The MATLAB `mean` function calculates the average of an array of values, returning the mean of the specified dimension by default, and can be used as follows:
data = [1, 2, 3, 4, 5];
average = mean(data);
Understanding the Mean
What is Mean?
The mean is a key statistical measure that indicates the central tendency of a set of values. It is commonly referred to as the average and is calculated by summing all the values in a dataset and then dividing by the number of values. Understanding the mean is critical in data analysis, as it provides a quick way to represent the typical value of a dataset.
Types of Mean
Arithmetic Mean
The arithmetic mean is the most widely-used form of mean. It is calculated using the formula:
\[ \text{Arithmetic Mean} = \frac{\sum_{i=1}^{n} x_i}{n} \]
where \(x_i\) represents each value in the dataset and \(n\) is the total number of values. The arithmetic mean is particularly useful when all data points contribute equally to the outcome.
Weighted Mean
In contrast to the arithmetic mean, the weighted mean accounts for the relative importance of each value. The formula is:
\[ \text{Weighted Mean} = \frac{\sum_{i=1}^{n} w_i x_i}{\sum_{i=1}^{n} w_i} \]
where \(w_i\) represents the weight associated with each \(x_i\). This mean is especially beneficial when certain values in the dataset should carry more significance, such as scores weighted by their credit hours in a course.
Real-World Applications of Mean
The mean is utilized across various fields:
- Finance: To compute the average return on investments.
- Engineering: In quality control processes to find the average output.
- Science: When analyzing measurements or experimental data.
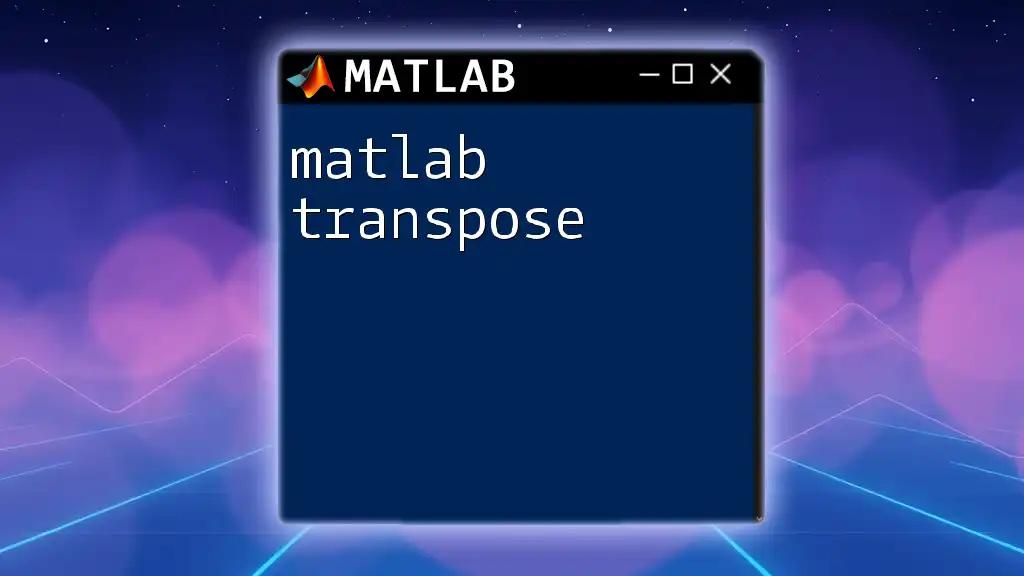
MATLAB Commands for Calculating Mean
The `mean()` Function
Syntax
In MATLAB, the primary function to compute the mean is `mean()`. The syntax is as follows:
mean(X, dim)
- X: Input array (vector or matrix).
- dim: Dimension along which the mean is calculated (1 for columns, 2 for rows).
Examples
Simple Example with a Vector
For instance, if we want to calculate the mean of a simple dataset:
data = [2, 4, 6, 8, 10];
average = mean(data);
disp(average); % Outputs 6
In this case, the output is 6, which represents the average of the numbers.
Example with a Matrix
When calculating the mean for multi-dimensional data, we can use the `mean()` function with a matrix:
matrix = [1, 2, 3; 4, 5, 6; 7, 8, 9];
row_avg = mean(matrix, 2); % Calculates mean across rows
column_avg = mean(matrix, 1); % Calculates mean across columns
disp(row_avg); % Outputs [2; 5; 8]
disp(column_avg); % Outputs [4; 5; 6]
Here, `row_avg` will return the means of each row, while `column_avg` gives the means for each column.
Additional Options and Output Types
Specifying Dimensions
Understanding how dimensions work in MATLAB is crucial when dealing with matrices. Specifying the dimension in the `mean()` function allows you to control whether you want to calculate the mean across rows or columns, which is essential for accurate data analysis.
Handling NaN Values
When working with real datasets, it's common to encounter NaN (Not a Number) values. MATLAB provides a way to handle these cases gracefully through the `omitnan` option. Using `nanmean()` (available in some MATLAB versions or toolboxes), you can calculate the mean while ignoring any NaN values:
data_with_nan = [1, 2, NaN, 4];
mean_no_nan = mean(data_with_nan, 'omitnan');
disp(mean_no_nan); % Outputs 2.3333
This ensures that your mean calculation remains accurate and reflective of your true data distribution.
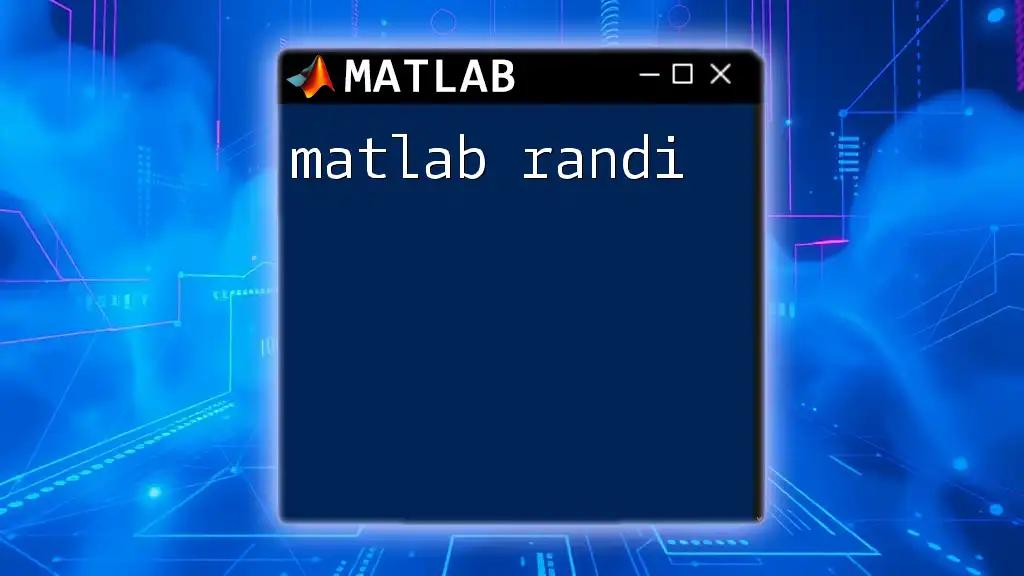
Visualizing Mean with MATLAB
Using Bar Graphs
Visualizing the mean can greatly enhance data interpretation. Given a dataset with categories, you can represent the mean through bar graphs, making the information clearer.
Here’s how to create a bar graph to show mean values:
categories = {'A', 'B', 'C'};
mean_values = [3, 5, 7];
bar(categories, mean_values);
xlabel('Categories');
ylabel('Mean Values');
title('Mean Values of Different Categories');
This visualization helps to quickly grasp how mean values differ across categories.
Boxplots for Insightful Representation
Boxplots serve as an excellent tool for statistical representation, showing not only the mean but also the spread and skewness of the data. Although the specifics of creating boxplots are beyond the `mean()` function, they often complement mean analysis by providing a fuller picture of the dataset.

Advanced Mean Analysis
Weighted Mean Calculation in MATLAB
To calculate a weighted mean in MATLAB, you can use basic arithmetic alongside the `sum()` function:
data = [1, 2, 3, 4];
weights = [0.1, 0.2, 0.3, 0.4];
weighted_mean = sum(data .* weights) / sum(weights);
disp(weighted_mean); % Outputs 3
This code snippet demonstrates how to find the weighted mean by multiplying values by their respective weights and dividing by the sum of those weights.
Using `mean` in Statistical Tables
When working with statistical tables, you can employ the `mean()` function to calculate means for various categories and structures, thereby enriching your data analysis.
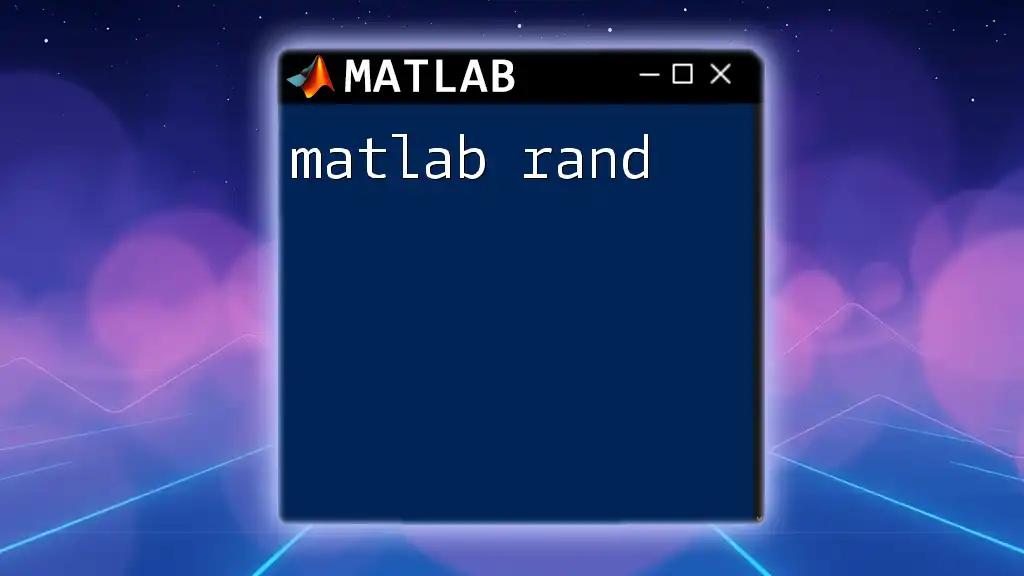
Common Errors and Debugging Tips
Inconsistent Dimensions
One frequent error when using the `mean()` function arises from inconsistencies in matrix dimensions. Always ensure that when calculating the mean across a specific dimension, that the data is structured correctly. If MATLAB returns an error about dimensions, check your dataset alignment.
Handling Large Datasets
For large datasets, performance can be a concern. It is wise to use data reduction techniques or to compute statistics incrementally. Utilizing MATLAB's efficient programming practices will help optimize the mean calculation process.
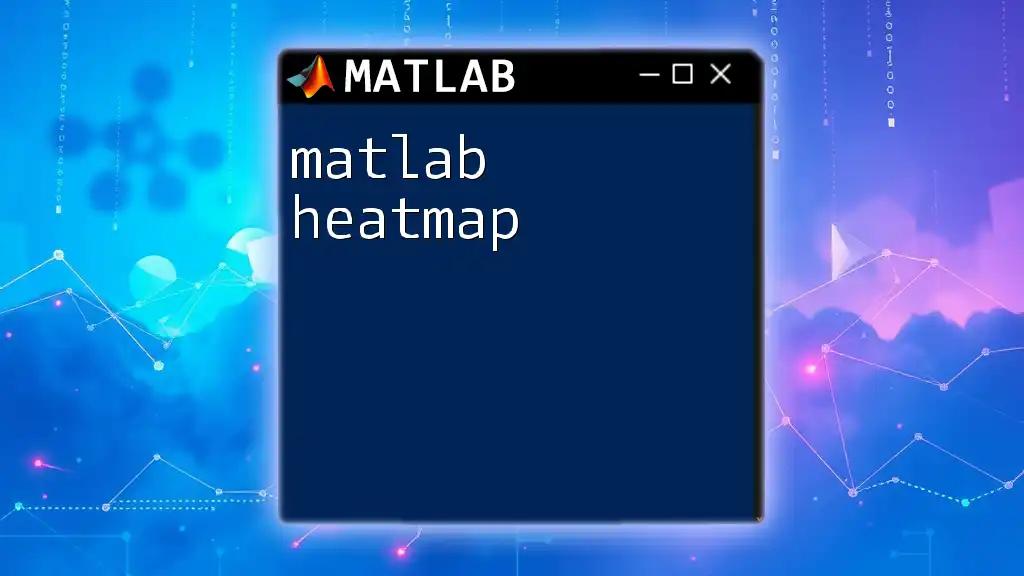
Conclusion
Understanding the `matlab mean` function is invaluable for anyone involved in data analysis and statistics using MATLAB. The mean serves not only as a fundamental statistic but also as a gateway to deeper data insights. By mastering the various commands and visualizations, you can elevate your MATLAB skills and enhance your analytical capabilities. Engaging with different datasets will further refine your understanding and application of these concepts.
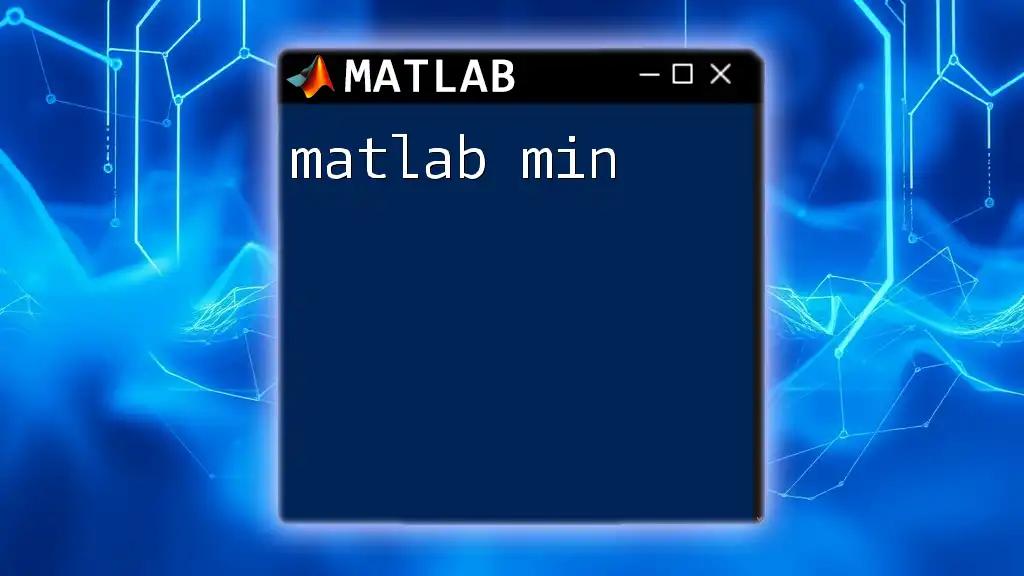
Additional Resources
References
To continue your learning journey, explore more about MATLAB statistics in official documentation and online courses related to statistical analysis and data visualization.
Tutorials and Further Learning
Expand your knowledge by diving into other statistical functions in MATLAB such as median, mode, and standard deviation to complement your understanding of mean calculations.