In MATLAB, the `dicomwrite` function allows you to create a DICOM file from image data, enabling efficient storage and sharing of medical imaging information.
Here's an example of how to use the `dicomwrite` function in MATLAB:
% Create a sample image and metadata
img = imread('exampleImage.png'); % Read a sample image
info = dicominfo('template.dcm'); % Load DICOM metadata template
% Write the image data to a DICOM file
dicomwrite(img, 'outputImage.dcm', info);
Understanding DICOM Files
What is DICOM?
DICOM, or Digital Imaging and Communications in Medicine, is a standard used for storing, transmitting, and sharing medical imaging information. It not only includes the image data but also encapsulates important metadata such as patient information and imaging parameters. This makes it an essential format in the healthcare industry, particularly for modalities like X-rays, MRIs, and CT scans.
Characteristics of DICOM Files
DICOM files are unique due to their specific file structure, which includes unique identifiers (UIDs) that help in cataloging images efficiently. Each file also contains extensive metadata describing the image such as:
- Patient identifiers (name, ID, birthdate)
- Study information (study instance UID, date, and time)
- Image characteristics (pixel data, image dimensions, imaging modality)
The ability to manage and distribute DICOM files across different imaging networks is vital for consistent patient care.
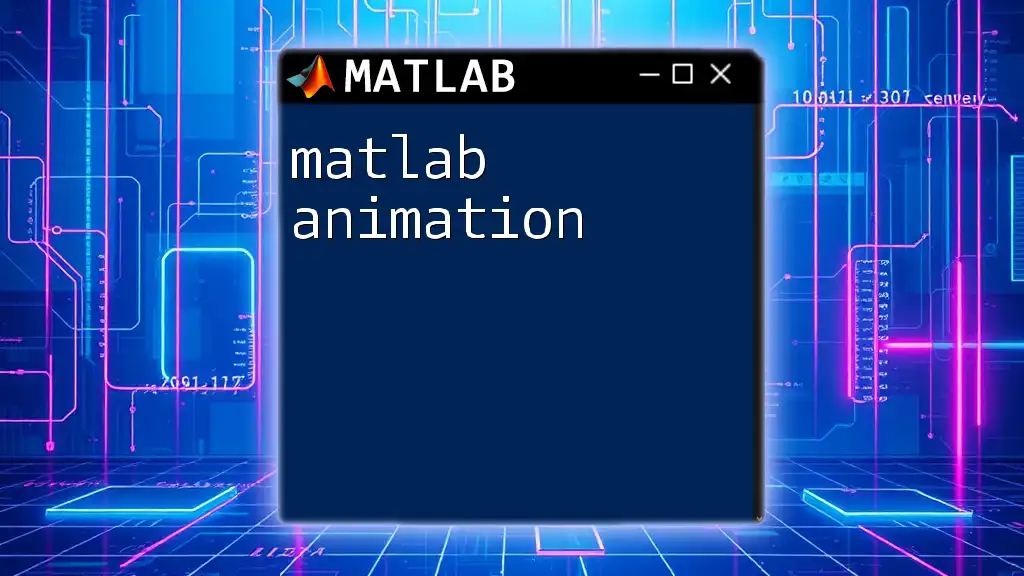
Why Use MATLAB for DICOM?
MATLAB offers powerful and versatile tools for handling DICOM files, which enhances both efficiency and effectiveness in medical imaging workflows. The robust Image Processing Toolbox provides essential algorithms for image enhancement, analysis, and visualization. Additionally, MATLAB’s scripting capabilities facilitate automation, allowing users to handle large volumes of DICOM files seamlessly.
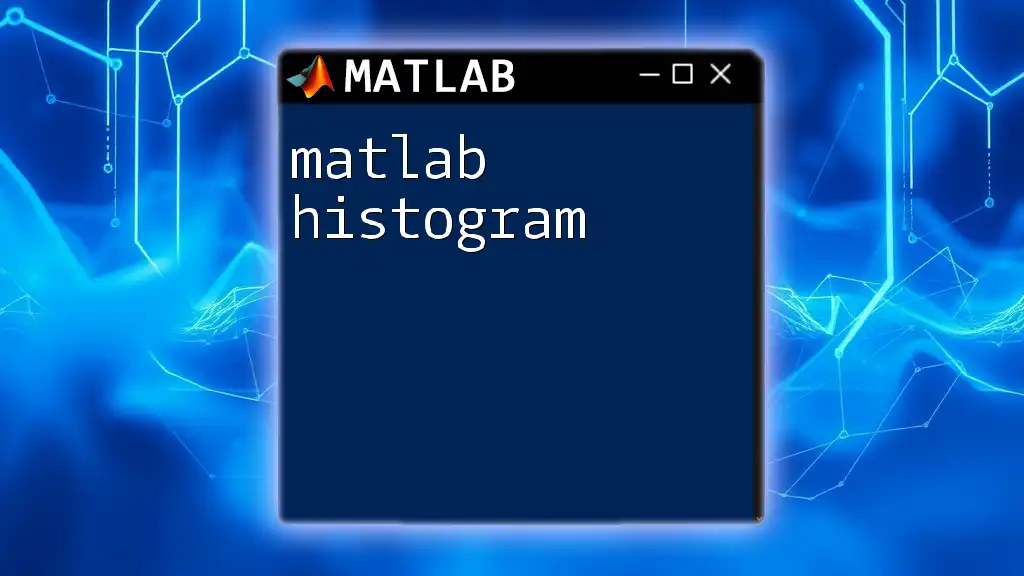
Getting Started with MATLAB for DICOM
Setting Up Your MATLAB Environment
To get started, ensure that you have the necessary toolboxes installed in MATLAB before diving into DICOM operations. Among the essential toolboxes are:
- Image Processing Toolbox: This toolbox is crucial for performing image analysis and manipulation.
- Image Acquisition Toolbox: This helps in acquiring images from various sources.
Installation can be done via the MATLAB Add-On Explorer, which provides a straightforward interface for adding functionalities.
Basic MATLAB Commands for DICOM
Reading DICOM Files
To open and visualize DICOM files, you can use the `dicomread` function in MATLAB. This command easily retrieves the image data for visualization or further processing. Here’s a simple example:
img = dicomread('example.dcm');
imshow(img, []);
title('DICOM Image');
This code snippet reads a DICOM file named `'example.dcm'` and displays the image.
Reading DICOM Metadata
Understanding the metadata associated with DICOM files is equally important. You can access metadata using the `dicominfo` function, which retrieves all relevant information about the DICOM file. Here’s how to do it:
info = dicominfo('example.dcm');
disp(info);
This command will display information such as patient name, study date, and imaging modality, providing context for the image data.
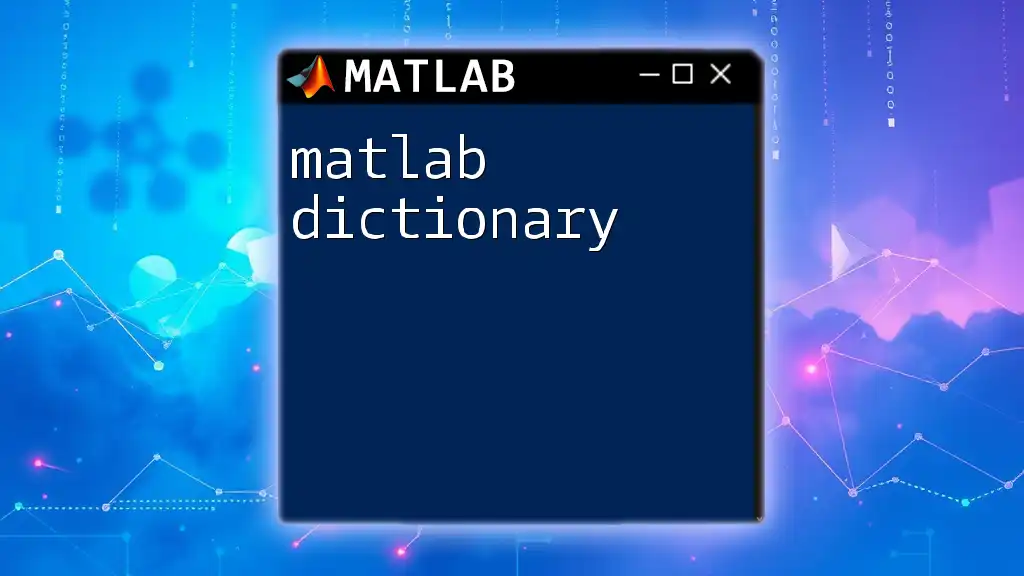
Processing DICOM Images in MATLAB
Basic Image Processing Techniques
A crucial aspect of working with DICOM files in MATLAB is the ability to enhance image quality. Common techniques include histogram equalization and contrast adjustment. Here’s how you can apply histogram equalization:
enhancedImg = histeq(img);
imshow(enhancedImg);
title('Enhanced DICOM Image');
Histogram equalization improves the contrast of the image, making features more distinguishable.
Advanced Visualization Techniques
MATLAB also allows you to visualize DICOM data in three dimensions, which is particularly useful for understanding volumetric data from CT or MRI scans. Here’s a code snippet that demonstrates how to create a 3D volume rendering:
volumeData = cat(3, dicomread('slice1.dcm'), dicomread('slice2.dcm'));
volshow(volumeData);
title('3D Visualization of DICOM Data');
By combining multiple slices, you can create a more comprehensive view of the anatomical structures being studied.
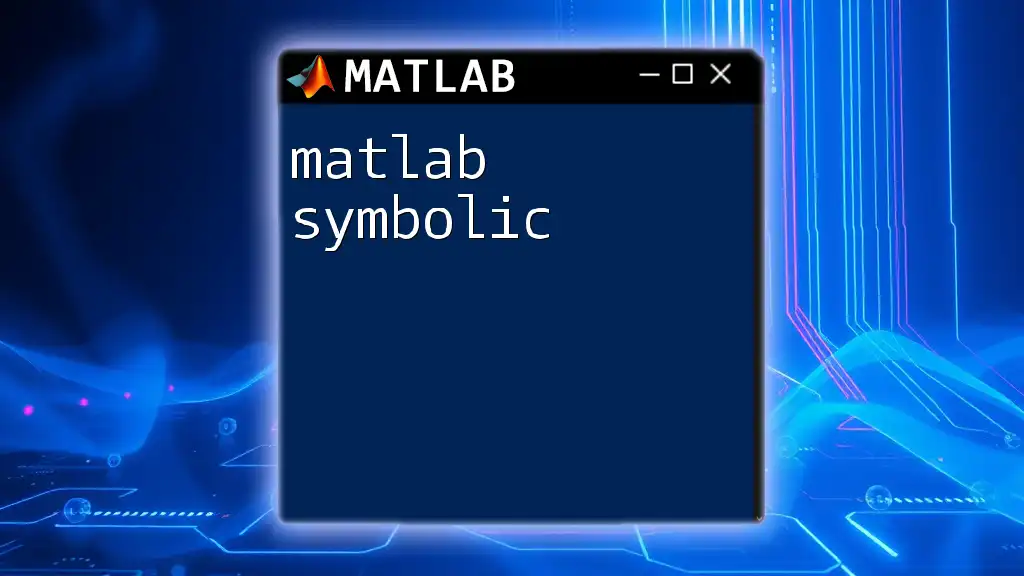
Storing and Writing DICOM Files
Saving Edited DICOM Files
Once processed, you may want to save the modified images back to DICOM format. The `dicomwrite` function is designed for this purpose. Ensure that you retain the important metadata when saving the image:
dicomwrite(enhancedImg, 'enhanced_example.dcm', info);
disp('DICOM file saved successfully.');
This command saves the enhanced image as a new DICOM file while retaining the original metadata from the `info` structure.
Configuring DICOM Metadata
Sometimes, it may be necessary to modify the metadata before saving. MATLAB allows you to customize these fields. For instance:
info.SeriesDescription = 'Enhanced Image Processing';
dicomwrite(enhancedImg, 'enhanced_example.dcm', info);
This snippet updates the `SeriesDescription` field of the metadata, providing a useful context for future reference.
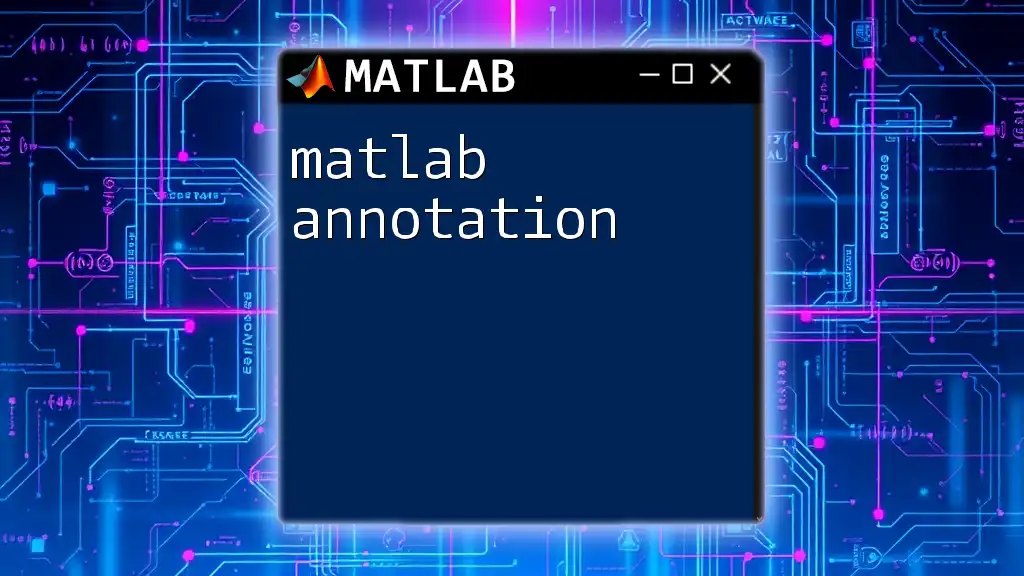
Practical Applications of DICOM in MATLAB
Examples in Medical Imaging Research
MATLAB’s powerful capabilities for processing DICOM images have been leveraged in numerous medical imaging research projects. Common applications involve:
- Image segmentation: Identifying and isolating specific structures, like tumors.
- Feature extraction: Analyzing images to derive quantifiable metrics important for diagnostics.
Real-world projects often result in significant advancements in both research and clinical practices by improving diagnostic accuracy and treatment planning.
Integrating DICOM with Machine Learning
In recent years, the role of artificial intelligence in medical imaging has grown rapidly. By integrating MATLAB DICOM capabilities with machine learning, researchers are able to extract features from DICOM images to train predictive models. This can lead to breakthroughs in automated diagnostics and treatment recommendations.
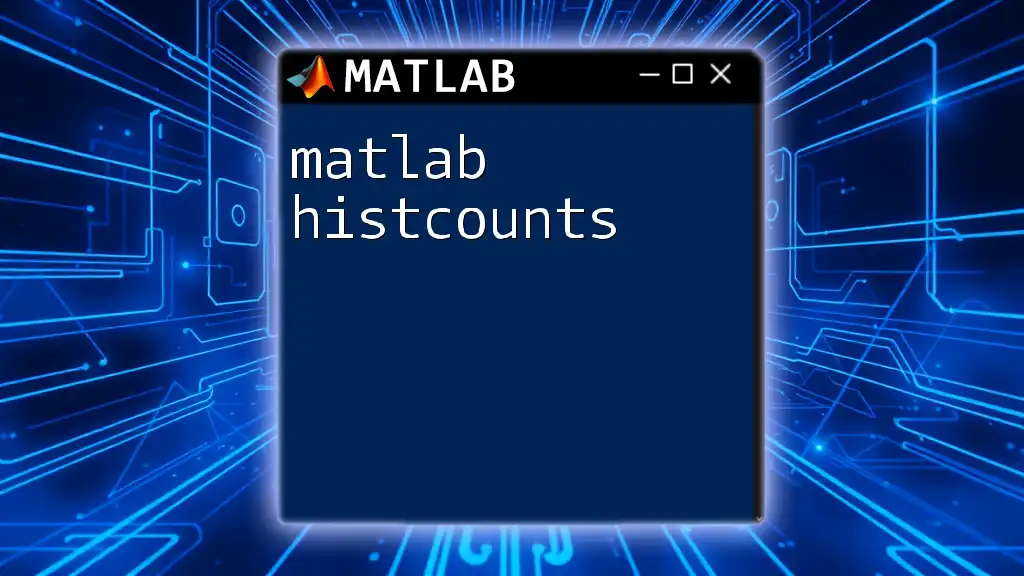
Conclusion
In summary, MATLAB serves as a robust tool for working with DICOM files, enabling medical professionals and researchers to efficiently read, process, visualize, and save medical images. The combination of MATLAB's powerful functions and intuitive syntax makes it an excellent choice for anyone looking to delve into medical imaging.
Feel encouraged to experiment with the commands provided in this guide, and explore the vast potential that lies in combining MATLAB with DICOM technology. By leveraging these tools effectively, you can significantly enhance your capabilities in medical imaging and research.
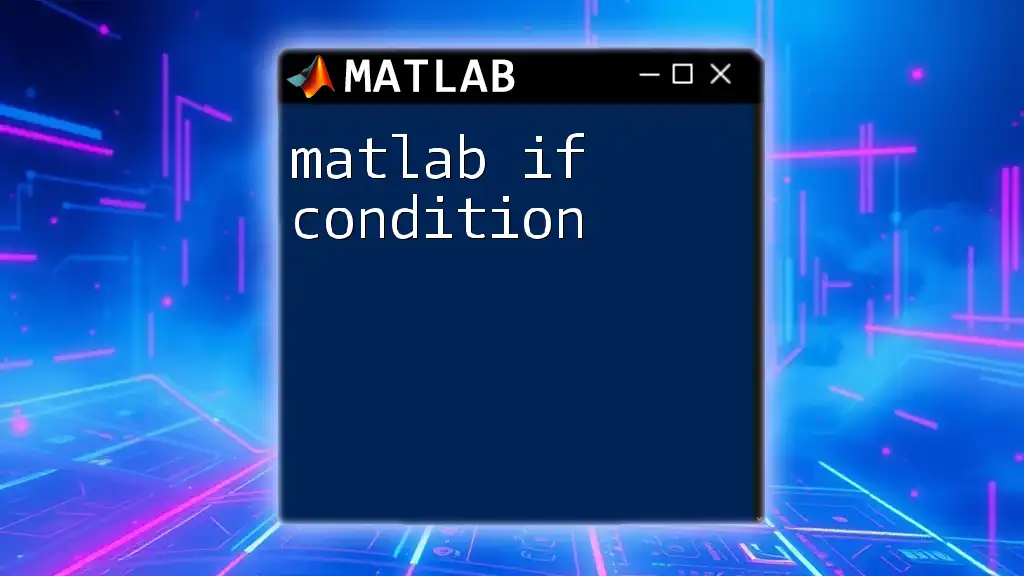
Call to Action
As you continue to explore the world of DICOM and MATLAB, consider joining our dedicated MATLAB community for additional resources, support, and networking opportunities. Happy coding!