based on a predefined mathematical function.
Using the `contour` Function
To create a basic contour plot, use the `contour` function. This command takes your x, y, and z matrices as inputs.
contour(X, Y, Z);
title('Basic Contour Plot');
xlabel('X-axis');
ylabel('Y-axis');
In this code snippet, `contour(X, Y, Z)` generates the contour plot of the data, while `title`, `xlabel`, and `ylabel` functions add labels and a title to the plot.
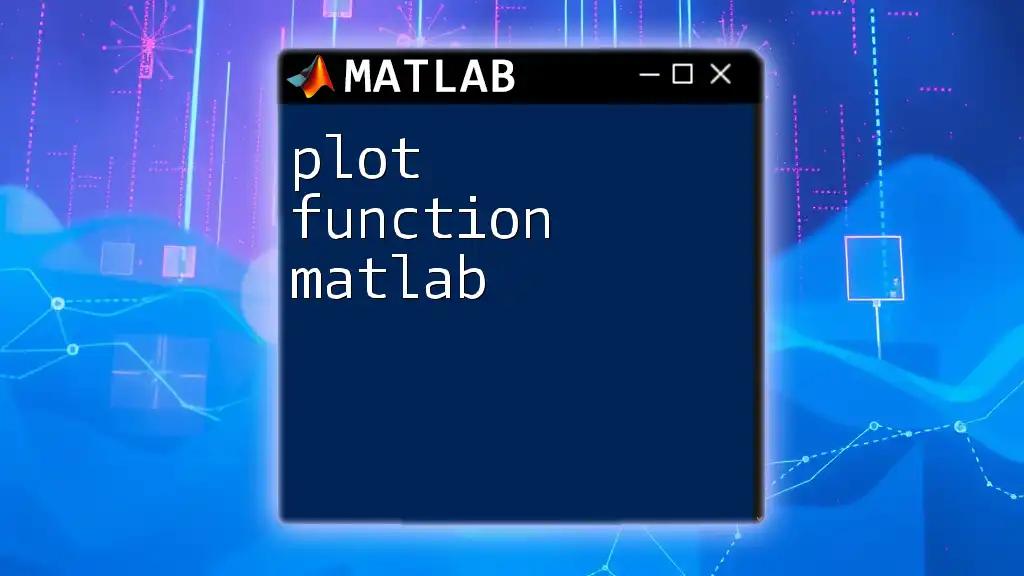
Customizing Your Contour Plot
Changing Line Styles and Colors
To enhance the visual appeal and readability of your contour plot, you can customize the color scheme. The `colormap` function changes the color palette, and `caxis` adjusts the scale of the color mapping.
colormap(jet);
This code changes the contour color map to the "jet" palette, providing a smooth gradient that highlights variations in the data.
Adding Contour Labels
Adding labels to the contour lines can help in interpreting the data represented by each level of the contour plot. You can easily enable contour labels by using the `ShowText` parameter in the `contour` function.
contour(X, Y, Z, 'ShowText', 'on');
This modification labels each contour line, allowing you to quickly reference the respective z-values at different points.
Adjusting Axis Limits
For better clarity, it’s important to set appropriate axis limits. You can define the limits of your x and y axes using the `xlim` and `ylim` functions, respectively. This helps focus the visualization on the regions of interest in the data.
xlim([-3 3]);
ylim([-3 3]);
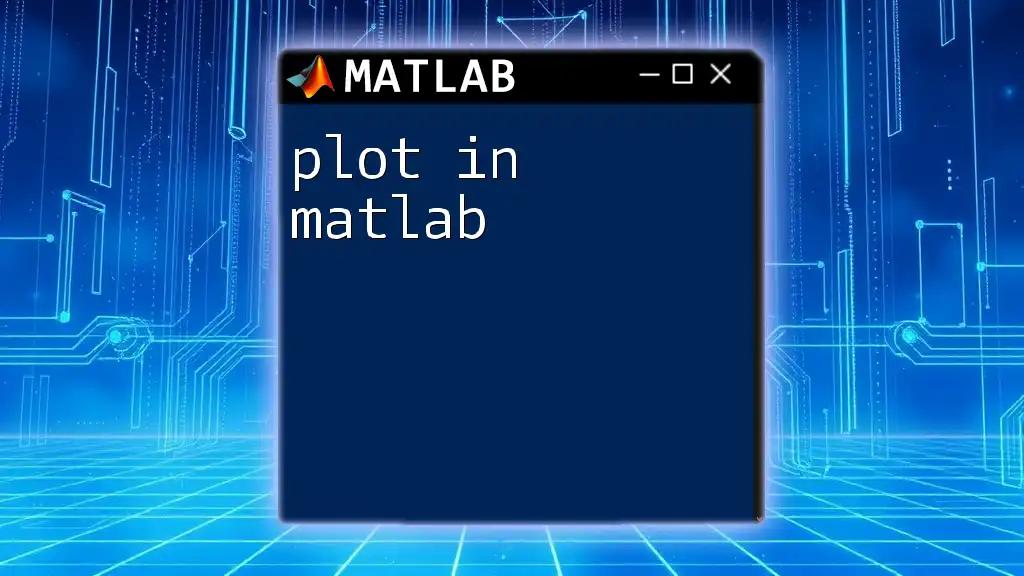
Advanced Contour Plot Techniques
Filled Contour Plots
Beyond basic contour plots, filled contour plots can provide more information at a glance. Use the `contourf` function to create a filled version of your contour visualization.
contourf(X, Y, Z);
Filled contours allow you to easily see gradients and areas of interest in your data, as each region between contour lines is filled with color.
3D Surface Visualization
To complement your contour analysis, you can visualize the same dataset in three dimensions using the `surf` function. This adds depth to your understanding of how the variables interact in a multi-dimensional space.
surf(X, Y, Z);
The 3D surface plot offers a tactile sense of height and depth, making it easier to spot peaks, valleys, and slopes in data distributions.
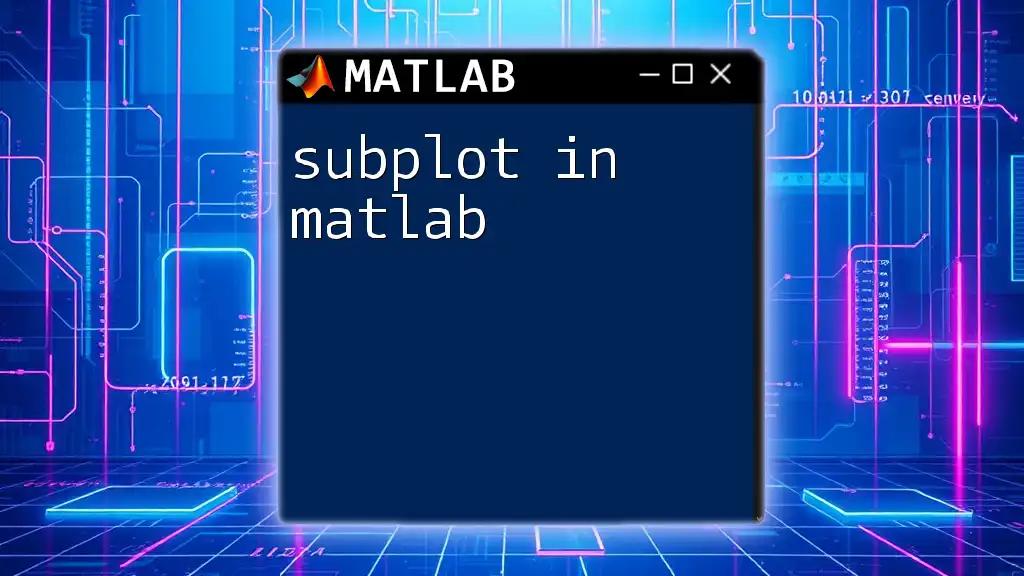
Real-World Applications of Contour Plots
Engineering Design
Contour plots are extensively used in engineering fields to analyze fluid dynamics or mechanical stress. For example, engineers may use contour maps to visualize pressures over a given area, enabling them to make more informed design decisions.
Environmental Science
In environmental studies, contour plots can visually represent topography, such as elevation maps and other geospatial data, allowing scientists to analyze habitats or ecosystems based on terrain and elevation changes.
Data Analysis
In data analysis, contour plots can summarize complex datasets for statistical interpretation. By visualizing data density or any multi-variable relationship, analysts can gain insights that inform decision-making processes in business and research.
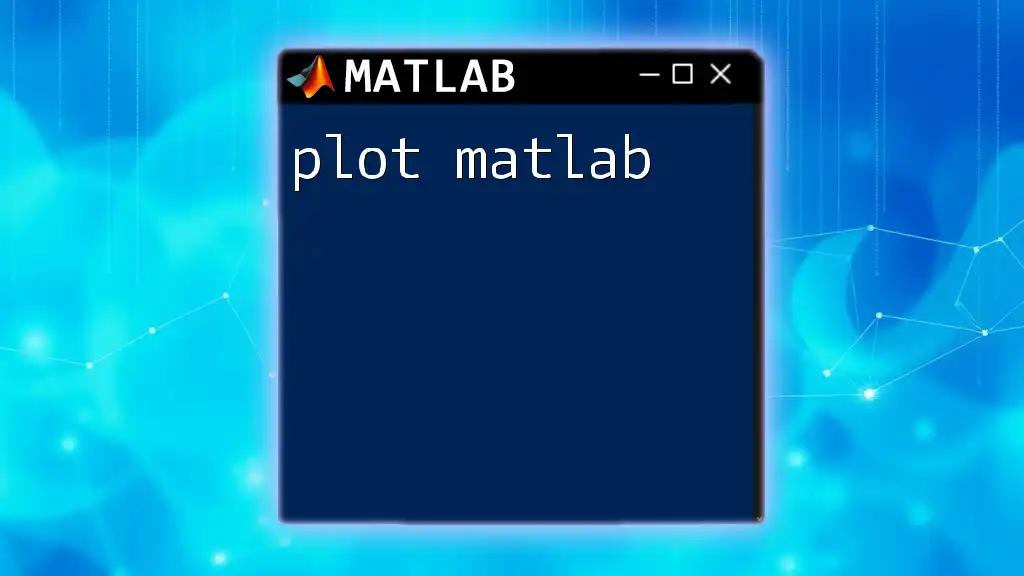
Troubleshooting Common Issues
Common Errors and Solutions
While working on your contour plot, you may encounter several common mistakes. These can include issues such as incorrect data dimensions or improper use of functions. To address these:
- Ensure your x, y, and z matrices have compatible dimensions.
- Double-check your function syntax for any typographical errors.
By writing clean, tested code and referring to the MATLAB documentation, you can troubleshoot and refine your contour plots effectively.
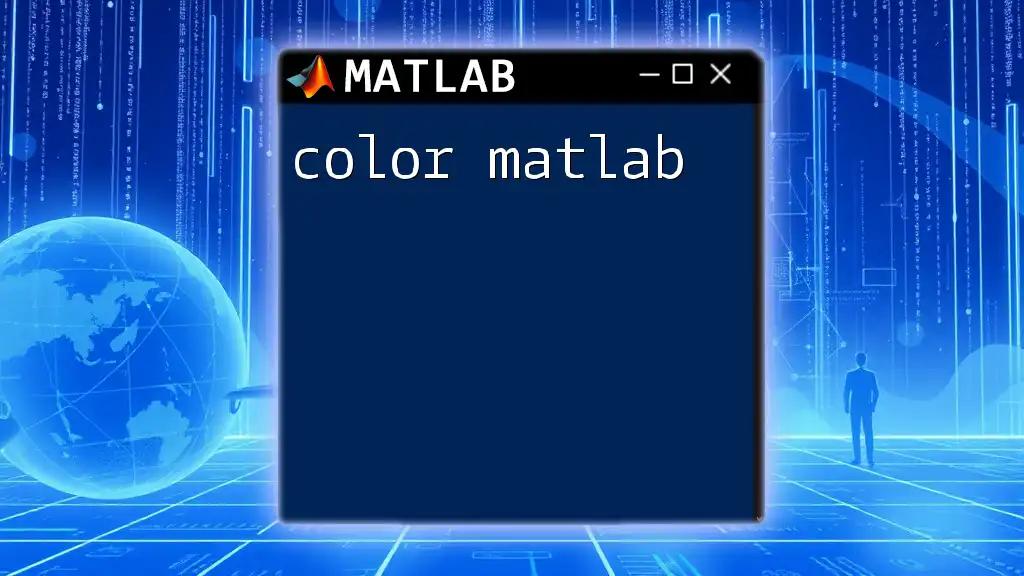
Conclusion
Understanding how to plot contour in MATLAB not only enhances your data visualization techniques but also equips you with a valuable skill applicable across various disciplines. By mastering the creation and customization of contour plots, you can present complex relationships in an easily interpretable format. As you build your skills, don’t hesitate to explore further resources, documentation, and user communities to expand your MATLAB journey.
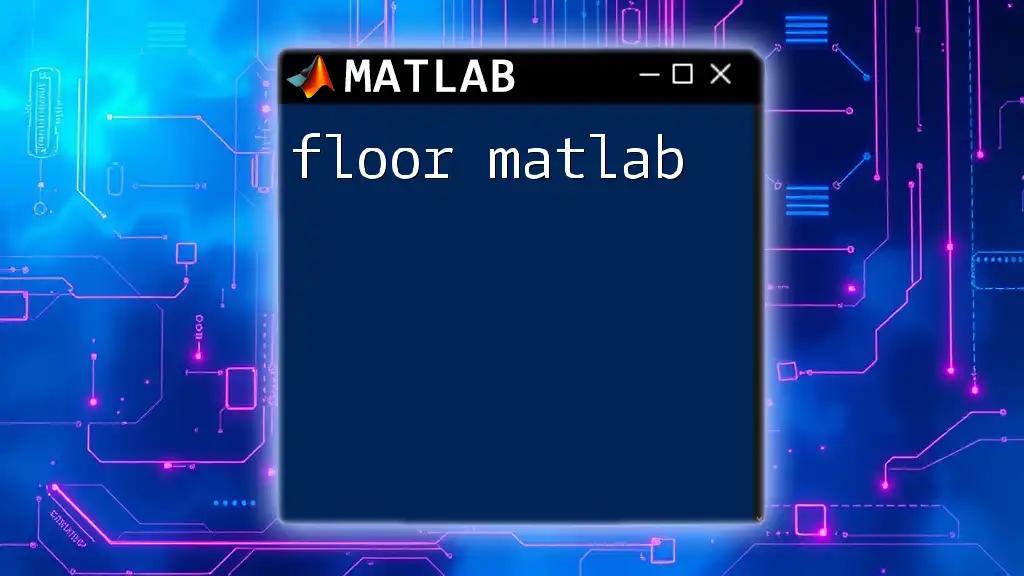
Additional Resources
For continued learning, consider exploring the official MATLAB documentation for contour plotting, online tutorials, and forums where MATLAB users gather to share insights and support each other. Engaging with the community can significantly accelerate your learning and provide additional perspectives on using MATLAB for data visualization.