A MATLAB 3D array is a multidimensional array that allows you to store data in three dimensions, which can be easily manipulated for various applications such as image processing or numerical simulations.
Here’s a simple example of creating and accessing a 3D array in MATLAB:
% Creating a 3D array of size 3x3x3
A = zeros(3, 3, 3);
% Assigning values to the first slice
A(:, :, 1) = [1, 2, 3; 4, 5, 6; 7, 8, 9];
% Assigning values to the second slice
A(:, :, 2) = [9, 8, 7; 6, 5, 4; 3, 2, 1];
% Accessing an element in the 3D array
element = A(2, 2, 1); % Accessing the element at row 2, column 2, and slice 1
Understanding the Basics of MATLAB Arrays
The Concept of Arrays in MATLAB
In MATLAB, arrays are fundamental data types used to store collections of values. An array can be classified into various types based on its dimensions, such as one-dimensional (1D), two-dimensional (2D), and three-dimensional (3D). Understanding these differences is essential when working with MATLAB 3D arrays, as it allows for more complex data arrangements.
How Arrays Work
Arrays in MATLAB are stored in contiguous blocks of memory. Each element of an array can be of different data types, including numeric, character, or logical types. MATLAB uses a column-major order to store multi-dimensional arrays, which means that it fills the columns of the array before filling the rows. This affects how you will access and manipulate array elements.
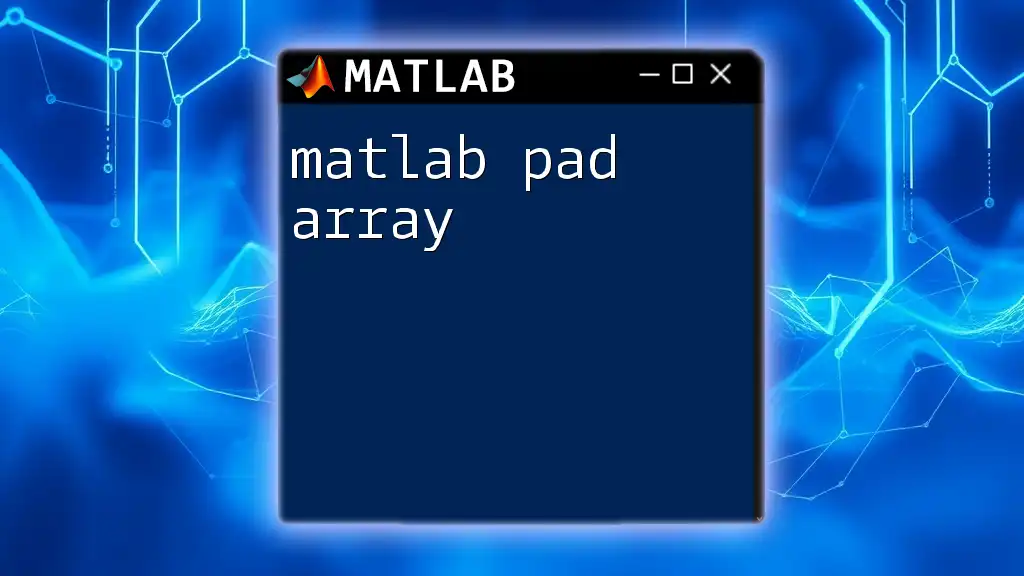
Creating 3D Arrays in MATLAB
Syntax for Creating 3D Arrays
Creating a MATLAB 3D array is simple and straightforward. The basic syntax follows the structure:
A = zeros(m, n, p); % Creates a 3D array filled with zeros
In this case, `m`, `n`, and `p` represent the dimensions of the array. For example, if you want an array of size 3x4x5 filled with zeros, you would write:
A = zeros(3, 4, 5); % Creates a 3D array with dimensions 3x4x5
Different Methods to Create 3D Arrays
Using `reshape` Function
The `reshape` function is a powerful tool that allows you to transform an existing array into a 3D format. For instance, if you start with a one-dimensional array, you can reshape it as follows:
B = rand(1, 60); % Create a 1D array filled with random numbers
C = reshape(B, [3, 4, 5]); % Reshape to a 3D array with dimensions 3x4x5
This example showcases how easily a linear array can be reorganized into a multi-dimensional array, which is particularly useful in data simulation and modeling tasks.
Using Direct Initialization
Another approach to create a 3D array is by directly initializing it with specified values. This method can be efficient for small or predefined datasets. Here's how you can do that:
D = cat(3, [1, 2; 3, 4], [5, 6; 7, 8], [9, 10; 11, 12]); % Concatenation example
In this example, the `cat` function combines three 2D matrices along the third dimension to form a single 3D array.
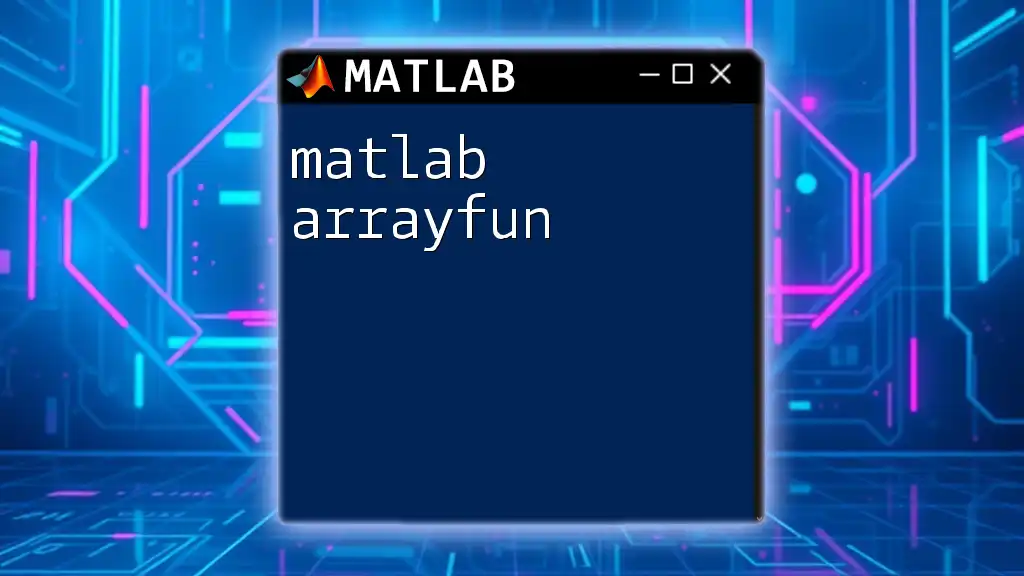
Accessing Elements in a 3D Array
Indexing Syntax
Accessing elements within a MATLAB 3D array requires specifying the row, column, and depth. The syntax looks like this:
E = C(2, 3, 1); % Accesses element at indices (2, 3, 1)
This command retrieves the value located at the second row, third column, and the first slice of the 3D array.
Techniques for Slicing and Dicing
Additional powerful features of MATLAB allow for extracting subarrays known as slicing. For instance, to extract all rows and columns of the second matrix in a 3D array:
F = C(:, :, 2); % Extracts all rows and columns from the second matrix
This capability makes it easy to analyze specific portions of data without needing to manipulate the entire array.
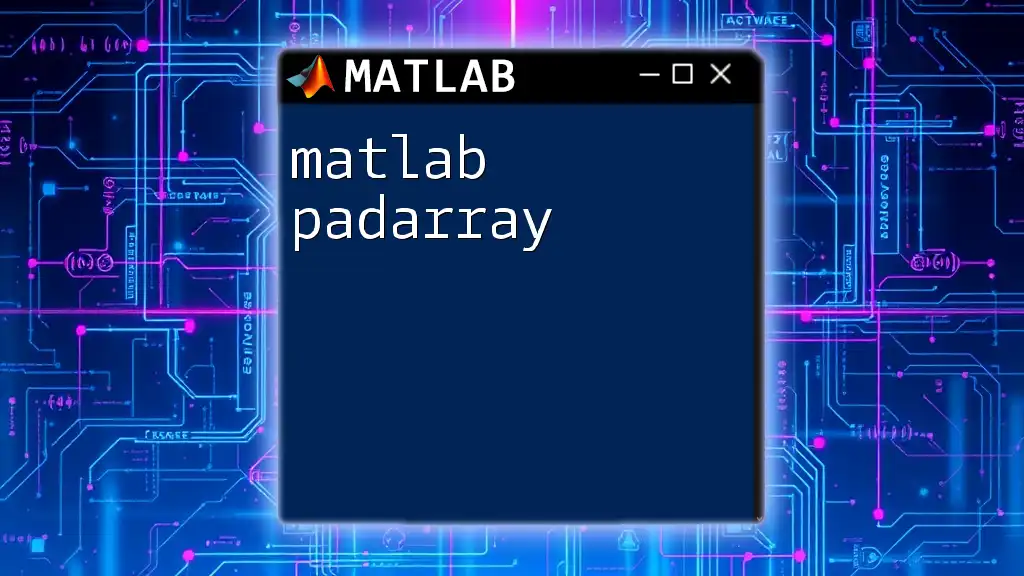
Manipulating 3D Arrays
Basic Operations on 3D Arrays
MATLAB allows for basic arithmetic operations on 3D arrays, much like 2D arrays. For instance, you can easily add a scalar to each element of the array.
G = C + 1; % Adds 1 to each element in the 3D array
This kind of operation is beneficial when normalizing or scaling data sets.
Advanced Functions
Using `sum`, `mean`, and `max`
MATLAB provides built-in functions such as `sum`, `mean`, and `max`, which can perform calculations across specified dimensions of a 3D array. For example, to sum elements across the third dimension, you can use:
H = sum(C, 3); % Sums along the third dimension
This command provides a new array that contains the sum of values slice-by-slice across the specified dimension.
Logical Operations
Working with logical conditions is also possible with 3D arrays. For example, if you want to create a logical array of true/false values based on a condition, you can do:
I = C > 5; % Creates a logical array indicating elements greater than 5
This allows users to filter and analyze their data based on specified criteria.
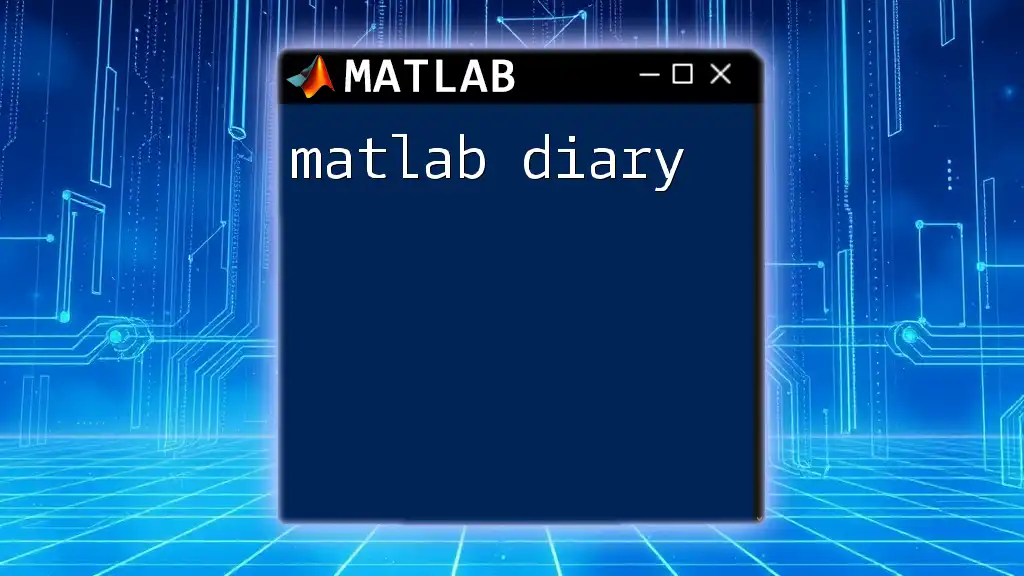
Visualizing 3D Arrays
Plotting Techniques
Visualization is crucial when working with complex data stored in MATLAB 3D arrays. MATLAB provides numerous built-in functions to help visualize such data. For instance, to create a surface plot of a particular slice of the 3D array:
surf(C(:, :, 1)); % Plots the first slice of the 3D array
This can provide insights into the data structure and help identify patterns or anomalies.
Advanced Visualization
Using `isosurface` and `slice`
For more advanced visualization, functions like `isosurface` and `slice` play an integral role in presenting 3D data effectively. To generate an isosurface at a specific threshold, you can use:
isosurface(C, 5); % Generates a 3D isosurface at value 5
This offers a 3D representation of data where certain conditions are met, allowing for intricate visual analyses.
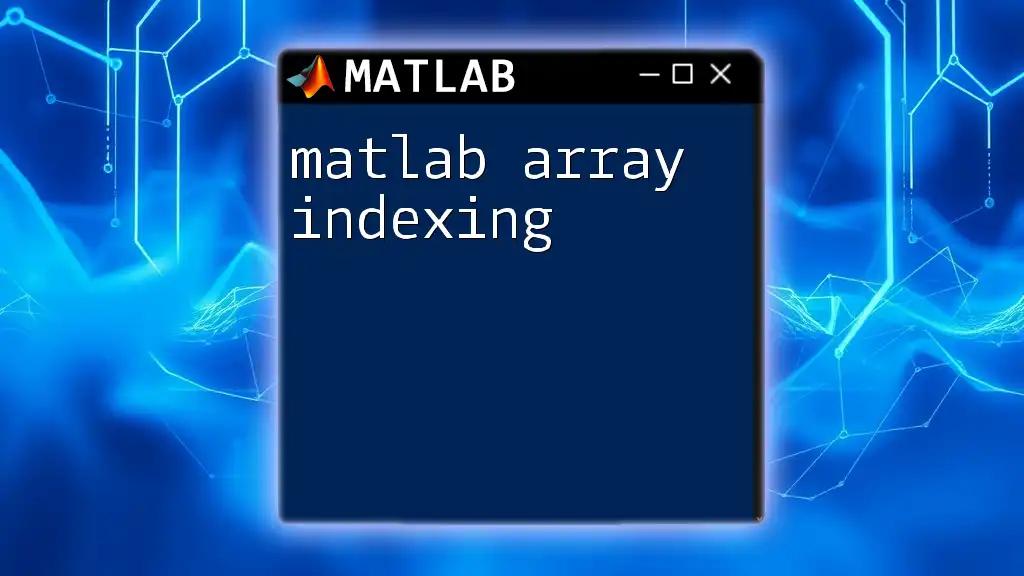
Applications of 3D Arrays
Fields that Utilize 3D Arrays
The significance of MATLAB 3D arrays extends across multiple fields. Industries such as engineering, medical imaging, and computer graphics routinely apply 3D arrays for organizing and visualizing complex datasets. These applications enhance simulation capabilities and decision-making processes.
Real-World Examples
Real-world applications of MATLAB 3D arrays include modeling weather patterns where data can change across various heights and times, or in medical imaging, where volumetric data is processed for analysis and visualization.
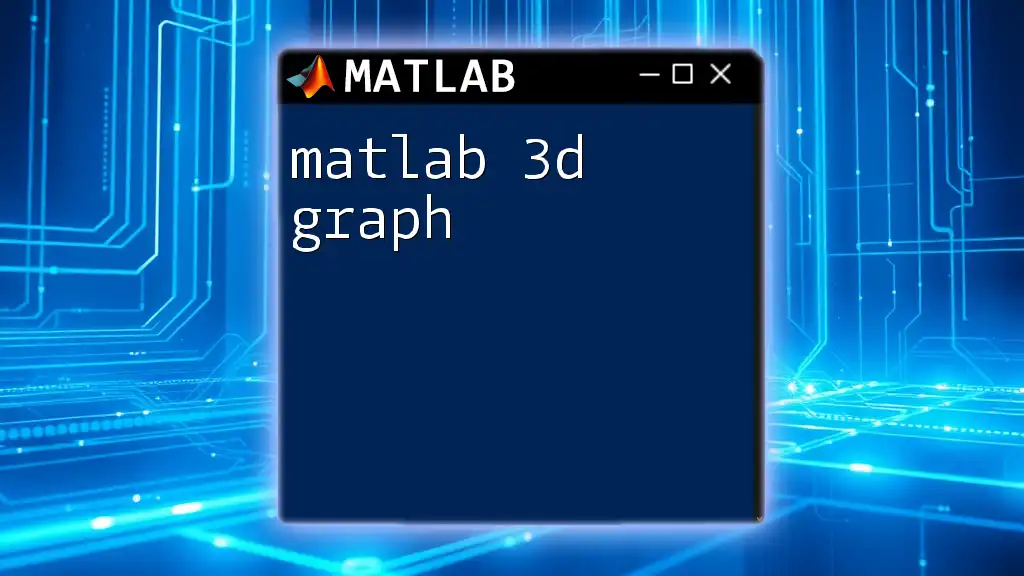
Troubleshooting Common Issues
Common Errors and Solutions
When working with MATLAB 3D arrays, you may encounter common programming errors, such as dimension mismatch during operations or incorrect indexing. Pay attention to error messages, which often provide insight into where the issue lies. Debugging in MATLAB can be simplified by checking the sizes of your arrays using `size()` and ensuring your operations are dimensionally compatible.
Best Practices
To maximize efficiency and minimize errors when working with 3D arrays, adopt the following best practices:
- Initialize arrays properly to avoid unexpected results.
- Use functions like `reshape` and `cat` effectively to manage data flow.
- Comment your code extensively for clarity, particularly in complex structures.
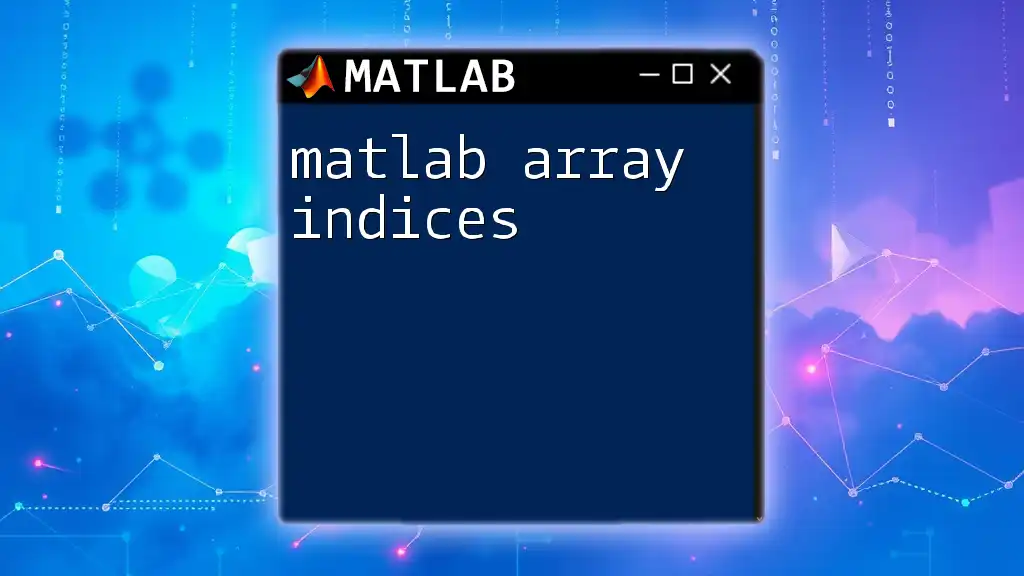
Conclusion
In summary, MATLAB 3D arrays are a vital tool for data manipulation and visualization, providing significant versatility in how data is structured and accessed. From creating and accessing the data to complex visualizations, mastering 3D arrays enhances your ability to work with multi-dimensional data efficiently. By exploring the various methods and practices discussed, users can unlock new possibilities in their MATLAB applications.