In MATLAB, a case statement (also known as a switch statement) allows you to execute different blocks of code based on the value of a variable, streamlining complex conditional checks.
Here’s a simple example:
x = 2;
switch x
case 1
disp('x is one');
case 2
disp('x is two');
case 3
disp('x is three');
otherwise
disp('x is something else');
end
Understanding the `case` Statement
Definition of the `case` Statement
A MATLAB case statement is a powerful control flow tool that allows you to execute different blocks of code based on the value of a specified variable. It is part of the `switch` structure, which efficiently evaluates a variable and matches it against several possible cases. Unlike traditional `if-else` constructs that require separate evaluations for each condition, the `case` statement provides a more streamlined approach, particularly when dealing with multiple conditions.
When to Use the `case` Statement
The case statement is particularly useful in scenarios that involve discrete values or enumerated options. When you have multiple specific values that need to be evaluated, using a `case` statement can improve both readability and performance. For example, if you are switching among several status codes or days of the week, a `case` statement enhances code clarity and efficiency.
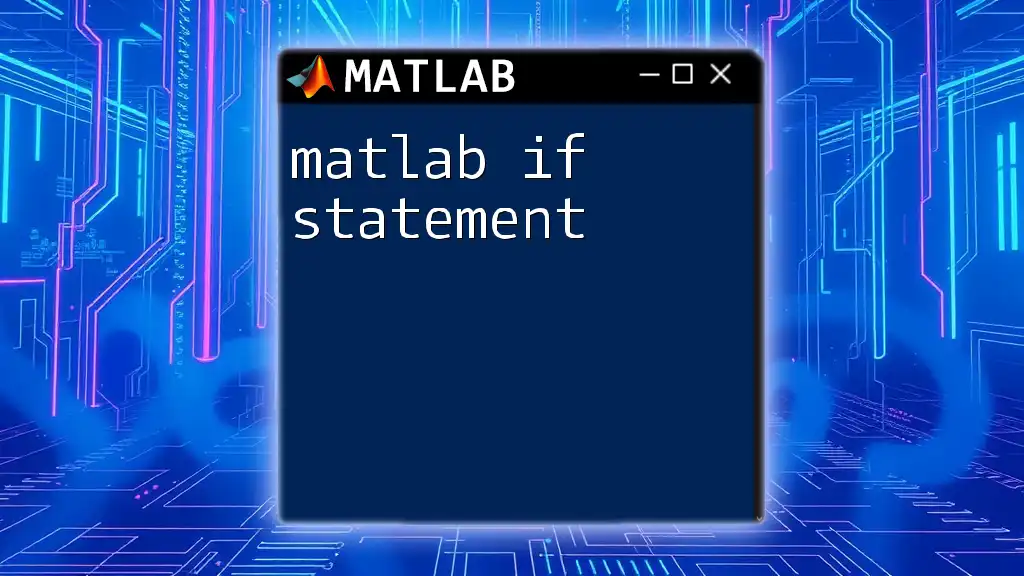
Syntax of the `case` Statement
Basic Structure
The basic syntax of a MATLAB case statement consists of three main components: the `switch` statement, one or more `case` statements, and an optional `otherwise` clause. Here’s how it’s structured:
switch variableName
case value1
% Code to execute for case value1
case value2
% Code to execute for case value2
otherwise
% Code to execute if no cases match
end
In this structure:
- The `switch` keyword initiates the statement and specifies the variable to evaluate.
- Each `case` keyword is followed by a value to match against the `variableName`.
- The `otherwise` clause serves as a catch-all for any values not explicitly handled.
Example of Syntax in Use
To help solidify understanding, consider this example where a variable named `color` determines the output:
color = 'blue';
switch color
case 'red'
disp('The color is red.');
case 'blue'
disp('The color is blue.');
otherwise
disp('Color not recognized.');
end
In this case, when `color` is set to `'blue'`, the output will be: The color is blue.
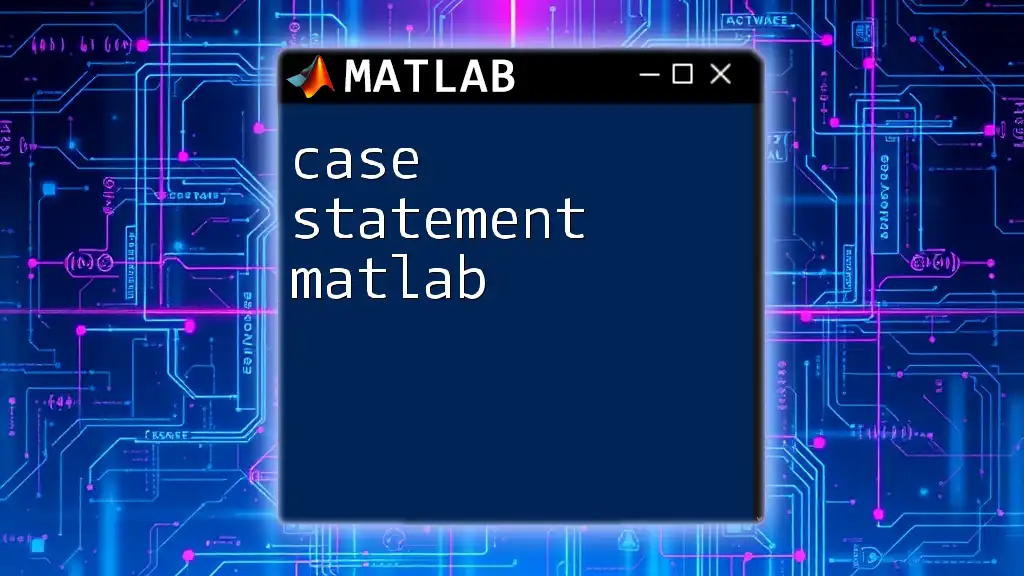
Detailed Breakdown of Components
The Switch Statement
The `switch` keyword acts as a decision-making construct that evaluates the variable specified. It simplifies the process of checking multiple potential values by directing the flow of control based on the value of one variable.
The Case Keyword
Following the `switch`, the `case` keyword indicates each possible match. Each `case` can handle a distinct value. If a match occurs, that block of code executes, bypassing any remaining cases.
The Otherwise Clause
The `otherwise` clause serves as the default action when no other cases are satisfied. It is essential for error handling and ensuring that your program gracefully manages unexpected inputs, thereby enhancing robustness.
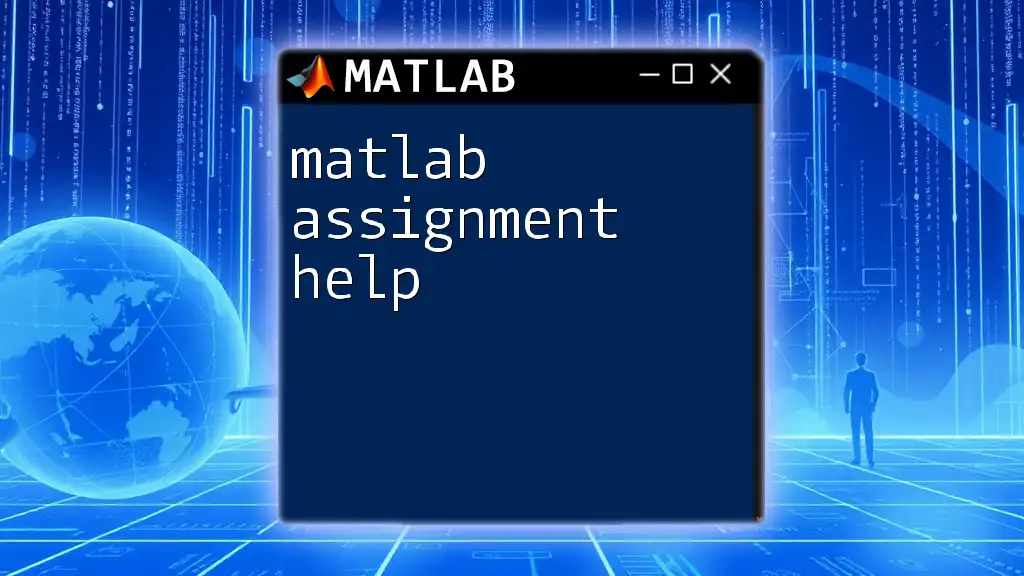
Practical Examples
Example 1: Simple Case Statement
Let’s take a look at a basic example using weekdays:
day = 'Wednesday';
switch day
case 'Monday'
disp('Start of the week.');
case 'Friday'
disp('Almost weekend.');
otherwise
disp('Midweek day.');
end
In this instance, if `day` is set to `'Wednesday'`, the output will be: Midweek day.
Example 2: Case Statement with Numerical Values
MATLAB also allows numerical comparisons in `case` statements. Here’s an example based on a scoring system:
score = 88;
switch score
case num2cell([90:100])
disp('Excellent!');
case num2cell([75:89])
disp('Good Job!');
case num2cell([0:74])
disp('Needs Improvement.');
end
Here, since `score` is `88`, the output will be: Good Job! This showcases how the case statement can effectively manage ranges using numerical arrays.
Example 3: Using Multiple Conditions in a Case Statement
Each `case` block can also evaluate multiple conditions, as seen in this example concerning colors:
color = 'green';
switch color
case {'red', 'blue'}
disp('Primary color.');
case {'green', 'orange'}
disp('Secondary color.');
otherwise
disp('Uncommon color.');
end
When `color` is set to `'green'`, the output will be: Secondary color. This allows for efficient grouping of similar conditions.
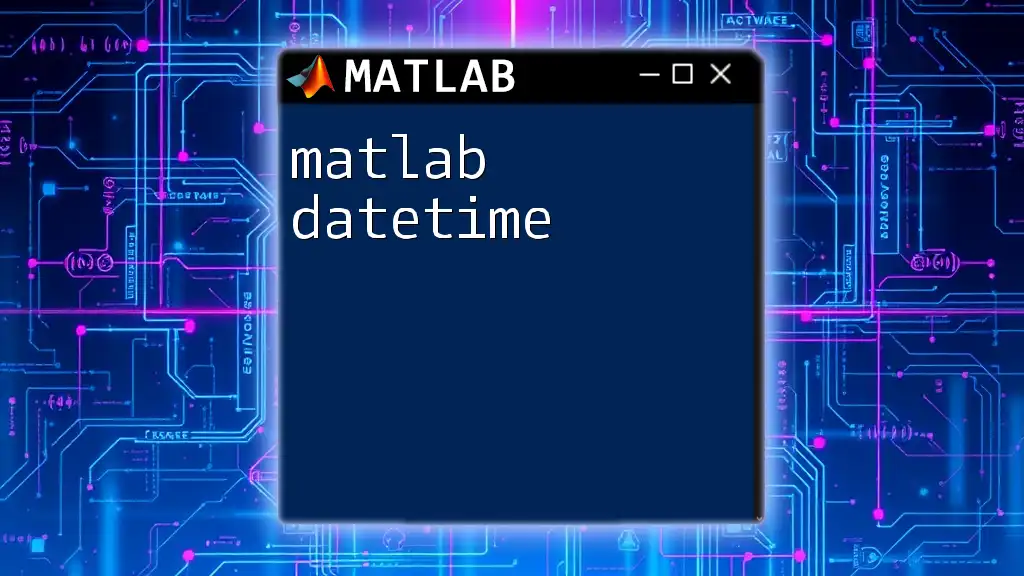
Common Mistakes to Avoid
Syntax Errors
When using the `case` statement, it’s easy to make syntax errors, especially in the declaration of the switch and case syntax. Always ensure that each `case` is properly defined, and check for misplaced keywords or missing `end` statements. A syntax error will prevent the code from executing.
Logical Errors
Logicians need to be aware of potential logical errors within their case blocks. For instance, if overlapping conditions exist, make sure that the more specific cases are evaluated before the more general ones. Always validate your input values to prevent unexpected behaviors.
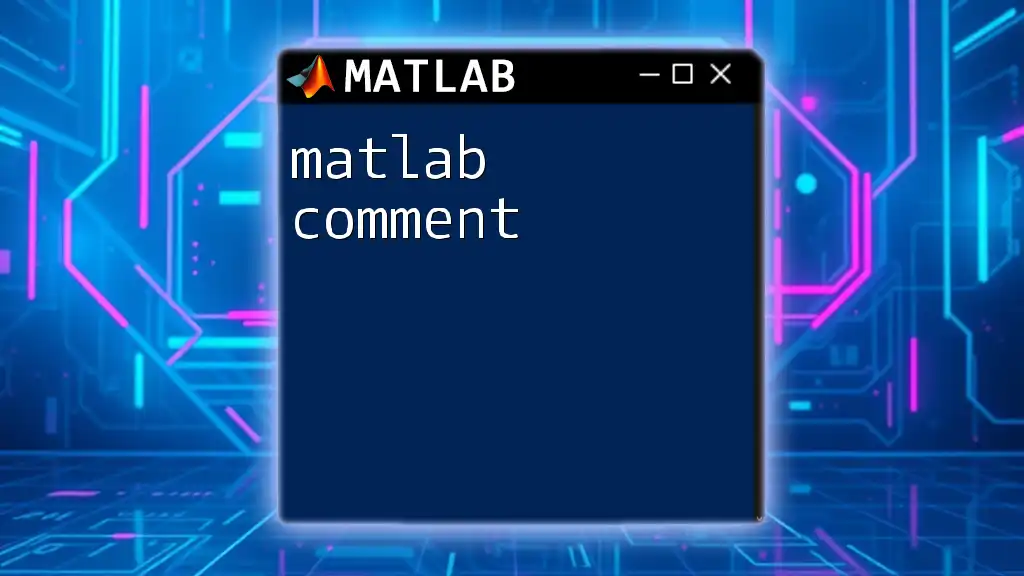
Best Practices
Readability
Keeping your code readable is paramount. Use clear and descriptive variable names, comment on complex logic, and consider the organization of your case statements. Readability improves maintenance and collaboration with others.
Performance Considerations
In terms of performance, case statements are generally quicker than `if-else` structures when checking multiple discrete values. For scenarios involving several conditions, opt for the `case` statement to enhance execution speed.
Documentation
Finally, effective documentation of your code helps others understand your logic. Always include comments that explain the purpose of each case, especially when the logic becomes complex. This will serve as a useful reference for anyone who works with your code in the future.
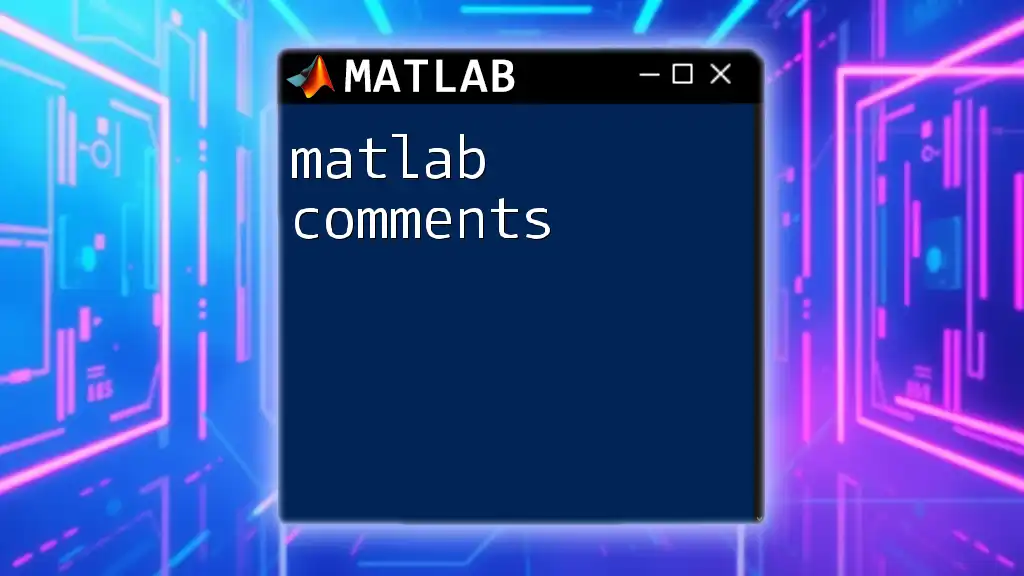
Conclusion
The MATLAB case statement is an invaluable tool in the MATLAB programming language, providing a clean and efficient way to handle multiple conditions. Understanding its syntax and application can save time and enhance the structure of your code.
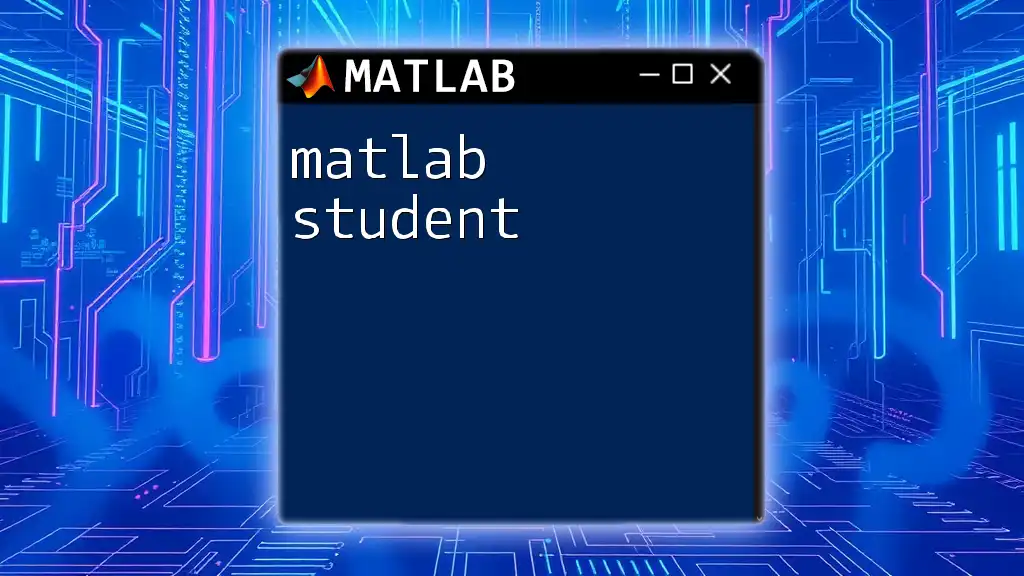
Further Resources
For more information on the case statement and MATLAB programming practices, consider visiting the official MATLAB documentation or exploring online courses specific to MATLAB programming.
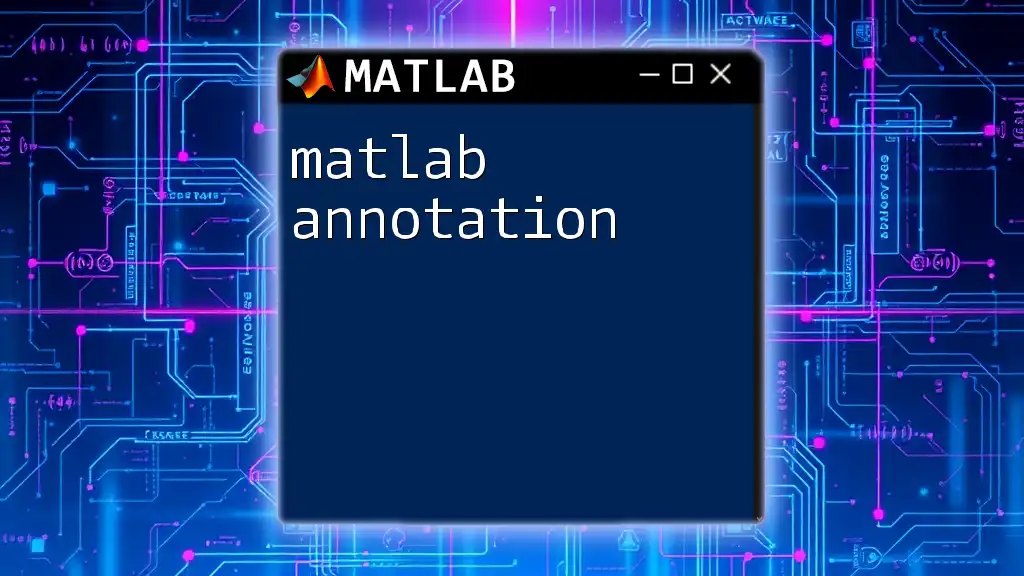
Call to Action
Explore how the MATLAB case statement can simplify your code by integrating it into your next programming project. For further assistance and structured learning, consider enrolling in a MATLAB course offered by our company!