The `dir` command in MATLAB is used to list the files and folders in the current directory or specified path.
% List all files and folders in the current directory
files = dir;
What is the `dir` Command?
The `dir` command is an essential MATLAB function that allows users to quickly access and manage files within a directory. Understanding how to effectively utilize this command can greatly enhance your workflow, especially if you are dealing with multiple files or need to navigate through complex directory structures.
Definition
At its core, the `dir` command is designed to provide a list of files and directories within a specified folder. It serves as a straightforward way to ascertain what is available in a particular location on your file system.
Purpose
The primary purpose of the `dir` command extends beyond mere visibility; it is instrumental for file management and organization within MATLAB. Whether you are compiling data, running simulations, or processing scripts, knowing how to summon a list of relevant files is fundamental to maintaining an efficient workspace.
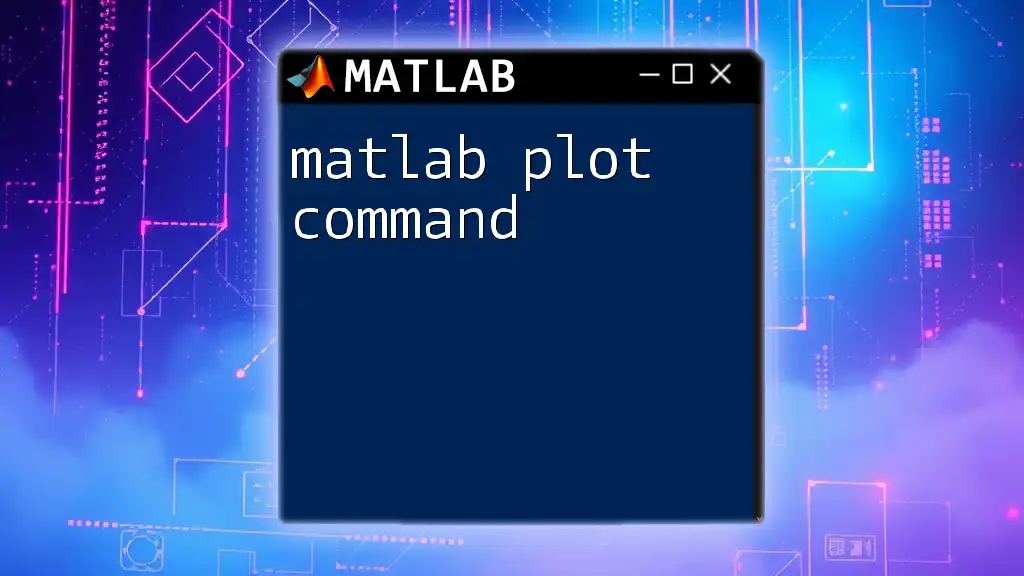
Basic Usage of the `dir` Command
Syntax
The basic syntax of the `dir` command can be summarized as follows:
dir(<directory>)
This simple command allows users to call on the contents of any specified directory.
Example: Listing Files in the Current Directory
One of the most common usages of the `dir` command is to list all files in your current working directory. The command can be executed without any arguments:
files = dir;
disp(files);
The output from this command is a structured array that captures attributes of each item, such as the file name, size, and date modified. Understanding these details can assist in locating specific files and gauging their relevance to your project.
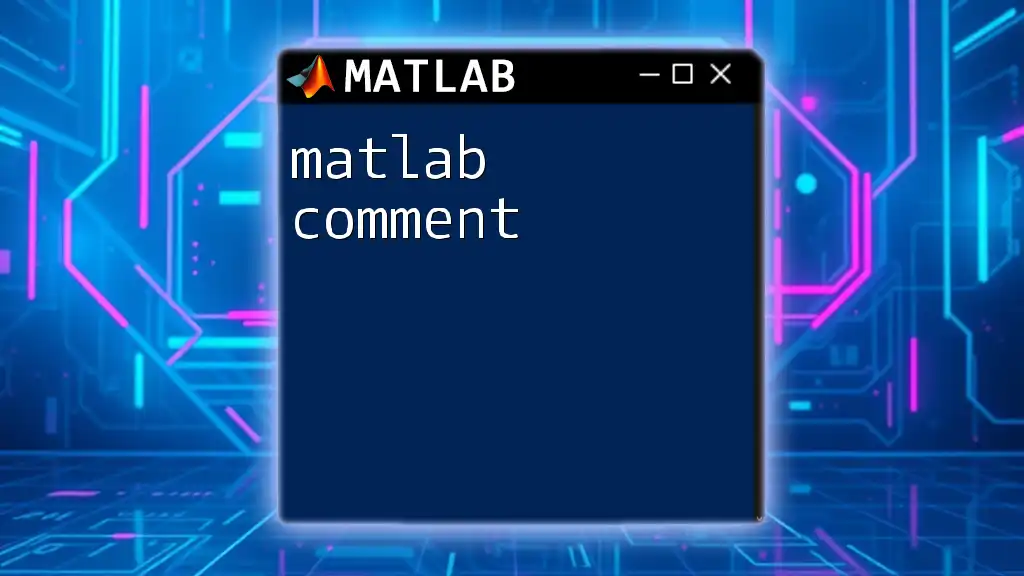
Options for Specifying Directories
Listing Files in Specific Directories
Sometimes you may want to target a specific directory beyond the current workspace. This can be achieved by providing the full path:
dir('C:\Your\Specific\Directory');
This capability allows you to access files in different locations, enabling efficient data organization and retrieval.
Using Wildcards
What are Wildcards?
Wildcards are powerful characters that represent one or more unspecified characters. The most common wildcard characters are `*` (which represents any number of characters) and `?` (which represents a single character).
Example: Filtering Specific File Types
Using wildcards with the `dir` command can be extremely beneficial. For instance, to list all MATLAB files in your current directory, you can utilize the following command:
dir('*.m'); % Lists all MATLAB files in the current directory
In this case, `*.m` filters the results to return only files with the .m extension, making it easier to focus on specific types of data or scripts.
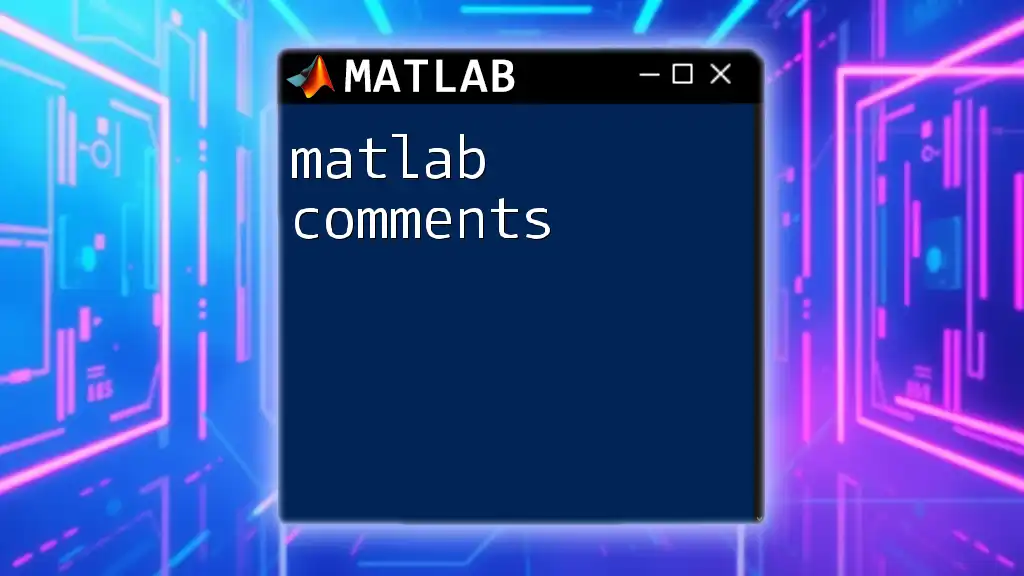
Understanding the Output of `dir`
Structure of the Output
When executing the `dir` command, you receive a structured array containing multiple fields for each file: name, date, bytes, and isdir. This allows not just visibility of files but also insights into their properties.
Accessing Specific Fields
Once you have the output from the `dir` command, you can access specific fields easily. For example, to obtain only the filenames, you can use:
filenames = {files.name}; % Get only the file names
This extracts just the names, which can be particularly useful for further processing or analysis.
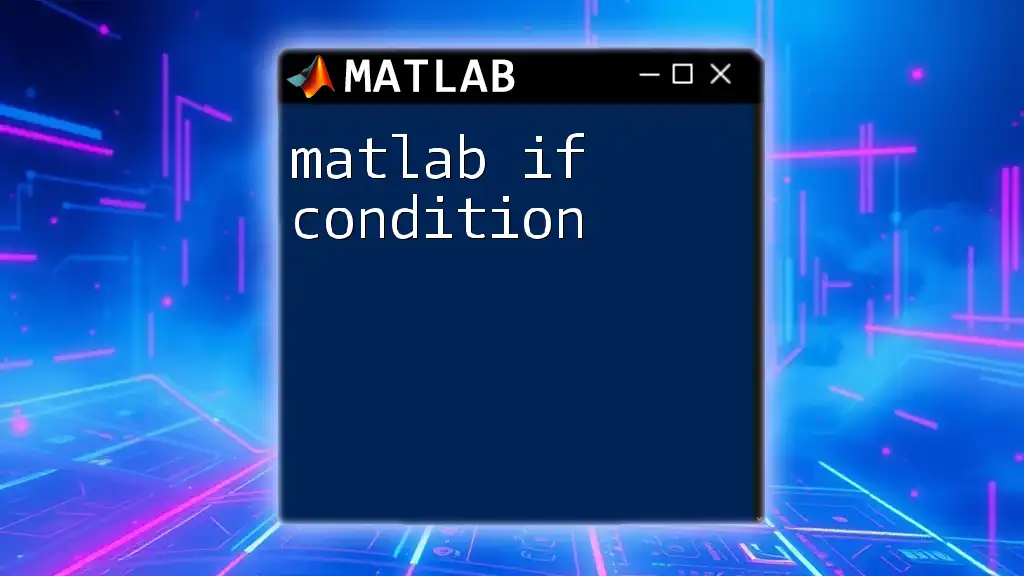
Additional Options and Flags
Using `dir` with Recursion
The `dir` command can also navigate through subdirectories by using recursion. If you want to list all MATLAB files in the current directory and any subdirectories, use this command:
dir('**/*.m'); % Lists all MATLAB files in current and subdirectories
This powerful feature ensures that no relevant files are missed, regardless of their location in the directory hierarchy.
Combining with Other Functions
The flexibility of the `dir` command becomes even more pronounced when combined with other MATLAB functions. For instance, using `fullfile` can enhance file path management considerably:
allFiles = dir('*.m');
for i = 1:length(allFiles)
fullPath = fullfile(pwd, allFiles(i).name);
disp(fullPath);
end
In this example, we loop through all `.m` files and print their full paths. Combining commands like this allows for efficient file manipulation and data organization.
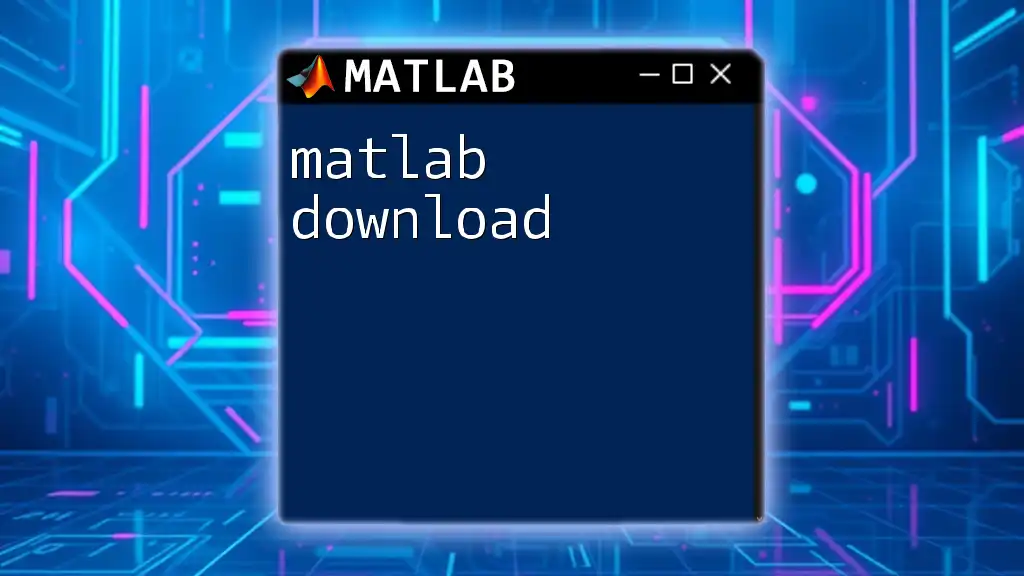
Common Use Cases for the `dir` Command
Checking for Specific File Existence
One practical application of the `dir` command is ensuring that a required file exists before proceeding with further code execution. This can help avoid errors and streamline workflows. For example, you can check if a file named `data.mat` exists with the following logic:
if any(strcmp({files.name}, 'data.mat'))
disp('File exists!');
else
disp('File does not exist.');
end
Automating File Processing Tasks
The `dir` command is a cornerstone for automation in file handling tasks. By listing the contents of a directory, you can easily program MATLAB to perform actions on multiple files, such as loading, processing, or analyzing datasets in bulk.
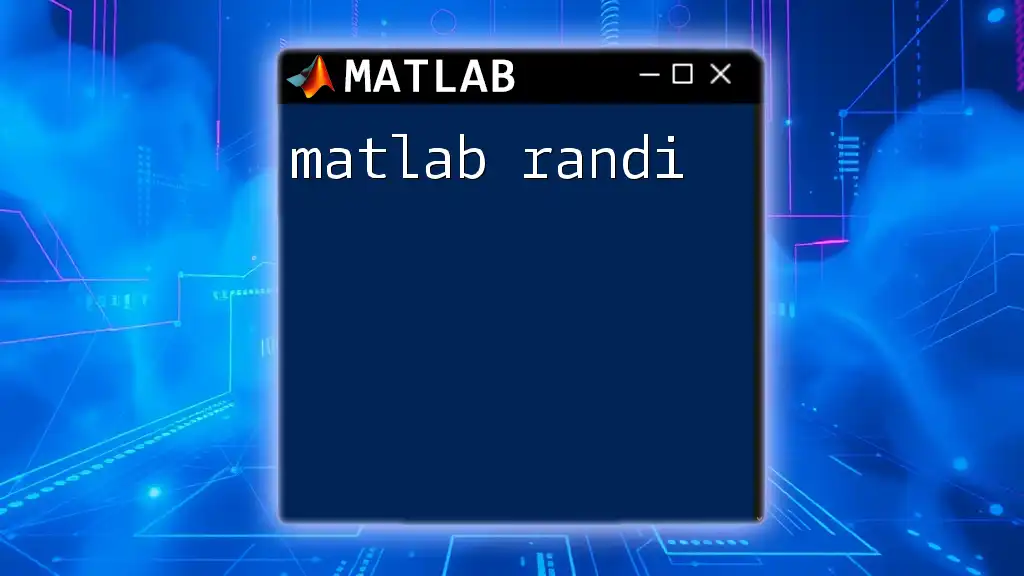
Conclusion
The MATLAB `dir` command is a fundamental tool that enhances your ability to manage files efficiently. By mastering this command, you can simplify your workflow, ensuring that you are able to locate and manipulate files rapidly. Don't hesitate to experiment with the various functionalities outlined here to see how they can streamline your operations in MATLAB.
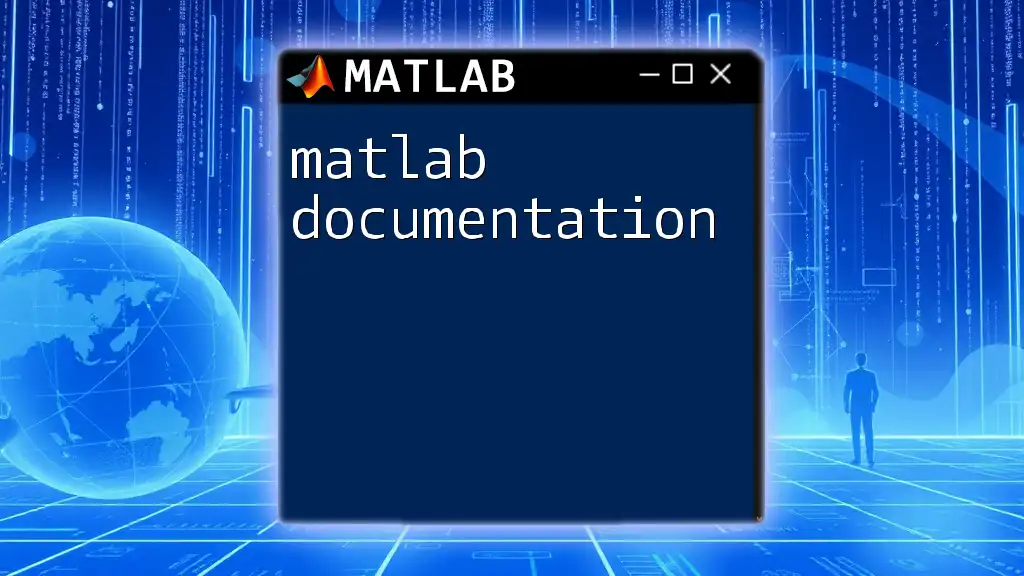
Additional Resources
For further information, consider checking out the official MATLAB documentation on the `dir` command. This resource provides a deeper dive into its capabilities and offers a wealth of examples. Additionally, exploring related topics such as file management or data organization in MATLAB can provide further context and utility.
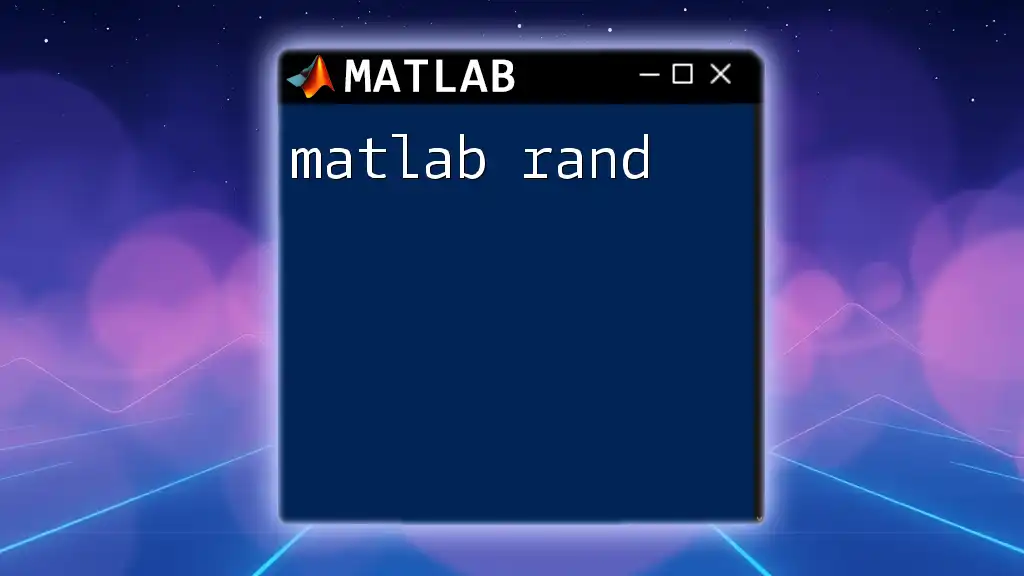
Call to Action
Now that you have a comprehensive understanding of the `dir` command, it’s time to put it into practice! Open MATLAB, execute the commands discussed, and see how they can improve your coding efficiency and project organization. Share your experiences and any tips you uncover along the way!