To reverse an array in MATLAB, you can use the `flip` function or indexing:
reversedArray = flip(originalArray); % Using the flip function
% or
reversedArray = originalArray(end:-1:1); % Using indexing
Understanding Arrays in MATLAB
What is an Array?
In MATLAB, an array is a fundamental data type that represents a collection of values organized in a structured format. These can be numeric, character-based, or logical. Arrays can be one-dimensional (vectors) or two-dimensional (matrices) and serve as a foundation for numerous mathematical and computational applications within MATLAB programming.
Why Reverse an Array?
Reversing an array can be crucial in various scenarios, such as data manipulation, algorithm development, and scientific computations. For instance, you might want to analyze time-series data in reverse order to identify trends, compare sequences, or even implement certain algorithms that require processing data from the end to the beginning. Understanding how to effectively reverse arrays enhances your MATLAB programming capabilities.
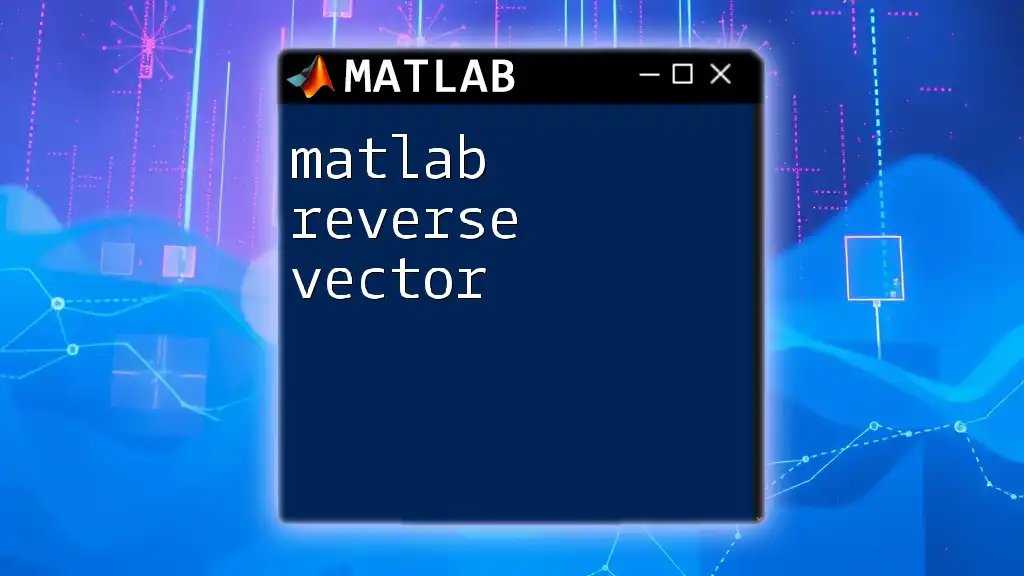
How to Reverse an Array in MATLAB
Using the `flip` Function
One of the most straightforward ways to reverse an array in MATLAB is by using the `flip` function. This built-in function is specifically designed to reverse the order of elements along a specified dimension of an array.
Example Code Snippet:
originalArray = [1, 2, 3, 4, 5];
reversedArray = flip(originalArray);
disp(reversedArray); % Output: [5, 4, 3, 2, 1]
In the example above, `flip(originalArray)` effectively rearranges the elements of the array from last to first. This method is efficient, easy to implement, and works seamlessly for vectors and matrices alike.
Using Indexing to Reverse an Array
MATLAB's powerful indexing capabilities allow you to reverse an array without relying on any functions. By utilizing the `end` keyword in conjunction with a specified range, you can directly access elements in reverse order.
Code Snippet Example:
originalArray = [1, 2, 3, 4, 5];
reversedArray = originalArray(end:-1:1);
disp(reversedArray); % Output: [5, 4, 3, 2, 1]
In this snippet, `originalArray(end:-1:1)` starts from the last element and works backward to the first. This indexing approach is not only straightforward but also provides a performance advantage in some cases, especially with larger datasets.
Reversing Multidimensional Arrays
Reversing arrays can become more complex in a multidimensional context, such as with matrices. However, MATLAB provides specific ways to reverse both rows and columns.
Reversing Rows
To reverse the rows of a 2D array, you can use the `flip` function with an additional argument specifying the dimension.
Example:
matrix = [1, 2, 3; 4, 5, 6; 7, 8, 9];
reversedRows = flip(matrix, 1);
disp(reversedRows);
In this example, `flip(matrix, 1)` reverses the order of rows in the 2D matrix, effectively flipping it vertically.
Reversing Columns
Conversely, if you want to reverse the columns, you specify the second dimension in the `flip` function.
Example:
reversedColumns = flip(matrix, 2);
disp(reversedColumns);
Here, `flip(matrix, 2)` flips the matrix horizontally, reversing the order of elements in each row.
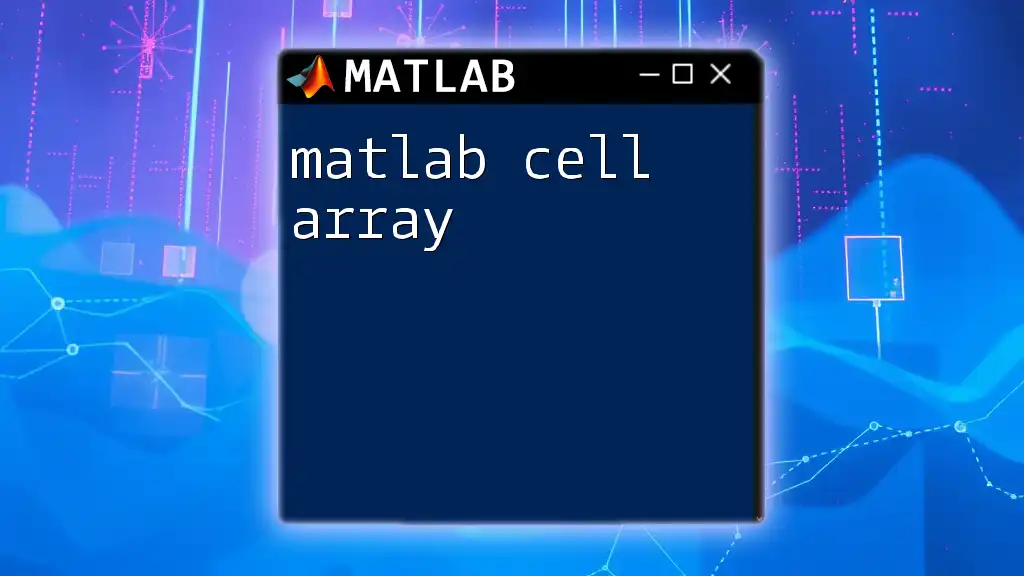
Special Cases in Reversing Arrays
Reversing a String Array
MATLAB also supports string arrays, and these can be reversed using the same methods outlined above. Understanding how to manipulate string arrays broadens your skills in data processing.
Code Snippet:
stringArray = ["apple", "banana", "cherry"];
reversedStrings = flip(stringArray);
disp(reversedStrings); % Output: ["cherry", "banana", "apple"]
In this case, the `flip` function effectively rearranges the string elements, demonstrating its versatility across different data types.
Reversing a Cell Array
Cell arrays contain elements of varying data types and require a unique approach for manipulation. To reverse a cell array, `flipud` (flip up-down) can be particularly useful.
Example Code:
cellArray = {1, 'apple', 3; 4, 5, 'banana'};
reversedCells = flipud(cellArray);
disp(reversedCells);
Using `flipud` ensures that each cell's content is preserved while the arrangement changes. This method highlights the adaptability of MATLAB when dealing with complex data types.
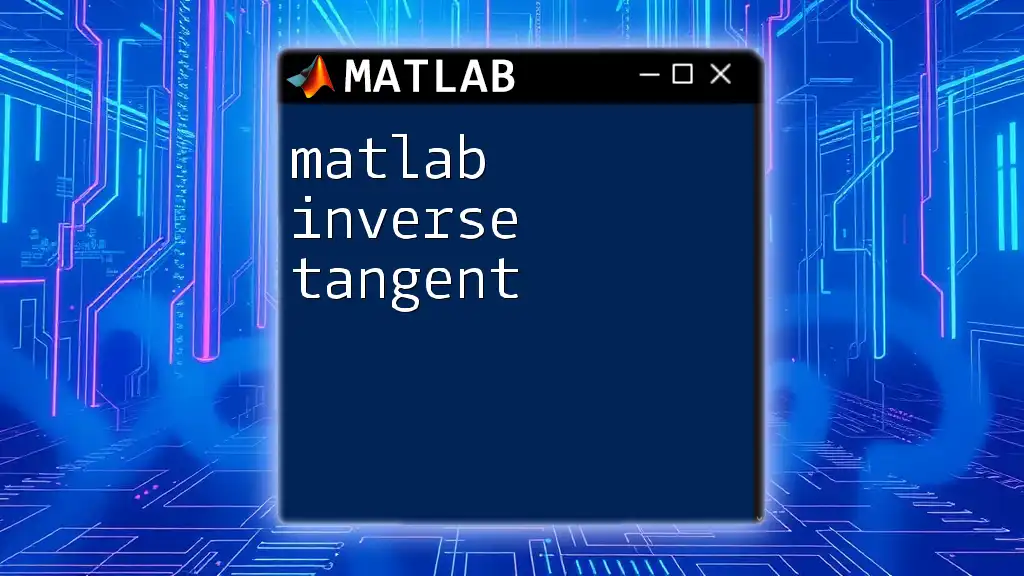
Performance Considerations
Efficiency of Reversing Methods
When it comes to performance, the choice of method for reversing an array can make a significant difference, especially with large datasets. The `flip` function is optimized for efficiency, but simple indexing can also be beneficial in many cases. In contexts where performance is critical, it's advisable to profile your code and select the method that provides the best balance between readability and speed.
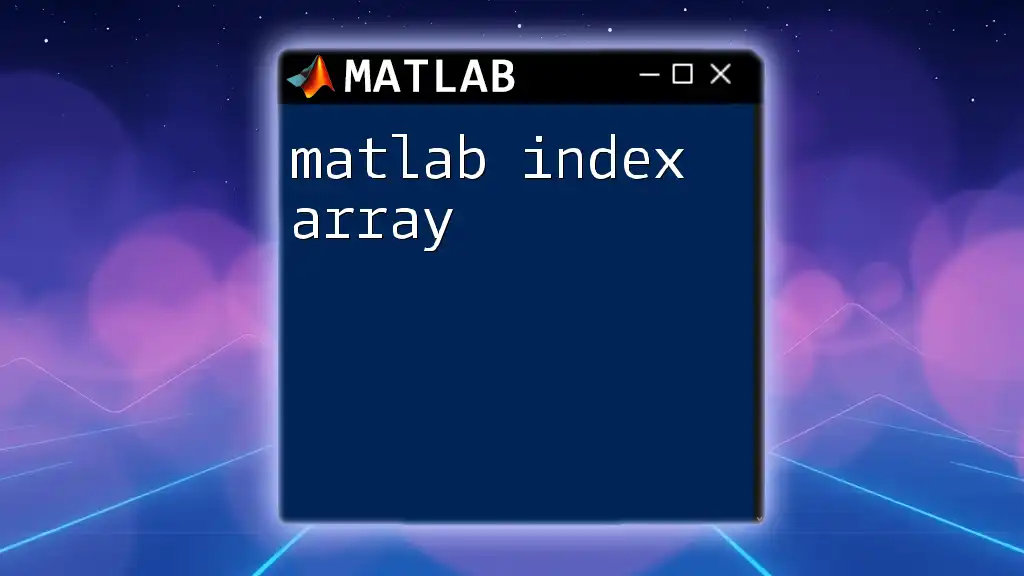
Practical Applications of Reversing Arrays in MATLAB
Case Study: Data Analysis
Consider a scenario in data analysis where you have a time-series dataset. Reversing the dataset can provide insights into patterns that may otherwise go unnoticed. For instance, analyzing customer purchase behaviors backward can help identify trends and predict future behaviors effectively.
Using Reversed Arrays in Algorithms
Reversing arrays can also play a crucial role in algorithm efficiency. For example, many sorting algorithms perform better when data is processed from the end to the start, as it can optimize comparisons and swaps.
function sortedArray = reverseSort(data)
reversedData = flip(data);
sortedArray = sort(reversedData);
end
originalData = [5, 3, 8, 1, 4];
result = reverseSort(originalData);
disp(result);
In this example, the data is first reversed, then sorted. This approach illustrates how reversing can enhance algorithm efficiency and outcomes.
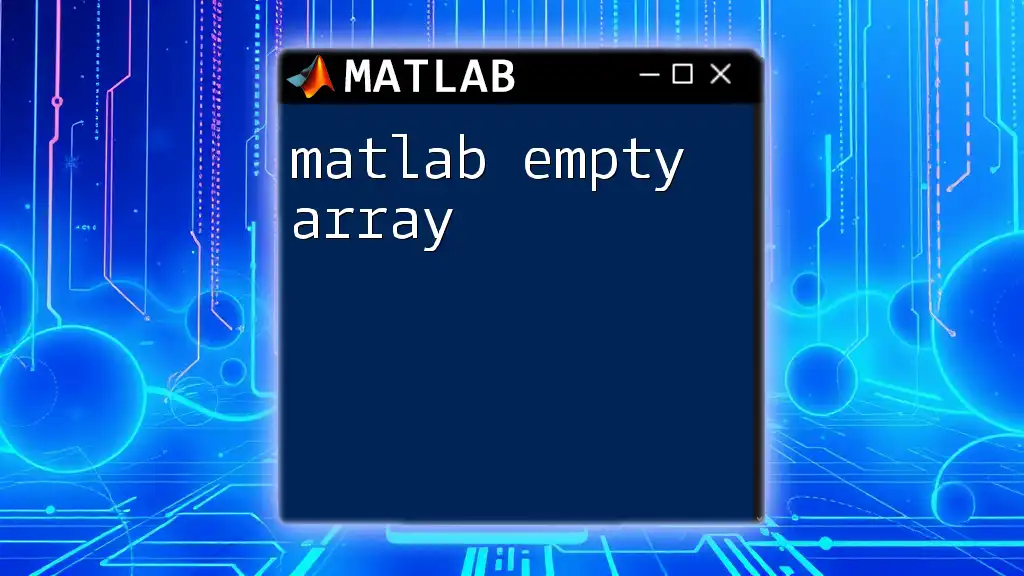
Conclusion
Reversing arrays is an essential skill in MATLAB programming, with applications across various domains including data analysis and algorithm implementation. Mastering techniques such as using the `flip` function and efficient indexing not only improves your coding efficiency but also expands your problem-solving toolkit in MATLAB.
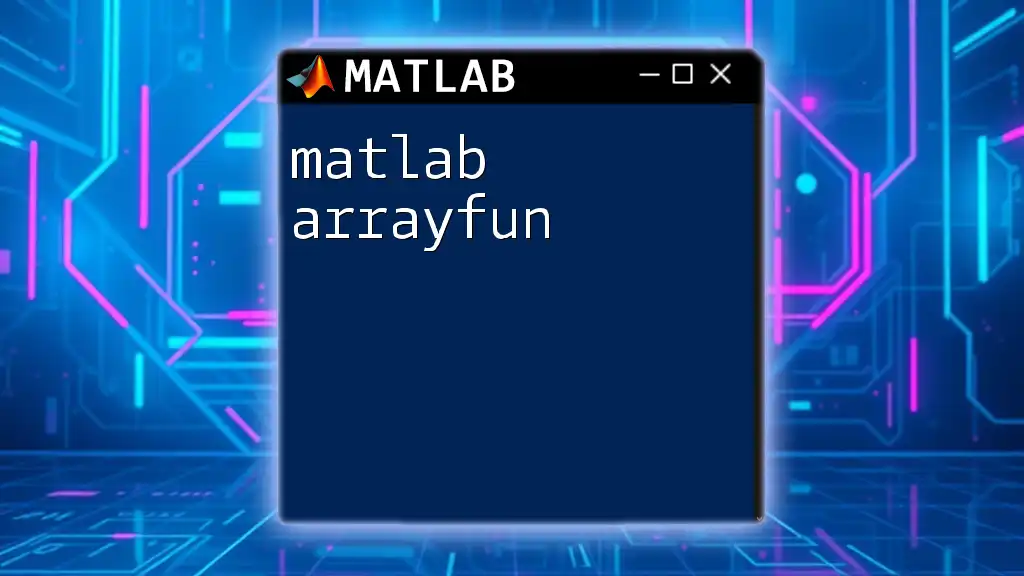
Additional Resources
To further enhance your understanding, consider exploring the official MATLAB documentation on array manipulation and seeking additional tutorials or resources that offer practical exercises. Engaging with community forums can also provide insights from other users, enriching your learning experience.
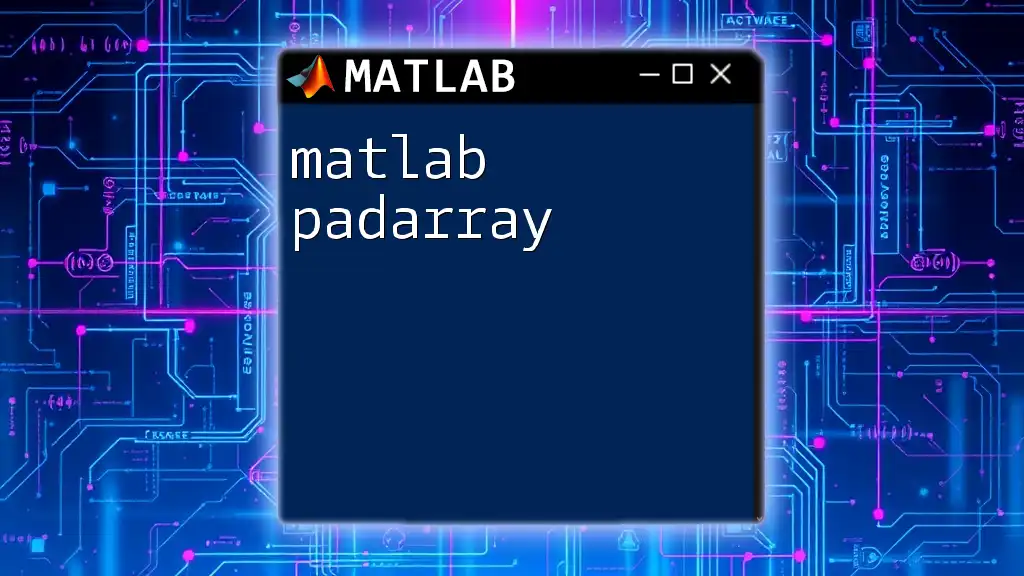
FAQs about Reversing Arrays in MATLAB
As you delve into the topic of reversing arrays, you may encounter questions that can help clarify your understanding. Common inquiries often relate to the best approaches for different array types, performance considerations, and specific coding scenarios. Exploring these FAQs can deepen your comprehension and provide practical solutions tailored to your programming needs.