`varargin` is a special input variable in MATLAB that allows a function to accept an arbitrary number of input arguments.
Here’s a simple example demonstrating its use:
function myFunction(varargin)
for i = 1:length(varargin)
disp(['Input argument ' num2str(i) ': ' num2str(varargin{i})]);
end
end
Understanding `varargin`
What is `varargin`?
`varargin` is a powerful feature in MATLAB that allows you to define functions with a variable number of input arguments. This means that you can create functions that can accept any number of inputs, providing flexibility and versatility in your coding.
Why Use `varargin`?
Using `varargin` can significantly improve the usability of your functions. With `varargin`, you can:
- Handle variable numbers of inputs, allowing users to pass in different data without changing the function signature.
- Enhance code readability, making your code more user-friendly as it allows users to provide only the necessary parameters.
- Create adaptable functions that can cater to different requirements, leading to reusable code.
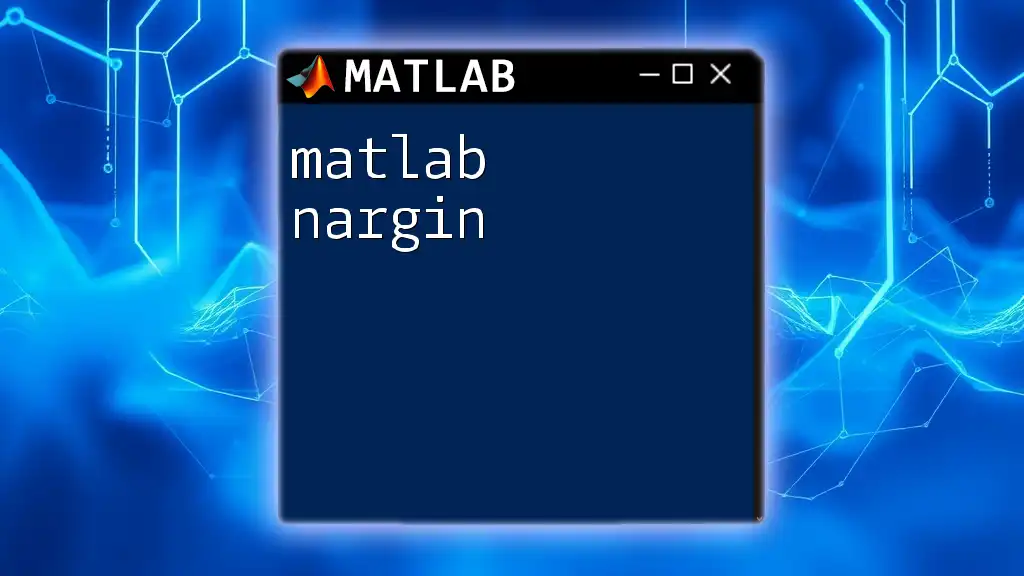
Creating Functions with `varargin`
Defining Functions with `varargin`
To define a function with `varargin`, you simply include it as the last input argument in the function definition. This enables the function to accept any number of additional input parameters.
For example, consider the following simple function that makes use of `varargin`:
function myFunction(varargin)
disp('Number of input arguments:');
disp(nargin);
disp('Input arguments:');
disp(varargin);
end
In this code, `myFunction` can accept any number of input arguments, and it will display how many arguments were passed, along with the actual arguments.
Accessing Input Arguments
Once you’ve defined a function using `varargin`, you can access each input through standard indexing. Here's a code snippet that demonstrates how to retrieve specific input arguments:
function myFunction(varargin)
a = varargin{1}; % Access first argument
b = varargin{2}; % Access second argument
fprintf('You provided: %d and %d\n', a, b);
end
In this example, the function retrieves the first two input arguments and displays them. If fewer than two arguments are provided, this will lead to an error, which illustrates the need for careful input management in your code.
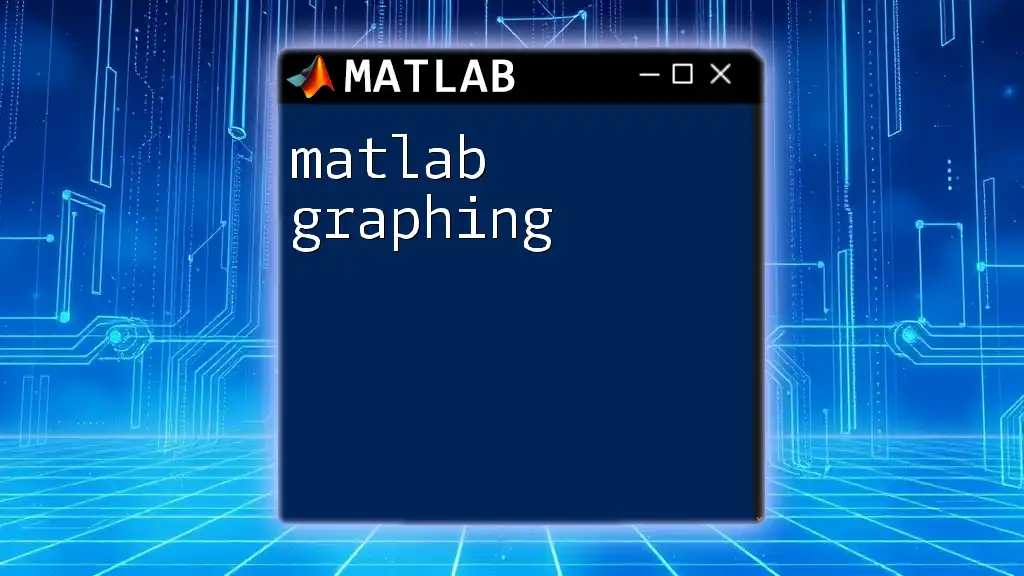
Practical Applications of `varargin`
Creating Functions with Optional Parameters
One of the most significant advantages of using `varargin` is the ability to create functions that accept optional parameters. The `inputParser` class allows for elegant handling of optional inputs and default values. An example of this is shown below:
function myFunction(a, varargin)
p = inputParser;
addOptional(p, 'b', 10); % Default value for b is 10
parse(p, varargin{:});
b = p.Results.b;
fprintf('Values are: a = %d, b = %d\n', a, b);
end
In this function, the user can provide a value for `b`, or if it is not provided, it defaults to `10`. This is especially useful for functions where certain parameters are not always necessary.
Dynamic Function Behavior
`varargin` enables you to design functions that can adapt their behavior based on the number or type of inputs they receive. For instance, consider a summation function that handles a dynamic number of input values:
function totalSum = dynamicSum(varargin)
totalSum = sum(cell2mat(varargin));
end
This function takes in a variable number of inputs and computes their total sum, demonstrating how `varargin` can make your functions elegantly dynamic.
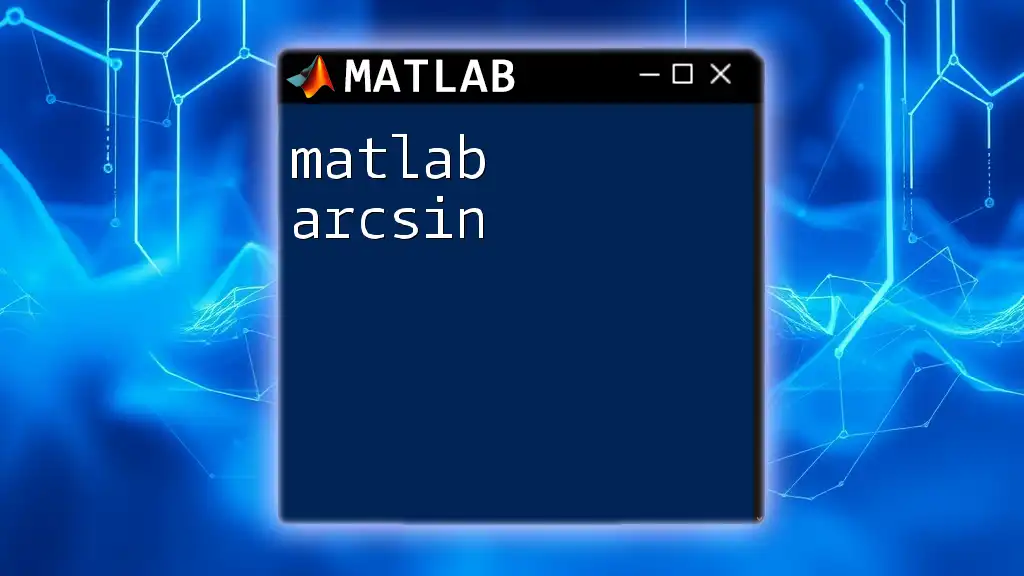
Advanced Features of `varargin`
Combining `varargin` with Other Input Structures
MATLAB allows you to combine `varargin` with `varargout` to offer flexible output options as well. This combination is ideal for functions that need to return multiple outputs flexibly. Here is an example:
function [sumVal, prodVal] = calcStats(varargin)
sumVal = sum(cell2mat(varargin));
prodVal = prod(cell2mat(varargin));
end
In this function, both the sum and product of all input values are computed and returned, showcasing how `varargin` can enhance the capability of your functions.
Error Handling in Functions with `varargin`
Proper error handling within `varargin` functions is crucial. You can use assertions and input validation to manage arguments effectively. For instance:
function myFunction(varargin)
assert(nargin > 0, 'At least one input required.');
% further processing...
end
This function will throw an error if no input arguments are passed, ensuring that the user receives feedback on how to use the function correctly.
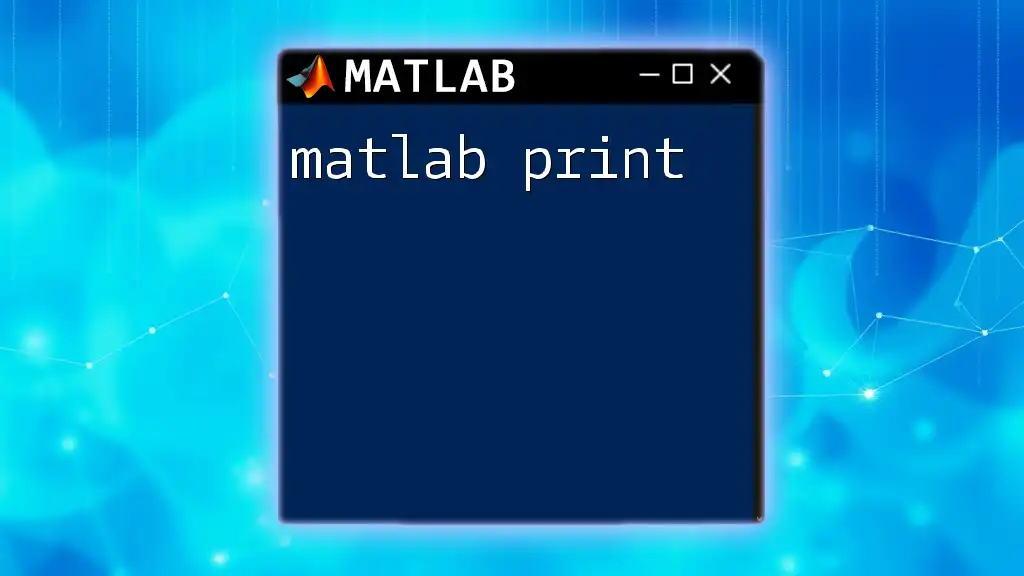
Common Pitfalls and Best Practices
Common Mistakes When Using `varargin`
While `varargin` is powerful, there are common mistakes to avoid:
- Not checking the number of inputs: Always ensure you've received the expected number of inputs before accessing `varargin`.
- Assuming input types: Since `varargin` can handle any type, it's easy to mismanage types if not handled carefully.
Best Practices for Using `varargin`
To make the best use of `varargin`, consider the following best practices:
- Document your function: Clearly specify what inputs are expected, especially if some are optional.
- Use input validation: Always validate inputs to ensure your function behaves predictably.
- Be clear in your output: If your function supports multiple outputs, utilize `varargout` to make usage intuitive.
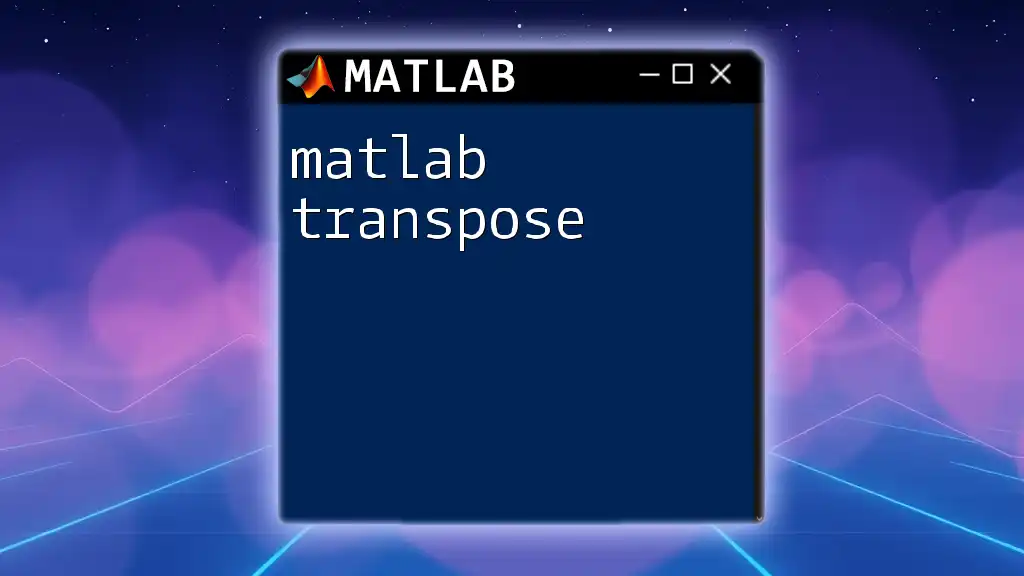
Conclusion
In summary, understanding and utilizing `matlab varargin` opens up a world of possibilities for creating adaptable, robust, and flexible functions within the MATLAB environment. By following the strategies outlined in this guide and implementing `varargin` effectively, you’ll be able to enhance the usability of your code significantly, leading to improved efficiency and user satisfaction.
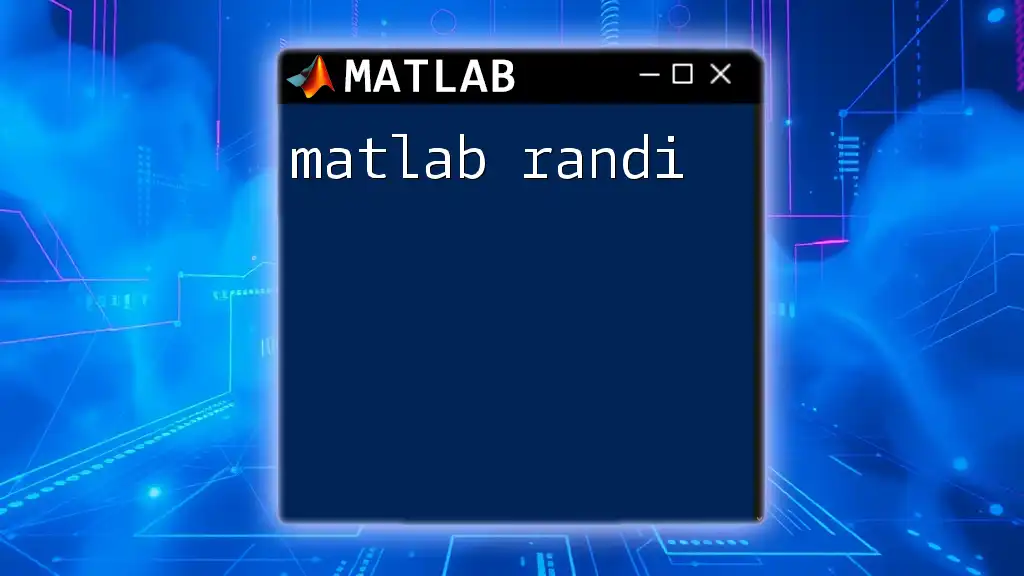
Additional Resources
Further Reading
For more detailed information, refer to the official MATLAB documentation on `varargin` and other relevant programming concepts.
Practice Exercises
To solidify your understanding, tackle some practice problems that require you to implement functions using `varargin`. Experiment with optional parameters and various input combinations to gain firsthand experience with this powerful feature.