In MATLAB, an array of arrays, often referred to as a cell array, allows you to store arrays of different sizes and types within a single variable.
C = {1, [2, 3, 4], 'Hello'; [5, 6], 7, {8, 9}};
Understanding MATLAB Arrays
Types of Arrays
1D Arrays (Vectors)
In MATLAB, a one-dimensional array, or vector, is a fundamental structure used to store a sequence of numbers or characters. For example, a row vector can be created as follows:
row_vector = [1, 2, 3, 4, 5];
A column vector can be generated similarly:
column_vector = [1; 2; 3; 4; 5];
Both forms are essential for performing calculations and operations in MATLAB.
2D Arrays (Matrices)
Two-dimensional arrays, or matrices, extend this concept, allowing for tabular data representation. They can be initialized with consistent row lengths:
matrix = [1, 2, 3; 4, 5, 6; 7, 8, 9];
Matrices play a critical role in linear algebra, data processing, and image manipulation.
What Is an Array of Arrays?
Definition and Structure
An "array of arrays" in MATLAB refers to a structure where one array contains other arrays. This concept allows you to create hierarchical data structures and represents complex data types efficiently.
Applications
Arrays of arrays can be particularly useful in fields such as data analysis, machine learning, and image processing, where complex data organization is necessary. For instance, arrays of arrays enable the structuring of student records with names and grades, facilitating easier data handling and manipulation.
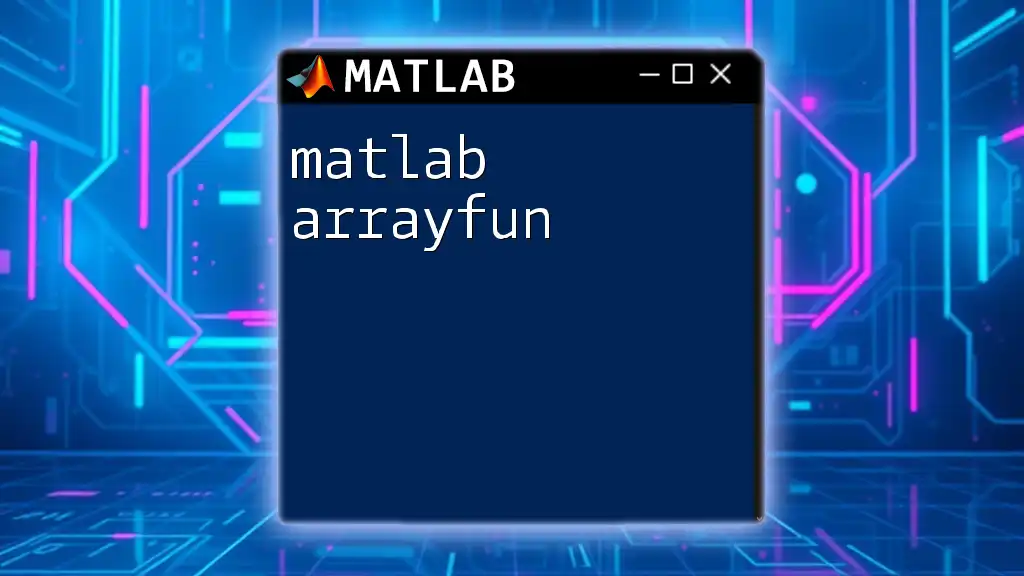
Creating Arrays of Arrays in MATLAB
Using Cell Arrays
What Are Cell Arrays?
Cell arrays differ from regular arrays in that they can hold varying types of data, including numbers, strings, and other arrays. This flexibility makes them ideal for creating arrays of arrays.
How to Create a Cell Array
You can create a cell array using curly brackets `{}`. For instance:
C = {1, 2, [3, 4]; 'a', 'b', 'c'};
This cell array comprises numeric values and character arrays, demonstrating its versatile capabilities. The first row can contain numbers while the second row has characters, showcasing how cell arrays can hold different data types seamlessly.
Using Multidimensional Arrays
Creating Multidimensional Arrays
Multidimensional arrays go beyond 2D matrices, comprising numerous layers. You can concatenate matrices along a specified dimension using the `cat` function. Consider this example:
A = cat(3, [1, 2; 3, 4], [5, 6; 7, 8]);
In this case, `A` is a 3D array composed of two 2D matrices. Understanding multidimensional arrays is vital for managing complex datasets in applications like image processing, where images can be represented as 3D arrays (height, width, color channels).
Arrays of Structs
Definition of Struct Arrays
Struct arrays are a powerful way to represent data using named fields. Each element of the struct can contain various types of data, making it an excellent choice for organizing complex datasets.
Creating Struct Arrays
You can easily create struct arrays with fields in MATLAB. For example:
S(1).name = 'Alice';
S(1).age = 30;
S(2).name = 'Bob';
S(2).age = 25;
This creates an array of structs where each struct holds information about a student. By accessing different fields, you can efficiently manage and retrieve student data.
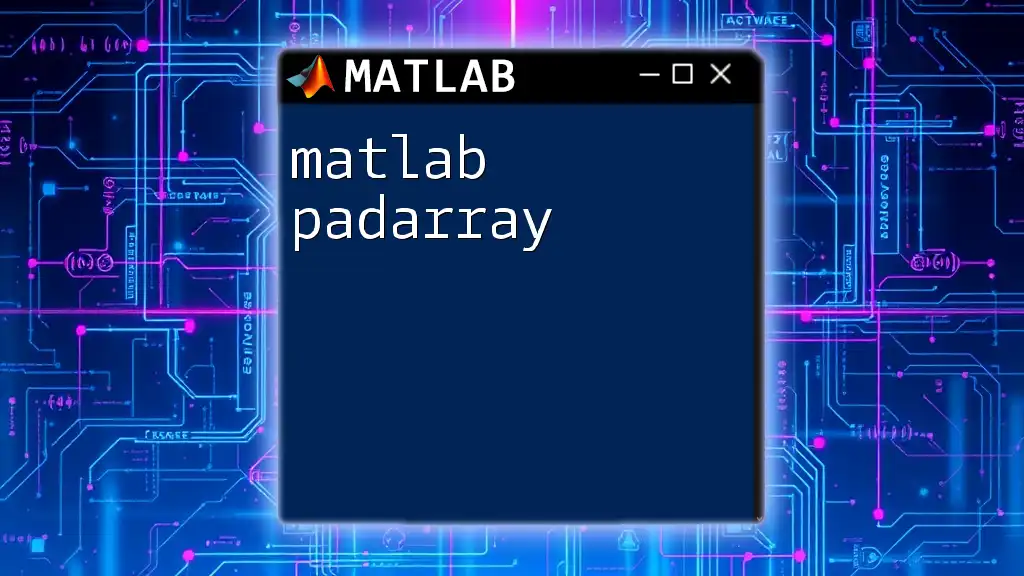
Accessing and Modifying Elements in Arrays of Arrays
Accessing Elements
Accessing Cell Array Elements
To access elements within a cell array, it is crucial to know the distinction between curly braces `{}` and parentheses `()`. Curly braces allow access to the contents of the cell, while parentheses allow access to the cell itself. For instance:
val = C{1, 3}; % Accessing the third element in the first row
This example retrieves the array `[3, 4]`.
Accessing Elements in Multidimensional Arrays
Accessing elements in a multidimensional array follows a straightforward syntax. For example:
val = A(1, 1, 1); % Accessing the first element of the first slice
This returns the value `1`, demonstrating how to pinpoint specific data within a 3D structure.
Modifying Elements
Modifying Cell Array Elements
Changing values within a cell array is simple. You can directly link a new value to a specific cell. For instance:
C{2, 1} = 'changed';
Here, the value in the cell at the second row and first column is updated to `'changed'`.
Modifying Elements in Struct Arrays
You can also update elements in struct arrays effortlessly. For example:
S(1).age = 31; % Changing Alice’s age.
This updates Alice's age, showcasing how easy it is to manage data within struct arrays.
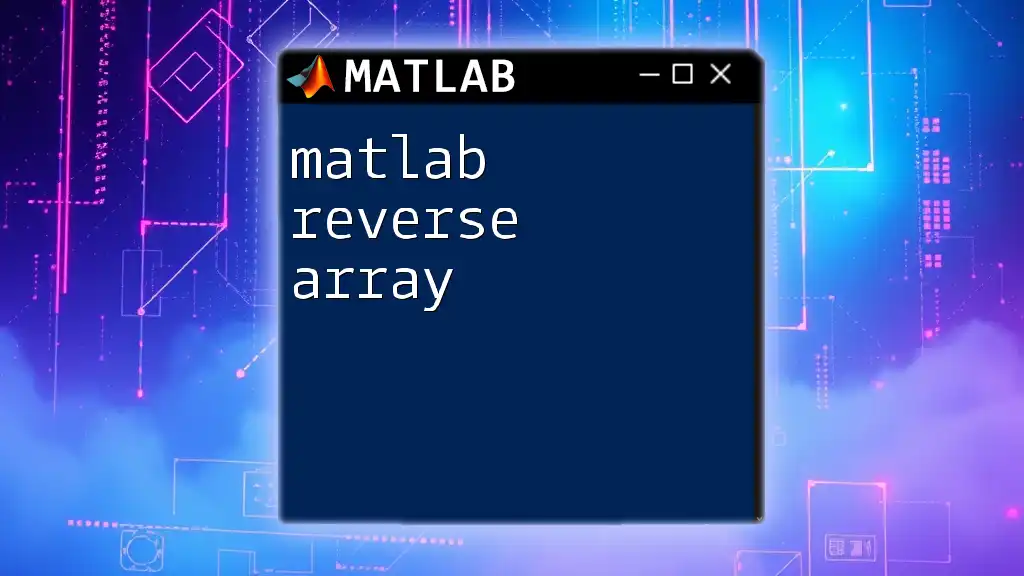
Practical Examples
Case Study 1: Student Grades
Create an Array of Arrays for Student Grades
Consider a scenario where you want to manage student grades. You can create an array of arrays like this:
grades = { 'Alice', [85, 90, 92]; 'Bob', [78, 88, 91] };
This structure allows you to store each student's name alongside their list of grades.
Access Average Grade
To calculate the average grade for a student, you can use:
average_alice = mean(grades{1, 2});
This command computes the average of Alice's grades efficiently.
Case Study 2: Image Data Handling
Using Arrays of Arrays for Image Data
In image processing, you can represent images as arrays of arrays. For instance:
R = [255, 0, 0; 255, 0, 0]; % Red channel
G = [0, 255, 0; 0, 255, 0]; % Green channel
B = [0, 0, 255; 0, 0, 255]; % Blue channel
Image = cat(3, R, G, B);
Here, `Image` holds three layers representing the RGB channels, allowing for image manipulation and processing.
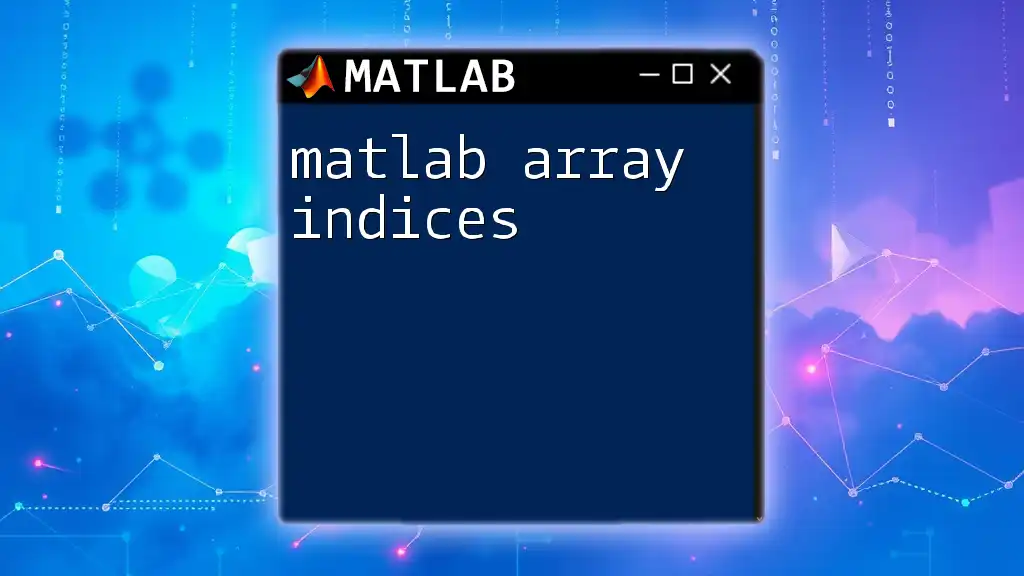
Best Practices for Working with Arrays of Arrays
Efficient Data Organization
Choosing the Right Type of Array
Understanding when to use cell arrays, multidimensional arrays, or structs is crucial for efficiency. Generally, if your data types vary, opt for cell arrays; for homogeneous data and complex structures, structs are your best bet.
Performance Considerations
Speed and Memory Efficiency
Different array types have varying implications on performance. For operations that involve repeated accesses of elements, consider multidimensional arrays as they allow for faster computation compared to cell arrays, which are more flexible but slower due to their dynamic nature.
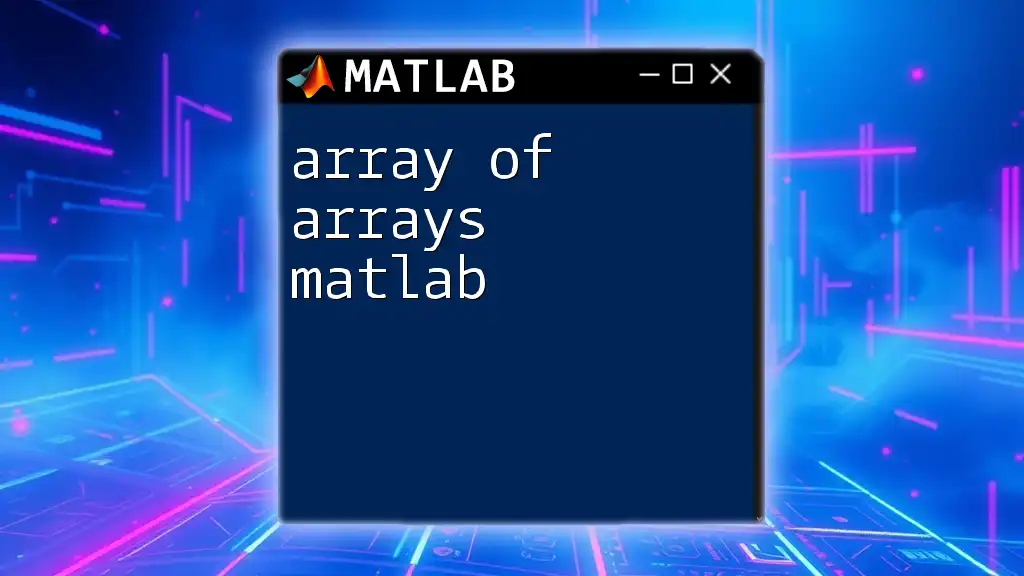
Troubleshooting Common Issues
Error Messages Explained
Common Pitfalls with Arrays of Arrays
Errors often arise from mismatched dimensions or incorrect use of syntax. Ensure you are aware of the structure you have defined when accessing elements, and always use proper indices.
Debugging Tips
Using MATLAB Debugging Tools
MATLAB provides powerful debugging tools that can help you step through code and examine the values of variables. Use breakpoints strategically to track down issues in your array handling.
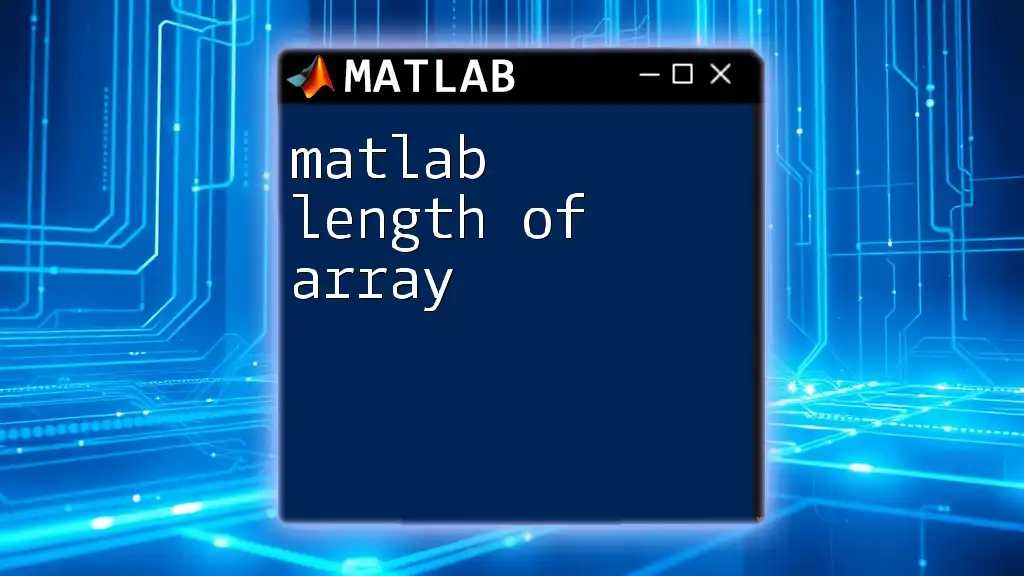
Conclusion
Recapping the concepts we've covered, matlab arrays of arrays offer a flexible and powerful means of managing complex data structures. From cell arrays to multidimensional arrays and structs, each type has specific applications and advantages. Armed with these insights, you can efficiently tackle data organization, analysis, and manipulation in MATLAB.
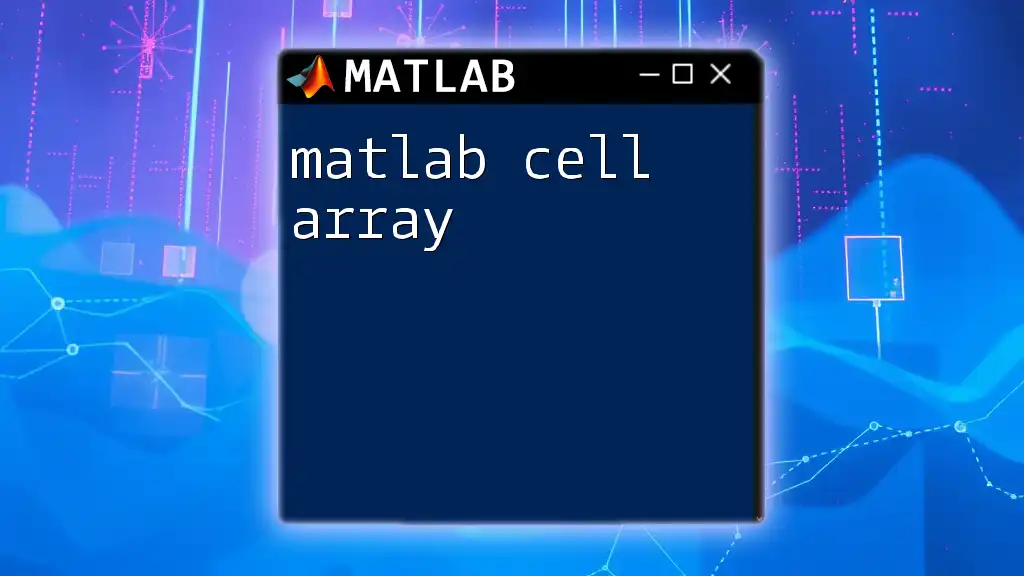
Call to Action
Try your hand at creating and manipulating arrays of arrays in MATLAB. Experiment with different scenarios, and don't hesitate to explore more tutorials on our platform for in-depth learning and practice!