In MATLAB, you can perform matrix multiplication using the asterisk (`*`) operator, which computes the product of two matrices where the number of columns in the first matrix must equal the number of rows in the second matrix.
C = A * B; % Multiplies matrix A by matrix B
Understanding Matrix Multiplication in MATLAB
Understanding Matrices in MATLAB
In MATLAB, a matrix is a fundamental data structure used for storing numbers in a two-dimensional format, consisting of rows and columns. Matrices are critical in various applications, including engineering disciplines, data analysis, machine learning, and more. Understanding how to manipulate matrices efficiently is vital for effective programming in MATLAB. Mastery of matrix operations is not just beneficial but essential for anyone looking to harness the full potential of MATLAB.
Basics of Matrix Multiplication
Before diving into practical applications, it is crucial to grasp the mathematical principles that underpin matrix multiplication.
Matrix multiplication involves combining two matrices to produce a third matrix. However, it is important to remember that only matrices with compatible dimensions can be multiplied together. For instance, if matrix A has dimensions m x n (m rows and n columns), it can only be multiplied by matrix B if B has dimensions of n x p. The resulting matrix C will have dimensions m x p.
The operation involves taking the dot product of the rows of the first matrix with the columns of the second. To calculate each element of the resulting matrix, multiply corresponding elements and sum the results. This operation is crucial in various scientific computations.
Matrix Multiplication Syntax in MATLAB
The basic syntax for multiplying two matrices in MATLAB is straightforward.
You can perform matrix multiplication using the asterisk (`*`) operator:
C = A * B;
This command will multiply matrices A and B, returning the result in matrix C. MATLAB adheres strictly to matrix multiplication rules, so ensure that the dimensions are compatible to avoid errors.
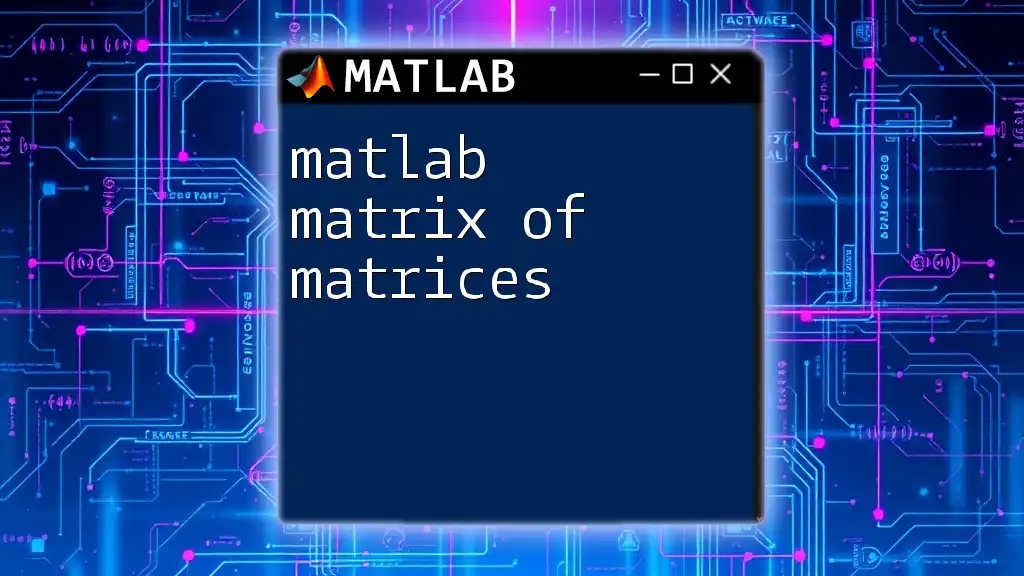
Step-by-Step Guide to Multiply Matrices
Creating Matrices in MATLAB
To perform operations like matrix multiplication, you first need to define your matrices in MATLAB. You can create a matrix by enclosing numbers in square brackets.
Here’s how you can create two matrices:
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
In this example, matrix A is a 2x2 matrix, as is matrix B. The semicolon indicates the end of a row, allowing the construction of multi-row matrices.
Performing Matrix Multiplication
With our matrices defined, we can now perform matrix multiplication. When we multiply matrices A and B, the operation in MATLAB proceeds as follows:
C = A * B;
The resulting matrix C can be calculated as:
- The element in the first row, first column of C is computed as follows: (15) + (27) = 19
- The element in the first row, second column of C: (16) + (28) = 22
- The element in the second row, first column of C: (35) + (47) = 43
- The element in the second row, second column of C: (36) + (48) = 50
Thus, matrix C is:
C = [19, 22; 43, 50];
Multiple Matrix Multiplication
You can also chain multiple matrix multiplications. For example, if you have another matrix \(D\):
D = [1, 2; 3, 4];
You can compute the product of A, B, and D as follows:
result = A * B * D;
In this case, MATLAB will evaluate the multiplication from left to right. First, it multiplies A and B to obtain an intermediate result, which is then multiplied by D.
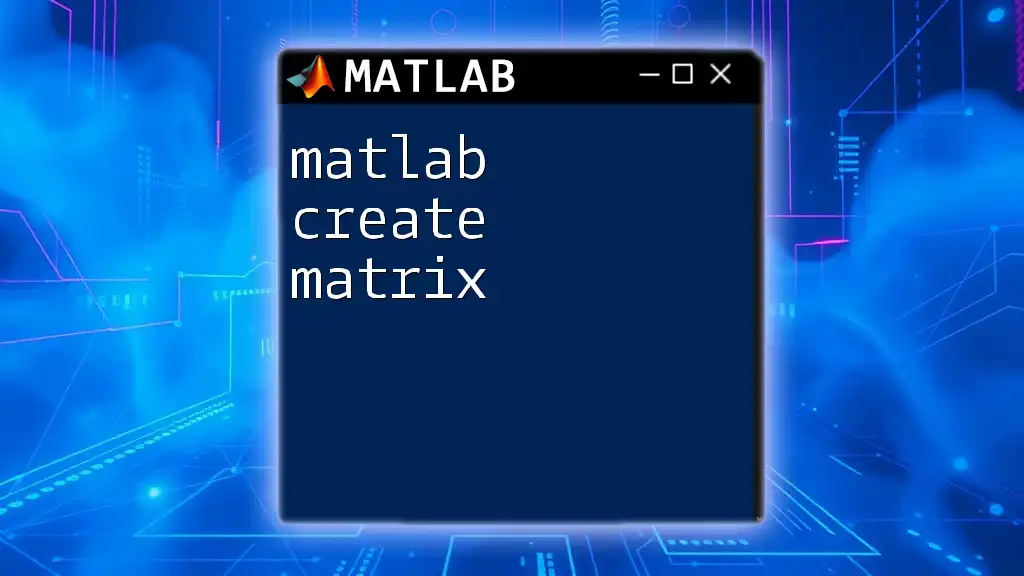
Common Errors and Troubleshooting
Dimension Errors
One common issue when working with matrix multiplication in MATLAB arises from incompatible dimensions. If you attempt to multiply two matrices whose sizes do not align, MATLAB will return an error message, typically stating "Inner matrix dimensions must agree."
To troubleshoot this, always check the dimensions of the matrices you intend to multiply. You can view the size of a matrix with the `size` function:
size(A) % Returns the dimensions of matrix A
Using Transpose to Adjust Dimensions
Sometimes, adjusting matrix dimensions using transposition can resolve incompatibility issues. The transpose operator (`'`) in MATLAB flips the matrix over its diagonal.
For instance, if matrix A is a 2x2 matrix and you need to perform operations that require a 2x1 shape, you can transpose it:
A_transpose = A'; % Transpose of A
Now, A_transpose can be multiplied appropriately with matrices that require it to be in a columnar format.
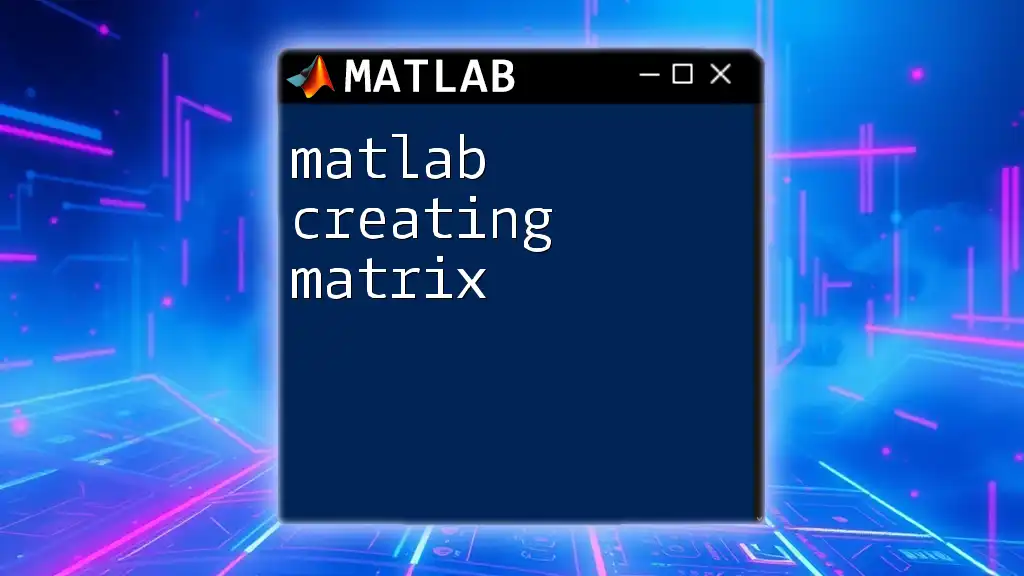
Advanced Topics in Matrix Multiplication
Element-wise Multiplication vs Matrix Multiplication
It is essential to differentiate between element-wise multiplication and matrix multiplication in MATLAB. While matrix multiplication uses the `` operator, element-wise multiplication employs the `.` operator.
For example:
E = A .* B; % Element-wise multiplication
In this operation, each corresponding element of matrices A and B is multiplied together, resulting in:
E = [1*5, 2*6; 3*7, 4*8]; % Result is [5, 12; 21, 32]
Sparse Matrices in MATLAB
As datasets grow larger, standard matrices can become inefficient in terms of memory usage. Sparse matrices are an efficient alternative for handling large matrices filled predominantly with zeros. Using sparse matrices speeds up computations and decreases memory consumption.
You can create a sparse matrix in MATLAB using:
S = sparse(A); % Create a sparse version of A
S can be multiplied with another matrix as follows:
S_result = S * B; % Multiply sparse matrix S with the dense matrix B
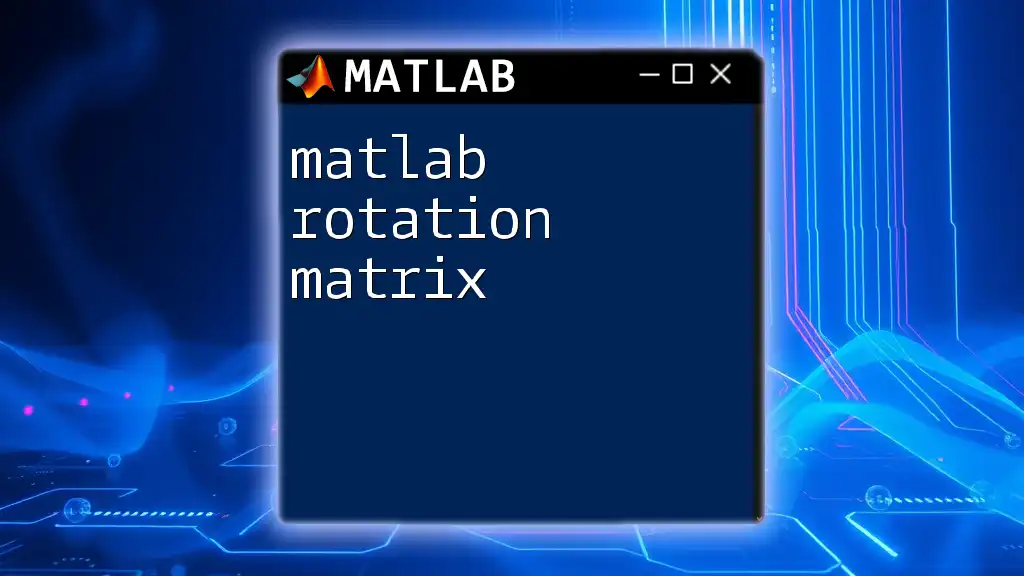
Practical Applications of Matrix Multiplication
Applications in Data Science
In data science, matrix multiplication plays a critical role in various computational algorithms, particularly in machine learning. Many algorithms, including neural networks and regression analysis, rely on matrix operations to process and analyze large datasets efficiently.
Applications in Engineering and Simulations
In engineering, matrix multiplications are frequently used in simulations, control systems, and structural analysis. For example, in finite element analysis (FEA), engineers use matrices to model and solve complex structural problems, requiring extensive matrix manipulations and multiplications.
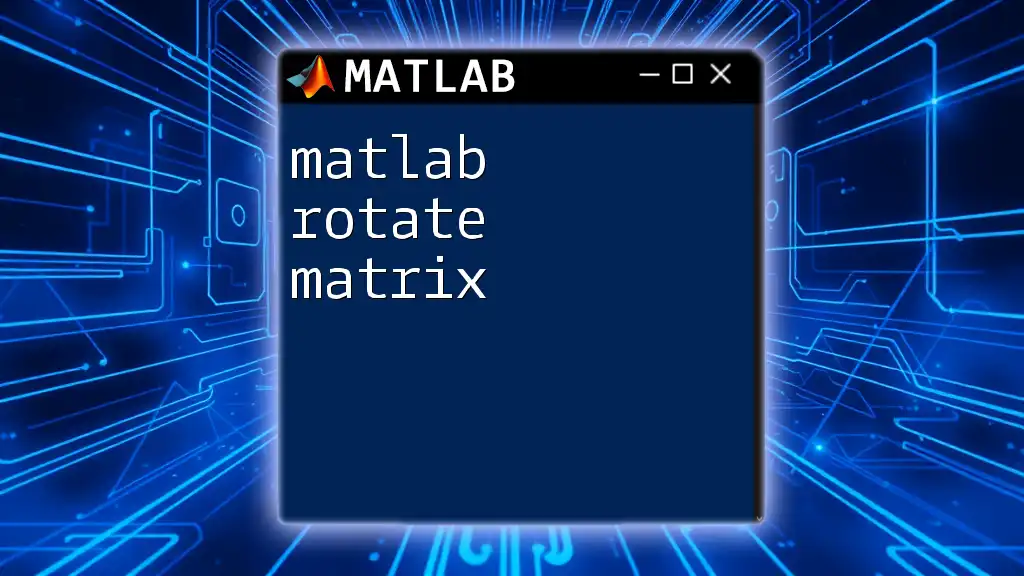
Conclusion
Throughout this article, we delved into the fundamental concepts of matrix multiplication in MATLAB, understanding how to define matrices, perform multiplication, troubleshoot common errors, and explore advanced topics. By practicing these skills, you will enhance your proficiency in MATLAB and tackle increasingly complex mathematical problems effectively.
Encouragement to Practice
To reinforce your understanding, I encourage you to experiment with various matrices of different shapes and sizes. The more you practice, the more confident you will become in performing matrix operations effectively in MATLAB.
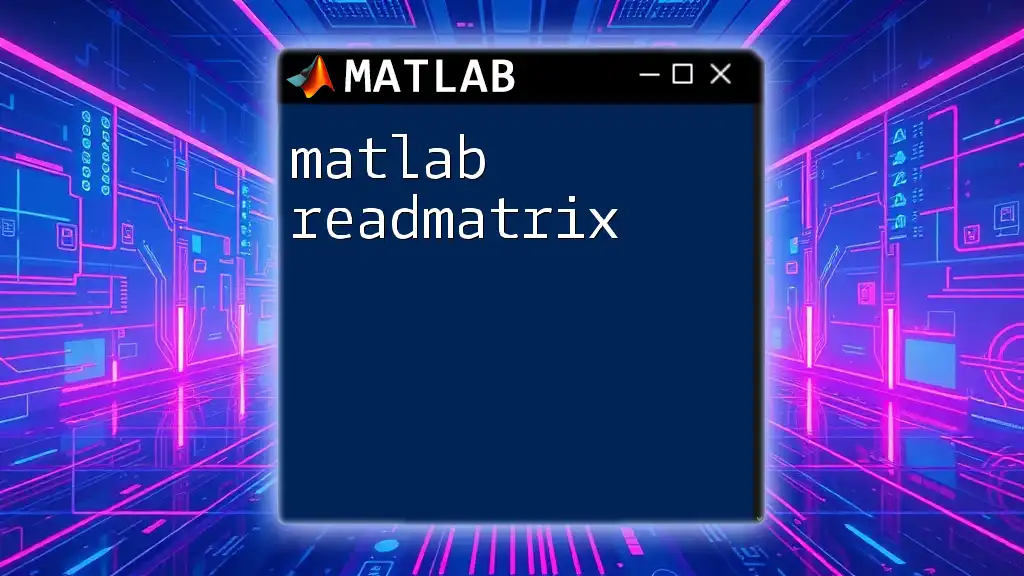
Additional Resources
For further exploration, refer to the [MATLAB documentation on matrix operations](https://www.mathworks.com/help/matlab/matlab-matrices.html) and consider online courses that delve deeper into MATLAB programming and matrix manipulations.