In MATLAB, you can prompt the user for input using the `input` function, allowing for interactive data entry during program execution.
Here’s a simple example:
userInput = input('Please enter a number: ');
Understanding the `input` Command
What is the `input` Command?
In MATLAB, the `input` command is essential for creating interactive scripts and functions. It allows the programmer to prompt users for information during the execution of a script, thus enhancing user engagement and offering flexibility in how the program runs. The capability to ask for user input is critical for many applications, from simple calculations to complex data manipulations.
Syntax of the `input` Command
The basic structure of the `input` command is straightforward. It requires a prompt string that defines what the user should enter. For example:
userInput = input('Enter a value: ');
In this example, userInput stores the value entered by the user after MATLAB displays the prompt `'Enter a value: '`. Understanding the syntax is crucial as it lays the foundation for effectively gathering data.
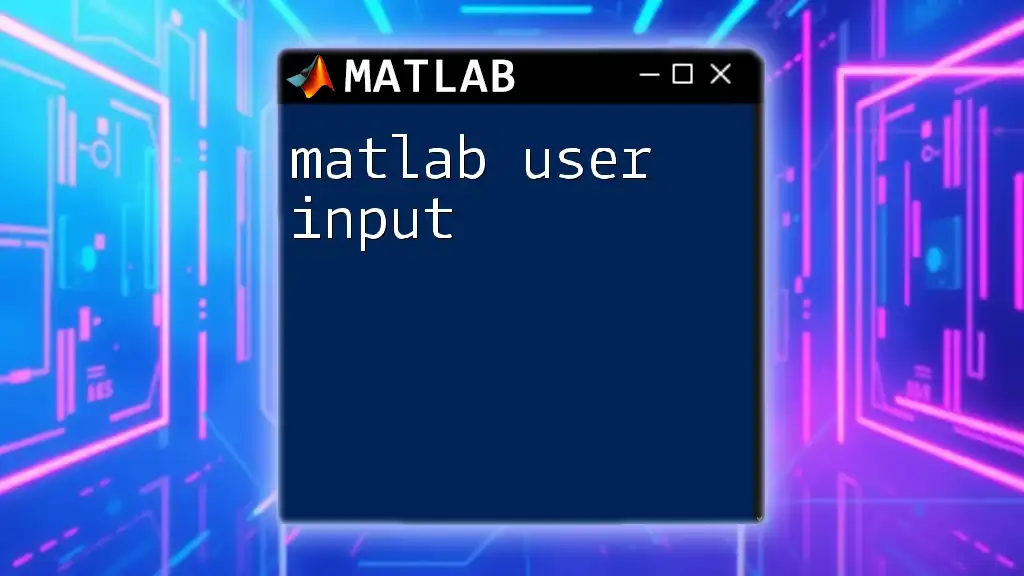
Types of Input: Numeric and String
Obtaining Numeric Input
To obtain numeric input, you can directly use the `input` command without any additional options. For instance:
age = input('Enter your age: ');
In this example, the variable age will capture the numeric input entered by the user. MATLAB interprets this input as a numeric variable. However, it is essential to note that if the user types in a non-numeric value, MATLAB will throw an error.
Thus, to ensure only valid numeric input is processed, consider implementing input validation in your scripts.
Handling String Input
When the intention is to capture string input, the `input` command offers a useful option: the 's' character. This ensures that any data entered by the user is treated as a string. For example:
name = input('Enter your name: ', 's');
Here, the variable name will store whatever string the user inputs, providing greater flexibility for textual data. Utilizing string inputs is common in applications such as name capture for greeting functions or collecting user responses.
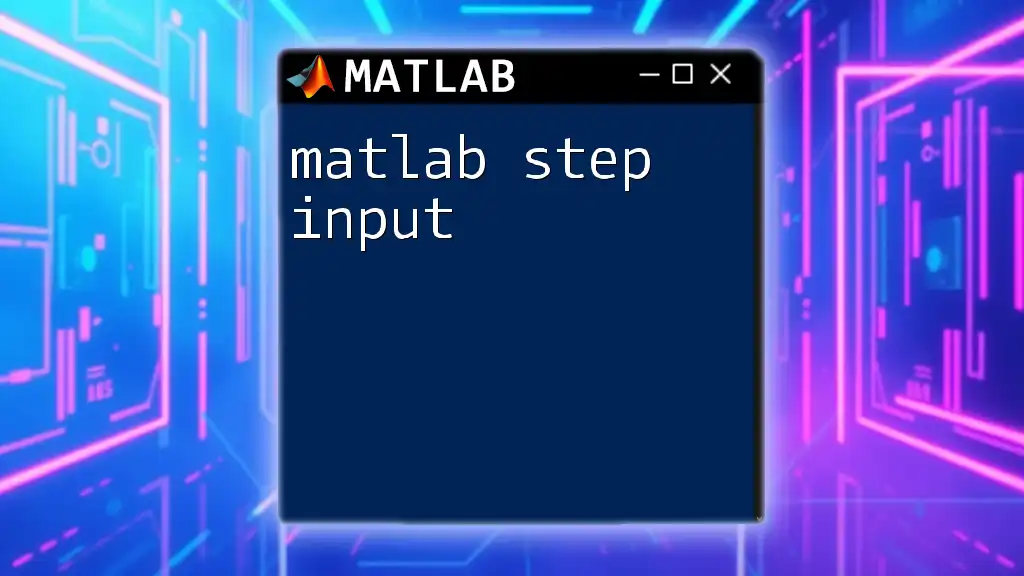
Using Input in Functions
Creating Functions that Accept Input
MATLAB allows you to craft custom functions that accept input from users. This ability elevates the interactivity and utility of your functions. For instance:
function greeting = sayHello()
name = input('What is your name? ', 's');
greeting = ['Hello, ' name '!'];
end
In this straightforward function, sayHello, the program prompts the user for their name and constructs a personalized greeting. Understanding how to implement user input in functions is vital when developing applications that require user-specific data.
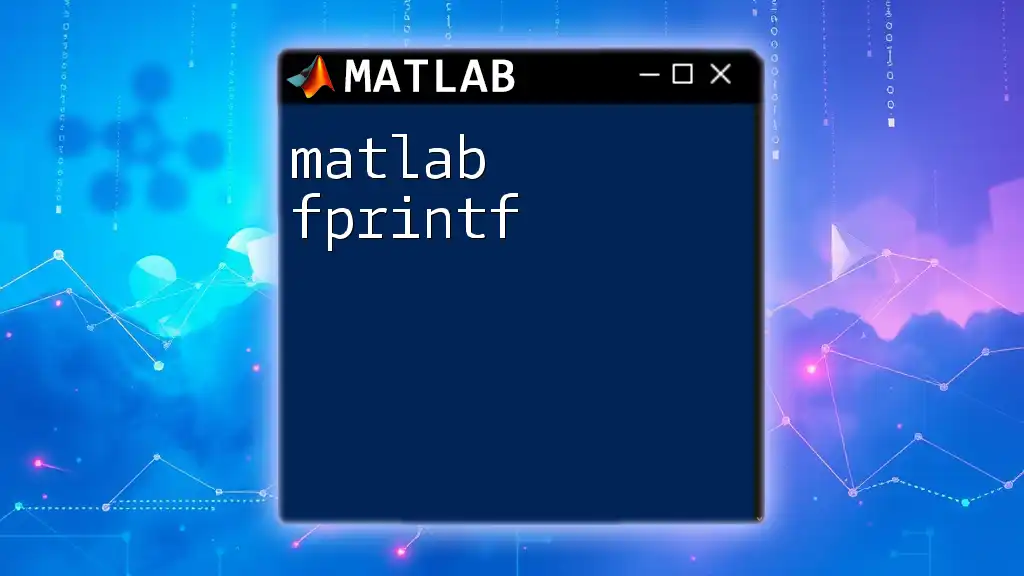
Input Validation Techniques
Basic Validation Techniques
Validating user input is a critical step to ensure your program runs smoothly without encountering errors due to unexpected data types. For example, if a user is prompted to enter their age, you should validate that the input is a positive number:
while true
age = input('Enter your age: ');
if isnumeric(age) && age > 0
break;
else
disp('Please enter a valid age.');
end
end
In this code snippet, the program continues to prompt the user until a valid numeric value greater than zero is received. This kind of input validation enhances the robustness of your scripts.
Advanced Input Validation with `try-catch`
When designing more complex applications, it’s prudent to implement advanced error handling using the `try-catch` construct in MATLAB. This allows for cleaner handling of errors while validating user input:
while true
try
age = input('Enter your age: ');
assert(isnumeric(age) && age > 0, 'Age must be a positive number.');
break;
catch e
disp(e.message);
end
end
In this case, if the input does not meet the conditions specified in the assertion, the program will catch the error and display a meaningful message, prompting the user to try again. This makes your applications more user-friendly and decreases frustration.
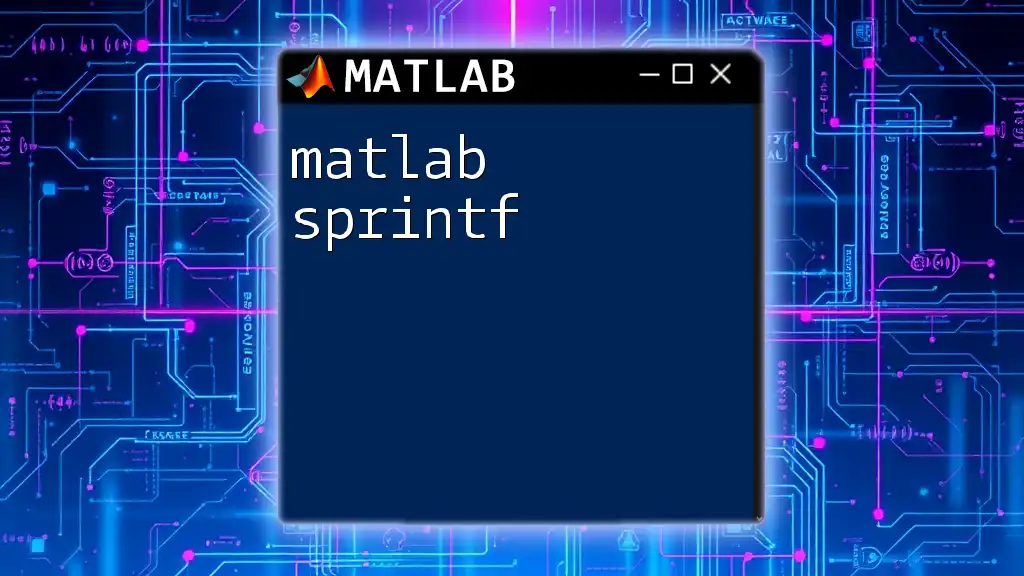
Best Practices for Using Input
Keeping User Prompts Clear and Concise
It’s crucial to craft clear and concise prompts for users. Vague instructions can lead to confusion and incorrect data entry. For instance, when asking a yes/no question, structure the prompt like this:
response = input('Do you want to continue? (yes/no): ', 's');
This formulation clearly states what the user should enter, thus improving user experience.
Testing and Debugging User Inputs
Finally, it’s vital to test and debug user inputs continuously. As you write scripts that prompt users, utilize MATLAB’s debugging tools to identify and rectify any issues with user entries. Testing input conditions helps you discover edge cases that might lead to errors or unexpected behavior in your scripts.
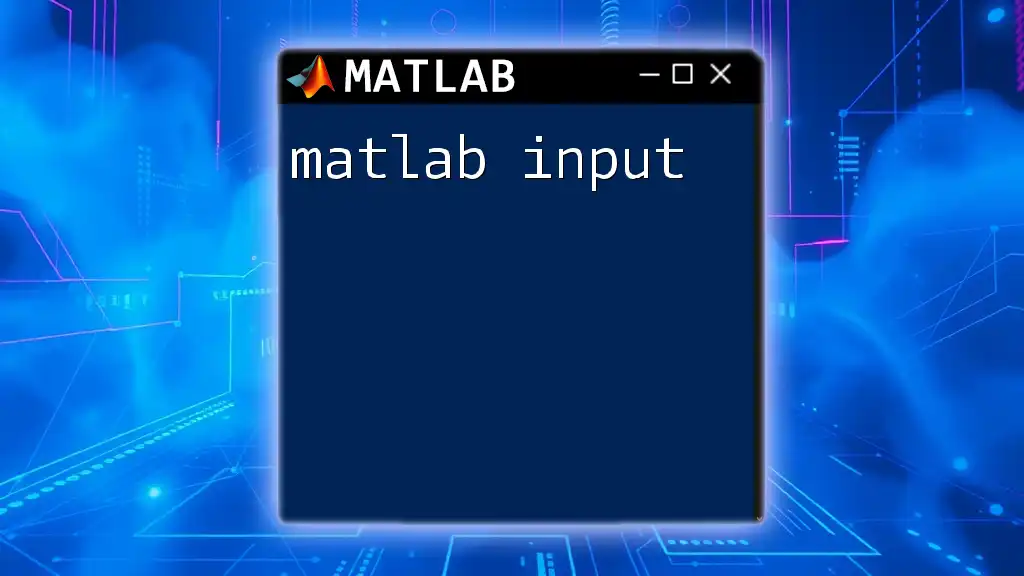
Conclusion
In summary, mastering how to ask for input in MATLAB is fundamental for creating interactive and user-centered applications. By utilizing the `input` command effectively, validating data rigorously, and implementing best practices, you can enhance the usability and functionality of your MATLAB scripts. Embrace these techniques, and your programming projects will be significantly enriched by the ability to engage with users through dynamic input.