The Fast Fourier Transform (FFT) in MATLAB is an efficient algorithm to compute the discrete Fourier transform, allowing users to analyze frequency components of signals quickly.
% Example of using FFT in MATLAB
x = [0 1 2 3 4 5]; % Sample signal
y = fft(x); % Compute the FFT
disp(y); % Display the FFT results
What is FFT?
Fast Fourier Transform (FFT) is a powerful mathematical algorithm that efficiently computes the Discrete Fourier Transform (DFT) and its inverse. FFT is critical in various fields such as signal processing, communications, and image analysis for transforming signals between time and frequency domains. Understanding how to leverage MATLAB for FFT can substantially enhance your data analysis capabilities.
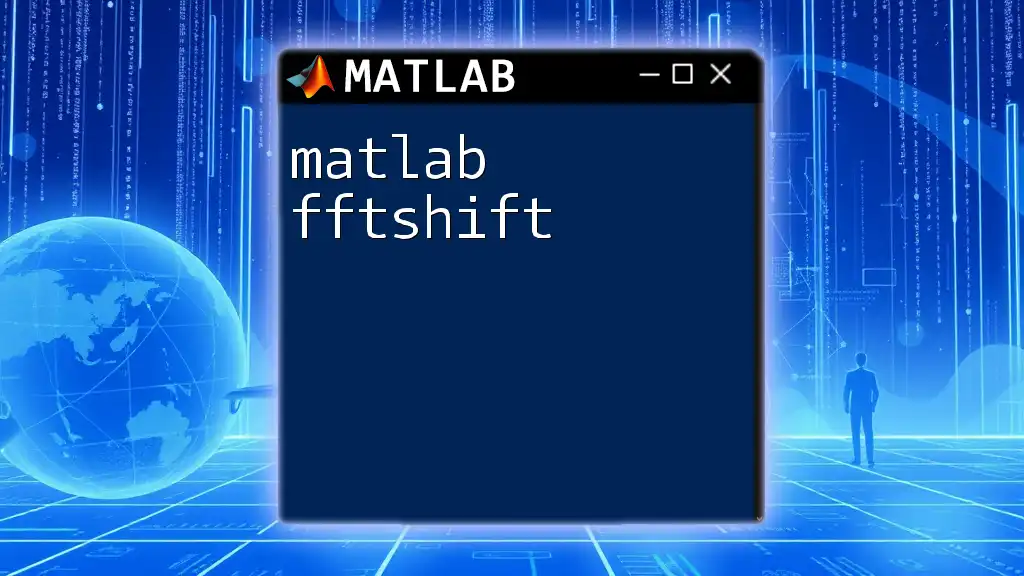
Applications of FFT
The applications of FFT are extensive. Some of its common uses include:
- Audio Processing: Analyzing sound signals for frequency components, filtering noise, and synthesizing audio.
- Image Processing: Manipulating images for compression, filtering, and transforming image data.
- Communications: Modulating and demodulating signals for effective transmission and reception.
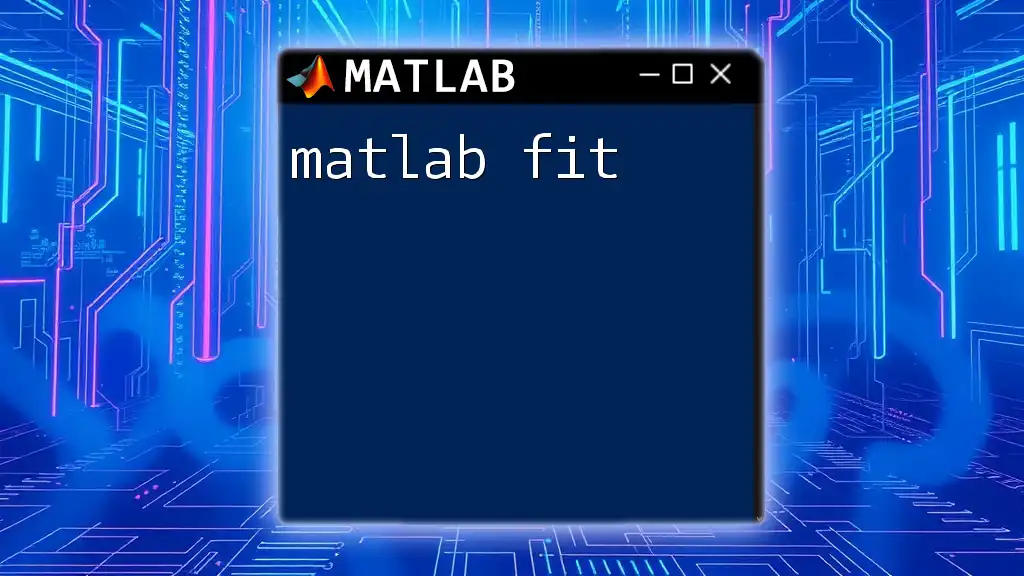
Why Use MATLAB for FFT?
MATLAB is preferred for FFT applications due to its user-friendly environment and built-in functions that simplify complex calculations. With high-level functions like `fft`, MATLAB allows users to perform FFT operations without deep knowledge of the underlying mathematics involved.
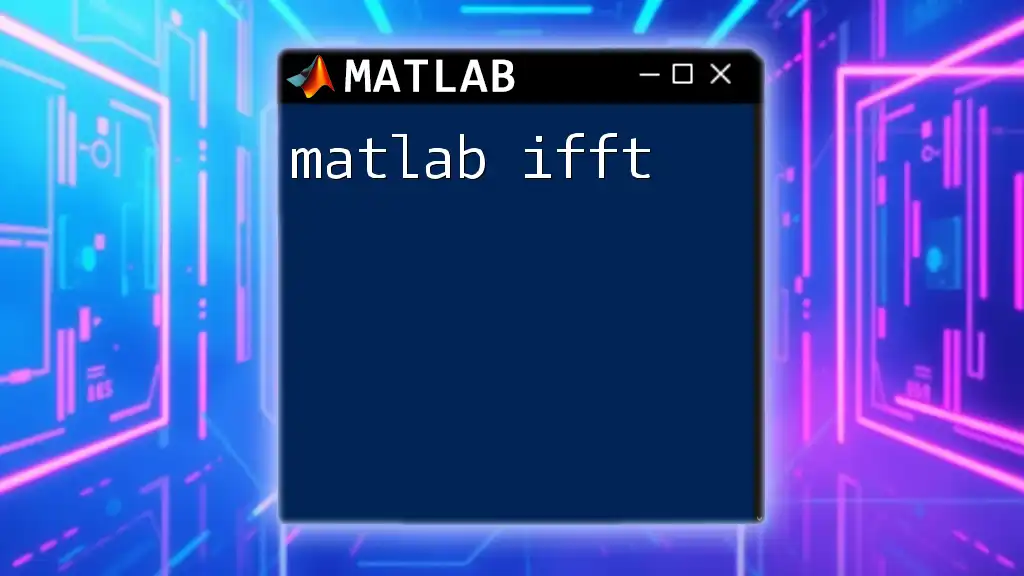
Overview of Fourier Transform
The Fourier Transform converts a signal from the time domain to the frequency domain by decomposing it into its constituent frequencies.
Continuous vs. Discrete Fourier Transform
- The Continuous Fourier Transform (CFT) applies to continuous functions, while the Discrete Fourier Transform (DFT) applies to discrete datasets.
- The transformation not only changes the representation of the signal but also reveals periodic characteristics of the data.
Key Concepts
Frequency denotes the number of occurrences of a repeating event in a unit of time. Bandwidth represents the range of frequencies contained in a signal, while resolution refers to the ability to distinguish between close frequencies.
Mathematical Formula of Fourier Transform
The mathematical expression for the Fourier Transform is:
$$ X(f) = \int_{-\infty}^{\infty} x(t)e^{-j2\pi ft} dt $$
where:
- \( X(f) \) is the Fourier Transform of the signal \( x(t) \),
- \( j \) is the imaginary unit,
- \( f \) is the frequency.
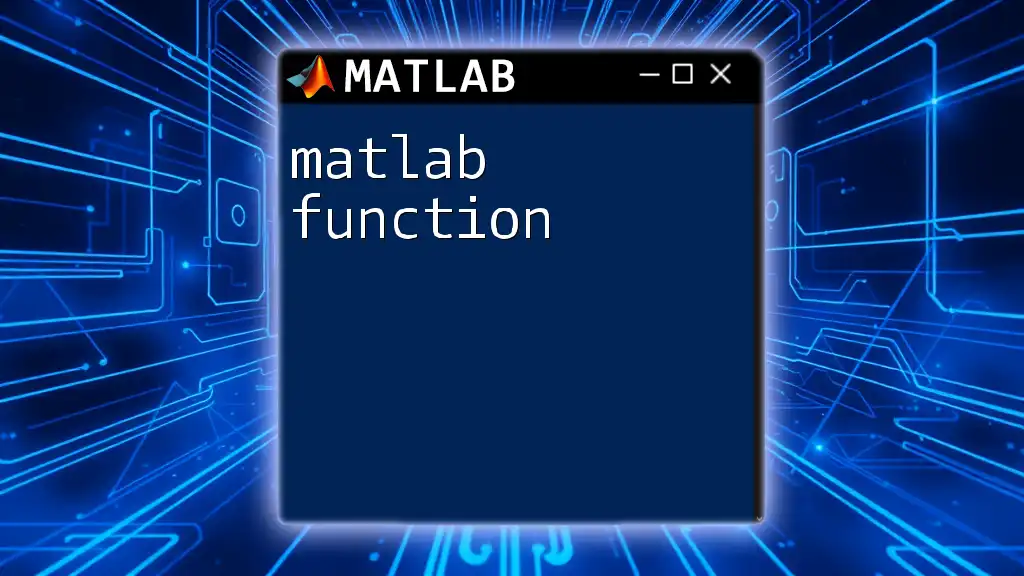
Getting Started with FFT in MATLAB
Setting Up MATLAB
Before diving into MATLAB FFT, ensure you have MATLAB installed and familiarize yourself with its interface.
Basic Syntax for FFT Command
In MATLAB, the basic syntax to perform the FFT is simple:
Y = fft(X)
Here, `X` represents the input signal, and `Y` will contain the frequency domain representation of the signal. A straightforward example demonstrates how this command can be utilized and visualized.
Example Usage:
To get started, let’s consider a basic example of using `fft`:
fs = 1000; % Sampling frequency
t = 0:1/fs:1-1/fs; % Time vector
x = sin(2*pi*100*t) + sin(2*pi*200*t); % Example signal
Y = fft(x);
In this example:
- We sample a signal consisting of two sine waves, one at 100 Hz and the other at 200 Hz.
- The FFT of the signal is computed and held in `Y`.
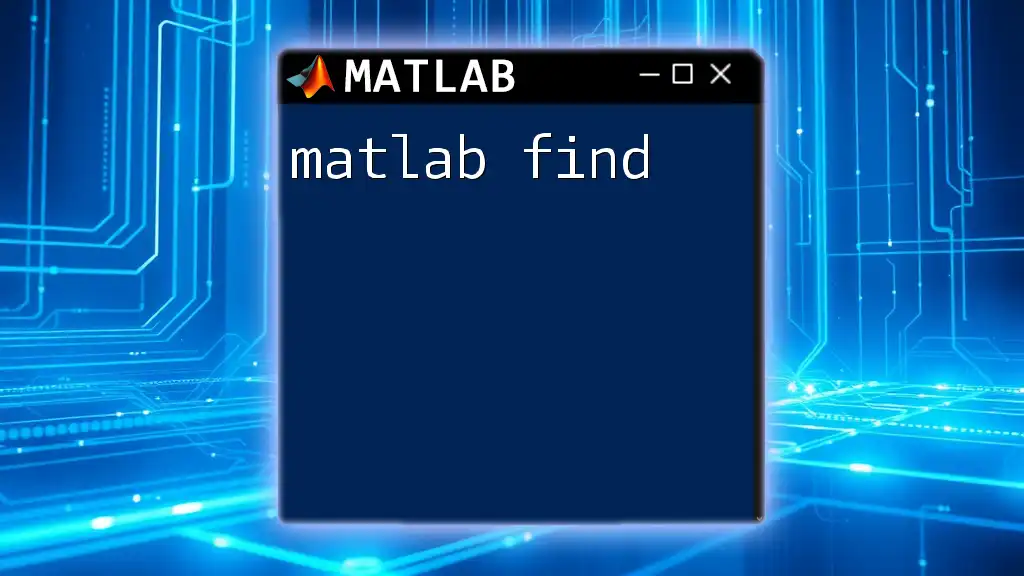
Utilizing the FFT Function
Working with Real Signals
MATLAB’s `fft` function is designed to handle real-valued signals directly. As demonstrated, generating a sample signal is straightforward.
Analyzing Frequency Components
One of the critical activities after executing FFT is analyzing the magnitude and phase components of the resulting signal. You can compute the magnitude and visualize the frequency spectrum as follows:
n = length(x); % Length of the signal
f = (0:n-1)*(fs/n); % Frequency range
plot(f, abs(Y)); % Plotting magnitude spectrum
This helps in observing how much of each frequency is present in the original signal.
Magnitude and Phase Spectrum
Understanding the magnitude and phase spectra can reveal extensive information about the frequency characteristics of the signal. For visualization:
subplot(2,1,1), plot(f, abs(Y)); title('Magnitude Spectrum');
subplot(2,1,2), plot(f, angle(Y)); title('Phase Spectrum');
Normalizing the FFT Output is crucial to ensure that the results can be interpreted accurately. Achieving normalization is done as follows:
Y_normalized = Y/n; % Normalize FFT output
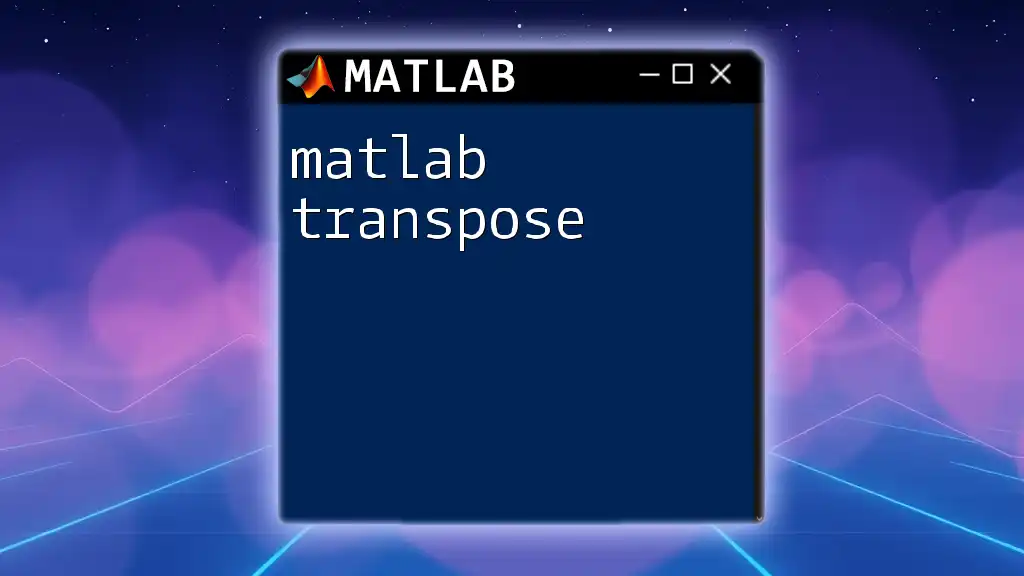
Advanced FFT Techniques
Multi-dimensional FFT
For multidimensional data such as images, MATLAB provides functionality for performing the FFT in multiple dimensions. This can be accomplished using the `fft2` function:
Z = fft2(image); % 2D FFT on an image
Real FFT with `fftreal`
In some cases, if you are dealing with purely real inputs, you may use specialized functions like `fftreal` (if available) to enhance performance by reducing computational workload.
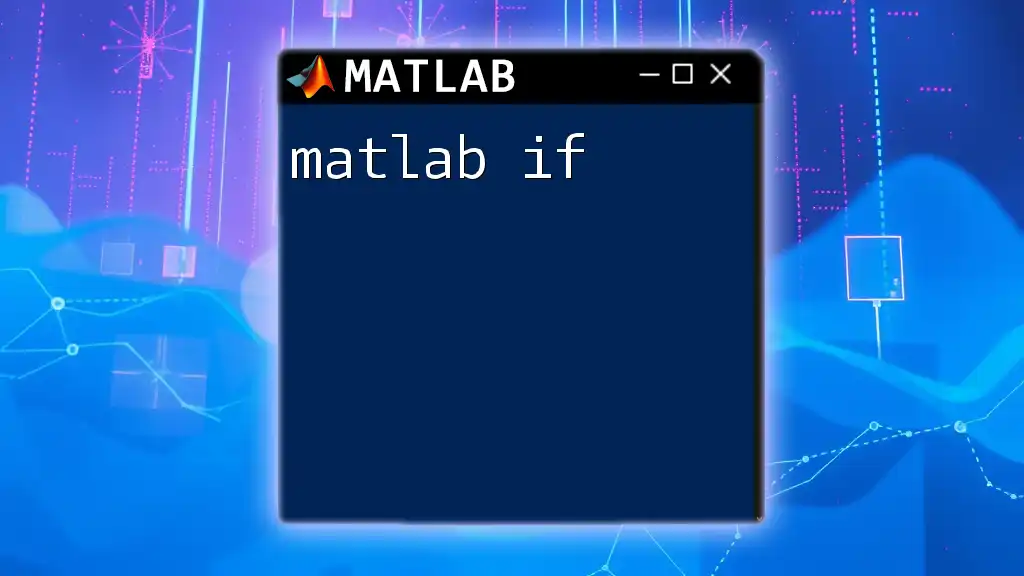
Performance Optimization
Speed Considerations
The significance of FFT arises primarily from its computational efficiency compared to a naive implementation of DFT. The complexity of FFT operations is \( O(n \log n) \), making it considerably faster for large datasets.
Using `fft` with Large Datasets
When working with large datasets, consider using zero-padding, which can improve frequency resolution:
X_padded = [x, zeros(1, N-length(x))]; % Zero-padding the signal
Y_padded = fft(X_padded); % Perform FFT
This allows more frequent data points in the frequency domain and better visual interpretation.
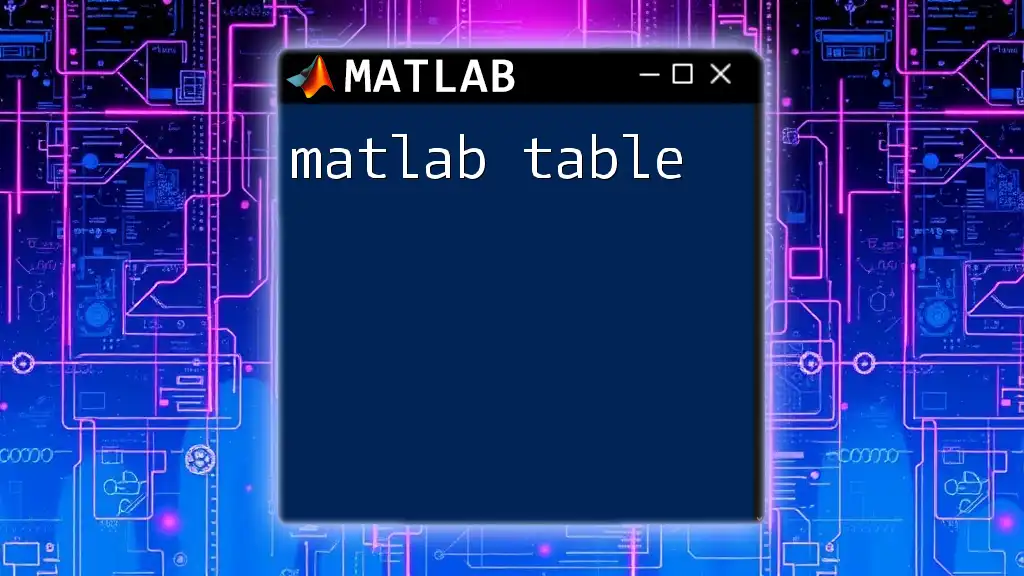
Common Issues and Troubleshooting
Interpreting FFT Results
While working with FFT, interpreting results from the frequency domain can be tricky. Common issues include:
- Aliasing, which occurs if the sampling frequency is too low in relation to the signal frequencies. To resolve this, ensure your sampling frequency is at least twice the highest frequency present in your signal.
MATLAB Warnings and Errors
When applying FFT, especially with large or complex datasets, be prepared for warnings or errors. Typical MATLAB errors could include dimension mismatches, which can often be resolved by ensuring all input arrays are correctly sized.
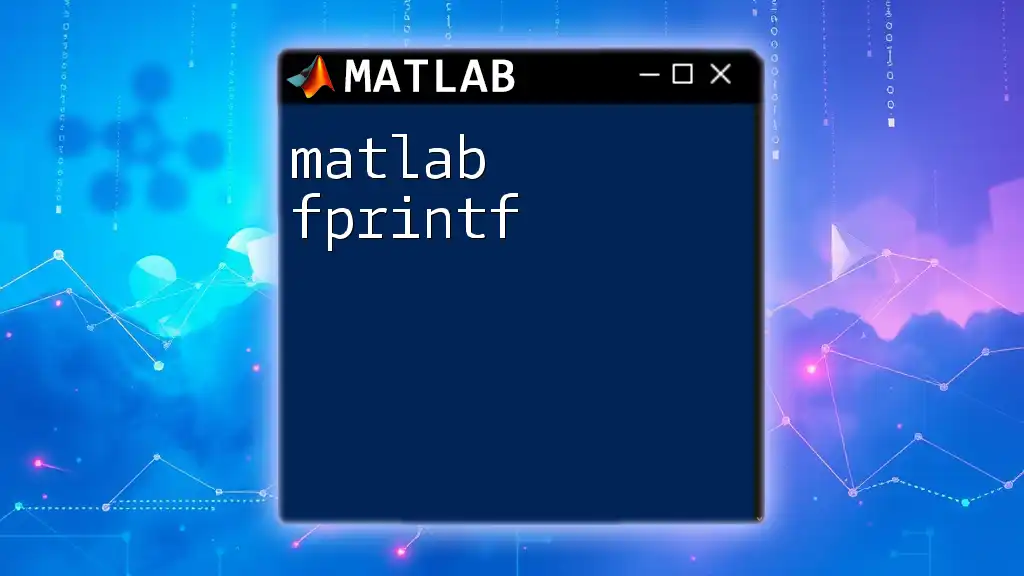
Practical Examples with Real Data
Example 1: Audio Signal Analysis
Consider a simple example of analyzing an audio signal. Following these steps will guide you through:
- Loading audio data:
[x, fs] = audioread('audiofile.wav'); % Load audio file
- Applying FFT:
Y_audio = fft(x);
- Plotting results:
f_audio = (0:length(Y_audio)-1)*(fs/length(Y_audio)); % Frequency vector
plot(f_audio, abs(Y_audio)); % Magnitude spectrum of audio signal
Example 2: Image Processing Using FFT
You can also apply FFT to analyze an image. The approach is relatively similar:
- Loading and processing a grayscale image:
image = imread('imagefile.png');
gray_image = rgb2gray(image); % Convert to grayscale
Z = fft2(double(gray_image)); % 2D FFT
- Visualizing the result:
magnitude_spectrum = log(1 + abs(Z)); % Compute the magnitude spectrum
imshow(magnitude_spectrum, []);
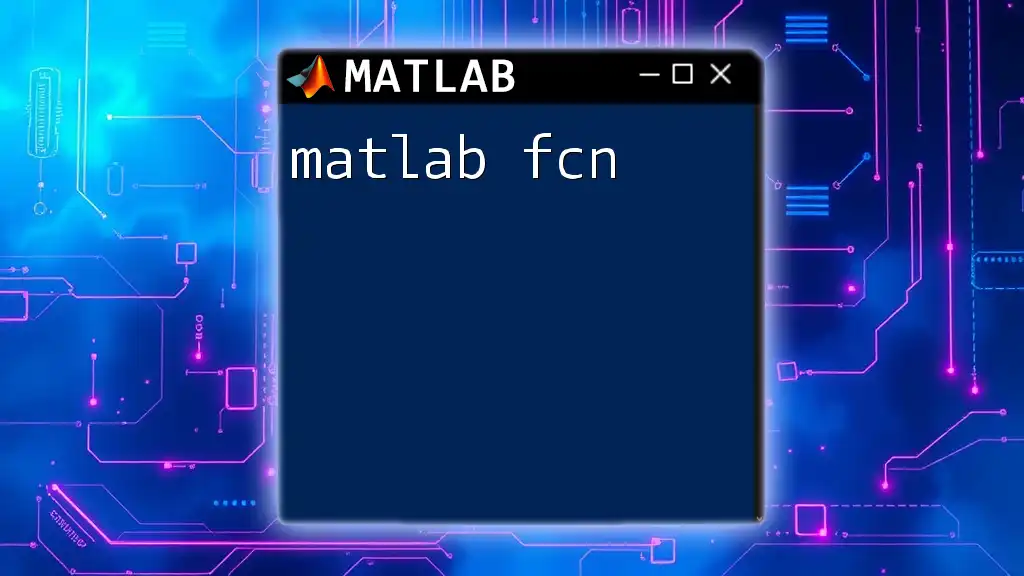
Conclusion
In summary, mastering MATLAB FFT can transform how you analyze signals and images by providing efficient means to shift between time and frequency domains. By practicing the commands and using the examples discussed, you will enhance your ability to utilize MATLAB for advanced signal processing tasks.
Encouraging exploration of additional resources and documentation will also help solidify your understanding. Begin your journey today with FFT in MATLAB, and experience the difference it can bring to your analytical capabilities!