A MATLAB matrix of matrices, commonly referred to as a cell array, allows you to store multiple matrices of varying sizes in a single variable, facilitating more flexible data management.
Here’s an example of creating a matrix of matrices using a cell array:
% Create a cell array containing different matrices
matrixOfMatrices = { [1, 2; 3, 4], [5; 6], [7, 8, 9; 10, 11, 12] };
% Accessing individual matrices
firstMatrix = matrixOfMatrices{1}; % Access the first matrix: [1, 2; 3, 4]
Understanding Matrices in MATLAB
What is a Matrix?
A matrix is a rectangular array of numbers arranged in rows and columns. In MATLAB, matrices form the cornerstone of its functionality, allowing for complex numerical computations. A simple example of a 2D matrix in MATLAB is as follows:
% Creating a simple 2D matrix
A = [1, 2; 3, 4];
disp(A);
This code snippet creates a 2x2 matrix, where each element can be accessed using row and column indices.
MATLAB Matrix Basics
In MATLAB, understanding matrix dimensions and indexing is crucial. Each matrix has dimensions defined by the number of rows and columns it contains. You can easily access any element by specifying its row and column position. For instance:
% Accessing elements of a matrix
element = A(1,2); % Accessing element in the first row, second column
In this example, the variable `element` will hold the value `2`, which resides in the first row and second column of matrix `A`.
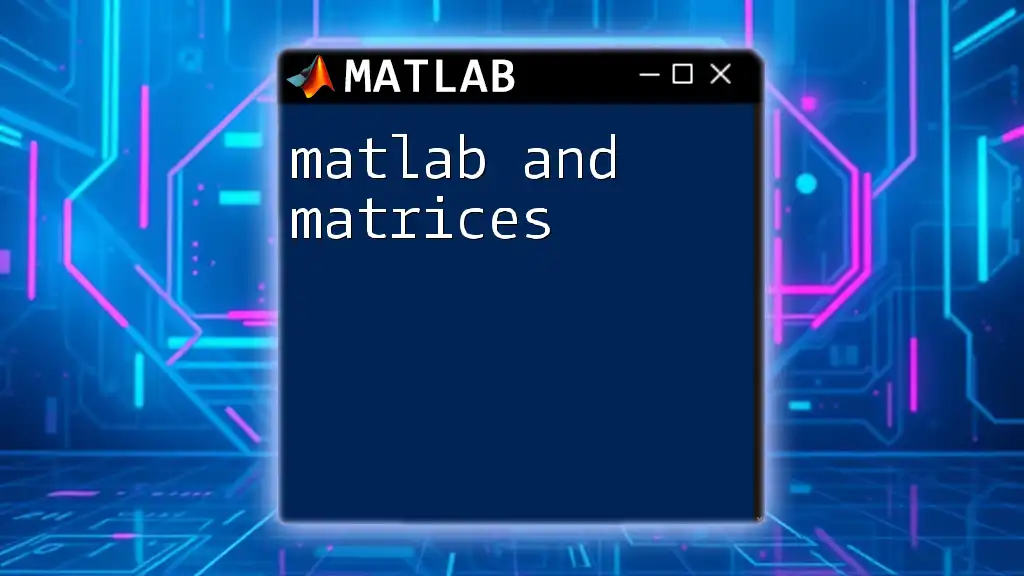
Introduction to a Matrix of Matrices
Definition of a Matrix of Matrices
A matrix of matrices refers to a structure where one matrix contains multiple matrices as its elements. This allows for hierarchical data organization, which is particularly useful in various applications like data analysis, machine learning, and image processing. For example, you can store different datasets or sub-matrices as elements within a larger matrix.
Structure and Characteristics
The structure of a matrix of matrices significantly differs from a standard matrix. While a standard matrix has a uniform shape, a matrix of matrices can hold matrices of varying dimensions. For example:
% Creating a matrix of matrices
M = {[1, 2; 3, 4], [5; 6]};
In this code snippet, `M` contains two matrices: the first matrix is 2x2, and the second is 2x1. This flexibility opens doors to complex data structures within MATLAB.
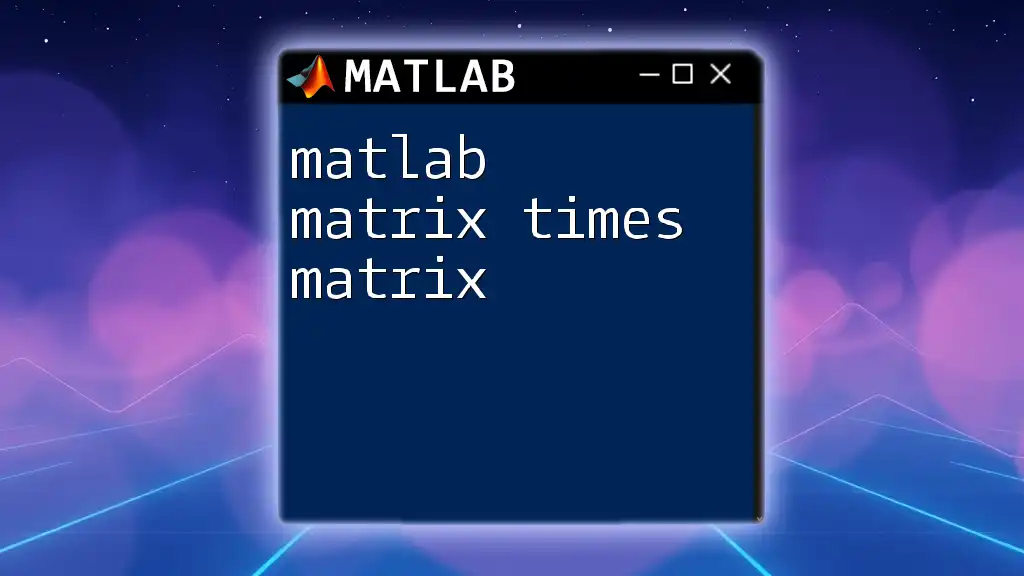
Creating a Matrix of Matrices in MATLAB
Step-by-Step Guide
Creating a matrix of matrices in MATLAB is straightforward. You can initialize it by using cell arrays, which allow for the storage of matrices of different sizes. Here's how to do this:
% Creating a matrix of matrices
A = {ones(2), eye(3)};
In this example, `A` contains two matrices: a 2x2 matrix filled with ones and a 3x3 identity matrix. The use of curly braces `{}` is key; they allow for a combination of matrices with varying dimensions.
Visual Representation
Visualizing the matrices within a matrix of matrices can enhance understanding. You can easily display these matrices using `cellfun`:
cellfun(@(x) disp(x), A); % Display each matrix in the matrix of matrices
This command iterates over each matrix `x` in `A` and displays it. It is an efficient way to verify the contents of your matrix of matrices.
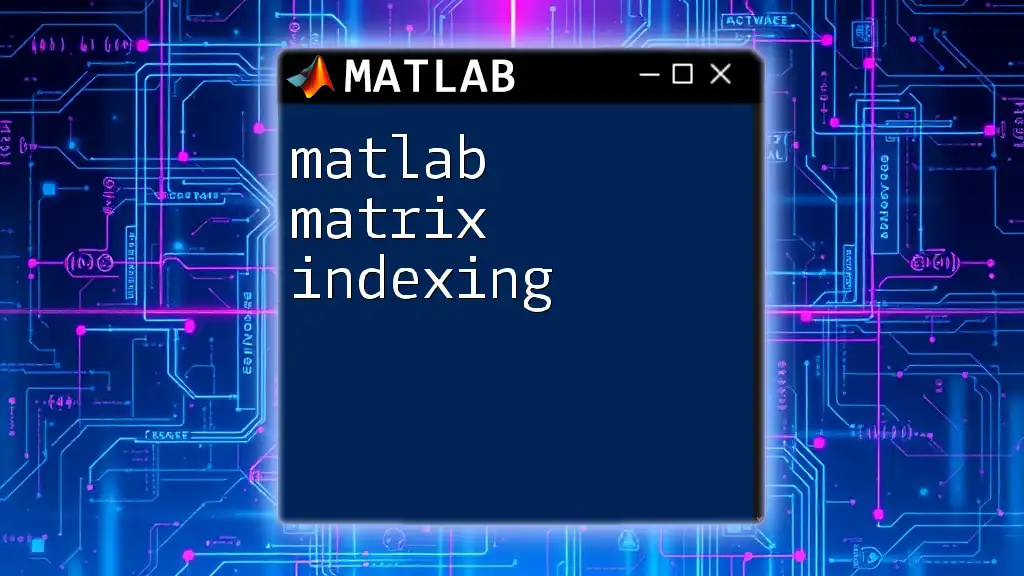
Accessing Elements in a Matrix of Matrices
Indexing Techniques
To access individual matrices within a matrix of matrices, you need to use curly braces. This is a critical aspect of MATLAB syntax when working with cell arrays. For instance, you can access the first matrix like this:
% Accessing the first matrix
firstMatrix = A{1};
Once you have accessed a matrix, you can also access specific elements within it using standard indexing techniques.
Example Use Case
To demonstrate element access further, let's retrieve a specific element from the first matrix in our matrix of matrices:
% Accessing an element within the first matrix
value = A{1}(2, 1); % Accessing the element in second row, first column
This command will set the variable `value` to `1`, as that is the content in the second row and first column of the first matrix in `A`.
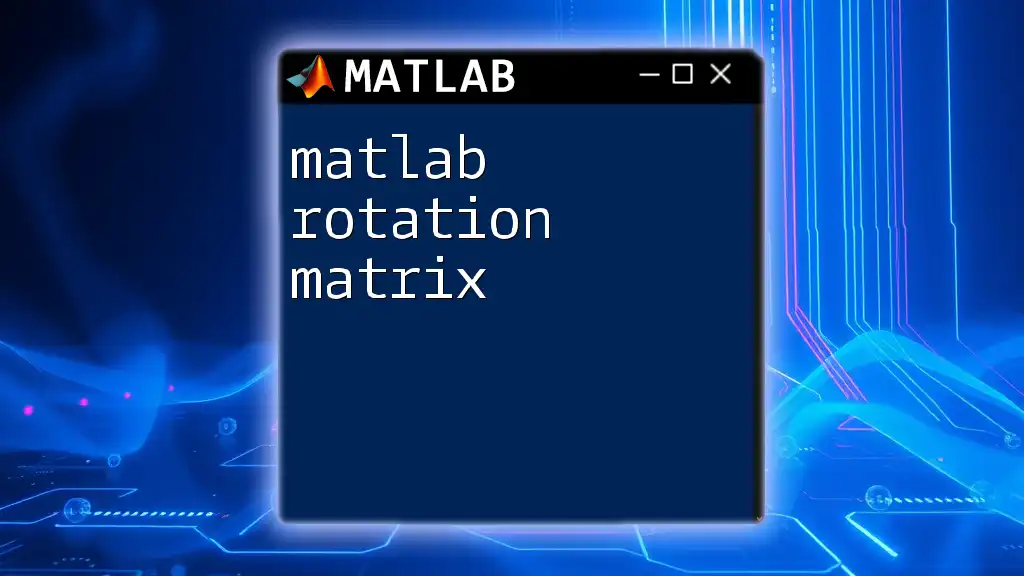
Manipulating a Matrix of Matrices
Adding and Deleting Matrices
You may need to modify your matrix of matrices by adding or deleting specific matrices. To append a new matrix, simply use the `end` keyword:
% Adding a new matrix
A{end+1} = [7, 8; 9, 10];
In this example, a new matrix `[[7, 8], [9, 10]]` is added to the end of `A`.
If you need to delete a matrix from your structure, you can use the `clear` command in conjunction with the indexing:
% Deleting the second matrix
A(2) = []; % Removes the second matrix from A
Modifying Elements
To modify existing elements within a matrix of matrices, you again use the curly braces to pinpoint the location:
% Modifying an element
A{1}(1,2) = 99; % Changing value in the first matrix
In this instance, the value at the first row and second column of the first matrix in `A` is changed to `99`.
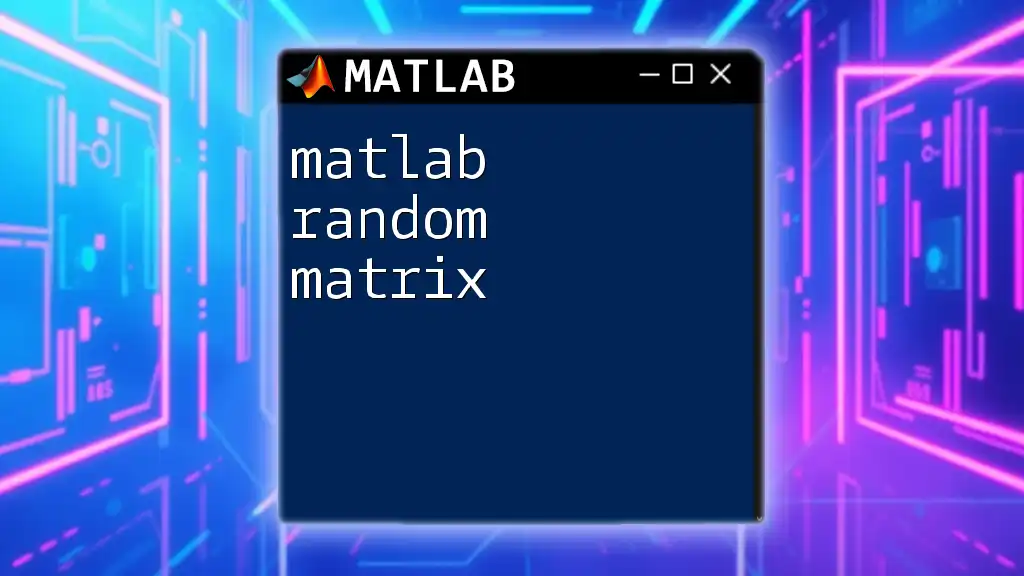
Applications of a Matrix of Matrices
In Data Analysis
In data analysis, a matrix of matrices can help organize different datasets efficiently. By storing various attributes of a dataset as separate matrices, you can conduct operations on them collectively or individually without redundancy. For instance, you could have one matrix for sales data, another for inventory, and yet another for customer data.
In Image Processing
In image processing, matrices of matrices can represent images where different color channels (like RGB) can be stored as separate matrices. This technique allows for easier manipulation of images by isolating these channels for adjustments or transformations.
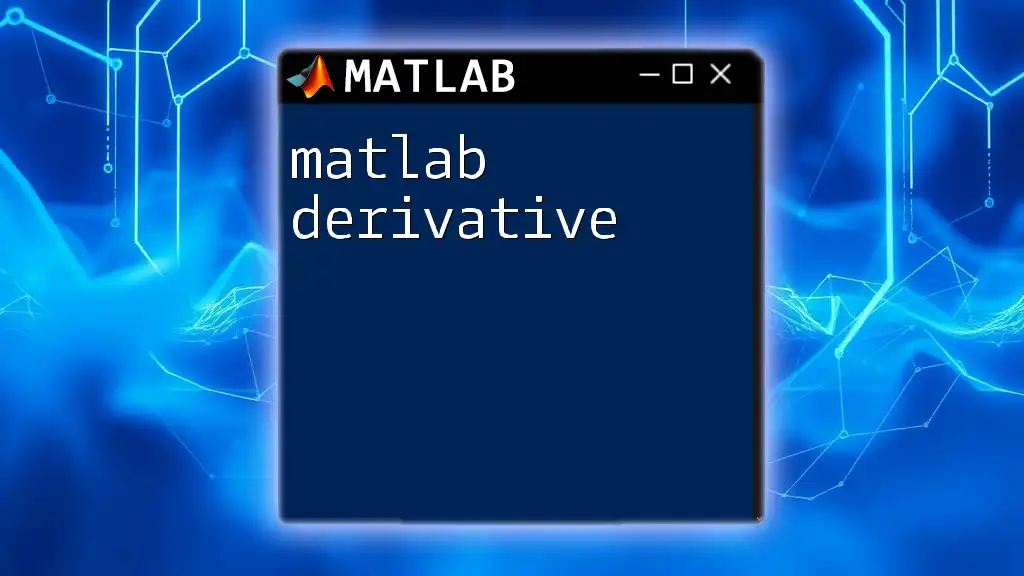
Conclusion
Understanding the concept of a MATLAB matrix of matrices offers vast possibilities for data management and computational tasks. Mastering how to create, access, and manipulate these structures can significantly enhance your workflow in MATLAB. As you practice with the examples provided in this guide, you'll find yourself more adept at handling complex data scenarios with efficiency and precision.