The `normrnd` function in MATLAB generates random numbers from a normal distribution specified by the mean and standard deviation.
Here’s a simple example of how to use the `normrnd` function:
% Generate 5 random numbers from a normal distribution with mean 0 and standard deviation 1
randomNumbers = normrnd(0, 1, [1, 5]);
Understanding Normal Distribution
Basics of Normal Distribution
The normal distribution is a fundamental concept in statistics, characterized by its symmetrical bell-shaped curve. It signifies that most observations cluster around the central peak and probabilities taper off as values move away from the mean. This distribution is critical in various statistical methods and applications.
Parameters of Normal Distribution
To effectively use the normal distribution, you need to understand its parameters:
-
Mean (μ): This is the average of the distribution and determines the location of the center of the graph.
-
Standard Deviation (σ): A measure of the spread or dispersion of the distribution. A smaller standard deviation indicates that the data points are closer to the mean, while a larger standard deviation suggests a wider spread.
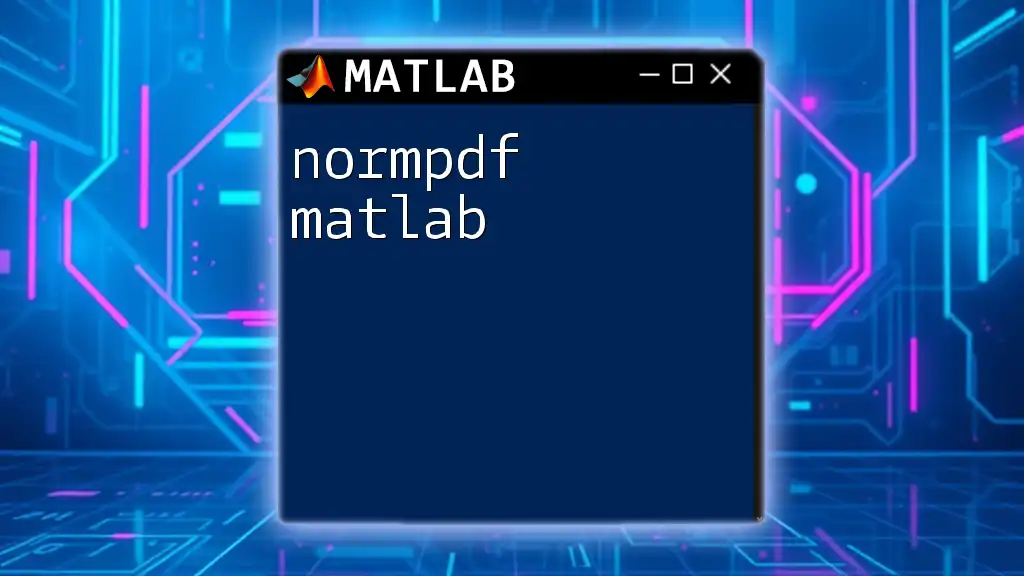
Using `normrnd` in MATLAB
Function Syntax
In MATLAB, the `normrnd` function is used to generate random numbers from a normal distribution. The basic syntax is as follows:
R = normrnd(mu, sigma, n, m)
-
R: The resulting matrix of random numbers.
-
mu: The mean of the normal distribution.
-
sigma: The standard deviation of the distribution.
-
n, m: These parameters define the dimensions of the output matrix.
Parameters Explained
Understanding the parameters is essential for using `normrnd` effectively:
-
mu: A scalar value or an array defining the means of your normal distributions.
-
sigma: Likewise, it can be a scalar or an array where each entry corresponds to the standard deviation of the respective mean.
-
n, m: These are the number of rows and columns of the output matrix, giving you control over the size of your generated data.
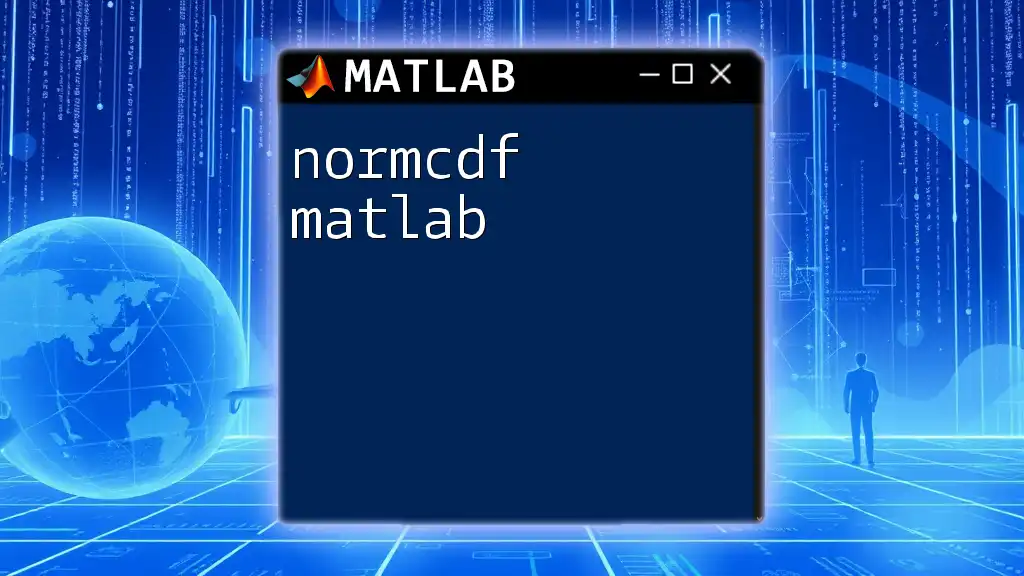
Examples of `normrnd` Usage
Example 1: Basic Random Number Generation
Let’s start with a simple example of generating random numbers from a standard normal distribution where the mean is 0 and the standard deviation is 1.
mu = 0;
sigma = 1;
R = normrnd(mu, sigma, 1, 10);
disp(R);
In this example, `R` will contain 10 random numbers drawn from the standard normal distribution. This is an effective way to create sample data for statistical analysis.
Example 2: Generating a Matrix of Random Numbers
Now, let’s generate a matrix of random numbers with different mean and standard deviation values.
mu = 5;
sigma = 2;
R = normrnd(mu, sigma, 5, 5);
disp(R);
Here, the output will be a 5x5 matrix of random numbers where each number is generated based on a normal distribution with a mean of 5 and a standard deviation of 2. This can be particularly useful when you need a larger dataset for simulations or modeling.
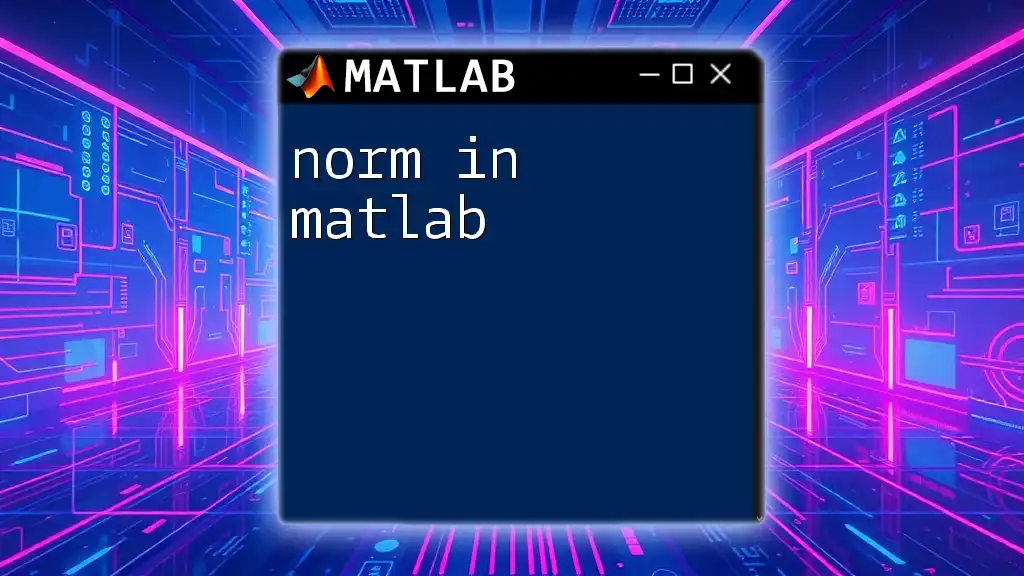
Visualizing Data from `normrnd`
Plotting Random Samples
Visual representation is key for understanding statistical data. By plotting the generated data alongside the theoretical normal distribution, you can gain insights into how well your random samples represent what you expect.
mu = 0;
sigma = 1;
R = normrnd(mu, sigma, 1000, 1);
histogram(R, 'Normalization', 'pdf');
hold on;
x = -4:0.1:4;
y = normpdf(x, mu, sigma);
plot(x, y, 'r', 'LineWidth', 2);
title('Histogram of Random Samples and Normal Distribution PDF');
hold off;
In this code snippet, we generate 1000 samples from a standard normal distribution and visualize it using a histogram. By overlaying the probability density function (PDF) of the theoretical distribution, you can evaluate how accurately your random samples follow the expected distribution.
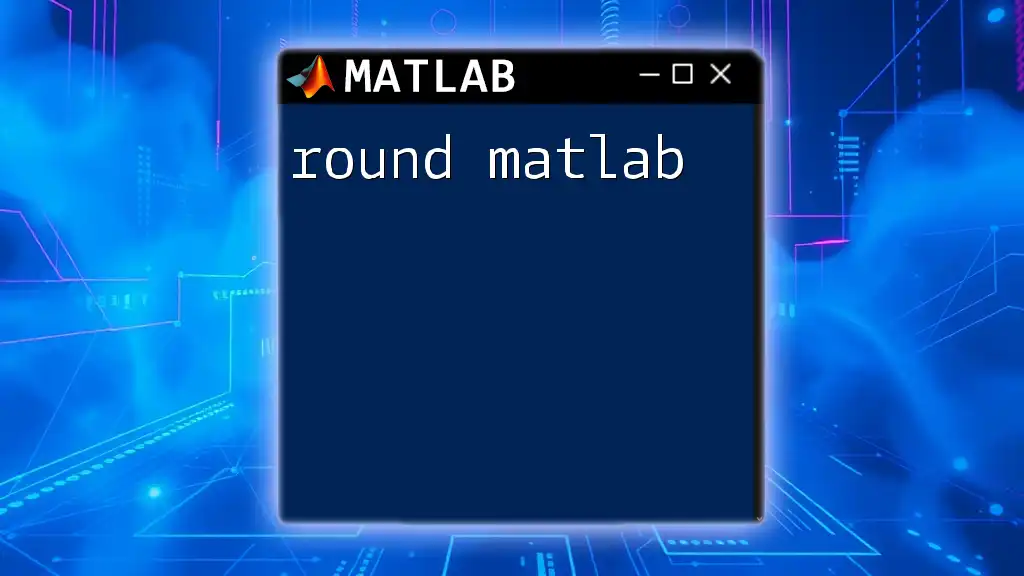
Applications of `normrnd`
Statistical Simulations
`normrnd` is widely used for statistical simulations. In situations where modeling real-world phenomena is necessary, such as in finance, healthcare, or engineering, `normrnd` enables analysts to create datasets that approximate the randomness inherent in those fields.
Testing Hypotheses
It also plays a pivotal role in hypothesis testing. For example, researchers may need to verify if their sample data follows a specific distribution. Using `normrnd`, they can generate reference datasets against which they can compare their actual results.
Monte Carlo Simulations
In more advanced contexts, `normrnd` is integral to Monte Carlo simulations. These are mathematical techniques that allow researchers to account for uncertainty in their models by running simulations with randomly generated data to see a range of potential outcomes.
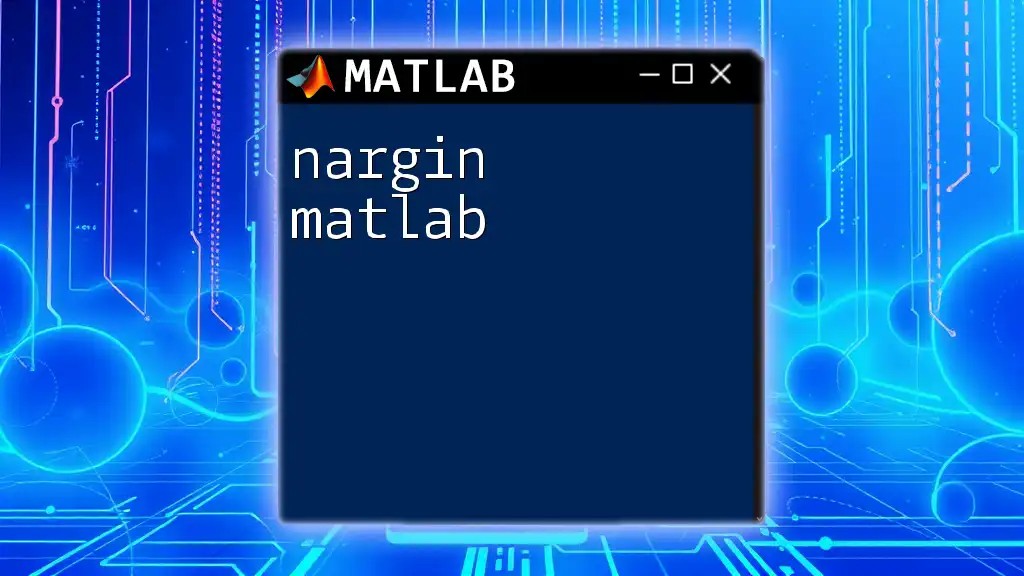
Advanced Usage and Customizations
Vectorized Operations
MATLAB is optimized for vector and matrix operations, which can enhance computational efficiency. With `normrnd`, you can generate data for multiple distributions at once.
mu = [1, 2, 3];
sigma = [1, 1, 1];
R = normrnd(mu, sigma, [1000, 3]);
This generates 1000 samples for three different normal distributions with means of 1, 2, and 3, respectively, making it a powerful tool for multi-dimensional analyses.
Using `normrnd` with Other Functions
Combining `normrnd` with other built-in MATLAB functions can further enhance your analysis. For instance, using `mean` and `std` on the output from `normrnd` can help verify your data's statistical properties:
data = normrnd(10, 5, [1000, 1]);
data_mean = mean(data);
data_std = std(data);
Here, you can check if the generated data roughly follow the defined normal distribution parameters.
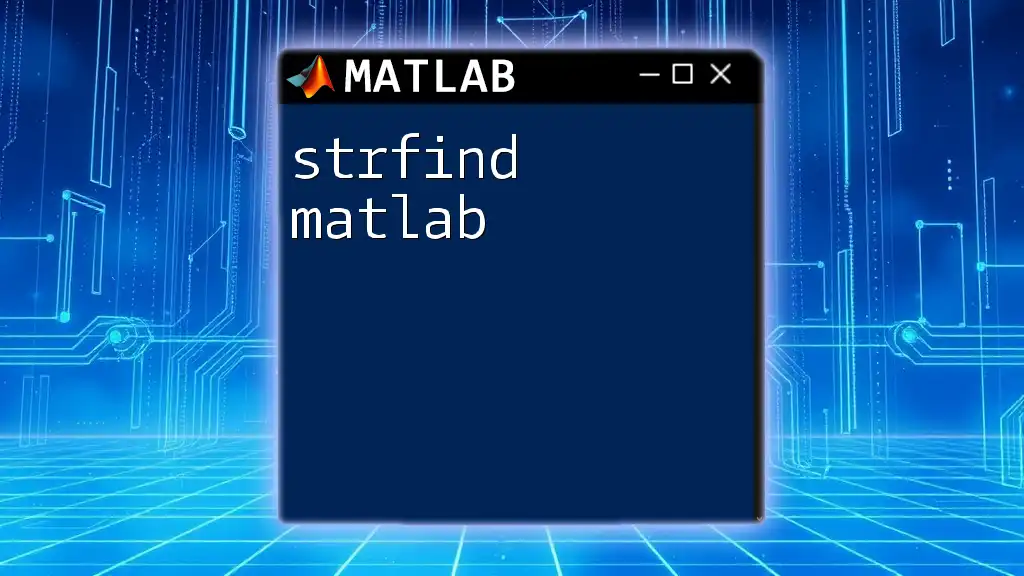
Troubleshooting Common Issues
Common Errors in `normrnd` Usage
One common mistake is misunderstanding the dimensions of the output. If `mu` and `sigma` are not compatible with the dimensions specified, MATLAB will throw an error. Always ensure the dimensions match up, or MATLAB may return an error or unexpected results.
Interpreting Outputs
After running `normrnd`, interpreting the output correctly is crucial. Randomness may generate unexpected values, but understanding the properties of your specified normal distribution will help you make sense of the data.
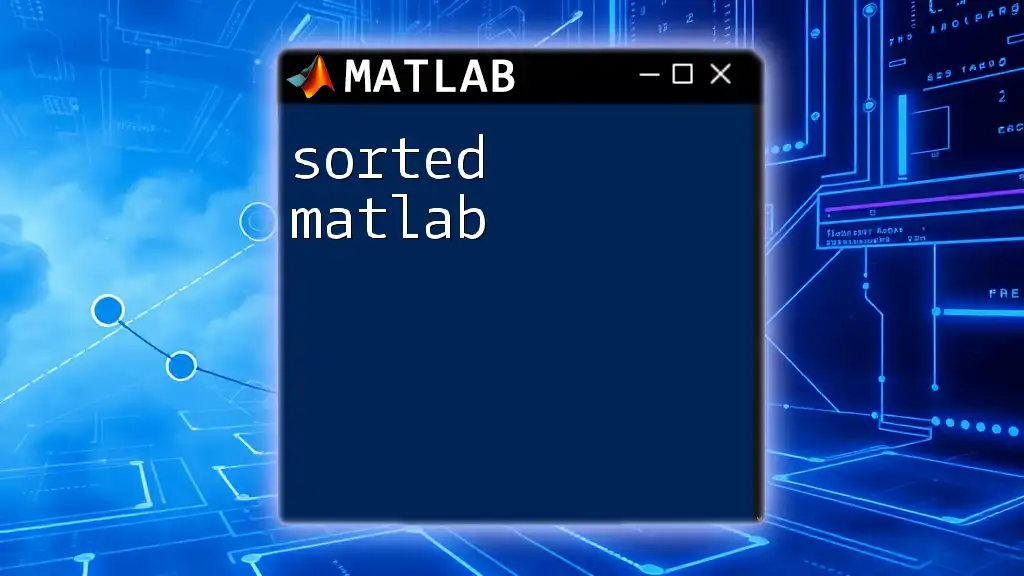
Conclusion
Summary of Key Takeaways
The `normrnd` function in MATLAB is a valuable tool for generating random numbers from a normal distribution. Mastery of this function enhances your ability to conduct statistical analyses, develop simulations, and interpret data effectively.
Further Reading and Resources
To deepen your understanding, consider referring to MATLAB’s official documentation on `normrnd` and explore related statistical topics through textbooks or online courses.
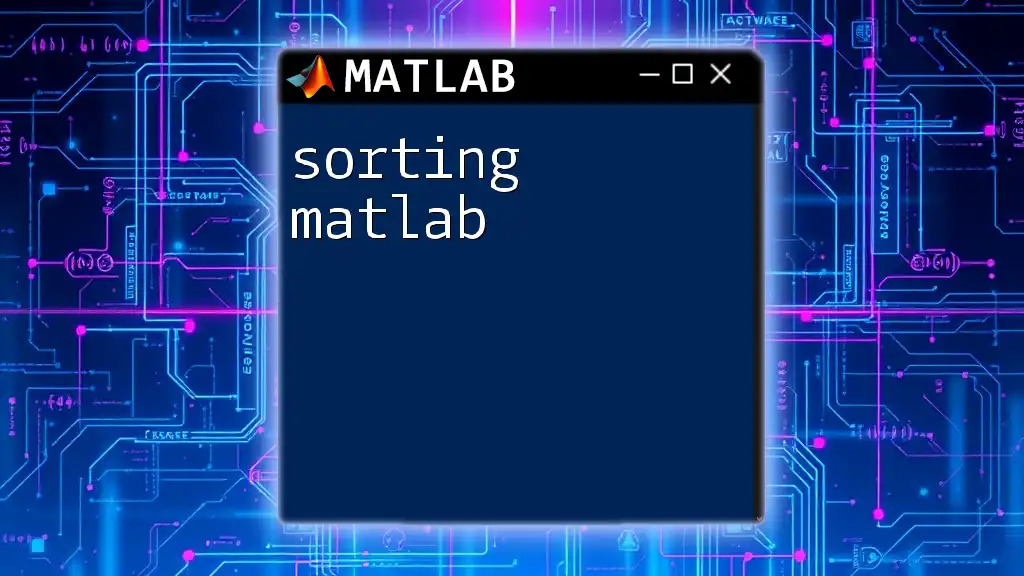
Call to Action
Encouraging Readers to Practice
Experiment with `normrnd` in your MATLAB environment. Try varying the means and standard deviations, and generate larger datasets. The best way to learn is through practice.
Join Our Community
For more tips and tricks related to MATLAB, consider joining our community. Sign up for courses or newsletters to stay updated and improve your data analysis skills.