The `arrayfun` function in MATLAB applies a specified function to each element of an array, returning an array of the same size with the results.
Here is a code snippet demonstrating its usage:
% Define a simple function to square a number
squareFunc = @(x) x.^2;
% Create an array
array = [1, 2, 3, 4, 5];
% Apply the function to each element using arrayfun
squaredArray = arrayfun(squareFunc, array);
% Display the result
disp(squaredArray);
What is `arrayfun`?
`arrayfun` is a powerful and flexible function in MATLAB that allows users to apply a specified function to each element of an array. This capability promotes cleaner code and often results in faster execution times compared to traditional looping constructs. The core idea of `arrayfun` is enabling a functional programming style, wherein functions are treated as first-class objects.
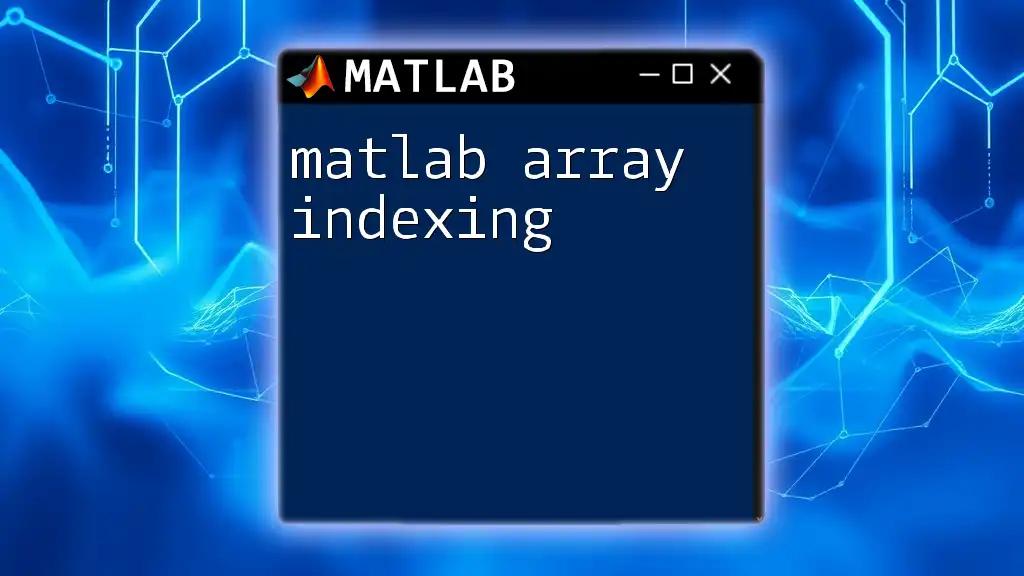
Why Use `arrayfun`?
Using `arrayfun` offers numerous advantages:
-
Code Readability: It often makes the code much more concise and expressive compared to for-loops. Instead of writing multiple lines for a loop, a simple one-liner can achieve the same result.
-
Performance Improvements: When working with large datasets, `arrayfun` can be optimized to run faster since MATLAB implements specific algorithms for its operations that can outperform standard loops.
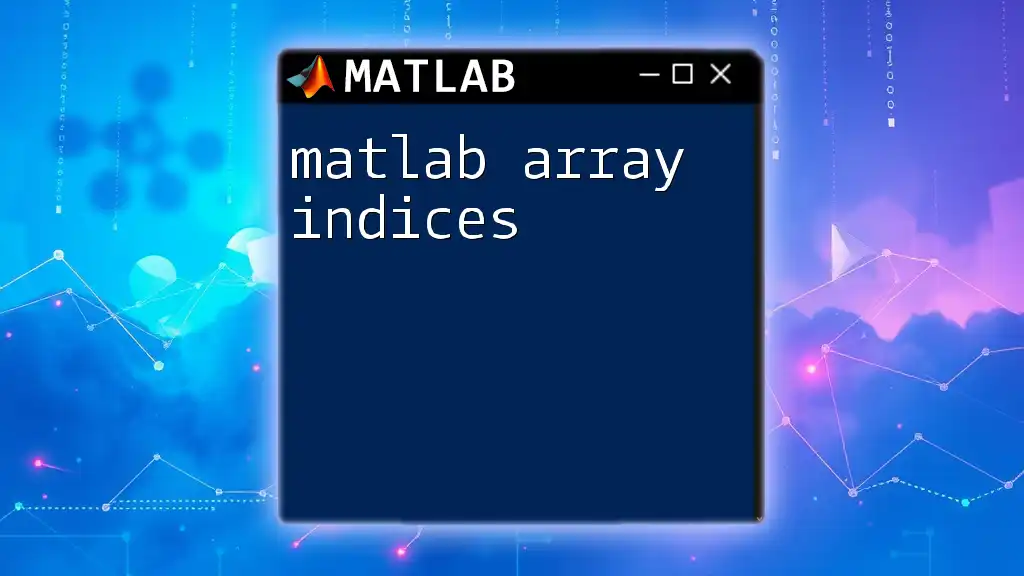
Syntax of `arrayfun`
To utilize `arrayfun`, the syntax is straightforward:
B = arrayfun(func, A)
Here, `func` refers to the function that you want to apply to each element of the array `A`, and `B` will contain the result of applying `func` to `A`. The output can be a numeric array or a cell array, depending on the type of function you use.
Return Types of `arrayfun`
The return type of `arrayfun` depends on whether the function provided returns scalar values or non-scalar values. If the output is consistently of the same type and size, `arrayfun` generates an array output. If not, it returns a cell array to accommodate varied sizes and types of data.
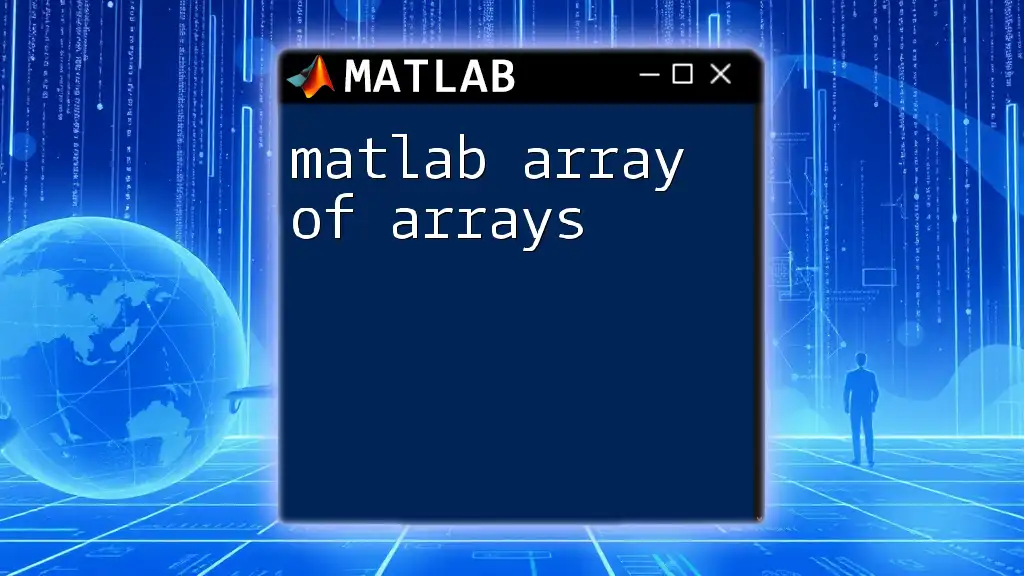
How to Use `arrayfun`
Basic Examples
Applying a Simple Function
A basic use of `arrayfun` could be to square each element of an array:
A = [1, 2, 3, 4];
B = arrayfun(@(x) x.^2, A);
disp(B);
In this example, the array `A` becomes `[1, 4, 9, 16]` after applying the squaring function to each element.
Using Anonymous Functions
More complex operations can also be easily applied using anonymous functions. For instance, if you want to find the cube of each element:
A = [1, 2, 3, 4];
cube = @(x) x^3;
B = arrayfun(cube, A);
disp(B);
Here, `B` will result in `[1, 8, 27, 64]`.
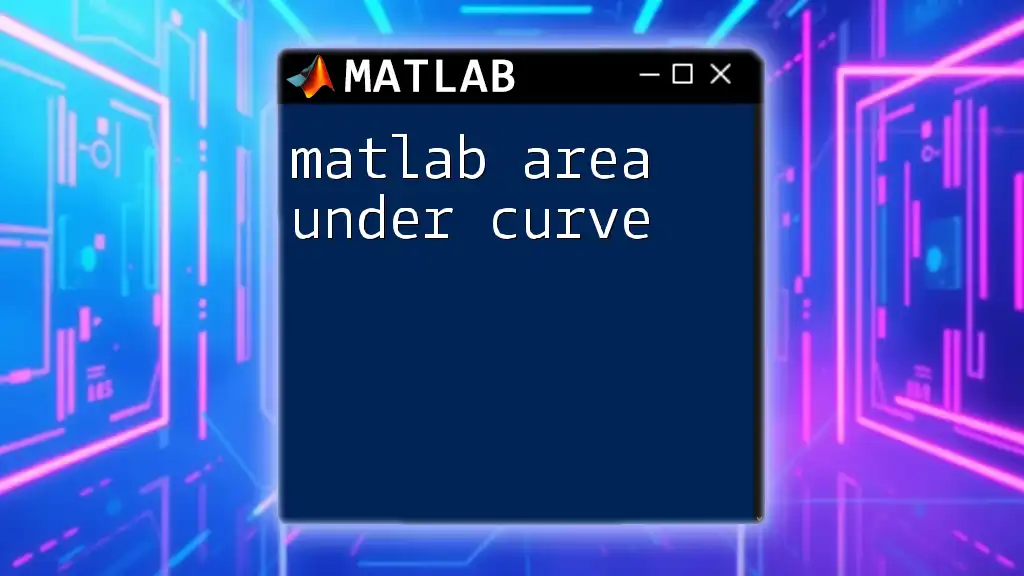
Working with Different Data Types
Numeric Arrays
`arrayfun` excels with numeric data, easily applying mathematical functions across an entire dataset.
Cell Arrays
Using `arrayfun` with Cell Arrays
Cell arrays present a unique challenge as they can contain different data types. `arrayfun` can handle this gracefully:
C = {'hello', 'world'};
uppercaseC = arrayfun(@upper, C, 'UniformOutput', false);
In this example, `uppercaseC` would contain `{'HELLO', 'WORLD'}` as the result of converting each string to uppercase.
Struct Arrays
Accessing and Modifying Struct Fields
`arrayfun` can also be used to modify fields in an array of structures. For example, if you have a struct where you want to double the value field:
S(1).value = 10; S(2).value = 20;
S = arrayfun(@(x) setfield(x, 'value', x.value * 2), S);
This will update the `value` field of each struct to 20 and 40 respectively.
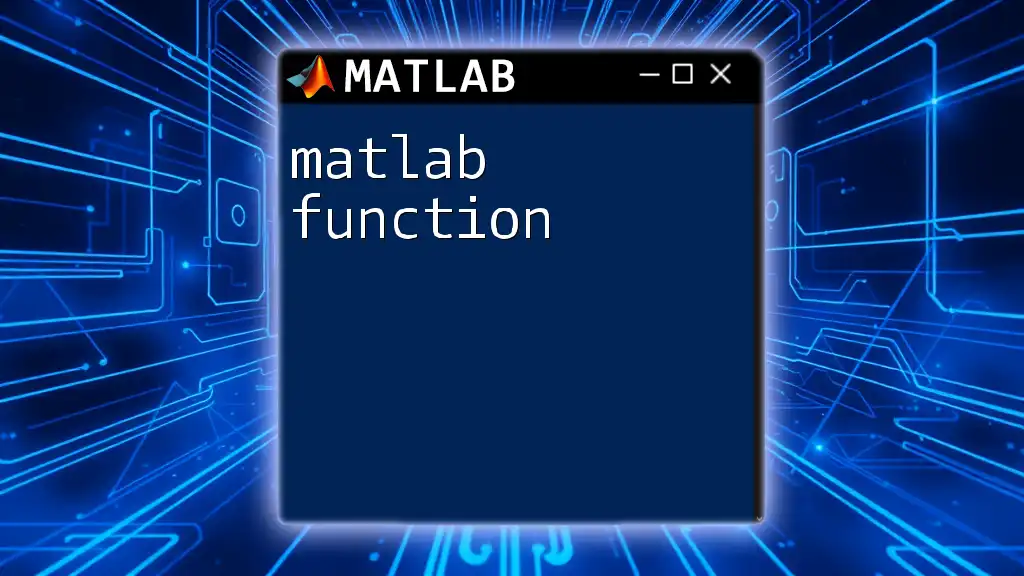
Advanced Usage of `arrayfun`
Specifying Additional Parameters
Sometimes, the function you want to apply requires additional parameters. You can specify such parameters directly in the call to `arrayfun`:
addFunc = @(x, y) x + y;
B = arrayfun(addFunc, A, 5); % Adds 5 to each element of A
Now, if `A` was `[1, 2, 3]`, `B` will yield `[6, 7, 8]`.
Handling Variable-Length Outputs
It's also possible to use `arrayfun` with functions that yield variable-length outputs. For example:
funcVarLength = @(x) {x, x^2};
C = arrayfun(funcVarLength, A, 'UniformOutput', false);
In this case, `C` would be a cell array containing pairs of each element and its square.
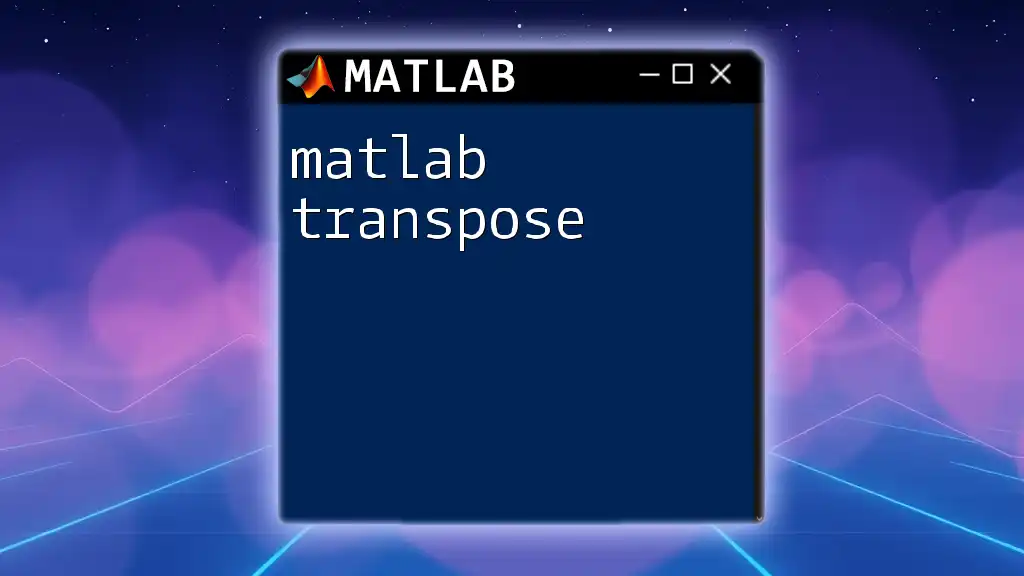
Performance Considerations
Comparing `arrayfun` with For-Loops
When working with large arrays, `arrayfun` can often outperform for-loops due to MATLAB's internal optimization strategies. It eliminates the overhead of indices management and function calls in loops, which can significantly reduce execution time.
Memory Usage and Optimization Tips
While `arrayfun` is efficient, it can consume memory differently than traditional loops, particularly if the function returns large arrays. Keep an eye on variable sizes and types, especially when operating with larger datasets.
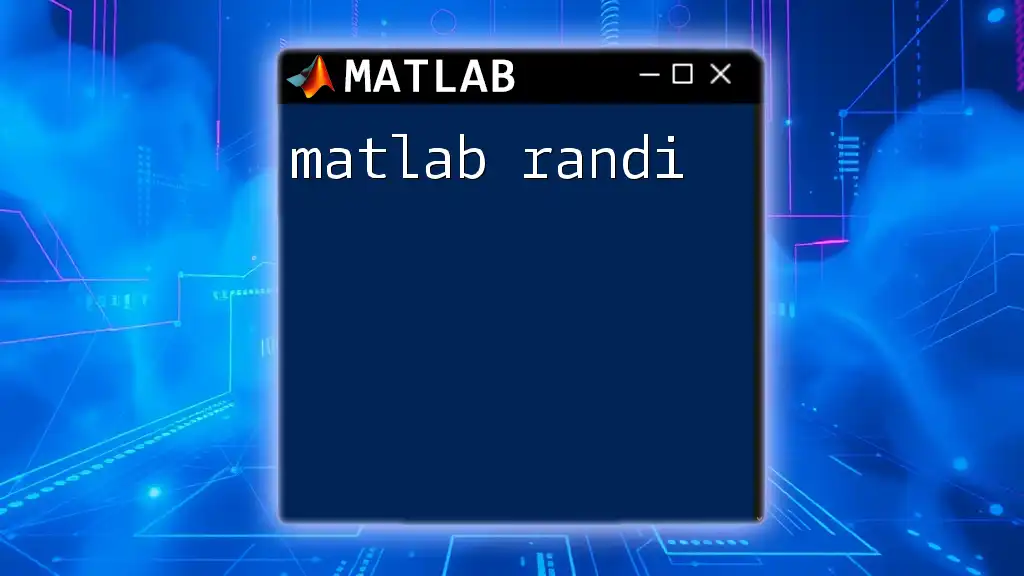
Common Pitfalls and Troubleshooting
Understanding Output Dimensions
One common issue is related to the output dimensions, especially when combining outputs of varying sizes. Ensure that the function applied produces outputs that are of consistent dimensions if you desire a straightforward numeric return.
Debugging Tips
If you encounter issues, consider using breakpoints to inspect the function’s behavior or test smaller subsets of your data to isolate problems.
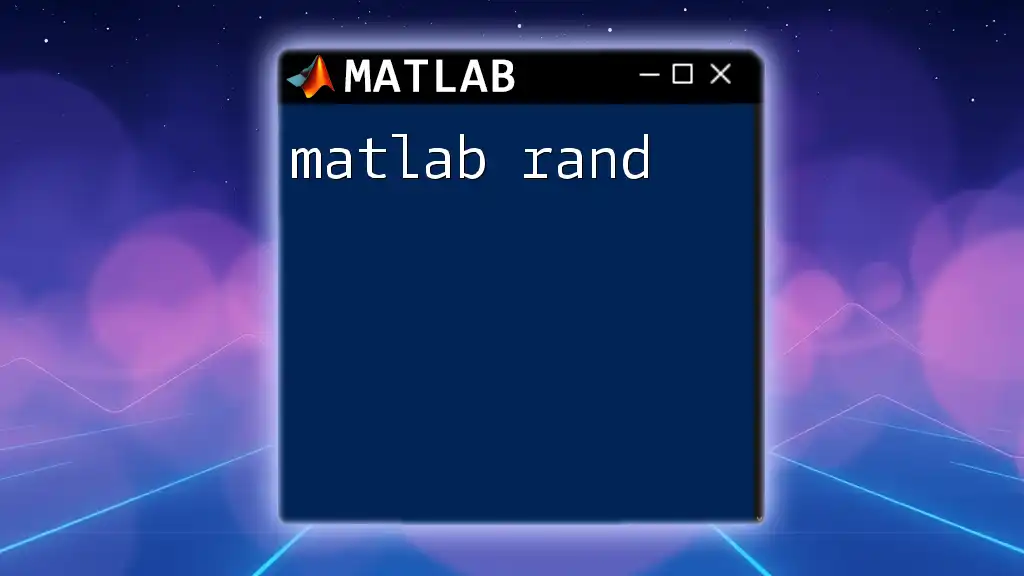
Practical Applications of `arrayfun`
Use Cases in Data Analysis
`arrayfun` is particularly useful in data manipulation tasks, where you might need to process large datasets quickly, such as applying transformations or computations across columns or rows of data.
Image Processing Applications
In image processing, `arrayfun` can be effectively used for pixel-level manipulations, such as filtering operations or adjustments based on pixel values.
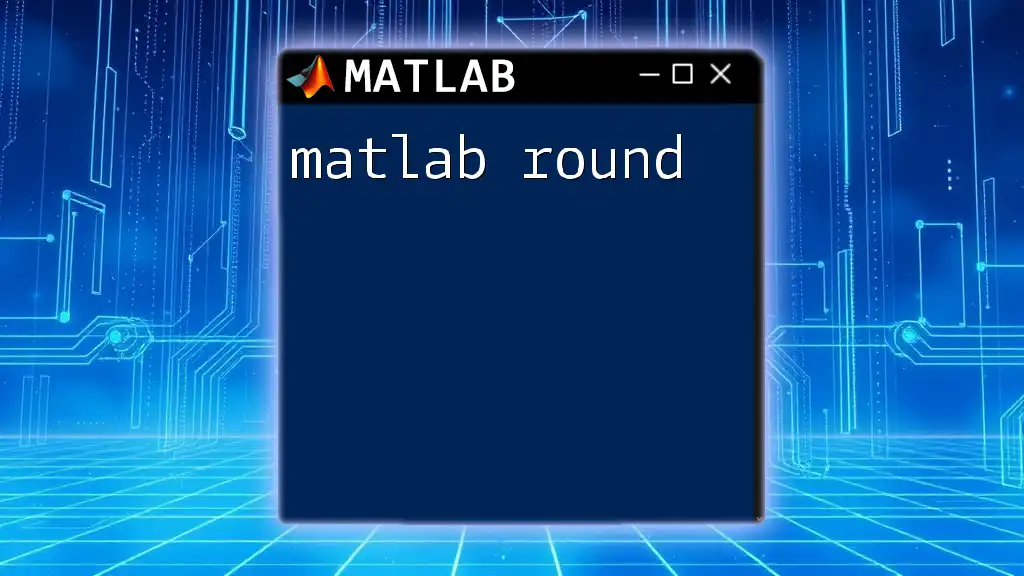
Conclusion
In this guide, we thoroughly explored the `matlab arrayfun` function, uncovering its syntax, basic and advanced usage, and real-world applications. With its ability to streamline coding and improve performance, `arrayfun` is an essential tool in the MATLAB arsenal. We encourage you to experiment and explore the capabilities that `arrayfun` offers for various functions in your data-related tasks.
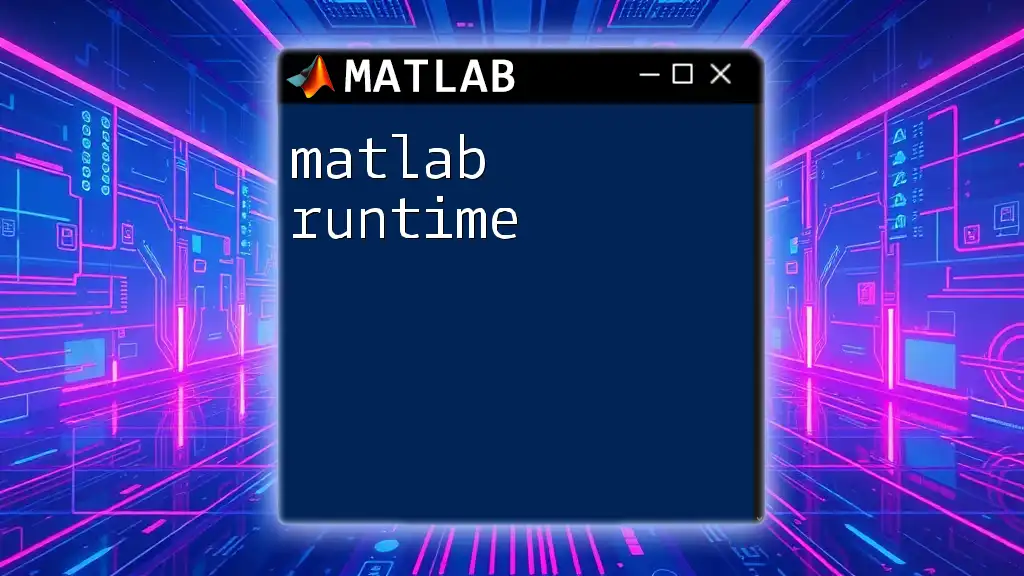
Additional Resources
For a deeper dive into the topic, consider consulting the official MATLAB documentation on `arrayfun`, where you will find additional examples, nuances, and tips for best practices. Exploring online courses or specialized blogs on MATLAB can also enhance your understanding and capabilities with this powerful function.