In MATLAB, you can append values to an existing array by using the concatenation operator, which combines the original array with the new values you want to add.
A = [1, 2, 3]; % Original array
A = [A, 4, 5]; % Appending values 4 and 5
Understanding MATLAB Arrays
What is an Array in MATLAB?
In MATLAB, an array is a fundamental data structure that allows you to store and manipulate collections of numbers or other data types. The power of MATLAB lies in its handling of arrays, which can be one-dimensional (vectors), two-dimensional (matrices), or even multi-dimensional. Each type of array serves a different purpose, but they all share common functionalities.
Arrays are crucial for organizing and manipulating data, whether you're performing mathematical computations, data analysis, or creating algorithms. Understanding how to effectively manage arrays is essential for anyone looking to leverage the full capabilities of MATLAB.
Basic Array Creation
Creating an array in MATLAB is straightforward. You can use square brackets `[]` to define an array, and separate elements with commas or spaces. For example, a simple array can be created as follows:
myArray = [1, 2, 3, 4];
This creates a one-dimensional array with four elements. For multi-dimensional arrays, you can use semicolons to separate rows:
myMatrix = [1, 2; 3, 4; 5, 6];
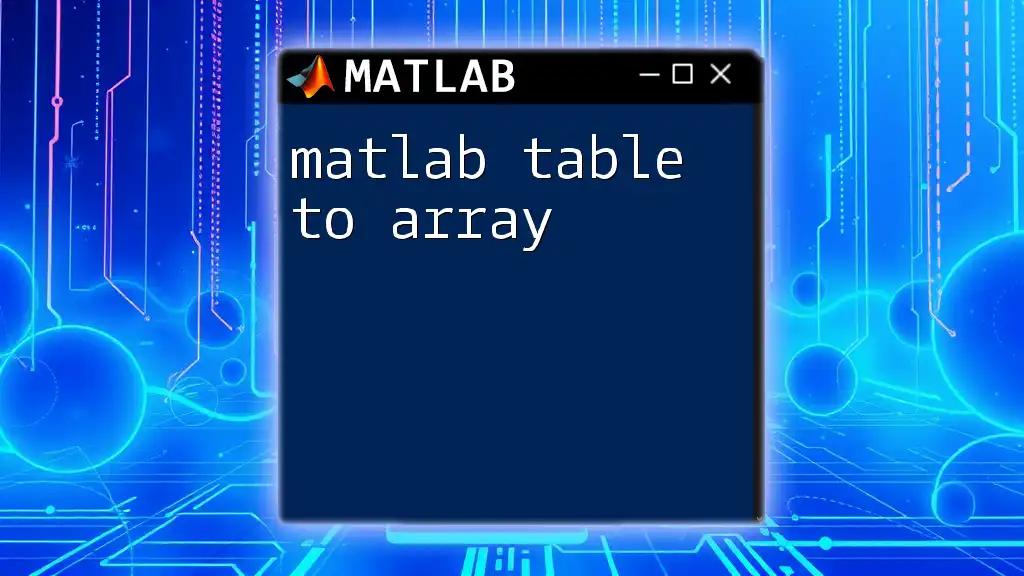
Appending Elements to Arrays
The Concept of Appending
Appended elements are new items added to an existing array. Appending is a common operation when you want to expand the dataset incrementally. This could be the addition of new measurements, results from calculations, or the outcome of any iterative processes. Knowing how to append effectively helps maintain data integrity and structure.
Various Methods to Append to an Array
Method 1: Using Concatenation
MATLAB provides a flexible way to combine arrays using concatenation. There are two main types: horizontal and vertical concatenation.
-
Horizontal Concatenation combines arrays side by side. Here’s an example:
A = [1, 2, 3]; B = [4, 5]; C = [A, B]; % Result: [1, 2, 3, 4, 5]
-
Vertical Concatenation stacks arrays on top of each other. Here’s how you can do it:
A = [1; 2; 3]; B = [4; 5]; C = [A; B]; % Result: [1; 2; 3; 4; 5]
This versatility in concatenating arrays allows for flexible data organization as you grow your datasets.
Method 2: Using the `end` Keyword
The `end` keyword in MATLAB is a powerful tool for dynamically appending elements to an array. It signifies the last index of the array and allows you to add new elements without needing to know the current size of the array. Here's an example of adding a single element using this method:
A = [1, 2, 3];
A(end + 1) = 4; % Result: [1, 2, 3, 4]
This approach is intuitive and makes appending new data straightforward.
Method 3: Using the `append` Function
Currently, MATLAB does not have a dedicated `append` function for arrays. Instead, you should rely on concatenation, as explained previously. Using built-in MATLAB operations efficiently is essential for optimal code performance.
Method 4: Appending Rows or Columns to a Matrix
When working with matrices, appending can also involve adding entire rows or columns. Here’s how to append a new row to a matrix:
matrix = [1, 2; 3, 4];
newRow = [5, 6];
matrix = [matrix; newRow]; % Result: [1, 2; 3, 4; 5, 6]
This method allows for substantial manipulation of matrix structures as you expand your datasets.
Performance Considerations
When appending elements to large arrays, performance can be a concern. Each time you append, MATLAB may need to allocate new memory for the array, which can slow down your code significantly, particularly in loops. To mitigate this, consider preallocating arrays to the maximum required size initially, which can enhance efficiency during iterative processes.
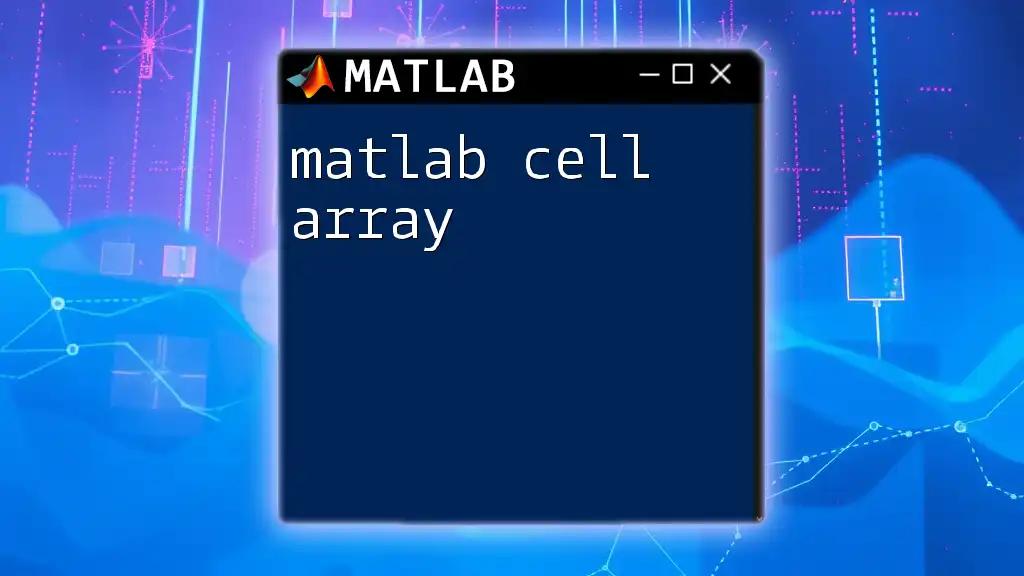
Common Pitfalls When Appending
Data Type Mismatch
One common issue that arises during array appending is the data type mismatch. In MATLAB, arrays must contain elements of the same type. Attempting to append a different data type can result in an error. For instance:
A = [1, 2, 3];
% Trying to append a string will cause an error
A(end + 1) = 'text'; % Error: cannot convert to type double.
Always ensure that the types of the elements you are appending match the existing array.
Appending in a Loop
Appending elements in a loop without preallocating your array can lead to inefficient memory usage and performance issues. When you repeatedly append elements, MATLAB dynamically resizes the array, which can be computationally expensive. Here's a comparison:
-
Inefficient appending in a loop:
for i = 1:100 A(i) = i; % Not preallocated end
-
Preallocated version:
A = zeros(1, 100); % Preallocate for i = 1:100 A(i) = i; % Efficient end
Preallocating public arrays saves time and resources, creating more efficient code.
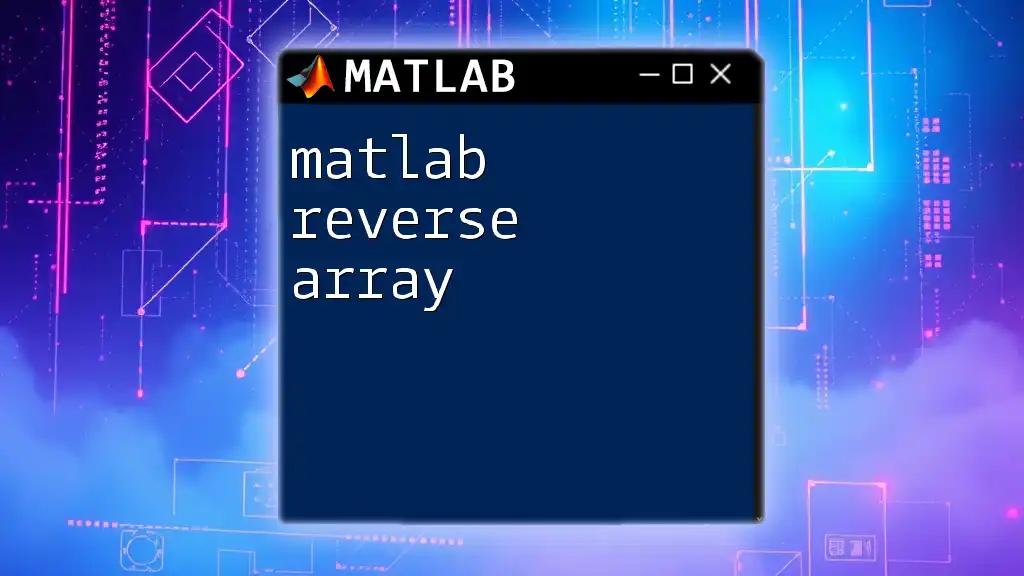
Best Practices for Appending to Arrays
To ensure efficient code when using MATLAB, follow these best practices:
- Always preallocate large arrays to minimize performance issues.
- Leverage built-in functions wisely for array manipulation.
- Be mindful of data types during appending to avoid errors.
By adhering to these best practices, you can significantly enhance the performance and efficiency of your MATLAB scripts.

Conclusion
Knowing how to append to arrays in MATLAB is a vital skill that enhances your capability to manipulate data efficiently. By understanding the various methods of appending, common pitfalls, and best practices, you can work effectively with arrays and maintain the integrity of your data processes.
With practice, these techniques will become second nature, allowing you to focus on more complex tasks and data analysis. Exploring further resources and experimenting with different array operations will solidify your understanding and improve your MATLAB skills.