A MATLAB cell array is a data type that allows you to store arrays of varying sizes and types, enabling more flexible data manipulation.
% Creating a cell array containing different types of data
myCellArray = {1, 'Hello', [2, 3, 4], true; 3.14, 'World', rand(2), false};
What is a Cell Array?
A cell array in MATLAB is a type of data structure that allows you to store arrays of varying types and sizes. Unlike standard arrays, which require all elements to be of the same type, cell arrays provide the flexibility to hold different data types, such as numeric values, strings, and even other arrays. This makes them particularly useful for handling heterogeneous data.
Why Use Cell Arrays in MATLAB?
The ability to combine various data types in a single structure is one of the primary advantages of using a MATLAB cell array. This versatility is beneficial when working with datasets that contain mixed content. For example, you might want to store a series of measurements alongside metadata. Cell arrays allow you to achieve this conveniently and efficiently.
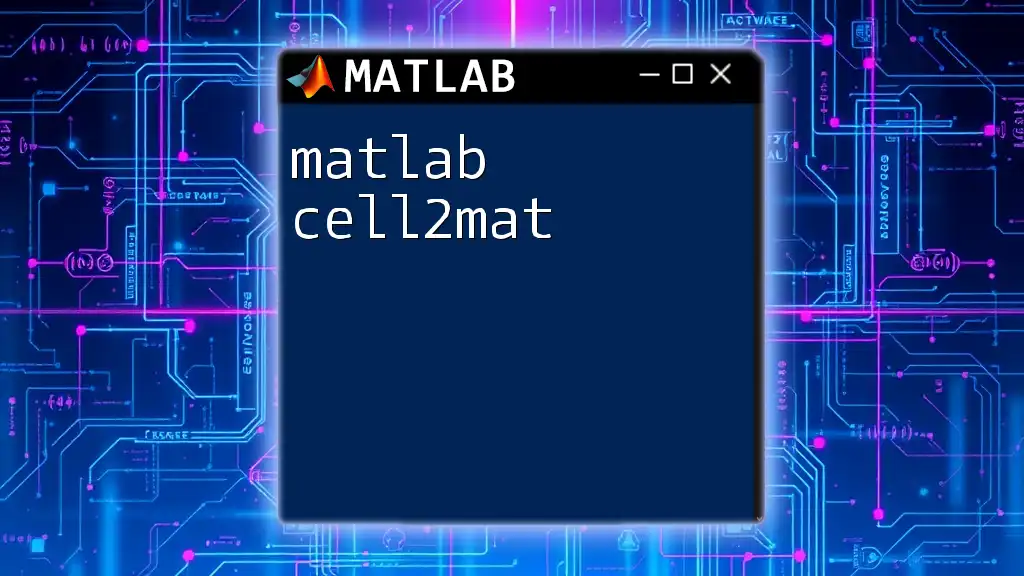
Creating Cell Arrays
Syntax for Creating a Cell Array
Creating a MATLAB cell array is straightforward. You can directly define it using curly braces. Here's a simple example:
C = {1, 'text', rand(3)};
This command creates a 1x3 cell array where:
- The first cell contains a numeric value.
- The second cell contains a string.
- The third cell contains a 3x3 matrix of random numbers.
Creating Cell Arrays with Functions
You can also initialize cell arrays using the `cell` function, which creates a cell array filled with empty values:
C = cell(3, 2); % Creates a 3x2 cell array
Another method to create a cell array is by converting an existing matrix or array into a cell format. The `num2cell` function can be useful for this purpose:
A = [1, 2; 3, 4];
C = num2cell(A);
After executing these commands, `C` will be a 2x2 cell array with each element of `A` stored in a separate cell.
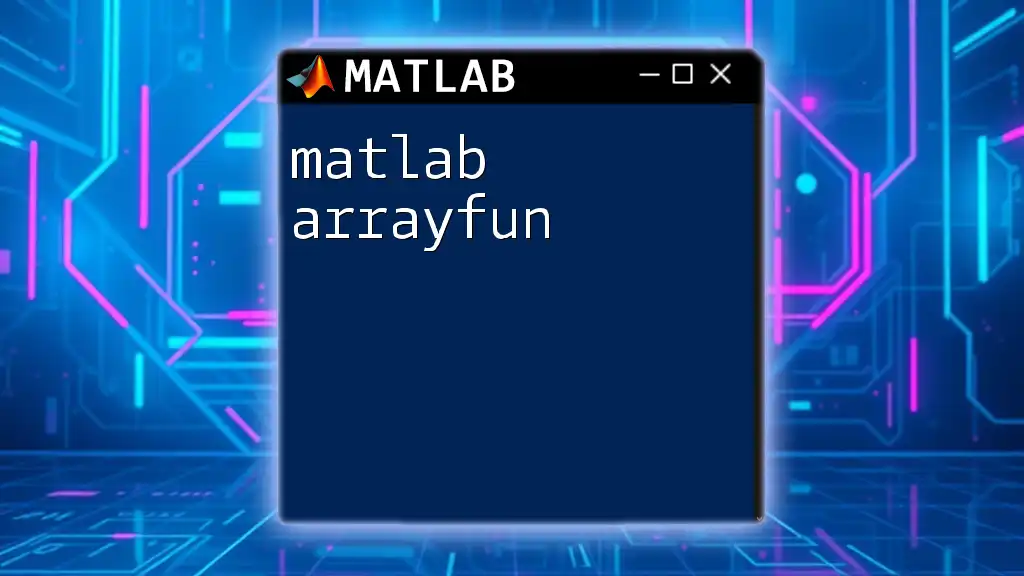
Accessing Elements in Cell Arrays
Indexing Basics
Cell arrays are accessed using two different types of indexing. Curly braces `{}` are used to access the contents of a cell, while parentheses `()` return a subarray consisting of the cells themselves. For example:
myArray = {1, 2, 3; 'A', 'B', 'C'};
val = myArray{1, 2}; % Accesses the element 2
In this case, `val` will store the numeric value `2`.
Retrieving Entire Rows or Columns
If you need to retrieve entire rows or columns from a MATLAB cell array, you can follow this approach:
To access a specific row:
row = myArray(1, :); % Retrieves first row
To access a specific column:
col = myArray(:, 2); % Retrieves second column
These commands allow for efficient manipulation and extraction of data from cell arrays.
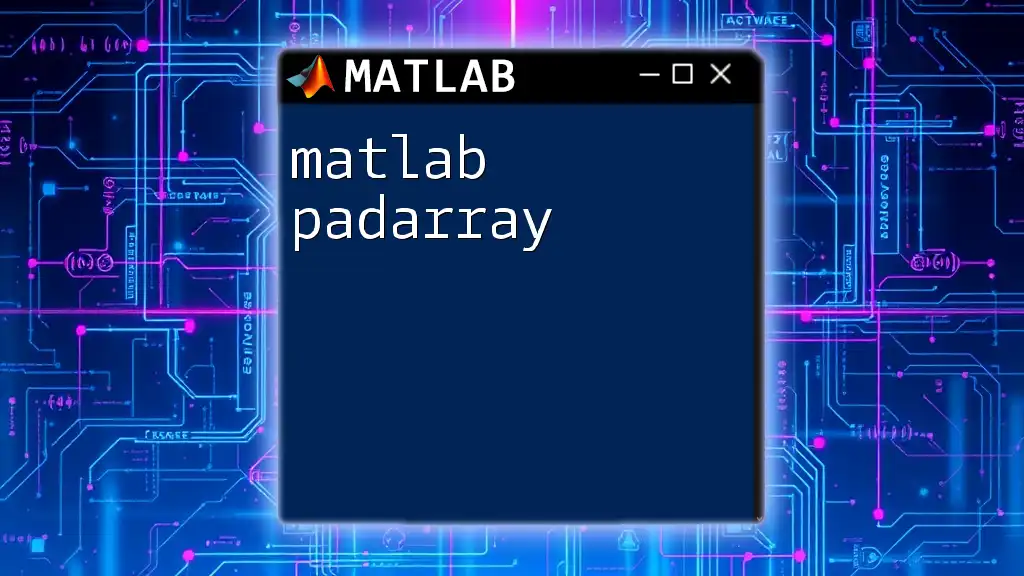
Modifying Elements in Cell Arrays
Changing Cell Contents
Updating the contents of a cell in a MATLAB cell array can be easily done using curly brace indexing:
myArray{2, 1} = 10; % Updates 'A' to 10
This line replaces the content of the second row and first column with the number `10`.
Adding New Cells
Cell arrays can grow dynamically, which makes adding new cells a breeze. You can assign a value to a cell that doesn’t currently exist:
myArray{3, 3} = 'New Entry'; % Adding a new element
This code adds a new cell at the position (3, 3) within the array, demonstrating the flexibility of working with cell arrays.
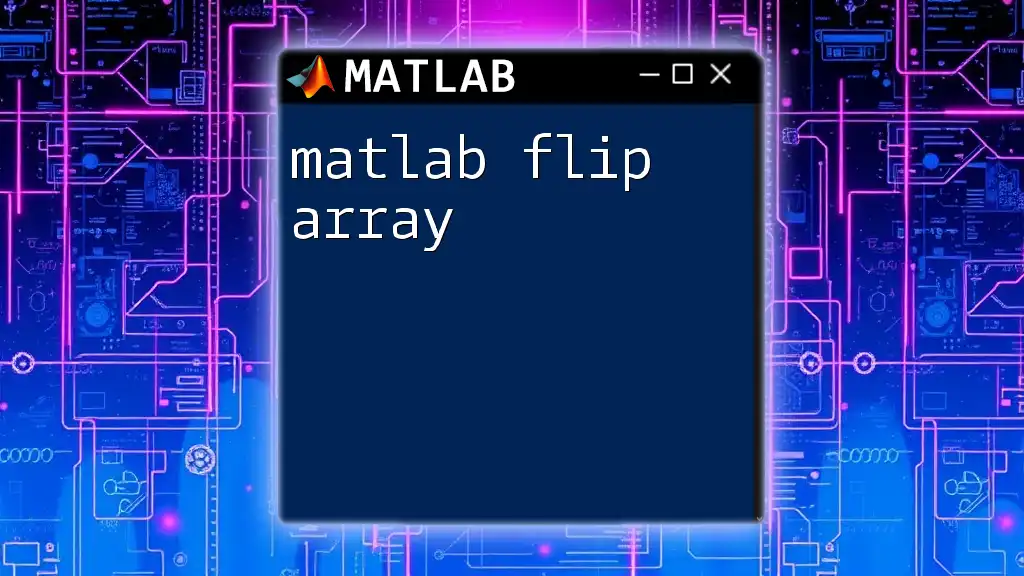
Important Functions for Cell Arrays
Common Functions Overview
Several MATLAB functions are tailored for cell array operations. One notable function is `cellfun`, which applies a specified function to every element in the cell array. For instance, if you want to obtain the lengths of strings contained in a cell array:
lengths = cellfun(@length, myArray); % Returns lengths of each cell
Other Useful Functions
The function `iscell` can be helpful when you need to confirm if a variable is a cell array:
isCellArray = iscell(myArray); % Check if it’s a cell array
Understanding these built-in functions will significantly enhance your ability to manipulate and utilize MATLAB cell arrays effectively.
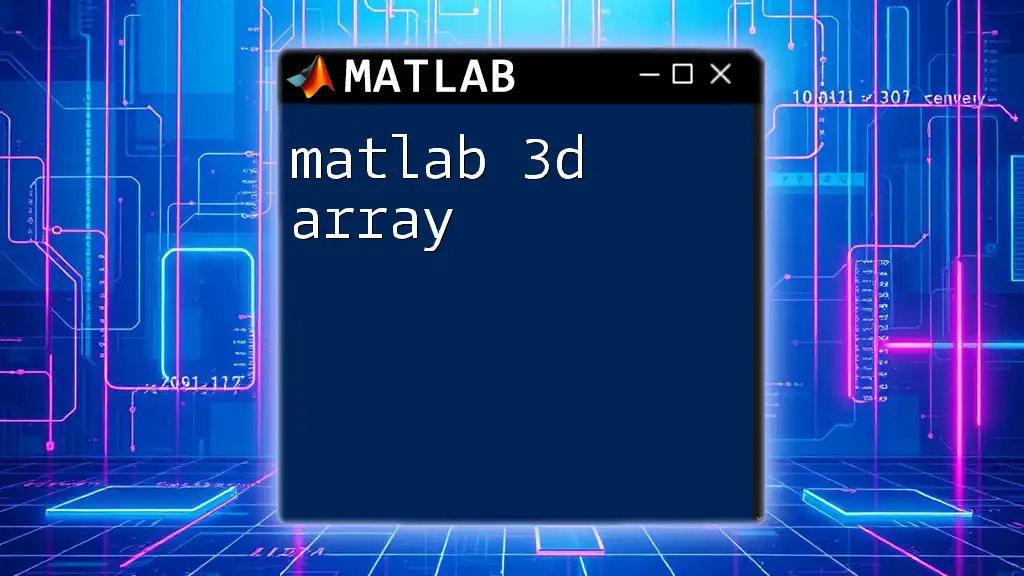
Practical Applications of Cell Arrays
Storing Mixed Data Types
Cell arrays shine when storing mixed data types. For example, if you have a dataset containing numerical values and corresponding labels, a cell array provides a clean and efficient way to manage this information. This approach is often seen in data analytics when capturing the results alongside metadata.
Dynamic Data Handling
When working with simulations or real-time data processing, you often need to update and modify data structures on the fly. Cell arrays support this functionality well, allowing you to append new data seamlessly or replace existing entries based on your needs.
File Importing and Storage with Cell Arrays
Cell arrays come handy when you read data from files, such as CSVs. MATLAB’s `readcell` function allows you to directly import data into a cell array:
C = readcell('data.csv'); % Reading a CSV file into a cell array
This command reads mixed data types from the `data.csv` file and stores them efficiently in the cell array `C`.
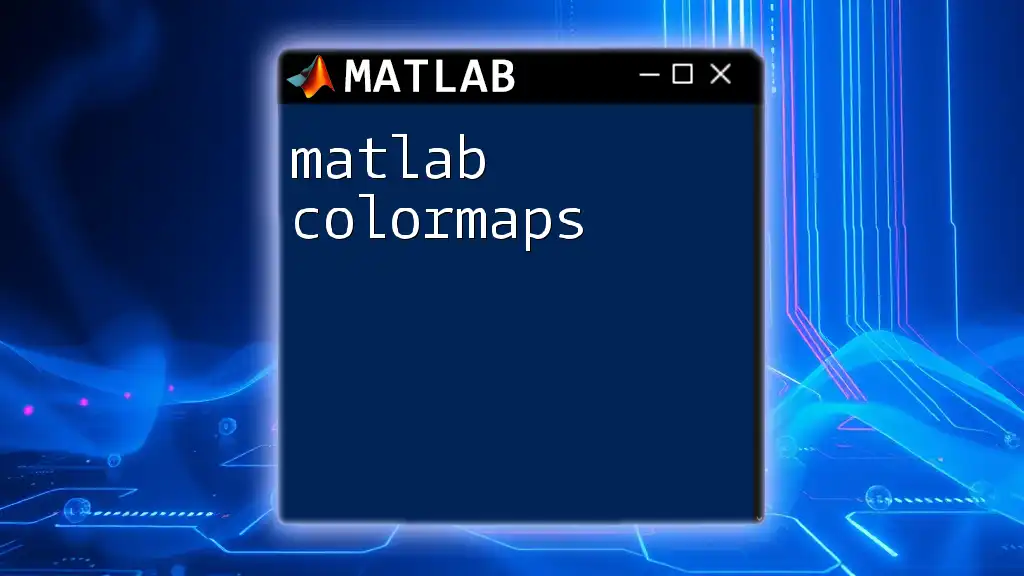
Best Practices for Using Cell Arrays
Performance Considerations
While MATLAB cell arrays are powerful, it’s essential to understand when to use them effectively. They are best suited for cases requiring mixed data types. However, if your data is uniform (e.g., all numeric), it’s often better to use standard arrays for better performance and memory efficiency.
Memory Management
You should manage memory carefully, particularly when working with larger datasets. Although cell arrays grow dynamically, excessive resizing can lead to performance issues. Preallocating the size of your cell arrays whenever possible can mitigate these concerns.
Code Clarity
Writing clear and maintainable code is crucial, especially when working with complex data structures like cell arrays. Use comments to document your code and explain what each operation does, ensuring readability for your future self or other collaborators.
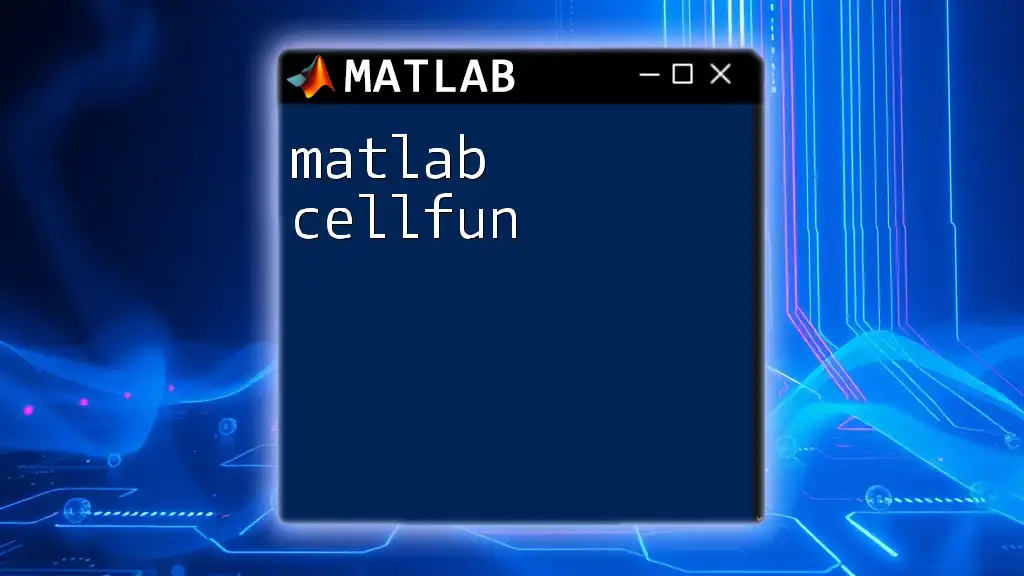
Troubleshooting Common Issues
Mismatched Data Types
One common issue when working with MATLAB cell arrays is trying to perform operations that assume uniform data types. Make sure to check the contents of each cell before applying functions that may not handle mixed data types gracefully.
Matrix Dimension Conflicts
When dynamically resizing or accessing cell arrays, you may encounter dimension conflicts. Always ensure that cells you try to access exist and that you're not attempting to retrieve elements outside the bounds of the array.
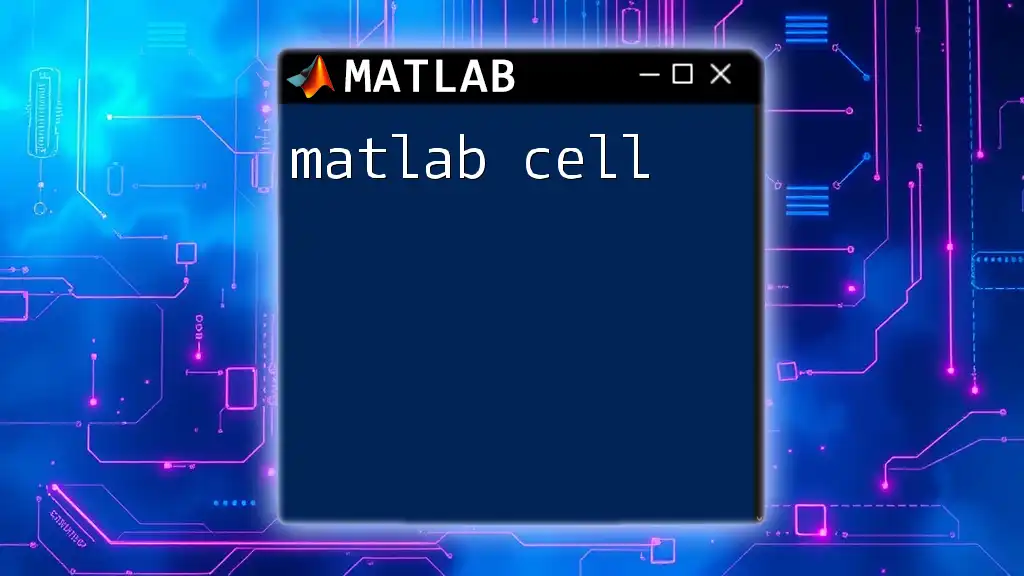
Conclusion
MATLAB cell arrays are invaluable tools for handling varied and complex datasets. Their flexibility allows developers to store multiple data types together, making them ideal for specific applications. By mastering their creation, manipulation, and troubleshooting, you enhance your programming capabilities, paving the way for effective data management and analysis.
Now that you understand the ins and outs of MATLAB cell arrays, don’t hesitate to experiment. Incorporate them into your projects, and explore their potential in your coding practices. Stay tuned for more tutorials packed with tips and tricks to boost your MATLAB skills!