In MATLAB, you can compare two strings to determine if they are identical using the `strcmp` function, which returns `true` if the strings match and `false` otherwise.
result = strcmp('apple', 'APPLE'); % This will return false because the comparison is case-sensitive.
What is String Comparison in MATLAB?
String comparison refers to the ability to determine relationships between strings based on specific criteria in MATLAB. This functionality is crucial for a wide range of applications, including data analysis, input validation, and conditional programming. Understanding how to compare strings effectively can help you make more informed decisions in your coding projects.
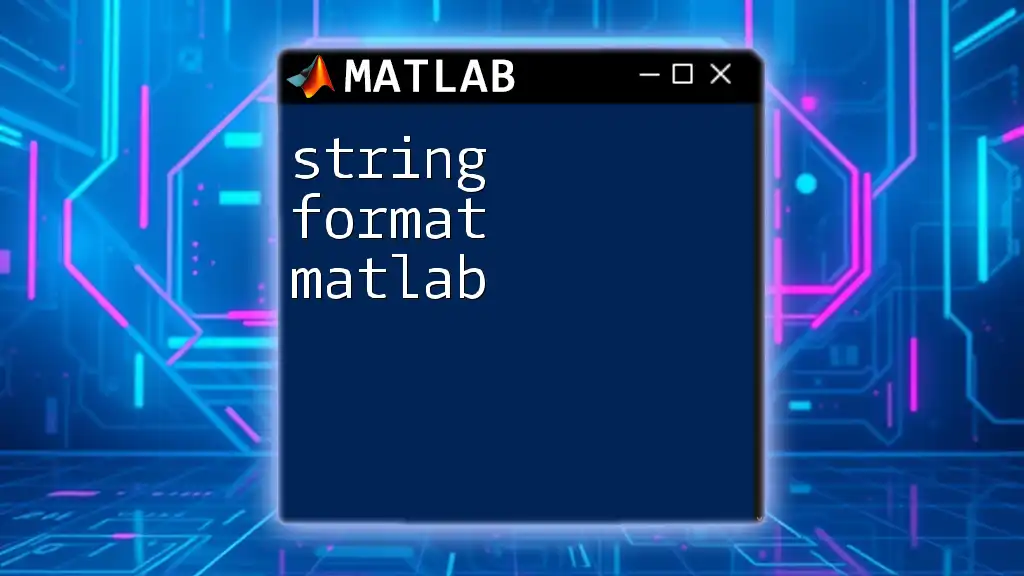
Key MATLAB Functions for String Comparison
strcmp
The `strcmp` function is the cornerstone for comparing two strings in MATLAB for equality. It returns a logical value: true if the strings are identical, and false otherwise.
Syntax:
strcmp(s1, s2)
Example:
s1 = 'Hello';
s2 = 'Hello';
result = strcmp(s1, s2); % Returns true
This example shows that both strings are identical. Always remember that `strcmp` is case-sensitive, meaning 'Hello' and 'hello' would return false. This function is particularly useful when you need to confirm user inputs or validate string data.
strcmpi
For scenarios where case should not matter, the `strcmpi` function offers a case-insensitive string comparison.
Syntax:
strcmpi(s1, s2)
Example:
s1 = 'Hello';
s2 = 'hello';
result = strcmpi(s1, s2); % Returns true
In this case, `strcmpi` understands that the two strings are equivalent, making it ideal for user input validations where casing may vary.
strcmpeq
The `strcmpeq` function extends the capabilities of traditional comparisons by treating strings as equal only if they are identical in both content and case.
Syntax:
strcmp(s1, s2, 'equal')
Example:
s1 = 'World';
s2 = 'world';
result = strcmp(s1, s2, 'equal'); % Returns false
This function is useful when exact matches are required, ensuring that no discrepancies in casing will allow for a false positive in string equality checks.
strncmp
The `strncmp` function allows for comparing the first N characters of two strings. This can be particularly useful when you only need to confirm that the prefixes of two strings match.
Syntax:
strncmp(s1, s2, N)
Example:
s1 = 'MATLAB programming';
s2 = 'MATLAB coding';
result = strncmp(s1, s2, 6); % Returns true
By comparing just the first six characters, you can quickly verify similar beginnings in string data.
strncmpi
Just like `strncmp`, but case-insensitive, `strncmpi` checks the first N characters of two strings without regard for the case.
Syntax:
strncmpi(s1, s2, N)
Example:
s1 = 'MATLAB';
s2 = 'matlab rocks';
result = strncmpi(s1, s2, 6); % Returns true
This is beneficial in situations where you might have prefixes that one user inputs in uppercase while another uses lowercase.
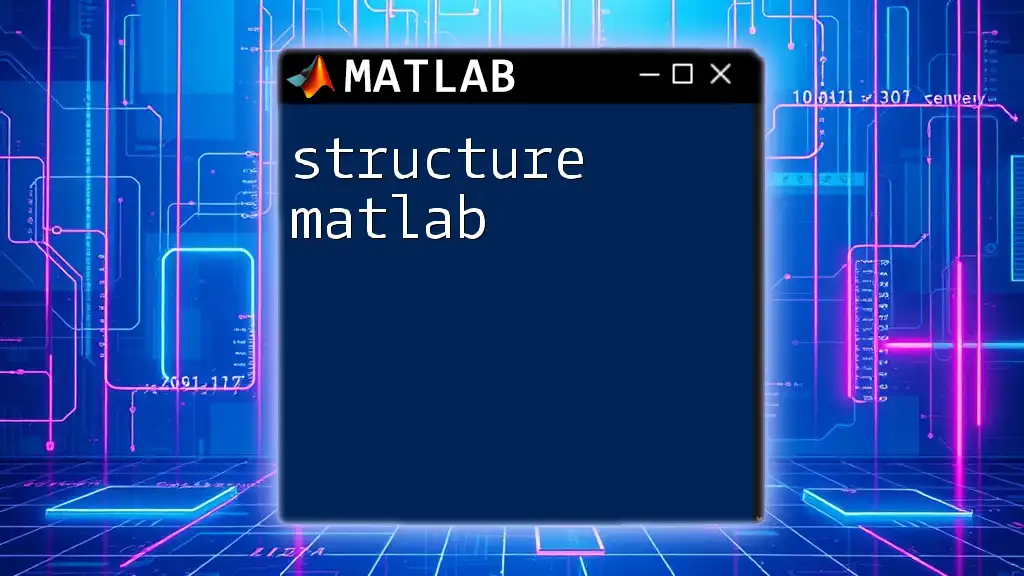
Advanced String Comparison Techniques
Using loops for custom comparisons
For more complex scenarios where built-in functions may not suffice, you can create custom string comparison functions.
Example:
function is_equal = customStrcmp(s1, s2)
is_equal = true;
if length(s1) ~= length(s2)
is_equal = false;
else
for i = 1:length(s1)
if s1(i) ~= s2(i)
is_equal = false;
break;
end
end
end
end
In this implementation, the function performs a character-by-character comparison. This method can be tailored to include specific requirements or additional complexity, such as ignoring certain characters.
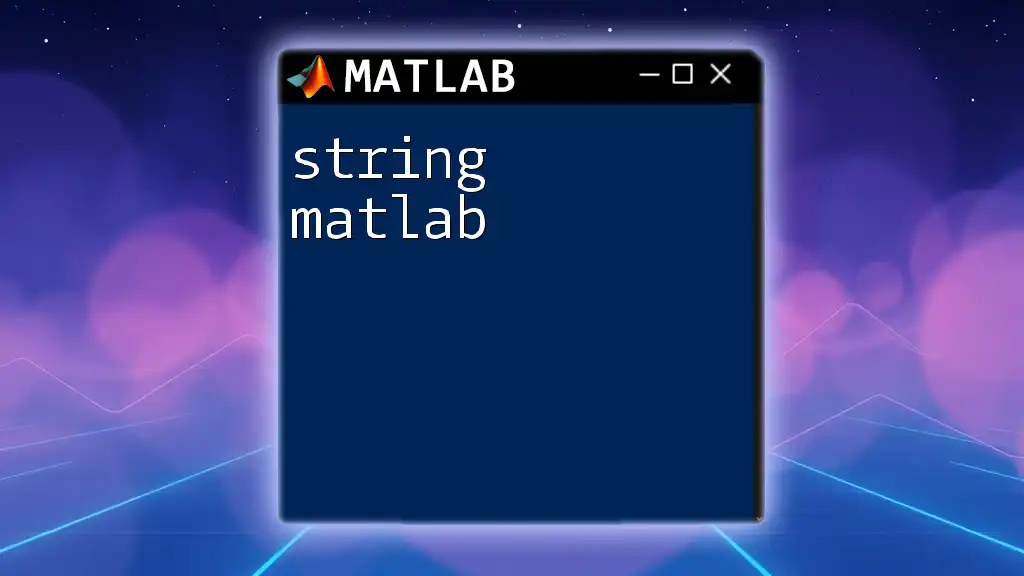
Common Pitfalls
Case Sensitivity
When utilizing the string comparison functions, it's essential to remember that many of them are case-sensitive. If you forget this, you may inadvertently fail to recognize strings that are logically equivalent.
Whitespace Issues
Whitespace can significantly affect string comparisons. For instance, comparing the strings 'Hello ' (with a space) and 'Hello' will yield false. Before performing comparisons, it’s advisable to trim whitespace using:
strtrim(inputString)
This allows for a cleaner comparison and avoids unnecessary errors.
Character Encoding Conflicts
Another common pitfall arises from different character encodings. If two strings are represented differently in terms of encoding (like Unicode vs. ASCII), comparisons may lead to unexpected results. Always ensure consistency in your string data encoding.
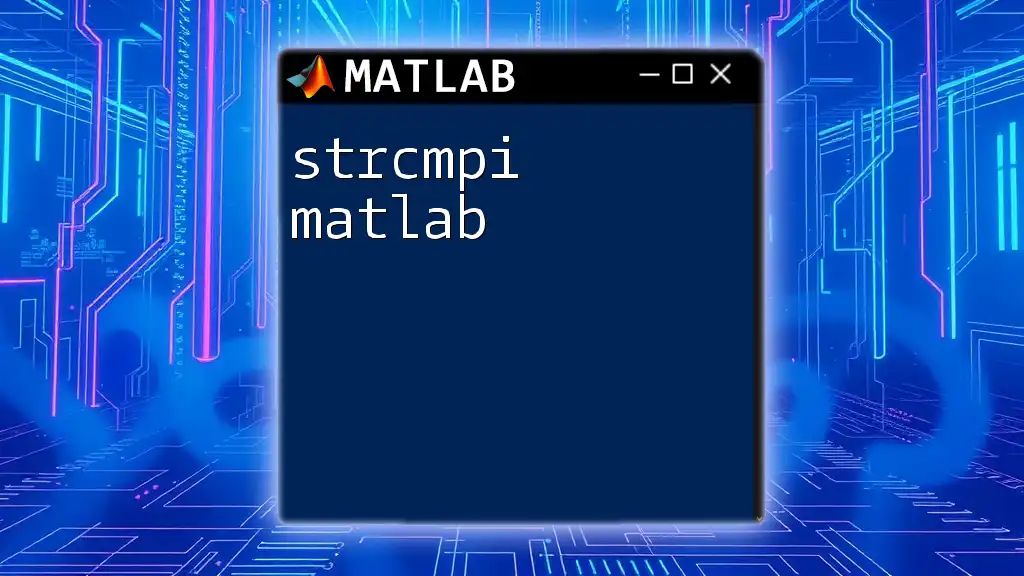
Best Practices for String Comparison in MATLAB
To maximize efficiency and accuracy in your MATLAB programming, adhere to these best practices:
-
Maintain Consistency: Always use the same casing format for string inputs when possible, as this prevents unnecessary complications and ensures smoother comparisons.
-
Trimming Strings: Utilize `strtrim` to remove unnecessary whitespace before conducting any comparison to ensure that only meaningful content is evaluated.
-
Choosing the Right Function: Be conscious of which comparison function best suits your needs. Case sensitivity and specific comparison criteria should guide your decision.
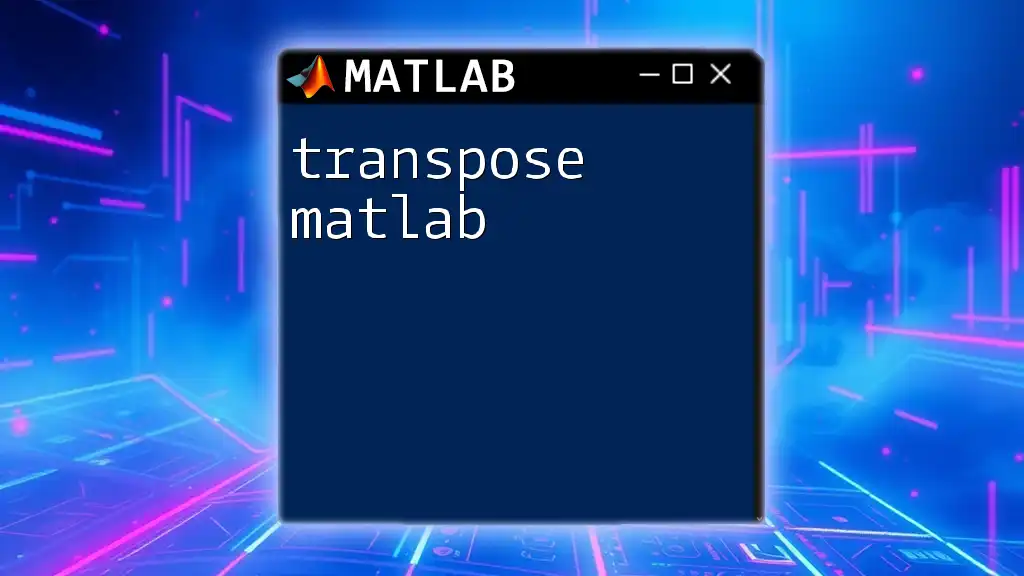
Conclusion
String comparison is an essential element in MATLAB programming, helping to validate data and make critical decisions based on input. By mastering the various functions available for string comparison—such as `strcmp`, `strcmpi`, `strncmp`, and others—you can ensure that your code effectively handles string data. Always remember to consider case sensitivity and whitespace to avoid common pitfalls, enabling you to write more robust and reliable MATLAB applications.
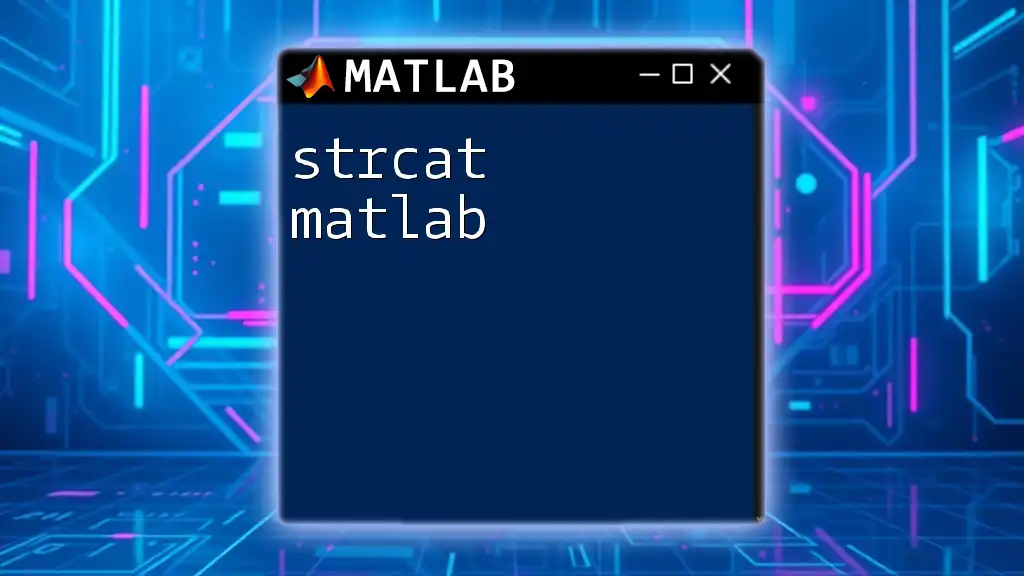
Additional Resources
For a deeper understanding of string manipulation and comparisons in MATLAB, refer to the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/strcmp.html). Exploring tutorials and community discussions can also enhance your know-how and practical skills.