`linprog` in MATLAB is a function used for linear programming that helps solve optimization problems by minimizing a linear objective function subject to linear equality and inequality constraints.
Here's a code snippet demonstrating how to use `linprog`:
f = [1; 2]; % Coefficients of the objective function
A = [1, 1; -1, 2; 2, 1]; % Coefficients for the inequality constraints
b = [3; 2; 4]; % Right-hand side of the inequality constraints
lb = [0; 0]; % Lower bounds for the variables
[x, fval] = linprog(f, A, b, [], [], lb); % Call linprog to solve the problem
disp('Optimal solution:');
disp(x);
disp('Minimum value of the objective function:');
disp(fval);
What is Linear Programming?
Linear programming (LP) is a mathematical method for determining a way to achieve the best outcome in a given mathematical model, which is typically expressed as a linear relationship. LP is widely used across various fields, including operations research, economics, and engineering, due to its powerful capability to solve optimization problems. By establishing an objective function to maximize or minimize—often tied to costs, profits, or efficiency—linear programming helps businesses and researchers make informed decisions.
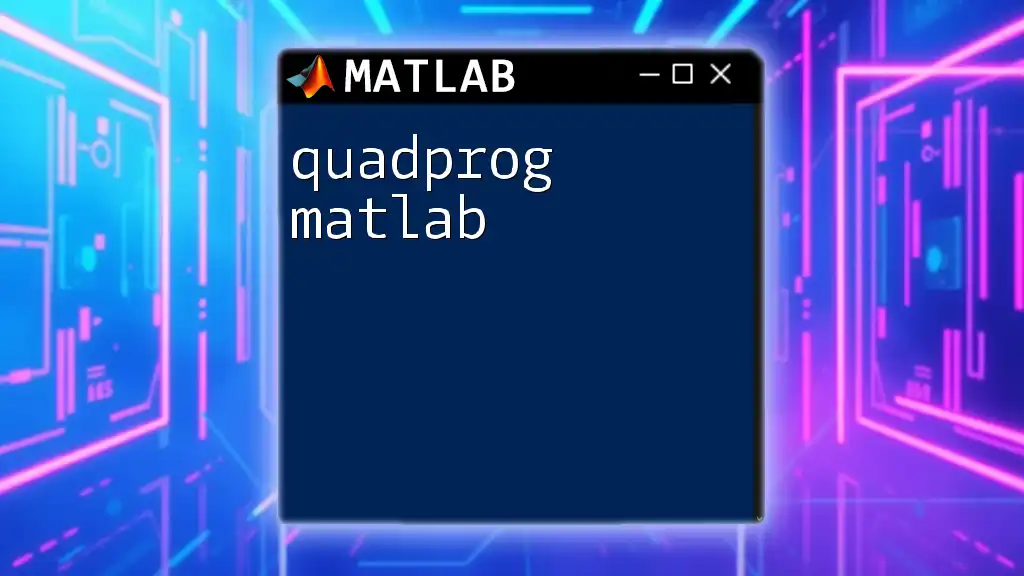
Overview of `linprog` Function
In MATLAB, the `linprog` function is the primary tool for solving linear programming problems. It is designed to find the values of variables that minimize a linear objective function while satisfying a set of linear inequalities and equalities. This function seamlessly integrates into MATLAB's environment, making it a popular choice among engineers and data scientists.
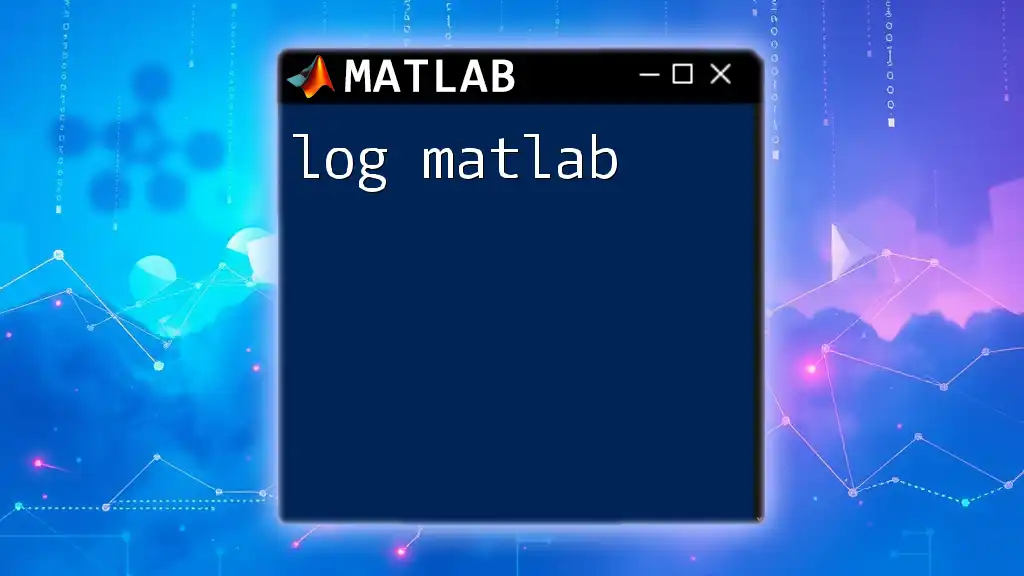
Getting Started with `linprog`
Prerequisites for Using `linprog`
Before diving into `linprog`, ensure you have a basic understanding of MATLAB and are familiar with linear programming concepts. Being comfortable with MATLAB’s interface and syntax will make learning `linprog` more straightforward.
Installing MATLAB
If you're new to MATLAB, you’ll first need to install it. Follow these steps:
- Visit the official MathWorks website.
- Choose the appropriate MATLAB package for your needs.
- Download and install the software.
- Ensure you have access to the Optimization Toolbox, which includes `linprog`.
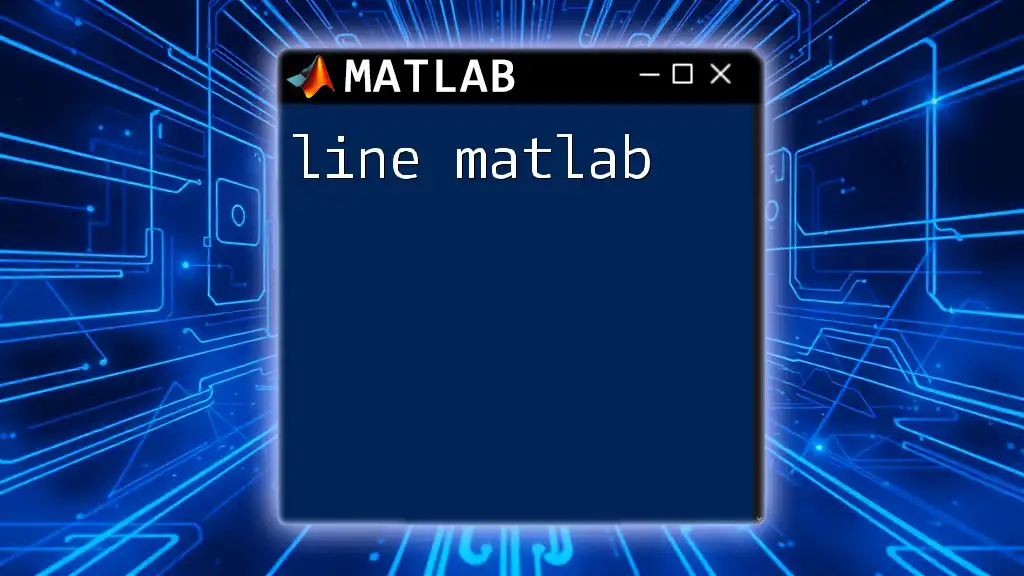
Understanding the Syntax of `linprog`
The basic syntax of the `linprog` function is as follows:
x = linprog(f, A, b, Aeq, beq, lb, ub)
Parameters Explained
Objective Function: `f`
The objective function defines what you are trying to minimize or maximize. The vector `f` contains the coefficients for the variables in your objective function. For example, if you're minimizing costs in a manufacturing scenario, the coefficients may represent the costs associated with different products.
Inequality Constraints: `A` and `b`
These define the boundaries within which the solution can exist. The constraints are expressed in the form:
\[ Ax \leq b \]
- `A` is a matrix where each row represents a constraint.
- `b` is a vector where each element corresponds to the limit of each constraint.
Equality Constraints: `Aeq` and `beq`
These constraints ensure that certain relationships hold exactly:
\[ Aeq \cdot x = beq \]
Like `A` and `b`, `Aeq` specifies the coefficients of the equality constraints, while `beq` contains their corresponding right-hand side values.
Lower and Upper Bound Constraints: `lb` and `ub`
These parameters restrict the variable values. Setting boundaries enables you to model real-world conditions such as minimum production rates or resource limits. If a variable can only take positive values, you would define it through the lower bound.
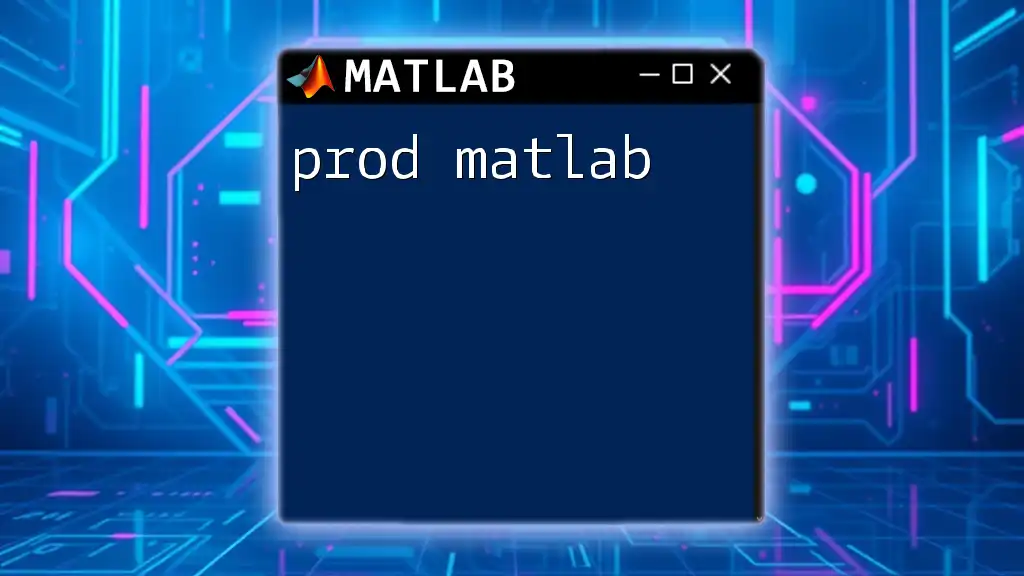
Setting Up a Simple Linear Programming Problem
Example Problem Statement
Let’s consider a simple problem:
Objective: Minimize the costs of producing two products (let's call them Product A and Product B) under specific constraints related to resources.
Let’s define the problem using the following data:
-
Objective Function Coefficients: `f = [2; 3]`, where 2 is the cost of Product A and 3 is the cost of Product B.
-
Resource Constraints:
- We can use up to 4 units of resources, defined as \(x_1 + x_2 \leq 4\).
- The second constraint allows up to 6 units, defined as \(2x_1 + x_2 \leq 6\).
-
Non-negativity Constraints: Both products cannot be produced in negative quantities, meaning \(x_1 \geq 0\) and \(x_2 \geq 0\).
Formulating the Problem
To implement this problem in MATLAB, we would define the following:
f = [2; 3]; % Coefficients for x1 and x2
A = [1, 1; 2, 1]; % Coefficients for inequality constraints
b = [4; 6]; % Limits for the inequalities
lb = [0; 0]; % Non-negativity constraints
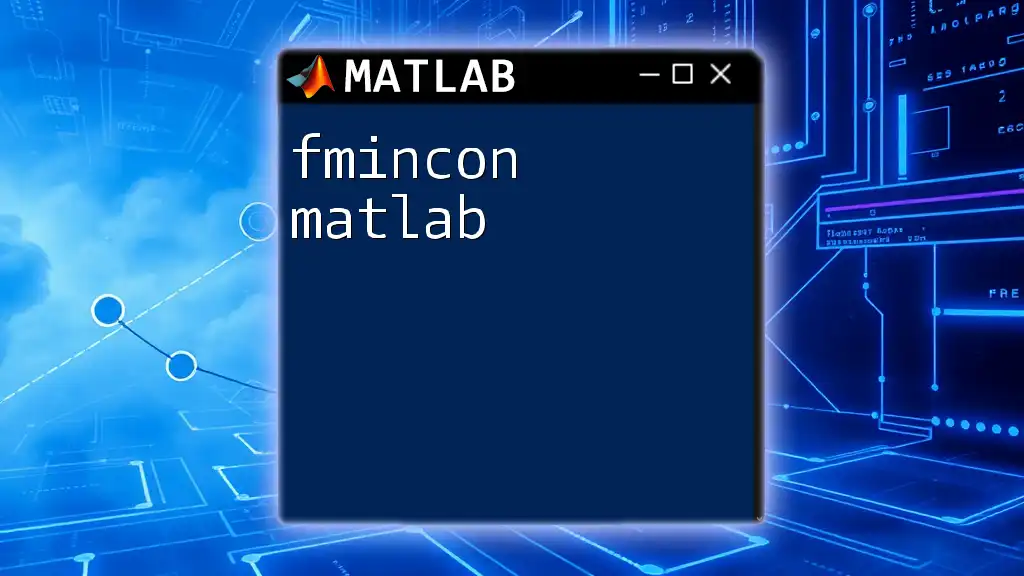
Running the `linprog` Function
Executing the Command
Now that we have formulated our problem, we can solve it using the `linprog` function. Here’s how:
options = optimoptions('linprog', 'Display', 'final'); % Set display options
[x, fval] = linprog(f, A, b, [], [], lb, []);
In this code snippet:
- `options` allows us to specify how much information we want from MATLAB during optimization.
- `x` is the vector of optimal production levels for Products A and B.
- `fval` gives us the minimum cost achieved.
Interpreting the Output
After running the `linprog` function, MATLAB returns the solution vector `x`. For instance, if the output is:
x =
2
2
This means the optimal production levels are 2 units of Product A and 2 units of Product B. The `fval` output gives you the minimum cost at that production level, which can be evaluated by substituting `x` back into the objective function.
Handling Warnings and Errors
While using `linprog`, you may encounter warnings or errors, such as infeasible solutions or unbounded solutions. Common issues include:
- Infeasibility: This arises when no solutions meet the constraints. Review your constraints to ensure they are not contradictory.
- Unbounded solutions: Occur when the feasible region is not bounded. Analyze your inequality constraints for completeness.
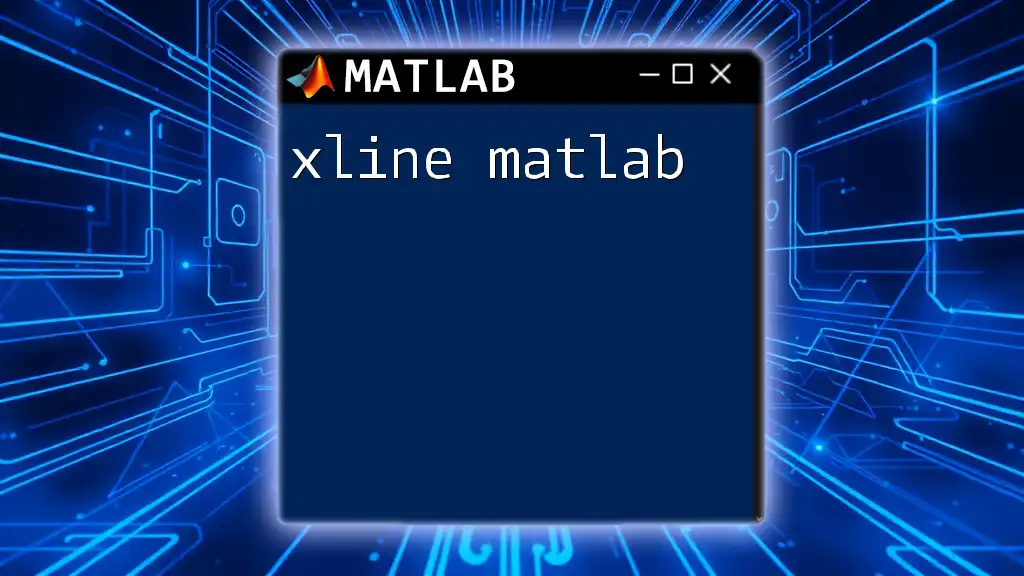
Advanced Usage of `linprog`
Using Options for Customization
The `linprog` function allows multiple customization options, enabling users to adjust settings to enhance performance and convergence:
options = optimoptions('linprog','Algorithm','dual-simplex', 'MaxIterations',500);
This command specifies using the dual-simplex algorithm, which is often more efficient for certain types of problems. You can adjust `MaxIterations` based on your specific needs.
Dealing with Large Scale Problems
For complex problems with a significant number of variables and constraints, you may want to:
- Utilize sparse matrices to improve computational efficiency.
- Apply different algorithms available in MATLAB for optimization.
Sensitivity Analysis
Sensitivity analysis is essential for understanding how changes in input parameters affect the optimal solution. Techniques include:
- Varying coefficients within `f` and observing changes in `x` and `fval`.
- Using the `sensitivity` function to explore how changes in constraints affect outcomes.
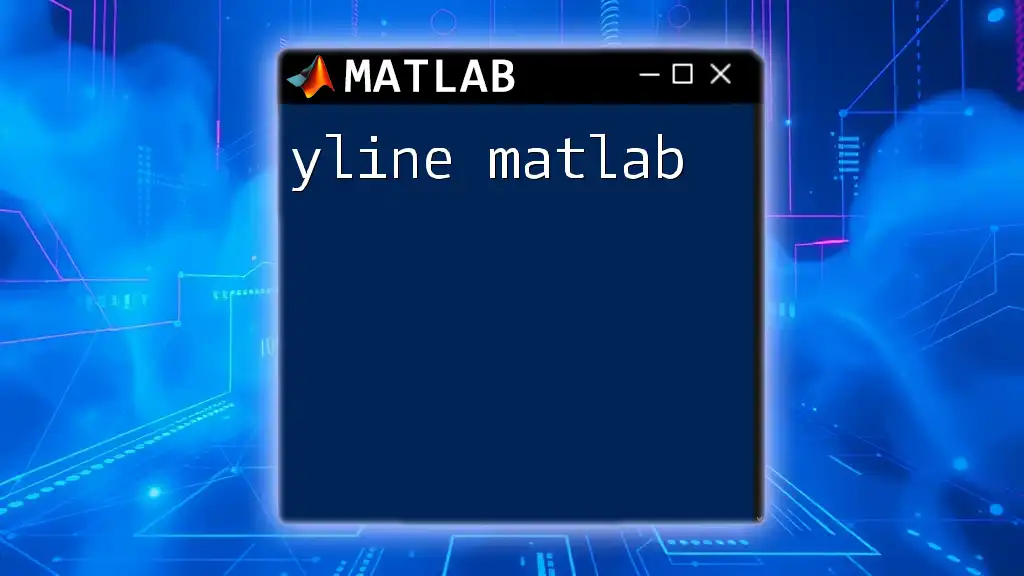
Real-World Applications of `linprog`
Case Studies
`linprog` has found applications in various industries:
- Finance: Portfolio optimization where objective functions aim to minimize risk for a given level of return.
- Manufacturing: Production planning to minimize costs while satisfying demand constraints.
- Logistics: Vehicle routing problems aiming to minimize transportation costs and time constraints.
Academic Research Contributions
Many researchers in fields like operations research and economics utilize `linprog` in their work, creating models to test theories and simulations concerning resource allocation, cost minimization, and more.
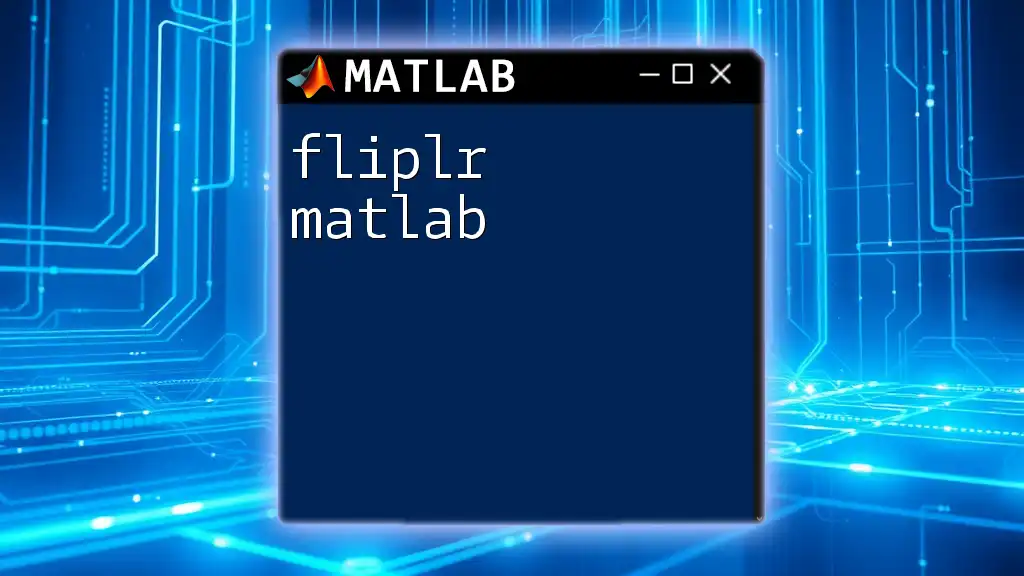
Conclusion
In this guide, you've explored the powerful capabilities of the `linprog` function in MATLAB for linear programming. From formulating your problem and executing the command to interpreting results and applying it to real-world challenges, mastering `linprog` can significantly improve decision-making processes in various domains.
Resources for Further Learning
For those looking to expand their knowledge further, consider exploring the official [MATLAB documentation](https://www.mathworks.com/help/optim/ug/linprog.html) on `linprog`. Additional resources, including online courses and tutorials, may also provide added insights into optimization techniques.
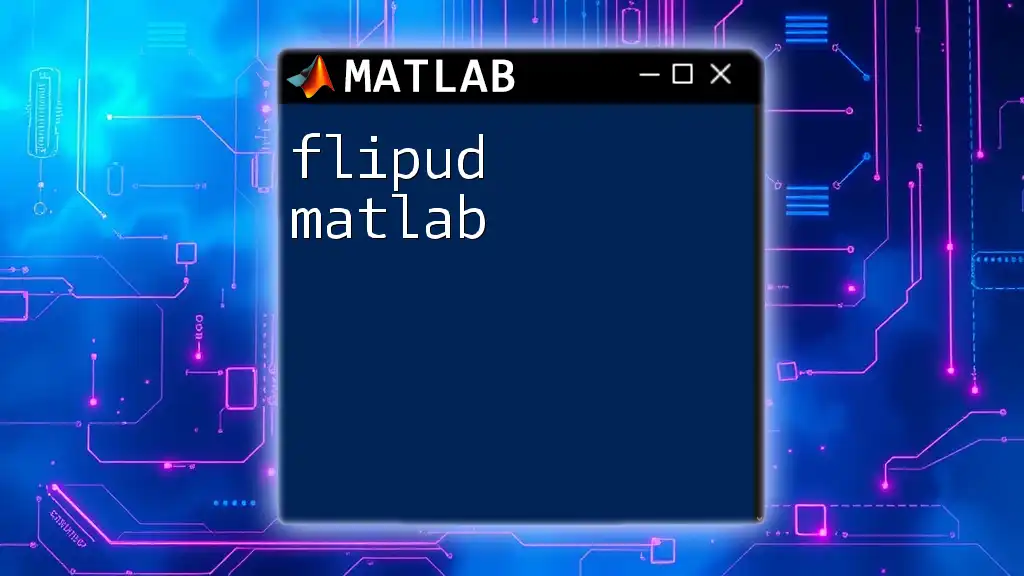
FAQs about `linprog`
-
What type of problems can `linprog` solve? `linprog` can efficiently solve problems involving linear constraints and linear objective functions, such as resource allocation and dual pricing models.
-
Can `linprog` handle non-linear constraints? No, `linprog` is specifically designed for linear problems. For non-linear constraints, consider using MATLAB's `fmincon` or `fminunc` functions.
-
How to interpret solution infeasibility messages? An infeasibility message indicates that no solution exists that meets all constraints. Revisit constraints and ensure there are no contradictions.