MATLAB symbolic computation allows users to perform algebraic operations on mathematical expressions exactly, rather than numerically, enabling manipulation of variables and equations symbolically.
Here’s a simple example:
syms x
expr = x^2 + 3*x + 2;
factored_expr = factor(expr);
disp(factored_expr)
Getting Started with MATLAB Symbolic
The MATLAB Symbolic Toolbox is an essential tool for engineers, scientists, and researchers looking to perform symbolic computations. To get started with this powerful toolbox, you first need to ensure it is installed. You can easily install the Symbolic Math Toolbox from the MATLAB Add-On Explorer via the Home tab in MATLAB.
When you're ready to dive in, familiarize yourself with the basic syntax and commands used in symbolic computation. A fundamental command is `syms`, which declares symbolic variables. Here’s an example of how to declare two symbolic variables:
syms x y
Core Concepts of Symbolic Math
Declaring Symbolic Variables
Symbolic variables are the backbone of symbolic computations in MATLAB. By using the `syms` command, you can create variables that can represent mathematical symbols rather than numerical values. This opens up a variety of possibilities for algebraic manipulations, calculus operations, and more.
For example, if you declare the variables `x` and `y`, you can subsequently create expressions involving these variables:
expr = x^2 + y^2;
Understanding Symbolic Expressions
Creating and manipulating symbolic expressions is central to using MATLAB symbolic effectively. After declaring your symbolic variables, you can form expressions just like you would in traditional mathematics.
For instance, if you want to simplify an expression, you can use the `simplify` function:
simplified_expr = simplify(expr);
This command will transform your expression into a more manageable form, reducing complexity and making it easier to differentiate or integrate.
Solving Equations Symbolically
MATLAB provides an efficient way to solve algebraic equations symbolically. You start by defining an equation. Here's how to create a simple quadratic equation:
eq = x^2 + 2*x + 1 == 0;
Once you have your equation set up, you can find its solutions using the `solve` function:
solution = solve(eq, x);
This command will return the roots of the quadratic equation, demonstrating how symbolic computation can provide exact solutions where numerical methods may fall short.
Differentiation and Integration
Symbolic Differentiation
A hallmark of the Symbolic Math Toolbox is the ability to differentiate functions symbolically. Symbolic differentiation allows you to compute the derivative of an expression without approximating its behavior, yielding precise results.
Here's how you can differentiate an expression:
derivative = diff(expr, x);
This command computes the derivative of `expr` with respect to `x`, providing clear insight into how the function behaves as `x` changes.
Symbolic Integration
Symbolic integration is equally powerful, enabling you to find antiderivatives or definite integrals symbolically. For example, you can compute the integral of an expression using the `int` function:
integral = int(expr, x);
This will return the integral of the previously defined expression with respect to `x`, showcasing the toolbox's capability for handling calculus operations.
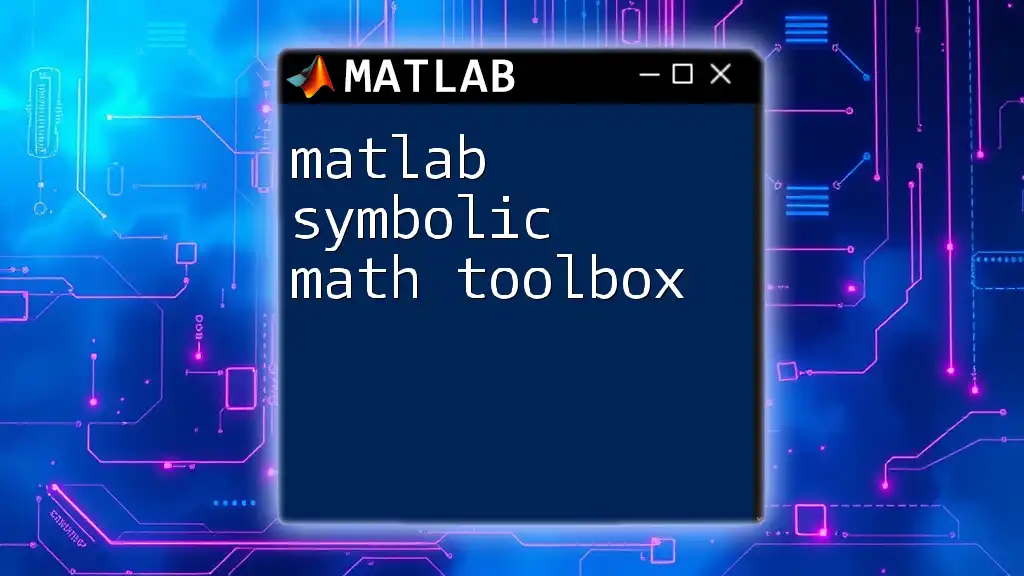
Advanced Symbolic Operations
Series Expansion
Understanding series expansions is essential for approximating functions around specific points. MATLAB makes this straightforward with the `taylor` function, which generates a Taylor series expansion of a specified function. For instance:
taylor_exp = taylor(exp(x), x);
This command expands the exponential function around the variable `x`, creating a polynomial that approximates the function near a specified point.
Laplace Transforms
Laplace transforms are a fundamental tool in engineering, particularly in control theory and signal processing. With the symbolic toolbox, you can compute Laplace transforms easily. Here’s a basic example:
syms t s
laplace_transform = laplace(exp(-t), t, s);
This transforms the exponential function into the frequency domain, providing insights valuable for system analysis.
Working with Matrices Symbolically
You can also create symbolic matrices, which are crucial for linear algebra computations in symbolic form. This is particularly useful in systems of equations, eigenvalue problems, and other applications.
To create a symbolic matrix, you can structure it like so:
A = [syms a b; syms c d];
Once your symbolic matrix is defined, you can perform various operations, such as addition, multiplication, and finding eigenvalues:
eig_values = eig(A);
These operations allow for symbolic manipulation that is essential for understanding complex systems.
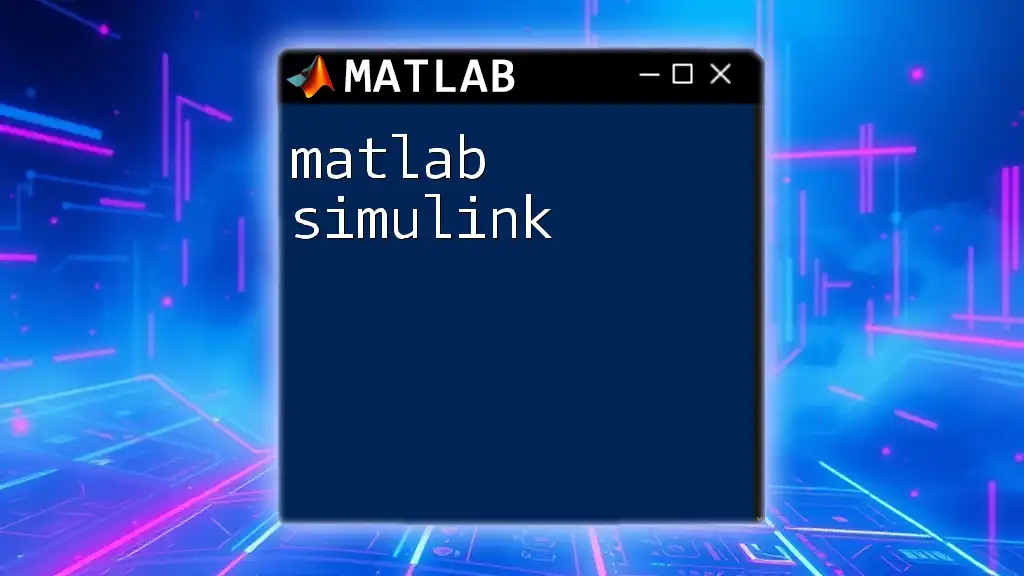
Plotting Symbolic Functions
Visualizing symbolic expressions can help in understanding their behavior. MATLAB provides the `fplot` function, which simplifies the process of plotting. For instance, you can plot a symbolic expression over a specified range:
fplot(expr, [-10, 10]);
This command will create a graph of the expression `expr` from `-10` to `10`, making it easy to visualize changes and behaviors across values.
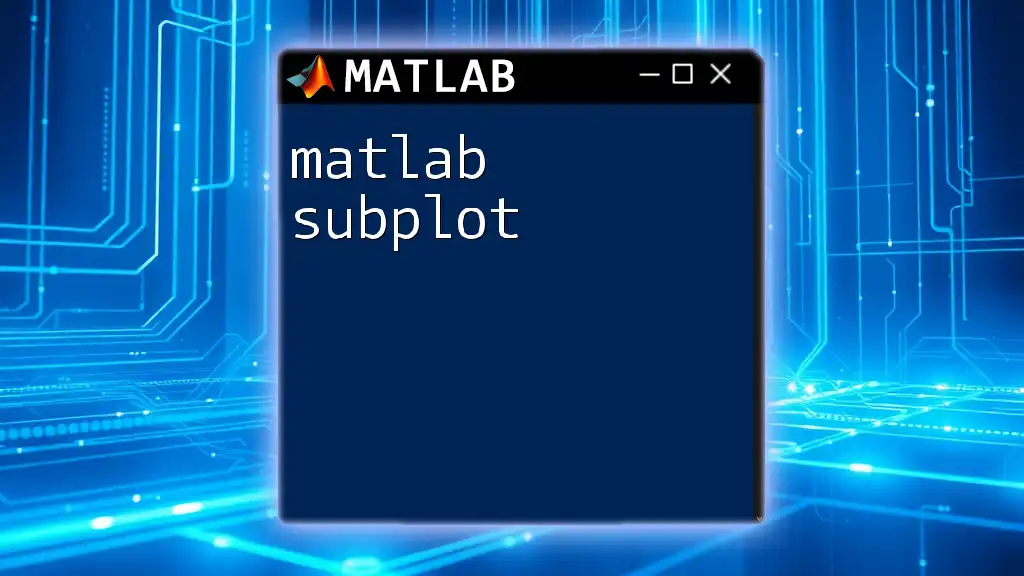
Applications of Symbolic Math
The applications of symbolic computation in MATLAB are extensive and invaluable. It serves as a powerful tool in various domains, helping to solve real-world problems. For instance, symbolic math can be utilized in system dynamics, optimization problems, and control theory, providing precise solutions that numerical methods may not yield directly.
Case Studies
Consider a case study in control systems where engineers must model and analyze system behavior. MATLAB's symbolic toolbox allows them to create transfer functions, compute stability criteria, and derive performance metrics without delving into numerical approximations.
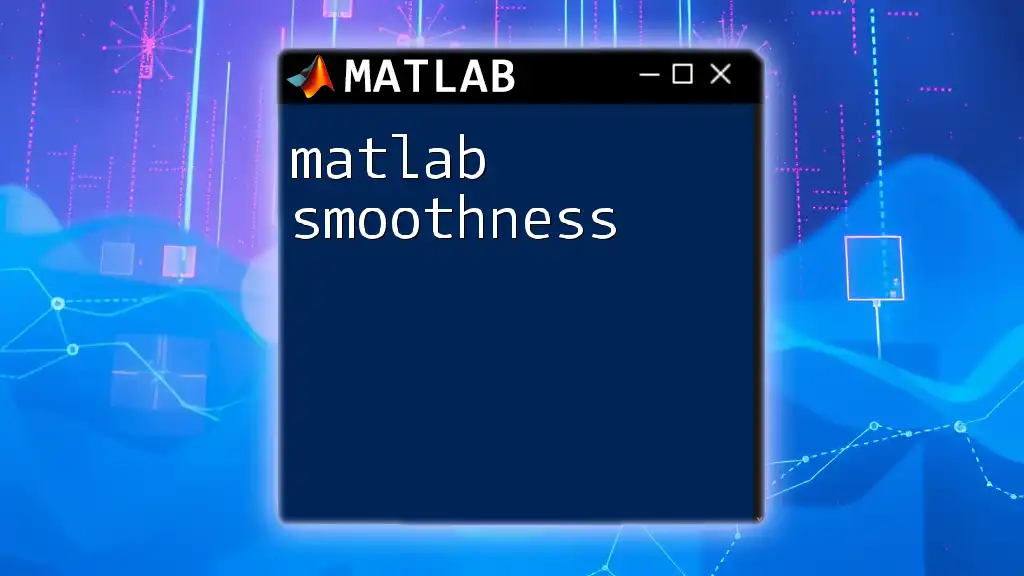
Troubleshooting Common Issues
When working with MATLAB symbolic, it’s essential to be aware of common pitfalls. Errors often arise from incorrectly declared variables or misunderstandings of symbolic functions. Always ensure that your symbolic variables are declared with `syms` before using them in expressions or equations.
If you encounter errors, refer to MATLAB’s documentation or forums to find solutions, as many common challenges have been discussed and resolved by the community.
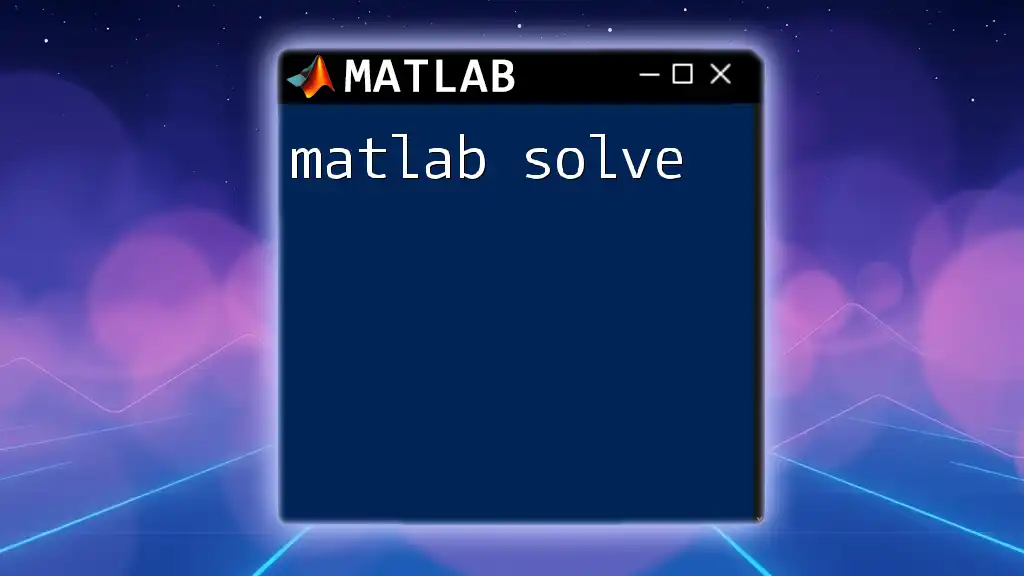
Conclusion
The MATLAB Symbolic Toolbox is an indispensable resource for professionals in engineering, science, and mathematics. By mastering its features—from declaring symbolic variables to performing complex integrations and differentiations—you can unlock a realm of possibilities for symbolic computation. This guide serves as a basis for further exploration, encouraging you to dive into the powerful capabilities that MATLAB offers in symbolic mathematics.
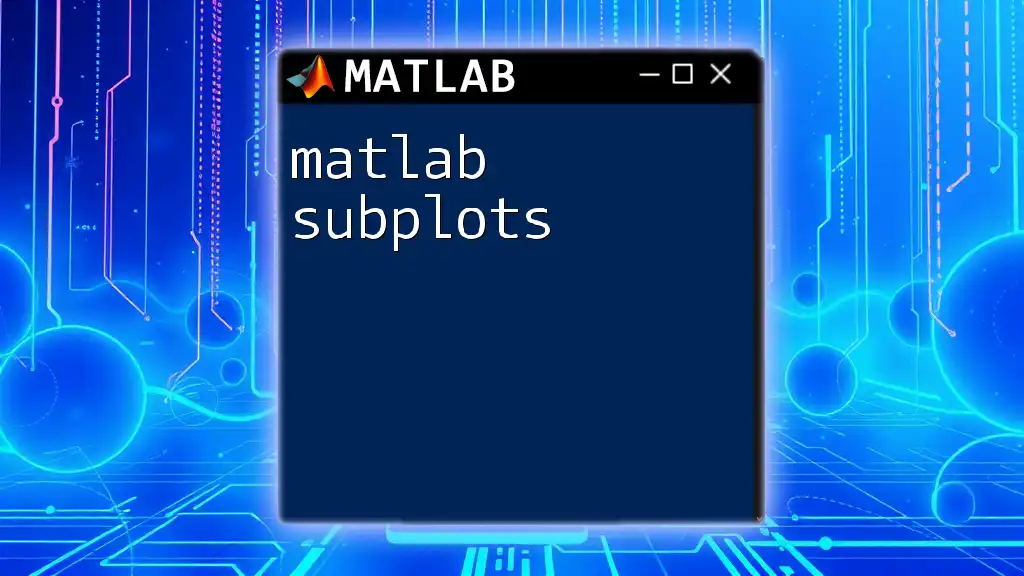
Additional Resources
For further learning, consider exploring textbooks on symbolic computation, MATLAB's official documentation, and interactive tutorials available online. These resources will deepen your understanding and enhance your skills in MATLAB symbolic operations.