The `padarray` function in MATLAB adds padding to an array with specified sizes and padding values, allowing for modifications of the array's dimensions and structure.
A = [1 2; 3 4]; % Original array
B = padarray(A, [1 1], 0); % Pad with zeros on all sides
Understanding Padding
What is Padding?
Padding, in the context of arrays, refers to the addition of extra elements around the edges of a data structure. This process is fundamental in various data analysis and processing tasks, especially in image manipulation and numerical computations. By adding padding, you create a buffer area that can help to avoid boundary issues when applying operations like convolution or when reshaping data.
Why Use Padding?
Padding becomes essential in scenarios where maintaining consistent array sizes is necessary. For instance, during the convolution of images, the edges of the original image may not produce results due to lack of neighboring data. By using padding, you ensure that all elements, including those at the borders, are considered in computations.
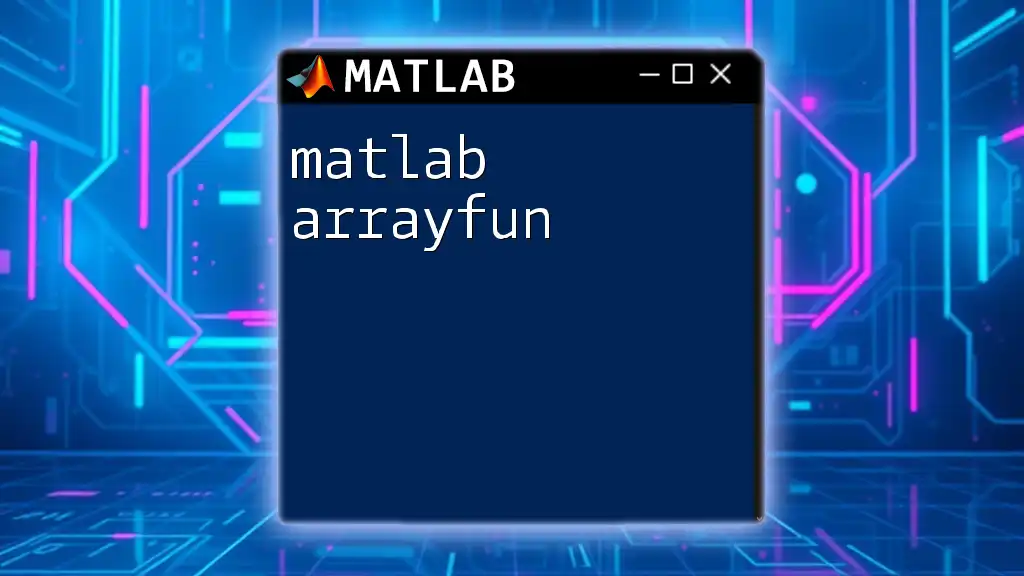
MATLAB `padarray` Function
Syntax
The basic syntax of the `padarray` function is straightforward and can accommodate various use cases:
B = padarray(A, padsize)
B = padarray(A, padsize, padval)
B = padarray(A, padsize, padval, direction)
Input Arguments
A: The Input Array
The input array, denoted as A, can be of varying dimensions including 1D, 2D, or even N-dimensional arrays. It forms the base upon which padding is to be applied.
padsize: Size of Padding
The padsize parameter specifies the amount of padding to be added along each dimension of the array. This parameter can be a single integer for uniform padding in all dimensions or a vector containing padding sizes for each dimension. For instance:
B = padarray(A, [2, 3]); % Pads 2 rows and 3 columns
In this example, the array A will receive two additional rows on top and three additional columns on the right.
padval: Value to Pad
padval allows you to define what value will be used to fill the newly created padding area. By default, MATLAB uses zeros for padding. However, if you want to use a different value (e.g., NaN, 5, etc.), you can specify it like this:
B = padarray(A, [1, 2], 0); % Zero-padding
B = padarray(A, [1, 2], NaN); % NaN-padding
Each of these examples exemplifies how to customize the padding value based on your needs.
direction: Padding Direction
The direction parameter determines where the padding is applied. It can take the following values:
- 'pre': Padding is added before the original data.
- 'post': Padding is added after the original data.
- 'both': Padding is symmetrically distributed on both sides.
Here’s how each option would work:
B = padarray(A, 2, 0, 'pre'); % Pads at the beginning
B = padarray(A, 2, 0, 'post'); % Pads at the end
By choosing the correct direction, you ensure that your data retains its integrity and intended format after padding.
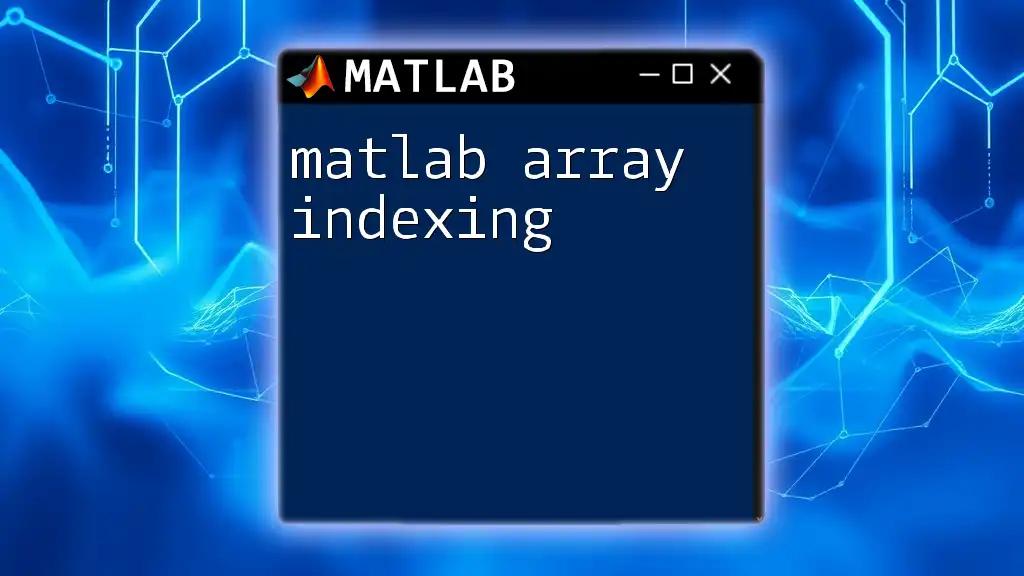
Examples of Using `padarray`
Example 1: Basic Padding
To illustrate basic padding, let’s start with a simple 2D array:
A = [1 2; 3 4];
B = padarray(A, [2, 1], 5);
In this case, the array A is padded with the value 5. The resulting array B will display five as the new padding value on two additional rows and one additional column.
Example 2: Advanced Padding Techniques
Padding is particularly useful in image processing. Let’s say we want to perform convolution on an image:
img = imread('image.png');
padded_img = padarray(img, [10, 10], 0, 'both');
Here, we’re padding the image with zeros (black) on all sides, ensuring that the convolution process can include the boundaries of the image. This approach prevents losing any critical data during computation.
Example 3: Dynamic Padding Based on Size
Sometimes, padding needs to adjust dynamically based on input size. The following function demonstrates how to implement this flexibility:
function B = dynamicPadding(A)
padSize = size(A); % Use current array size as padding sizes
B = padarray(A, padSize, 0, 'both'); % Pad with zeros on both sides
end
This function captures the dimensions of array A and applies symmetric padding, which is especially useful in modules where array dimensions might vary unpredictably.
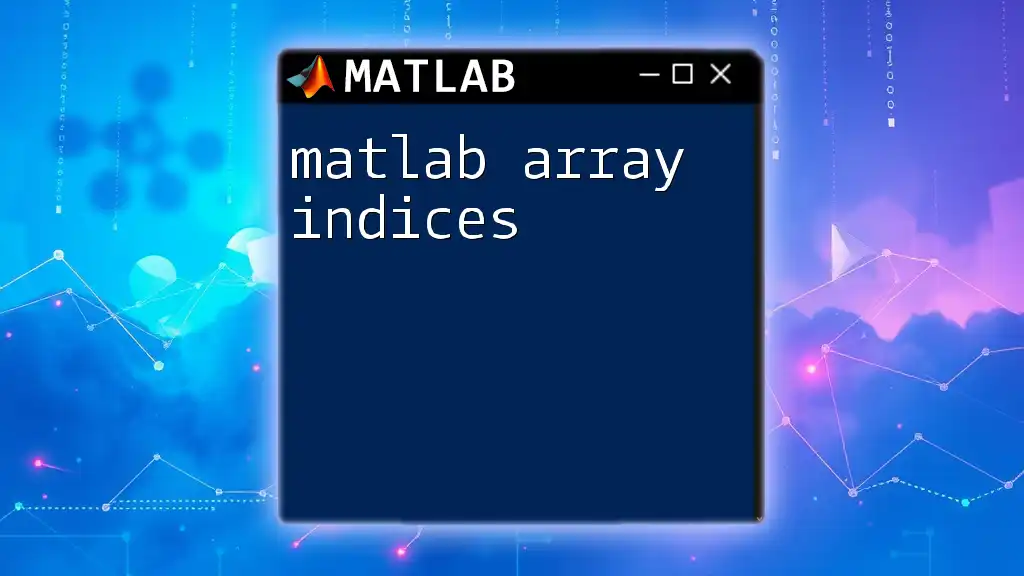
Common Errors and Troubleshooting
Error: Mismatched Dimensions
One common issue when using `padarray` arises from mismatches between array dimensions and specified padding sizes. If you attempt to add padding of incompatible sizes, MATLAB will generate an error. Ensure that your padsize matches the dimensions of your array to avoid such problems.
Error: Invalid `padsize`
Another frequent source of errors is specifying an invalid padsize. For example, trying to pad a 2D array with a 3-element vector will lead to an error since it does not correspond to the dimensions of the array. Always confirm that the padsize corresponds to the correct number of dimensions to prevent such mistakes.
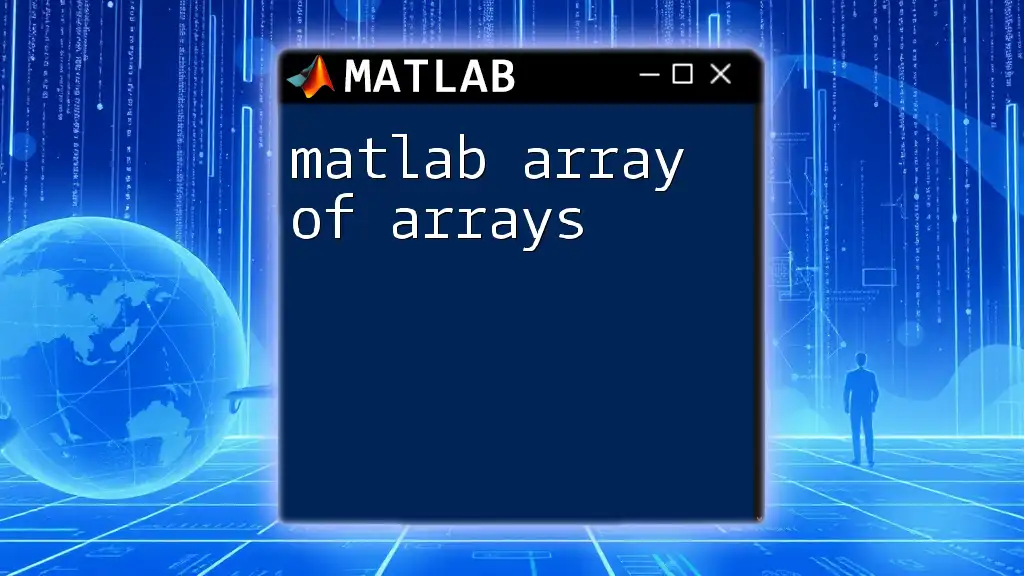
Best Practices for Using `padarray`
When utilizing the `padarray` function, consider the following best practices:
- Use Meaningful Padding Values: Choose the padding value according to the context of your data. For instance, when working with images, using zeros or NaNs can significantly affect the outcome of subsequent processing steps.
- Evaluate Necessity: Assess whether padding is necessary for your specific task, especially with large datasets, as excessive padding may lead to inefficiencies in computation and analysis.
- Monitor Performance: Be cautious with performance; using padding extensively on large arrays can increase memory usage and slow down calculations.
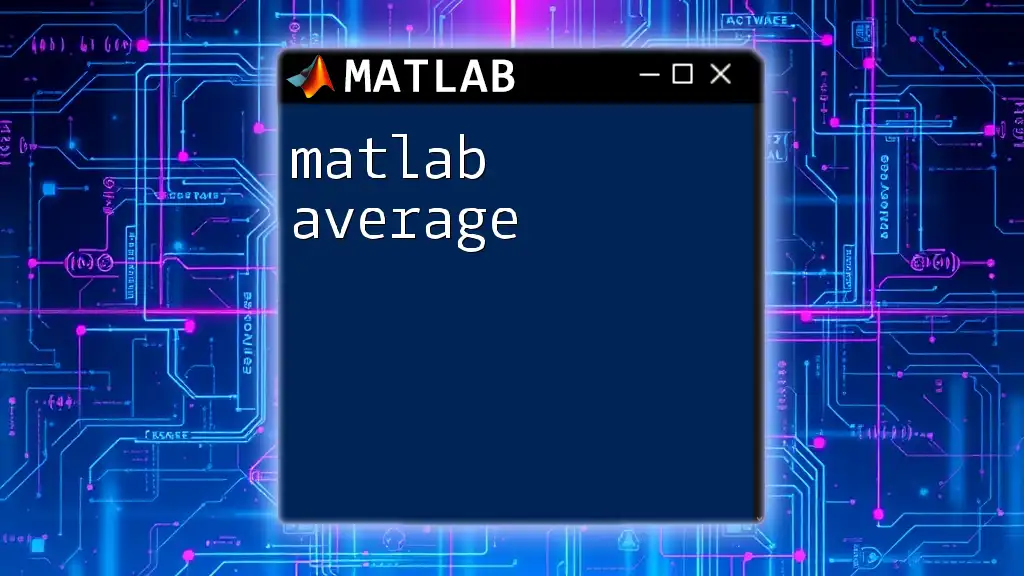
Conclusion
The `padarray` function in MATLAB is an essential tool for managing data arrays effectively, particularly in ensuring that all data gets processed during various operations. By mastering this function, you can significantly enhance your data handling skills, especially in image processing and numerical analysis. Practice with the examples provided to deepen your understanding and discover the versatile applications of `padarray` in your future projects.
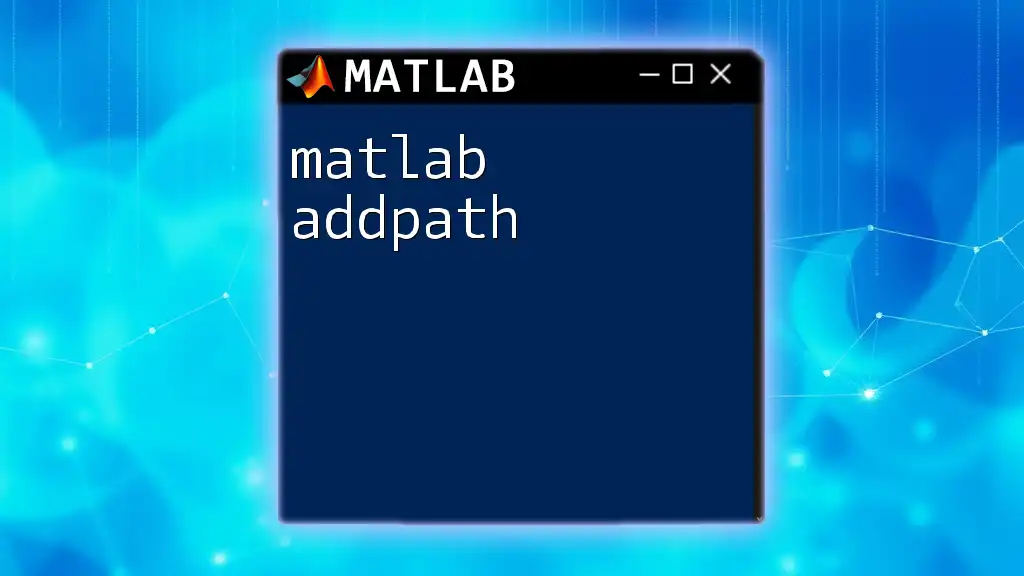
Additional Resources
Official MATLAB Documentation
For further reading and advanced topics, explore MATLAB’s official documentation on the `padarray` function to unlock more features and functionalities.
Community and Forums
Engage with MATLAB communities and forums, such as MathWorks Community, Stack Overflow, and others, to share knowledge, acquisition, and troubleshooting tips related to `padarray` and other MATLAB functions.
Future Learning Topics
Once you’re comfortable with the `padarray` function, consider exploring related subjects like image processing techniques using MATLAB, data normalization strategies, or matrix manipulation methods to widen your MATLAB expertise.