The `tic` and `toc` commands in MATLAB are used to measure the elapsed time between two points in your code, allowing for performance analysis of your scripts.
Here’s a simple code snippet demonstrating how to use `tic` and `toc`:
tic; % Start the stopwatch
pause(2); % Simulate a process by pausing for 2 seconds
elapsedTime = toc; % Stop the stopwatch and save the elapsed time
fprintf('Elapsed time: %.2f seconds\n', elapsedTime); % Display the elapsed time
Understanding `tic` and `toc`
What is `tic`?
The `tic` command in MATLAB is a powerful tool designed to start the stopwatch timer. By using `tic`, you can measure the amount of time it takes for various code segments to execute. It's particularly useful in various situations where you want to optimize performance and understand how long your computations take.
When to use `tic`? Consider scenarios where your computations get lengthy, or when assessing the efficiency of different algorithms. By timing code execution, you can make informed decisions about where to focus your optimization efforts.
What is `toc`?
Following the `tic` command, the `toc` command halts the timer and outputs the total elapsed time. It provides insight into how long the section of code you wanted to measure has taken to run, which is crucial for performance evaluation.
Utilizing `toc` after `tic` gives you an easy way to profile code, which is valuable for understanding performance bottlenecks in your applications.
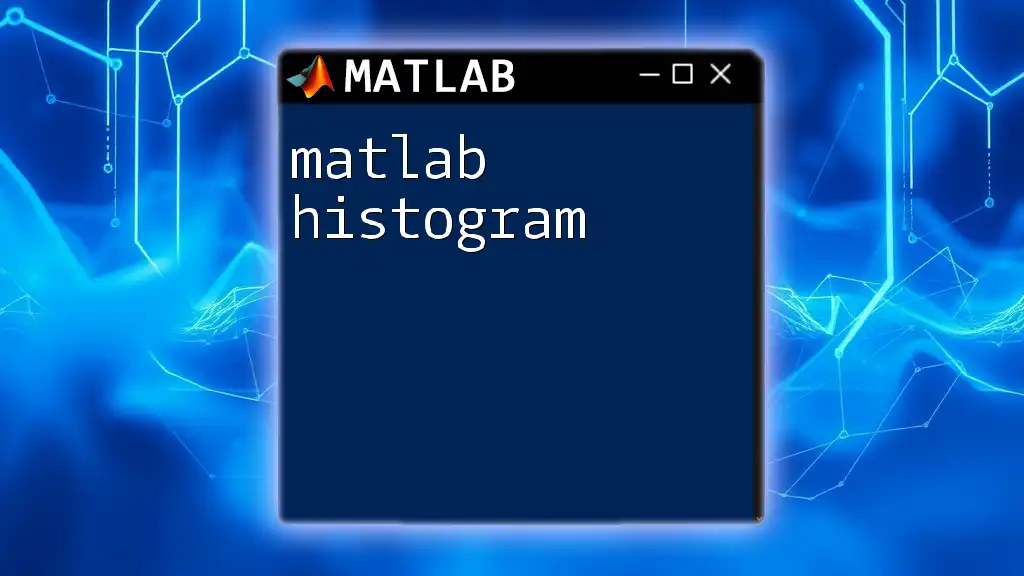
How to Use `tic` and `toc`
Basic Syntax
To use `tic` and `toc` together, you simply place `tic` before the code you wish to measure and `toc` immediately after. Here's a simple example:
tic
% Code to measure
pause(2) % Example: simulating a delay of 2 seconds
toc
In this example, `pause(2)` simulates a delay of 2 seconds. When you run this code, `toc` will output the elapsed time, which should be approximately 2 seconds.
Timing Multiple Sections of Code
Using Multiple `tic` and `toc`
You can also use `tic` and `toc` to measure different non-continuous sections of code. This is useful when you want to profile various parts of your program separately. Here’s how to do it:
tic
% First code segment
pause(1)
toc
tic
% Second code segment
pause(3)
toc
In this case, you’ll get two outputs: the first indicating that the first segment took about 1 second and the second confirming that the second code segment took approximately 3 seconds. Each `toc` provides the elapsed time for its corresponding `tic`.
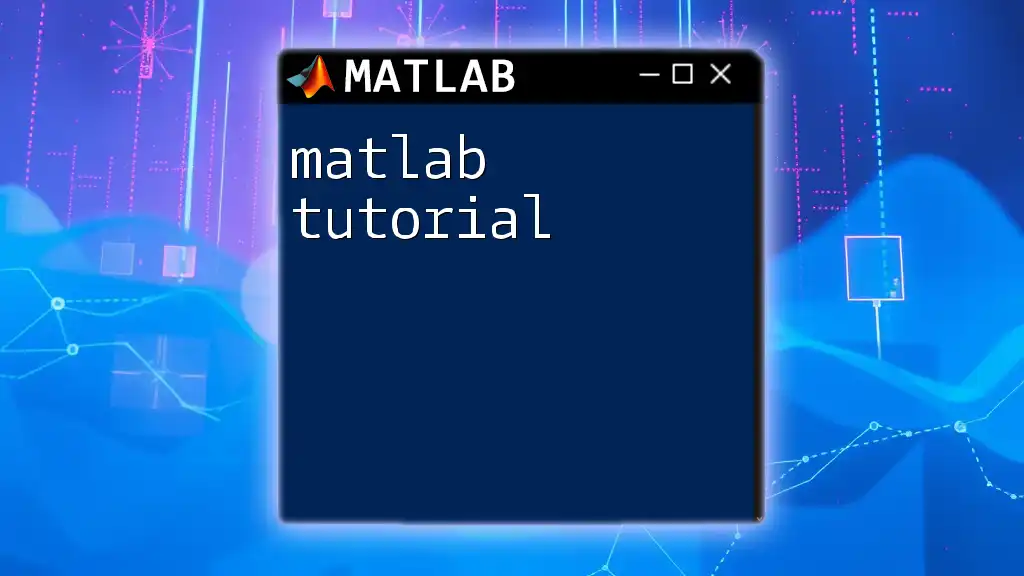
Advanced Timing Techniques
Using `tic` and `toc` in Functions
You can encapsulate `tic` and `toc` inside functions to profile their execution time. This is especially useful when you want to gauge how long a specific function takes alone. Here’s a demonstration:
function myFunction()
tic
% Code to measure
pause(4)
elapsedTime = toc;
fprintf('Elapsed time: %.2f seconds\n', elapsedTime);
end
When you call `myFunction()`, it will pause for 4 seconds and then print the elapsed time. This allows you to measure the performance of functions directly and make adjustments accordingly.
Storing Elapsed Time
Sometimes, you may want to save the results of your timing for further analysis later on. Storing elapsed time allows you to compare performance over different runs or algorithmic variations. Here’s an illustrative example:
tic
pause(1)
elapsedTime1 = toc;
tic
pause(2)
elapsedTime2 = toc;
fprintf('Elapsed time 1: %.2f seconds\n', elapsedTime1);
fprintf('Elapsed time 2: %.2f seconds\n', elapsedTime2);
In this example, you store the elapsed time for two different delays and print them. This process is invaluable in giving you metrics that can guide your optimization strategies.
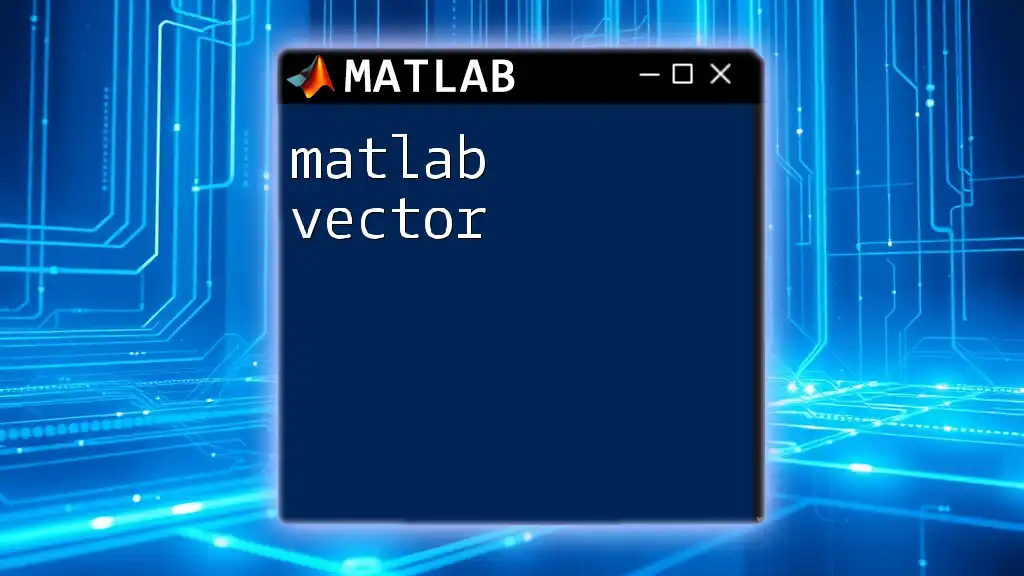
Common Gotchas and Best Practices
Precision and Accuracy
When using `tic` and `toc`, keep in mind that various factors can influence the accuracy of your timing results. For instance, system load from other applications running on your computer can introduce variability in execution times. Testing the same code multiple times and averaging the results can help mitigate these effects.
Best Practices for Effective Timing
To ensure that your timing data is meaningful, consider the following best practices:
- Code Placement: Always place `tic` at the very beginning of the code segment you want to time and `toc` immediately after. This creates a clear and concise measurement scope.
- Avoid Nested Timing: While it’s technically possible to have `tic` and `toc` inside other functions or loops, it can complicate your output and interpretation. Try to limit the scope of your timing to major blocks of code or individual functions.
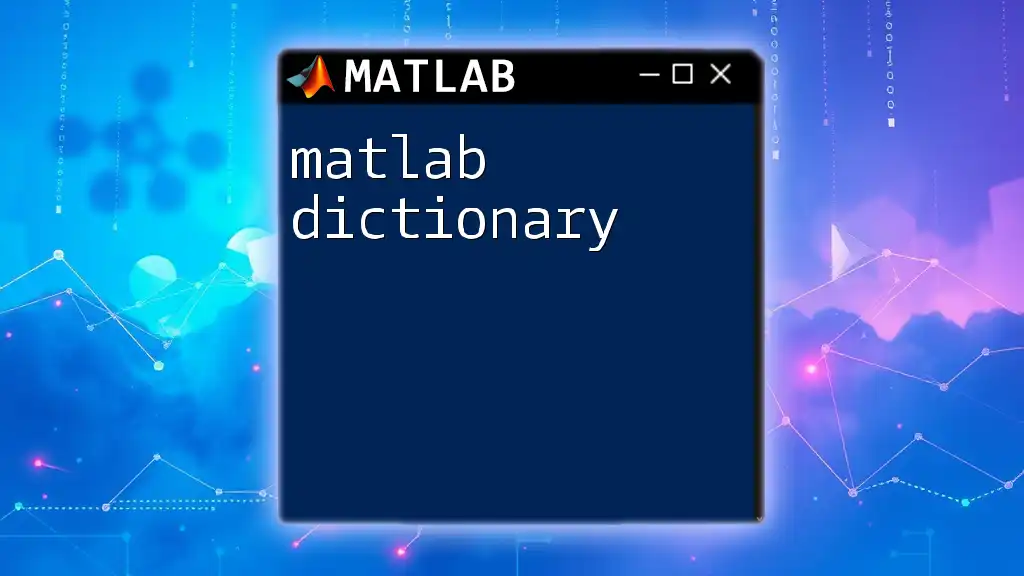
Practical Applications of Timing in MATLAB
Performance Optimization
`tic` and `toc` can be instrumental in identifying performance bottlenecks in your MATLAB code. When you time different segments of your code, you may discover unexpectedly long execution times in certain sections. Armed with this knowledge, you can then refactor or optimize the problematic sections for increased efficiency.
Benchmarking Algorithms
Another powerful application of `matlab tic toc` is in comparative performance testing of different algorithms. By timing their execution and comparing the results, you can choose the most efficient algorithm for your needs. This can significantly enhance the performance of your code, especially in scenarios where the choice of algorithm dramatically influences execution time.
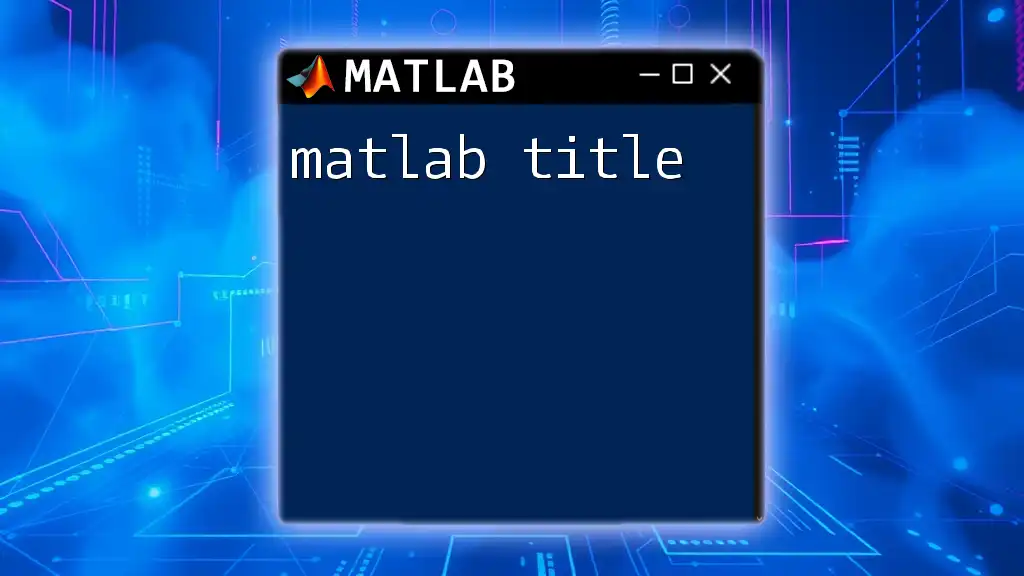
Conclusion
In summary, the `tic` and `toc` commands are essential tools in a MATLAB programmer's toolbox for measuring and optimizing code execution times. By incorporating these commands into your workflow, you gain valuable insights into the performance of your applications, enabling you to make informed decisions about enhancements and optimizations.
Experimenting with `tic` and `toc` in your MATLAB projects can yield significant improvements in both performance and efficiency, making it a practice worth adopting.
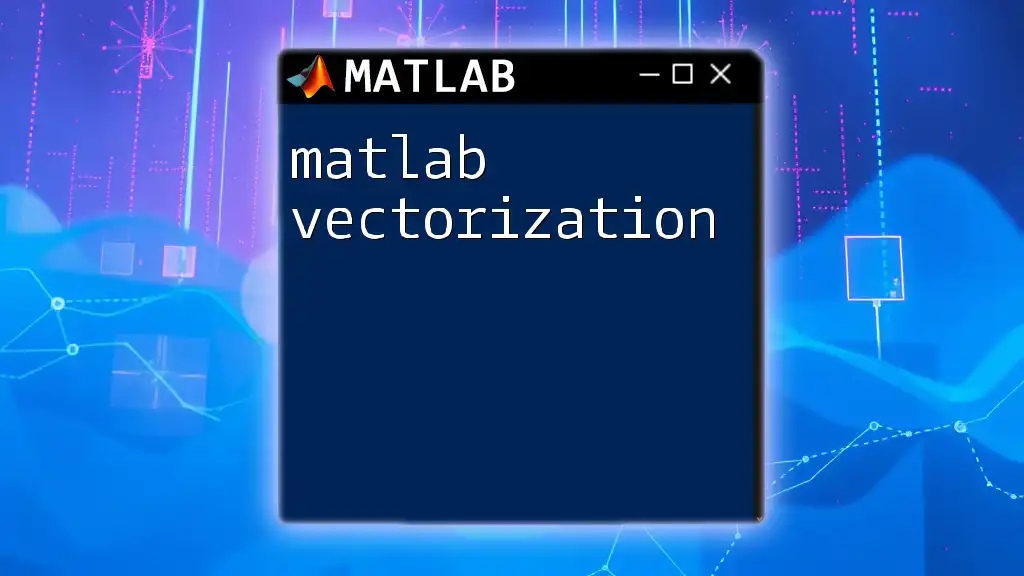
Additional Resources
For more in-depth information on the implementation and usage of timing functions in MATLAB, you can refer to the official MATLAB documentation. Books, tutorials, and tailored courses focused on performance optimization will further enhance your understanding and mastery of these essential commands.
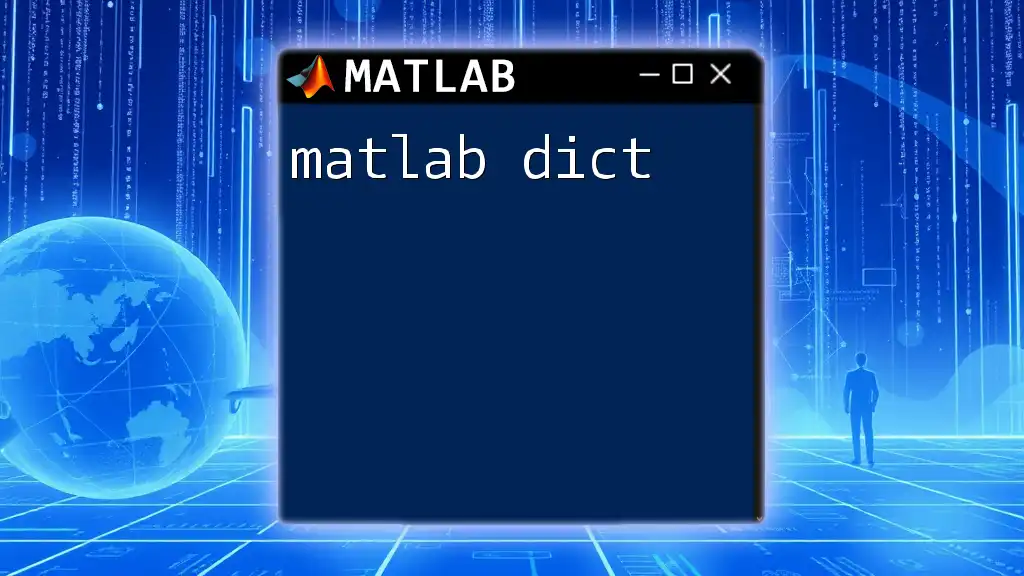
Call to Action
Join our MATLAB community today! Engage in classes and forums designed to help you hone your MATLAB skills. With the right guidance and practice, you can turn your coding challenges into opportunities for learning and growth.